Python - First Identity Object Oriented
Posted by Spinicrus on Sat, 15 Feb 2020 20:32:30 +0100
Wedge
Now you are a developer of a game company. Now you need to develop a game called Dog and Human Warfare. Just think, Dog and Human Warfare requires at least two roles. One is human and the other is dog. People and dogs have different skills, such as people fighting with sticks and dogs. Dogs can bite people. How can you describe these different roles and their functions?
You have searched through all the skills you have acquired and written the following code to describe the two roles
def person(name,age,sex,job):
data = {
'name':name,
'age':age,
'sex':sex,
'job':job
}
return data
def dog(name,dog_type):
data = {
'name':name,
'type':dog_type
}
return data
The above two methods are equivalent to creating two models in which each person and each dog in the game have the same attributes.At the beginning of the game, how do you create a specific person or dog based on the specific information passed in by a person or a dog?
d1 = dog("Li Lei","Pekingese")
p1 = person("Strictly handsome",36,"F","Operation and maintenance")
p2 = person("egon",27,"F","Teacher")
Two character objects have been generated. Dogs and people have different functions. Dogs bite people and people beat them, right? How can we do that?Think, you can write another function for each function, which function you want to execute, call it directly, right?
def bark(d):
print("dog %s:wang.wang..wang..."%d['name'])
def walk(p):
print("person %s is walking..." %p['name'])<br><br>
walk(p1)
bark(d1)
The above functions are simply perfect!
But after a while of careful play, you accidentally did the following
p1 = person("Strictly handsome",36,"F","Operation and maintenance")
bark(p1) #The way to pass a person's object to a dog
In fact, there's nothing wrong with the code you wrote.Obviously, people can't invoke the functions of a dog, but there are no restrictions in your routine. How do you achieve this restriction at the code level?
def person(name,age,sex,job):
def walk(p):
print("person %s is walking..." % p['name'])
data = {
'name':name,
'age':age,
'sex':sex,
'job':job,
'walk':walk
}
return data
def dog(name,dog_type):
def bark(d):
print("dog %s:wang.wang..wang..."%d['name'])
data = {
'name':name,
'type':dog_type,
'bark':bark
}
return data
Restrict Functionality New Code

d1 = dog("Li Lei","Pekingese")
p1 = person("Strictly handsome",36,"F","Operation and maintenance")
p2 = person("egon",27,"F","Teacher")
Produce specific people and Wang

d1['bark'](p1) #Ways to Pass People to Dogs
Unable to call
You're so smart that you're able to restrict people from using themselves.
The programming idea you just used is really simple object-oriented programming. After we created two models to represent all the people and dogs in the game, the barking or walking of the rest of the dog is not important for both models.The interaction between the specific person and the dog is waiting for you to use it.If you fight with a dog, it's up to you to walk or stick your dog.Then every decision you make may have an impact on your game.This is also uncertain.It's not the same thing that we've done before when we've written code and gone on track.
Nevertheless, we have only finished a very small part of the game.Many other functions are not implemented.
Just now you just prevented two completely different roles from mixing functions. But is it possible that the same role has different attributes?For example, everyone has beaten cs. There are police and terrorists in cs, but because they are all people, you write a role called person(). Both police and terrorists can shoot each other, but the police can't kill hostages. Terrors can. How can this be achieved?Just think about it. Simple. Just add a judgment to the function of hostage killing. If it's a policeman, don't let it be ok ay.Yes, it solves the problem of hostage killing, but you will find that there are many differences and similarities between the police and the terrorists. If you make a separate judgement in each of these differences, you will be dead.(
When you think about it, just write two roles. Anyway, there are so many differences. My brother can't write two roles because they have a lot of commonalities. Writing two different roles means that the same function should be rewritten, isn't it my brother?.
Well, the topic will be here for you, and you can't understand your IQ any more!
Process-Oriented VS Object-Oriented
The core of process-oriented programming is process (pipelining thinking). Process is the step to solve a problem. Process-oriented programming is like carefully designing a pipeline to consider when and what to do with it.
The advantage is that it greatly reduces the complexity of writing programs by simply following the steps to be executed and stacking the code.
The disadvantage is that a set of pipelining or processes is used to solve a problem, and the code is all in one go.
Scenarios: Once you have completed a scenario that rarely changes, well-known examples include the Linux kernel, git, and Apache HTTP Server.
The core of object-oriented programming is object (God-like thinking). To understand what an object is, you must treat yourself as God. In the eyes of God, everything that exists in the world is an object, and what does not exist can be created.Object-oriented programming is like designing a travel story to the west. The problem to be solved in the past is to pass the classics to Dongtu Datang. It requires four people to think about solving this problem: Tang Monk, Sha Monk, Pig Bajie, Sun Wukong. Everyone has their own characteristics and skills (this is the concept of the object, the attributes and methods of the features and skills of the object respectively). However, this*It was not fun, so a group of ghosts and monsters were arranged. In order to prevent the four Masters and Apprentices from being killed on the way to take the route, a group of fairies were arranged to escort them. These were all objects.Then the Sutra begins, and the four masters and apprentices fight with the ghosts and gods until they finally get the sutra.The Tathagata does not care at all what process the four Masters and Apprentices follow.
Object-oriented programming
The advantage is that it solves the extensibility of the program.Individual modifications to an object are immediately reflected in the system as it is easy to modify the characteristics and skills of a character parameter in a game.
Disadvantages: poor controllability prevents pipelining of process-oriented programming to accurately predict the process and results of a problem. Object-oriented programs begin by interacting with objects to solve problems, and even God cannot predict the end result.As a result, we often see that a gamer's modification of a certain parameter is likely to result in the appearance of bullying skills. Cutting down three people with one knife will cause the game to lose its balance.
Application Scenario: Software with constantly changing needs, changes in general needs are concentrated in the user layer, Internet applications, internal enterprise software, games, etc. are all good places for object-oriented programming to show itself.
Object-oriented programming is not all in python.
Object-oriented programming can make the maintenance and extension of programs simpler, and greatly improve the efficiency of program development. In addition, object-oriented programs can make your code logic easier for others to understand, and make team development easier.
Learn about some nouns: class, object, instance, instantiation
Class: A class of things with the same characteristics (people, dogs, tigers)
Object/instance: a specific thing (next door flowers, downstairs prosperity)
Instantiation: Class ->Object process (this is not obvious in life, let's explain it later)
Initial Classes and Objects
Everything in python is an object, and types are essentially classes, so believe it or not, you've used classes for a long time
>>> dict #type dict Is Class dict
<class 'dict'>
>>> d=dict(name='eva') #instantiation
>>> d.pop('name') #towards d Send a message to execute d Method pop
'eva'
From the example above, a dictionary is a kind of data structure. When I say a dictionary, you know what is represented by {} with k-v key-value pairs. It also has some ways of adding, deleting, and altering.But once I say the dictionary, do you know what's in it?No, so we say that for a class, it has the same characteristic attributes and methods.
The specific {'name':'eva'} dictionary is a dictionary, it can use all the methods of the dictionary, and it has specific values, it is an object of the dictionary.An object is a specific individual that already exists.
Another example is popular. For example, you have a zoo now and you want to describe it. Every animal in the zoo is a class: tigers, swans, crocodiles, bears.They all have the same attributes, such as height, weight, birth time and species, and different movements, such as crocodiles swimming, swans flying, tigers running, bears eating.
But these tiger bears are not specific animals, they are a class of animals.Although they all have height and weight, you cannot determine how much this value is.If you are given a specific tiger at this time and you are not dead, then you can measure him and weigh him. Are these values specific?Then the specific tiger is a specific instance and an object.More than this one, in fact, each specific tiger has its own height and weight, so each tiger is a tiger object.
