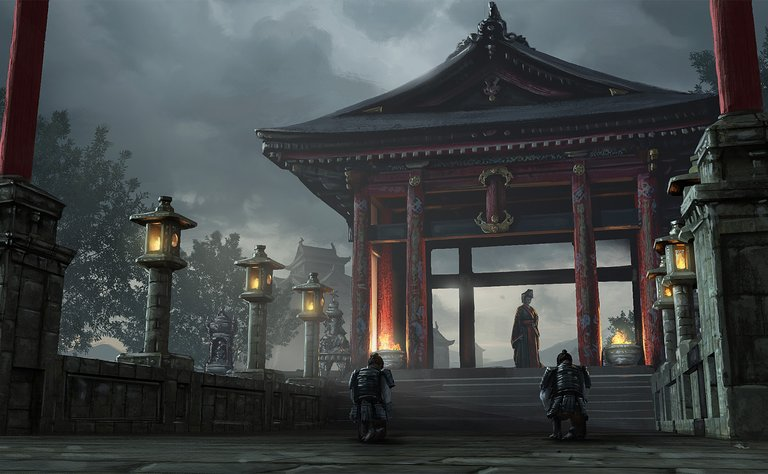
php and ajax technology
With the arrival of web2.0, Ajax has gradually become the mainstream, what is ajax, the development mode of ajax, the advantages, and the use of technology.(ajax overview, technology used by ajax, issues to be noted, application of Ajax technology in PHP)
What is ajax, the development model of ajax, advantages.
ajax, created by jesse james garrett, is asynchronous javascript and xml, asynchronous javascript and xml technology. ajax is not a new language or technology. It is a combination of javascript, xml, css, dom and many other technologies, which enables client-side asynchronous request operations and can communicate with the server without refreshing the page, thus reducing the waiting time of users.
ajax development mode:
Browser (client) http transport, http request, web server data store, back-end processing, inheritance system, server-side.
Client (browser) JavaScript calls, ajax engine http requests, http transport, web and xml servers, data storage, back-end processing, inheritance system (server).
Advantages: Reduce the burden on the server by transferring part of the work borne by the server to the client without refreshing the update page, calling external data such as xml, based on standardized and widely supported technologies.
JavaScript is an interpretive programming language that adds dynamic script code to a web page.
xmlhttprequest
ie browser put xmlhttp
var http_request = new ActiveXObject("Msxml2.XMLHTTP");
var http_request = new ActiveXObject("Microsoft.XMLHTTP");
Other browsers such as mozailla,safari
var http_request = new XMLHttpRequest();
if(window.XMLHttpRequest){ http_request = new XMLHttpRequest(); }else if(window.ActiveXObject){ try{ http_request = new ActiveXObject("Msxml2.XMLHTTP"); }catch(e){ try{ http_request = new ActiveXObject("Msxml2.XMLHTTP"); }catch(e){ try{ http_request = new ActiveXObject("Microsoft.XMLHTTP"); }catch(e){} } }
Common methods for XMLHttpRequest objects:
The open() method sets the url for the asynchronous request target
open("method", "url" [,asyncFlag [,"userName" [, "password"]]])
The send() method is used to send requests to the server
send(content)
setRequestHeader() method
The setRequestHeader() method sets a value for the http header of the request
setRequestHeader("label","value")
label specifies http header, value specifies HTTP header setting value
The setRequestHeader() method cannot be used until the open() method has passed
abort() method
The abort() method is used to stop the current asynchronous request
getAllResponseHeaders() method
The getAllResponseHeaders() method is used to complete http header information as a string.
Common properties of xmlhttpRequest object
The onreadystatechange triggers this event handler for each state change, typically invoking a JavaScript function.
Status of readyState request:
0 is uninitialized 1 is downloading 2 is loaded 3 In Interaction 4 For Completion
Response from the responseText server, representing a string
Response from the responseXML server, representing xml
Status returns the server's http status code
statusText returns the text corresponding to the http status code
The xml language is an extensible markup language that provides a format for describing structured data.The data that the xmlHttpRequest object exchanges with the server, usually in xml format.
dom is a document object model and defines a set of interfaces for parsing xml documents.
