JAVA basic learning
method
Java's main memory space
When the code is placed in the method area to execute the main file first, when the main method is executed, the memory space required by the method is allocated in the stack, which is a data structure
Stack data structure
Stack data structure: stack
What is a data structure?
The data structure is usually a container for storing data, and the container may have different structures. Data structure has nothing to do with java language. Data structure is an independent subject
What are the common data structures?
Array, linked list, graph binary, queue... java language has written the data structure, and programmers can use it directly.
Together with data structures are algorithms: sorting, searching, etc
Proficient in data structures and algorithms will improve the efficiency of the program
Method area execution time memory transformation
Push
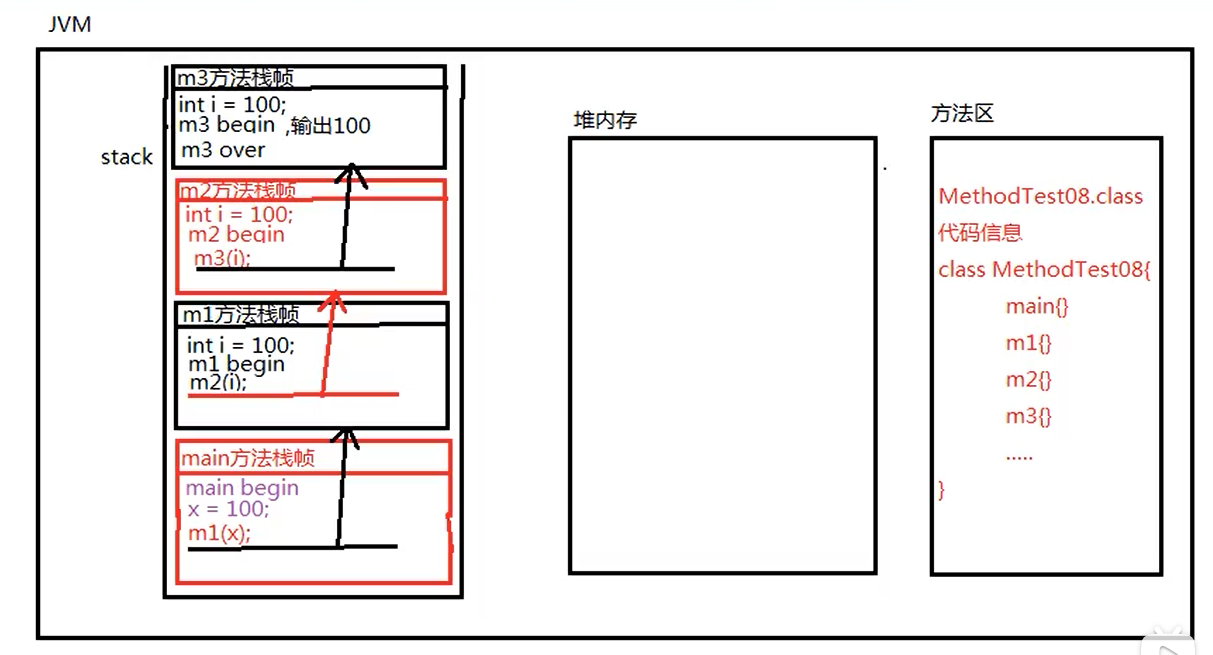
Bomb stack
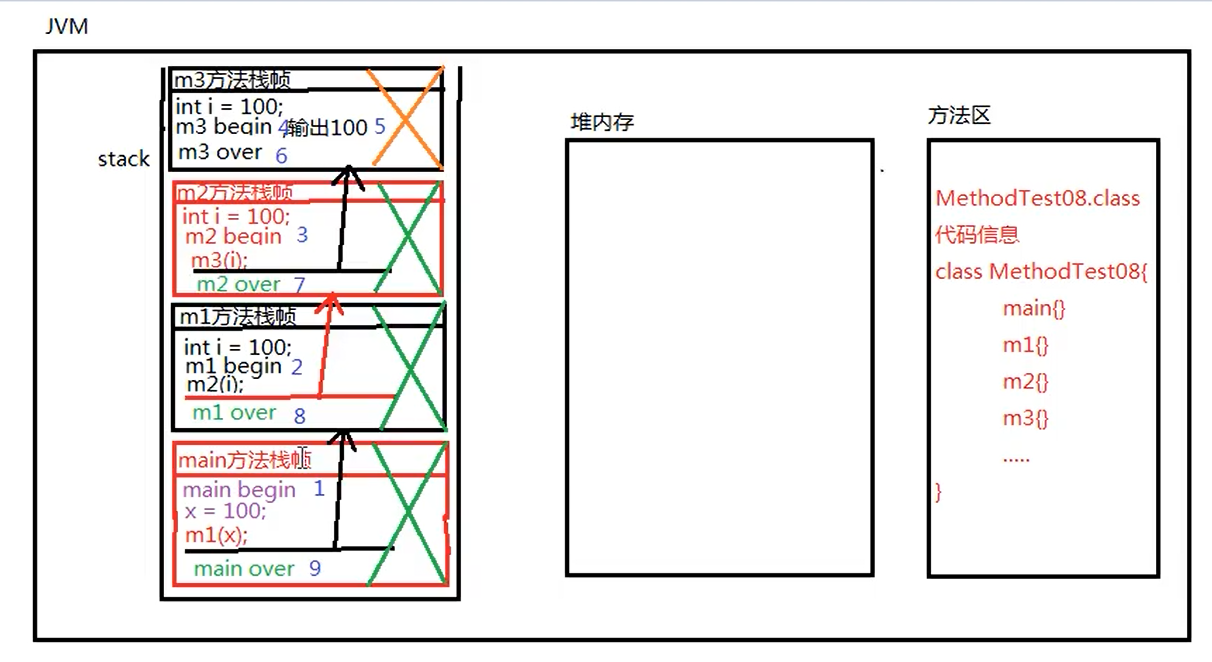
The three i and x are local variables. After the method ends, the memory space is released
package Method; //Local variables are only valid in the method body. After the method ends, the local variable memory will be invalidated //There is data first in the method area. Code fragments are placed in the method area to store class bytecode //Stack memory: when a method is called, the memory space required by the method is allocated in the stack //The method will not allocate space in the stack if it is not called. The method will allocate space in the stack only when it is called, and it is pressing the stack when it is called //After the method is executed, the space required by the method will be released to bounce the stack. /* Method calls are called: stack up and allocate space Method end: pop the stack to free up space Stack storage: the memory required in the execution of the method and the local variables of the method */ public class test01 { //Main method entry public static void main(String[] args){ System.out.println("main begin"); /* int a = 100; int b = a; The principle here is: copy the number 100 saved in the a variable and pass it to b, So a and b are two different memory spaces, two local variables */ int x = 100; m1(x); System.out.println("main begin"); } public static void m1(int i){ //i is a local variable System.out.println("m1 begin"); m2(i); System.out.println("m1 over"); } public static void m2(int i){ System.out.println("m2 begin"); m3(i); System.out.println("m2 over"); } public static void m3(int i){ System.out.println("m3 begin"); System.out.println(i); System.out.println("m3 over"); } }
main begin m1 begin m2 begin m3 begin 100 m3 over m2 over m1 over main begin
Method summary
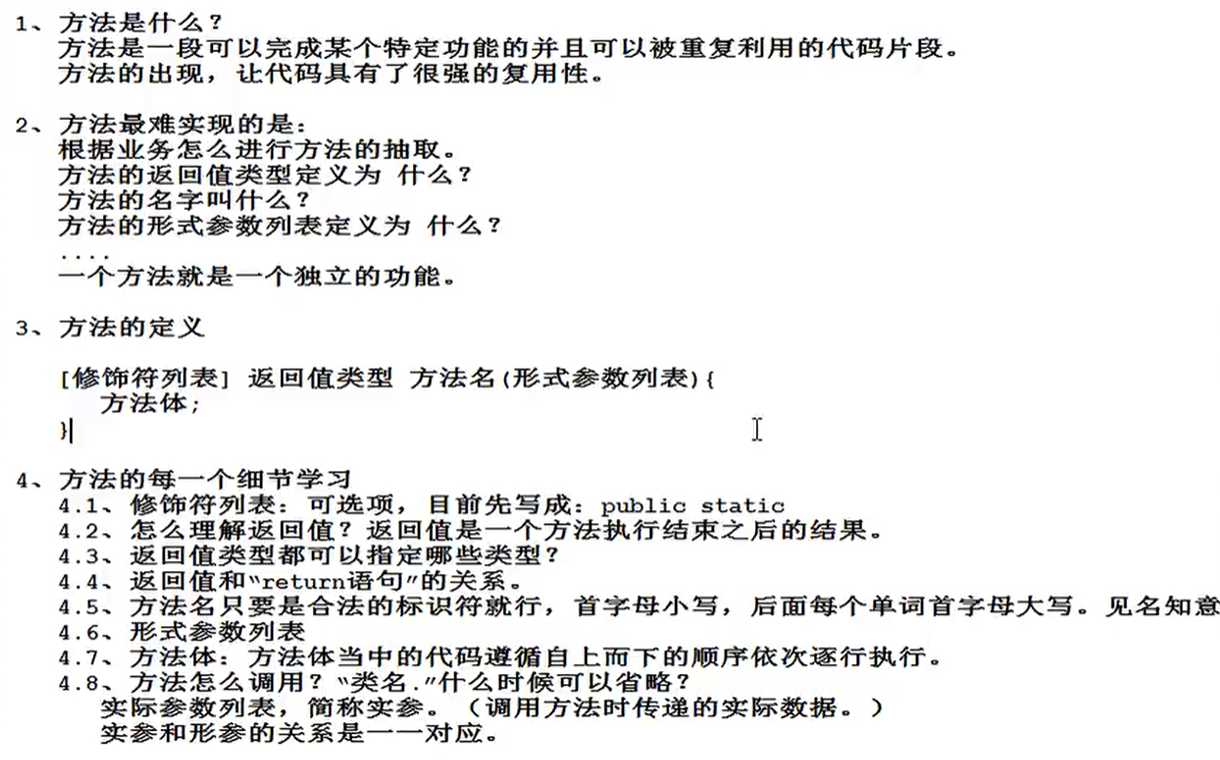
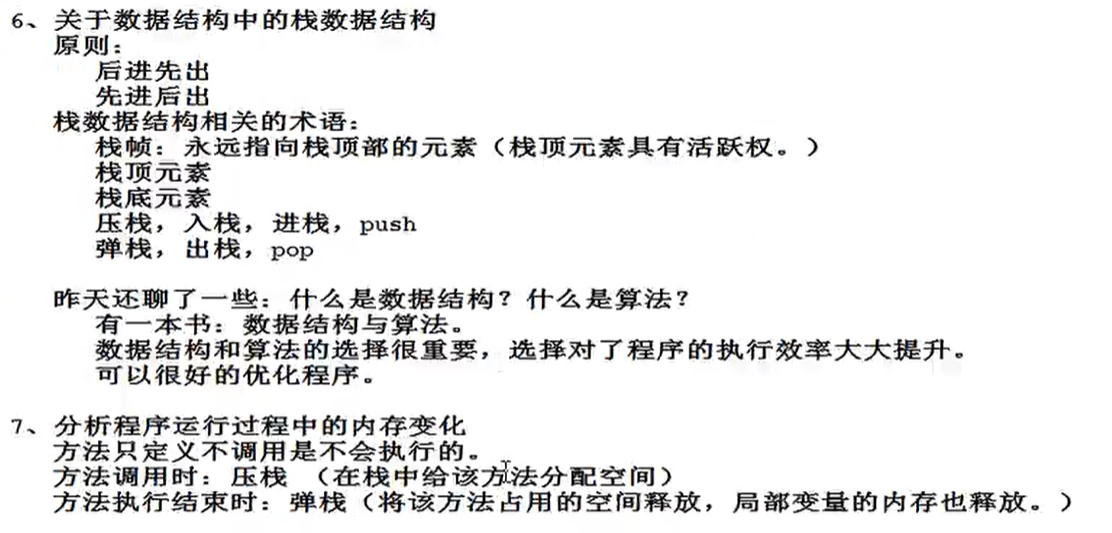
Method overload
package Method; //The program does not use method overloading to analyze the shortcomings of the program? //Programmers need to remember too many method names //The code is not beautiful enough public class OverloadTest01 { //Main method public static void main(String[] args){ int x = sumInt(10,20); System.out.println(x); long y = sumLong(20L,10L); System.out.println(y);; double z = sumDouble(10.00,21.00); System.out.println(z); } public static int sumInt(int a, int b){ return a+b; } public static long sumLong(long a, long b){ return a+b; } public static double sumDouble(double a, double b){ return a+b; } }
package Method; /* Using method overloading mechanism Advantage 1: neat and beautiful code Advantage 2: method names with similar functions can be overlapped How to distinguish languages in java language? First, the java compiler first distinguishes by method names, but the same method name is allowed in the Java language If the method names are the same, the compiler will distinguish the methods by their parameter types */ public class overloadTest02 { public static void main(String[] args){ System.out.println(sum(10, 20)); System.out.println(sum(10l, 20l)); System.out.println(sum(20.0, 10.0)); } public static int sum(int a,int b){ System.out.println("int Sum"); return a+b; } public static long sum(long a,long b){ System.out.println("long Sum"); return a+b; } public static double sum(double a,double b){ System.out.println("double Sum"); return a+b; } }
When will method overloading occur?
In a class, function 1 and function 2 have similar functions; It can be considered that their method names are consistent, so that the code is beautiful and easy to write later
When does the code overload methods?
