I. Integer int
Meaning: Integers are numbers without decimal parts. Including natural number, 0 and negative number.
For example: 100-5
In Python 3, there is only one type of integer, independently of integers and long integers. Using Python's built-in function type, you can see the data type that the variable refers to. Python integer types are different from those expressed in other languages. The range of integer values in other languages is related to machine digits. For example, on 32-bit machines, the range of integer values is - 2 ^ 31 to 2 ^ 31, and on 64-bit machines, the range of integer values is - 2 ^ 63 to 2 ^ 63. Python integers can represent values only depending on the size of memory supported by the machine, that is, Pyhton can represent large numbers, which can exceed the range of values represented by machine digits.
1. Representation of literal values of integers:
a. decimal representation:
10 200 9999999 9999999999999999999999999999
B. Binary representation: (0b begins, followed by 0 and 1)
0b111
0b101
0b1111111111
c. Octal representation: (0o begins, followed by 0-7)
0o177 #127 0o11 #9
d. Hexadecimal representation: (0x at the beginning, followed by 0~9, A~F, a~f)
0x11 #17 0xFF #255 0x1234ABCD
2. Constructors of Integers
int(x,base=10) #base Representation is decimal,The default is 10 int(x=0) #Convert a number or string to an integer and return 0 if no parameters are given
2. Floating-point float
Floating-point numbers are numbers with decimal parts.
1. Expressions:
a. In decimal form:
3.14 3.1 3. 0.14 .14
b. Scientific counting:
#decimal+e/E+Positive and negative sign (positive sign can be omitted)+index 6.18E-1 2.9979e8
2. Constructing Functions of Floating Points
float(obj) #Converts a string or number to a floating point number and returns 0 if no parameters are given
3. complex
Virtual numbers cannot exist alone. They always form a complex with a real part with a value of 0.0. Complex numbers are divided into two parts, real part + imaginary part (image), and imaginary part ends with J or J.
1. Literal value:
1j (2j) 1+1J 3-4J (-100+100J)
2. The constructor of complex numbers:
complex(r=0.0, i=0.0) #Create a complex number with numbers(Real part is r, Imaginary part i)
4. Boolean type bool
Types used to represent true and false States
True denotes truth (condition satisfies or holds)
False denotes false (condition is not satisfied or not valid)
Explain:
- True has a value of 1
- The value of False is 0.
Constructor of bool type
bool(x) #use x Create a Boolean Value(True/False)
The case of bool(x) returning false values
None #Null value False #Boolean false value 0 #Integer 0 0.0 #Floating point 0 0j #Plural 0 '' #Empty string () #Empty tuple [] #Null list {} #Empty dictionary set() #Empty set
frozenset() #Empty fixed set
b'' #Empty byte string
bytearray(b'') #Null byte array
V. Null-valued None objects
Explain:
- None is a special object that represents nonexistence
- None is different from False.
- None is not zero.
- None is not an empty string.
- None and any other data type are always returned to False.
- None has its own data type, NoneType.
- You can assign None to any variable, or any variable to a None worthy object, but you cannot create other NoneType objects.
Effect:
- Used for occupying
- Used to unbind variables
6. String str
Effect:
Used to record text (text) information
Explain:
In non-commentary, all parts enclosed in quotation marks are strings
Types of quotation marks
Single quotes
"Double quotation marks"
'''Three single quotation marks
"""triple double quotation marks"
1. Representation of strings:
a. The literal representation of empty strings
'' "" '''''' """"""
b. Literal representation of non-empty strings
'hello world' "hello world" '''hello world''' """hello world"""
The difference between single quotation mark and double quotation mark:
- Double quotation marks in single quotation marks are not terminators
- Single quotation marks in double quotation marks are not terminators
Three quotation mark strings:
Effect:
Line breaks in triple quote strings are automatically converted to line breaks'n'
Three quotation marks can contain single and double quotation marks.
