Flask Foundation
Flash overview
What is the Web Framework
Web Application Framework (Web Application Framework) or simple web framework (Web Framework) represents a collection of libraries and modules, enabling web application developers to write applications without worrying about low-level details such as protocols and thread management.
What is Flask
Flask is a Web application framework written in Python. It was developed by * * Armin Ronacher * *, who led an international team of Python enthusiasts called Pocco. Flask is based on Werkzeug WSGI toolkit and Jinja2 template engine. Both are Pocco projects.
WSGI
Web Server Gateway Interface (WSGI) has been used as the standard for Python Web application development. WSGI is the specification of the common interface between web server and web application.
Werkzeug
It is a WSGI toolkit, which implements request, response object and utility function. This makes it possible to build a web framework on it. The Flask framework uses Werkzeug as one of its foundations.
jinja2
jinja2 is a popular template engine for Python. The Web template system combines templates with specific data sources to present dynamic Web pages.
Flask is often referred to as a microframe. It is designed to keep the core of the application simple and scalable. Flash does not have a built-in abstraction layer for database processing, nor does it form validation support. Instead, flask supports extensions to add such functionality to applications. Some of the popular flash extensions will be discussed later in this tutorial.
Flash environment
Flash installation conditions
Python 2.6 or later is usually required to install Flask. Although Flask and its dependencies are applicable to Python 3 (Python 3.3 and above), many Flask extensions do not support it correctly. Therefore, it is recommended to install Flask on Python 2.7.
Install virtualenv for the development environment
virtualenv is a virtual Python environment Builder. It can help users create multiple Python environments in parallel. Therefore, it can avoid compatibility problems between different versions of libraries.
The following command is used to install virtualenv:
pip install virtualenv
This command requires administrator privileges. You can add "sudo" before * * pip "on Linux / Mac OS. If you are using Windows, please log in as an administrator. On Ubuntu, virtualenv can be installed using its package manager.
Sudo apt-get install virtualenv
After installation, a new virtual environment is created in the folder.
mkdir newproj
cd newproj
python -m venv newproj
To activate the corresponding environment on # Linux / OS X #, use the following command:
venv/bin/activate
To activate the corresponding environment on Windows *, you can use the following command:
venv\scripts\activate
We are now ready to install Flask in this environment:
pip install Flask
The above commands can be run directly without the need for a system wide virtual environment.
Flash application
Hello Word program
It is preferred to import the flash module in the project. One object of the Flask class is our WSGI application.
The flag constructor uses * * current module (_ name _) As a parameter.
The route() * * function of the Flask class is a decorator that tells the application which URL should call the relevant function.
# -*- coding: utf-8 -*- from flask import Flask app = Flask(__name__) @app.route('/') def hello(): return "hello word" if __name__ == "__main__": app.run(host='0.0.0.0')
In the above example, the '/' URL is the same as * * hello_world() * * function binding. Therefore, when the home page of the web server is opened in the browser, the output of this function will be rendered.
Run and access the page
app.run() is equivalent to executing the flash run operation on the command line, and flash automatically starts its own installed web service
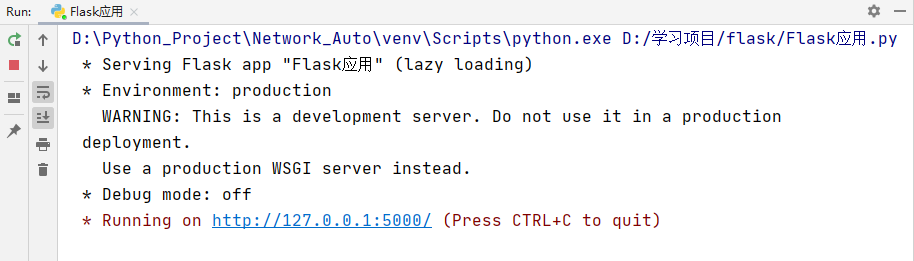
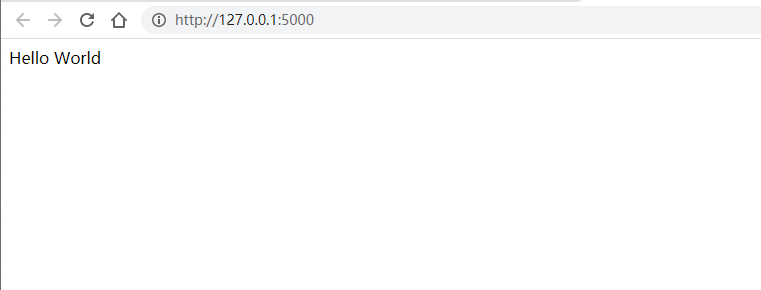
app.route()
@app.route(rule, options)
-
**The rule * * parameter indicates the binding with the URL of the function.
-
**options * * is the parameter list to be forwarded to the underlying Rule object.
app.run()
The * * run() * * method of Flask class runs the application on the local development server. The run() method has four parameters to choose from
app.run(host, port, debug, options)
- host = '0.0.0.0' enable external access. Other hosts can access web services through local IP
**DEBUG and setting py
**
if __name__ == "__main__": app.run(host='0.0.0.0', debug=True)
- debug=True enables the dubug mode. As long as the code changes, the latest code will be reloaded
- debug=False is the default mode. The code will not be loaded automatically when it changes. It is suitable for the same environment
app.config['ENV'] = 'development' app.config['DEBUG'] = True
- After using Flask(name) to build the app object, add the above code. The function is the same as debug=True
# settings.py ENV = 'development' DEBUG = True
# app.py import sttings app.config.from_object(settings)
- Directly on app Setting the environment status in py will affect the main programs, so settings is introduced Py file, just in app Py can be used in settings Pre defined configuration in PY
app.run() method parameter table
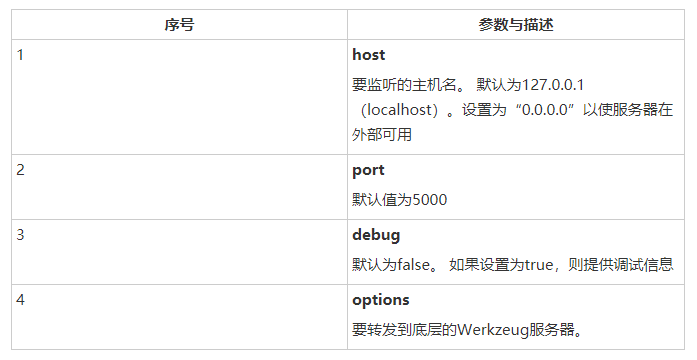
Flask routing
Flask routing access
Modern Web frameworks use routing techniques to help users remember application URL s. You can access the desired page directly without navigating from the home page.
The * * route() * * decorator in Flask is used to bind URL s to functions. For example:
from flask import Flask app = Flask(__name__) @app.route("/hello") def hello(): return "hello, Word111111111" if __name__ == '__main__': app.run(debug=True)
Here, the URL * * '/ Hello' rule is bound to hello_ The world() function. Therefore, if the user accesses http: / / localhost: 5000 / hello * * URL, * * hello_ The output of the world() * * function will be rendered in the browser.