- Condition 1: in the same class
- Condition 2: same method name
- Condition 3: different parameter lists
- Different types of parameters; The number of parameters is different; The order of parameters is different
be careful:
- Method overloading is independent of the return value type of the method
- Method overloading is independent of the modifier list of the method
package Method; /* When will method overloading occur? In a class, function 1 and function 2 have similar functions It can be considered that their method names are consistent, so that the code is beautiful and easy to write later When does the code overload methods? Condition 1: in the same class Condition 2: same method name Condition 3: different parameter lists Different types of parameters; The number of parameters is different; The order of parameters is different */ /* Do you recognize println() as a method name */ public class overloadTest03 { public static void main(String[] args){ //println is the name of the method. It is written by sun company and can be used directly //The println() method must be overloaded //For println, just remember a method name //The parameter type can be passed freely, which indicates that println() is overloaded System.out.println(10); System.out.println("12"); System.out.println(true); } }
Method recursion
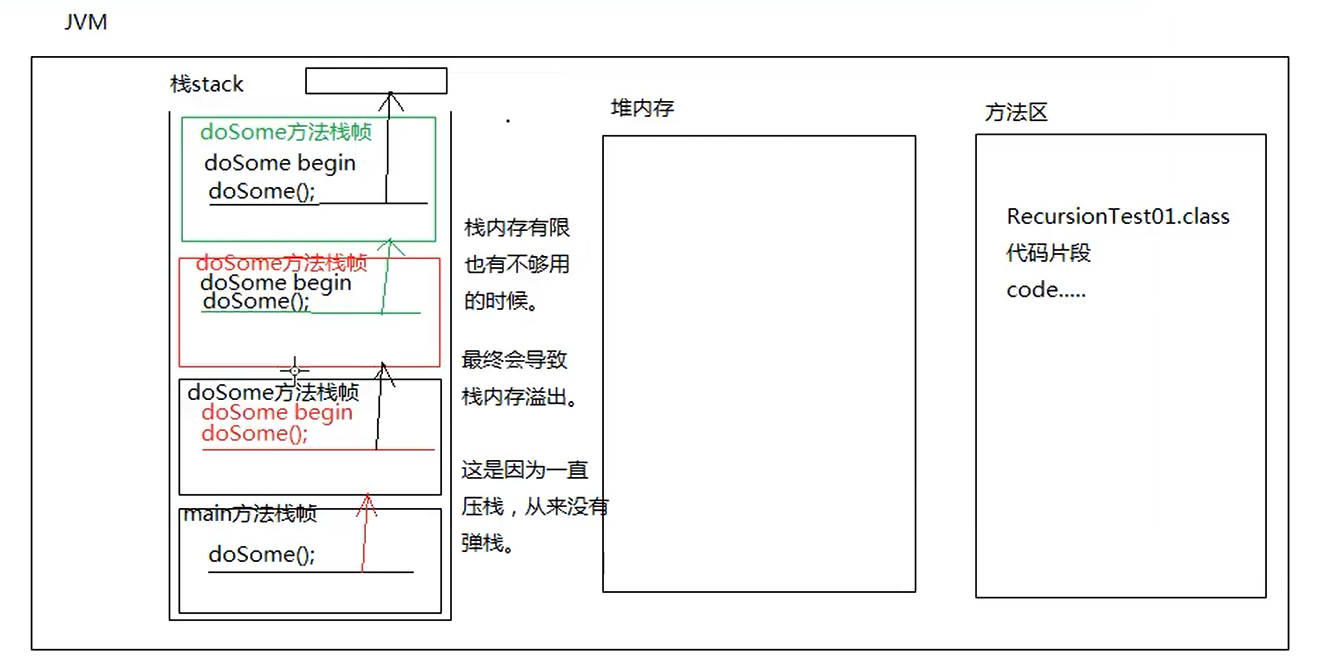
package Method; /* 1.What is method recursion? The method calls itself, which is the recursion of the method 2.When recursion occurs, the program has no end condition, and a stack overflow error will occur So recursion must end the condition 3. */ public class RecursionTest01 { public static void main(String[] args){ doSome(); } public static void doSome(){ System.out.println("doSome begin"); //Calling method: doSome() is a sail, can doSome method be called? Certainly. //At present, this method has no stop conditions. What problems will happen to the computer? doSome(); //This line will never be implemented System.out.println("doSome over"); } } The code will have a memory overflow error of the stack
1. What is method recursion?
The method calls itself, which is the recursion of the method
2. When recursion occurs, the program does not have an end condition, and a stack overflow error will occur, so recursion must end the condition
3. If recursion assumes an end condition, will stack overflow errors not occur?
Assuming that the end condition is correct and legal, sometimes there will be stack memory errors in recursion, because the recursion may be too deep and take up not enough memory, because it is always pressing the stack.
4. In the actual development, it is not recommended to use recursion easily. If you can use the for loop while loop instead, try to use the loop as much as possible, because the efficiency of the loop is high, the memory consumed by recursion is small, and the memory consumed by recursion is relatively large. In addition, improper use of recursion may lead to the death of JWM (but in rare cases, there is no way to implement it without recursive program)
5. In the actual development, if you encounter the error of stack overflow, how to solve this problem?
- Step 1: check whether the end condition of recursion is correct
- Step 2: the recursion condition is correct. At this time, you need to manually adjust the initialization size of JVM stack memory to make the stack memory larger.
- Step 3: adjust the size. If there is still an error at runtime, you can only continue to expand the size of stack memory.
- The java-x parameter allows you to view the parameters for resizing the stack
package Method; /* The sum of 1-n is not calculated recursively */ public class RecursionTest02 { public static void main(String[] args){ int N = 100; int res = sum(N); System.out.println(res); } public static int sum(int n){ int result = 0; for(int i = 1; i<= n ; i++){ result += i; } return result; } }
package Method; /* Use recursion to sum 1-n */ public class RecursionTest03 { public static void main(String[] args){ System.out.println(sum(100)); } public static int sum(int n) { if (n == 1) { return 1; } return n + sum(n - 1); } }
Recursive memory graph
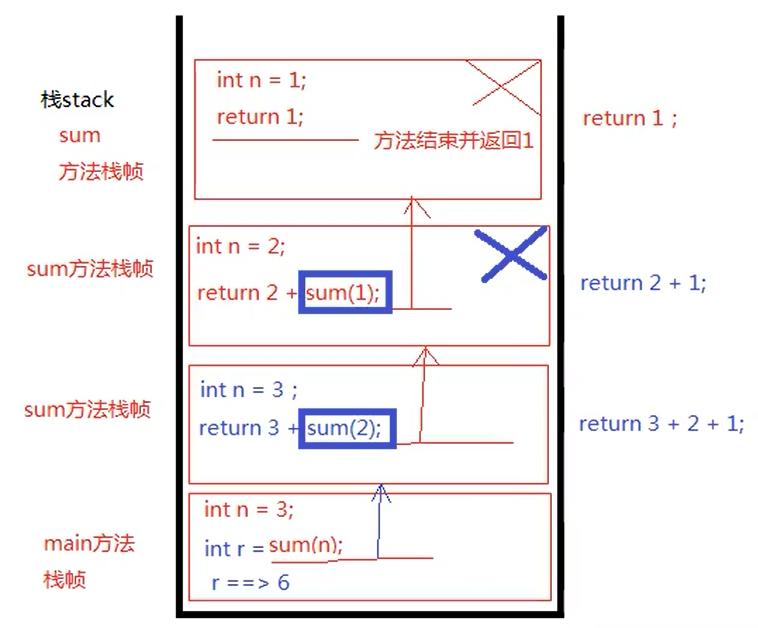
Recursive factorization
package Method; /* Factorization using recursion */ public class RecursionTest03 { public static void main(String[] args){ System.out.println(jicheng(5)); } public static int jicheng(int n){ if(n ==1 ){ return 1; } return n * jicheng(n-1); } }
Understanding object oriented
1. What is the difference between process oriented and object-oriented?
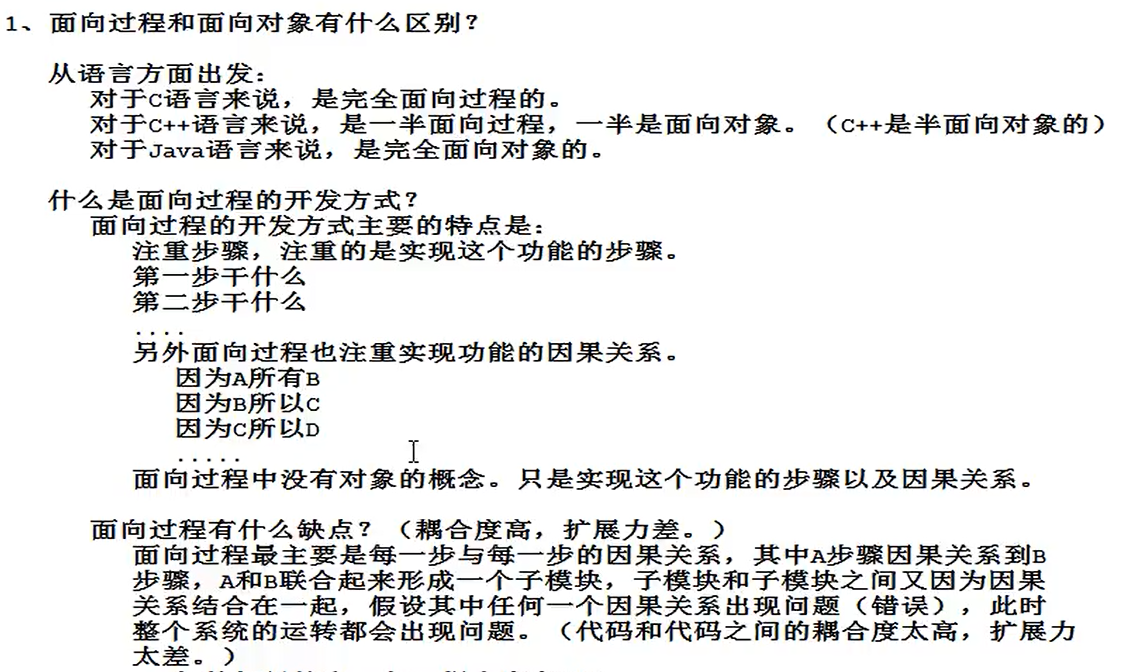
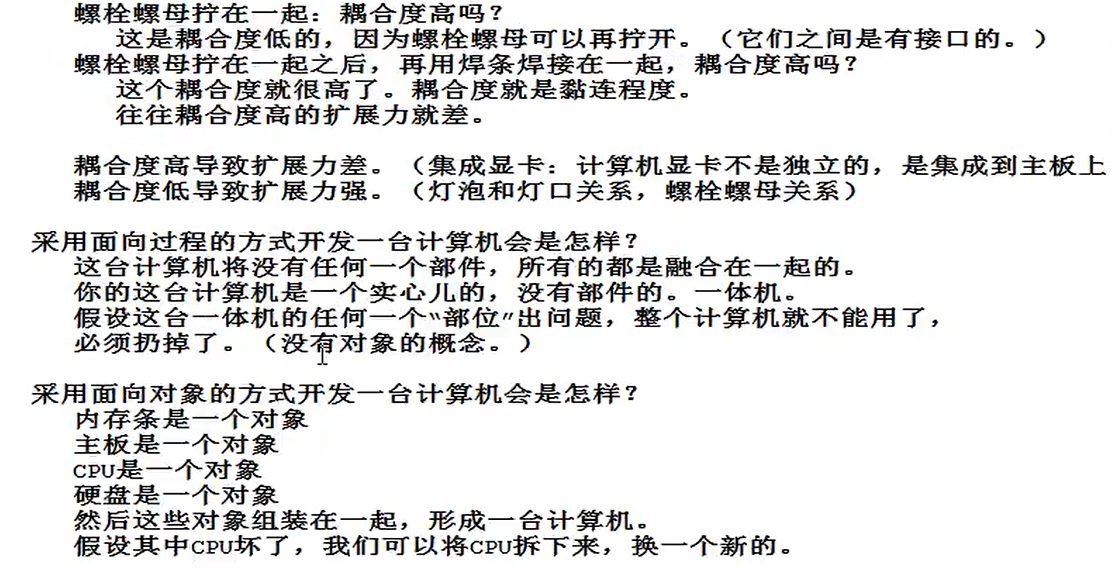

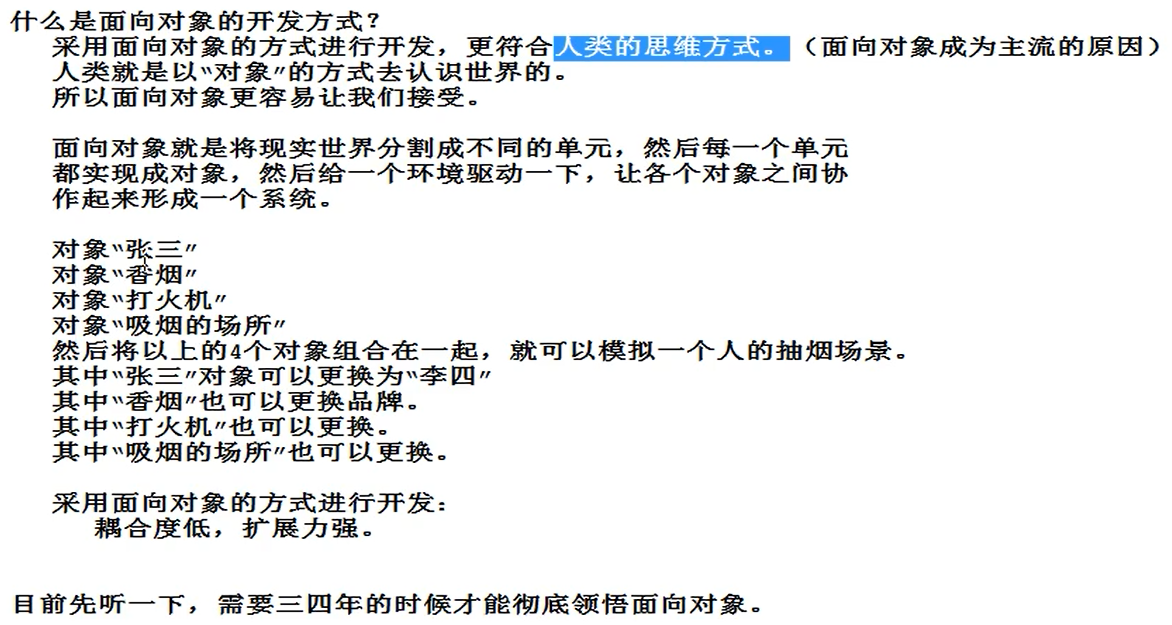
2. When we run through the whole system in an object-oriented way, three terms are involved:
OOA: Object Oriented Analysis
OOD: Object Oriented Design
OOP: Object Oriented Programming
The whole software development process is run through by OO
The process of implementing a software:
Analysis A ➡ Design D ➡ Programming P
3. Three characteristics of object-oriented
Encapsulation, inheritance, polymorphism
Any object-oriented programming language includes these three features: for example, python
Note: java is just one of the object-oriented languages.
4. Concept of class and object
What is a class?
In fact, class does not exist in the real world. It is an abstract concept, a template, and a result of our human brain's thinking, summary and abstraction
Stars are a class
What is an object? (real individuals)
An object is an entity that actually exists. An object has another name called an instance
The process of creating objects through classes is called instantiation
Yao Ming is an object
Andy Lau is an object
Both objects are stars
In the java language, if you want to get the "object", you must first define the "class". The "object" is created through the "class" template.
A class is a template: it describes the "common characteristic information" of all objects
An object is an individual created through a class
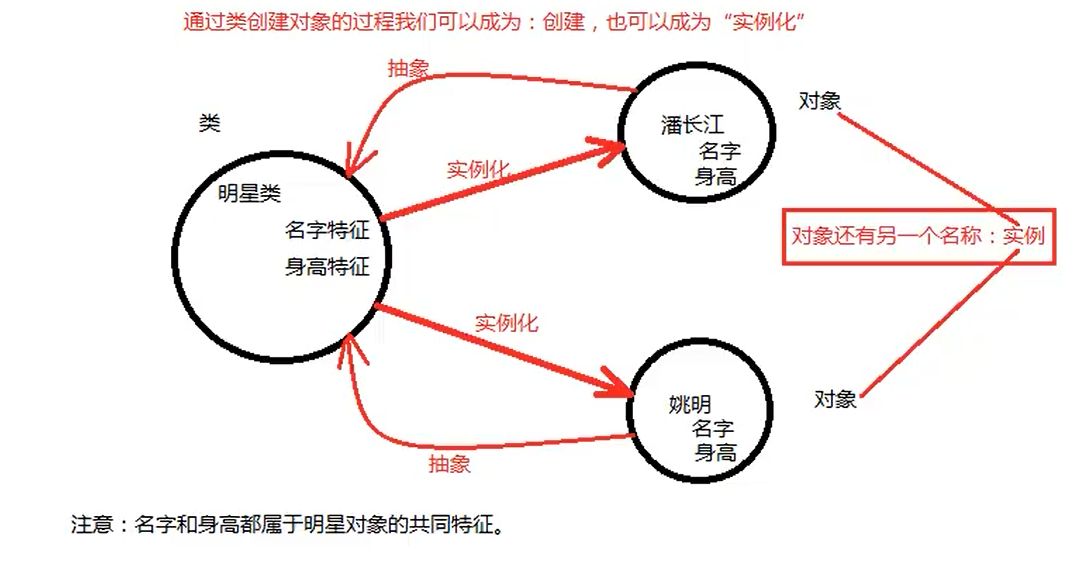

Class - [instantiation] - > object (instance)
Object - [Abstract] - > class
Common features of classes
A class is a template that describes a common feature. What are the common features?