Use AJAX technology to detect user names in PHP
<script language="javascript"> var http_request = false; function createRequest(url) { //Initialize the object and issue an XMLHttpRequest request http_request = false; if (window.XMLHttpRequest) { //Other browsers such as Mozilla http_request = new XMLHttpRequest(); if (http_request.overrideMimeType) { http_request.overrideMimeType("text/xml"); } } else if (window.ActiveXObject) { //IE Browser try { http_request = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { http_request = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) {} } } if (!http_request) { alert("Cannot create XMLHTTP Example!"); return false; } http_request.onreadystatechange = alertContents; //Specify response method http_request.open("GET", url, true); //Make an HTTP request http_request.send(null); } function alertContents() { //Processing information returned by the server if (http_request.readyState == 4) { if (http_request.status == 200) { alert(http_request.responseText); } else { alert('The page you requested found an error'); } } } </script> <script language="javascript"> function checkName() { var username = form1.username.value; if(username=="") { window.alert("Please fill in your user name!"); form1.username.focus(); return false; } else { createRequest('checkname.php?username='+username+'&nocache='+new Date().getTime()); } } </script>
<?php header('Content-type: text/html;charset=GB2312'); //Specify encoding format for sending data as GB2312 $link=mysql_connect("localhost","root","root"); mysql_select_db("db_database",$link); $GB2312string=iconv( 'UTF-8', 'gb2312//IGNORE', $RequestAjaxString); //Ajax uses encodeURIComponent to encode the Chinese language to be submitted first mysql_query("set names gb2312"); $username=$_GET[username]; $sql=mysql_query("select * from tb_user where name='".$username."'"); $info=mysql_fetch_array($sql); if ($info){ echo "Sorry!User name[".$username."]Already registered!"; }else{ echo "Congratulations!User name[".$username."]Not registered!"; } ?>
Category Add
<script language="javascript"> var http_request = false; function createRequest(url) { //Initialize the object and issue an XMLHttpRequest request http_request = false; if (window.XMLHttpRequest) { //Other browsers such as Mozilla http_request = new XMLHttpRequest(); if (http_request.overrideMimeType) { http_request.overrideMimeType("text/xml"); } } else if (window.ActiveXObject) { //IE Browser try { http_request = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { http_request = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) {} } } if (!http_request) { alert("Cannot create XMLHTTP Example!"); return false; } http_request.onreadystatechange = alertContents; //Specify response method http_request.open("GET", url, true); //Make an HTTP request http_request.send(null); } function alertContents() { //Processing information returned by the server if (http_request.readyState == 4) { if (http_request.status == 200) { sort_id.innerHTML=http_request.responseText; //Set element content for sort_id HTML text substitution } else { alert('The page you requested found an error'); } } } </script> <script language="javascript"> function checksort() { var txt_sort = form1.txt_sort.value; if(txt_sort=="") { window.alert("Please fill in the article category!"); form1.txt_sort.focus(); return false; } else { createRequest('checksort.php?txt_sort='+txt_sort); } } </script>
<?php $link=mysql_connect("localhost","root","root"); mysql_select_db("db_database",$link); $GB2312string=iconv( 'UTF-8', 'gb2312//IGNORE', $RequestAjaxString); //Ajax uses encodeURIComponent to encode the submitted Chinese first mysql_query("set names gb2312"); $sort=$_GET[txt_sort]; mysql_query("insert into tb_sort(sort) values('$sort')"); header('Content-type: text/html;charset=GB2312'); //Specify encoding format for sending data as GB2312 ?> <table border="0" cellpadding="0" cellspacing="0"> <tr> <td> <select name="select" > <?php $link=mysql_connect("localhost","root","root"); mysql_select_db("db_database23",$link); $GB2312string=iconv( 'UTF-8', 'gb2312//IGNORE', $RequestAjaxString); //Ajax uses encodeURIComponent to encode the Chinese language to be submitted first mysql_query("set names gb2312"); $sql=mysql_query("select distinct * from tb_sort group by sort"); $result=mysql_fetch_object($sql); do{ header('Content-type: text/html;charset=GB2312'); //Specify encoding format for sending data as GB2312 ?> <option value="<?php echo $result->sort;?>" selected><?php echo $result->sort;?></option> <?php }while($result=mysql_fetch_object($sql)); ?> </select>
xml basic technology
Learn about xml and how to parse documents using simpleXML
Ways to traverse, modify, save, and create xml documents
xml syntax
xml document structure, xml declaration, processing instructions, annotations, xml elements, xml attributes, using cdata tags, xml command space.
