introduction
Yesterday, I finished process control and talked about some of the simplest built-in methods of basic data types. Let's continue with the built-in method today. There are many built-in methods. Don't be afraid. As long as you use more, you will be able to write it when you think of it.
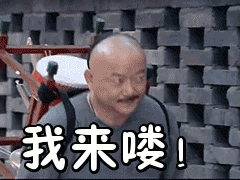
String built-in method
Remove leading and trailing unilateral characters
If we want to remove the first and last characters, we will consider strip(), but when we just remove the characters on one side. rstrip() can remove only the tail characters, and lstrip() can remove only the head characters.
text = '**abcd**' # Removes the specified symbol from the tail* print(text.rstrip('*')) # '**abcd' # Remove the specified symbol of the head* print(text.lstrip('*')) # 'abcd**'
Case change
Older people who surf the Internet should know that the previous verification codes were case sensitive, but now they are not needed. This is due to the case of the built-in method. lower() is to lowercase all English characters in the string, and upper() is to uppercase all English characters in the string. islower() determines whether a line of string is lowercase, and isupper() determines whether a line of string is uppercase. The results of these two built-in methods are Boolean.
text = 'kinGnie' # Make all strings lowercase text.lower() # 'kingnie' # Make all strings uppercase text.upper() # 'KINGNIE' # Determine whether all strings are lowercase text.islower() # False # Determine whether the string is capitalized text.isupper() # False
Determine the beginning and end of the string
Startswitch () is used to judge whether the first character of the string is the specified character, and endswitch () is used to judge whether the tail of the string is the specified character.
text = 'asdlkgjd' # Judge first character text.startswith('a') # True text.startswith('ab') # False # Judge trailing character text.endswith('d') # True text.endswith('jd') # True
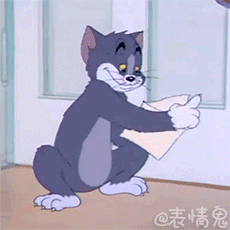
Format output
In the previous issue, we talked about a way to format output -- placeholders. However, in the early years, the python development team has proposed to eliminate the placeholder% s. Today, let's introduce some other methods of formatting output, mainly format.
# Use {} for placeholders print('I this year{}Years old, my name is{}. '.format(18, 'Jin Yemin')) # I am 18 years old. My name is Jin Yemin. # Indexed placeholder print('I this year{0}Years old, my name is{1},{0}I'm strong at the age of.'.format(18, 'Jin Yemin')) # I am 18 years old. My name is Jin Yemin. # With variable name print('I this year{age}Years old, my name is{name},{age}I'm strong at the age of.'.format(age = 18, name = 'Jin Yemin')) # I am 18 years old. My name is Jin Yemin. # Variable outside type age = 18 name = 'Jin Yemin' print(f'I this year age Years old, my name is name,age I'm strong at the age of.')
Splice string
The plus sign can splice two strings, * copy many strings and splice them. Join loops the strings with the for statement and splices them with the specified symbol. Remember that the element spliced by the join must be a string, and the numeric type will report an error
# Low splicing efficiency of plus sign print('dsa' + 'dsa') # 'dsadsa # Copy with asterisk print('dsa' * 5) # dsadsadsadsadsa # Circular splicing of strings using join print('|'.join('dsadsa')) # d|s|a|d|s|a
Replace the specified character in the string
replace() is used to replace characters. The first parameter is the character to be replaced, the second parameter is the character to be replaced, and the third character is to replace several characters.
# Specified number of replacements print('fa fa fa lidasljaljkljaksljd'.replace('fa', 'mo', 2)) # mo mo fa lidasljaljkljaksljd # replace all print('fa fa fa lidasljaljkljaksljd'.replace('fa', 'mo')) # mo mo mo lidasljaljkljaksljd
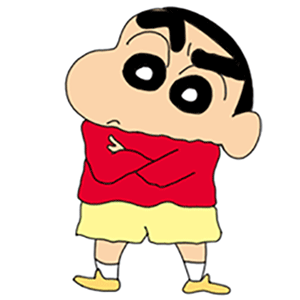
Judge whether the string is a pure number
# isdigit() text = '4646465456' print(text.isdigit(text)) # True text1 = 'sadasd465465' print(text.isdigit(text)) # False
Finds the corresponding index value of the specified character
The built-in method content of the string from here is used infrequently.