In python, features are represented by variables and skills are represented by functions. Therefore, a category of things with the same characteristics and skills is a'class', and an object is a specific one of these things.
Knowledge about classes
Initial Knowledge Class
statement
def functionName(args):
'Function Document String'
Function Body
'''
Class class name:
'Document string of class'
Class Body
'''
#Let's create a class
class Data:
pass
class Person: #Define a human
role = 'person' #The Role of ManattributeAll people
def walk(self): #Everyone can walk, that is, there is a walkMethod, also known as dynamic properties
print("person is walking...")
Classes serve two purposes: attribute reference and instantiation
Attribute references (class names. attributes)
class Person: #Define a human
role = 'person' #People's role attributes are people
def walk(self): #Everyone can walk, that is, there is a way to walk
print("person is walking...")
print(Person.role) #Viewer's role attribute
print(Person.walk) #Referencer's Walking Method, note that this is not a call
instantiation
A bracketed class name is an instantiation that automatically triggers the operation of the u init_u function, which can be used to customize its own characteristics for each instance
class Person: #Define a human
role = 'person' #People's role attributes are people
def __init__(self,name):
self.name = name # Each character has its own nickname;
def walk(self): #Everyone can walk, that is, there is a way to walk
print("person is walking...")
print(Person.role) #Viewer's role attribute
print(Person.walk) #Referencer's Walking Method, note that this is not a call
The process of instantiation is the process of class->object
Originally, we only had one Person class. In this process, we generated an egg object with its specific name, attack power and life value.
Syntax: object name = class name (parameter)
egg = Person('egon') #Class name()Is equivalent to execution Person.__init__()
#Execution complete__init__()An object is returned.This object resembles a dictionary with properties and methods that belong to this person.
View Properties & Call Methods
print(egg.name) #View Property Direct Object Name.Property Name
print(egg.walk()) #Call method, object name.Method Name()
About self
self: The first parameter that automatically passes the object/instance itself to u init_ during instantiation, and you can give it an individual name, but normal people don't.
Because you make a fool of others and you don't know it
Supplementary Class Properties
One: Where exactly are the properties of the classes we define stored?There are two ways to view
Dir (class name): a list of names is found
Class name. u dict_u: A dictionary was found with key as attribute name and value as attribute value
Two: Special class attributes
Class name. u name_u#Class name (string)
Class name. u doc_u#Document string for class
Class name. u base_u#First parent of class (when talking about inheritance)
Class name. u bases_u#Tuples of all the parent classes of the class (when talking about inheritance)
Class name. u dict_u#Dictionary properties of the class
Class name. u module_u#Module where the class definition is located
Class name. u class_u#Instance corresponding class (only in newer classes)
Knowledge about objects
Back to our dog fight: now we need to make a little change to our kind
In addition to walking, humans should have some attacking skills.
class Person: # Define a human
role = 'person' # People's role attributes are people
def __init__(self, name, aggressivity, life_value):
self.name = name # Each character has its own nickname;
self.aggressivity = aggressivity # Each character has its own attack power;
self.life_value = life_value # Each role has its own life value;
def attack(self,dog):
# People can attack a dog, and the dog here is also an object.
# If a person attacks a dog, its life value will decrease according to the person's aggression.
dog.life_value -= self.aggressivity
An object is an example of a class that actually exists, that is, an instance
Objects/instances serve only one purpose: attribute references
egg = Person('egon',10,1000)
print(egg.name)
print(egg.aggressivity)
print(egg.life_value)
Of course, you can also refer to a method, because a method is also an attribute, just a function-like attribute, which we also call dynamic attributes.
Referencing dynamic properties does not execute this method; to call the same method as calling a function, parentheses are required after it
print(egg.attack)
I know there are a lot of things you might not understand in classes.Let's use functions to explain this kind of thing. What's the object? Just use this understanding secretly. Don't tell others
def Person(*args,**kwargs):
self = {}
def attack(self,dog):
dog['life_value'] -= self['aggressivity']
def __init__(name,aggressivity,life_value):
self['name'] = name
self['aggressivity'] = aggressivity
self['life_value'] = life_value
self['attack'] = attack
__init__(*args,**kwargs)
return self
egg = Person('egon',78,10)
print(egg['name'])
Object-Oriented Summary - Fixed Mode of Definition and Call
Class class name:
Def u init_u (self, parameter 1, parameter 2):
Property 1 of self.object =parameter 1
Property 2 of self.object = parameter 2
def method name (self):pass
def method name 2(self):pass
Object Name = Class Name (1,2) #Object is an instance and represents something specific
#Class name (): Class name + parentheses instantiates a class, which is equivalent to calling the u init_u method
#Parameters in parentheses, parameters do not need to pass self, other parameters correspond to one-to-one in init
#Result returns an object
Object name. Object's property 1 #View the object's properties, just use the object name. Property name
Object name. Method name ()#Call a method in a class, using object name directly. Method name ()
Exercise 1: Output the following information at the terminal
Xiao Ming, 10 years old, male, goes up the mountain to cut wood
Xiao Ming, 10 years old, male, driving Northeast
Xiao Ming, 10 years old, male, favorite big health care
Lao Li, 90, male, goes up the mountain to cut wood
Lao Li, 90, male, drives to the Northeast
Lao Li, 90, male, loves big health care
Lao Zhang...
Interaction between objects
Now that we have a human being, we can get a real person by giving it some specific properties.
Now let's create another dog. Dogs can't hit people anymore, they can only bite people, so we give them a bite method.
With dogs, we need to instantiate a real dog.
Then people and dogs can fight.Let's get them to fight now!
Create a Dog
class Dog: # Define a dog class
role = 'dog' # Dogs are all dog characters
def __init__(self, name, breed, aggressivity, life_value):
self.name = name # Each dog has its own nickname;
self.breed = breed # Each dog has its own breed;
self.aggressivity = aggressivity # Each dog has its own attack;
self.life_value = life_value # Each dog has its own life value;
def bite(self,people):
# Dogs can bite people, and here the dog is also an object.
# Dogs bite people, then people's life value decreases depending on the aggression of the dog
dog.life_value -= self.aggressivit
Instantiate a real dihar
ha2 = Dog('Fructus Erbiae','Siberian Husky',10,1000) #Create a real dog ha2
Interactive egon hit ha2
print(ha2.life_value) #Have a look ha2 Value of Life
egg.attack(ha2) #egg Fight ha2 Once
print(ha2.life_value) #ha2 10 blood points dropped
Complete code

class Person: # Define a human
role = 'person' # People's role attributes are people
def __init__(self, name, aggressivity, life_value):
self.name = name # Each character has its own nickname;
self.aggressivity = aggressivity # Each character has its own attack power;
self.life_value = life_value # Each role has its own life value;
def attack(self,dog):
# People can attack a dog, and the dog here is also an object.
# If a person attacks a dog, its life value will decrease according to the person's aggression.
dog.life_value -= self.aggressivity
class Dog: # Define a dog class
role = 'dog' # Dogs are all dog characters
def __init__(self, name, breed, aggressivity, life_value):
self.name = name # Each dog has its own nickname;
self.breed = breed # Each dog has its own breed;
self.aggressivity = aggressivity # Each dog has its own attack;
self.life_value = life_value # Each dog has its own life value;
def bite(self,people):
# Dogs can bite people, and here the dog is also an object.
# Dogs bite people, then people's life value decreases depending on the aggression of the dog
people.life_value -= self.aggressivity
egg = Person('egon',10,1000) #Create a real person egg
ha2 = Dog('Fructus Erbiae','Siberian Husky',10,1000) #Create a real dog ha2
print(ha2.life_value) #Have a look ha2 Value of Life
egg.attack(ha2) #egg Fight ha2 Once
print(ha2.life_value) #ha2 10 blood points dropped
egon War Hash

from math import pi
class Circle:
'''
//A circle class is defined.