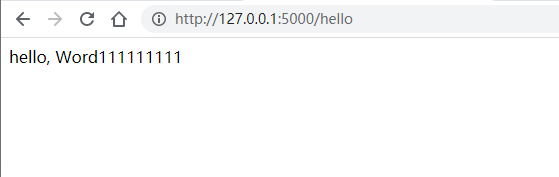
Request and response
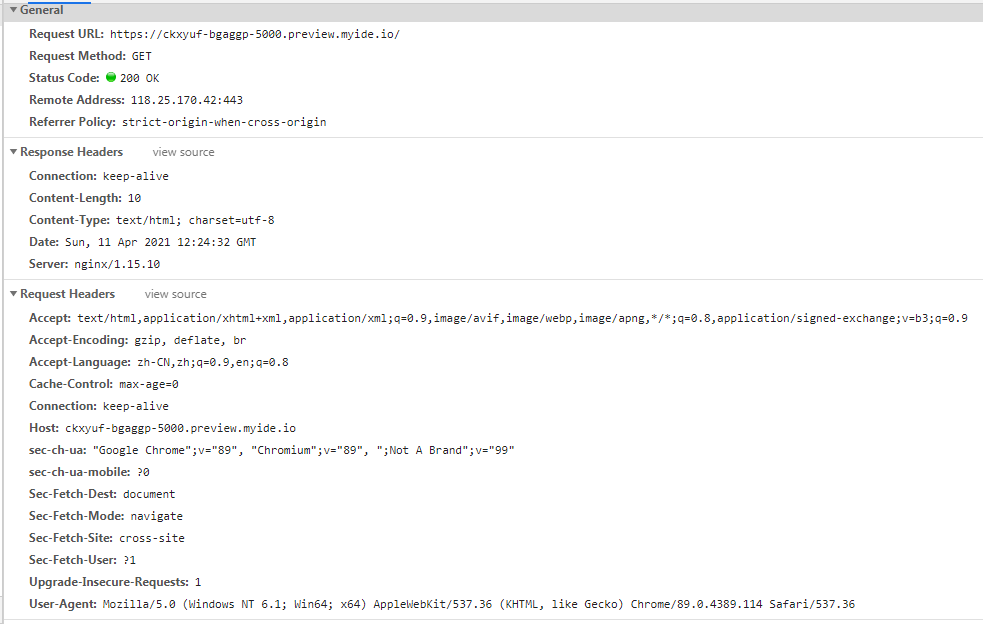
add_rul_rule() routing binding
Add of application object_ url_ The rule () function can also be used to bind a URL to a function, as with app route().
The purpose of the decorator can also be represented by the following:
from flask import Flask app = Flask(__name__) def hello(): return "hello, Word111111111" if __name__ == '__main__': # Equivalent to @ app Route ('/ Hello') because app The bottom layer of route is actually add_url_rule method app.add_url_rule('/', 'hello', hello) app.run(debug=True)
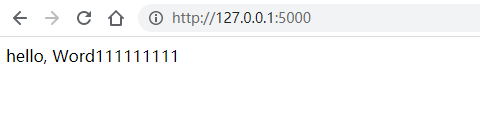
Flag variable rule
The URL can be built dynamically by adding variable parts to the rule parameters. This variable section is marked * *. It is passed as a keyword parameter to the function associated with the rule.
In the following example, the rule parameter of the route() decorator contains a string attached to the URL '/ Hello'. Therefore, if you enter in the browser http://localhost:5000/hello/xiaochen As URL * *, then * * 'Xiaochen' * * will be provided as a parameter to the * * hello() * * function.
from markupsafe import escape from flask import Flask app = Flask(__name__) """ visit: http://127.0.0.1:5000/hello/xiaochen Output: Hello xiaochen! the last one/The following contents are passed to the previous one as parameters URL page """ @app.route('/hello/<name>') def hello_name(name): return 'Hello %s!' % name if __name__ == '__main__': app.run()
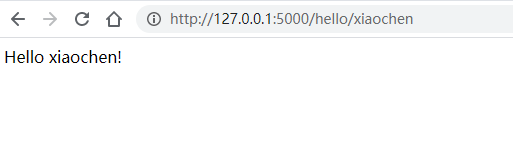
Types supported by variable rules
In addition to the default string variable section, you can also use the following converters to build rules:
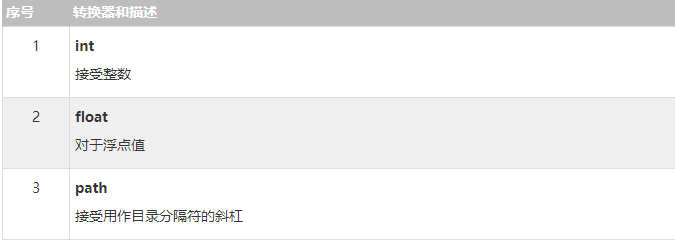
Sample code
from markupsafe import escape from flask import Flask app = Flask(__name__) @app.route('/user/<username>') def show_user_profile(username): # show the user profile for that user return 'User %s' % escape(username) """ In addition to strings, you can also pass in int(Integer) float(Floating point number) path(belt/Separator (path) see show_post,show_float,show_subpath Three methods show the results """ @app.route('/post/<int:post_id>') def show_post(post_id): # show the post with the given id, the id is an integer return 'Post Number %d' % post_id @app.route('/float/<float:revNo>') def show_float(float_num): return 'Float Number %f' % float_num @app.route('/path/<path:subpath>') def show_subpath(subpath): # show the subpath after /path/ return 'Subpath %s' % escape(subpath) if __name__ == '__main__': print(show_user_profile(username='yangxiaochen')) print(show_post(post_id=1)) print(show_subpath(subpath='/project')) app.run()
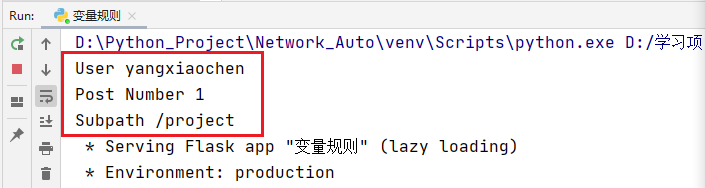
Flash URL construction
url_for() construct URL
**url_ The for() * * function is useful for dynamically building URLs for specific functions. The function takes the name of the function as the first parameter and one or more keyword parameters, each corresponding to the variable part of the URL.
from flask import Flask, redirect, url_for app = Flask(__name__) """ visit: http://127.0.0.1:5000/admin Output: Hello Admin """ @app.route('/admin') def hello_admin(): return 'Hello Admin' """ visit: http://127.0.0.1:5000/guest/xiaochen Output: Hello xiaochen as Guest """ @app.route('/guest/<guest>') def hello_guest(guest): return 'Hello %s as Guest' % guest """ visit: http://127.0.0.1:5000/user/admin Auto redirect to http://127.0.0.1:5000/admin Output: Hello Admin visit: http://127.0.0.1:5000/user/xiaochen Auto redirect to http://127.0.0.1:5000/guest/xiaochen Output: Hello xiaochen as Guest """ @app.route('/user/<name>') def hello_user(name): if name =='admin': return redirect(url_for('hello_admin')) else: return redirect(url_for('hello_guest',guest = name)) if __name__ == '__main__': app.run(debug = True)
**The User() function checks whether the received parameters match 'admin'. If there is a match, the URL is used_ For() redirects the application to hello_admin() function, otherwise redirect to the Hello that passes the received parameter to it as a guest parameter_ Guest() * * function.