Use of three quotation mark strings:
#Single quotation mark 'welcome to beijing\nwelcome to beijing\nI am studing!' #Double quotation marks "welcome to beijing\nwelcome to beijing\nI am studing!" #triple quotes s='''welcome to beijing welcome to beijing! I am studing!'''
2. Representing special characters with escape sequences
In the literal value of a string, a character is represented by a character backslash () followed by some characters.
Escaped Character Table in Strings
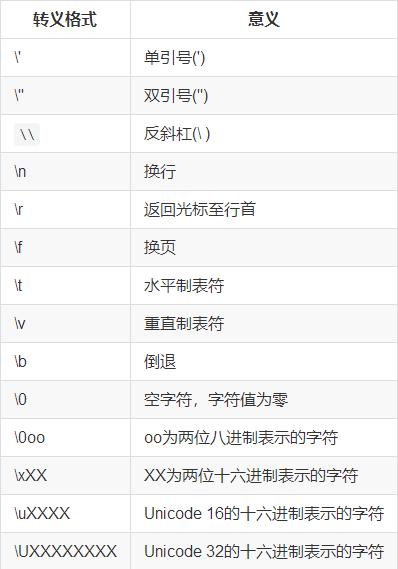
raw string (original string)
Effect:
Invalidate escaped characters
Literal format:
r'String content' r"String content" r'''String content''' r"""String content"""
Example:
a='C:\newfile\test.py' print(a) print(len(a)) #Get the length of the string a=r'C:\newfile\test.py' print(a) print(len(a)) #Get the length of the string
3. String operation:
a. Arithmetic operators:
+ += * *=
+ Plus Operator for String Mosaic
Example:
x = 'abcd' + 'efg' print(x) # abcdefg x += '123' print(x) # abcdefg123
* Operators are used to generate duplicate strings
x = '123' y = x * 2 # y = '123123' x *= 3 # x = '123123123' x = 3 x *= '123' # x = '123123123'
b. Comparisons of Strings
Operator:
> >= < <= == !=
Example:
'A' < 'B' # True 'B' < 'a' # True 'ABC' > 'AB' # True 'AD' < 'ABC' # False 'ABC' == 'abc' # False
C.in/not in operator
Effect:
In is used in sequences, dictionaries, collections, to determine whether a value exists in a container, to return True if it exists, or to return False if it does not.
Format:
Object in container
Example:
s = 'welcome to tarena!' 'to' in s # True 'weimingze' in s # False
4. index Index
The python string str is a sequence of characters that cannot be changed.
Index grammar:
String [integer expression]
Explain:
- python sequences can use indexes to access objects (elements) in the sequence
- The forward index of python sequence starts at 0, the second index is 1, and the last index is len(s) -1.
- The reverse index of python sequence starts with - 1, - 1 represents the last, - 2 represents the penultimate, and the first is - len(s)
Example:
s = 'ABCDE' print(s[0]) # A print(s[1]) # B print(s[4]) # E
5. slice
Effect:
Re-compose a string by extracting a part of the corresponding elements from the string sequence
Syntax:
String [(Beginning Index b): (Ending Index e)(: (Step Size s)] Note: () The part enclosed in () can be omitted.
Explain:
- The start index is where the slice begins to cut 0 for the first element and - 1 for the last element.
- The end index is the end index of the slice (but does not include the end index)
- Step size is the direction and offset of the slice moving after each retrieval of the current index
Explanation of step size:
- There is no step size, which is equivalent to moving an index backwards after the value has been taken (default is 1)
- When the step size is positive integer, take forward slice:
- The default step size is 1, the default start index is 0, and the default end index is len(s).
- When the step size is a negative integer, take the reverse slice:
- In reverse slicing, the default starting position is the last element and the default ending position is the previous position of the first element.
