A man of lofty ideals cherishes the short days while a man of sorrow knows the long nights. —— Jin Fu Xuan's Miscellaneous Poems
Study hard! We don't have much time left!
First fill in what I forgot to say yesterday. Yesterday's Insertion method Compared with Recursive method and In-situ reverse sequence method Neither extra space to save extra pointers nor recursive calls to functions are required, which is more efficient.
Here is today's topic. It's relatively simple.
Topic: Given the list head - > 1 - > 2 - > 3 - > 4 - > 5 - > 6 - > 7 - > 8, write the algorithm, output 8, 7, 6, 5, 4, 3, 2, 1; but can not change the list structure. That is, after the output list, the list is still head - > 1 - > 2 - > 3 - > 4 - > 5 - > 6 - > 7 - > 8.
Today's topic is not the reverse list, but the reverse output. If we don't consider the following requirements, it's easy to think that if we first reverse the list and then output it in sequence, then the output will be reverse. There are three methods of reverse linked list, each of which has its advantages and disadvantages. So how do we output the list in reverse order without changing its structure? The method given below is recursion.
Recursion has already been mentioned before. Today I'll review it and solve this problem by the way. We say that recursive programs have two elements: recursive definition and recursive termination conditions. First of all, to output the reverse order of head - > 1 - > 2 - > 3 - > 4 - > 5 - > 6 - > 7 - > 8, we need to output the reverse order of 2 - > 3 - > 4 - > 5 - > 6 - > 7 - > 8 first, and then output the first node. But to output the reverse order of 2 - > 3 - > 4 - > 5 - > 6 - > 7 - > 8, we need to output the reverse order of 3 - > 4 - > 5 - > 6 - > 7 - > 8 first, and then output 2; similarly, until the last element 8, the direct output is the reverse order. Then output 7, 6, 5... recursively. This implements reverse output, and we haven't changed either the next domain or the data domain of any node, nor the linked list structure. After the above analysis: recursion is defined as: first output the reverse order of the sub-list except the current node, then output the current node; recursive termination condition is: when only one element in the list or the list is empty. You may not feel the change in the list structure. The following is implemented in code. Let's see if we can meet the requirements of the topic.
First construct the list, as I said before.—— python algorithm-002 single linked list reverse recursion method There are explanations:
#First construct node objects class LNode: """ //Define linked list objects """ def __init__(self, x): self.data = x self.next = None #Constructing linked list function def creatLink(x): i = 1 head = LNode(None) tmp = None cur = head while i <= x: tmp = LNode(i) cur.next = tmp cur = tmp i += 1
Here we begin to implement today's algorithm:
def ReversePrint(head): """ //Inverse output without changing the list structure """ #First determine whether the list is empty or not, then return directly if head.next is None or head is None: return firstNode=head.next # Used to traverse linked lists, but also the first node of the sub-linked list ReversePrint(firstNode) # Recursive Call Sublist print(firstNode.data) # Output the data of the current traversal node return head # Used to judge whether the structure of linked list has changed or not
This is the code to implement the algorithm, much shorter than yesterday, I believe it is easy to understand! Let's see how it works.
Main program:
if __name__ == '__main__': head=creadLink(8) #Let's look at the structure of the linked list first. print("BeforeReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next #Inverse Output Link List print("ReversePrint:") head = ReversePrint(head) #Then see if the reverse list has changed. print("BeforeReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next
The output of the program is as follows:
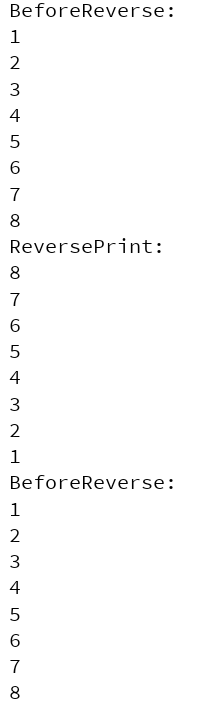
All code:
#First construct node objects class LNode: """ //Define linked list objects """ def __init__(self, x): self.data = x self.next = None #Constructing linked list function def creatLink(x): i = 1 head = LNode(None) tmp = None cur = head while i <= x: tmp = LNode(i) cur.next = tmp cur = tmp i += 1 return head def ReversePrint(head): """ //Inverse output without changing the list structure """ #First determine whether the list is empty or not, then return directly if head.next is None or head is None: return firstNode=head.next # Used to traverse linked lists, but also the first node of the sub-linked list ReversePrint(firstNode) # Recursive Call Sublist print(firstNode.data) # Output the data of the current traversal node return head # Used to judge whether the structure of linked list has changed or not if __name__ == '__main__': head=creatLink(8) #Let's look at the structure of the linked list first. print("BeforeReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next #Inverse Output Link List print("ReversePrint:") head = ReversePrint(head) #Then see if the reverse list has changed. print("BeforeReverse:") cur = head.next while cur != None: print(cur.data) cur = cur.next
Okay, that's all for today's algorithm. I'd like to apologize to you first. The thing is this: when I write an article, I wrote the code by myself, but the comments are not as many as they are now, and some details I have not noticed need to be changed, so I will add some things in the analysis, inevitably there will be multiple blanks, and write notes in another line. Here comes the desperate place! In Python, indentation is used to divide code blocks, which looks beautiful and in fact beautiful, but it is absolutely impossible to mix space and Tab keys, so you must pay attention to only one kind of code!!! But I haven't noticed this in the previous articles. Today's direct code replication should work (but I don't recommend it). This leads to the students can not copy directly run, but also report some strange errors... Sorry, I was wrong! At the same time, it is not recommended that you copy it directly. Writing by yourself will find many interesting things. I won't change the first few articles, leave them for you to change slowly. But this will not happen in future articles.
One more thing, in my github (Web site is English, mobile phone endpoint viewcode can be seen, the computer can directly see), the above is my own direct operation of the code, the title is the corresponding, but the code may be slightly different. The above topics are more, but there is no such detailed explanation. If you want to learn, you can study them by yourself.
Mo Tao's slanders are like deep waves, Mo Yan's relocation of visitors is like sand.
Thousands of clean-ups, though hard, blow all the sand to gold.
—— Liu Yuxi's "Waves and Sands"
Come on.