Open browser and enter URL - http://localhost:5000/user/admin
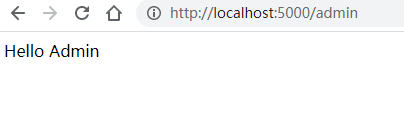
Enter the following URL in your browser - http://localhost:5000/user/mvl
**
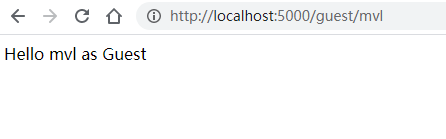
Flash response object
The Response object returns the data type
settings.py
ENV = 'development' DEBUG = True
app.py
# -*- coding: utf-8 -*- import test from flask import Flask, redirect, url_for, Response, make_response import settings app = Flask(__name__) app.config.from_object(settings) @app.route('/index1') def index1(): return '<h1>Return string type</h1>' @app.route('/index2') def index2(): return {'a': 'beijing', 'b': 'shanghai', 'c': 'cehngdu'} @app.route('/index3') def index3(): return 'Tuple type', 200 @app.route('/index4') def index4(): response = Response('<h1>adopt Response return HTML type</h1>') print(response.content_type) print(response.headers) print(response.status_code) print(response.status) return Response('<h1>adopt Response return HTML type</h1>') if __name__ == '__main__': app.run()
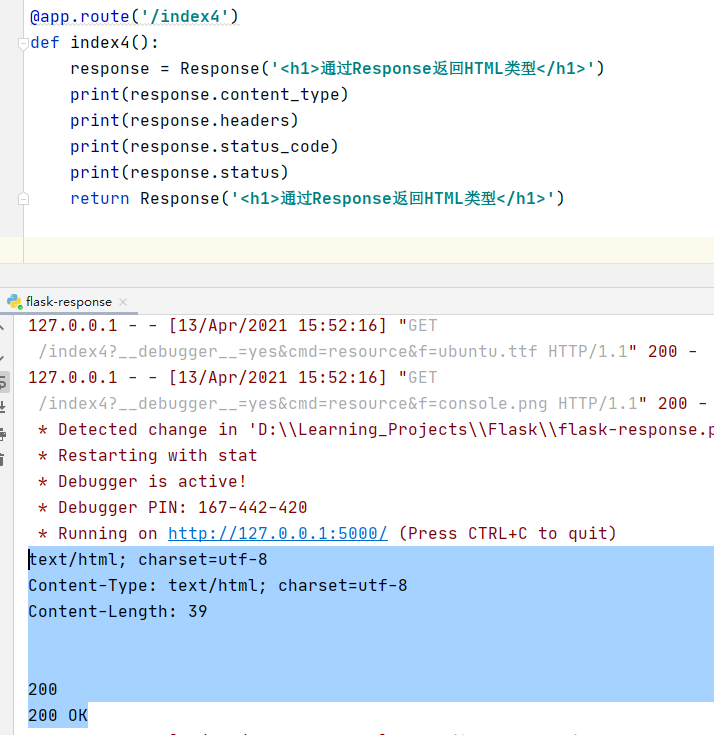
Flash HTTP method
Http protocol is the basis of data communication in the world wide web. Different methods of retrieving data from the specified URL are defined in the protocol.
The following table summarizes the different http methods:
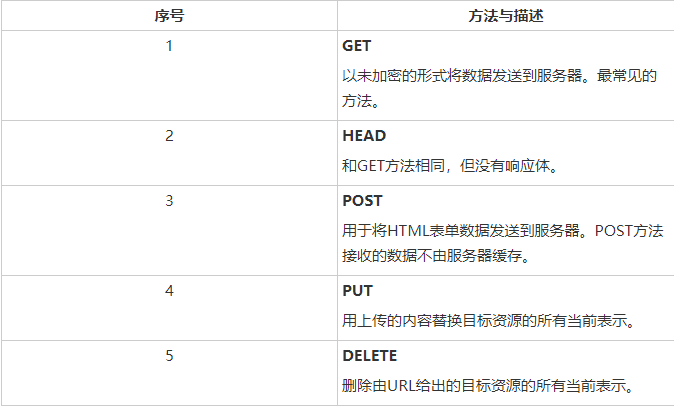
By default, flash routes respond to GET requests. However, you can change this preference by providing method parameters for the * * route() * * decorator:
# ending=utf-8 from flask import Flask, redirect, url_for, request app = Flask(__name__) """ Serial number Method and description 1,GET: Send data to the server in unencrypted form. The most common method. 2,HEAD: and GET The method is the same, but there is no response body. 3,POST: Used to HTML Send the form data to the server. POST The data received by the method is not cached by the server. 4,PUT: Replace all current representations of the target resource with the uploaded content. 5,DELETE: Delete by URL All current representations of the target resource given. """ """ By default, Flask Routing response GET Request. However, you can route()The decorator provides method parameters to change this preference. login()Method coordination login.html File test post/get visit: login method Flask Routing response POST,GET request When login.html in method = "post"When, access login.html And submit the form and get the return: This is"POST"Method to access the page and submit the content: test login.html in method = "get"When, access login.html And submit the form and get the return: This is the method"GET"Visit the page and submit the content: test """ @app.route('/success/<function>,<content>') def success(function, content): return 'This is"{}"Method to access the page and submit the content:{}'.format(function, content) """Judge the request type and pass the request type and form content through url_for Forward to/success Display""" @app.route('/login', methods=['POST', 'GET']) def login(): if request.method == 'POST': text = request.form['nm'] return redirect(url_for('success', function='POST', content=text)) else: text = request.args.get('nm') return redirect(url_for('success', function='GET', content=text)) if __name__ == '__main__': app.run(debug=True)
<html> <body> <form action = "http://localhost:5000/login" method = "post"> <p>Enter Name:</p> <p><input type = "text" name = "nm" /></p> <p><input type = "submit" value = "submit" /></p> </form> </body> </html>
- When login HTML method = "post", access login HTML and enter the content to submit the form to view the returned results:
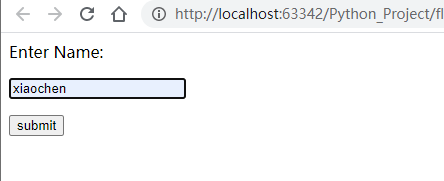
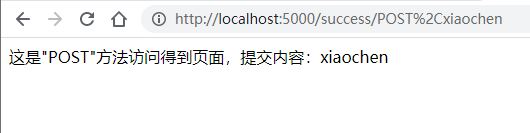
- When login HTML method = "get", access login HTML and enter the content to submit the form to view the returned results:
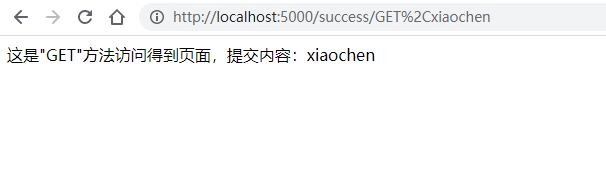
Flash template
Template concept
In large applications, putting business logic and presentation content together will increase code complexity and maintenance cost
- A template is actually a file containing response text, in which placeholders (variables) are used to represent the dynamic part, telling the template engine that its specific value needs to be obtained from the data used
- The process of replacing variables with real values and returning the final string is called 'rendering'
- Flask uses Jinja2 as a template engine to render templates
Benefits of using templates
- The view function is only responsible for business logic and data processing (business logic)
- The template takes the data result of the view function for display (view display)
- Clear code structure and low coupling
Basic use of template
Create a templates folder under the project to store all template files, and create corresponding template files html files in the directory
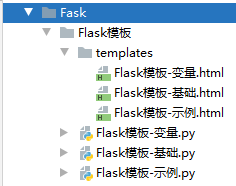
Flash template - Basic py
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('Flask Template-Basics.html') if __name__ == '__main__': app.run()
Flash template - Basic html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> My template html content </body> </html>
