Hello, everyone. Meet again. I'm Quan dujun. I've prepared the Idea registration code for you today.
There are nine container components in QT, namely combo box QGroupBox, scroll area QScrollArea, toolbox QToolBox, tab QTabWidget, control stack QWidgetStack, frame QFrame, component QWidget, MDI window display area QMdiArea and docking window QDockWidget. This blog mainly introduces: combo box QGroupBox, scroll area QScrollArea, toolbox QToolBox, tab QTabWidget
1, QGroupBox group box
QGroupBox provides support for building group boxes. The group box usually has a border and a title bar, which is used as a container part, in which various window parts can be arranged. The title of the group box is usually displayed at the top, and its position can be set to one of the methods of left, center, right and automatic adjustment. The widget in the grouping box can get the focus of the application. The widget in the grouping box is the sub window of the grouping box. Usually, the addWidget() method is used to add the sub widget to the grouping box.
Qt example: \ examples \ Qt-5.9 3 \ widgets \ widgets \ GroupBox provides an example of QGroupBox
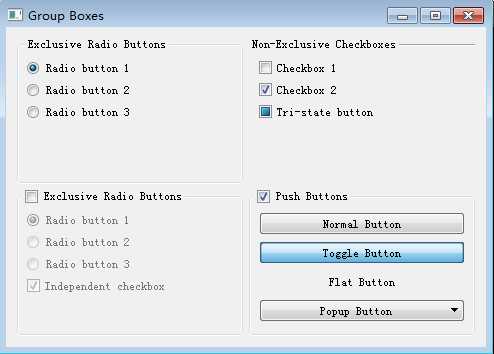
(1) Attributes
Q_PROPERTY(QString title READ title WRITE setTitle) Q_PROPERTY(Qt::Alignment alignment READ alignment WRITE setAlignment) Q_PROPERTY(bool flat READ isFlat WRITE setFlat) Q_PROPERTY(bool checkable READ isCheckable WRITE setCheckable) Q_PROPERTY(bool checked READ isChecked WRITE setChecked DESIGNABLE isCheckable NOTIFY toggled USER true)
(2) Signal and slot
public Q_SLOTS: void setChecked(bool checked); Q_SIGNALS: void clicked(bool checked = false); void toggled(bool);
(3) Example
#include "mainwindow.h" #include <QApplication> #include <QObject> #include <QGroupBox> #include <QPushButton> #include <QCheckBox> #include <QRadioButton> #include <QVBoxLayout> int main(int argc, char *argv[]) { QApplication a(argc, argv); QGroupBox *pGroupBox = new QGroupBox(QObject::tr("GroupBox test")); QPushButton *pBtn = new QPushButton(QObject::tr("button")) ; QCheckBox* pCheckBox = new QCheckBox(QObject::tr("checkbox test")); QRadioButton* pRadio = new QRadioButton(QObject::tr("radiobtn test")); QVBoxLayout *layout = new QVBoxLayout; layout->addWidget(pBtn); layout->addWidget(pCheckBox); layout->addWidget(pRadio); pGroupBox->setLayout(layout); pGroupBox->setCheckable(true); pGroupBox->show(); return a.exec(); }
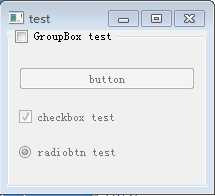
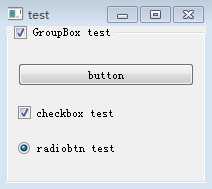
2, QScrollArea scroll area
The QScrollArea scroll area component is used to display the frame of the content of the child control. If the size of the child control exceeds the size of the frame, you can use the scroll bar to view the whole child control conveniently. QScrollArea can add scroll bars to any QWidget, but the scroll bars added to general custom forms are not displayed.