State characteristics and action characteristics
Class = attribute + method
- Attribute from: Status
- Method from: action
5. What is the role of Java software engineers in development?
java is a bridge between the virtual world and the real world
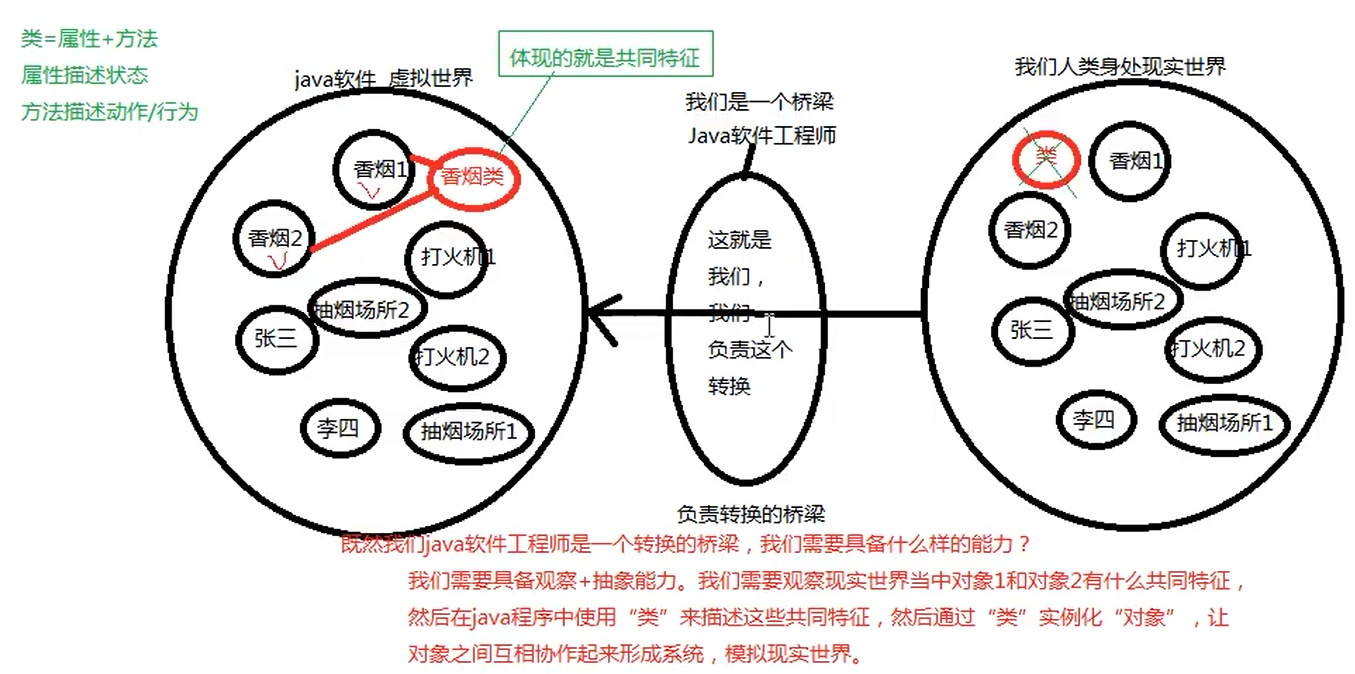
6. Definition of class
How to define a class and what is the syntax format?
[Modifier list] class Class name{ //Class = attribute + method //Attributes exist as "variables" in code //Method description action; Property description status }
Why do attributes exist as "variables"?
Attribute corresponds to data, which can only be put into variables
Conclusion: attributes are variables
Variable: divided according to the location of occurrence:
- Variables declared in the method body: local variables
- Method declared variables: member variables
package lei; /* 1.Common characteristics of students: Student id int, name String, gender char, address String Note: attributes are member variables */ //Students is both a class name and a type name, belonging to the reference data type public class Students { //attribute //Member variable int xuehao; String name; int age; boolean gender; String adress; }
package lei; /* Object creation and use What is the syntax for creating objects? */ public class StudentTest { public static void main(String[] args){ //You can use the of the Student class /* Syntax for creating objects; new new Class name (); new is an operator dedicated to the creation of objects Data type variable name = new class name (); There are two data types: basic data type and reference data type java All classes in are reference data types */ Students s = new Students(); //Students is a reference data type //new Students() is an object } }
7. Compilation process
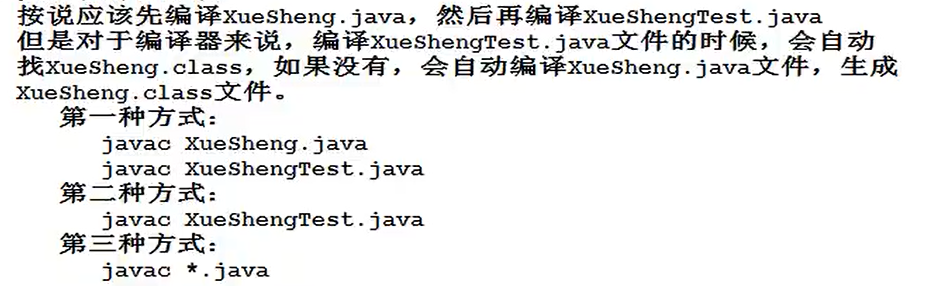
Creation and use of objects
Object creation
Class name variable name = new class name ();
This completes the creation of the object
Variables must be declared before they can be accessed after assignment
Note: for member variables, there is no manual assignment, but the system assigns values automatically, which is the default value
Type default
byte 0
short 0
long 0L
int 0
float 0.0F
double 0.0
boolean flase
char \u000
Reference data class null
What are instance variables
An instance is an object, which is also called an instance;
Instance variables are actually object level variables
The object and its instance variables are stored in heap memory
When accessing instance variables, do you have to create objects first?
//Can I access student names directly through class names? //The student name is an instance variable, which is an object level variable //Do you have an object before you can have a name //Instance variables cannot be accessed directly through the class name //System.out.println(Students.name);
What is the difference between objects and references?
Objects are generated through new and stored in heap memory
References are: but any variable that holds a memory address and points to an object in heap memory is called a reference
A reference is a variable that stores the memory address of an object
Generally speaking, as long as the memory address of an object is stored in this variable, this variable is called a reference
The object is: new from the heap
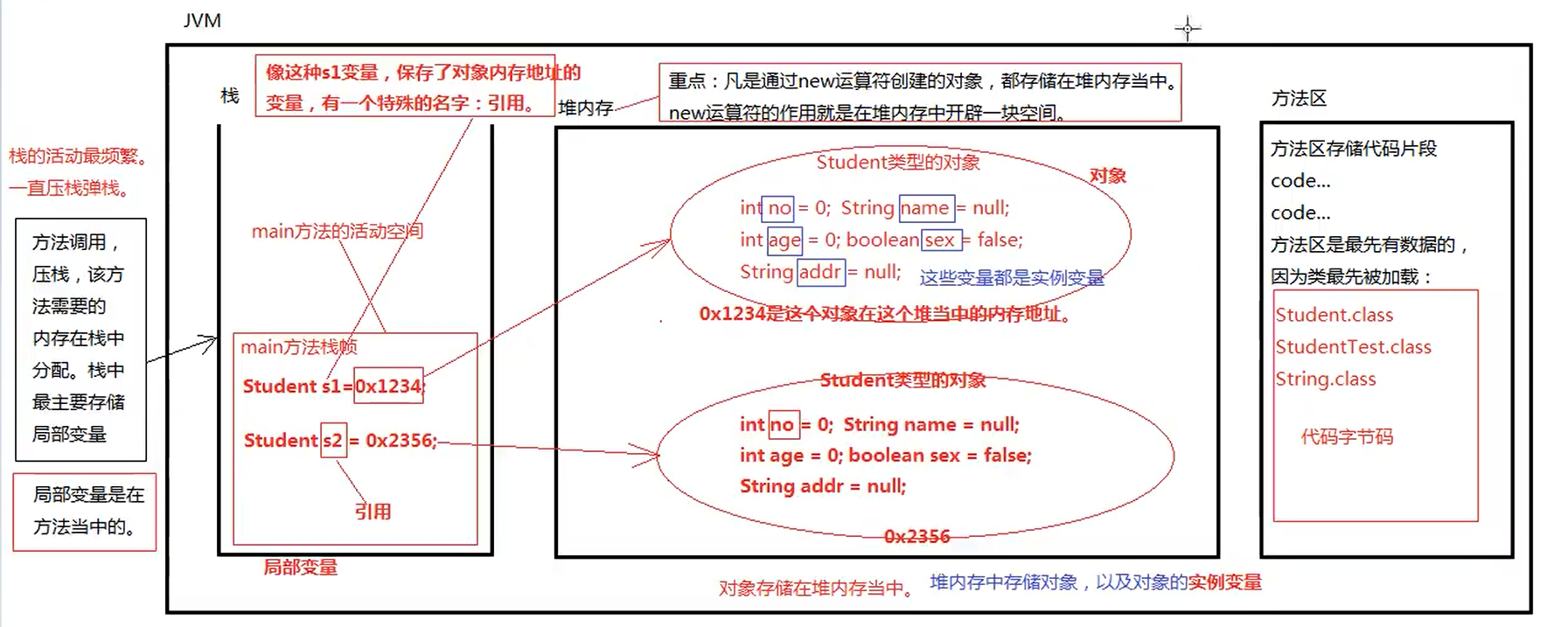
The access ID cannot use User.id, which is an error. Instance variables cannot be accessed by class name. The access of ID must first create an object and then have an object to access the ID of the object
Think: must a reference be a local variable? Not necessarily.
The three main memory in the JVM
Stack: it mainly stores local variables and class object addresses, the space required for method execution, and local variables; as long as they are local variables in the method body, they are allocated in the stack
Heap memory: mainly stores objects and instance variables of objects
Method area: mainly stores code fragments
Memory diagram example:
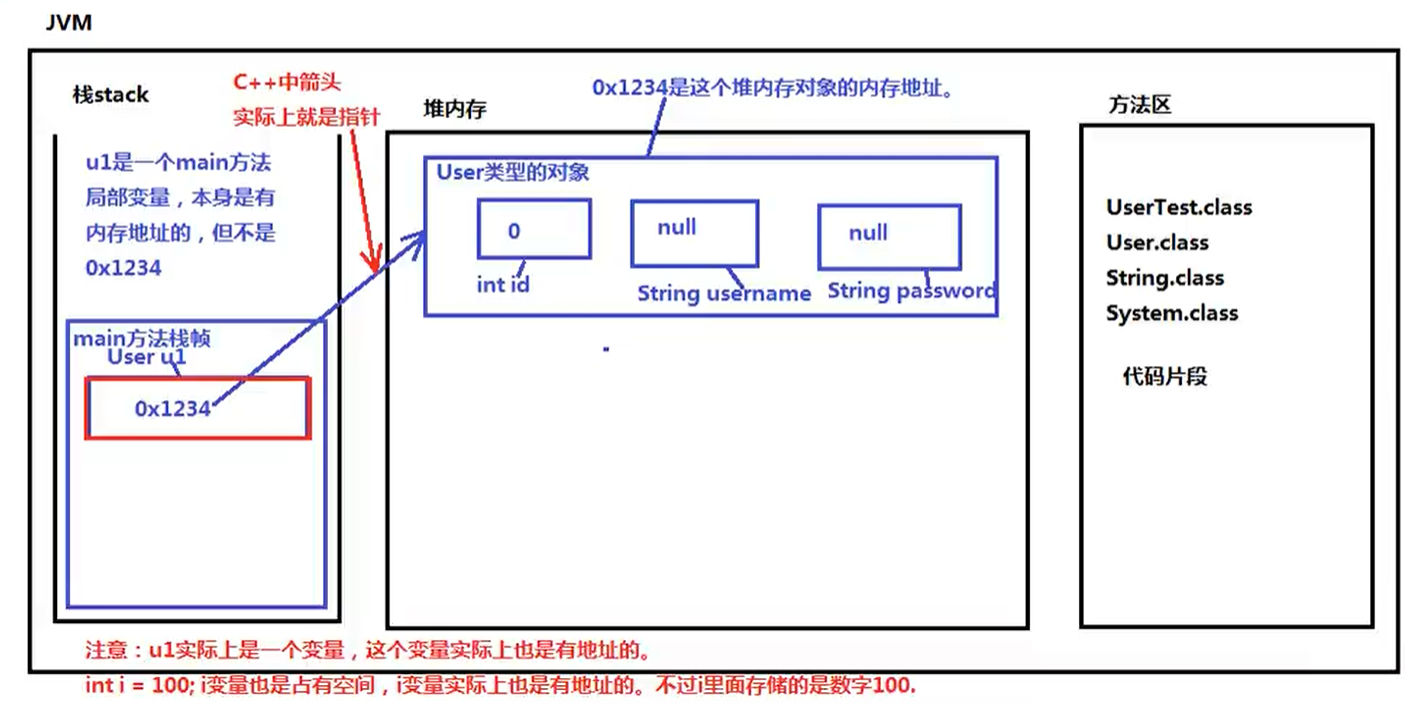
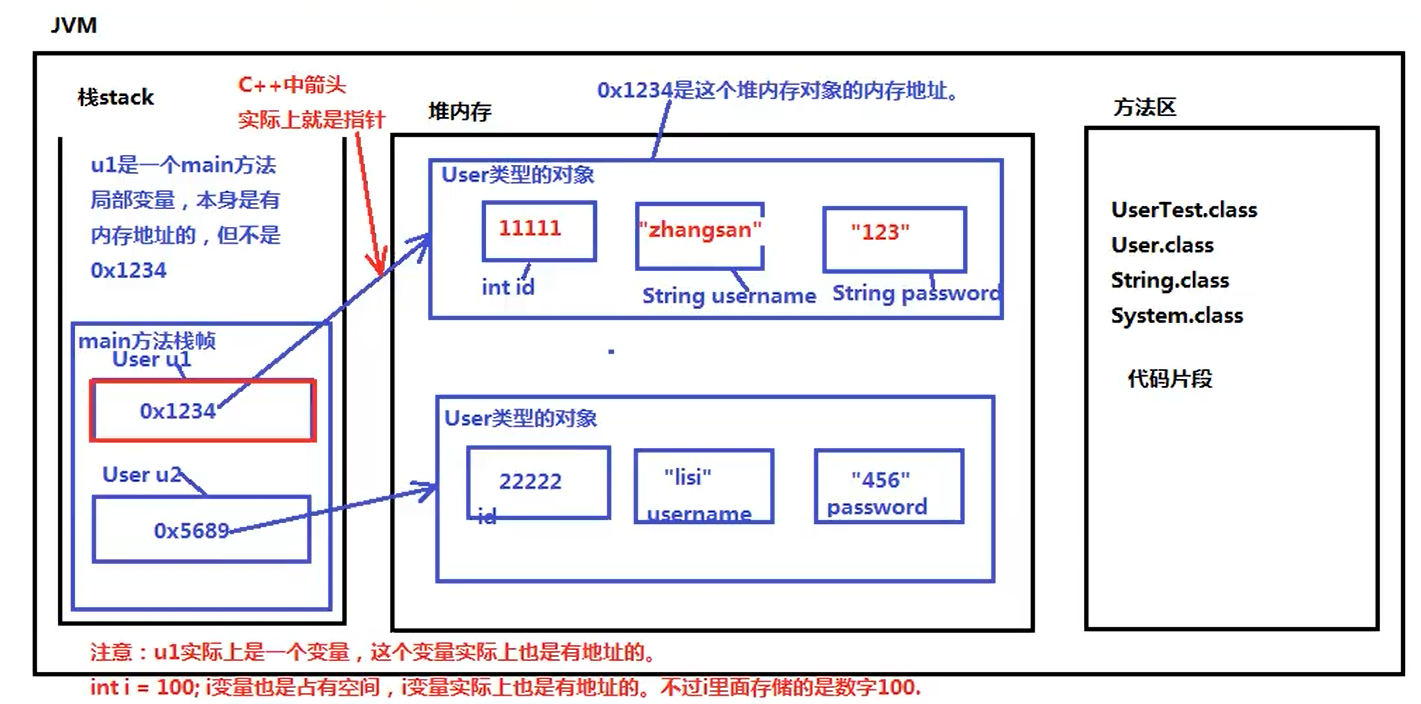
Example 2:
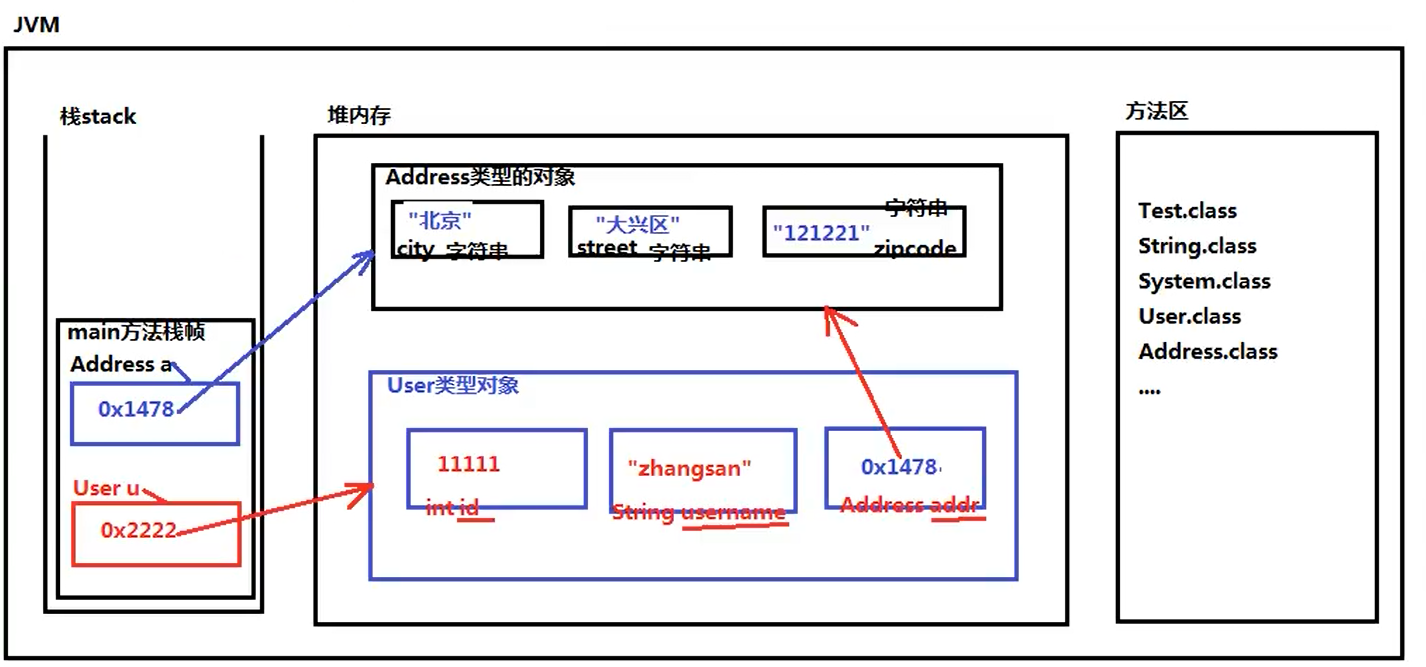
package lei; /* Remember: you can order what's in it All instance variables are accessed through "reference." */ public class Test01 { public static void main(String[] args){ Address a = new Address(); a.city = "Beijing"; a.street = "Daxing District"; a.zipcode = "10010"; System.out.println(a.city); System.out.println(a.street); System.out.println(a.zipcode); Users user1 = new Users(); user1.add = a; user1.id = 123; user1.name = "yxy"; /*System.out.println("============"="Represents assignment operation ================== =); int x = 100; int y = x; Means to copy the value 100 saved in the x variable to y */ /* Add K = new Add(); Add m = k; Add k = 0x1111; Then m = 0x1111; */ //In java, only values are passed. Values may be variables, addresses, and so on System.out.println(user1.id); System.out.println(user1.name); System.out.println(user1.add.city); System.out.println(user1.add.zipcode); System.out.println(user1.add.street); } } /* *A reference is a variable that stores the memory address of an object** Generally speaking, as long as the memory address of an object is stored in this variable, this variable is called a reference *The object is: new from the heap** * * Think: must references be local variables? * Not necessarily */