//Provides methods for calculating area and perimeter
'''
def __init__(self,radius):
self.radius = radius
def area(self):
return pi * self.radius * self.radius
def perimeter(self):
return 2 * pi *self.radius
circle = Circle(10) #Instantiate a circle
area1 = circle.area() #Calculate the area of a circle
per1 = circle.perimeter() #Calculate Circumference
print(area1,per1) #Print Circle Area and Perimeter
A simple example to help you understand object-oriented
Class Namespaces and Object and Instance Namespaces
Creating a class creates a namespace for the class that stores all the names defined in the class, called attributes of the class
Classes have two properties: static and dynamic
- Static attributes are variables defined directly in a class
- Dynamic attributes are methods defined in classes
Class's data properties are shared to all objects
>>>id(egg.role)
4341594072
>>>id(Person.role)
4341594072
The dynamic properties of a class are bound to all objects
>>>egg.attack
<bound method Person.attack of <__main__.Person object at 0x101285860>>
>>>Person.attack
<function Person.attack at 0x10127abf8>
Creating an object/instance creates a namespace for the object/instance, which holds the name of the object/instance, called its properties
In obj.name, the name is first found in obj's own namespace. If it is not found, the name is found in the class. If it is not found, the parent class is found. If it is not found, an exception is thrown.
Object-oriented combinatorial usage
In addition to inheritance, there is another important way of software reuse: combining
Combination refers to the combination of classes in which objects of another class are used as data attributes.
class Weapon:
def prick(self, obj): # This is an active skill for the equipment,Kill each other
obj.life_value -= 500 # Assume attack power is 500
class Person: # Define a human
role = 'person' # People's role attributes are people
def __init__(self, name):
self.name = name # Each character has its own nickname;
self.weapon = Weapon() # Bind a weapon to a character;
egg = Person('egon')
egg.weapon.prick()
#egg Combining the objects of a weapon, you can directly egg.weapon To use all methods in a composite class
A ring is composed of two circles. The area of a ring is the area of the outer circle minus the area of the inner circle.The perimeter of a ring is the perimeter of the inner circle plus the perimeter of the outer circle.
At this point, we will first implement a circle class and calculate the perimeter and area of a circle.Then combine instances of circles in the Annulus class as their own attributes
from math import pi
class Circle:
'''
//A circle class is defined.
//Provides methods for calculating area and perimeter
'''
def __init__(self,radius):
self.radius = radius
def area(self):
return pi * self.radius * self.radius
def perimeter(self):
return 2 * pi *self.radius
circle = Circle(10) #Instantiate a circle
area1 = circle.area() #Calculate the area of a circle
per1 = circle.perimeter() #Calculate Circumference
print(area1,per1) #Print Circle Area and Perimeter
class Ring:
'''
//A torus class is defined
//Method of providing the area and perimeter of a ring
'''
def __init__(self,radius_outside,radius_inside):
self.outsid_circle = Circle(radius_outside)
self.inside_circle = Circle(radius_inside)
def area(self):
return self.outsid_circle.area() - self.inside_circle.area()
def perimeter(self):
return self.outsid_circle.perimeter() + self.inside_circle.perimeter()
ring = Ring(10,5) #Instantiate a ring
print(ring.perimeter()) #Calculate the perimeter of a ring
print(ring.area()) #Calculate the area of a ring
The relationship between a class and a combined class is established by combining. It is a kind of "yes" relationship, such as when the professor has a birthday and when he teaches python courses.
class BirthDate:
def __init__(self,year,month,day):
self.year=year
self.month=month
self.day=day
class Couse:
def __init__(self,name,price,period):
self.name=name
self.price=price
self.period=period
class Teacher:
def __init__(self,name,gender,birth,course):
self.name=name
self.gender=gender
self.birth=birth
self.course=course
def teach(self):
print('teaching')
p1=Teacher('egon','male',
BirthDate('1995','1','27'),
Couse('python','28000','4 months')
)
print(p1.birth.year,p1.birth.month,p1.birth.day)
print(p1.course.name,p1.course.price,p1.course.period)
'''
Run Results:
1 27
python 28000 4 months
'''
When there is a significant difference between classes, and smaller classes are the components required by larger classes, composition is better
Initial Object Oriented Summary
Define a human
class Person: # Define a human
role = 'person' # People's role attributes are people
def __init__(self, name, aggressivity, life_value, money):
self.name = name # Each character has its own nickname;
self.aggressivity = aggressivity # Each character has its own attack power;
self.life_value = life_value # Each role has its own life value;
self.money = money
def attack(self,dog):
# People can attack a dog, and the dog here is also an object.
# If a person attacks a dog, its life value will decrease according to the person's aggression.
dog.life_value -= self.aggressivity
Define a dog class
class Dog: # Define a dog class
role = 'dog' # Dogs are all dog characters
def __init__(self, name, breed, aggressivity, life_value):
self.name = name # Each dog has its own nickname;
self.breed = breed # Each dog has its own breed;
self.aggressivity = aggressivity # Each dog has its own attack;
self.life_value = life_value # Each dog has its own life value;
def bite(self,people):
# Dogs can bite people, and here the dog is also an object.
# Dogs bite people, then people's life value decreases depending on the aggression of the dog
people.life_value -= self.aggressivity
Next, a new weapon class is created.
class Weapon:
def __init__(self,name, price, aggrev, life_value):
self.name = name
self.price = price
self.aggrev = aggrev
self.life_value = life_value
def update(self, obj): #obj Who's going to bring this equipment
obj.money -= self.price # People who use this weapon will spend less money on it
obj.aggressivity += self.aggrev # Bring this equipment with you to increase your attack
obj.life_value += self.life_value # Bring this device with you can add life
def prick(self, obj): # This is an active skill for the equipment,Kill each other
obj.life_value -= 500 # Assume attack power is 500
Test Interaction
lance = Weapon('Lance',200,6,100)
egg = Person('egon',10,1000,600) #Create a real person egg
ha2 = Dog('Fructus Erbiae','Siberian Husky',10,1000) #Create a real dog ha2
#egg Fight alone"Fructus Erbiae"Feeling exhausted, I decided to save my life and buy a weapon
if egg.money > lance.price: #If egg More money than equipment, you can buy a spear
lance.update(egg) #egg Spend money on a spear to defend yourself and improve your attributes
egg.weapon = lance #egg Equipped with a lance
print(egg.money,egg.life_value,egg.aggressivity)
print(ha2.life_value)
egg.attack(ha2) #egg Fight ha2 Once
print(ha2.life_value)
egg.weapon.prick(ha2) #Launch Weapon Skills
print(ha2.life_value) #ha2 Cunning men won with weapons, half empty slot
Follow this line of thought to design classes and objects bit by bit, and eventually you can fully implement a battle game.
