---Resume content start---
Remembering common Python techniques can help improve code design, reduce errors, and save time.
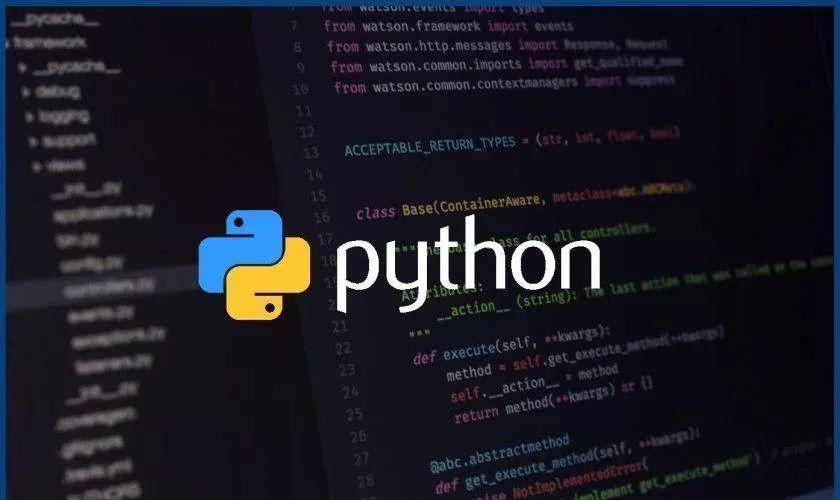
Python is an interpretive language, and its readability and ease of use make it more and more popular. As mentioned in the Zen of Python:
Beauty is better than ugliness, clarity is better than obscurity.
In your daily coding, the following tips can bring you unexpected results.
1. String inversion
The following code snippet uses slicing operation in Python to realize string inversion:
# Reversing a string using slicing
my_string = "ABCDE"
reversed_string = my_string[::-1]
print(reversed_string)
# Output
# EDCBA
2. Initial capital
In the following code snippet, you can capitalize a String by using the title() method of the String class:
my_string = "my name is chaitanya baweja"
# using the title() function of string class
new_string = my_string.title()
print(new_string)
# Output
# My Name Is Chaitanya Baweja
3. Take the elements of the string
The following code snippet can be used to find out all the elements that make up a string. We use the feature that only non repeated elements can be stored in the set:
my_string = "aavvccccddddeee"
# converting the string to a set
temp_set = set(my_string)
# stitching set into a string using join
new_string = ''.join(temp_set)
print(new_string)
# Output
# acedv
4. Output String/List repeatedly
String/List can be multiplied. In this method, they can be used for any multiplication.
n = 3 # number of repetitions
my_string = "abcd"
my_list = [1,2,3]
print(my_string*n)
# abcdabcdabcd
print(my_string*n)
# [1,2,3,1,2,3,1,2,3]
There is an interesting use to define a list of n constants:
n = 4
my_list = [0]*n # n indicates the length of the desired list
# [0, 0, 0, 0]
5. List derivation
List derivation provides a more elegant way to work with lists. In the following code snippet, multiply the elements in the old list by 2 to create a new list:
original_list = [1,2,3,4]
new_list = [2*x for x in original_list]
print(new_list)
# [2,4,6,8]
6. Exchange two variable values
Python does not need to create an intermediate variable to exchange the values of two variables. It can be easily implemented:
a = 1
b = 2
a, b = b, a
print(a) # 2
print(b) # 1
7. String splitting
You can use the split() method to split a string into multiple substrings, or you can pass the separator as a parameter to split it.
string_1 = "My name is Chaitanya Baweja"
string_2 = "sample/ string 2"
# default separator ' '
print(string_1.split())
# ['My', 'name', 'is', 'Chaitanya', 'Baweja']
# defining separator as '/'
print(string_2.split('/'))
# ['sample', ' string 2']
8. String splicing
The join() method can combine a string list into a string. In the following code snippet, I use it to splice all strings together:
list_of_strings = ['My', 'name', 'is', 'Chaitanya', 'Baweja']
# Using join with the comma separator
print(','.join(list_of_strings))
# Output
# My,name,is,Chaitanya,Baweja
9. Palindrome detection
Previously, we have said how to flip a string, so palindrome detection is very simple:
my_string = "abcba"
if my_string == my_string[::-1]:
print("palindrome")
else:
print("not palindrome")
# Output
# palindrome
10. Element repetition times
There are many ways to do this in Python, but my favorite is counter. Counter calculates the number of times each element appears. Counter() returns a dictionary, with the element as the key and the number of times it appears as the value. We can also use the most common () method to get the element with the most words.