# find method s1 = 'king wolf kin zinz' print(s1.find('f')) # 8 # find can also use the index value to set a search range. print(s1.find('f', 1, 8)) # -1 return - 1 if not found #index method s1 = 'king wolf kin zinz' print(s1.index('f')) # 8. If the index method is not found, an error will be reported
Text position change
# Center center s1 = 'king' print(s1.center(10,'-')) # '--- king ---' centers the string, and the insufficient number is supplemented with the specified symbol # ljust left align s1 = 'king' print(s1.ljust(10, '-')) # 'king ------' left align the string, and the insufficient number is supplemented with the specified symbol # Align right s1 = 'king' print(s1.rjust(10, '-')) # '------- king' right aligns the string, and the insufficient number is supplemented with the specified symbol # zfill right aligned, if not enough, use 0 to supplement s1 = 'king' print(s1.zfill(10)) # '000000king' right aligns the string, and the insufficient number is supplemented with the specified symbol
Escape character
Backslash plus some English letters are escape characters, which are of special use
# String with escape character print('dlsjdal\ndadsasasd') ''' 'dlsjdal dadsasasd' ''' # Cancel escape print(r'dlsjdal\ndadsasasd') # 'dlsjdal\ndadsasasd'
Case of letters
# title case message = 'dasdsa' print(message.capitalize()) # Dasdsa # Flip Case of letters message = 'DasDsadA' print(message.swapcase()) # dASdSADa # Capitalize each word message = 'my dear friend is king' print(message.title()) # My Dear Friend Is King
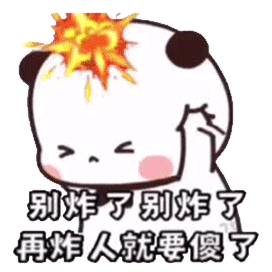
List built-in method
Type conversion
List type conversion is basically used for data types that can be used for loops, such as strings, tuples, sets, and dictionaries.
num_dict = {'name': 'king', 'age': 18} print(list(num_dict)) # ['name ','age'], as the old saying goes, the value in the key value pair can only be obtained through the key num_set = {1, 2, 3, 4} print(list(num_set)) # [1, 2, 3, 4] num_tuple = (1, 3, 4, 6) print(list(num_tuple)) # [1, 3, 4, 6] num_str = 'sdads' print(list(num_str)) # ['s', 'd', 'a', 'd', 's']
basic operation
The index value, slice, statistical number and member operation of the list are no different from those of the string type, except that the list takes the element as the individual and the string takes the character as the individual.
num_list = ['king', 'tom', 'job', 'kim', 'jason'] # Index value num_list[0] # 'king' # section num_list[0:3] # ['king', 'tom', 'job'] num_list[0:4] # ['king', 'job'] # Number of statistical elements len(num_list) # 5 # member operator ls = 'king' print(ls in num_list) # True
Add element to list
Add 'single' element to the tail
# No matter what form the object in the append bracket is, entering the list is only one element num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list.append('min') print(num_list) # ['king', 'tom', 'job', 'kim', 'jason', 'min'] num_list.append([1, 2, 4, 8]) print(num_list) # ['king', 'tom', 'job', 'kim', 'jason', 'min', [1, 2, 4, 8]]
Insert a single 'element' at the specified location
''' insert Is to insert the specified index value, and the elements at and after the original index value are moved back. insert Enter the index value for the first parameter and the insert object for the second parameter. ''' num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list.insert(0,'min') print(num_list) # ['min', 'king', 'tom', 'job', 'kim', 'jason']
Merge list
The plus sign can be used to merge the list, but it is inefficient and takes up too much space. The plus sign is to open up a new memory space and put the combined values in the new memory space. Extend is to modify the original memory space. Of course, like extend, + = is appended to the original list.