Access results:
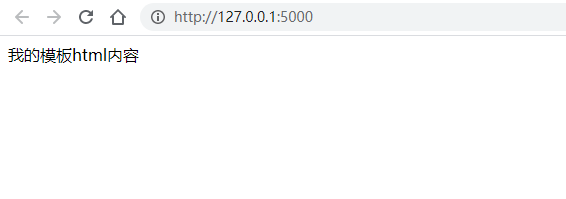
Flash template - variable py
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): # Data transferred into the template my_str = 'Hello Word' my_int = 10 my_array = [3, 4, 2, 1, 7, 9] my_dict = { 'name': 'xiaoming', 'age': 18 } return render_template('Flask Template-variable.html', my_str=my_str, my_int=my_int, my_array=my_array, my_dict=my_dict ) if __name__ == '__main__': app.run()
Flash template - variable html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> My template html content {{ my_str }} {{ my_int }} {{ my_array }} {{ my_dict }} </body> </html>
Access results:
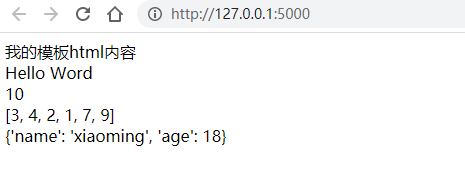
Flash template - example py
from flask import Flask, render_template app = Flask(__name__) """ html The file structure is fixed as a template, and variables are used to occupy the space rander_template Pass in variables for rendering be similar to format Functions, by key=value Incoming mode """ @app.route('/') def index(): # Pre define the content to be transferred into the html template my_int = 18 my_str = 'curry' my_list = [1, 5, 4, 3, 2] my_dict = { 'name': 'durant', 'age': 28 } # render_template method: render template # Parameter 1: template name parameter n: data transferred to the template # The return method can be returned to the browser for access res = render_template('Flask Template-Examples.html', my_int=my_int, my_str=my_str, my_list=my_list, my_dict=my_dict) return res if __name__ == '__main__': app.run(debug=True)
Flash template - example html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <h2>I'm a template</h2> {{ my_int }} <br> {{ my_str }} <br> {{ my_list }} <br> {{ my_dict }} <hr> <h2>Templated list Data acquisition</h2> <hr> {{ my_list[0] }} <br> {{ my_list.1 }} <hr> <h2>Dictionary data acquisition</h2> <hr> {{ my_dict['name'] }} <br> {{ my_dict.age }} <hr> <h2>Arithmetic operation</h2> <br> {{ my_list.0 + 10 }} <br> {{ my_list[0] + my_list.1 }} </body> </html>
Access results:
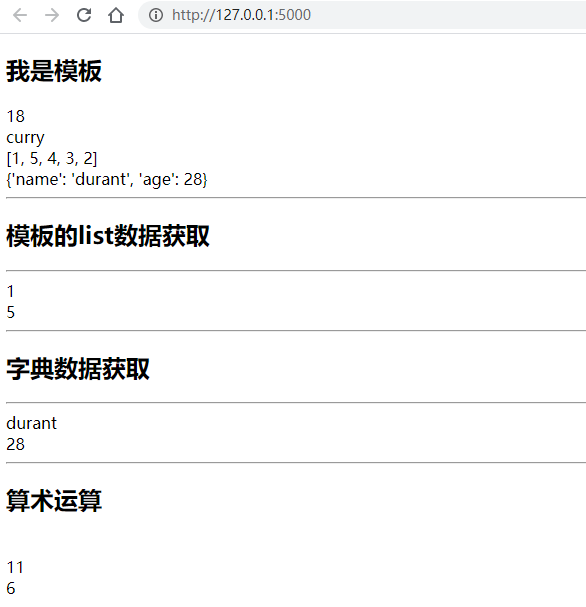
Flash static file
Web applications often require static files, such as javascript files or CSS files that support the display of web pages. Usually, the web server is configured and provides these services for you, but during the development process, these files are from next to your package or module_ static_ Place it in the * * / static provider folder.
In the following example, in index Call hello. HTML on the OnClick event of the HTML button in HTML JS, which is rendered on the "/ * * URL of the flash application.
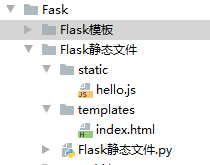
Flash static file py
from flask import Flask, render_template app = Flask(__name__) @app.route("/") def index(): return render_template("index.html") if __name__ == '__main__': app.run(debug = True)
index.html
<html> <head> <!-- Script type="text/javascript";url_for Redirect to static Catalog hello.js file--> <script type = "text/javascript" src = "{{ url_for('static', filename = 'hello.js') }}" ></script> </head> <body> <!-- Input type="button"(Button);corresponding hello.js File function="sayHeelo()";Button display content="Say Hello"--> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </body> </html>
heloo.js
function sayHello() { alert("Hello World") }
Access results:
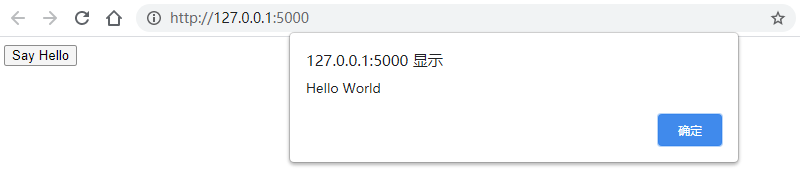
Flash request object
The data from the client web page is sent to the server as a global request object. In order to process the request data, it should be imported from the flash module.
The important attributes of the Request object are listed below:
-
Form - it is a dictionary object that contains key and value pairs for form parameters and their values.
-
**args * * - parse the contents of the query string, which is a question mark (?) Part of the URL after.
-
**Cookies * * - dictionary object that holds Cookie names and values.
-
Files - data related to uploaded files.
-
Method - the current request method.
Flash sends the form data to the template
Request object method
# -*- coding: utf-8 -*- from flask import Flask, redirect, url_for, request import settings app = Flask(__name__) app.config.from_object(settings) @app.route('/index') def index(): print(request.headers, '\n') print(request.path, '\n') print(request.full_path, '\n') print(request.base_url, '\n') print(request.url, '\n') return 'Flask Request Object method' app.run()
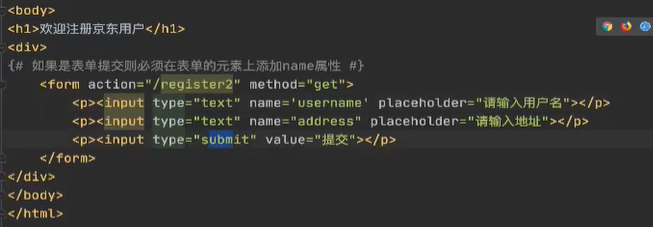
request.args.get() get access value
- If the web page access method is get and there is a form, full_path will carry parameter information to the URL? Format rendering of key = value & key = value
request.form.get() post access value
- If the web page access method is post and there is a form, the front end will return the form object to the back end in dictionary form
Examples
We have seen that the http method can be specified in the URL rule. The * * Form data received by the trigger function can be collected as a dictionary object and forwarded to the template to render it on the corresponding web page.