Example:
s = 'ABCDE' a = s[1:4] # a -> 'BCD' a = s[1:] # a -> 'BCDE' a = s[:2] # a -> 'AB' a = s[:] # a -> 'ABCDE' a = s[4:2] # a -> '' a = s[2:10000] # a -> 'CDE' Start index/End index crosses boundaries a = s[::2] # a -> 'ACE' a = s[1::2] # a -> 'BD' a = s[::-1] # a -> 'EDCBA' a = s[::-2] # a -> 'ECA' a = s[4:0:-2] # a -> 'EC'
6. Common string methods
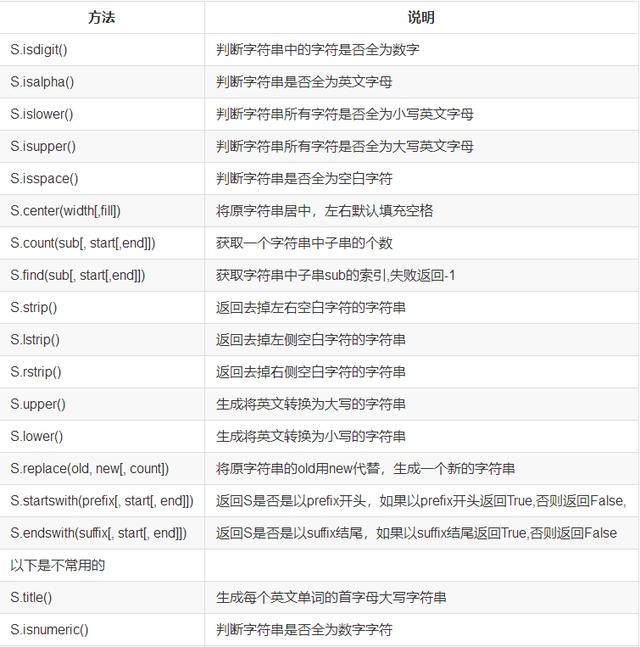
Blank character
It refers to invisible characters such as spaces, horizontal tabs (\t), newline characters (\n).
String text parsing method
#Use strings sep Segmentation as a delimiter s Character string,Returns a list of partitioned strings, #When no parameters are given,Segmentation using blank characters as separators s.split(sep=None) #Connect Strings in Iterable Objects,Return to an intermediary s Segmented strings s.join(iterable)
Example:
s = 'Beijing is capital' L = s.split(' ') # L = ['Beijing', 'is', 'capital'] s = '\\' L = ['C:', 'Programe files', 'python3'] s2 = s.join(L) # s2 = b'C:\Programe files\python3'
7. Several functions of a string:
a. Constructor of strings
str(obj='') #Converting objects to strings
Example:
s = 123 print(str(s) + '456') # 123456
b. String Coding Conversion Function
ord(c) #Returns a string of Unicode Coding value chr(i) #Return i The character corresponding to this value
Example:
print(ord('A')) # 65 print(ord('in')) #
c. Converting integers to string functions
hex(i) #Converting integers to hexadecimal strings
oct(i) #Converting integers to octal strings
bin(i) #Converting integers to binary strings
8. String Formatting Expressions
Operator:
%
Effect:
Generate strings in the desired format
Syntax:
- Format string% parameter value
- Format string%(parameter value 1, parameter value 2,...)
The% in the format string is a placeholder, and the placeholder position will be replaced by the parameter value.
Placeholders and type codes in formatted strings:
Format grammar between placeholders and type codes:
% [Format grammar] type code
Format grammar:
- Left alignment
- + Display positive sign
- 0 zero fill
- Width (integer)
- Width. Accuracy (integer)
Example:
'%10d' % 123 #' 123' '%-10d' % 123 #'123 ' '%10s' % 'abc' #' abc' '%-5s' % 'abc' #'abc ' '%-5d' % 123 #'00123' '%7.3f' % 3.1415926 #'003.142'
list:
Explain:
- Lists are made up of a series of specific elements. There is no correlation between elements, but there is a sequence between them.