package lei; public class Address { String city; String street; String zipcode; }
package lei; public class Users { int id; String name; Address add; }
Property reference
package lei; public class T { A a; public static void main(String[] args){ A a = new A(); B b = new B(); C c = new C(); D d = new D(); T t = new T(); c.d = d; b.c = c; a.b = b; t.a = a; System.out.println(t.a.b.c.d.i); } } class A{ B b; } class B{ C c; } class C{ D d; } class D{ int i = 100; }
Null pointer
package lei; /* Null pointer exception */ public class NullPointerTest { public static void main(String[] args){ //Create customer object Customer c = new Customer(); //id to access this object System.out.println(c.id); //Reassign id c.id = 45; System.out.println(c.id); c =null; /* There is no problem with the compiler, because the compiler only checks the syntax. The compiler finds that c is a Customer type and there is an id attribute in the Customer type, so it can However, the existence of the object is required during operation, but the object is gone. Only one exception can be thrown */ System.out.println(c.id); /* Null pointer error Exception in thread "main" java.lang.NullPointerException at lei.NullPointerTest.main(NullPointerTest.java:16) */ } } class Customer{ int id; //For instance variables in member variables, you should first create objects and then access them through "reference" }
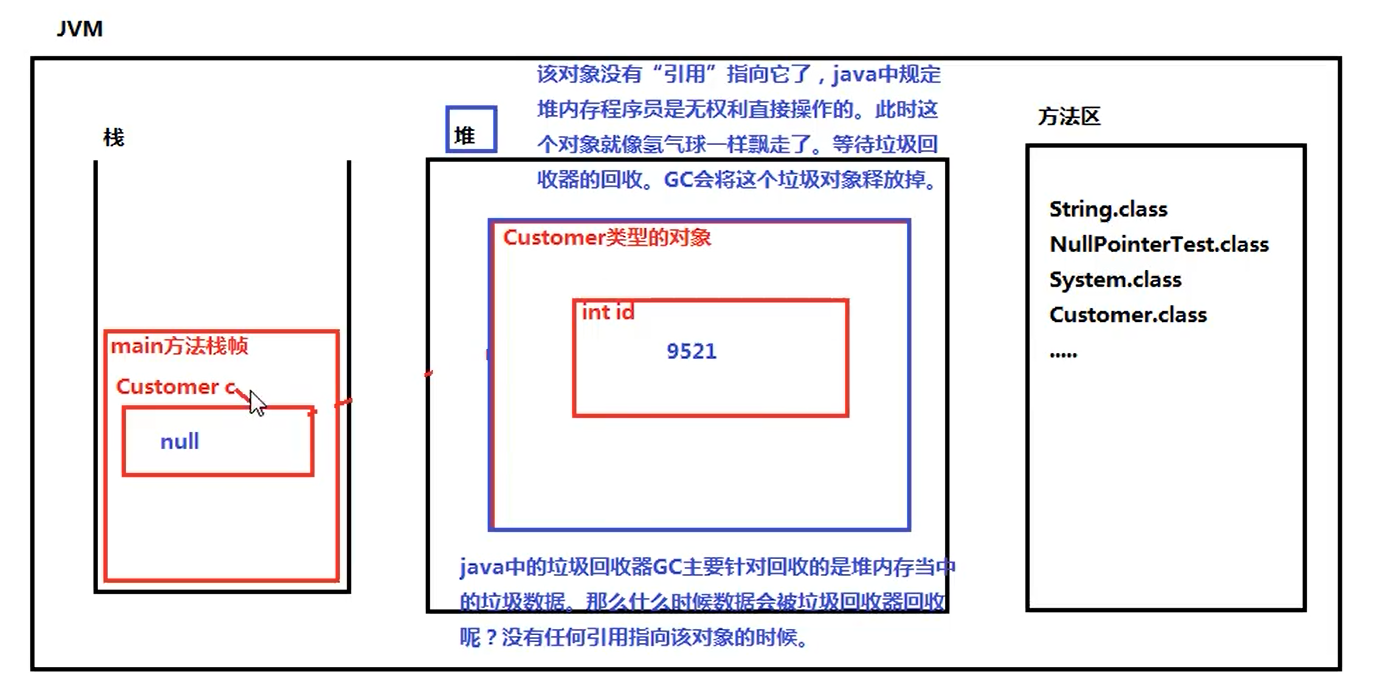
As for the garbage collector GC, in the java language, the java collector is mainly aimed at heap memory. When a java object has no reference to the object, the GC will consider releasing the garbage data to be recycled.
The condition of null pointer exception is that null pointer exception will occur when null reference accesses instance related data
Parameter passing during method call
java stipulates that when parameters are passed, they have nothing to do with the type. Whether it is a basic type or a reference data type, it is agreed that they are the values saved in the box, and a copy is copied and passed on
package canshuchuandi; //Parameter passing of analyzer //java stipulates that when parameters are passed, they have nothing to do with the type. Whether it is a basic type or a reference data type, it is agreed that they are the values saved in the box, and a copy is copied and passed on public class Test01 { public static void main(String[] args){ int i = 10; add(i); System.out.println("main-->" + i); //10 } public static void add(int i){ // i in the add field //Copy the number 10 stored in the i variable box in main and pass it to the add method i++; System.out.println("add-->" + i); //11 } }
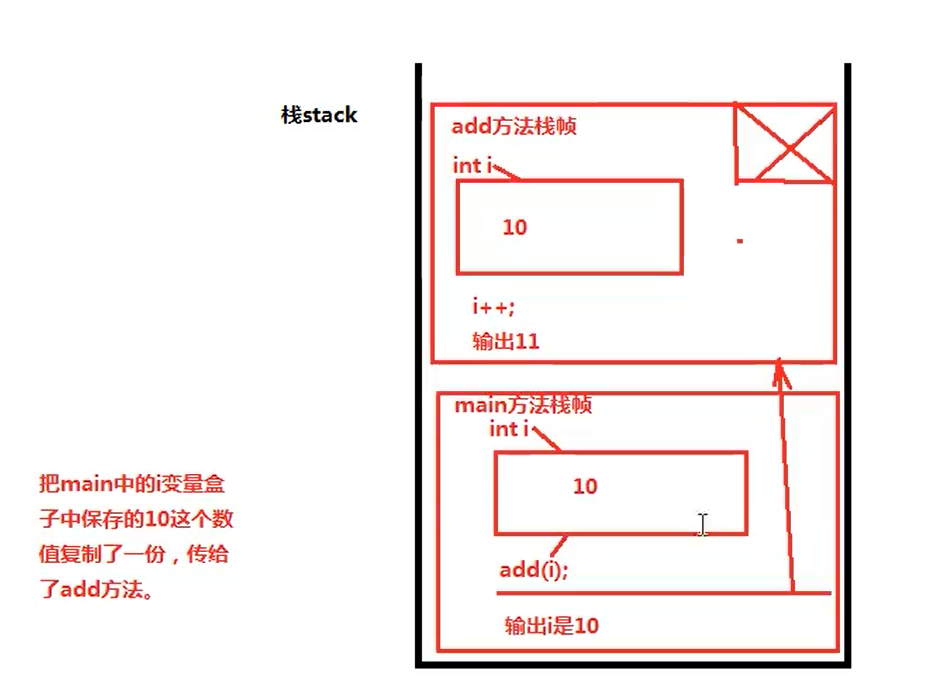
package canshuchuandi; public class Test02 { public static void main(String[] args) { Person p = new Person(); p.age = 10; add(p); System.out.println("main -->" + p.age); //11 } //Methods can be basic data types or reference data types, as long as they are legal data types public static void add(Person p){ p.age++; System.out.println("add -->" + p.age); //11 } } class Person{ int age; }
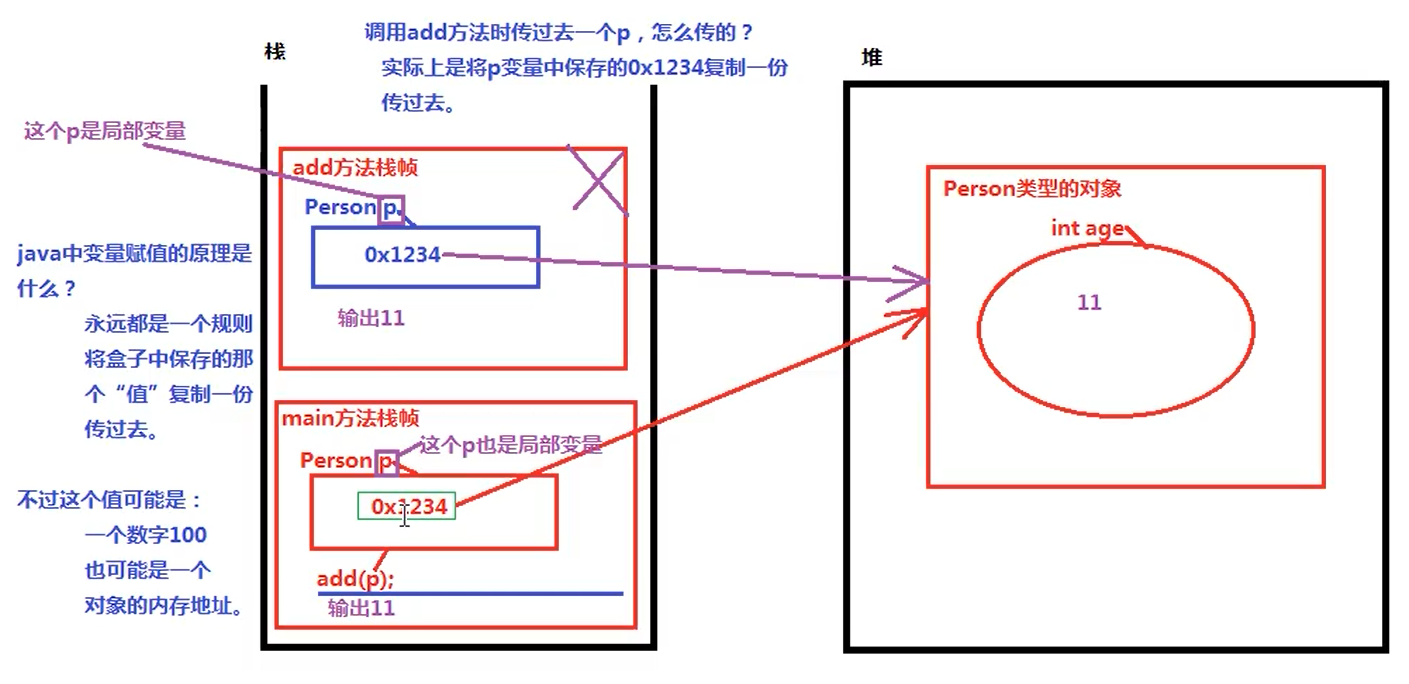
These two p are different local variables
Construction method Constructor
1. What is a construction method and what is its use?
Construction method is a special method. It can create objects and initialize instance variables,
In other words, the construction method is used to create objects and assign values to their attributes (Note: the system will assign default values when instance variables are not assigned manually)
2. When a class does not provide any constructor, but the system will actually provide a parameterless constructor by default (this method will be called the default constructor)
3. How to call the constructor?
Use the new operator to construct the method; Syntax format: new construction method name (actual parameter list)
4. What is the grammatical structure of the construction method?
[Modifier list] Construction method name(Formal parameter list){ Construction method body; }
Note: 1. At present, the modifier list is written in public, and do not write in public static
2. The constructor name and class name must be consistent
3. The constructor name does not need to specify any return value type and write void
Syntax structure of common methods
[Modifier list] Return value type method name(Formal parameter list){ Method body; }
5. Nonparametric construction method and parametric construction method
-
When no constructor is provided in a class, the system provides a parameterless constructor by default. This parameterless constructor is called the default constructor.