num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list.extend([1, 2, 3, 4]) # ['king', 'tom', 'job', 'kim', 'jason', 1, 2, 3, 4]
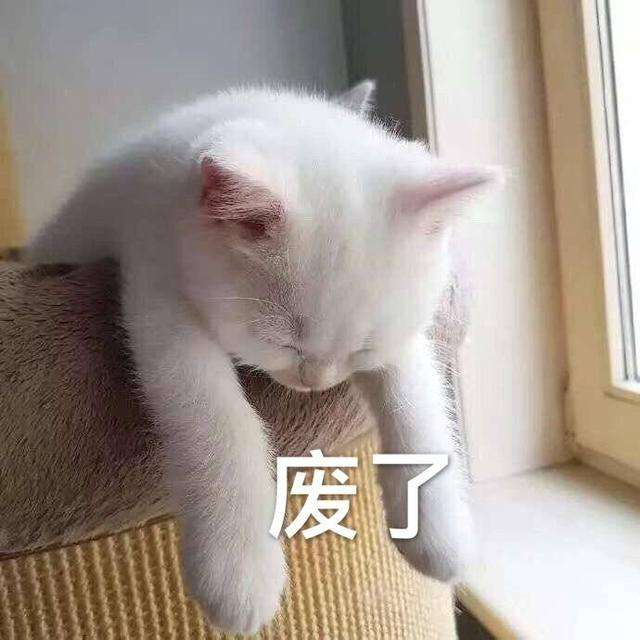
Delete from list
General deletion
num_list = ['king', 'tom', 'job', 'kim', 'jason'] del num_list[0] print(num_list) # ['tom', 'job', 'kim', 'jason']
Indicates that the Taoist surname is deleted
num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list.remove('king') print(num_list) # ['tom', 'job', 'kim', 'jason']
Delayed deletion
# pop deferred deletion can also be regarded as taking it out of the list and using it alone num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list.pop() # 'jason' defaults to moving the last one out num_list.pop(0) # 'king' can also use the index to move out the specified element
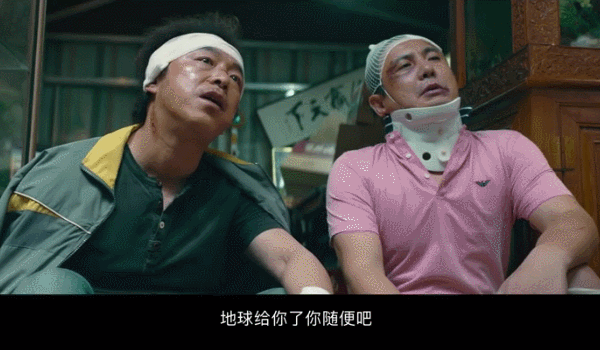
Modify list elements
# Rebind variable values directly to the index num_list = ['king', 'tom', 'job', 'kim', 'jason'] num_list[0] = 'yes' print(num_list) # ['yes', 'tom', 'job', 'kim', 'jason']
sort
num_list = [44, 55, 77, 88, 11] num_list.sort() print(num_list) # [11, 44, 55, 77, 88] default from small to large num_list.sort(reverse=True) print(num_list) # [88, 77, 55, 44, 11] reverse means to flip from large to small
Flip
num_list = [44, 55, 77, 88, 11] num_list.reverse() print(num_list) # [11, 88, 77, 55, 44]
Comparison operation
# List comparison mainly refers to the comparison of corresponding indexes. num_list = [44, 55, 77, 88, 11] num_lstwo = [1, 2, 4] print(num_list > num_lstwo) # True
Counts the number of occurrences of elements in the list
ls1 = [11, 22, 66, 11, 11, 33, 77, 11, 11] print(ls.count(11)) # 5
clear list
ls1 = [11, 22, 66, 11, 11, 33, 77, 11, 11] ls1.clear() print(ls1) # []
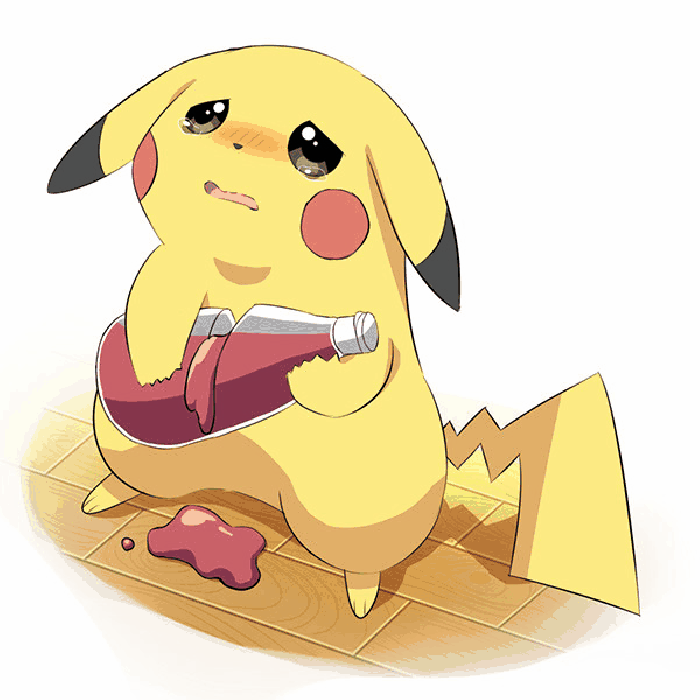
Variable type and immutable type
The variable type mainly changes the value and the address remains unchanged. The immutable type mainly changes the value and the address. Take the list as an example. When the list is of variable type, the list points to the index value in the memory space. When the elements in the list change, the variable value pointed to by the index value changes, and the list still points to the memory space where the index value is located, while the variable names of these immutable types directly point to the variable value. When the variable value changes, Only the memory address pointed to by the variable name has changed.