- Lists are containers
- List is a kind of sequence
- Lists are sequences that can be changed
sequence in python
1. String str
2. list list
3. tuple
4. bytes
5. byte array bytearray
1. Create literal values for empty lists:
L=[] #L Binding an empty list
2. Create a non-empty list:
L=[1,2,3,4] L=["Beijing","Shanghai","Tianjin"] L=[1,'two',3,'Four'] L=[1,2,[3.1,3.2,3.3],4]
3. list Construction (Creation) Function list
#Generate an empty list, equivalent to[] list() #Create a list with iteratable objects list(iterable)
Example:
L=list() #L An empty list, equal to[] L=list("ABCD") # L->['A','B','C','D'] L = list(range(1, 10, 2))
4. Operation of lists:
a. Arithmetic operations
+ += * *=
+ For splicing lists
x = [1,2,3] y = [4,5,6] z = x + y # z = [1,2,3,4,5,6]
+= For splicing the original list with the left Iterable object to generate a new list
x = [1,2,3] x += [4,5,6] # x = [1,2,3,4,5,6] x = [1,2,3] x += 'ABC' # += The right side must be an iterative object
* Generate duplicate lists
x = [1,2,3] * 2 # x = [1,2,3,1,2,3]
*= Used to generate duplicate lists while binding new lists with variables
x = [1, 2] x *= 3 # x = [1,2,1,2,1,2]
b. Comparisons of lists:
Operator:
< <= > >= == !=
Example:
x = [1,2,3] y = [2,3,4] x != y # True x > [1,2] # True x < y # True [1,3,2] > [1,2,3] # True ['AB', 'CD'] > ['AC', 'BD'] # False [1, 'two'] > ['two', 1] # TypeError
c. in / not in the list
Explain:
- Determine whether a data element exists in a container (list), return True if it exists, or False if it exists
- The return value of not in is the opposite of the in operator
Example:
x = [1, 'Two', 3.14, 'Four'] 1 in x # True 2 in x # False 3 not in x # True 'Four' not in x # False
5. Index index of list
Index grammar of lists:
List [Integer Expressions]
Usage:
The index values of lists are identical to those of strings
The index of the list is divided into forward index and reverse index.
Example:
L = ['A', 2, 'B', 3] print(L[1]) # 2 x = L[2] # x = 'B'
Index assignment of lists
Lists are variable sequences, and elements in lists can be changed by index assignment
Syntax:
List [index]= expression
Example:
x = [1,2,3,4] x[2] = 3.14 # Change the value of the third element
6. Slices of lists
Syntax:
List [:]
The [:]
The slicing value of a list returns a list with rules equal to the slicing rule of a string.
Example:
x = list(range(9)) y = x[1:9:2] # y = [1,3,5,7]
Slice assignment syntax for lists:
List [Slice] = Iterable Objects
Explain:
The right side of the slice assignment operator must be an iterative object
Example:
L = [2,3,4] L[0:1] = [1.1, 2.2] print(L) # [1.1, 2.2, 3, 4] L = [2,3,4] L[:] = [7,8] L = [2,3,4] L[1:2] = [3.1, 3.2, 3.3] # [2,3.1, 3.2, 3.3,4] L = [2,3,4] L[1:1] = [2.1, 2.2] # [2, 2.1, 2.2, 3, 4] L = [2,3,4] L[0:0] = [0, 1] # L=[0, 1, 2,3,4] L = [2,3,4] L[3:3] = [5,6] # L=[2,3,4, 5,6] L = [2,3,4] L[1:2] = []
Slices whose slice step is not 1 are assigned:
L = list(range(1, 9)) L[1::2] = [2.2, 4.4, 6.6, 8.8] print(L) # [1, 2.2, 3, 4.4, 5, 6.6, 7, 8.8]
Notes for slicing:
For slice assignment with step size not equal to 1, the number of elements provided by the iterative object on the right side of the assignment operator must be equal to the number of segments sliced out.
Such as:
L = [1,2,3,4,5,6] L[::2] = 'ABCD' # Wrong L[::2] = 'ABC' # Right.