-
If a class manually provides a construction method, the system will not provide a parameterless construction method. It is recommended to write the parameterless construction method manually, so there will be no problem
-
Both parameterless constructors and parameterless constructors can be called
-
Does the constructor support method overloading?
Method overloading can be supported. There can be multiple construction methods in a class, and the names of all construction methods are the same
Method overloading has one feature:
In the same class, the method names are the same and the parameter lists are different
-
For instance variables, as long as you do not assign values manually in the construction method, the system will assign values by default
-
Constructor: creates an object and assigns a value to the attribute
package Constructor; /* Construction method: 1.What is a construction method? What's the usage? Construction method is a special method. It can create objects and initialize instance variables, In other words, the construction method is used to create objects and assign values to their attributes (Note: the system will assign default values when instance variables are not assigned manually) 2.When a class does not provide any constructor, but the system will actually provide a parameterless constructor by default (this method will be called the default constructor) 3.How to use the call constructor? Use the new operator to construct the method Syntax format: new construction method name (actual parameter list) 4.What is the syntax structure of the construction method? void cannot be written without return value type [Modifier list] constructor name (formal parameter list){ Construction method body; } Note: 1. At present, the modifier list is written in public, and do not write in public static 2.Constructor name and class name must be consistent 3.The constructor name does not need to specify any return value type or write void Syntax structure of common methods [Modifier list] return value type method name (formal parameter list){ Method body; } */ public class Test01 { public static void main(String[] args){ //Call parameterless constructor new Students(); //Call normal method Test01.doSome(); doSome(); //Call the parameterless constructor of the Students class //Create an object of type students Students s1 = new Students(); System.out.println(s1); //Output hash value //Call constructor with parameters Students s2 = new Students(100); System.out.println(s2); } //Common construction method public static void doSome(){ System.out.println("ds"); } } class Students{ int no; int age; String name; //When Students do not have a constructor, the system will actually provide a parameterless constructor by default //Construction method public Students(){ System.out.println("No parameter execution"); } public Students(int no) { this.no = no; } //heavy load //public void Students(String name){} public Students(String name){ this.name = name; } }
Call different construction methods to create objects
package Constructor; public class Test03 { public static void main(String[] agrs){ //Call different construction methods to create objects Vip v1 = new Vip(); Vip v2 = new Vip(111,"y"); Vip v3 = new Vip(222,"x","123"); Vip v4 = new Vip(333,"y","123",true); System.out.println(v1.no); System.out.println(v1.name); System.out.println(v1.birth); System.out.println(v1.sex); System.out.println("----------------------"); System.out.println(v2.no); System.out.println(v2.name); System.out.println(v2.birth); System.out.println(v2.sex); System.out.println("----------------------"); System.out.println(v3.no); System.out.println(v3.name); System.out.println(v3.birth); System.out.println(v3.sex); System.out.println("----------------------"); System.out.println(v4.no); System.out.println(v4.name); System.out.println(v4.birth); System.out.println(v4.sex); } }
0 null null false ---------------------- 111 y null false ---------------------- 222 x 123 false ---------------------- 333 y 123 true Process finished with exit code 0
Three characteristics of object-oriented - encapsulation, inheritance and polymorphism
With encapsulation, there is inheritance. With inheritance, there is polymorphism
encapsulation
What is encapsulation? What's the use?
In real life, many real examples are encapsulated, such as mobile phones, televisions, etc
Package to protect the internal components. In addition, after packaging, we can't see the internal complex structure for our users.
Function of encapsulation:
If the data in a class body is encapsulated, the caller of the code does not need to care about the complex implementation of the code, but can access it through a simple entry. In addition, the data with high security level in the class body is encapsulated, and external personnel cannot access it at will to ensure the security of the data.
How to package
Step 1: attribute privatization, private
Step 2: provide a simple operation entry to the outside world, and provide public set methods and get methods. Both methods do not have static, and they are object level methods
You can set up checkpoints in the set method to protect the security of data
Note: in the java development specification, the set method and get method must meet the following formats and cannot be static
get Method requirements: public return type get+Attribute names are capitalized(No parameter){ return xxx; }
set Method requirements: public void+Attribute names are capitalized (with one parameter){ xxx = Parameters; }
Without encapsulation:
package fengzhuang; //Accessing Person in an external program public class PersonTest { public static void main(String[] args){ //Create Person object Person p1 = new Person(); //There are two operations to access the properties of an object: one is to read data and the other is to change data p1.age = 50; System.out.println(p1.age); //In the external program of Person, you can operate age at will } } class Person { //Instance variables, attributes int age; }
With encapsulation:
package fengzhuang; //Accessing Person in an external program public class PersonTest { public static void main(String[] args) { //Private means private. After being modified by this keyword, the data can only be accessed in this class. This attribute cannot be accessed except this class /* Person age,Completely inaccessible externally p1.age = 20; //read System.out.println(p1.age); */ Person p1 = new Person(); //get and set methods cannot be called through the class name. p1.setAge(-1); int age = p1.getAge(); System.out.println(age); } }
package fengzhuang; //This is a Person without encapsulation /* public class Person { //Instance variables, attributes int age; } */ //Start to encapsulate the code without exposing complex data //Encapsulated to protect internal data and ensure its security public class Person{ //Property privatization private int age; //Provide simple operation access to the outside /* Write special methods to complete reading and writing (get, set) The public set method and get method are provided externally, and both methods do not have static. They are object level methods */ //Note: in the java development specification, the set method and get method should meet the following formats /* get Method requirements: public Return value type get + property name initial capital (no parameter){ return xxx; } set Method requirements: public void+Attribute names are capitalized (with one parameter){ xxx = Parameters; } */ public int getAge(){ return age; } public void setAge(int age) { if(age <0 || age >150){ System.out.println("Illegal age"); return; //Program termination } this.age = age; } }
static keyword
Static variables are initialized when the class is loaded
- All static keywords are class related and class level
- All static modifications are accessed in the way of "class name"
- Static modified variable: static variable
- Static modification method: static method
Classification of variables:
Variables are divided according to the declared position: variables in the method body are called local variables;
Variables outside the method are called member variables.
Member variables can be divided into:
Instance variable
Static variable
1. When the country is not a static variable: it will lead to a waste of memory, and the country will be put in the heap
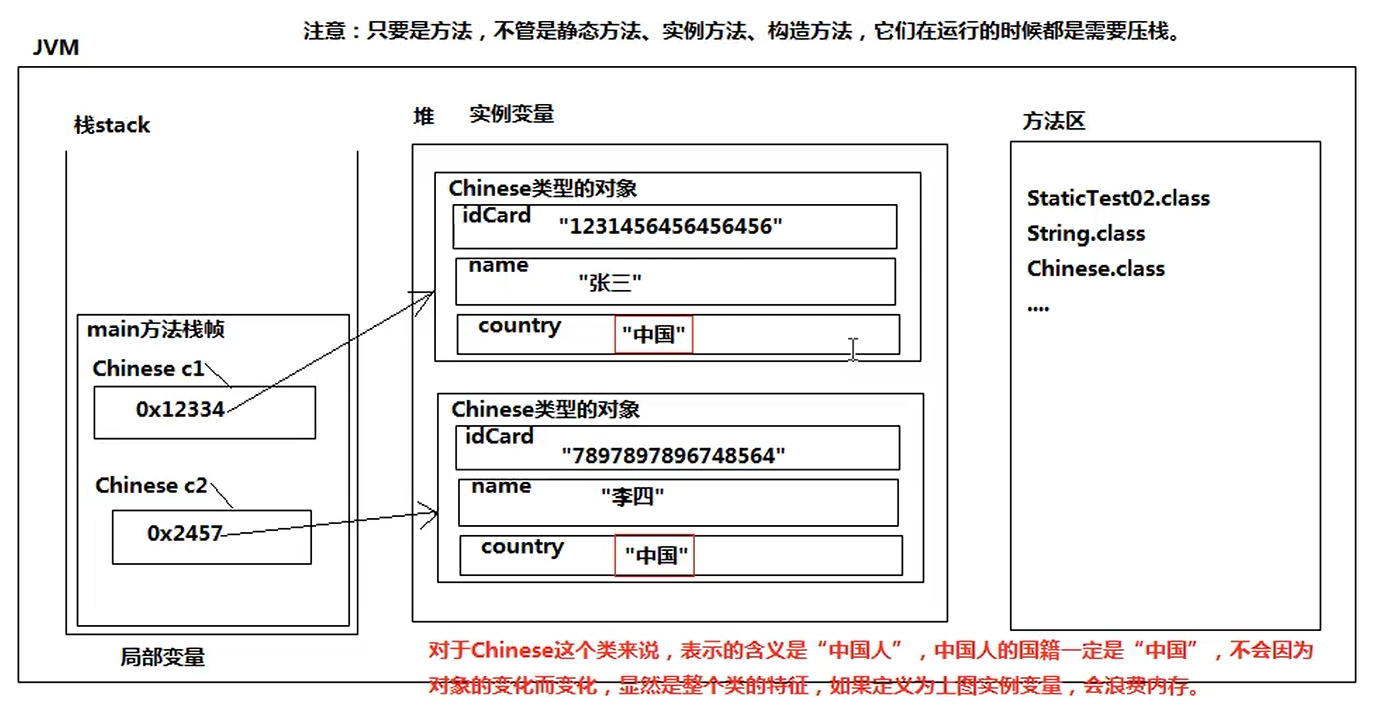
package Staticpackage; /* When is it declared static and when is it declared instance */ public class Test02 { public static void main(String[] args){ Chinese c1 = new Chinese("123456","yxy"); Chinese c2 = new Chinese("123456789","yxa"); } } class Chinese{ //The ID number should be an instance variable, one person per copy. //The name should also be an instance variable String idCard; String name; //Nationality is a fixed value, so it is a static variable String country; public Chinese() { } public Chinese(String idCard, String name) { this.idCard = idCard; this.name = name; } }
2. When country is a static variable, the static variable is placed in the method area
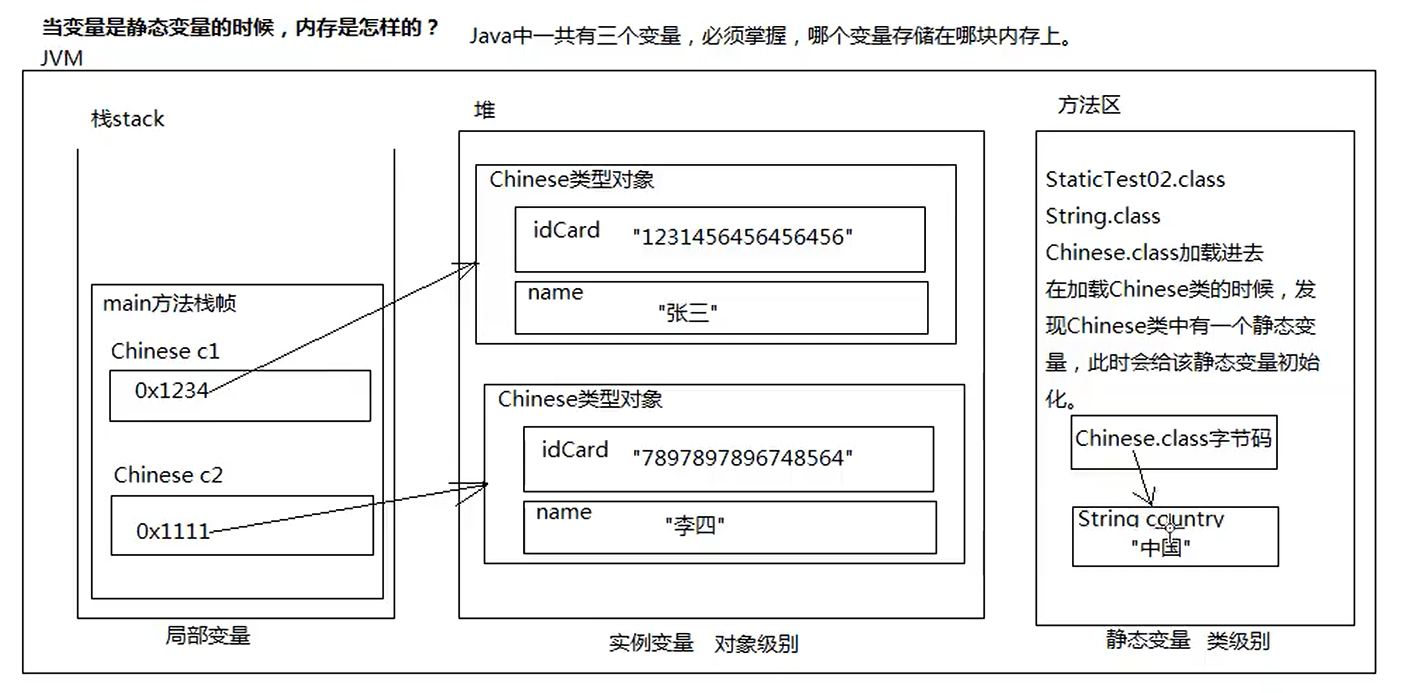
package Staticpackage; /* When is it declared static and when is it declared instance If a property value of this object is the same, it is not recommended to define it as an instance variable. It is recommended to define it as a class level feature and a static variable, which can be retained in the method area to save overhead */ public class Test02 { public static void main(String[] args){ //Output of static variables System.out.println(Chinese.country); Chinese c1 = new Chinese("123456","yxy"); Chinese c2 = new Chinese("123456789","yxa"); System.out.println(c1.idCard); System.out.println(c1.name); System.out.println(c2.idCard); System.out.println(c2.name); //System.out.println(Chinese.idCard); //The program reports an error because idCard is an instance variable and must be accessed by reference } } class Chinese{ //The ID number should be an instance variable, one person per copy. //The name should also be an instance variable String idCard; String name; //Declared as a static variable also has an initial value, which is null. This initial value is initialized when the class is loaded //And static variables exist in the method area //Nationality is a fixed value, so it is a static variable static String country = "China"; public Chinese() { } public Chinese(String idCard, String name) { this.idCard = idCard; this.name = name; } }
3. Null pointer exception
package Staticpackage; /* Instance: should be accessed by referencing Static: it is recommended to use "class name." to access Conclusion: Null pointer exception: null pointer exception will occur only when it is related to "null reference" and "access" instance " */ public class Test03 { public static void main(String[] args){ System.out.println(Chinese.country); //create object Chinese c1 = new Chinese("11", "yxy"); System.out.println(c1.country); System.out.println(c1.name); System.out.println(c1.idCard); //Null reference c1 = null; //No null pointer exception will occur, because static variables do not need the existence of objects. In fact, this code is still System.out.println(Chinese.country); System.out.println(c1.country); } } class Chinese{ String idCard; String name; public Chinese() { } static String country = "China"; public Chinese(String x, String y){ idCard = x; name = y; } }
4. Use of static methods
package Staticpackage; public class Test04 { public static void main(String[] args){ Test04.dosome(); Test04 st = new Test04(); st.doOther(); } //Static methods do not require new objects and use class names public static void dosome(){ System.out.println("Static method do some"); } //Instance methods need new objects, which are accessed by "reference". " public void doOther(){ System.out.println("Example method"); } }
5. Things in class
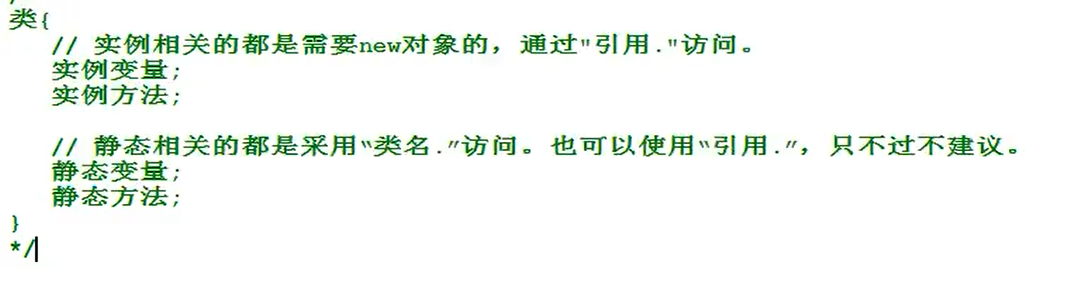
6. When are methods defined as static
Reference standard: if an instance variable is accessed in the method body, it must be an instance method. In most cases, the methods of a tool class are generally static (static methods do not need new objects and directly use type calls, which is extremely convenient. Tool classes are for convenience, so the methods in tool classes are generally static) Definition of example method: different objects have different test results. We can think that the test is something related to the object, so we define the test as example method
package Staticpackage; /* When is it defined as an instance method and when is it defined as a static method Reference standard: when the instance variable is accessed in the method body, it must be the instance method In most cases, the methods of tool classes are generally static (static methods do not need new objects, but directly use type calls) Extremely convenient. Tool classes are for convenience, so the methods in tool classes are generally static) Definition of example method: different objects have different test results. We can think that the test is something related to the object, so we define the test as example method */ //Class = attribute + method public class Test05 { public static void main(String[] args){ } } class User{ //Instance variable, object r required private int id; private String name; //First, name is at the object level. //Print the user's name, which is the instance method public void printName(){ } // new an object before you can use the get and set methods public void setId(int i){ id = i; } public int getId(){ return id; } }
7. Reason why static variable cannot be defined in main method
Only classes have static variables. Methods can only operate on static variables and cannot attempt to define static variables in methods
Static static block
- Static can define static blocks of code
Syntax structure: static{ java sentence; java sentence; }
-
Execution time: executed when the class is loaded, and only once
-
Note: multiple static code blocks can be written in a class. Static code blocks are executed when the class is loaded and before the main method is executed. Static code blocks are generally executed in top-down order
-
The purpose of static code block is to record the project log
Special timing: the timing of class loading and code release
package Staticpackage; /* static You can define static code blocks Syntax structure: static{ java sentence; java sentence; } Execution time: Class is executed when it is loaded, and only once characteristic: Multiple static code blocks can be written in a class. Static code blocks are executed when the class is loaded and before the main method is executed. Static code blocks are generally executed in top-down order** */ public class Test06 { //Static code block static{ System.out.println("A"); } //Multiple static code blocks can be defined in a class static{ System.out.println("B"); } public static void main(String[] agrs){ System.out.println("hello word"); } static{ System.out.println("C"); } }
Static variables are initialized during class loading, and static code blocks are executed during class loading, so static code blocks can access static variables,
The initialization of instance variables starts at the time of the new object, and the empty memory will be opened up when the construction method is executed
Summary: stack, heap, method area
Stack: as long as the method is executed, it will press the stack. The stack will provide the space required by the method and store local variables in the stack
Heap: store instance variables in the heap and new objects
Method area: class information, stored code fragments and static variables
So far, what is the execution code with sequential requirements?