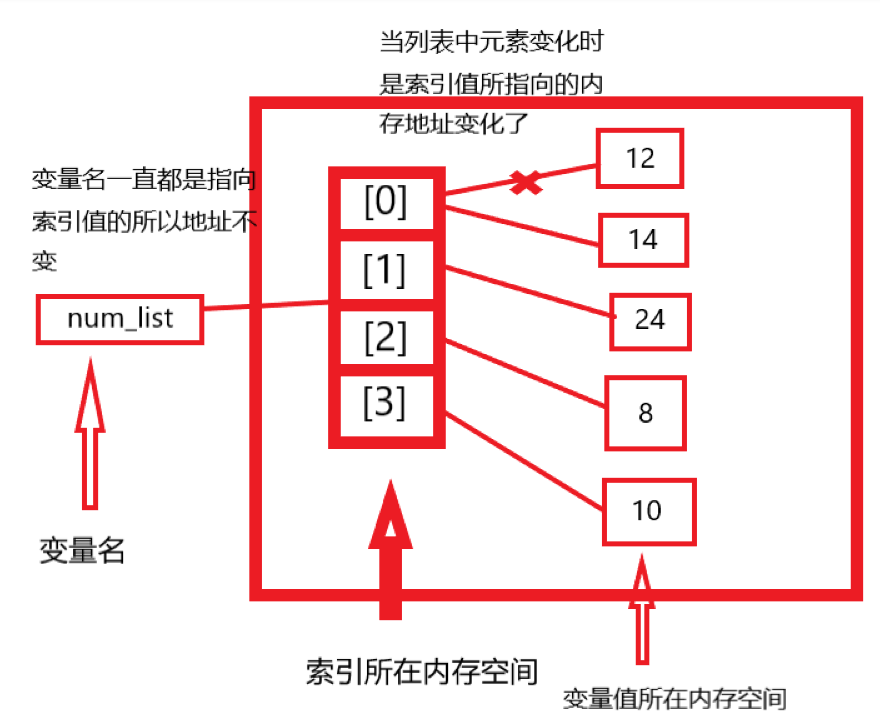
The case of immutable types is the case of index values and variable values.
Queue and stack
Queue is first in first out, which is similar to queuing to buy things. When you arrive first, you buy good things first and go first. The stack is similar to potato chips. The first potato chips will be taken out‘
# Queue similar ls1 = [11, 22, 66, 11, 11, 33, 77, 11, 11] ls2 = [] for i in ls1: ls2.append(i) while len(ls1) > 1: ls2.pop(0) # Stack similar ls1 = [11, 22, 66, 11, 11, 33, 77, 11, 11] ls2 = [] for i in ls1: ls2.append(i) while len(ls1) > 1: ls2.pop()
summary
Guys, today's content is mainly backrest and multi-use. It's not very difficult. Come on!
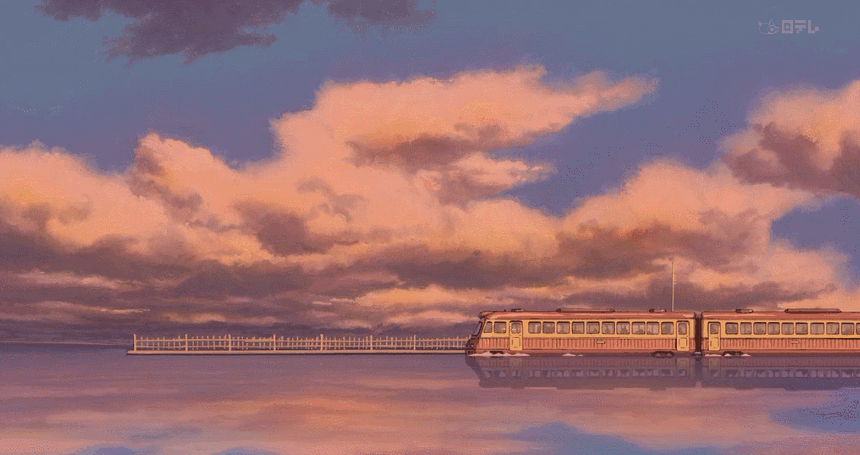