Processing of multiple AIDL files connected by a Binder connection pool
Note beforehand:
I am also a beginner, so this article starts from a beginner's point of view, if there are inappropriate places, please leave a message to teach me, thank you.
If you don't understand the use of AIDL and the Binder mechanism, you can refer to my previous articles. Android Foundation - The Binder Mechanism of AIDL on Application Layer for Beginners,http://blog.csdn.net/qq_30379689/article/details/52253413
Preface:
According to our previous use of AIDL, one AIDL interface must be satisfied for one service.If our application requires more than one AIDL for more than one module, then more than one Service end is needed. Service is the four components with a high memory footprint, which affects the performance of the application.So we need to put all the AIDLs into one service to manage them.
Welcome to my CSDN blog, Hensen_blog, http://blog.csdn.net/qq_30379689
How Binder Connection Pooling works:
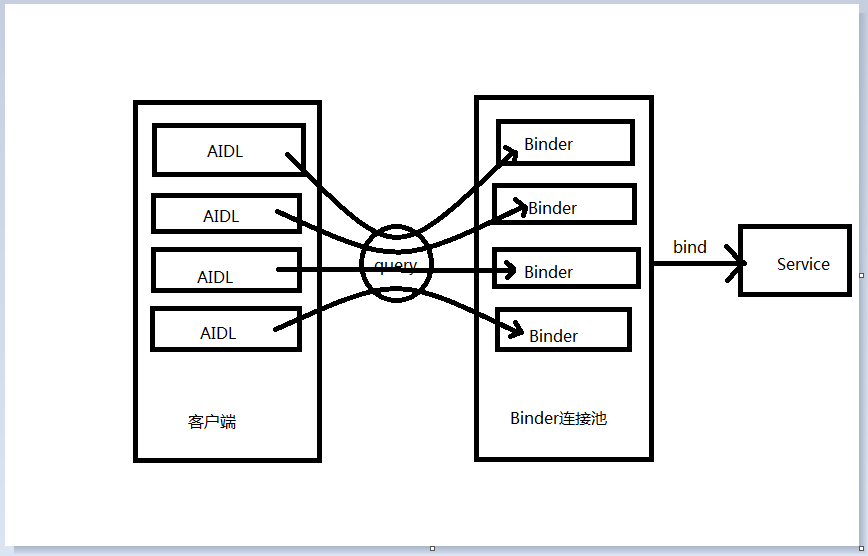
Service-side operations
Step 1: Create the aidl file needed by the two modules and create a Binder connection pool aidl file to compile Gradle
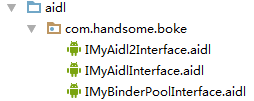
-
interface IMyAidlInterface {
-
int add(int num1,int num2);
-
}
-
interface IMyAidl2Interface {
-
String print_A();
-
String print_B();
-
}
-
interface IMyBinderPoolInterface {
-
IBinder queryBinder(int binderCode);
-
}
Step 2: Create two modules to implement the aidl file class and create a Binder connection pool class and implement, create a server
-
public class IMyAidlImpl extends IMyAidlInterface.Stub {
-
@Override
-
public int add(int num1, int num2) throws RemoteException {
-
return num1 + num2;
-
}
-
}
-
public class IMyAidl2Impl extends IMyAidl2Interface.Stub {
-
@Override
-
public String print_A() throws RemoteException {
-
return "A";
-
}
-
-
@Override
-
public String print_B() throws RemoteException {
-
return "B";
-
}
-
}
-
public class BinderPool {
-
-
private static final int BINDER_1 = 0;
-
private static final int BINDER_2 = 1;
-
-
-
-
-
public static class IMyBinderPoolImpl extends IMyBinderPoolInterface.Stub {
-
-
@Override
-
public IBinder queryBinder(int binderCode) throws RemoteException {
-
IBinder binder = null;
-
switch (binderCode) {
-
case BINDER_1:
-
binder = new IMyAidlImpl();
-
break;
-
case BINDER_2:
-
binder = new IMyAidl2Impl();
-
break;
-
default:
-
break;
-
}
-
return binder;
-
}
-
}
-
}
-
public class MyService extends Service {
-
-
private Binder myBinder = new BinderPool.IMyBinderPoolImpl();
-
-
@Override
-
public IBinder onBind(Intent intent) {
-
return myBinder;
-
}
-
-
@Override
-
public void onCreate() {
-
super.onCreate();
-
}
-
-
@Override
-
public void onDestroy() {
-
super.onDestroy();
-
}
-
}
Step 3: Configure Service in manifests
-
<service
-
android:name=".Aidl.MyService"
-
android:exported="true" />
Step 4: Start the service in code
-
public class LoginActivity extends AppCompatActivity {
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_login);
-
-
startService(new Intent(this, MyService.class));
-
}
-
}
Client Operations
Step 1: Copy the server-side aidl file to the client and compile Gradle
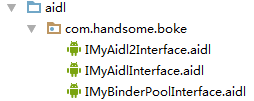
Step 2: Write the code for the Binder connection pool and explain it in the code
Step 3: Use in code
-
public class MainActivity extends AppCompatActivity {
-
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_main);
-
new Thread(new Runnable() {
-
@Override
-
public void run() {
-
-
startBinderPool();
-
}
-
}).start();
-
}
-
-
private void startBinderPool() {
-
BinderPool binderPool = BinderPool.getInstance(this);
-
-
IBinder binder2 = binderPool.queryBinder(BinderPool.BINDER_2);
-
IMyAidl2Interface myAidl2Interface = IMyAidl2Interface.Stub.asInterface(binder2);
-
try {
-
String str1 = myAidl2Interface.print_A();
-
String str2 = myAidl2Interface.print_B();
-
Log.i("------", "Aidl2 Results:" + str1);
-
Log.i("------", "Aidl2 Results:" + str2);
-
} catch (RemoteException e) {
-
e.printStackTrace();
-
}
-
-
IBinder binder = binderPool.queryBinder(BinderPool.BINDER_1);
-
IMyAidlInterface myAidlInterface = IMyAidlInterface.Stub.asInterface(binder);
-
try {
-
int res = myAidlInterface.add(1, 3);
-
Log.i("------", "Aidl1 Calculation results:" + res);
-
} catch (RemoteException e) {
-
e.printStackTrace();
-
}
-
}
-
}
Step 4: Start the server, then start the client, view the Log, test results
-
08-25 00:13:04.025 3538-3566/com.handsome.app2 I/-----------: Aidl2 results: A
-
08-25 00:13:04.025 3538-3566/com.handsome.app2 I/-----------: Aidl2 Result: B
-
08-25 00:13:04.028 3538-3566/com.handsome.app2 I/-----------: Aidl1 results: 4