In the following example, the '/' URL renders a web page with a form (student.html). The filled data will be published to the '/ result' URL that triggers the '/ result() * * function.
**The result() * * function collects the request in the dictionary object Form , and send it to result html.
This template dynamically renders HTML tables of form data.
File structure:
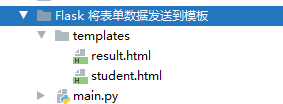
main.py
from flask import Flask, render_template, request app = Flask(__name__) @app.route('/') def student(): return render_template('student.html') @app.route('/result',methods = ['POST', 'GET']) def result(): # Get current request method if request.method == 'POST': # Gets the dictionary object, which contains the key value pair information of form parameters and their values result = request.form return render_template("result.html",result = result) """ Run the program and access by default'/'Page, i.e student.html Form page. student.html After completing the form page, click submit to send the data to'/result'page '/result'URL The bound function is result() therefore result Methods can be request.method Get current request method When request.method == 'POST'When, through request.form Get dictionary object Finally passed render_template Method call'result.html'And pass in result Dictionary for rendered output """ if __name__ == '__main__': app.run(debug = True)
student.html
<form action="http://localhost:5000/result" method="POST"> <p>Name <input type = "text" name = "Name" /></p> <p>Physics <input type = "text" name = "Physics" /></p> <p>Chemistry <input type = "text" name = "chemistry" /></p> <p>Maths <input type ="text" name = "Mathematics" /></p> <p><input type = "submit" value = "submit" /></p> </form>
result.html
<!doctype html> <table border = 1> {% for key, value in result.items() %} <tr> <th> {{ key }} </th> <td> {{ value }}</td> </tr> {% endfor %} </table>
Access results:
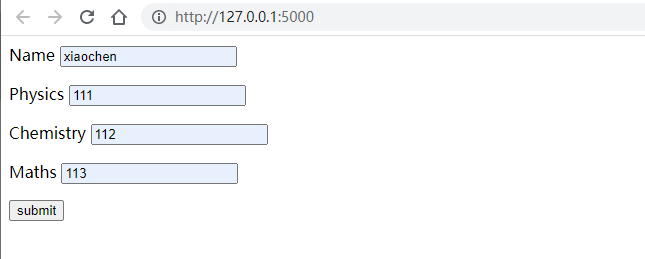
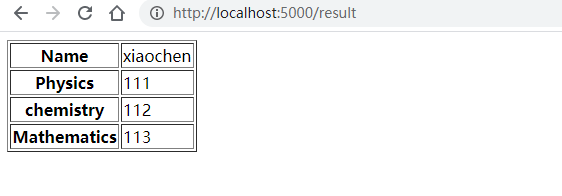
Flask Cookies
Cookie s are stored on the client's computer in the form of text files. Its purpose is to remember and track the data related to customer use, so as to obtain better visitor experience and website statistics.
The Request object contains the properties of the cookie. It is the dictionary object of all cookie variables and their corresponding values, which has been transmitted by the client. In addition, cookies also store the expiration time, path and domain name of their website.
In Flask, the processing steps of cookie s are:
- Set cookie s