7.del statement for deleting list elements
Syntax:
del list [index]
del list [slice]
Example:
L = [1,2,3,4,5,6] del L[0] # L = [2,3,4,5,6] del L[-1] # L = [2,3,4,5] L = [1,2,3,4,5,6] del L[::2] # L = [2, 4, 6]
8. Commonly used list methods
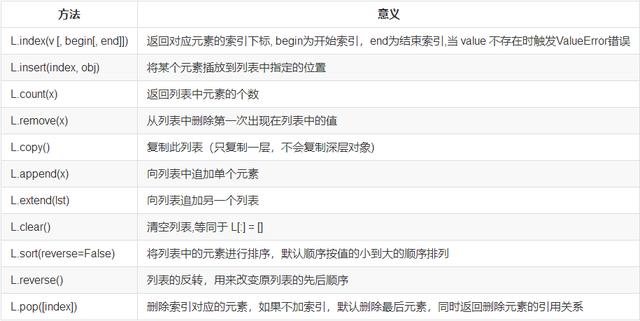
tuple
Explain:
Tuples are immutable sequences. Like list s, tuples can store elements of any type. Once tuples are generated, they cannot be changed.
Tuple representation: enclosed in parentheses (), individual elements are enclosed in commas (,) to distinguish between a single object and a tuple
1. Representation of tuples
a. Create character values for empty tuples
t = ()
b. Create literal values for non-empty tuples
t = 200, t = (20,) t = (1,2,3) t = 100, 200, 300
c. Error examples of tuples:
t = (20) # t Bound integer x, y, z = 100, 200, 300 # Sequential assignment x, y, z = 'ABC' x, y, z = [10, 20, 30]
2. tuple, the constructor of tuples
#Generate an empty tuple, equivalent to() tuple() #Generating a tuple with an iterative object tuple(iterable)
Example:
t = tuple() t = tuple(range(10)) t = tuple('hello') t = tuple([1,2,3,4])
3. Arithmetic operations of tuples:
Arithmetic oper a tions:
+ += * *=
The usage is exactly the same as that of lists.
b. Comparisons of tuples:
< <= > >= == !=
Rules are exactly the same as lists
c.in/ not in
Rules are exactly the same as lists
4. Index Value
Rules are exactly the same as lists
Difference:
Tuples are immutable objects and do not support index and slice assignments
5. Slice Value
Rules are exactly the same as lists
Difference:
Tuples are immutable objects and do not support index and slice assignments
6. Tuple method:
#Used to retrieve tuples v Location of index T.index(v[,begin[,end]]) #Used to retrieve tuples v Number of T.count(v) #The above methods are the same. list Medium index,count Method
Example:
for x in reversed("ABCD"): print(x) # D C B A L = [8, 6, 3, 5, 7] L2 = sorted(L) print(L2) # [3, 5, 6, 7, 8] L3 = sorted(L, reversed=True) print(L3) # [8, 7, 6, 5, 3] print(L) # remain unchanged
Dictionary dict
Explain:
- A dictionary is a variable container that stores any type of data.
- Every data in a dictionary is indexed by a'key', unlike a sequence that can be indexed by subscripts.
- The data of dictionary is not sequential, and the storage of dictionary is disordered.
- Data in a dictionary is mapped to key-value storage
- The keys of a dictionary cannot be repeated and can only be used as keys of a dictionary with invariant types.
1. The literal representation of dictionaries:
Enclosed with {}, separate key-value pairs with colons (:), and separate key-value pairs with semicolons
a. Create an empty dictionary
d = {}
b. Create a non-empty dictionary:
d = {'name': 'tarena', 'age': 15} d = {'Full name': 'Xiao Zhang'} d = {1:'One', 2:'Two'}
2. dictionary constructor dict
dict() #Creating an empty dictionary is equivalent to {}
dict(iterable) #Initialization of a dictionary with iterative objects
dict(**kwargs) #Generating a dictionary by keyword parametric form
Example:
d = dict() d = dict([('name', 'tarena'), ('age',15)]) d = dict(name='tarena', age=15)
3. Key Index of Dictionary
Using the [] operator, we can get the'value'corresponding to the'key' in the dictionary.