Instance statement block
package Staticpackage; public class InstanceCode { //entrance public static void main(String[] args){ new InstanceCode(); new InstanceCode(); new InstanceCode("abc"); } //The instance statement block is not executed when the class is loaded { System.out.println("Instance statement execution"); } public InstanceCode() { System.out.println("Nonparametric construction"); } public InstanceCode(String name) { System.out.println("Parametric construction"); } }
The instance statement block is not executed when the class is loaded, it is executed before the constructor is executed, and it is executed once every time the new object is executed
The execution order of the learned code blocks
package Staticpackage; //Judge execution order public class CodeOrder { static{ System.out.println("A"); } public static void main(String[] args){ System.out.println("main begin"); new CodeOrder(); System.out.println("main over"); } public CodeOrder() { System.out.println("B"); } { System.out.println("C"); } static{ System.out.println("X"); } }
A X main begin C B main over
this keyword
-
this is a keyword, all lowercase
-
An object is a this. This is a variable and a reference. This stores the memory address of the current object and points to itself. Therefore, strictly speaking, this represents the "current object", which is stored in the heap memory.
-
This can only be used in instance methods. This is who calls this instance method, so this represents the current object.
-
It can be omitted in most cases
-
Why can't this be used in static methods?
this represents the current object, which is not required for static method calls
-
In the instance method or construction method, in order to distinguish between local variables and instance variables, this cannot be omitted
//this cannot be omitted package Staticandthis; /* this It can be used in instance methods, but not in static methods. this keyword can be omitted in most cases In the instance method or construction method, in order to distinguish between local variables and instance variables, this cannot be omitted */ public class Test09 { public static void main(String[] args){ System.out.println("---Nonparametric construction——————"); Students s = new Students(); s.setName("yxy"); s.setNo(1); System.out.println(s.getName()); System.out.println(s.getNo()); System.out.println("---Parametric construction——————"); Students s2 = new Students(2,"yx"); System.out.println(s2.getName()); System.out.println(s2.getNo()); } } class Students{ private int no; private String name; public Students() { } //this.no is an instance variable // no is a local variable public Students(int no, String name) { this.no = no; this.name = name; } public void setNo(int no) { this.no = no; } public void setName(String name) { this.name = name; } //getName actually gets the name of the current name public int getNo() { return no;//return this.name } public String getName() { return name; } }
package Staticandthis; /* 1.this Is a keyword, all lowercase 2.this What is it and how is it in terms of memory? this Is a variable and a reference. this saves the memory address of the current object and points to itself One object, one this So strictly speaking, this represents the "current object", which is stored in heap memory */ public class Test08 { public static void main(String[] args){ Customer c1 = new Customer("yxy"); c1.shopping(); //this stands for c1. c1 calls shopping. this is c1 Customer c2 = new Customer("x"); Student.m(); } } class Customer { String name; public Customer() { } public Customer(String s) { name = s; } //Customer shopping method example method public void shopping() { //c1 calls shopping, and this is c1 //c2 calls shopping, and this is c2 System.out.println(this.name + "Shopping");//The reference. Is this. this. Can be omitted } public static void doSome() { //this represents the current object, which is not required for static method calls // System.out.println(this); } } class Student{ //Instance variables must be accessed by referencing a new object String name = "z"; //So name cannot access public static void m(){ // System.out.println(name); //You can Student s = new Student(); System.out.println(s.name); //If the instance variable is directly accessed in the method, it must be an instance method } }
yxy Shopping z
-
New syntax: to call another constructor of this class through the current constructor, you can use the following syntax format:
This (actual parameter list);
Code reuse can be achieved by calling constructor 2 through constructor 1, but it should be noted that constructor 1 and constructor 2 are in the same class
The function of the new syntax is: code reuse
In the constructor: this() cannot be preceded by other statements, and can only appear once.
package Staticandthis; /* this` It can be used in addition to the instance method, but also in the construction method New syntax: call another constructor of this class through the current constructor. You can use the following syntax format: this (actual parameter list); */ public class Test10 { public static void main(String[] args) { Date d1 = new Date(); d1.setDay(10); Date d2 = new Date(2000, 01, 01); d1.detail(); d2.detail(); } } class Date{ private int year; private int month; private int day; public Date(){ //Error: the call to this must be the first statement in the constructor // System.out.println(11); /* this.year = 1970; this.month = 1; this.day = 1;*/ this(1970,1,1); } public Date(int year, int month, int day) { this.year = year; this.month = month; this.day = day; } //Provides a printing method public void detail(){ System.out.println(year + "," + month + "," + day); } public void setYear(int year) { this.year = year; } public void setMonth(int month) { this.month = month; } public void setDay(int day) { this.day = day; } public int getYear() { return year; } public int getMonth() { return month; } public int getDay() { return day; } }
summary

package Staticandthis; public class Test11 { public static void main(String[] args){ //Create account Account a = new Account("1000",2000,1.23); //Create customer object //A is to create a relationship between the Customer and the Account object //Assign the memory address of a directly to the variable act Customers c = new Customers("yx",a); c.getAct().deposit(100); c.getAct().withdraw(960); c.getAct().withdraw(2000); } } //Account class class Account{ private String id; private double balacen; private double annualInterestRate; public Account() { } public Account(String id, double balacen, double annualInterestRate) { this.id = id; this.balacen = balacen; this.annualInterestRate = annualInterestRate; } public void setId(String id) { this.id = id; } public void setBalacen(double balacen) { this.balacen = balacen; } public void setAnnualInterestRate(double annualInterestRate) { this.annualInterestRate = annualInterestRate; } public String getId() { return id; } public double getBalacen() { return balacen; } public double getAnnualInterestRate() { return annualInterestRate; } //Deposit method public void deposit(double money){ if(money > 0){ // balacen = money + balacen; // return balacen; // Call method to repair balance setBalacen(getBalacen() + money); System.out.println("Successfully deposited" + money); }else { System.out.println("Please enter the correct amount"); return; } } //Withdrawal method public void withdraw(double money){ if(money < getBalacen()){ //balacen = balacen -money; //return balacen; setBalacen(getBalacen() - money); System.out.println("Successfully removed" + money); }else { System.out.println("Sorry, your credit is running low"); return; } } } class Customers{ private String name; private Account act; public Customers() { } public Customers(String name, Account act) { this.name = name; this.act = act; } public String getName() { return name; } public Account getAct() { return act; } public void setName(String name) { this.name = name; } public void setAct(Account act) { this.act = act; } }
java three variables
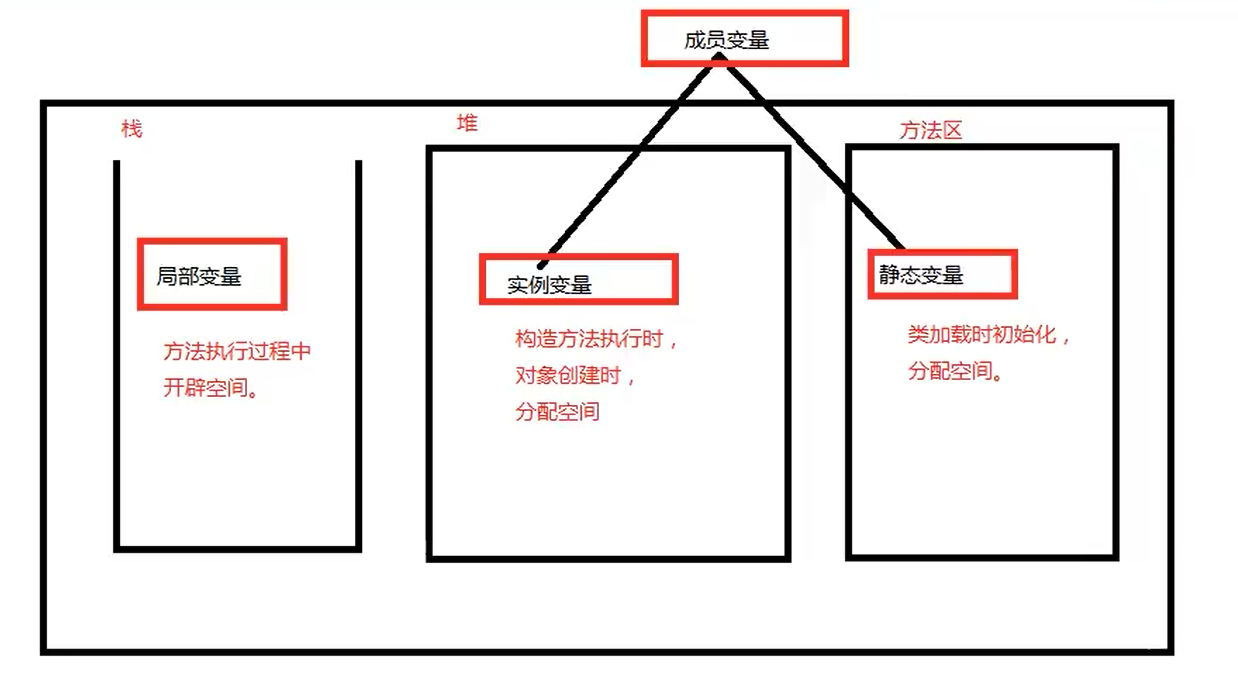
Summary:
How are all variables accessed
How are all methods accessed
What is in a class
No matter how the program changes, there is a fixed law
All instance related objects are created first and accessed through "reference"
All statically related are accessed directly by "class name"
Conclusion:
As long as the calling method a and the called method b are in the same class:
this.
Class name. Can be omitted
package Staticandthis; /* Class body{ Instance variables; Example method; Static variables; Static method; Construction method; Static code block; Instance statement block; Method (){ Local variables; } } */ public class Review { //Before the program is executed, all classes are loaded into the JVM //The main method will not execute until the loading is completed static{ System.out.println("Review Class loading"); } //Static method public static void main(String[] args){ //local variable int i = 100; //Complete all the actions stu s1 = new stu(); s1.study(); } } //Student class class stu{ private int no; private String name; static String job = "study"; { System.out.println("Instance statement block. This construction method is executed once, and it will be executed once here"); } public stu(int no, String name) { this.no = no; this.name = name; } public stu() { //Default student ID and name this(100,"yxy"); } //Two instance methods are provided //Calling other instance methods of this class in the instance method public void study(){ //System.out.println(this.getName() + "studying hard"); System.out.println(name + "I'm studying hard"); //this. Can be omitted //eat(); this.eat(); } public void eat(){ System.out.println(name + "Eat in a restaurant"); //How static methods use class names //In the same class, the class name. Can be omitted stu.m1(); } //Two static methods are provided public static void m1(){ System.out.println("M1 method"); m2(); } public static void m2(){ System.out.println("M2 method"); System.out.println("state" + job);//stu.job } public int getNo() { return no; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
package Staticandthis; /* No matter how the program changes, there is a fixed law All instance related objects are created first and accessed through "reference" All statically related are accessed directly by "class name" Conclusion: As long as the calling method a and the called method b are in the same class: this. Class name. Can be omitted */ public class Review02 { int i = 100; static int j = 1000; public void m1(){} public void m2(){} public void x(){ // This method is an instance method. During the execution of this method, the current object exists m1(); //this.m1(); m2(); m3(); m4(); System.out.println(i); System.out.println(j); //Accessing static methods of other classes T.t2(); //Access instance methods of other classes T t = new T(); t.t1(); } public static void m3(){} public static void m4(){} /* Step 1: main The method is static. When calling the main method, the JVM directly uses the "class name." method, so there is no this in the main method Step 2: m1() m2()They are all instance methods. According to java syntax, you should first access the new object in the way of "reference" */ public static void main(String[] args){ // System.out.println(i); System.out.println(j); // m1(); // m2(); m3(); m4(); //To access the m1 m2 new object Review02 r = new Review02(); r.m1(); r.m2(); System.out.println(r.i); //Local variables can be accessed directly int k = 10; System.out.println(k); T t = new T(); t.t1(); T.t2(); } } class T{ public void t1(){} public static void t2(){} }
inherit
Role of inheritance:
Basic function: the subclass inherits the parent class, and the code can be reused
Main function: because of inheritance, there is later method coverage and polymorphism
Inherited related properties:
① If class B inherits class A, class A is called superclass, parent class and base class, and class B is called subclass, derived class and extended class.
② Inheritance in java only supports single inheritance and does not support multiple inheritance. C + + supports multiple inheritance, which also reflects the simplicity of java. In other words, it is not allowed to write code like this in java:
class B extends A,C{ }.