""" set up cookie,use key, value, max_age Data format for The default validity period is temporary cookie,The browser fails when it is closed Can pass max_age Set the validity period in seconds """ @app.route("/set_cookies") def set_cookie(): resp = make_response("success") resp.set_cookie("cookie", "xiaochen",max_age=3600) return resp
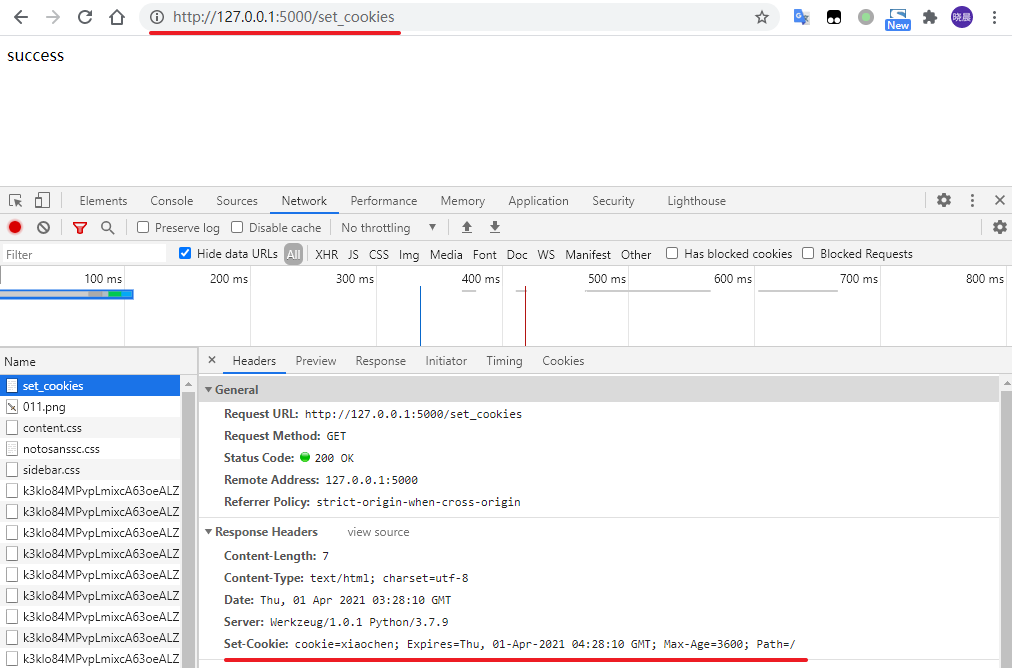
- Get cookie
""" obtain cookie,adopt request.cookies Returns a dictionary, which can obtain the corresponding values in the dictionary """ @app.route("/get_cookies") def get_cookie(): cookie_1 = request.cookies.get("cookie") return cookie_1
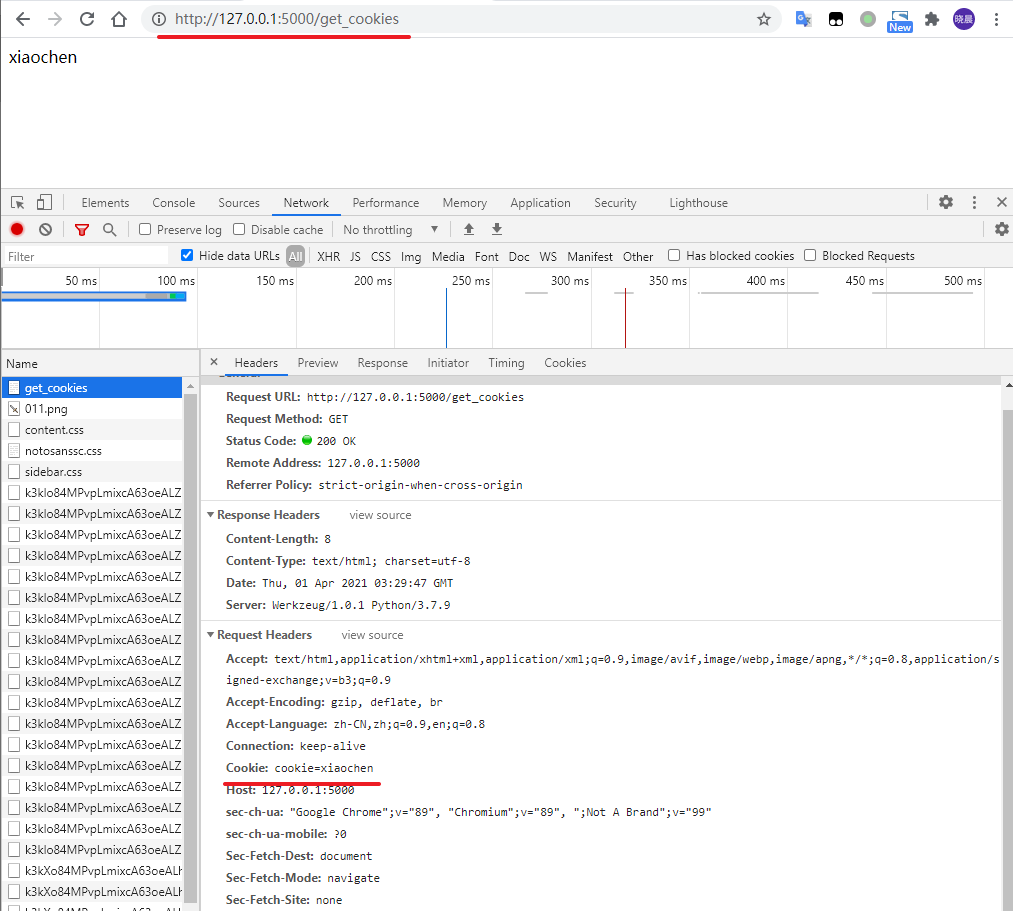
- delete cookie
""" The deletion here just makes cookie Expired, not deleted directly cookie delete cookie,adopt delete_cookie()The way inside is cookie Name of """ @app.route("/delete_cookies") def delete_cookie(): resp = make_response("del success") # Specifies the key specified when setting 'cookie' resp.delete_cookie("cookie") return resp
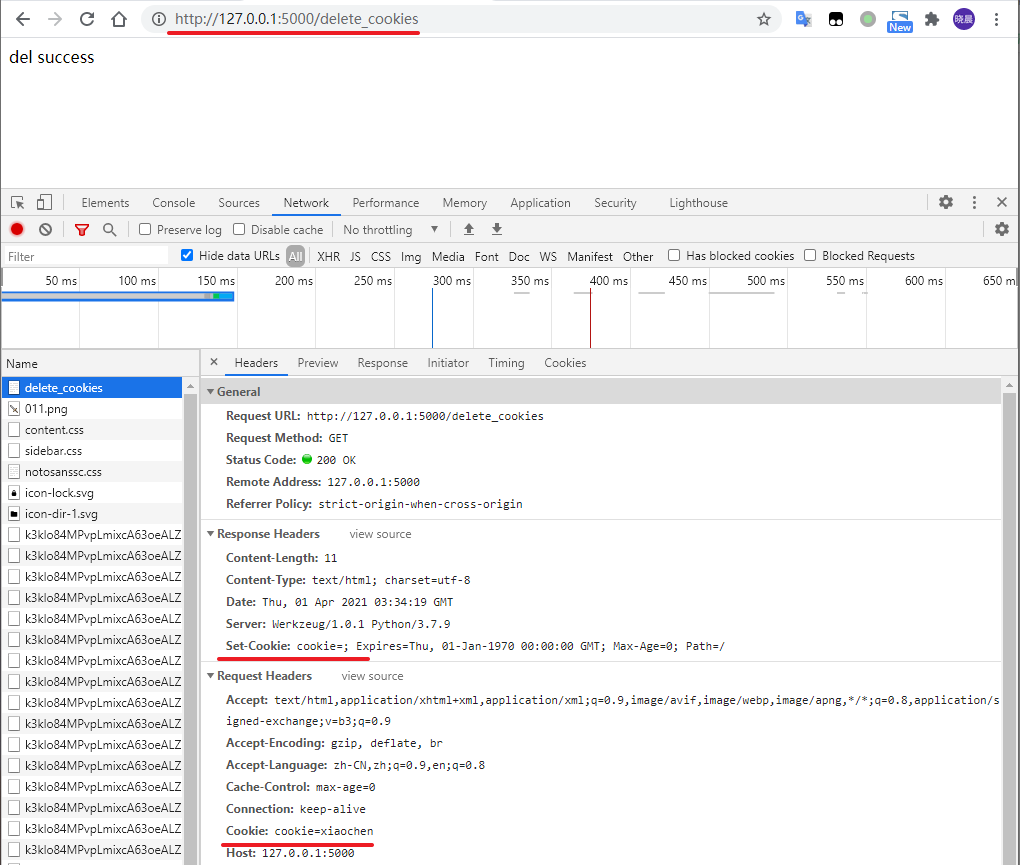
Full code:
from flask import Flask, make_response, request app = Flask(__name__) """ set up cookie,use key, value, max_age Data format for The default validity period is temporary cookie,The browser fails when it is closed Can pass max_age Set the validity period in seconds """ @app.route("/set_cookies") def set_cookie(): resp = make_response("success") resp.set_cookie("cookie", "xiaochen",max_age=3600) return resp """ obtain cookie,adopt request.cookies Returns a dictionary, which can obtain the corresponding values in the dictionary """ @app.route("/get_cookies") def get_cookie(): cookie_1 = request.cookies.get("cookie") return cookie_1 """ The deletion here just makes cookie Expired, not deleted directly cookie delete cookie,adopt delete_cookie()The way inside is cookie Name of """ @app.route("/delete_cookies") def delete_cookie(): resp = make_response("del success") # Specifies the key specified when setting 'cookie' resp.delete_cookie("cookie") return resp if __name__ == '__main__': app.run(debug=True)
Flash session
Unlike cookies, session data is stored on the server. Session is the time interval between a client logging in to the server and logging out of the server. The data that needs to be saved in this session is stored in a temporary directory on the server.
Assign a session ID to each client's session. The session data is stored at the top of the cookie and signed by the server in an encrypted manner. For this application, you need to define a SECRET_KEY.
Define secret_key
app.secret_key = 'fkdjsafjdkfdlkjfadskjfadskljdsfklj'
Setting session variables
The Session object is also a dictionary object that contains key value pairs of Session variables and associated values.