class QScrollArea : public QAbstractScrollArea
class QAbstractScrollArea : public QFrame
(1) Attributes
class Q_WIDGETS_EXPORT QAbstractScrollArea : public QFrame { Q_OBJECT Q_PROPERTY(Qt::ScrollBarPolicy verticalScrollBarPolicy READ verticalScrollBarPolicy WRITE setVerticalScrollBarPolicy) Q_PROPERTY(Qt::ScrollBarPolicy horizontalScrollBarPolicy READ horizontalScrollBarPolicy WRITE setHorizontalScrollBarPolicy) Q_PROPERTY(SizeAdjustPolicy sizeAdjustPolicy READ sizeAdjustPolicy WRITE setSizeAdjustPolicy) ....................................................... } class Q_WIDGETS_EXPORT QScrollArea : public QAbstractScrollArea { Q_OBJECT Q_PROPERTY(bool widgetResizable READ widgetResizable WRITE setWidgetResizable) Q_PROPERTY(Qt::Alignment alignment READ alignment WRITE setAlignment) ................................................ }
(2) Common member functions
QScrollArea: : QScrollArea(QWidget *parent = 0)
Construct a ScrollArea whose parent object is parent
void QScrollArea: : setWidget(QWidget *widget)
Set the control widget as a child control of the QScrollArea component
QWidget *SCrollArea: : takeWidget()
Delete child controls of QScrollArea
QWidget *QScrollArea: : widget()const
Returns the child control of QScrollArea
(3) Example
#include "mainwindow.h" #include <QApplication> #include <QObject> #include <QGroupBox> #include <QPushButton> #include <QCheckBox> #include <QRadioButton> #include <QVBoxLayout> #include <QScrollArea> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; QGroupBox *pGroupBox = new QGroupBox(QObject::tr("GroupBox test"), &w); QPushButton *pBtn = new QPushButton(QObject::tr("button")) ; QCheckBox* pCheckBox = new QCheckBox(QObject::tr("checkbox test")); QRadioButton* pRadio = new QRadioButton(QObject::tr("radiobtn test")); QScrollArea* pSCrollArea = new QScrollArea(&w); QVBoxLayout *layout = new QVBoxLayout; layout->addWidget(pBtn); layout->addWidget(pCheckBox); layout->addWidget(pRadio); pGroupBox->setLayout(layout); pGroupBox->setCheckable(true); // Add groupbox to scroll area pSCrollArea->setWidgetResizable(true); // Set scroll area size pSCrollArea->setBackgroundRole(QPalette::Dark); pSCrollArea->setHorizontalScrollBarPolicy(Qt::ScrollBarAsNeeded); pSCrollArea->setVerticalScrollBarPolicy(Qt::ScrollBarAsNeeded); pSCrollArea->setWidget(pGroupBox); w.setCentralWidget(pSCrollArea); w.setWindowTitle("container test"); w.setMinimumSize(50,50); w.show(); return a.exec(); }
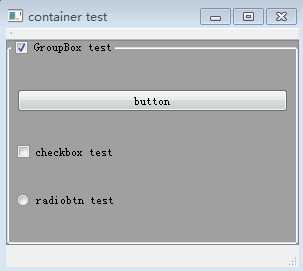
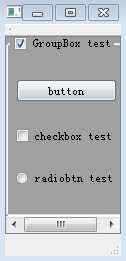
3, QToolBox toolbox
QToolBox provides a series of pages and compartments, just like the toolbox in Qt Creator.