Use the types module to confirm the differences between methods and functions
How to access objects of a custom class using a pickle
Three Object-Oriented Features
inherit
What is inheritance
Inheritance is a way to create new classes. In python, a newly created class can inherit one or more parent classes, which can also be called base or superclass, and a newly created class is called a derived or subclass
Class inheritance in python is divided into single inheritance and multiple inheritance
class ParentClass1: #Define Parent Class
pass
class ParentClass2: #Define Parent Class
pass
class SubClass1(ParentClass1): #Single inheritance, base class is ParentClass1,Derived classes are SubClass
pass
class SubClass2(ParentClass1,ParentClass2): #python Supports multiple inheritances, separating multiple inherited classes with commas
pass
View Inheritance
>>> SubClass1.__bases__ #__base__View only the first subclass inherited from left to right.__bases__Is to view all inherited parent classes
(<class '__main__.ParentClass1'>,)
>>> SubClass2.__bases__
(<class '__main__.ParentClass1'>, <class '__main__.ParentClass2'>)
Tip: If no base class is specified, python's class inherits the object class by default, which is the base class for all Python classes and provides implementations of common methods such as u str_u.
>>> ParentClass1.__bases__
(<class 'object'>,)
>>> ParentClass2.__bases__
(<class 'object'>,)
Inheritance and abstraction (abstraction before inheritance)
Abstraction is the extraction of similar or more similar parts.
The abstraction is divided into two levels:
1. Classify the parts of the objects that are similar to Barack Obama and Messi;
2. Select the more similar parts of the three classes: human, pig and dog as the*parent.
The primary role of abstraction is to categorize (to isolate concerns and reduce complexity)
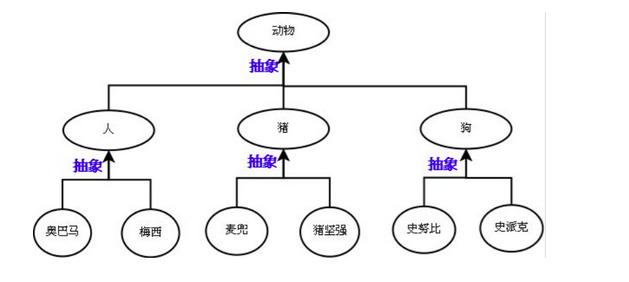
Inheritance: is based on the abstract result, to achieve it through the programming language, must first go through the abstract process, in order to express the abstract structure through inheritance.
Abstract is just an action, or a skill, in the process of analysis and design, through which classes can be derived
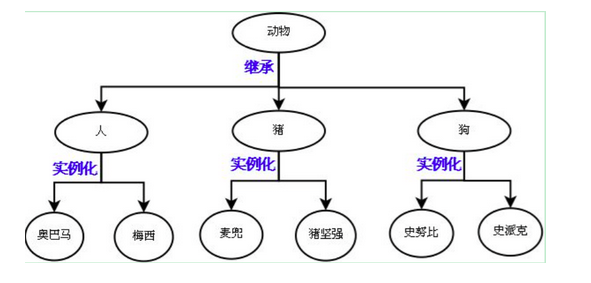
Inheritance and reuse


====================================Part One
for example
Cats can: eat, drink, climb trees
Dogs can: eat, drink and watch
If we want to create a class for cats and dogs, then we need to do all their functions for cats and dogs. The pseudocode is as follows:
#Cats and dogs have a lot of the same content
class cat:
def eat (self):
# do something
def drink (self):
# do something
def self:
# do something
Classdog:
def eat (self):
# do something
def drink (self):
# do something
def home watch (self):
#do something
====================================Part Two
It is easy to see that eating and drinking are functions of both cats and dogs, while we have written two separate cat and dog classes.If you use the idea of inheritance, implement the following:
Animals: eat, drink
Cats: Tree climbing (cats inherit animal functions)
Dogs: Housekeeping (Dogs inherit animal functions)
The pseudocode is as follows:
class animals:
def eat (self):
# do something
def drink (self):
# do something
#Writes another class name in parentheses after the class, indicating that the current class inherits another class
class cat (animal):
def self:
print'Miao'
#Writes another class name in parentheses after the class, indicating that the current class inherits another class
class dog (animal):
def home watch (self):
print'Wow'
=====================================Part Three
Code implementation of #inheritance
class Animal:
def eat(self):
Print ('%s to eat'%self.name)
def drink(self):
print ("%s drinks"%self.name)
class Cat(Animal):
def __init__(self, name):
self.name = name
self.breed ='cat'
def climb(self):
print('tree climbing')
class Dog(Animal):
def __init__(self, name):
self.name = name
self.breed='dog'
def look_after_house(self):
print('bark')
# #################### Execute ###### Execute ######
c1 = Cat('Little White's Black Cat')
c1.eat()
c2 = Cat('Little Black White Cat')
c2.drink()
d1 = Dog('Fat puppy')
d1.eat()
Example of using inheritance to solve code reuse
During the development of the program, if we define a class A and then want to create another class B, but most of the content of class B is the same as class A
It is impossible to write class B from scratch, using the concept of class inheritance.
Create a new class B by inheritance, let B inherit A, B will'inherit'all the attributes of A (data and function attributes), and achieve code reuse
class Animal:
'''
//Both man and dog are animals, so create an Animal base class
'''
def __init__(self, name, aggressivity, life_value):
self.name = name # People and dogs have their own nicknames;
self.aggressivity = aggressivity # People and dogs have their own attack power;
self.life_value = life_value # Both man and dog have their own values of life;
def eat(self):
print('%s is eating'%self.name)
class Dog(Animal):
pass
class Person(Animal):
pass
egg = Person('egon',10,1000)
ha2 = Dog('Fructus Erbiae',50,1000)
egg.eat()
ha2.eat()
Tip: Create a new class with existing classes, which will reuse most of the settings in the existing software, resulting in a lot of programming workload. This is often called software reuse, which can not only reuse your own classes, but also inherit others, such as standard libraries, to customize new data types. This will greatly shorten the software development cycle and large-scaleSoftware development is important.
derive
Of course, subclasses can also add their own new attributes or redefine them here themselves (without affecting the parent class), and it is important to note that once you redefine your own attributes and have the same name as the parent class, you will call the new attributes on your own.
class Animal:
'''
//Both man and dog are animals, so create an Animal base class
'''
def __init__(self, name, aggressivity, life_value):
self.name = name # People and dogs have their own nicknames;
self.aggressivity = aggressivity # People and dogs have their own attack power;
self.life_value = life_value # Both man and dog have their own values of life;
def eat(self):
print('%s is eating'%self.name)
class Dog(Animal):
'''
//Dogs, inheriting Animal classes
'''
def bite(self, people):
'''
//Derived: Dogs have biting skills
:param people:
'''
people.life_value -= self.aggressivity
class Person(Animal):
'''
//Human, Inherit Animal
'''
def attack(self, dog):
'''
//Derived: People have aggressive skills
:param dog:
'''
dog.life_value -= self.aggressivity
egg = Person('egon',10,1000)
ha2 = Dog('Fructus Erbiae',50,1000)
print(ha2.life_value)
print(egg.attack(ha2))
print(ha2.life_value)
Note: Attribute references, such as ha2.life_value, first look for life_value from the instance, then go to the class, and then to the parent class... until the top-level parent.
In subclasses, newly created function attributes with duplicate names may need to reuse the function with duplicate names in the parent class when editing functions within the function. The function should be called in the same way as the normal function, i.e., class name.func(), which is now the same as calling the normal function, so even the self parameter should pass values to it.
In python3, subclasses can also execute parent methods directly using the super method.