Session['username'] = 'admin'
Release session
session.pop('username', None)
Complete demonstration program and operation interpretation
# ending=utf-8 from flask import Flask, session, redirect, url_for, escape, request """Session Object is also a dictionary object that contains key value pairs of session variables and associated values.""" app = Flask(__name__) """definition secret_key,Signature for session encryption with server""" app.secret_key = 'fkdjsafjdkfdlkjfadskjfadskljdsfklj' """ Visit the home page and'username'Key has not been added to session Dictionary object, so return visitor_status Automatically jump to/login Page, because no data has been submitted at this time, it is still get Access, so return login_form After the user enters the account and clicks login, the data will be returned to/login Page, judge the access method as POST Then pass request.form obtain html Definition in form'username'The value of the key, assigned to session['username'] And pass url_for Forward to index method, index Method judgment'username'Exist, so return login_status login_status Display the login status and provide the logout option. Click the logout option and jump to/logout page logout()Method back through session.pop()Release the session and jump back to index Method """ @app.route('/') def index(): login_status = "Login user name is: {} <br> <b><a href = /logout>Click here to log out</a></b>" visitor_status = "You are not logged in <br><a href = /login></b> Click here to login</b></a>" if 'username' in session: username = session['username'] return login_status.format(username) return visitor_status """It is used to provide the login form and add it to the login form'username'Value assigned to session And jump to index method""" @app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': session['username'] = request.form['username'] return redirect(url_for('index')) login_form = ''' <form action = "" method = "post"> <p><input type ="text" name ="username"/></p> <p><input type ="submit" value ="Sign in"/></p> </form> ''' return login_form """Used to release the session and return the page to index""" @app.route('/logout') def logout(): # remove the username from the session if it is there session.pop('username', None) return redirect(url_for('index')) if __name__ == '__main__': app.run(debug=True)
- Visit the home page and return to the visitor_status
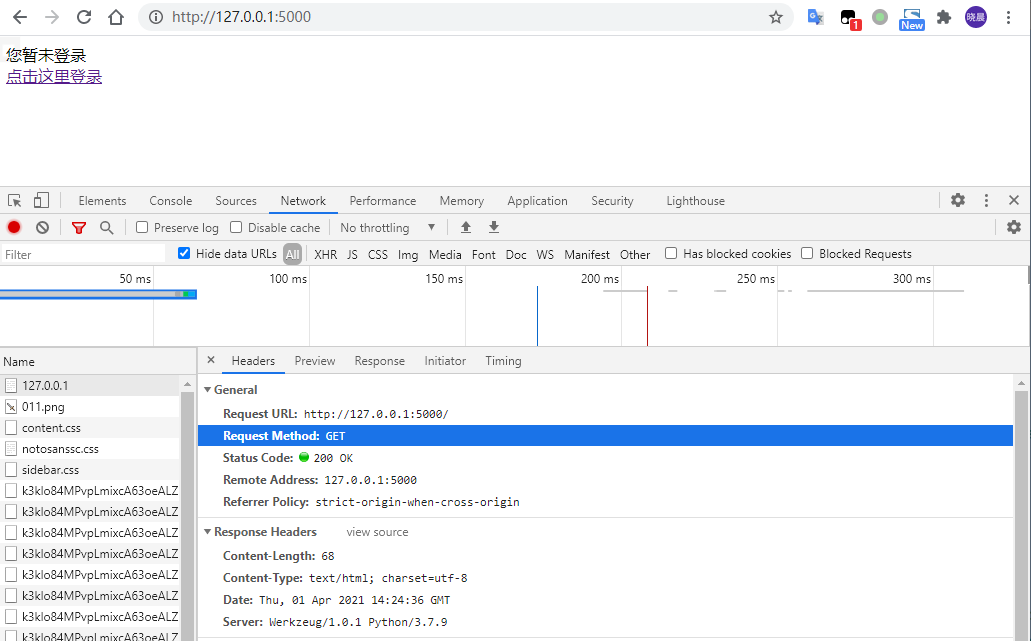
- Click login to jump to / login; Since there is no session for the first time, login is returned_ form
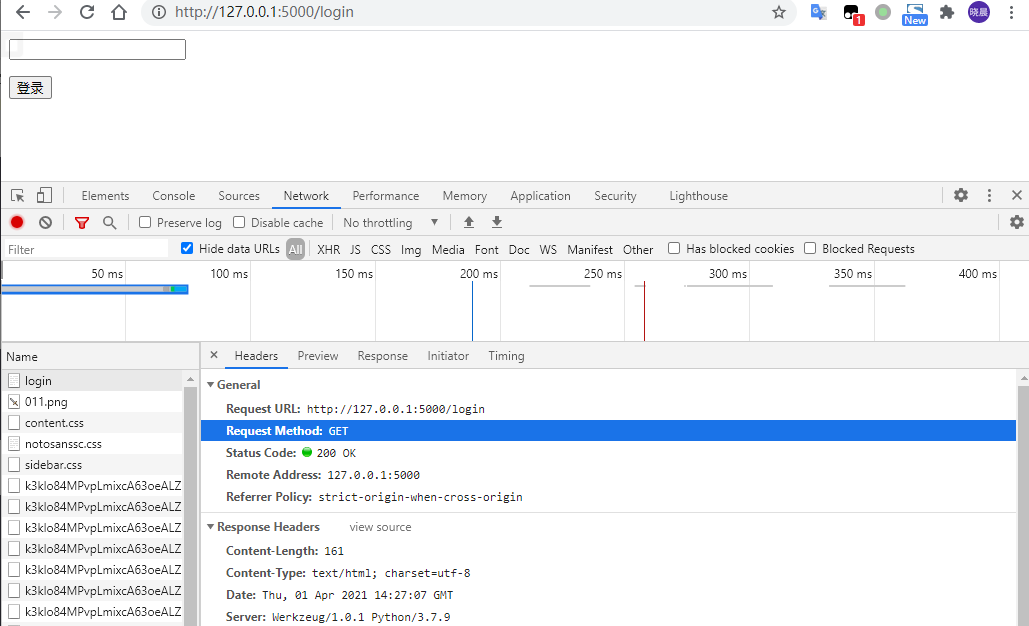
- After clicking login, the request method is changed to POST, and the session and jump index methods are set. Since the session exists, login is returned_ staatus
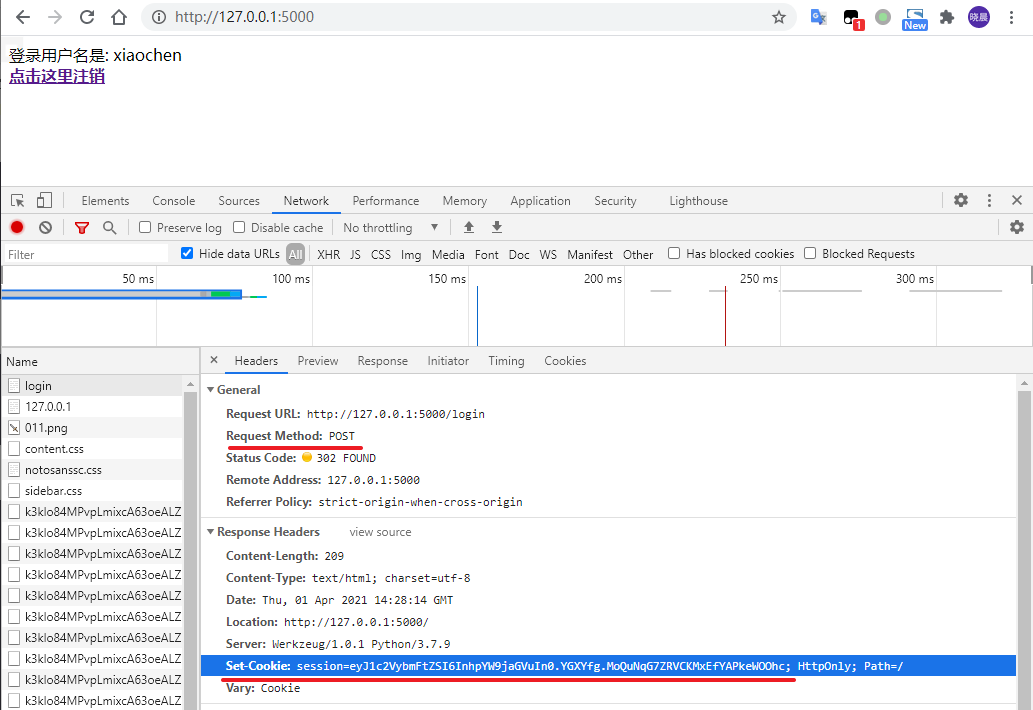
- After clicking log off, release the session and jump to the index method. At this time, the session no longer exists, so return to visitor_status
