Vue3.0 installation
npm i vue@next vue-loader@next npm install vue-router@next npm i webpack webpack-cli webpack-dev-server --save-dev
typescript installation dependency
npm install ts-loader --save-dev npm install typescript --save-dev npm install @vue/babel-plugin-jsx -D npm i @babel/preset-env @babel/preset-typescript @vue/babel-plugin-jsx --save-dev
Add in. babelrc or babel.config.js
//babel.config.js module.exports = { presets: [["@babel/preset-env"], "@babel/preset-typescript"], plugins: [["@vue/babel-plugin-jsx"]], };
webpack.module.rules configuration
{ test: /\.tsx?$/, use: [ { loader: "babel-loader", }, { loader: "ts-loader", }, ], }, { test: /\.js(x)*$/, loader: "babel-loader", },
be careful: webpack 5 requires at least Node.js 10.13.0 (LTS)
The problems encountered are as follows:
1. Vue3. X Vue router4. X is written in the following version:
Change the original mode: "history" to history: createWebHistory(). (set other modes in the same way).
//Install version "vue": "^3.0.2", "vue-loader": "^16.0.0-rc.1", "vue-router": "^4.0.0-rc.3"
Comparison between vue2 router and vue3 Router:
// vue2-router const router = new VueRouter({ mode: history , ... }) //vue3-router(vue-next-router) import { createRouter, createWebHistory } from vue-next-router const router = createRouter({ history: createWebHistory(), ... })
explain: 1. The history of vue3 router replaces the new option mode 2. The mode: 'history' option of vue2 router has been replaced with a more flexible name history. Depending on the mode used, replace it with the appropriate function:
"history":createWebHistory() "hash":createWebHashHistory() "abstract":createMemoryHistory()
Details: Click me to check
2. Cause of problem: TypeError:window.Vue.use is not a function
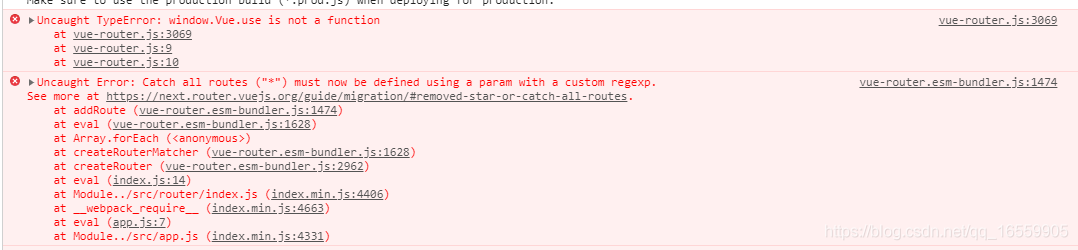
Problem Description:
I used Vue router ":" ^ 4.0.0-rc. 3 in package.json
However, the 3.4.9 version is referenced in index.html, so using vue3. X + Vue router4. X causes the following exception
<script src="https://unpkg.com/vue-router@3.4.9/dist/vue-router.js"></script>
Solution:
"vue-router": "^4.0.0-rc.3" //or is referenced in index.html <script src="https://unpkg.com/vue-router@next"></script>
3. vue-i18n use
According to vue-i18n, vue-i18n will soon be transferred to the consolidated organization. After that, it will be developed and maintained on intlify.
Question: vue-i18n will soon be transferred to intlify organization. After that, it will be developed and maintained on intlify,
See for details here Original writing:
import VueI18n from 'vue-i18n'; import messages from './404'; export const i18n = new VueI18n({ locale: 'zh-CN', messages, });
Now, it needs to be installed and used
//install //npm i @intlify/vue-i18n-loader //use import messages from "./404"; import { useI18n, createI18n } from "vue-i18n"; const i18n = createI18n({ locale: "zh-CN", messages, }); export { i18n };
More use: see also 4. For example: uncaught referenceerror:__ VUE_ PROD_ DEVTOOLS__ is not defined
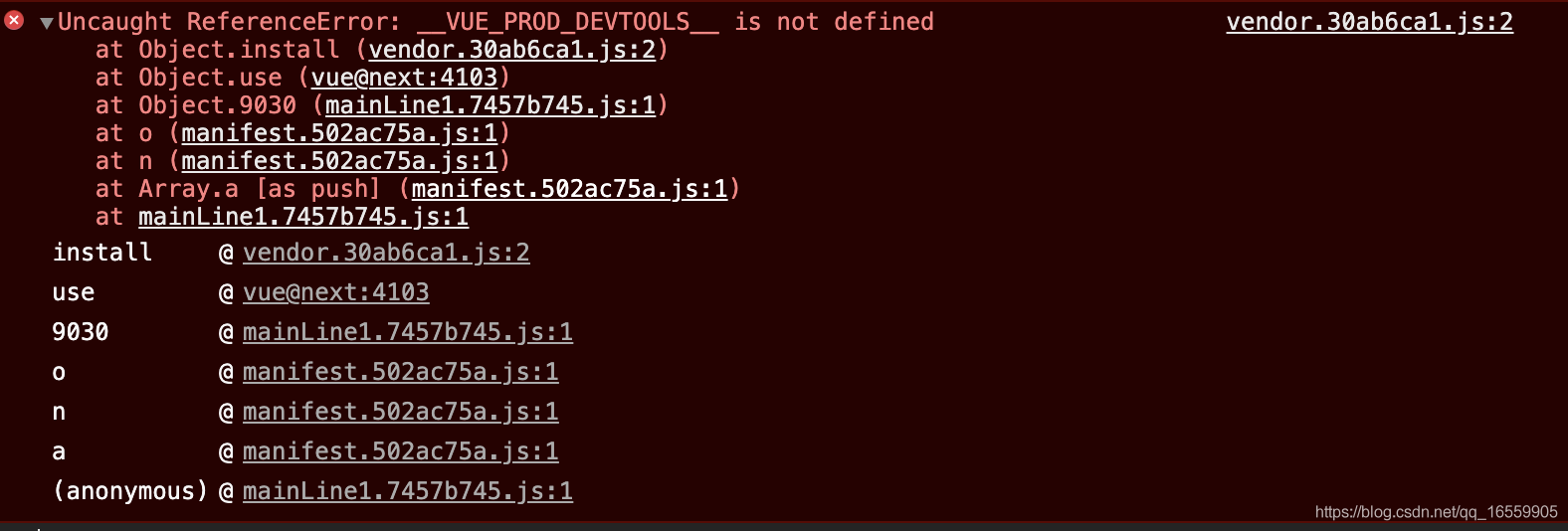
