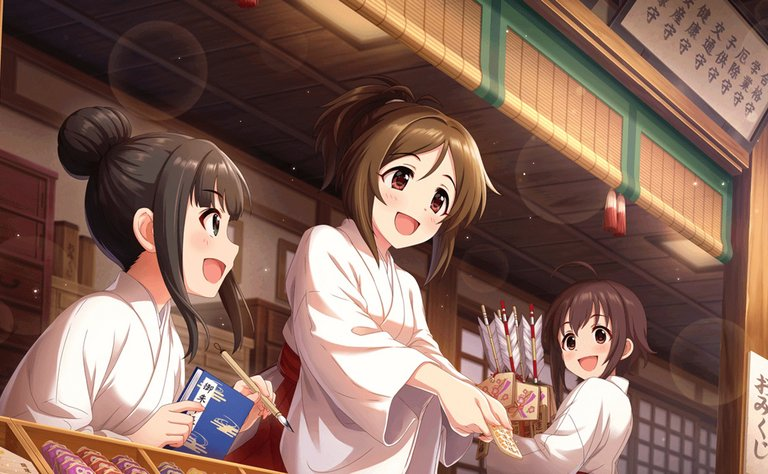
The thinkphp framework is a free, open source, fast and simple object-oriented lightweight PHP development framework.
Learn what is ThinkPHP overview, the directory structure of ThinkPHP projects, the controller of thinkphp, views, the process of building ThinkPHP projects, the configuration of thinkphp, the model of thinkphp, and familiarity with the built-in template engine.
The thinkphp framework is a lightweight PHP development framework with rich functions, which makes web application development simpler and faster.
Characteristic:
Class library import, url mode, compilation mechanism, query language, view model, grouping module, template engine, ajax support, caching mechanism.
Thinkphp supports a windows/unix server environment and can run on a variety of web services including Apache and iis.Download thinkPHP:
The directory structure of ThinkPHP
Automatically generate directories
Project catalog deployment scenario
Naming Specification
Project Construction Process
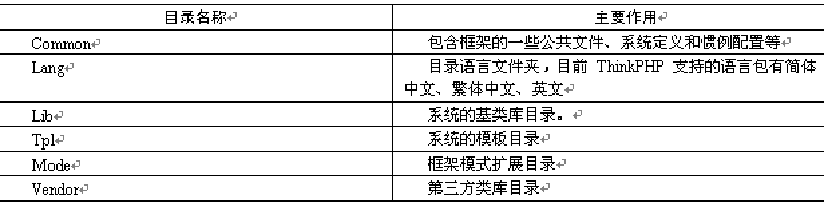
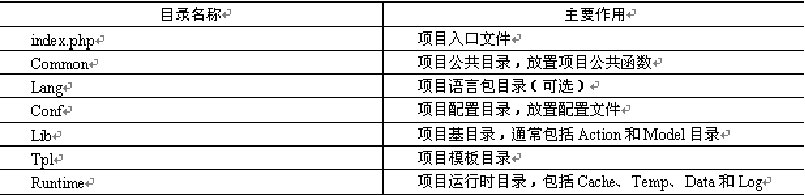
Automatically generate directories
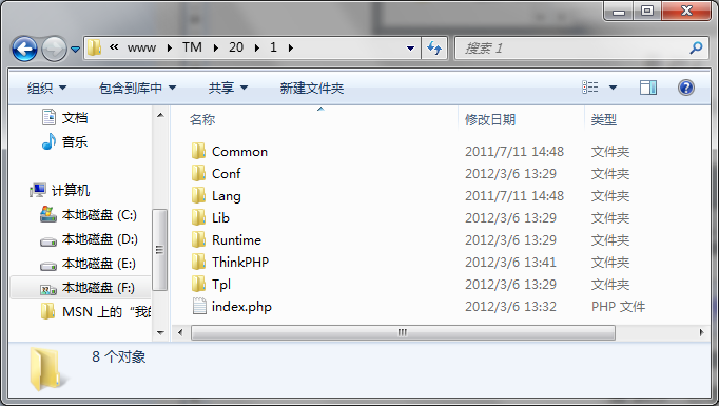
Project catalog deployment scenario
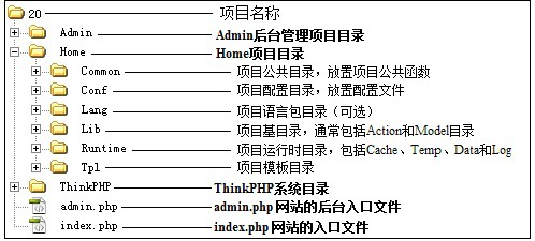
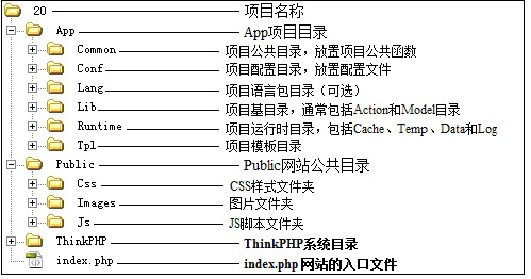
Project Construction Process
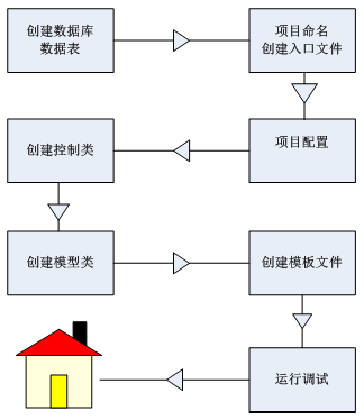
Configuration of ThinkPHP
Configuration Format
Debugging Configuration
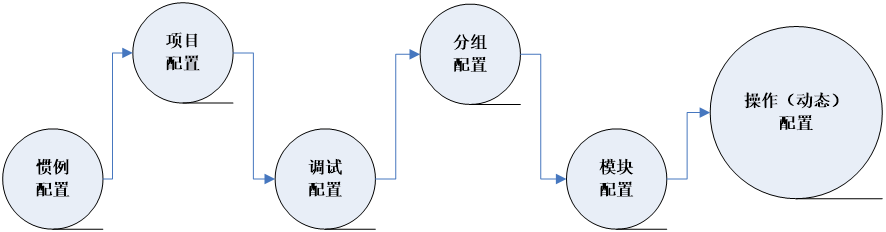
Controller for ThinkPHP
Controller
Cross-Module Calls
1. Naming models
2. Instantiation Model
3. Attribute Access
4. Connect to the database
5. Create data
6. Continuous operation
7. CURD Operation
<?php $db = array ( 'server' => 'localhost', 'port' => '3306', 'username' => 'root', 'password' => 'dada', 'database' => 'dada' ); $conn = @mysql_connect($db['server'].':'.$db['port'],$db['username'],$db['password']); if (! $conn) { echo "Server not connected!" . mysql_error(); } else { // Declare Character Set mysql_set_charset('utf8', $conn); // Select Database mysql_select_db($db['database'], $conn); }
<?php if (! isset ( $_SESSION )) { session_start (); } if (! isset ( $_SESSION ['userName'] )) { header ( "location:login.php" ); } $userName = $_SESSION ['userName']; // Access the database and query the student table for the assigned number of students require_once 'dbconfig.php'; if (! isset ( $_REQUEST ['id'] )) { header ( "location:index.php" ); } $id = $_REQUEST ['id']; $sql = "select * from student where id = $id"; // exit($sql); $result = mysql_query ( $sql ); $row = mysql_fetch_array ( $result )?> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Student Information</title> </head> <body> <div align='right'>User name:<?=$userName?> <a href='loginout.php'>Log out</a></a> </div> <div align='center'> <hr /> <h1>Student Information</h1> <form action='editdo.php' method='post'> <input type='hidden' name='id' value='<?=$row ['id']?