class A:
def hahaha(self):
print('A')
class B(A):
def hahaha(self):
super().hahaha()
#super(B,self).hahaha()
#A.hahaha(self)
print('B')
a = A()
b = B()
b.hahaha()
super(B,b).hahaha()
Help you understand super
class Animal:
'''
//Both man and dog are animals, so create an Animal base class
'''
def __init__(self, name, aggressivity, life_value):
self.name = name # People and dogs have their own nicknames;
self.aggressivity = aggressivity # People and dogs have their own attack power;
self.life_value = life_value # Both man and dog have their own values of life;
def eat(self):
print('%s is eating'%self.name)
class Dog(Animal):
'''
//Dogs, inheriting Animal classes
'''
def __init__(self,name,breed,aggressivity,life_value):
super().__init__(name, aggressivity, life_value) #Execute Parent Class Animal Of init Method
self.breed = breed #New attributes derived
def bite(self, people):
'''
//New skills have been derived: Dogs have biting skills
:param people:
'''
people.life_value -= self.aggressivity
def eat(self):
# Animal.eat(self)
#super().eat()
print('from Dog')
class Person(Animal):
'''
//Human, Inherit Animal
'''
def __init__(self,name,aggressivity, life_value,money):
#Animal.__init__(self, name, aggressivity, life_value)
#super(Person, self).__init__(name, aggressivity, life_value)
super().__init__(name,aggressivity, life_value) #Executing parent class init Method
self.money = money #New attributes derived
def attack(self, dog):
'''
//New skills have been derived: aggressive skills
:param dog:
'''
dog.life_value -= self.aggressivity
def eat(self):
#super().eat()
Animal.eat(self)
print('from Person')
egg = Person('egon',10,1000,600)
ha2 = Dog('Fructus Erbiae','Siberian Husky',10,1000)
print(egg.name)
print(ha2.name)
egg.eat()
The relationship between the derived class and the base class is established through inheritance, which is a'yes'relationship, such as white horse being a horse and human being being being an animal.
When there are many identical functions between classes, it is better to extract these common functions into base classes and inherit them, for example, the professor is a teacher.
>>> class Teacher:
... def __init__(self,name,gender):
... self.name=name
... self.gender=gender
... def teach(self):
... print('teaching')
...
>>>
>>> class Professor(Teacher):
... pass
...
>>> p1=Professor('egon','male')
>>> p1.teach()
teaching
Abstract class and interface class
Interface Class
Inheritance has two purposes:
One: inherit the methods of the base class and make your own changes or extensions (code reuse)
2. Declare that a subclass is compatible with a base class, define an interface class Interface, define some interface names (that is, function names) in the interface class and do not implement the functions of the interface, inherit the interface class, and implement the functions in the interface
class Alipay:
'''
//Alipay Payment
'''
def pay(self,money):
print('Alipay paid%s element'%money)
class Applepay:
'''
apple pay payment
'''
def pay(self,money):
print('apple pay Payments made%s element'%money)
def pay(payment,money):
'''
//Payment function, responsible for payment in general
//The object to be paid and the amount to be paid
'''
payment.pay(money)
p = Alipay()
pay(p,200)
Problems that arise easily in development
class Alipay:
'''
//Alipay Payment
'''
def pay(self,money):
print('Alipay paid%s element'%money)
class Applepay:
'''
apple pay payment
'''
def pay(self,money):
print('apple pay Payments made%s element'%money)
class Wechatpay:
def fuqian(self,money):
'''
//pay is implemented, but with a different name
'''
print('WeChat paid%s element'%money)
def pay(payment,money):
'''
//Payment function, responsible for payment in general
//The object to be paid and the amount to be paid
'''
payment.pay(money)
p = Wechatpay()
pay(p,200) #Error in execution
Interface Initial: Manually report exceptions: NotImplementedError to resolve problems encountered in development
class Payment:
def pay(self):
raise NotImplementedError
class Wechatpay(Payment):
def fuqian(self,money):
print('WeChat paid%s element'%money)
p = Wechatpay() #No error here
pay(p,200) #Wrong report here
Borrowing abc module to implement interface
from abc import ABCMeta,abstractmethod
class Payment(metaclass=ABCMeta):
@abstractmethod
def pay(self,money):
pass
class Wechatpay(Payment):
def fuqian(self,money):
print('WeChat paid%s element'%money)
p = Wechatpay() #Wrong if not adjusted
In practice, the first meaning of inheritance is not very significant or even often harmful.Because it makes subclasses strongly coupled with base classes.
The second meaning of inheritance is very important.It is also called interface inheritance.
Interface inheritance essentially requires "a good abstraction, which specifies a compatible interface so that external callers don't need to care about specific details and can process all objects of a particular interface equally" - which is programmatically called normalization.
Normalization allows high-level external users to indiscriminately process a collection of interface-compatible objects - just like linux's generic file concept, everything can be processed as a file, regardless of whether it's memory, disk, network, or screen (and, of course, for low-level designers, it's also possible to distinguish between a "character device" and a "block device" and make a decision)Targeted design: How detailed it is depends on the needs).
Depends on the inversion principle:
High-level modules should not depend on low-level modules, and both should depend on their abstraction; abstraction should not depend on details; and detail should depend on abstraction.In other words, program interfaces, not implementations
There is no keyword called interface in python at all. The code above just looks like an interface, but does not actually function as an interface. Subclasses do not need to implement an interface at all. If you do not want to imitate the concept of an interface, you can use a third-party module:
http://pypi.python.org/pypi/zope.interface
twisted zope.interface is used in twisted\internet\interface.py
Document https://zopeinterface.readthedocs.io/en/latest/
Design pattern: https://github.com/faif/python-patterns


Interfaces extract a group of functions that are common to each other and can be treated as a collection of functions.
Then let the subclasses implement the functions in the interface.
What this means is normalization, which means that as long as the classes are implemented on the same interface, all the classes will produce the same objects in use.
Normalization makes it possible for users not to care about the classes of objects, but to know that these objects have certain functions, which greatly reduces the difficulty of users.
For example, we define an animal interface, which defines interface functions such as running, eating, breathing, etc., so that the mouse class implements the interface and the squirrel class implements the interface. Each of them produces a mouse and a squirrel and sends them to you. Even if you can't tell which mouse is which, you know they will run, eat and breathe..
Another example is: we have a car interface, which defines all the functions of the car, and then Honda, Audi and Volkswagen all implement the car interface. That's easy. You just need to learn how to drive a car, then we will drive whether Honda, Audi or Volkswagen. You don't need to turn off when you drive.What kind of car do I drive? The operation (function call) is the same
Why use interfaces
abstract class
What is an abstract class
Like java, python has the concept of an abstract class but also needs to be implemented with modules. An abstract class is a special class that can only be inherited and cannot be instantiated
Why abstract classes
If a class is drawn from a bunch of objects with the same content, then an abstract class is drawn from a bunch of classes with the same content, including data and function properties.
For example, we have banana classes, apple classes, peach classes. The abstract class of fruit is the same thing that we extract from these classes. When you eat fruit, you either eat a specific banana or eat a specific peach.....You can never eat something called fruit.
From a design perspective, if a class is abstracted from a real object, then an abstract class is abstracted from a class.