XML Document Structure
<?xml version="1.0" encoding="gb2312" standalone="yes"?>
<?xml-stylesheet type="text/css" href="111.css"?>
XML declaration
<?xml version="1.0" encoding="gb2312" standalone="yes"?>
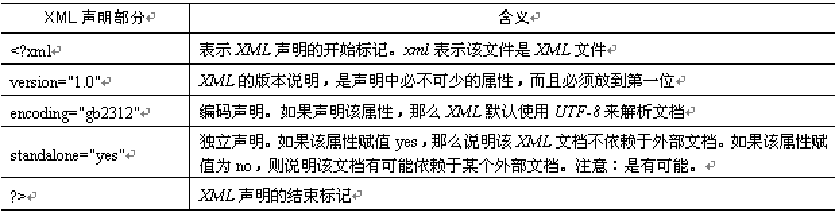
Processing instructions
<?xml-stylesheet type = "text/css" href="111.css"?>
<? Processing Instruction Name Processing Execution Information?>
xml-stylesheet: Style form processing instructions
type="text/css": set the style used by the document to be CSS
href="111.css": The address of the style file is set

XML attributes
<Label Property Name="Property Value" Property Name="...>Content</Label>
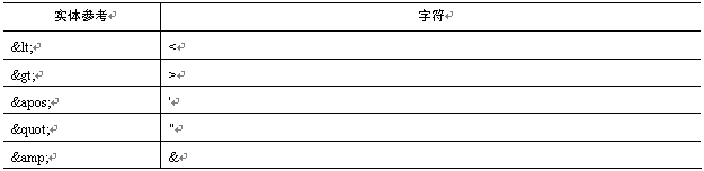
SimpleXML
Create SimpleXML Object
Simplexml_load_file() function that parses the specified file into memory
Simplexml_load_string() function that parses the created string into memory
Simplexml_load_date() function to import a domDocument object created using the dom function into memory
Traverse through all child elements
The children() method and foreach loop statement can iterate through all child node elements
Traverse through all attributes
attributes() method in SimpleXML object
<?xml version="1.0" encoding="GB2312"?> <exam> </exam>
<?php header('Content-Type:text/html;charset=utf-8'); ?> <?php /* First method */ $xml_1 = simplexml_load_file("5.xml"); print_r($xml_1); /* Second method */ $str = <<<XML <?xml version='1.0' encoding='gb2312'?> <Object> <ComputerBook> <title>PHP</title> </ComputerBook> </Object> XML; $xml_2 = simplexml_load_string($str); echo '<br>'; print_r($xml_2); /* Third method */ $dom = new domDocument(); $dom -> loadXML($str); $xml_3 = simplexml_import_dom($dom); echo '<br>'; print_r($xml_3); ?>
<?php header('Content-Type:text/html;charset=utf-8'); ?> <style type="text/css"> <?php $str = <<<XML <?xml version='1.0' encoding='gb2312'?> <object> <book> <computerbook>PHP</computerbook> </book> <book> <computerbook>PHP</computerbook> </book> </object> XML; $xml = simplexml_load_string($str); foreach($xml->children() as $layer_one){ print_r($layer_one); echo '<br>'; foreach($layer_one->children() as $layer_two){ print_r($layer_two); echo '<br>'; } } ?>
<?php $str = <<<XML <?xml version='1.0' encoding='gb2312'?> <object name='commodity'> <book type='computerbook'> <bookname name='22'/> </book> <book type='historybook'> <booknanme name='111'/> </book> </object> XML; $xml = simplexml_load_string($str); foreach($xml->children() as $layer_one){ foreach($layer_one->attributes() as $name => $vl){ echo $name.'::'.$vl; } echo '<br>'; foreach($layer_one->children() as $layer_two){ foreach($layer_two->attributes() as $nm => $vl){ echo $nm."::".$vl; } echo '<br>'; } } ?>
<?php header('Content-Type:text/html;charset=utf-8'); ?> <?php $str = <<<XML <?xml version='1.0' encoding='gb2312'?> <object name='commodity'> <book> <computerbook>P123</computerbook> </book> <book> <computerbook name='456'/> </book> </object> XML; $xml = simplexml_load_string($str); echo $xml[name].'<br>'; echo $xml->book[0]->computerbook.'<br>'; echo $xml->book[1]->computerbook['name'].'<br>'; ?>
<?php header('Content-Type:text/html;charset=utf-8'); $str=<<<XML <?xml version='1.0' encoding='gb2312'?> <object name='commodity'> <book> <computerbook type='12356'>123</computerbook> </book> </object> XML; $xml = simplexml_load_string($str); echo $xml[name].'<br />'; $xml->book->computerbook['type'] = iconv('gb2312','utf-8','PHP123'); $xml->book->computerbook = iconv('gb2312','utf-8','PHP456'); echo $xml->book->computerbook['type'].' => '; echo $xml->book->computerbook; ?>
<?php $xml = simplexml_load_file('10.xml'); $xml->book->computerbook['type'] = iconv('gb2312','utf-8','PHP1'); $xml->book->computerbook = iconv('gb2312','utf-8','PHP2'); $modi = $xml->asXML(); file_put_contents('10.xml',$modi); $str = file_get_contents('10.xml'); echo $str; ?>
