chart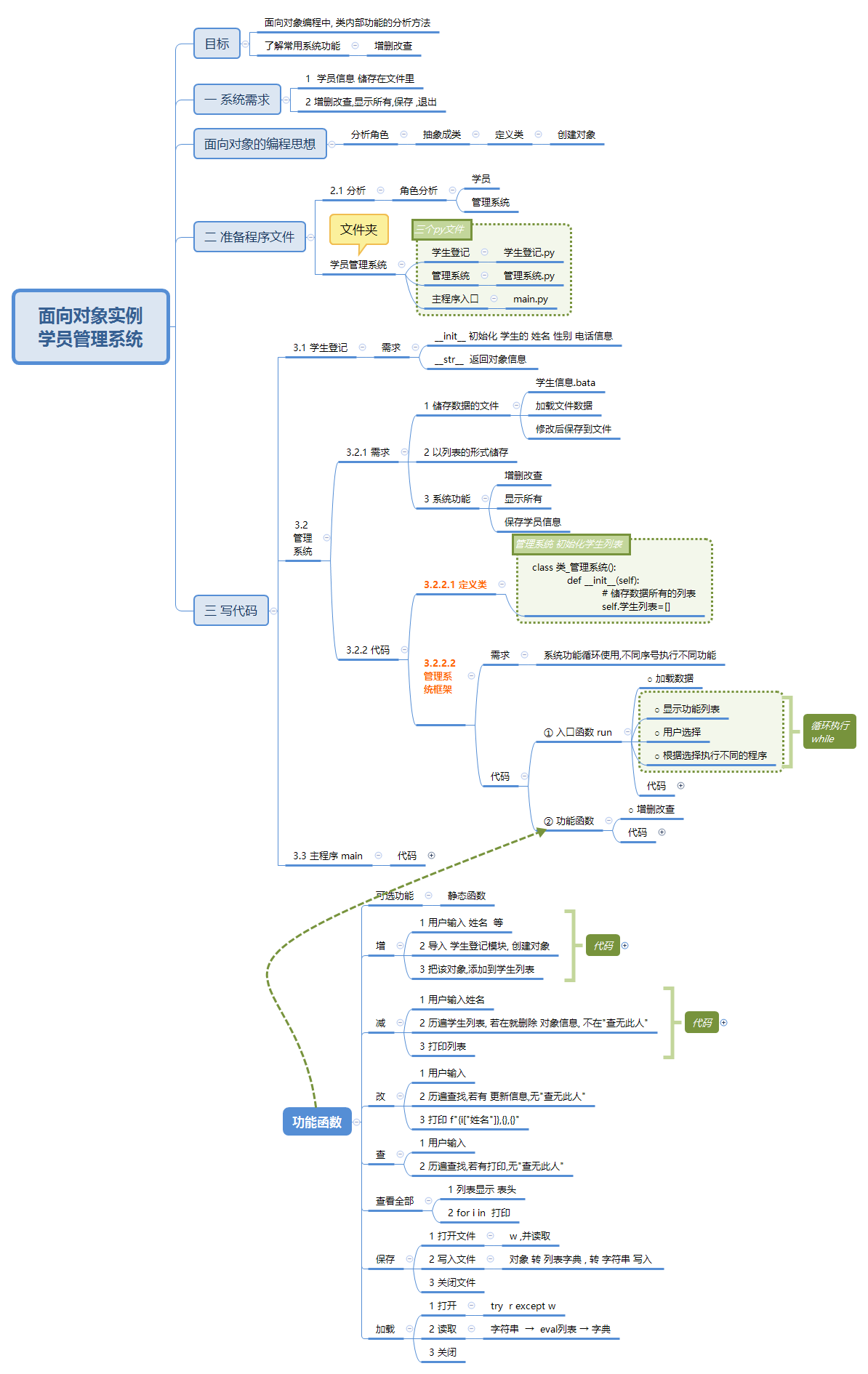
target
- Understand the analysis method of class internal function in the process of object-oriented programming development
- Understand common functions, add, delete, modify and query
I. system requirements
Use the object-oriented programming idea to complete the development of student management system, as follows
- System requirements: student data is stored in files
- System functions: ① add, delete, modify and check student information, ② display all student information, save student information, ③ exit the system and other functions
Object oriented programming idea: analyzing roles, abstracting them into classes, defining classes, and creating objects
II. Preparation of procedure documents
2.1 analysis
- Role analysis
- student
- management system
Precautions at work
1. In order to facilitate code maintenance, generally one role and one program file
2. The project shall have a main program entry, which is used to main py
2.2 creating program files
① Create a project directory, such as Student management system
② Create program file
- Program entry file: main py
- Student file: student registration py
- Management system files: management system py
III. writing procedure
3.1 student registration py
Requirements:
- Student information includes: Name Gender mobile number
- Add__ str__ Magic function, easy to view student object information
3.1.2 program code
class Student registration(): def __init__(self,full name,Gender,mobile phone): # Name Gender telephone self.full name=full name self.Gender=Gender self.mobile phone=mobile phone def __str__(self): # The destination output is not the memory address, but the specific content return f"{self.full name},{self.Gender},{self.mobile phone}" # Create an object to temporarily verify the above code # Xiao Ming = Student("Xiao Ming", "nv",17753355555) # Print (Xiao Ming) """Xiao Ming,nv,17753355555"""
3.2 management system py
Requirements:
- Where to store data: File student information data
- Load file data
- Save modified data to file
- Form of data storage: list storage of student objects
- system function
- Add, delete, modify and query
- Display all student information
- Save student information
3.2.1 definition class
class management system(): def __init__(self): # List used to store data self.Student list t=[]
3.2.2 management system framework
The system functions are required to be recycled, and the user inputs different function serial numbers to perform different functions
- step
- A defined program entry function
- Load data
- Display function menu
- User input function serial number
- Perform different functions according to the serial number entered by the user
- 2. Define system function functions, add / delete students, etc
- A defined program entry function
Structure 1: entry function
class class_management system(): def __init__(self): self.Student list=[] """ One entry function""" def run(self): # 1 load the file to obtain student data # 2 display function list # 3 user input selection function serial number # 4 realize different functions according to different serial numbers """Bifunctional function"""
> Above structure: Below class , ① init Initialize student list, ② Entry function run And function functions ,It's all the same ,
Structure 2: function function
class class_management system(): def __init__(self): self.Student list=[] """ One entry function""" def run(self): # 2 display function list self.Optional Features () #Class to call an instance method, use self """Bifunctional function""" # 2.1 display function menu @staticmethod def Optional Features (): #There is no self in the bracket of static function print("Please select the following functions:") print("1: increase")