From an implementation perspective, abstract classes differ from ordinary classes in that they have abstract methods, which cannot be instantiated, can only be inherited, and subclasses must implement Abstract methods.It's a bit like the interface, but it's different and we're going to get the answer
Implementing abstract classes in python

#Everything is a file
import abc #utilize abc Module implementation abstract class
class All_file(metaclass=abc.ABCMeta):
all_type='file'
@abc.abstractmethod #Define abstract methods without implementing functionality
def read(self):
'Subclasses must define read capabilities'
pass
@abc.abstractmethod #Define abstract methods without implementing functionality
def write(self):
'Subclasses must define write capabilities'
pass
# class Txt(All_file):
# pass
#
# t1=Txt() #Error, subclass does not define abstract method
class Txt(All_file): #Subclasses inherit Abstract classes, but must be defined read and write Method
def read(self):
print('Reading methods of text data')
def write(self):
print('Reading methods of text data')
class Sata(All_file): #Subclasses inherit Abstract classes, but must be defined read and write Method
def read(self):
print('Reading methods of hard disk data')
def write(self):
print('Reading methods of hard disk data')
class Process(All_file): #Subclasses inherit Abstract classes, but must be defined read and write Method
def read(self):
print('Method of reading process data')
def write(self):
print('Method of reading process data')
wenbenwenjian=Txt()
yingpanwenjian=Sata()
jinchengwenjian=Process()
#So we're all normalized,That is, the idea that everything is a file
wenbenwenjian.read()
yingpanwenjian.write()
jinchengwenjian.read()
print(wenbenwenjian.all_type)
print(yingpanwenjian.all_type)
print(jinchengwenjian.all_type)
Abstract class and interface class
The essence of an abstract class or a class refers to the similarity of a set of classes, including data attributes (such as all_type) and function attributes (such as read, write), while interfaces only emphasize the similarity of function attributes.
An abstract class is a direct concept between a class and an interface with some of the properties of the class and interface that can be used to implement a normalized design.
There is no such thing as interface classes in python, and we should have some basic concepts even if we don't define interfaces through specialized modules.
1. Multiple Inheritance
In inheriting Abstract classes, we should try to avoid multiple inheritances.
When inheriting interfaces, we encourage you to inherit more interfaces
Interface Isolation Principle:
Use multiple specialized interfaces instead of a single overall interface.That is, clients should not rely on unnecessary interfaces.
2. Implementation of the method
In Abstract classes, we can make basic implementations of some abstract methods.
In the interface class, any method is just a specification, and the specific functions need to be implemented by subclasses.
Diamond Inheritance
Inheritance order

class A(object):
def test(self):
print('from A')
class B(A):
def test(self):
print('from B')
class C(A):
def test(self):
print('from C')
class D(B):
def test(self):
print('from D')
class E(C):
def test(self):
print('from E')
class F(D,E):
# def test(self):
# print('from F')
pass
f1=F()
f1.test()
print(F.__mro__) #Only newer forms have this property to view linear lists, classical classes do not have this property
#New Class Inheritance Order:F->D->B->E->C->A
#Classic Class Inheritance Order:F->D->B->A->E->C
#python3 Unified in China are all new-style classes
#pyhon2 Medium Talent Class and Classic Class
Inheritance order
Inheritance Principle
How python actually implements inheritance, and for each class you define, python calculates a method resolution order (MRO) list, which is a simple linear order list of all base classes, such as
>>> F.mro() #Equivalent to F. u mro_u
[<class '__main__.F'>, <class '__main__.D'>, <class '__main__.B'>, <class '__main__.E'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>]
To implement inheritance, python looks for base classes from left to right on the MRO list until it finds the first class that matches this property.
This MRO list is constructed by a C3 linearization algorithm.Let's not delve into the math behind this algorithm, which is essentially a merge of MRO lists of all parent classes and follows three guidelines:
1. The subclass will be checked before the parent
2. Multiple parent classes are checked according to their order in the list
3. If there are two legal choices for the next class, choose the first parent class
Inheritance Summary
The role of inheritance
Reduce code reuse
Improving code readability
Canonical programming mode
Several nouns
Abstract: Abstraction is the extraction of similar or more similar parts.It is a process from titling to abstraction.
Inheritance: The subclass inherits the methods and properties of the parent class
Derivation: Subclasses create new methods and attributes based on parent methods and attributes
Abstract class and interface class
1. Multiple Inheritance
In inheriting Abstract classes, we should try to avoid multiple inheritances.
When inheriting interfaces, we encourage you to inherit more interfaces
2. Implementation of the method
In Abstract classes, we can make basic implementations of some abstract methods.
In the interface class, any method is just a specification, and the specific functions need to be implemented by subclasses.
Diamond Inheritance
New Class: Width First
Classic Class: Depth First
polymorphic
Polymorphism refers to the fact that a class of things has multiple forms
Animals come in many forms: human, dog, pig*
import abc
class Animal(metaclass=abc.ABCMeta): #Same kind of things:Animal
@abc.abstractmethod
def talk(self):
pass
class People(Animal): #One of the animal forms:people
def talk(self):
print('say hello')
class Dog(Animal): #Animal Form Two:Dog
def talk(self):
print('say wangwang')
class Pig(Animal): #Animal Form Three:pig
def talk(self):
print('say aoao')
Files come in many forms: text files, executable files
import abc
class File(metaclass=abc.ABCMeta): #Same kind of things:file
@abc.abstractmethod
def click(self):
pass
class Text(File): #One of the shapes of a file:text file
def click(self):
print('open file')
class ExeFile(File): #Form Two of the File:Executable File
def click(self):
print('execute file')
Polymorphism
What is polymorphic dynamic binding (sometimes referred to as polymorphism when used in the context of inheritance)
Polymorphism is the use of an instance regardless of its type
In object-oriented methods, polymorphisms are generally expressed as follows:
Send the same message to different objects (!!!obj.func(): is a method func that calls obj, also known as a message func that sends obj, and different objects behave differently when they receive it (that is, a method).
That is, each object can respond to a common message in its own way.The so-called message is to call a function, and different behaviors refer to different implementations, that is, to execute different functions.
For example: teacher. The bell rings (), student. The bell rings (), the teacher performs the after-work operation, and the student performs the after-school operation. Although the two messages are the same, the effect of the execution is different.
Polymorphism
peo=People()
dog=Dog()
pig=Pig()
#peo,dog,pig All animals,Animals must have talk Method
#So we don't have to think about what the three are.,And use it directly
peo.talk()
dog.talk()
pig.talk()
#Further more,We can define a unified interface to use
def func(obj):
obj.talk()
Duck type
Comma moment:
Python advocates the duck type,'If it looks like, sounds like, and walks like a duck, then it's a Duck'
python programmers typically write programs based on this behavior.For example, if you want to write a custom version of an existing object, you can inherit it
You can also create a completely new object that looks and acts like it but has no relationship with it, which is often used to preserve the looseness of the program components.
Example 1: Utilize various'file-like'objects defined in the standard library, although they work like files, they do not inherit the methods of built-in file objects
Example 2: Sequence types come in many forms: strings, lists, tuples, but they have no direct inheritance relationship
#Both look like ducks,Both look like files,So you can use it as a file
class TxtFile:
def read(self):
pass
def write(self):
pass
class DiskFile:
def read(self):
pass
def write(self):
pass
encapsulation
[Encapsulation]
Hide the properties and implementation details of the object and provide public access only.
[Benefits]
1. Isolate changes;
2. Easy to use;
3. Improve reusability;
4. Improve security;
[Packaging principle]
1. Hide everything you don't need to provide to the outside world;
2. Hide properties and provide public access to them.
Private variables and private methods
Hide properties in python starting with a double underscore (set to private)
private variable
#Actually, this is just a distortion operation
#All names in a class that begin with a double underscore, such as__x Will automatically distort to:_Class name__x Form:
class A:
__N=0 #Class data properties should be shared,Grammatically, however, you can set a class's data properties to be private, such as__N,Will distort to_A__N
def __init__(self):
self.__X=10 #Deformation to self._A__X
def __foo(self): #Deformation to_A__foo
print('from A')
def bar(self):
self.__foo() #Only within a class can it pass through__foo Form access to.
#A._A__N It is accessible, that is, it is not strictly a restriction on external access, it is just a grammatical distortion
This automatic distortion is characterized by:
1. The u x defined in the class can only be used internally, such as self. u x, which refers to the result of distortion.