>'/> <table width=300> <tr> <td align='center'>School Number</td> <td><input type='text' name='studentId' value='<?=$row ['studentId']?>' /></td> </tr> <tr> <td align='center'>Full name</td> <td><input type='text' name='name' value='<?=$row ['name']?>' /></td> </tr> <tr> <td align='center'>class</td> <td><input type='text' name='className' value='<?=$row ['className']?>' /></td> </tr> <tr> <td align='center'>Birthday</td> <td><input type='text' name='birthday' value='<?=$row ['birthday']?>' /></td> </tr> <tr> <td align='center'>Gender</td> <td> <input type='radio' name='sex' value='male' <?=$row ['sex']=='male'?'checked':''?>>male </input> <input type='radio' name='sex' value='female' <?=$row ['sex']=='female'?'checked':''?>>female</input> </td> </tr> <tr> <td align='center'>Nation</td> <td><input type='text' name='nation' value='<?=$row ['nation']?>' /></td> </tr> <tr> <td colspan=2 align='center'><input type='submit' value='Confirm Modification' /></td> </tr> </table> </form> </div> </body> </html>
<?php require_once 'dbconfig.php'; header ( "content-type:text/html;charset=utf-8" ); // Get Form Data $id = $_REQUEST ['id']; $studentId = $_REQUEST ['studentId']; $name = $_REQUEST ['name']; $className = $_REQUEST ['className']; $birthday = $_REQUEST ['birthday']; $sex = $_REQUEST ['sex']; $nation = $_REQUEST ['nation']; // Quoted string data types are used in sql statements, and number fields are free to use $sql = "update student set studentId ='$studentId',name = '$name',className = '$className',birthday = '$birthday',sex ='$sex',nation='$nation' where id = $id"; if (mysql_query ( $sql )) { echo "Successful modification!!!<br/>"; echo "<a href='index.php'>Back to Home Page</a>"; } else { echo "Modification failed!!!<br/>"; echo "<a href='index.php'>System Error</a>"; }
<?php if (! isset ( $_SESSION )) { session_start (); } if (! isset ( $_SESSION ['userName'] )) { header ( "location:login.php" ); } $userName = $_SESSION ['userName']; // Access database, query student table require_once 'dbconfig.php'; $sql = "select * from student"; $result = mysql_query ( $sql ); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Student Information</title> </head> <body> <div align='right'>User name:<?=$userName?> <a href='loginout.php'>Log out</a></a> </div> <hr /> <h1>Student Information</h1> <table border=1> <tr> <th>School Number</td> <th>Full name</td> <th>class</td> <th>Birthday</td> <th>Gender</td> <th>Nation</td> <th>operation</th> </tr> <?php while ( $row = mysql_fetch_array ( $result ) ) { echo "<tr>"; echo "<td>" . $row ['studentId'] . "</td>"; echo "<td>" . $row ['name'] . "</td>"; echo "<td>" . $row ['className'] . "</td>"; echo "<td>" . $row ['birthday'] . "</td>"; echo "<td>" . $row ['sex'] . "</td>"; echo "<td>" . $row ['nation'] . "</td>"; echo "<td>" ."<a href=\"edit.php?id='". $row ['id'] ."'\">edit</a></td>"; echo "</tr>"; } ?> </table> </body> </html>
