1.
What is the pointer
2.
Pointer and pointer type
3.
Field pointer
4.
Pointer operation
5.
Pointers and arrays
6.
Secondary pointer
7.
Pointer array
1. What is the pointer?
What is the pointer?
Two key points of pointer understanding:
1. A pointer is the number of the smallest unit in memory, that is, the address
2. Pointer in spoken language usually refers to pointer variable, which is used to store memory address
summary
: pointer is the address. In colloquial language, pointer usually refers to pointer variable.
Pointer variable
We can use & (get address operator) to get the actual memory address of the variable and store the address in a variable
A variable is a pointer variable
#include <stdio.h> int main() { int a = 10;//Open up a space in memory int *p = &a;//Here we take the address of variable a, and we can use the & operator. //The a variable takes up 4 bytes. Here, the address of the first byte of the 4 bytes of a is stored in the p variable //In, p is a pointer variable. return 0; }
Summary:
Pointer variable, a variable used to store the address. (the values stored in the pointer are treated as addresses).
The question here is:
- How big is a small unit? (1 byte)
- How to address?
After careful calculation and trade-off, we find that it is more appropriate to give a corresponding address to a byte.
For
32
Bit machine, assuming
32
For each address line, it is assumed that the high level (high voltage) and low level (low voltage) generated by each address line during addressing is (1 or
0
);
So
32
The address generated by the root address line will be:
00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000001
...
11111111 11111111 11111111 11111111
Here it is
2
of
32
Address to the power.
Each address identifies a byte, then we can give
(
2^32Byte == 2^32/1024KB ==
2^32/1024/1024MB==2^32/1024/1024/1024GB == 4GB
)
4G
Address the idle.
Here we understand:
stay
32
The address is
32
individual
0
perhaps
1
To form a binary sequence, the address has to be used
4
A byte of space to store, so
The size of a pointer variable should be
4
Bytes.
If in
64
On the machine, if any
64
What is the size of a pointer variable
8
Bytes to store an address.
Summary:
Pointers are used to store addresses, which uniquely identify an address space.
The size of the pointer is
32
Bit platform is
4
Bytes, in
64
Bit platform is
8
Bytes.
2. Pointer and pointer type
Here we are discussing the type of pointer
As we all know, variables have different types, such as integer, floating point, etc. Does the pointer have a type?
To be exact: Yes.
int num = 10; p = #
Will
&num
(
num
Save to
p
In, we know
p
Is a pointer variable. What is its type?
We give the pointer variable the corresponding type.
char *pc = NULL; int *pi = NULL; short *ps = NULL; long *pl = NULL; float *pf = NULL; double *pd = NULL;
Here you can see that the pointer is defined as follows:
type + *
.
Actually:
char*
Type pointer is used to store
char
Address of the type variable.
short*
Type pointer is used to store
short
Address of the type variable.
int*
Type pointer is used to store
int
Address of the type variable.
2.1 pointer + - integer
#include <stdio.h> int main() { int a = 10; int* pa = &a; char* pc = &a; printf("%p\n", pa); printf("%p\n", pc); printf("%p\n", pa + 1); printf("%p\n", pc + 1); return 0; }
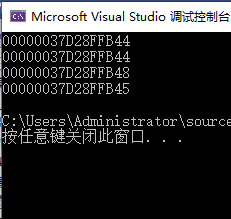
Summary:
The type of pointer determines how far the pointer moves forward or backward.
2.2 dereference of pointer
#include <stdio.h> int main() { int n = 0x11223344; char *pc = (char *)&n; int *pi = &n; *pc = 0; //Focus on observing the changes of memory during debugging. *pi = 0; //Focus on observing the changes of memory during debugging. return 0; }
Summary:
The type of pointer determines how much permission you have to dereference the pointer (can operate several bytes).
For example:
char*
Pointer dereference can only access one byte, and
int*
The dereference of the pointer can access four bytes
3. Field pointer
Concept: Wild pointer means that the position pointed by the pointer is unknowable (random, incorrect and unrestricted)
3.1 cause of formation
1. Pointer not initialized
#include <stdio.h> int main() { int *p;//Local variable pointer is uninitialized and defaults to random value *p = 20; return 0; }
2. Pointer cross-border access
#include <stdio.h> int main() { int arr[5] = { 1,2,3,4,5 }; int i = 0; int* p = arr; for (i = 0; i < 10; i++) { printf("%d ", *p); //When the range pointed to by the pointer exceeds the range of array arr, p is the wild pointer p++; } return 0; }
3. The space pointed by the pointer is released
3.2 how to avoid wild pointer
1.
Pointer initialization
2.