2. This distortion is actually for external distortion, which is not accessible by the name u x.
3. The u x defined in the subclass does not override the u x defined in the parent class because the subclass is distorted to: _subclass name u x, while the parent is distorted to: _parent name u x, that is, attributes starting with a double downslide line cannot be overridden when inherited to the subclass.
The problem with this distortion is that:
1. This mechanism does not really restrict us from accessing attributes directly from outside. Knowing the class name and attribute name, we can spell the name: _class name_u attribute, and then we can access it, such as a. _A_u N
2. The process of distortion only takes effect inside the class, and it is not distorted by the assignment operation after definition.
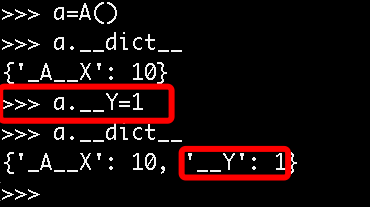
Private Method
3. In inheritance, a parent can define a method as private if it does not want its subclasses to override its own methods
#Normal Conditions
>>> class A:
... def fa(self):
... print('from A')
... def test(self):
... self.fa()
...
>>> class B(A):
... def fa(self):
... print('from B')
...
>>> b=B()
>>> b.test()
from B
#hold fa Defined as private, i.e.__fa
>>> class A:
... def __fa(self): #Defined as_A__fa
... print('from A')
... def test(self):
... self.__fa() #Only the class you belong to will prevail,That is, call_A__fa
...
>>> class B(A):
... def __fa(self):
... print('from B')
...
>>> b=B()
>>> b.test()
from A
Encapsulation and Extensibility
Encapsulation is about clearly distinguishing between inside and outside so that class implementers can modify what's inside the encapsulation without affecting the external caller's code; while external users only know one interface (function), as long as the interface (function) name and parameters remain the same, the user's code will never change.This provides a good basis for collaboration -- or, as long as the basic convention of interfaces is unchanged, code changes are not a concern.
#Designer of class
class Room:
def __init__(self,name,owner,width,length,high):
self.name=name
self.owner=owner
self.__width=width
self.__length=length
self.__high=high
def tell_area(self): #The external interface hides the internal implementation details, at this point we want the area
return self.__width * self.__length
#User
>>> r1=Room('Bedroom','egon',20,20,20)
>>> r1.tell_area() #Consumer Call Interface tell_area
#Designers of classes, which easily extend functionality, do not require users of classes to change their code at all
class Room:
def __init__(self,name,owner,width,length,high):
self.name=name
self.owner=owner
self.__width=width
self.__length=length
self.__high=high
def tell_area(self): #The external interface hides the internal implementation, and what we want is volume,Internal logic has changed,Simply fix the following line to get a very simple implementation,And external calls are not aware,This method is still used, but functionality has changed
return self.__width * self.__length * self.__high
#For still in use tell_area People with interfaces can use new functionality without changing their code at all
>>> r1.tell_area()
Property property
What is attribute property
Property is a special property that executes a function (function) and returns a value when accessed


Example 1: BMI index (bmi is calculated, but it clearly sounds like an attribute rather than a method, and it would be easier to understand if we made it an attribute)
BMI values for adults:
Too light: less than 18.5
Normal: 18.5-23.9
Overweight: 24-27
Obesity: 28-32
Very obese, above 32
Body mass index (BMI) = body weight (kg) height ^2 (m)
EX: 70kg÷(1.75×1.75)=22.86
Example 1

class People:
def __init__(self,name,weight,height):
self.name=name
self.weight=weight
self.height=height
@property
def bmi(self):
return self.weight / (self.height**2)
p1=People('egon',75,1.85)
print(p1.bmi)
import math
class Circle:
def __init__(self,radius): #The radius of a circle radius
self.radius=radius
@property
def area(self):
return math.pi * self.radius**2 #Calculate area
@property
def perimeter(self):
return 2*math.pi*self.radius #Calculate Perimeter
c=Circle(10)
print(c.radius)
print(c.area) #Can be accessed as data properties area,Triggers the execution of a function,Calculate a value dynamically
print(c.perimeter) #Ditto
'''
//Output results:
314.1592653589793
62.83185307179586
'''
Example 2: Perimeter and area of a circle
Why use property
After defining a function of a class as an attribute, obj.name when an object is reused does not even notice that its name is a function that is executed and then calculated. This feature is used in a way that follows the principle of uniform access
In addition, look at
ps: There are three ways of object-oriented encapsulation:
[public]
In fact, this is not encapsulated, it is open to the public
[protected]
This kind of encapsulation is not public to the public, but it is public to friends or subclasses (the image says "son"), but I don't know why people don't say "daughter", just like "parent" means "parent", but Chinese is called "parent").
[private]
This encapsulation is not public to anyone
python does not grammatically build all three of them into its own class mechanism. In C++, all data is typically set to private, then set and get methods (interfaces) are provided to set and retrieve them, which can be achieved in python through the property method.
class Foo:
def __init__(self,val):
self.__NAME=val #Hide all data properties
@property
def name(self):
return self.__NAME #obj.name Visited by self.__NAME(This is where the true values are stored)
@name.setter
def name(self,value):
if not isinstance(value,str): #Type check before setting values
raise TypeError('%s must be str' %value)
self.__NAME=value #After type checking,Will value value Store in a real location self.__NAME
@name.deleter
def name(self):
raise TypeError('Can not delete')
f=Foo('egon')
print(f.name)
# f.name=10 #Throw an exception'TypeError: 10 must be str'
del f.name #throw'TypeError: Can not delete'
A static property property property is essentially an implementation of get, set, delete

class Foo:
@property
def AAA(self):
print('get Run me when it's time')
@AAA.setter
def AAA(self,value):
print('set Run me when it's time')
@AAA.deleter
def AAA(self):
print('delete Run me when it's time')
#Only attributes AAA Definition property Only then can it be defined AAA.setter,AAA.deleter
f1=Foo()
f1.AAA
f1.AAA='aaa'
del f1.AAA
class Foo:
def get_AAA(self):
print('get Run me when it's time')
def set_AAA(self,value):
print('set Run me when it's time')
def delete_AAA(self):
print('delete Run me when it's time')
AAA=property(get_AAA,set_AAA,delete_AAA) #Built-in property Three parameters and get,set,delete One-to-one correspondence
f1=Foo()
f1.AAA
f1.AAA='aaa'
del f1.AAA
How to use it?
class Goods:
def __init__(self):
# Original price
self.original_price = 100
# Discount
self.discount = 0.8
@property
def price(self):
# Actual price = Original price * Discount
new_price = self.original_price * self.discount
return new_price
@price.setter
def price(self, value):
self.original_price = value
@price.deleter
def price(self):
del self.original_price
obj = Goods()
obj.price # Get commodity prices
obj.price = 200 # Modify original price
print(obj.price)
del obj.price # Delete original price
classmethod
class Classmethod_Demo():
role = 'dog'
@classmethod
def func(cls):
print(cls.role)
Classmethod_Demo.func()
staticmethod
class Staticmethod_Demo():
role = 'dog'
@staticmethod
def func():
print("When used in the general method")
Staticmethod_Demo.func()

class Foo:
def func(self):
print('in father')
class Son(Foo):
def func(self):
print('in son')
s = Son()
s.func()
# Please say the output of the above code and explain why?
Exercise 1

class A:
__role = 'CHINA'
@classmethod
def show_role(cls):
print(cls.__role)
@staticmethod
def get_role():
return A.__role
@property
def role(self):
return self.__role
a = A()
print(a.role)
print(a.get_role())
a.show_role()
# __role What identities are in the class?
# What does the above code output?
# What is the function of the three decorators?What are the differences?
Exercise 2
More Object Oriented Description
Object-Oriented Software Development
After learning python's class mechanism, many people will encounter a problem in production or be confused. This is really normal, because any program development is designed before programming. python's class mechanism is just a programming method. If you insist on holding the class and your problem to death, it will take a minute to become more confused. In the past, softwareDevelopment is relatively simple, from task analysis to program writing to program debugging, which can be done by one person or a group.However, with the rapid increase of software size, any problems facing the software are very complex, there are too many factors to consider. The errors and hidden errors, unknown errors in a software may reach an astonishing degree, which is not completely solved at the design stage.