Because the program does not allow the use of undefined variables, the current use of vue 3.x will report an error. (I haven't tried Vue's new vite)
Solution: Replace them when compiled using webpack.DefinePlugin:
const webpack = require('webpack'); plugins: [ new webpack.DefinePlugin({ __VUE_OPTIONS_API__: JSON.stringify(true), __VUE_PROD_DEVTOOLS__: JSON.stringify(false) }) ],
Reference link: Click me to check
5. Set the environment variable process.env.node in the webpack configuration_ ENV
// webpack.config.js module.exports = { // webpack mode (mode parameter): different built-in optimizations are performed in different modes mode: process.env.NODE_ENV === 'production' ? 'production' : 'development' }
NODE_ENV is a variable that needs to be set for the current environment variable in a specific application. Generally, it will be set when executing different commands Because this variable is set differently for different operating systems, for example, if it is set to development mode,
//window system set NODE_ENV=development //mac system export NODE_ENV=development
This is not convenient for switching, so cross env is provided. It is a cross platform third-party package that needs to be installed when using,
//Setting the environment variable: cross env node_ Env = development npm i --save-dev cross-env
For example: package.json
{ "scripts": { // It is set as the development environment and packaged and deployed according to the configuration of webpack.dev.js "dev": "cross-env NODE_ENV=development webpack --config webpack.config.js" } }
6. When TypeScript references a resource file, it prompts for an error handling scheme that cannot be found
Problem Description: reference video or picture in tsx, and prompt wrong reference in file text editor
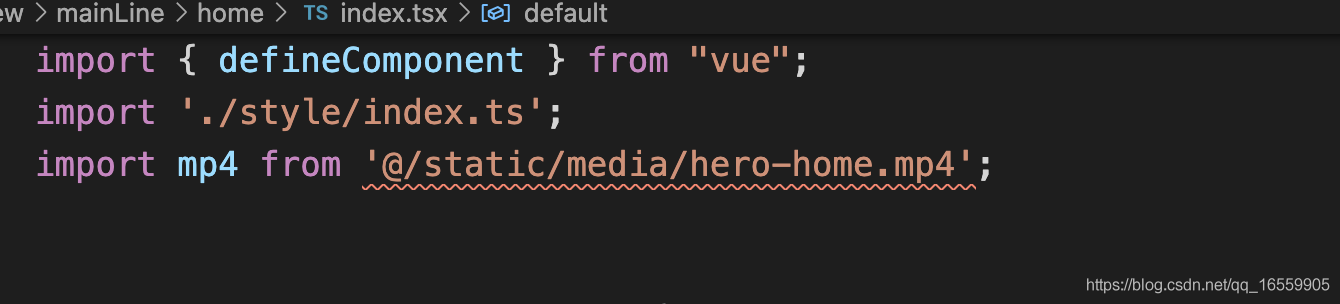
Problem: ERROR: ts2307: cannot find module '@ / static / media / hero home. MP4' or its corresponding type declarations
Reason: the file can be found, and the page can be opened and displayed normally at the same time, but the compilation fails, resulting in the failure of follow-up!
Solution: Note: typescript cannot recognize non code files (available under JS). If you need to identify this file resource in ts, you can declare the file type first.
Create a new TS file, such as global.d.ts (. D.ts is the abbreviation of typescript declaration file), and put it in the main code folder.
In the ts file, add declarations of various file types, such as:
declare module '*.svg' { interface Svg { content: string; id: string; viewBox: string; node: any; } const svg: Svg; export default svg; } declare module '*.png' { const png: string; export default png; } declare module '*.mp3' { const mp3: string; export default mp3; } declare module '*.mp4' { const mp3: string; export default mp4; } declare module '*.gif' { const png: string; export default png; }
It can also be abbreviated as declare module '*.png';
When the project is compiled, the contents of the file are automatically read. Then you can identify the resource file
reference resources: Point me here