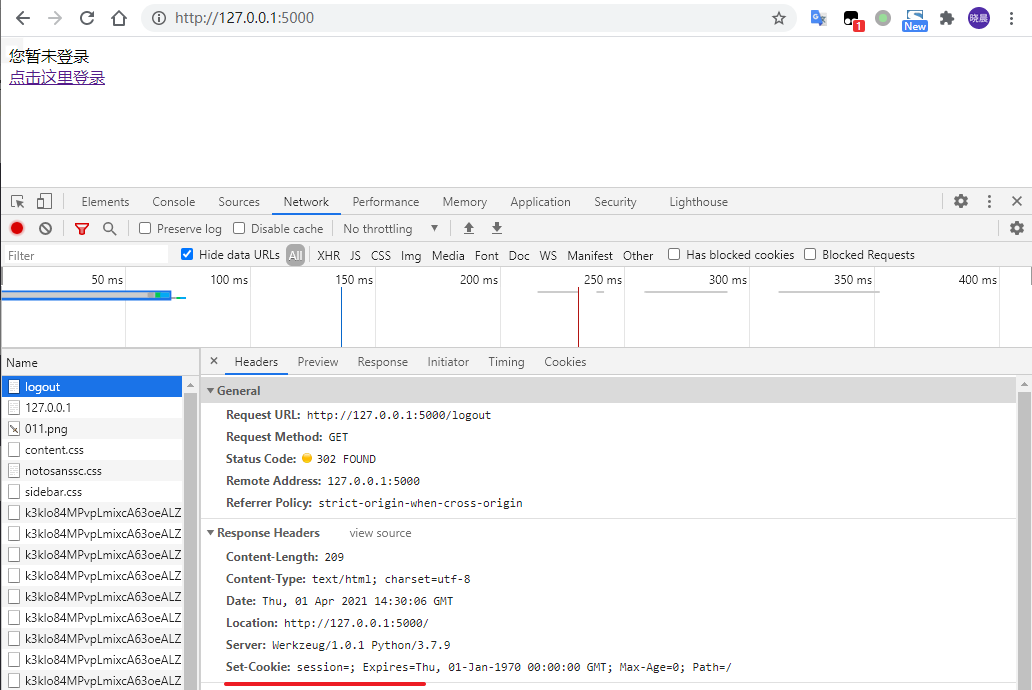
Flash redirection and errors
Flash redirection
The Flask class has a * * redirect() * * function. When called, it returns a response object and redirects the user to another target location with the specified status code.
The redirect() function uses
Flask.redirect(location, statuscode, response)
In the above functions:
-
The location parameter is the URL that should redirect the response.
-
The statuscode is sent to the browser header. The default value is 302.
-
The response parameter is used to instantiate the response.
Status code standardization
The following status codes have been standardized:
- HTTP_300_MULTIPLE_CHOICES
- HTTP_301_MOVED_PERMANENTLY
- HTTP_302_FOUND
- HTTP_303_SEE_OTHER
- HTTP_304_NOT_MODIFIED
- HTTP_305_USE_PROXY
- HTTP_306_RESERVED
- HTTP_307_TEMPORARY_REDIRECT
Redirection example
from flask import Flask, redirect, url_for, render_template, request """call redirect()Function, which returns a response object and redirects the user to another target location with the specified status code.""" # Initialize the Flask application app = Flask(__name__) """ Visit the home page through render_template Method render output login.html login.html The page provides a form for users to enter account information login()Method by obtaining login.html Access mode extraction'username'Value of If the access method is POST And'username'Redirect to if there is a value/success """ @app.route('/') def index(): return render_template('login.html') @app.route('/login', methods=['POST', 'GET']) def login(): if request.method == 'POST' and request.form['username'] == 'admin': return redirect(url_for('success')) return redirect(url_for('index')) @app.route('/success') def success(): return 'logged in successfully' if __name__ == '__main__': app.run(debug=True)
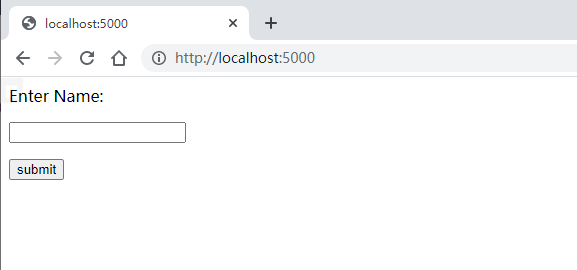
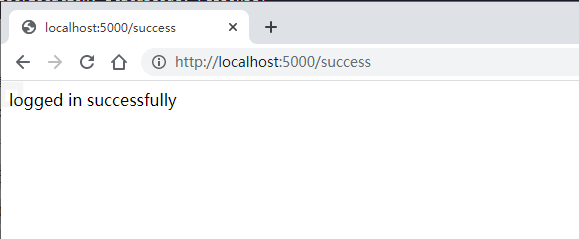
Flash error handling
The abort() method returned an error code
Flask.abort(code)
The Code parameter takes one of the following values:
-
**400 * * - for error requests
-
**401 * * - for unauthenticated
-
**403 **- Forbidden
-
**404 * * - not found
-
**406 * * - not acceptable
-
**415 * * - for unsupported media types
-
**429 * * - too many requests
Return error code example
from flask import Flask, redirect, url_for, render_template, request, abort app = Flask(__name__) @app.route('/') def index(): return render_template('log_in.html') @app.route('/login',methods = ['POST', 'GET']) def login(): if request.method == 'POST': if request.form['username'] == 'admin' : return redirect(url_for('success')) else: abort(401) else: return redirect(url_for('index')) @app.route('/success') def success(): return 'logged in successfully' if __name__ == '__main__': app.run(debug = True)
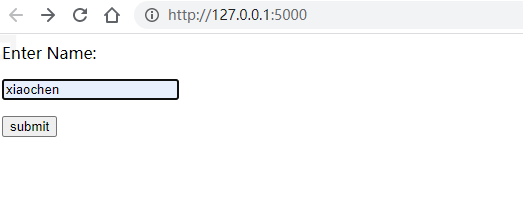
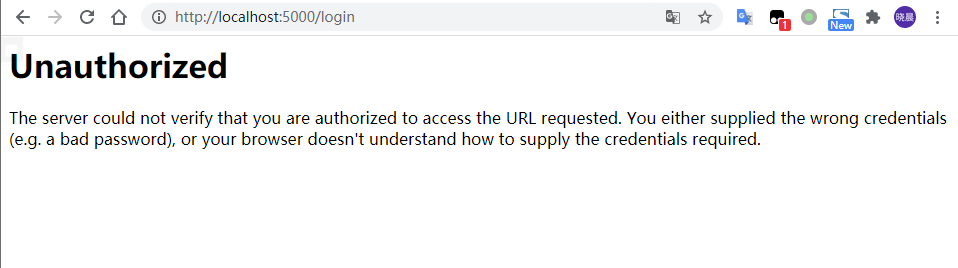