1. Objectives
- Learn to convert the pictures ['jpg', 'png', 'jpeg', 'bmp') in the current folder into base64 and save them to icon JS file;
- Learn to read image files and convert the files into base64 strings;
- Learn to get all the picture files in the folder;
- Learn to save the string of base64 into icon JS file.
2. Introduce dependent modules
import os import base64
3. Get all the files under the folder
- Read all file and folder names under the current given path
- Returns a list of all file and folder names
# Get all files in the folder def get_all_file(path): names = None try: names = os.listdir(path) except Exception as e: print(e) else: return names
4. Get the picture list in the file list
- Loop through the list of incoming files and folders
- Get file suffix name by cutting
- Judge whether the suffix is ['jpg', 'png', 'jpeg', 'bmp'] picture suffix
- Yes, save it to the picture list
- Returns a list of collected picture names
# Gets the list of pictures in the file list def get_all_images(files): images = [] try: for name in files: suffix = name.split('.').pop() if suffix in ['jpg', 'png', 'jpeg', 'bmp']: images.append(name) except Exception as e: print(e) else: return images
5. Get the list of base64
- Create and write icon Contents list of JS file
- Circular picture name list
- Convert each picture to a base64 string
- Store the key value pair consisting of the picture name and [base64 string] into the list
- Loop to complete the output of js file
- Return icon JS content list
# Get the list of base64 def get_images_base64(path, files): jss = [ "const icon = {\n" ] try: for name in files: suffix = name.split('.')[0] img_url = f'{path}/{name}' base_img = img_to_base64(img_url) jss.append(f' "{suffix}Icon": "{base_img}",\n') except Exception as e: print(e) else: jss.append("}\n") jss.append("module.exports = icon;") return jss
6. Create js file and write content
- Create the passed in js name
- Write content list to file
# Create js file and write content def create_js_folder(path, foldername, base_imgs): try: with open(path + "/" + foldername + ".js", "w",encoding="utf-8") as js: js.writelines(base_imgs) except Exception as e: print(e)
7. Complete code
#!/usr/bin/env python """ @Author : Rattenking @CSDN : https://blog.csdn.net/m0_38082783 """ import os import time import base64 # Convert pictures to base64 def img_to_base64(path): with open(path,"rb") as f: base64_data = base64.b64encode(f.read()) return f'data:image/jpg;base64,{base64_data.decode()}' # Gets the list of pictures in the file list def get_all_images(files): images = [] try: for name in files: suffix = name.split('.').pop() if suffix in ['jpg', 'png', 'jpeg', 'bmp']: images.append(name) except Exception as e: print(e) else: return images # Get all files in the folder def get_all_file(path): names = None try: names = os.listdir(path) except Exception as e: print(e) else: return names # Create js file and write content def create_js_folder(path, foldername, base_imgs): try: with open(path + "/" + foldername + ".js", "w",encoding="utf-8") as js: js.writelines(base_imgs) except Exception as e: print(e) # Get the list of base64 def get_images_base64(path, files): jss = [ "const icon = {\n" ] try: for name in files: suffix = name.split('.')[0] img_url = f'{path}/{name}' base_img = img_to_base64(img_url) jss.append(f' "{suffix}Icon": "{base_img}",\n') except Exception as e: print(e) else: jss.append("}\n") jss.append("module.exports = icon;") return jss if __name__ == "__main__": start_time = int(round(time.time() * 1000)) path = "./" # Get all files files = get_all_file(path) # Get all pictures images = get_all_images(files) # Convert picture list to base64 string icons = get_images_base64(path, images) # Create js file of icon create_js_folder(path, "icon", icons) end_time = int(round(time.time() * 1000)) print(f'The picture conversion time is:{end_time - start_time}ms')
8. Summary
- Due to the development of wechat applet, it is inconvenient to replace many small icons on the server. It is necessary to convert the icons to base64, but it is troublesome to convert them one by one[ Batch transfer pictures to base64 tool exe ]Tool, you can quickly turn the icon in the current folder into an icon JS file. In development, you only need to read the corresponding picture name in this file!
9. Use
Put the folder where the icon is stored into the conversion tool

Run the tool to generate icon js

Introduce icon in the usage page js
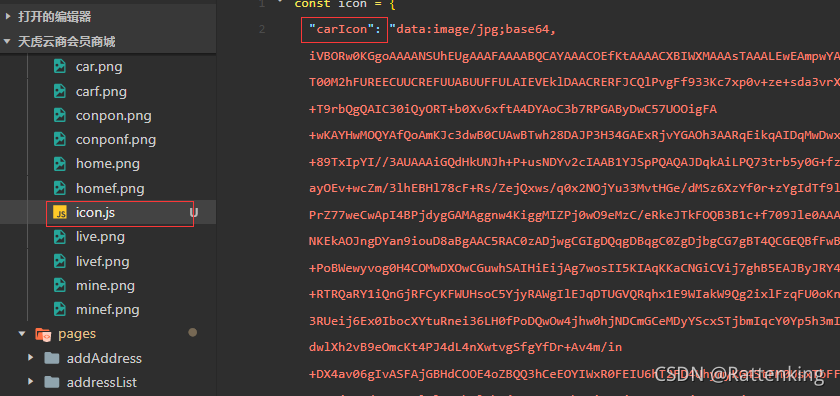
Note that the generated base64 image name is the suffix Icon after the original image name

Icon used in wxml interface
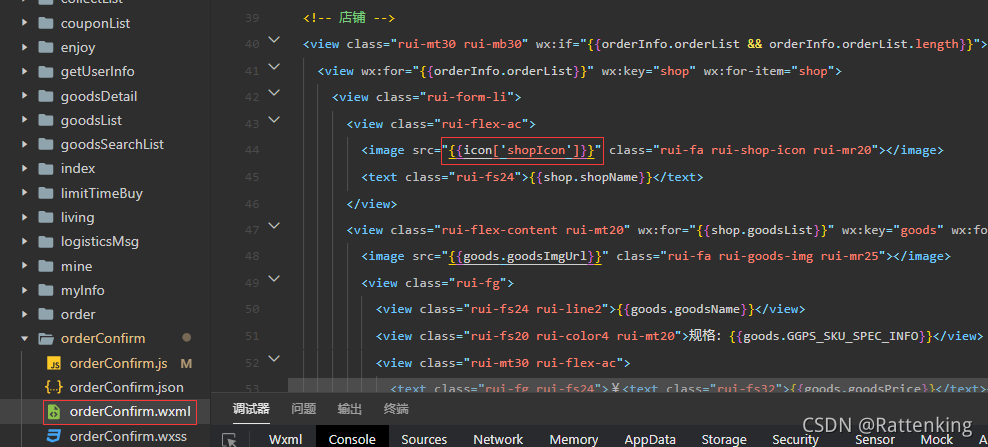
To update the icon, you only need to put the icon in the folder and re run the tool [it is convenient and fast, and there is no need to modify and replace the previous code]