③ Although multiple inheritance is not supported in java, sometimes it will have the effect of indirect inheritance,
For example, class C extends B and class B extends A. that is, C inherits B directly, but C also inherits a indirectly.
④ java stipulates that a subclass inherits from the parent class, and all the rest can be inherited except the constructor. However, private properties cannot be accessed directly in the subclass, which can be accessed indirectly
⑤ If a class in java does not inherit any classes, it inherits the Object class by default. The Object class is the root class (ancestral class) provided by the java language, that is, an Object is born with all the characteristics of the Object type.
⑥ Inheritance also has some disadvantages. For example, inheriting the Account class from the CreditAccount class will lead to a very high degree of coupling between them. The CreditAccount class will be affected immediately after the Account class is changed
Inherit from Object
Test extensions
1. After the subclass inherits the parent class, can the subclass object be used to call the parent class method?
In essence, after a subclass inherits from the parent class, it owns the methods inherited from the parent class. In fact, what is called is not the parent class's method, but the subclass's own method, which belongs to the subclass
package Extends; /* Test: the subclass inherits the parent class. Can the parent class object be called with the subclass object? In essence, after a subclass inherits from the parent class, it owns the methods inherited from the parent class. In fact, what is called is not the parent class's method, but the subclass's own method, which belongs to the subclass */ public class Test02 { public static void main(String[] args){ Cats c = new Cats(); c.move(); System.out.println(c.name); } } class Animal{ //The name is not encapsulated String name = "x";//Give a default value public void move(){ System.out.println(name + "Moving"); } } //Cats will inherit everything from Animal class Cats extends Animal{ }
2. What conditions can inheritance be used in actual development?
Anything that can be described by "is a" can be inherited
For example: cat is a animal
Suppose there is a Class A and a class B in the future development. If there are duplicate codes in class A and class B, can they inherit? Not necessarily. Let's see if they can be described with "is a"
class Customer{
String name; / / name
// setter and getter
}
class Product{
String name; / / name
// setter and getter
}
class Product extends Customer{
}
The above inheritance is a failure, because: Product is a Customer is against ethics.
3. Any class does not inherit from any class. It inherits from Object by default. What is in Object?
Why is java easy to learn?
Because Java has built-in a huge class library, programmers do not need to write code from 0. Programmers can carry out "secondary" development based on this huge class library. (the development speed is fast, because the built-in Library of JDK realizes many basic functions.)
For example, String is a String class written by SUN, and System is a System class written by SUN. These classes can be used directly.
Where is the JDK source code?
C:\Program Files\Java\jdk-13.0.2\lib\src.zip
Can you understand the following code now?
System.out.println("Hello World!");
In System.out, there are no parentheses after out, indicating that out is a variable name.
In addition, System is a class name. Directly use the class name System.out to indicate that out is a static variable.
System.out returns an object, and then accesses the println() method in the form of "object."
package Extends; //Blue is the keyword in idea //Black identifier //System.out.println("Hello word"); //In the above code, System, out and println are identifiers public class Test03 { static Student s = new Student(); public static void main(String[] args){ //entrance Test03.s.ex();//Classes. Static variables. Methods System.out.println("Hello word"); } } class Student{ public void ex(){ System.out.println("examination."); } }
One of the objects is called toString(). We tested it and found that:
System.out.println (Reference);
When directly outputting a "reference", the println() method will automatically call "reference. toString()" first, and then output the execution result of the toString () method.
package Extends; /* Inherit Object by default. What are the methods in Object? package java.lang; import jdk.internal.vm.annotation.IntrinsicCandidate; public class Object { @IntrinsicCandidate public Object() {} @IntrinsicCandidate public final native Class<?> getClass(); @IntrinsicCandidate public native int hashCode(); public boolean equals(java.lang.Object obj) { return (this == obj); } @IntrinsicCandidate protected native java.lang.Object clone() throws CloneNotSupportedException; public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); } @IntrinsicCandidate public final native void notify(); @IntrinsicCandidate public final native void notifyAll(); public final void wait() throws InterruptedException { wait(0L); } public final native void wait(long timeoutMillis) throws InterruptedException; public final void wait(long timeoutMillis, int nanos) throws InterruptedException { if (timeoutMillis < 0) { throw new IllegalArgumentException("timeoutMillis value is negative"); } if (nanos < 0 || nanos > 999999) { throw new IllegalArgumentException( "nanosecond timeout value out of range"); } if (nanos > 0 && timeoutMillis < Long.MAX_VALUE) { timeoutMillis++; } wait(timeoutMillis); } @Deprecated(since="9") protected void finalize() throws Throwable { } } */ public class Test05 { public static void main(String[] args){ Test05 t = new Test05(); String r = t.toString(); System.out.println(r); //Extends. Test05@1b6d3586 1b6d3586 can be regarded as the memory address of the object in the heap memory. In fact, it is the result of the memory address obtained by the hash algorithm product p = new product(); String r2 = p.toString(); System.out.println(r2); System.out.println(p.toString()); //What if you output references directly System.out.println(p);//The toString method is called by default } } class product{ /* public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); } */ }
Extends.Test05@1b6d3586 Extends.product@4554617c Extends.product@4554617c Extends.product@4554617c
Method coverage and polymorphism mechanism
Method coverage
When to use method overrides?
After the subclass inherits from the parent class, when the inherited method cannot meet the business requirements of the current subclass, the subclass has the right to rewrite the method. It is necessary to Override the method. Method Override is also called method Override override
Conditions covered by the method
Condition 1: two classes must have inheritance relationship
The two roles of inheritance: basic role and important role
Basic function: code reuse
Important role: Method coverage and polymorphism mechanism
Condition 2: the rewritten method and the inherited method have
The same return value type,
Same method name,
Same formal parameter list
Condition 3: the access permission cannot be lower, but higher
Condition 4: the rewritten method cannot throw more or less than the previous method
package Override; /* When will method override be considered: after the subclass inherits the parent class, when the inherited method cannot meet the business requirements of the current subclass, the subclass has the right to rewrite the method, and it is necessary to override the method, Method Override is also called method Override Important conclusions: When the subclass overrides the method inherited from the parent class, the subclass object must call the overridden method Review the following methods: When does the opening rate use method overload? overload If the functions of a class are similar, it is recommended to define the same name, so that the code is beautiful and convenient for programming What conditions satisfy method overloading? Condition 1: in the same class Condition 2: same method name Condition 3: different parameter lists (number, order and type) How do we write code that constitutes method coverage at the code level? Condition 1: two classes must have inheritance relationship The two roles of inheritance: basic role and important role Basic function: code reuse Important role: Method coverage and polymorphism mechanism Condition 2: the rewritten method and the inherited method have The same return value type, Same method name, Same formal parameter list Condition 3: the access permission cannot be lower, but higher Condition 4: the rewritten method cannot throw more or less than the previous method */ public class Test02 { public static void main(String[] args){ Brid b =new Brid(); b.move(); b.sing(1); Cat c = new Cat(); c.move(); } } class Animal{ public void move(){ System.out.println("Animals are moving!"); } //The parent class threw an exception /*public void move() throws Error{ System.out.println("Animals are moving! "); }*/ //Lower permissions can be accessed by public /*protected void move(){ System.out.println("Animals are moving! "); }*/ public void sing(int i){ System.out.println("Animal singing"); } } class Brid extends Animal{ //Override, override and override the move method //It is best to copy the methods in the parent class intact (manual writing is not recommended) //Method override is to override the inherited method public void move(){ System.out.println("The bird is flying!"); } //A child class cannot throw more exceptions than a parent class /*public void move() { System.out.println("Animals are moving! "); }*/ //Protected means protected and not open to public //Error: access permission is too low, /* protected void move(){ System.out.println("Birds are flying "); }*/ //Not enough method coverage //However, it can constitute a method overload public void sing(){ System.out.println("Bird singing"); } } class Cat extends Animal{ public void move(){ System.out.println("The cat is walking"); } }
matters needing attention
- Note 1: method overrides are only for methods and have nothing to do with attributes
- Note 2: private methods cannot be overridden
- Note 3: constructors cannot be inherited, so constructors cannot be overridden
- Note 4: Method coverage can only be used for governance methods. Static methods have no coverage significance
Classic case
package Override; //A method covers classic cases public class Test03 { public static void main(String[] args){ //The first method /*Chinese c = new Chinese("Chinese "); c.speak(); American a = new American("American "); a.speak();*/ //The second method Chinese c = new Chinese(); c.setName("Chinese"); c.speak(); American a = new American(); a.setName("American"); a.speak(); } } class People{ private String name; public People() { } public People(String name) { this.name = name; } public String getName() { return name; } public void setName(String name) { this.name = name; } public void speak(){ System.out.println(name + "Talking"); } } class Chinese extends People{ public Chinese(String name) { super(name); } public Chinese() { } public void speak(){ //name is a private property. name can only be called by calling grtName() System.out.println(this.getName() + "Speak Chinese"); } } class American extends People{ public American(String name) { super(name); } public American() { } public void speak(){ //name is a private property. name can only be called by calling grtName() System.out.println(this.getName() + "Speak English"); } }
package Override; /* About toString() method in Object 1.toString()What is the function of the method? Convert java objects to string form 2.Object What is the default implementation of the toSting() method in the class? public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); } toSrting: It means to convert to String Meaning: calling the toString method of a Java object can convert the Java object into string form. 3.Is the default implementation given by the toString() method sufficient? */ public class Test04 { public static void main(String[] args){ MyDate t1 = new MyDate(); //Convert object to string form //MyDate@776ec8df Is the result before overriding the toString method //Desired output: mm / DD / yyyy //Output after rewriting on January 1, 1970 System.out.println(t1.toString()); //When outputting a reference, the println method will automatically call the referenced toString method System.out.println(t1); MyDate t2 =new MyDate(2000,8,7); System.out.println(t2.toString()); System.out.println(t2); } } class MyDate{ private int year; private int month; private int day; public MyDate() { //default time this(1970,1,1); } public MyDate(int year, int month, int day) { this.year = year; this.month = month; this.day = day; } public int getYear() { return year; } public int getMonth() { return month; } public int getDay() { return day; } public void setYear(int year) { this.year = year; } public void setMonth(int month) { this.month = month; } public void setDay(int day) { this.day = day; } public String toString() { return year + "year" + month + "month" + day + "day"; } }
What is the difference between method overloading and method overriding?
Method overloading occurs in the same class.
Method overrides occur between parent and child classes with inheritance relationships.
Method overloading is a class with the same method name and different parameter lists.
Method overrides are parent-child classes with inheritance relationship, and the overridden method must be consistent with the previous method:
The method name, parameter list and return value type are consistent.
polymorphic
Concepts of upward and downward transformation
First: upward transformation
Child -- > parent (automatic type conversion)
Second: downward transformation
Parent -- > child (casts, casts are required)
be careful:
Java allows both upward and downward transformation
Whether it is upward transformation or downward transformation
There must be an inheritance relationship between the two types. If there is no inheritance relationship, the compiler will report an error
What is polymorphism?
Multiple states
Java programs are divided into compilation phase and running phase
Let's analyze the compilation phase first:
For the compiler, the compiler only knows that the type of a2 is Animal
Therefore, when compiling, the compiler will go to the Animal.class bytecode file to find the move method. After finding the move method on the binding, the compilation is successful and the static binding is successful
Analysis operation phase:
In the run-time stage, the java objects in the heap memory are actually Cat objects, so when moving, the objects that really participate in the move are Cat. Therefore, the run-time stage will dynamically execute the move() method of Cat objects. This process belongs to the binding of the run-time stage and dynamic binding
Polymorphisms represent multiple forms:
A form when compiling
Running is a form.
Risk of downward transformation
ClassCastException error will be generated
How to avoid: generation of ClassCastException
New content, operator: instanceof
First: instanceof can dynamically determine the type of object pointed to by the reference at run time
Second: the syntax of referencing instanceof:
(reference instanceof type)
Third: the operation result of instanceof operator can only be: true/fasle
Fourth: c is a reference. The c variable holds the memory address and points to the object in the heap
Suppose (c instanceof Cat) is True; Indicates that the java object in c memory is a Cat
Suppose (c instanceof Cat) is false; Indicates that the java object in c memory is not a Cat
instanceof dynamically judges in the running phase.
instanceof operator must be used whenever and wherever the type is transformed downward to avoid ClassCastException
package duotai; /* Polymorphic basic grammar 1.Before learning polymorphic basic grammar, we need to popularize two concepts: First: upward transformation Child -- > parent Second: downward transformation Parent -- > child be careful: Java Allow upward transformation and downward transformation Whether it is upward transformation or downward transformation There must be an inheritance relationship between the two types. If there is no inheritance relationship, the compiler will report an error 2.Polymorphism refers to: The parent type refers to an object that points to a subclass Including compilation phase and run phase Compile time: method of binding parent type Run time: methods for dynamically binding subtypes 3.When to use downward transformation? Do not cast casually Methods unique to subclass objects, using downward transformation */ public class Test01 { public static void main(String[] args){ Animal a1 = new Animal(); a1.move(); Cat c1 = new Cat(); c1.move(); Bird b1 = new Bird(); b1.move(); /* 1.Animal There is an inheritance relationship between and Ca 2.Animal Is the parent class and Animal is the child class 3.Cat is a Animal A reference to a parent type allows pointing to objects of a child type. Animal a2 = new Cat(); a2 Is a reference to the parent type new Cat()Is a subtype object Allow a2 this parent type to refer to an object of a child type. */ Animal a2 = new Cat(); Animal a3 = new Bird(); /* What is polymorphism? Multiple states Java The program is divided into compilation stage and running stage Let's analyze the compilation phase first: For the compiler, the compiler only knows that the type of a2 is Animal Therefore, when compiling, the compiler will look for the move method in the Animal.class bytecode file and find the move method on the binding Method, compilation succeeded, static binding succeeded Analysis operation phase: During the run phase, the java objects in the heap memory are actually Cat objects, so when moving, the objects that really participate in moving are Cat objects Therefore, the move() method of the Cat object will be dynamically executed in the runtime. This process belongs to the binding in the runtime and dynamic binding Polymorphisms represent multiple forms: A form when compiling Running is a form. */ a2.move(); a3.move(); //==================================================================== Animal a5 = new Cat(); //The analyzer must analyze the dynamic binding between the compile phase and the run phase //If CatchMouse is called, forced downward transformation must be performed //Because a5 is an Animal type and is converted to Cat, there is an inheritance relationship between Animal and Cat, so there is no error Cat x = (Cat)a5; x.CatchMouse(); //Is there a risk of downward transformation Animal a6 = new Bird();//On the surface a6, it's an Animal. When running, it's actually Brid //In the run phase, the heap memory actually creates a Bird object. In the actual run, the Bird object is converted into a Cat object, //There is no inheritance relationship between bird and Cat objects /* Cat y = (Cat)a6; y.CatchMouse();*/ //How to avoid: generation of ClassCastException /* New content, operator: instanceof First: instanceof can dynamically determine the type of object pointed to by the reference at run time Second: the syntax of referencing instanceof: (Reference instanceof type) Third: the operation result of instanceof operator can only be: true/fasle Fourth: c is a reference. The c variable holds the memory address and points to the object in the heap Suppose (c instanceof Cat) is True; Indicates that the java object in c memory is a Cat Suppose (c instanceof Cat) is false; Indicates that the java object in c memory is not a Cat instanceof Dynamic judgment in operation phase. instanceof operator must be used whenever and wherever the type is transformed downward to avoid ClassCastException */ System.out.println(a6 instanceof Cat); if(a6 instanceof Cat){//Cast if a6 Cat Cat y = (Cat)a6; y.CatchMouse(); } } } class Animal{ public void move(){ System.out.println("moving"); } } class Cat extends Animal{ @Override public void move() { System.out.println("Cat is Moving"); } //In addition to move, you should have your own unique behavior public void CatchMouse(){ System.out.println("catching"); } } class Bird extends Animal{ @Override public void move() { System.out.println("Bird is flying"); } public void Sing(){ System.out.println("bird is singing"); } }
package duotai; /* Programmers can observe the bottom layer, Bird and Cat The reason for instanceof judgment is that programmers may not see it in future development */ public class Test02 { public static void main(String[] args){ Animal x = new Bird(); Animal y = new Cat(); if(x instanceof Bird){ Bird a = (Bird)x; a.Sing(); }else if(x instanceof Cat){ Cat a = (Cat)x; a.CatchMouse(); } if(y instanceof Cat){ Cat b = (Cat)y; b.CatchMouse(); }else if(y instanceof Bird){ Bird b = (Bird)y; b.Sing(); } } }
package duotai; public class Test03 { public static void main(String[] args){ //main programmer A is responsible for writing Atest a = new Atest(); a.test(new Cat()); a.test(new Bird()); } } class Atest{ //Programmer B is responsible for writing //The parameter of the test() method is an Animal public void test(Animal a){ //The method you write will be called by others //When others call, they may pass a Bird to your test() method //Of course, it can be recognized //For me, I don't know what parameters will be passed to me when calling if(a instanceof Cat){ Cat c = (Cat) a; c.CatchMouse(); }else if(a instanceof Bird){ Bird b = (Bird) a; b.Sing(); } } }
The role of polymorphism in practical application
Reduce the coupling degree of the program and improve the expansion force of the program
public class Master{ public void feed(Dog d){} public void feed(Cat c){} } The above code indicates: Master and Dog as well as Cat The relationship is very close (high coupling). Resulting in poor expansion force. public class Master{ public void feed(Pet pet){ pet.eat(); } } The above representatives said: Master and Dog as well as Cat The relationship between the two countries broke away, Master The concern is Pet Class. such Master and Dog as well as Cat The degree of coupling is reduced and the scalability of the software is improved.