<?php //Message_XML class, inherits the DomDocument class of PHP5 class Message_XML extends DomDocument{ //attribute private $Root; //Method //Constructor public function __construct() { parent:: __construct(); //Create or read an XML document message.xml that stores message information if (!file_exists("message.xml")){ $xmlstr = "<?xml version='1.0' encoding='GB2312'?><message></message>"; $this->loadXML($xmlstr); $this->save("message.xml"); } else $this->load("message.xml"); } public function add_message($user,$address){ //Add data $Root = $this->documentElement; //Get message message $admin_id =date("Ynjhis"); $Node_admin_id= $this->createElement("admin_id"); $text= $this->createTextNode(iconv("GB2312","UTF-8",$admin_id)); $Node_admin_id->appendChild($text); $Node_user = $this->createElement("user"); $text = $this->createTextNode(iconv("GB2312","UTF-8",$user)); $Node_user->appendChild($text); $Node_address = $this->createElement("address"); $text= $this->createTextNode(iconv("GB2312","UTF-8",$address)); $Node_address->appendChild($text); $Node_Record = $this->createElement("record"); $Node_Record->appendChild($Node_admin_id); $Node_Record->appendChild($Node_user); $Node_Record->appendChild($Node_address); //Join under the root node $Root->appendChild($Node_Record); $this->save("message.xml"); echo "<script>alert('Added Successfully');location.href='".$_SERVER['PHP_SELF']."'</script>"; } public function delete_message($admin_id){ //Delete data $Root = $this->documentElement; $xpath = new DOMXPath($this); $Node_Record= $xpath->query("//record[admin_id='$admin_id']"); $Root->removeChild($Node_Record->item(0)); $this->save("message.xml"); echo "<script>alert('Delete succeeded');location.href='".$_SERVER['PHP_SELF']."'</script>"; } public function show_message(){ //Read data $root=$this-documentElement; $xpath=new DOMXPath($this); $Node_Record=$this->getElementsByTagName("record"); $Node_Record_length=$Node_Record->length; print"<table width='506' bgcolor='#FFFFCC'><tr>"; print"<td width='106' height='22' align='center'>"; print"<b>User name</b>"; print"</td><td width='400' align='center'>"; print"<b>Message message</b></td></tr>"; for($i=0;$i<$Node_Record->length;$i++){ $k=0; foreach($Node_Record->item($i)->childNodes as $articles){ $field[$k]=iconv("UTF-8","GB2312",$articles->textContent); $k++; } print"<table width='506' bgcolor='#FFFFCC'><tr>"; print"<td width='100' height='22' align='center'>"; print"$field[1]"; print"</td><td width='300' align='left'>"; print"$field[2]"; print"</td><td width='100' align='center'>"; print"<a href='?Action=delete_message&admin_id=$field[0]'>delete</a></td>"; print"</tr></table>"; }} public function post_message(){ print "<table width='506' bgcolor='#FFFFCC'><form method='post' action='?Action=add_message'>"; print "<tr><td width='106'height='22'> User name:</td><td><input type=text name='user' size=50></td></tr>"; print "<tr><td width='106' height='22'> Message message message:</td><td width='400'><textarea name='address' cols='48' rows='5' id='address'></textarea></td></tr>"; print "<tr><td width='106' height='30'> <input type='submit' value='Add data'></td><td align='right'><a href=?Action=show_message>View data</a> </td></tr></form></table>"; } } ?> <html> <head> <title>Define a PHP read XML class</title> <style> td,body{font-size:12px} a:link { text-decoration: none; } a:visited { text-decoration: none; } a:hover { text-decoration: none; } a:active { text-decoration: none; } </style> <meta http-equiv="Content-Type" content="text/html; charset=gb2312"></head> <body> <table width=506 height=50 border=0 cellpadding=0 cellspacing=0 bgcolor="#33BE6B"> <tr> <td width="506" height=50 valign="bottom" background="title.gif"><table width="506"> <tr> <td height="24" align="right" scope="col"> <a href=?Action=post_message>Add data</a> </td> </tr> </table></td> </tr> <?php $HawkXML = new Message_XML; $Action =""; if(isset($_GET['Action'])) $Action = $_GET['Action']; switch($Action){ case "show_message": //See $HawkXML->show_message(); break; case "post_message": //Submit $HawkXML->post_message(); break; case "add_message": //Add to $HawkXML->add_message($_POST['user'],$_POST['address']); break; case "delete_message": //delete $HawkXML->delete_message($_GET['admin_id']); break; } ?> </table> </body> </html>