Be careful that the pointer is out of bounds
3.
Pointer to space release
NULL
4.
Avoid returning the address of a local variable
5.
Check validity of pointer before use
#include <stdio.h> int main() { int a = 10; p = &a; int* p = NULL; //.... if (p != NULL) { *p = 20; } return 0; }
4. Pointer operation
- Pointer + - integer
- Pointer pointer
- Relational operation of pointer
4.1 pointer + - integer
#define N_VALUES 5 float values[N_VALUES]; float *vp; //Pointer + - integer; Relational operation of pointer for (vp = &values[0]; vp < &values[N_VALUES];) { *vp++ = 0; }
4.2 pointer - pointer
#include <stdio.h> int main() { int arr[10] = { 0 }; printf("%d\n", &arr[0] - &arr[9]); return 0; } //Premise: pointer pointer is that two pointers must point to the same space //Pointer - pointer, which gets the number of elements between the pointer and the pointer
4.3 pointer relation operation
for(vp = &values[N_VALUES]; vp > &values[0];) { *--vp = 0; }
Code simplification
,
This modifies the code as follows:
for(vp = &values[N_VALUES-1]; vp >= &values[0];vp--) { *vp = 0; }
In fact, most compilers can successfully complete the task, but we should avoid writing this, because the standard does not guarantee its feasibility.
Standard provisions:
A pointer to an array element is allowed to be compared with a pointer to the memory location after the last element of the array, but not with
A pointer to the memory location before the first element is compared.
5. Pointers and arrays
#include <stdio.h> int main() { int arr[10] = { 1,2,3,4,5,6,7,8,9,0 }; printf("%p\n", arr); printf("%p\n", &arr[0]); return 0; }
Operation results:
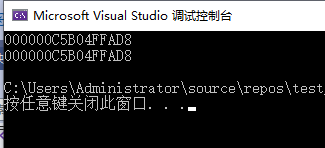
It can be seen that the array name and the address of the first element of the array are the same.
Conclusion:
The array name represents the address of the first element of the array
.
Then it is feasible to write code like this:
int arr[10] = {1,2,3,4,5,6,7,8,9,0}; int *p = arr;//p stores the address of the first element of the array
Since we can store the pointer as an address in the array.
For example:
#include <stdio.h> int main() { int arr[] = { 1,2,3,4,5,6,7,8,9,0 }; int* p = arr; //Pointer to the address of the first element of the array int sz = sizeof(arr) / sizeof(arr[0]); for (i = 0; i < sz; i++) { printf("&arr[%d] = %p <====> p+%d = %p\n", i, &arr[i], i, p + i); } return 0; }
Operation results:
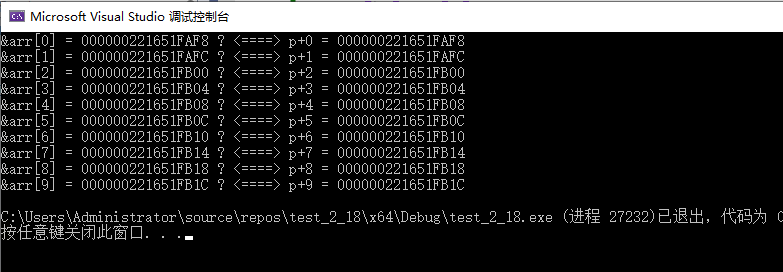
therefore
p+i
In fact, the calculation is an array
arr
Subscript
i
Your address.
Then we can access the array directly through the pointer.
As follows:
int main() { int arr[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 }; int *p = arr; //Pointer to the address of the first element of the array int sz = sizeof(arr) / sizeof(arr[0]); int i = 0; for (i = 0; i<sz; i++) { printf("%d ", *(p + i)); } return 0; }
6. Secondary pointer
Pointer variable is also a variable. If it is a variable, it has an address. Where is the address of pointer variable stored?
This is it.
Secondary pointer
.
The operations of the secondary pointer are:
*ppa
Pass on
ppa
Dereference the address in, so that you can find
pa
,
*ppa
In fact, the interview is
pa
.
int b = 20; *ppa = &b;//Equivalent to PA = & B;
**ppa
Pass first
*ppa
find
pa
,
Then yes
pa
To dereference:
*pa
, what did you find
a
.
**ppa = 30; //Equivalent to * pa = 30; //Equivalent to a = 30;
7. Pointer array
Is a pointer array a pointer or an array?
Answer: array. Is an array of pointers.
Array, we already know the integer array, the character array.
int arr1[5]; char arr2[6];
What about array pointers?
int* arr3[5];//What is it?
arr3
Is an array with five elements, each of which is an integer pointer.