Structure 3 plus while – this works with main Py can run
#--*coding:utf-8*-- class class_management system(): def __init__(self): self.Student list=[] """ One entry function""" def run(self): # 1 load the file to obtain student data pass while True: # 2 display function list self.Optional Features () #Class to call an instance method, use self # 3 user input selection function serial number Function serial number=int(input("Please select the function you want:")) # 4 realize different functions according to different serial numbers if Function serial number==1: self.increase() elif Function serial number==2: self.reduce() elif Function serial number==7: break """Bifunctional function""" # 2.1 display function menu static function @staticmethod def Optional Features (): #There is no self in the bracket of static function print("Please select the following functions:") print("1: increase") print("2: Delete") # 2.2 add def increase(self): print("increase") # 2.3 minus def reduce(self): print("reduce") # 2.4 modification # 2.5 check # 2.6 display all # 2.7 preservation # 2.8 loading # verification if __full name__=="__main__": One middle school=class_management system() print(One middle school.Optional Features ()) print(class_management system.Optional Features ())
3.3 main.py
demand
- Guided package "management system",
- Create the object and start the program
#--*coding:utf-8*-- # Guide Package from management system import * # Create object startup management system Second middle school=class_management system() Second middle school.run()
This big frame is ok, and the program can run normally
3.4 defining system function
3.4.1 add
-
The user is required to enter the name and gender to add the student to the system
-
step
- User input name... Input
- Create object = student registration class (name, gender, mobile phone)
- Add students to the list self Student list Append (student)
-
Initial 1 code
# 2.2 add def increase(self): # 1. Please input the user's name, etc # 2 create student object # 3 add the object to the student list
- code
#Adding student function requires creating Student object, so first import * student registration * module > from Student registration import * class management system(): .......... # 2.2 add def increase(self): # 1. Please input the user's name, etc full name=input("Please enter your name:") Gender=input("Please enter your gender:") mobile phone=input("Please enter your mobile phone:") # 2. Create a student object. Student registration should be introduced here py student=Student registration(full name,Gender,mobile phone) print(student) # 3 add the object to the student list self.Student list.append(student)d print(self.Student list)
3.4.2 minus
- The user is required to enter the name. If it is in the list, this student information will be deleted
- step
- User input name
- Go through the student data list and check whether it exists. If yes, delete it. If not, print "no person found"
- Initial 1 code
# 2.3 minus def reduce(self): # 1 user input student name # 2. If the history list is in, it will be deleted. If it is not in, it will prompt "no person found"
- code
#Adding student function requires creating Student object, so first import * student registration * module > from Student registration import * class management system(): .......... # 2.3 minus def reduce(self): # 1 user input student name Minus students=input("Please enter the name of the student you want to delete:") # 2. If the history list is in, it will be deleted. If it is not in, it will prompt "no person found" for i in self.Student list: if i.full name==Minus students: # Delete the student object self.Student list.remove(i) break # After going through the list, else:print("No one was found") print(self.Student list)