<?php //Message_XML class, inherits the DomDocument class of PHP5 class Message_XML extends DomDocument{ //attribute private $Root; //Method //Constructor public function __construct() { parent:: __construct(); //Create or read an XML document message.xml that stores message information if (!file_exists("message.xml")){ $xmlstr = "<?xml version='1.0' encoding='GB2312'?><message></message>"; $this->loadXML($xmlstr); $this->save("message.xml"); } else $this->load("message.xml"); } public function add_message($user,$address){ //Add data $Root = $this->documentElement; //Get message message $admin_id =date("Ynjhis"); $Node_admin_id= $this->createElement("admin_id"); $text= $this->createTextNode(iconv("GB2312","UTF-8",$admin_id)); $Node_admin_id->appendChild($text); $Node_user = $this->createElement("user"); $text = $this->createTextNode(iconv("GB2312","UTF-8",$user)); $Node_user->appendChild($text); $Node_address = $this->createElement("address"); $text= $this->createTextNode(iconv("GB2312","UTF-8",$address)); $Node_address->appendChild($text); $Node_Record = $this->createElement("record"); $Node_Record->appendChild($Node_admin_id); $Node_Record->appendChild($Node_user); $Node_Record->appendChild($Node_address); //Join under the root node $Root->appendChild($Node_Record); $this->save("message.xml"); echo "<script>alert('Added Successfully');location.href='".$_SERVER['PHP_SELF']."'</script>"; } public function show_message(){ //Read data $root=$this-documentElement; $xpath=new DOMXPath($this); $Node_Record=$this->getElementsByTagName("record"); $Node_Record_length=$Node_Record->length; print"<table width='506' bgcolor='#FFFFCC'><tr>"; print"<td width='106' height='22' align='center'>"; print"<b>User name</b>"; print"</td><td width='400' align='center'>"; print"<b>Message message</b></td></tr>"; for($i=0;$i<$Node_Record->length;$i++){ $k=0; foreach($Node_Record->item($i)->childNodes as $articles){ $field[$k]=iconv("UTF-8","GB2312",$articles->textContent); $k++; } print"<table width='506' bgcolor='#FFFFCC'><tr>"; print"<td width='100' height='22' align='center'>"; print"$field[1]"; print"</td><td width='400' align='left'>"; print"$field[2]"; print"</td>"; print"</tr></table>"; }} public function post_message(){ print "<table width='506' bgcolor='#FFFFCC'><form method='post' action='?Action=add_message'>"; print "<tr><td width='106'height='22'> User name:</td><td><input type=text name='user' size=50></td></tr>"; print "<tr><td width='106' height='22'> Message message message:</td><td width='400'><textarea name='address' cols='48' rows='5' id='address'></textarea></td></tr>"; print "<tr><td width='106' height='30'> <input type='submit' value='Add data'></td><td align='right'><a href=?Action=show_message>View data</a> </td></tr></form></table>"; } } ?> <html> <head> <title>Use XML To store a small amount of data</title> <style> td,body{font-size:12px} a:link { text-decoration: none; } a:visited { text-decoration: none; } a:hover { text-decoration: none; } a:active { text-decoration: none; } </style> <meta http-equiv="Content-Type" content="text/html; charset=gb2312"></head> <body> <table width=506 height=50 border=0 cellpadding=0 cellspacing=0 bgcolor="#33BE6B"> <tr> <td width="506" height=50 valign="bottom" background="title.gif"><table width="506"> <tr> <td height="24" align="right" scope="col"> <a href=?Action=post_message>Add data</a> </td> </tr> </table></td> </tr> <?php $HawkXML = new Message_XML; $Action =""; if(isset($_GET['Action'])) $Action = $_GET['Action']; switch($Action){ case "show_message": //See $HawkXML->show_message(); break; case "post_message": //Submit $HawkXML->post_message(); break; case "add_message": //Add to $HawkXML->add_message($_POST['user'],$_POST['address']); break; } ?> </table> </body> </html>
afterword
Okay, please leave a message in the message area to share your experience and experience.
Thank you for learning today. If you find this article helpful, you are welcome to share it with more friends. Thank you.
Thank!Thank you!Your sincere appreciation is my greatest motivation to move forward!
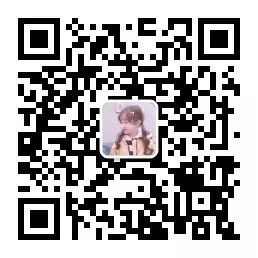