Three characteristics of object-oriented:
Encapsulation, inheritance, polymorphism
With encapsulation, with the concept of the whole.
Inheritance occurs between objects.
After inheritance, there is method coverage and polymorphism.
A software development principle is mentioned here:
Seven basic principles: OCP (open to expansion and closed to modification)
The purpose is to reduce program coupling and improve program expansion.
Abstract oriented programming is not recommended.
package duotai; public class Test04 { public static void main(String[] args){ Master m = new Master(); Dog d = new Dog(); Cats c = new Cats(); YingWu y = new YingWu(); m.feed(d); m.feed(c); m.feed(y); } } class Master{ public void feed(Pet p){ //When compiling, the compiler finds that p is a pet class and looks for the eat() method in the pet class. As a result, it finds it and the compiler passes //When it is found that the underlying layer is actually a Dog object, it will automatically call the eat method corresponding to the Dog object. p.eat(); } } class Pet{ //This method can not be implemented public void eat(){ } } class Dog extends Pet{ public void eat(){ System.out.println("dog eat"); } } class Cats extends Pet{ public void eat(){ System.out.println("Cat eat"); } } class YingWu extends Pet{ public void eat(){ System.out.println("YingWu eat"); } }
Explain the remaining problems
Private methods cannot be overridden.
Method coverage is only for instance methods, and static method coverage is meaningless. (this is because method coverage is usually combined with polymorphism)
Do static methods have method overrides?
Polymorphism is naturally associated with objects, and objects are not required for the execution of static methods,
Therefore, in general, we will say that there is no method coverage for static methods
Sum up two sentences:
Private cannot be overwritten.
Static does not talk about coverage.
In method overrides, the return value type of the method.
What conditions will constitute the coverage of the method when they are met?
1. Between two classes with inheritance relationship.
2. Method in parent class and method after subclass override:
Have the same method name, the same formal parameter list, and the same return value type.
After learning the polymorphism mechanism:
Can the "same return value type" be modified?
For the return value type to be the basic data type, it must be consistent.
For the return value type is a reference data type, the return value type can become smaller after rewriting (but it doesn't make much sense. No one writes this in actual development).
super keyword
super is a keyword, all lowercase
Comparative study of super and this
this:
this can appear in instance methods and construction methods
The syntax of this is: "this.", "this()"
this cannot be used in static methods
this. It can be omitted in most cases, and cannot be omitted when distinguishing instance variables and local variables
this() can only appear in the first line of the constructor. Call other methods in this class through the current constructor for code reuse
super:
super can appear in instance methods and construction methods
Super syntax is: "super.", "super()"
super cannot be used in static methods
super. It can be omitted in most cases,
super() can only appear in the first line of the constructor. Call other methods in the "parent class" through the current constructor. The purpose is to initialize the parent type feature when creating a subclass object
super()
Indicates that the constructor of the parent class is called through the constructor of the child class
Model the scenario in the real world: to have a son, you need an existing father
super() can only appear in the first line of the constructor. The constructor in the "parent class" is called through the current constructor. The purpose is to initialize the parent type feature when creating a subclass object.
Important conclusions
When the first line of a construction method:
If there is neither this() nor super(), there will be a super() by default;
Indicates that the parameterless constructor of the parent class is called through the constructor of the current subclass
Therefore, we must ensure that the parameterless construction method of the parent class exists
this() and super() cannot coexist. They can only exist in the first line of the constructor
No matter how tossed, the parameterless constructor of the parent class will be executed
package Super; /* 1. super Is a keyword, all lowercase 2. super Contrast with this this: this Can appear in instance methods and construction methods this The syntax is: "this.", "this()" this Cannot be used in static methods this. It can be omitted in most cases, and cannot be omitted when distinguishing instance variables from local variables this()It can only appear in the first line of the construction method. Other methods in this class can be called through the current construction method for the purpose of code reuse super: super Can appear in instance methods and construction methods super The syntax is: "super.", "super()" super Cannot be used in static methods super. In most cases, it can be omitted, super()It can only appear in the first line of the construction method. Call other methods in the "parent class" through the current construction method. The purpose is to initialize the parent type feature when creating a subclass object 3. super() Indicates that the constructor of the parent class is called through the constructor of the child class Model the scenario in the real world: to have a son, you need an existing father 4. Important conclusions When the first line of a construction method: If there is neither this() nor super(), there will be a super() by default; Indicates that the parameterless constructor of the parent class is called through the constructor of the current subclass Therefore, we must ensure that the parameterless construction method of the parent class exists 5. this()And super() cannot coexist. They can only exist in the first line of the constructor 6. No matter how tossed, the parameterless constructor of the parent class will be executed */ public class Test01 { public static void main(String[] args){ new B(); /* A Implementation of parameterless construction method of class B Implementation of parameterless construction method of class */ } } class A{ int i; public A(int i) { System.out.println("A Implementation of parameterized construction method of class"); } public A() { System.out.println("A Implementation of parameterless construction method of class"); } /* If a class does not provide any construction method manually, the system will provide a parameterless construction method by default */ } class B extends A{ public B() { //Call the constructor with parameters in the parent class //super();// Automatic /* A Implementation of parameterless construction method of class B Implementation of parameterless construction method of class */ this("123"); /* A Implementation of parameterless construction method of class B Implementation of parameterized construction method of class B Implementation of parameterless construction method of class */ System.out.println("B Implementation of parameterless construction method of class"); } public B(String a){ //super(); By default, some are called first /* A Implementation of parameterless construction method of class B Implementation of parameterized construction method of class B Implementation of parameterless construction method of class */ System.out.println("B Implementation of parameterized construction method of class"); } }
Execution order of super and this
package Super; public class Test02 { public static void main(String[] args){ new E(); } } class C{ public C() { System.out.println("C implement"); } } class D extends C{ public D() { System.out.println("D implement"); } public D(String name){ System.out.println("D name"); } } class E extends D { public E() { this("name"); System.out.println("E implement"); } public E(String name) { this(name,20); System.out.println("E name implement"); } public E(String name,int i) { super(name); System.out.println("E name i implement"); } } // C implement D name E name i implement E name implement E implement
Usage time of super (instance parameter)
package Super; /* Use super at the right time: */ public class Test03 { public static void main(String[] args){ CreditAccount ca1 = new CreditAccount(); System.out.println(ca1.getActno() + "," + ca1.getBalance() + "," + ca1.getCredit()); CreditAccount ca2 = new CreditAccount("a",123,111); System.out.println(ca2.getActno() + "," + ca2.getBalance() + "," + ca2.getCredit()); } } class Account{ /* Private methods can only be accessed in this class */ private String actno; //account number private double balance; //balance public Account() { } public Account(String actno, double balance) { this.actno = actno; this.balance = balance; } public String getActno() { return actno; } public double getBalance() { return balance; } public void setActno(String actno) { this.actno = actno; } public void setBalance(double balance) { this.balance = balance; } } //Other types of accounts: credit card accounts class CreditAccount extends Account{ private double credit; public CreditAccount(String actno, double balance, double credit) { /* this.actno = actno; this.balance = balance; The above two lines of code are in the right place and can be used: super(actno) Call the constructor of the parent class through the constructor of the child class */ super(actno,balance); this.credit = credit; } public CreditAccount() { } public void setCredit(double credit) { this.credit = credit; } public double getCredit() { return credit; } }
null,0.0,0.0 a,123.0,111.0
Memory map of super execution
Note: Although the constructor is called, a series of parent constructor are also called during the execution of the constructor, and the parent constructor continues to call its parent constructor, in fact, only one object is created
What exactly is "super"?
Super (argument): initializing the parent type feature of the current object does not create a new object. In fact, only one object can be created
What does the super keyword represent?
The super keyword represents the part of the parent type characteristics of the "current object"
example
Subtype and parent type have no property with the same name
package Super; public class Test04 { public static void main(String[] args){ Vip v = new Vip("z"); v.Shopping(); } } class Cumstor{ String name; public Cumstor() { } public Cumstor(String name) { this.name = name; } public void Shopping(){ } } class Vip extends Cumstor{ public Vip() { } public Vip(String name) { super(name); } public void Shopping(){ System.out.println(this.name + "shopping"); System.out.println(super.name + "shopping"); System.out.println(name + "shopping"); } } zshopping zshopping zshopping
Subtype and parent type have properties with the same name
package Super; /* this.And super. Can be omitted in most cases this.It cannot be omitted when distinguishing local variables from instance variables super.This attribute is in the parent class and also in the child class. If you want to access the attribute in the parent type in the child type, you can't omit it */ public class Test05 { public static void main(String[] args){ Vip v = new Vip("z"); v.Shopping(); } } class Cumstor{ String name; public Cumstor() { } public Cumstor(String name) { this.name = name; } public void Shopping(){ } } class Vip extends Cumstor{ //Suppose a subclass also has an attribute with the same name String name; public Vip() { } public Vip(String name) { super(name); } //Neither super nor this can appear in static methods public void Shopping(){ //this represents the current space System.out.println(this.name + "shopping"); //Super represents the parent type characteristics of the current object (super is a space in the object pointed to by this) System.out.println(super.name + "shopping"); System.out.println(name + "shopping"); } } // nullshopping zshopping nullshopping
Neither super nor this can appear in static methods
How does java distinguish between molecular class attributes and parent type attributes
this. And super. Can be omitted in most cases
this. Cannot be omitted when distinguishing between local variables and instance variables
super. This attribute exists in the parent class and in the child class. If you want to access the attribute in the parent type in the child type, you can't omit it
super cannot be used alone
Super is not a reference, super does not save memory addresses, and super does not point to any objects
super only represents the parent type characteristics inside the current object
package Super; /* Conclusions from the test. Super is not a reference, super does not save memory addresses, and super does not point to any objects super It only represents the parent type characteristics inside the current object */ public class Test06 { public void doSome(){ //If the reference is output, the referenced toSting method will be called automatically System.out.println(this); //System.out.println(this.toString()); //Error expecting '.' System.out.println(super.); } public static void main(String[] args){ Test06 t = new Test06(); t.doSome(); } }
package Super; public class Test07 { public static void main(String[] args){ Cat c = new Cat(); c.M(); } } class Animal{ public void move(){ System.out.println("Animal move"); } } class Cat extends Animal{ public void move(){ System.out.println("Cat move"); } public void M(){ this.move(); move(); super.move(); } }
- super. Property - access the properties of the parent class
- super. Method name (argument) - the method that accesses the parent class
- super. (argument) - access the constructor of the parent class
Memory map of super execution
Note: Although the constructor is called, a series of parent constructor are also called during the execution of the constructor, and the parent constructor continues to call its parent constructor, in fact, only one object is created
[external chain picture transferring... (img-L7U7fMuh-1634040754692)]
What exactly is "super"?
Super (argument): initializing the parent type feature of the current object does not create a new object. In fact, only one object can be created
What does the super keyword represent?
The super keyword represents the part of the parent type characteristics of the "current object"
example
Subtype and parent type have no property with the same name
package Super; public class Test04 { public static void main(String[] args){ Vip v = new Vip("z"); v.Shopping(); } } class Cumstor{ String name; public Cumstor() { } public Cumstor(String name) { this.name = name; } public void Shopping(){ } } class Vip extends Cumstor{ public Vip() { } public Vip(String name) { super(name); } public void Shopping(){ System.out.println(this.name + "shopping"); System.out.println(super.name + "shopping"); System.out.println(name + "shopping"); } } zshopping zshopping zshopping
[external chain picture transferring... (img-0aZuJNxt-1634040754692)]
Subtype and parent type have properties with the same name
package Super; /* this.And super. Can be omitted in most cases this.It cannot be omitted when distinguishing local variables from instance variables super.This attribute is in the parent class and also in the child class. If you want to access the attribute in the parent type in the child type, you can't omit it */ public class Test05 { public static void main(String[] args){ Vip v = new Vip("z"); v.Shopping(); } } class Cumstor{ String name; public Cumstor() { } public Cumstor(String name) { this.name = name; } public void Shopping(){ } } class Vip extends Cumstor{ //Suppose a subclass also has an attribute with the same name String name; public Vip() { } public Vip(String name) { super(name); } //Neither super nor this can appear in static methods public void Shopping(){ //this represents the current space System.out.println(this.name + "shopping"); //Super represents the parent type characteristics of the current object (super is a space in the object pointed to by this) System.out.println(super.name + "shopping"); System.out.println(name + "shopping"); } } // nullshopping zshopping nullshopping
[external chain pictures are being transferred... (IMG lhaj8n99-163400754693)]
Neither super nor this can appear in static methods
How does java distinguish between molecular class attributes and parent type attributes
this. And super. Can be omitted in most cases
this. Cannot be omitted when distinguishing between local variables and instance variables
super. This attribute exists in the parent class and in the child class. If you want to access the attribute in the parent type in the child type, you can't omit it
super cannot be used alone
Super is not a reference, super does not save memory addresses, and super does not point to any objects
super only represents the parent type characteristics inside the current object
package Super; /* Conclusions from the test. Super is not a reference, super does not save memory addresses, and super does not point to any objects super It only represents the parent type characteristics inside the current object */ public class Test06 { public void doSome(){ //If the reference is output, the referenced toSting method will be called automatically System.out.println(this); //System.out.println(this.toString()); //Error expecting '.' System.out.println(super.); } public static void main(String[] args){ Test06 t = new Test06(); t.doSome(); } }
package Super; public class Test07 { public static void main(String[] args){ Cat c = new Cat(); c.M(); } } class Animal{ public void move(){ System.out.println("Animal move"); } } class Cat extends Animal{ public void move(){ System.out.println("Cat move"); } public void M(){ this.move(); move(); super.move(); } }
- super. Property - access the properties of the parent class
- super. Method name (argument) - the method that accesses the parent class
- super. (argument) - access the constructor of the parent class