3.4.3 modification
The user is required to enter the name, update the object information if any, and create a new one if none
step
- User input name \ gender \ mobile phone
- Go through the student list, update it when it is, and "check no such person" when it is not
code
# 2.4 modification def change(self): # 1 user input name \ gender \ mobile phone Change students=input("Please enter the student name to modify:") # 2. Go through the student list, update it when it is, and "check no such person" when it is not for i in self.Student list: if i.full name==Change students: i.full name=Change students i.Gender=input("Please enter student gender:") i.mobile phone=input("Please enter the student's mobile phone:") print(f"The student information has been modified successfully,full name:{Change students},Gender:{i.Gender},mobile phone:{i.mobile phone}") break else:print("No one was found")
3.4.4 check
The user is required to enter the name to find out whether there is
code
# 2.5 check def check(self): Check students=input("Please enter the name of the student you want to find:") for i in self.Student list: if i.full name==Check students: print(f"The student information is as follows,full name:{Check students},Gender:{i.Gender},mobile phone:{i.mobile phone}") break else:print("No one was found")
3.4.5 view all
View all student information in the form of demand form
code
# 2.6 display all def View all(self): print("full name\t Gender\t mobile phone") for i in self.Student list: print(f"{i.full name}\t{i.Gender}\t{i.mobile phone}")
3.4.6 save student information
- It is required to save the modified student data to the file
- step
- Open file
- Write data to file
- Close file
1 is the data written to the file the memory address of the student object? No! It should be understandable data
2 what data type should be in the file? String dictionary [{"Wang Wu": "Wang Wu", "male", "1775"}]
- Extended dict
Function: collect the properties, methods and corresponding values of class objects or instance objects, and return a dictionary
class A(): a=0 # Class properties def __init__(self): self.b=1 #Instance object properties aa=A() # Returns all data in a class print(A.__dict__) # Return only instance object related print(aa.__dict__) """ {'__module__': '__main__', 'a': 0, ....} # Dictionary file {'b': 1} # Dictionary file """
-Initial code
# 2.7 preservation def preservation(self): # 1 open file f=open("Student information.bata","w") # 2 write data to file # 2.1 convert [Student object] to [list dictionary] [i.__dict__ for i in Student object] # 2.2 the file is written to str() as a string f.write(str(New student list)) # 3 close file f.close()
code
# 2.7 preservation def preservation(self): # 1 open file f=open("Student information.bata","w") # 2 write data to file # 2.1 note 1: file writing cannot be a memory address, [Student object]] is converted to [list dictionary] New student list=[i.__dict__ for i in self.Student list] """List derivation""" """[{"full name":"Wang Wu","Gender":"male","mobile phone":"111"}]""" print(New student list) # 2.2 note 2: the data in the file is required to be a string. The data type needs to be converted into a string before it can be written f.write(str(New student list)) # 3 close file f.close()
After saving, you can't see it in the pycharm directory. The saved file should be found in the resource manager
After opening the data file
[{'name':'aa ',' gender ':'bb', 'mobile phone':'132 '}]
3.4.8 loading student information
- Every time a requirement enters the system, the modified data is the data in the file??