from collections import Counter
my_list = ['a','a','b','b','b','c','d','d','d','d','d']
count = Counter(my_list) # defining a counter object
print(count) # Of all elements
# Counter({'d': 5, 'b': 3, 'a': 2, 'c': 1})
print(count['b']) # of individual element
# 3
print(count.most_common(1)) # most frequent element
# [('d', 5)]
11. Use of modifier
An interesting use of Counter is to find a modifier: a new word formed by changing the position (order) of the letters of a word or sentence. If two objects obtained by using Counter are equal, they are modifiers:
from collections import Counter
str_1, str_2, str_3 = "acbde", "abced", "abcda"
cnt_1, cnt_2, cnt_3 = Counter(str_1), Counter(str_2), Counter(str_3)
if cnt_1 == cnt_2:
print('1 and 2 anagram')
if cnt_1 == cnt_3:
print('1 and 3 anagram')
12,try-except-else
In Python, try except is used for exception capture. else can be used to execute when no exception occurs. If you need to execute some code, whether or not an exception has occurred, use final:
a, b = 1,0
try:
print(a/b)
# exception raised when b is 0
except ZeroDivisionError:
print("division by zero")
else:
print("no exceptions raised")
finally:
print("Run this always")
13. Enumeration traversal
In the following code snippet, traverse the values and corresponding indexes in the list:
my_list = ['a', 'b', 'c', 'd', 'e']
for index, value in enumerate(my_list):
print('{0}: {1}'.format(index, value))
# 0: a
# 1: b
# 2: c
# 3: d
# 4: e
14. Memory size of objects
The following code snippet shows how to get the memory size occupied by an object:
import sys
num = 21
print(sys.getsizeof(num))
# In Python 2, 24
# In Python 3, 28
15. Merge two dictionaries
In Python 2, use the update() method to merge. In Python 3.5, it's simpler. In the following code snippet, merge two dictionaries. When there is an intersection between the two dictionaries, use the latter to overwrite.
dict_1 = {'apple': 9, 'banana': 6}
dict_2 = {'banana': 4, 'orange': 8}
combined_dict = {**dict_1, **dict_2}
print(combined_dict)
# Output
# {'apple': 9, 'banana': 4, 'orange': 8}
16. Code execution time
In the following code snippet, time is used to calculate the execution time of the code:
import time
start_time = time.time()
# Code to check follows
a, b = 1,2
c = a+ b
# Code to check ends
end_time = time.time()
time_taken_in_micro = (end_time- start_time)*(10**6)
print(" Time taken in micro_seconds: {0} ms").format(time_taken_in_micro)
17. List expansion
Sometimes you don't know the nesting depth of your current list, but you want to expand them into a one-dimensional list. Here's how to do it:
from iteration_utilities import deepflatten
# if you only have one depth nested_list, use this
def flatten(l):
return [item for sublist in l for item in sublist]
l = [[1,2,3],[3]]
print(flatten(l))
# [1, 2, 3, 3]
# if you don't know how deep the list is nested
l = [[1,2,3],[4,[5],[6,7]],[8,[9,[10]]]]
print(list(deepflatten(l, depth=3)))
# [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Numpy flatten can better handle your formatted data.
18. Random sampling
In the following example, random sampling from a list is implemented using the random library.
import random
my_list = ['a', 'b', 'c', 'd', 'e']
num_samples = 2
samples = random.sample(my_list,num_samples)
print(samples)
Random sampling, I recommend using the "secrets" library to achieve more security. The following code snippet can only be run in Python 3:
import secrets # imports secure module.
secure_random = secrets.SystemRandom() # creates a secure random object.
my_list = ['a','b','c','d','e']
num_samples = 2
samples = secure_random.sample(my_list, num_samples)
print(samples)
#You can add this group 631441315 to communicate with each other. There are a lot of PDF books and tutorials in the group for free use! No matter which stage you have learned, you can get the corresponding tutorial!
19. Digitalization
The following code converts an integer number into a digitized object:
num = 123456
list_of_digits = list(map(int, str(num)))
print(list_of_digits)
# [1, 2, 3, 4, 5, 6]
20. Uniqueness check
The following code example checks whether the elements in the list are not duplicate:
def unique(l):
if len(l)==len(set(l)):
print("All elements are unique")
else:
print("List has duplicates")
unique([1,2,3,4])
# All elements are unique
unique([1,1,2,3])
# List has duplicates
21, summary
These are very useful codes that I find in my daily work. Thank you very much for reading this article. I hope it will help you.
---End of recovery content---