Syntax:
DICTIONARY [key]
a. Get data elements:
d = dict(name='tarena', age=15) print(d['age']) # 15
b. Add/modify dictionary elements
Dictionary [key] = expression
Example:
d = {} d['name'] = 'tarena' # Create a new key-value pair d['age'] = 15 # Create key-value pairs d['age'] = 16 # Modify key-value pairs
4.del statement deletes dictionary elements
Syntax:
del dictionary [key]
Example:
d = {'name': 'china', 'pos': 'asia'} del d['pos'] print(d) del d['name'] print(d) # {}
5. In/not in operator of dictionary
The in operator can be used to determine whether a'key'exists in a dictionary, and if it exists, it returns True or False.
not in is the opposite of in return value
Example:
d = {'a': 1, 'b': 2} 'a' in d # True 1 in d # False 100 not in d # True 2 not in d # True
6. Iterative access to dictionaries:
Dictionaries are iterative objects, and dictionaries can only iteratively access keys.
d = {'name': 'tarena', (2002, 1, 1): 'Birthday'} for x in d: print(x)
7. Dictionary Method
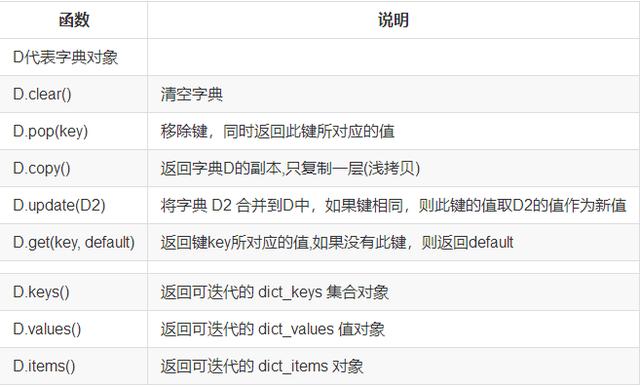
set Set
Explain:
- Sets are variable containers
- Data objects in a collection are unique (not repeated many times)
- A set is an unordered storage structure, and the data in the set is not sequential.
- Elements in a collection must be immutable objects
- Sets are iterative
- A collection is equivalent to a dictionary with only keys and no values (keys are the data of the collection)
1. The expression of a set:
a. Create empty collections
s = set() # set() Create an empty collection
b. Create non-empty collections
s = {1, 2, 3} # Three integers 1 in a set,2,3
2. Constructor set of sets
set() #Create an empty collection
set(iterable) #Create a new collection object with iteratable objects
Example:
s = set("ABC") s = set('ABCCBA') s = set({1:"One", 2:'Two', 5:'Five'}) s = set([1, 3.14, False]) s = set((2,3,5,7)) # s = set([1, 2, [3.1, 3.2], 4]) # Wrong [3.1, 3.2] is a variable object
3. Operation of sets:
A. & Generate the intersection of two sets
s1 = {1,2,3} s2 = {2,3,4} s3 = s1 & s2 # s3 = {2, 3}
b. | Generate the union of two sets
s1 = {1,2,3} s2 = {2,3,4} s3 = s1 | s2 # s3 = {1,2,3,4}
c. - Generating complements of two sets
s1 = {1,2,3} s2 = {2,3,4} # Generation belongs to s1,But it does not belong. s2 A collection of all elements s3 = s1 - s2
d. ^ Generates symmetric complements of two sets
s1 = {1,2,3} s2 = {2,3,4} s3 = s1 ^ s2 # s3 = {1, 4} # Equate to s3 = (s1 - s2) | (s2 -s1)
E. <Judging that one set is a subset of another
F. > Judging that a set is a superset of another set
s1 = {1, 2, 3} s2 = {2, 3} s2 < s1 # True Judgement subset s1 > s2 # True Judgement superset
g. ===!= The set is the same/different
s1 = {1, 2, 3} s2 = {2, 3, 1} s1 == s2 # True s1 != s2 # False # The data of a collection is not sequential
H.in/not in operator
Explain:
Equivalent to a dictionary, the in operator is used in a collection. When a value exists in a collection, it is true, otherwise it is false.