So software development is actually a complete set of specifications, what we have learned is only a small part of it, a complete development process, which requires defining the tasks of each stage, and proceeding to the next stage under the premise of ensuring the correctness of one stage, called software engineering
Object-oriented software engineering includes the following sections:
1. object oriented analysis (OOA)
In the system analysis phase of software engineering, analysts and users are required to combine to make a precise analysis and clear statement of users'needs, and to analyze what software systems should do, not how to do, in a large way.Object-oriented analysis should follow the object-oriented concepts and methods, in the analysis of tasks, from the relationship between objects and objects that exist objectively, from the related objects (object's "characteristics" and "skills") to the relations between objects, and use a class to identify objects with the same attributes and behaviors.
Establish a demand model that reflects this work situation, where the model is rough.
2 object oriented design (OOD)
Based on the requirements model formed during the object-oriented analysis stage, each part is designed specifically.
The first is the design of classes, which may contain multiple levels (using inheritance and derivation mechanisms).Then, on the basis of these classes, the ideas and methods of program design, including the design of algorithms, are presented.
The design phase does not involve any specific computer language, but rather a more general description tool, such as pseudocode or flowcharts
3 object oriented programming (OOP)
Based on the results of object-oriented design, choose a computer language to write it as a program, which can be python
4 object oriented test (OOT)
Before a program is written and handed over to the user, it must be rigorously tested to detect errors in the program and correct them.
Pair-oriented testing uses an object-oriented approach to testing, with classes as the basic unit of testing.
5 Object Oriented Soft Maintenance (OOSM)
Just as after-sales services and maintenance are required for any product, there are problems with the software when it is used, or if the software vendor wants to improve the performance of the software, it needs to modify the program.
Because the program is developed using an object-oriented method, it is easier to maintain the program.
Because of the encapsulation of objects, modifying one object has little effect on other objects. Using object-oriented method to maintain the program greatly improves the efficiency and scalability of software maintenance.
In the object-oriented method, the earliest development must be object-oriented programming (OOP). At that time, neither OOA nor OOD had been developed. In order to write object-oriented programs, programmers must go deep into the analysis and design field, especially in the design field. At that time, OOP actually contained two phases of OOD and OOP, which required more from programmers.Many people find it difficult to master.
Now designing a large software is strictly in accordance with the five stages of object-oriented software engineering. The work of the five stages is not completed by one person from beginning to end, but by different people, so the tasks of OOP phase are simpler.Programmers only need to write programs in object-oriented language according to the ideas put forward by OOd.
OOP is only a small part of a large software development process.
For full stack development, there are five stages. For simple problems, it is not necessary to strictly follow these five stages. Programmers often follow object-oriented methods for program design, including class design and program design.
Explanation of several concepts
1. Object-oriented programming looks tall, so when I'm programming, I should make sure I'm writing a whole class, so that the program I write must be good (Object-oriented only works in scenarios where scalability is a high requirement)
2. Many people like to talk about the three main object-oriented features (where did this come from, encapsulation, polymorphism, inheritance?There are too many bugs, let's call them the three main features for now), so when I'm programming based on object-oriented, I must let the classes I define contain all three of them, so it must be a good program to write
Good fellow, I said that the eighteen palms of the fallen dragon have eighteen palms, so every time you fight with someone, you have to fight from the first palm to the eighteenth palm to make it look like you are going to be there: in the face of the enemy, you have already fallen by the third palm. You said, No, you can get up for Lao Zi, Lao Zi has not finished show yet...
3. Classes have class attributes and instances have instance attributes, so when we define classes, we must define so many class attributes that we can't imagine what to do, so think hard, the more you define, the more nifty you are
This makes a serious error, the sooner the program is object-oriented, the sooner it dies, and what is object-oriented, because we want to combine data with functions, the overall structure of the program is not out, or you don't know all the issues that need to be considered, so you start object-oriented, which leads to you thinking there and thinking you think you know it all.And define a bunch of attributes that you won't use afterwards, or you can't figure out what to define, so you'll just keep thinking and you'll go crazy.
You've seen a company that wants to develop a software and start writing it. It must be a frequent meeting to discuss plans. See Section 8.
Common Object-Oriented Terms
Abstract/Implementation
Abstraction refers to the modeling of the essential manifestations, behaviors, and characteristics of real-world problems and entities, and the establishment of a related subset that can be used to draw program structures to implement such models.Abstraction includes not only the data properties of this model, but also the interfaces to these data.
An Abstract implementation is a realization of this data and its associated interfaces.The process of realizing should be transparent and irrelevant to the client program.(
Packaging/Interface
Encapsulation describes the concept of hiding data/information by providing interfaces and access functions to data properties.Accessing data directly from any client, regardless of the interface, is contrary to encapsulation unless the programmer allows it.As part of the implementation, clients do not need to know how data attributes are organized after encapsulation.In Python, all class attributes are public, but the name may be "confused" to prevent unauthorized access, but that's all. There are no other precautions.This requires a corresponding interface to the data at design time to prevent client programs from accessing encapsulated data attributes through nonstandard operations.
Note: Encapsulation is by no means equivalent to "hiding something you don't want others to see that you might modify in the future with private"
The real encapsulation is to think deeply, make a good abstraction, give a "complete and minimal" interface, and make the internal details transparent to the outside
(Note: Transparency means that external callers can get any functionality they want without being aware of the internal details.)
Synthesis
Composition extends the description of classes so that many different classes are combined into one large class to solve real problems.Composite describes an extremely complex system, such as a class composed of other classes, smaller components may be other classes, data attributes, and behavior, all of which together are "one" relationships with each other.
Derivation/Inheritance/Inheritance Structure
Derivation describes the derivation of new attributes from subclasses that retain all required data and behavior in an existing class type, but allow modifications or other custom operations without modifying the definition of the original class.
Inheritance describes how subclass attributes inherit from an ancestor class
The inheritance structure represents multiple "generations" derived and can be described as a "family tree", a continuous subclass, related to ancestor classes.
Generalization/Specialization
Inheritance-based
Generalization means that all subclasses have the same characteristics as their parent and ancestor classes.
Specialization describes the customization of all subclasses, that is, what attributes make it different from its ancestor classes.
Polymorphism and Polymorphism
Polymorphism refers to the multiple states of the same thing: water, which has many different states: ice, water vapor
The concept of polymorphism indicates how objects operate and access through their common attributes and actions, regardless of their specific classes.
Ice, water vapor, all inherit from water. They all have the same name as Cloud, but Ice, Cloud (), is a completely different process from Water Vapor, Cloud (), although it is invoked in the same way.
Self-reflection
Self-reflection, also known as reflection, shows how an object obtains information about itself at run time.If you pass an object to you, you can find out how capable it is, which is a powerful feature.If Python does not support some form of introspection, dir and type built-in functions will not work properly.And those special properties, like u dict_u, u name_u and u doc_u
Topics:
Python
Programming
Attribute
Linux