(1) Attributes
class Q_WIDGETS_EXPORT QToolBox : public QFrame { Q_OBJECT Q_PROPERTY(int currentIndex READ currentIndex WRITE setCurrentIndex NOTIFY currentChanged) Q_PROPERTY(int count READ count) . . . . . . . . . . . . . . . . . . . . }
(2) Common functions
Add an item, and set the icon, text, enable, tooltip, etc. of the item
int addItem(QWidget *widget, const QString &text); int addItem(QWidget *widget, const QIcon &icon, const QString &text); int insertItem(int index, QWidget *widget, const QString &text); int insertItem(int index, QWidget *widget, const QIcon &icon, const QString &text); void removeItem(int index); void setItemEnabled(int index, bool enabled); bool isItemEnabled(int index) const; void setItemText(int index, const QString &text); QString itemText(int index) const; void setItemIcon(int index, const QIcon &icon); QIcon itemIcon(int index) const; #ifndef QT_NO_TOOLTIP void setItemToolTip(int index, const QString &toolTip); QString itemToolTip(int index) const; #endif int currentIndex() const; QWidget *currentWidget() const; QWidget *widget(int index) const; int indexOf(QWidget *widget) const; int count() const; public Q_SLOTS: void setCurrentIndex(int index); void setCurrentWidget(QWidget *widget); Q_SIGNALS: void currentChanged(int index);
(3) Example
Idea: each item in QToolBox is a QWidget
QWidegt consists of QVBoxLayout. Each QVBoxLayout contains two qtoolbuttons
#include "mainwindow.h" #include <QApplication> #include <QToolBox> #include <QVBoxLayout> #include <QToolButton> #include <QGroupBox> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; QWidget *pGroup1 = new QWidget; QToolButton *pBtn1 = new QToolButton; pBtn1->setText("apple"); pBtn1->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn1->setAutoRaise(true); pBtn1->setArrowType(Qt::ArrowType::DownArrow); QToolButton *pBtn2 = new QToolButton; pBtn2->setText("babala"); pBtn2->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn2->setAutoRaise(true); QVBoxLayout *pLayout1 = new QVBoxLayout(pGroup1); pLayout1->setMargin(10); pLayout1->setAlignment(Qt::AlignmentFlag::AlignLeft); pLayout1->addWidget(pBtn1); pLayout1->addWidget(pBtn2); ////////////////////////////////////// QWidget *pGroup2 = new QWidget; QToolButton *pBtn3 = new QToolButton; pBtn3->setText("HUAWEI"); pBtn3->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn3->setAutoRaise(true); QToolButton *pBtn4 = new QToolButton; pBtn4->setText("IXOAMI"); pBtn4->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn4->setAutoRaise(true); QVBoxLayout *pLayout2 = new QVBoxLayout(pGroup2); pLayout2->setMargin(10); pLayout2->setAlignment(Qt::AlignmentFlag::AlignLeft); pLayout2->addWidget(pBtn3); pLayout2->addWidget(pBtn4); QToolBox* pToolBox = new QToolBox(&w); pToolBox->addItem(pGroup1, "fruit"); // When the first parameter is QToolBtn, the display is incorrect pToolBox->addItem(pGroup2, "telephone"); w.setCentralWidget(pToolBox); w.setWindowTitle("container test"); w.setMinimumSize(50,50); w.show(); return a.exec(); }
View Code
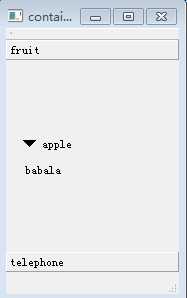
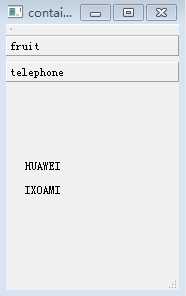
4, QTabWidget tab
There is a label option bar at the top or bottom of the QTabWidget tab component. Each label option has a page. To select which page, just click the corresponding label or press the specified ALT + letter shortcut key combination.