- step
- 1 [open file] attempts to open with r, if abnormal w opens
- 2 [read file] the file reads a string and needs to be restored to a list: [{}] → [Student object]
- 3 [close file]
- code
def load(self): # 1 [open file] attempts to open with r, if abnormal w opens try: f=open("Student information.bata","r") except: f=open("Student information.bata","w") else: # 2 [read file] the file reads a string and needs to be restored to a list: [{}] → [Student object] # 2.1 read file string data=f.read() # 2.2 restore string to list type New list=eval(data) # Create student registration class object student registration class (name, gender, mobile phone) and assign it to self Student list self.Student list=[Student registration(i["full name"],i["Gender"],i["mobile phone"]) for i in New list] finally: # 3 [close file] f.close()
==================================
IV. all codes
4.1 student registration py
#--*coding:utf-8*-- # Define class initialization information class Student registration(): # Initialize student information def __init__(self,full name,Gender,mobile phone): self.full name=full name self.Gender=Gender self.mobile phone=mobile phone # The return value, str, is used to convert the returned memory address into def __str__(self): return f"{self.full name},{self.Gender},{self.mobile phone}" # test if __name__=="__main__": Zhang San=Student registration("Zhang San","male",1775) # print("name \ tgender \ mobile phone") print(Zhang San) print(Student registration)
4.2 management system py
#--*coding:utf-8*-- from Student registration import * class class_management system(): def __init__(self): self.Student list=[] """ One entry function""" def run(self): self.load() while True: # 2 display function list self.Optional Features () #Class to call an instance method, use self # 3 user input selection function serial number Function serial number=int(input("Please select the function you want:")) # 4 realize different functions according to different serial numbers if Function serial number==1: self.increase() elif Function serial number==2: self.reduce() elif Function serial number==3: self.change() elif Function serial number==4: self.check() elif Function serial number==5: self.View all() elif Function serial number==6: self.preservation() elif Function serial number==7: self.load() elif Function serial number==8: break """Bifunctional function""" # 2.1 display function menu static function @staticmethod def Optional Features (): #There is no self in the bracket of static function print("Please select the following functions:") print("1: increase") print("2: Delete") print("3: change") print("4: check") print("5:View all") print("6:Save student information") print("7: load") # 2.2 add def increase(self): # 1. Please input the user's name, etc full name=input("Please enter your name:") Gender=input("Please enter your gender:") mobile phone=input("Please enter your mobile phone:") # 2. Create a student object. Student registration should be introduced here py Increase students=Student registration(full name,Gender,mobile phone) print(Increase students) # 3 add the object to the student list self.Student list.append(Increase students) print(self.Student list) # 2.3 minus def reduce(self): # 1 user input student name Minus students=input("Please enter the name of the student you want to delete:") # 2. If the history list is in, it will be deleted. If it is not in, it will prompt "no person found" for i in self.Student list: if i.full name==Minus students: # Delete the student object self.Student list.remove(i) break # After going through the list, else:print("No one was found") print(self.Student list) # 2.4 modification def change(self): # 1 user input name \ gender \ mobile phone Change students=input("Please enter the student name to modify:") # 2. Go through the student list, update it when it is, and "check no such person" when it is not for i in self.Student list: if i.full name==Change students: i.full name=input("Please enter student name:") i.Gender=input("Please enter student gender:") i.mobile phone=input("Please enter the student's mobile phone:") print(f"The student information has been modified successfully,full name:{i.full name},Gender:{i.Gender},mobile phone:{i.mobile phone}") break else:print("No one was found") # 2.5 check def check(self): Check students=input("Please enter the name of the student you want to find:") for i in self.Student list: if i.full name==Check students: print(f"The student information is as follows,full name:{Check students},Gender:{i.Gender},mobile phone:{i.mobile phone}") break else:print("No one was found") # 2.6 display all def View all(self): print("full name\t Gender\t mobile phone") for i in self.Student list: print(f"{i.full name}\t{i.Gender}\t{i.mobile phone}") # 2.7 preservation def preservation(self): # 1 open file f=open("Student information.bata","w") # 2 write data to file # 2.1 note 1: file writing cannot be a memory address, [Student object]] is converted to [list dictionary] New student list=[i.__dict__ for i in self.Student list] """List derivation""" """[{"full name":"Wang Wu","Gender":"male","mobile phone":"111"}]""" print(New student list) # 2.2 note 2: the data in the file is required to be a string. The data type needs to be converted into a string before it can be written f.write(str(New student list)) # 3 close file f.close() # 2.8 loading def load(self): # 1 [open file] attempts to open with r, if abnormal w opens try: f=open("Student information.bata","r") except: f=open("Student information.bata","w") else: # 2 [read file] the file reads a string and needs to be restored to a list: [{}] → [Student object] # 2.1 read file string data=f.read() # 2.2 restore string to list type New list=eval(data) # Create student registration class object student registration class (name, gender, mobile phone) and assign it to self Student list self.Student list=[Student registration(i["full name"],i["Gender"],i["mobile phone"]) for i in New list] # 3 [operation document] addition, deletion, modification and query # 4 [save file] save student data to the student list finally: # 5 [close file] f.close() # verification if __name__=="__main__": One middle school=class_management system() print(One middle school.Optional Features ()) print(class_management system.Optional Features ())
4.3 main program py
#--*coding:utf-8*-- # Guide Package from management system import * # Create object startup management system Student management system of No. 2 middle school=class_management system() Student management system of No. 2 middle school.run()