<html> <head> <title>Login</title> <meta http-equiv="Content-Type" content= "text/html; charset=utf-8" > </head> <body> <h1>1606 Sign in</h1> <form name="form1" method= "post" action= "logindo.php" > <table width="300" border= "0" align= "center" cellpadding= "2" cellspacing= "2" > <tr> <td width="150" ><div align= "right" >User name:</div></td> <td width="150" ><input type= "text" name= username ></td> </tr> <tr> <td><div align="right" >Password:</div></td> <td><input type="password" name= "passcode" ></td> </tr> </table> <p align="center" > <input type="submit" name= "Submit" value= "Sign in" > <input type="reset" name= "Reset" value= "Reset" > <a href='register.php'>register</a> </p> </form> </body> </html>
<?php header ( "content-type:text/html;charset=utf-8" ); if (! isset ( $_SESSION )) { session_start (); } if (isset ( $_SESSION ['userName'] )) { header ( "location:index.php" ); } elseif (! isset ( $_REQUEST ['username'] )) { header ( "location:login.php" ); } else { $username = $_POST ['username']; $passcode = $_POST ['passcode']; //Calculation Summary $password2 = sha1 ( $passcode ); require_once 'dbconfig.php'; // Query account list based on user name and password $sql = "select * from user where username= '$username' and password='$password2'"; $result = mysql_query ( $sql, $conn ); if ($row = mysql_fetch_array ( $result )) { $_SESSION ['userName'] = $username; header ( "location:index.php" ); } else { echo "<script>alert('ERROR Incorrect username or password!');</script>"; echo "Wrong username or password!<br/>"; echo "<a href='login.php'>Re-login</a>"; } } ?>
<?php if(!isset($_SESSION)){ session_start(); } session_destroy(); header("location:login.php");
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <h1 align='center'>Welcome to Registration</h1> <hr> <form action="registerdo.php" method='post'> <label>User name:</label><input type='text' name='username' /> <label>Password:</label><input type='text' name='password' /> <input type='submit' name='hh' value='Submit' /> </form> </body> </html>
<?php require_once 'dbconfig.php'; header("content-type:text/html;charset=utf-8"); //Get Form Data $username = $_REQUEST['username']; $password = $_REQUEST['password']; $password2 = sha1($password); //Quoted string data types are used in sql statements, and number fields are free to use $sql = "INSERT INTO user(id, username, password, status) VALUES (null,'$username','$password2',1)"; //exit($sql); if(mysql_query($sql)){ echo "Registration successful!!!<br/>"; echo "<a href='login.php'>Go Log In</a>"; }else{ echo "Registration failed!!!<br/>"; echo "<a href='register.php'>Re-registration</a>"; }
afterword
Okay, please leave a message in the message area to share your experience and experience.
Thank you for learning today. If you find this article helpful, you are welcome to share it with more friends. Thank you.
Thank!Thank you!Your sincere appreciation is my greatest motivation to move forward!
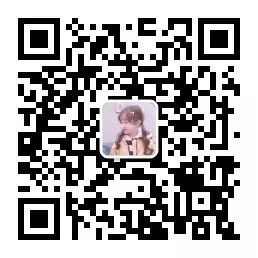