not in is the opposite of in return value
Example:
s = {1, 'Two', 3.14} 1 in s # True 2 in s # False 3.14 not in s # False 4 not in s # True
4. The method of collecting:
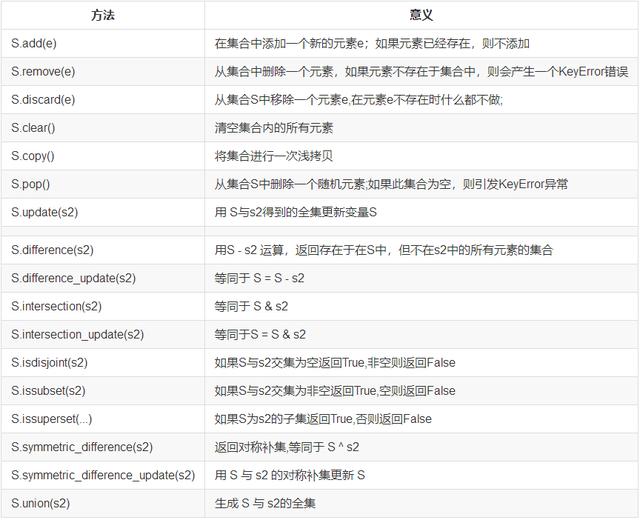
XI. frozenset of Fixed Sets
Explain:
Fixed sets are immutable, disordered, and contain unique elements
Effect:
Fixed sets can be used as keys in a dictionary or as values (elements) of a set.
1. Expressions of Fixed Sets
a. Create empty fixed sets
fs = frozenset()
b. Create non-empty fixed sets
fs = frozenset([2,3,5,7])
2. Constructor:
frozenset() frozenset(Iterable object) # with set Function consistency,Returns a fixed set
3. Operation of fixed sets:
Operational rules are equivalent to usage in set
4. Fixed set method:
All methods equivalent to a set remove methods that modify the set
12. bytes
Effect:
Storing data in bytes
Explain:
- Byte string is an immutable sequence of bytes
- Bytes are integers between 0 and 255
1. Expressions of byte strings
a. Create literal values for empty byte strings
b'' b"" b'''''' b"""""" B'' B"" B'''''' B""""""
b. Create literal values for non-empty byte strings:
b'ABCD' b'\x41\x42' b'hello tarena'
2. bytes, the constructor of byte strings
bytes() #Generating an empty byte string is equivalent to b'' bytes(Integer Iterable Objects) #Initialize a byte string with an iterative object bytes(integer n) #Generate n byte strings with zero values bytes(Character string, encoding='utf-8') #Generating a byte string with string conversion encoding
3. Operation of byte strings
Operational rules are equivalent to usage in str
Example:
b = b'abc' + b'123' # b=b'abc123' b += b'ABC' # b=b'abc123ABC' b'ABD' > b'ABC' # True b = b'ABCD' 65 in b # True b'A' in b # True
4. The difference between bytes and str:
- Bytes storage bytes (0-255)
- str stores Unicode characters (0-65535)
bytes and str conversion
a. Encoding
str ------> bytes
b = s.encode('utf-8')
b. Decode
bytes ------> str
s = b.decode('utf-8')
13. byte array bytearray
Variable byte sequence
1. Create constructors for byte arrays:
bytearray() creates an empty byte array Bytearray (integer) Bytearray Bytearray (string, encoding='utf-8') # The above parameters are equivalent to byte strings
2. Operation of byte arrays:
Byte arrays support index and slice assignment with the same rules as lists
3. By tearray:
B.clear() #Empty byte array B.append(n) #Append a byte(n For 0-255 Integer) B.remove(value) #Delete the first byte that appears, and if it does not, produce a ValueError error B.reverse() #Reverse the order of bytes B.decode(encoding='utf-8') # Decode B.find(sub[, start[, end]]) #lookup