(1) Attributes
TabPosition,TabShape,currentIndex,count,iconSize,TextElideMode,usesScrollButtons,documentMode,tabsClosable,movable,tabBarAutoHide
class Q_WIDGETS_EXPORT QTabWidget : public QWidget { Q_OBJECT Q_PROPERTY(TabPosition tabPosition READ tabPosition WRITE setTabPosition) Q_PROPERTY(TabShape tabShape READ tabShape WRITE setTabShape) Q_PROPERTY(int currentIndex READ currentIndex WRITE setCurrentIndex NOTIFY currentChanged) Q_PROPERTY(int count READ count) Q_PROPERTY(QSize iconSize READ iconSize WRITE setIconSize) Q_PROPERTY(Qt::TextElideMode elideMode READ elideMode WRITE setElideMode) Q_PROPERTY(bool usesScrollButtons READ usesScrollButtons WRITE setUsesScrollButtons) Q_PROPERTY(bool documentMode READ documentMode WRITE setDocumentMode) Q_PROPERTY(bool tabsClosable READ tabsClosable WRITE setTabsClosable) Q_PROPERTY(bool movable READ isMovable WRITE setMovable) Q_PROPERTY(bool tabBarAutoHide READ tabBarAutoHide WRITE setTabBarAutoHide) }
(2) Signal and slot
public Q_SLOTS: void setCurrentIndex(int index); void setCurrentWidget(QWidget *widget); Q_SIGNALS: void currentChanged(int index); void tabCloseRequested(int index); void tabBarClicked(int index); void tabBarDoubleClicked(int index);
(3) Example
#include "mainwindow.h" #include <QApplication> #include <QTabWidget> #include <QToolButton> #include <QVBoxLayout> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; QWidget *pGroup1 = new QWidget; QToolButton *pBtn1 = new QToolButton; pBtn1->setText("apple"); pBtn1->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn1->setAutoRaise(true); pBtn1->setArrowType(Qt::ArrowType::DownArrow); QToolButton *pBtn2 = new QToolButton; pBtn2->setText("babala"); pBtn2->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn2->setAutoRaise(true); QVBoxLayout *pLayout1 = new QVBoxLayout(pGroup1); pLayout1->setMargin(10); pLayout1->setAlignment(Qt::AlignmentFlag::AlignLeft); pLayout1->addWidget(pBtn1); pLayout1->addWidget(pBtn2); ////////////////////////////////////// QWidget *pGroup2 = new QWidget; QToolButton *pBtn3 = new QToolButton; pBtn3->setText("HUAWEI"); pBtn3->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn3->setAutoRaise(true); QToolButton *pBtn4 = new QToolButton; pBtn4->setText("IXOAMI"); pBtn4->setToolButtonStyle(Qt::ToolButtonStyle::ToolButtonTextBesideIcon); pBtn4->setAutoRaise(true); QVBoxLayout *pLayout2 = new QVBoxLayout(pGroup2); pLayout2->setMargin(10); pLayout2->setAlignment(Qt::AlignmentFlag::AlignLeft); pLayout2->addWidget(pBtn3); pLayout2->addWidget(pBtn4); QTabWidget *pTab = new QTabWidget(&w); pTab->addTab(pGroup1, "fruit"); pTab->addTab(pGroup2, "telephone"); w.setCentralWidget(pTab); w.setWindowTitle("container test"); w.setMinimumSize(50,50); w.show(); return a.exec(); }
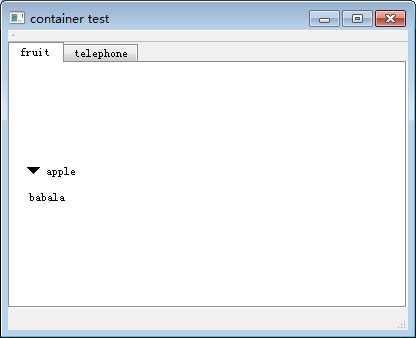
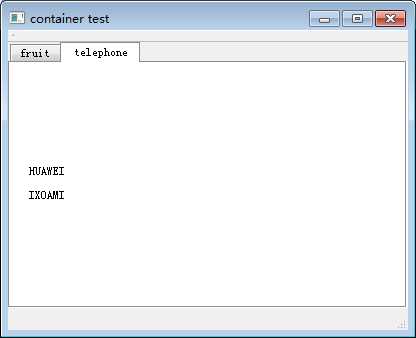
Publisher: full stack programmer, stack length, please indicate the source for Reprint: https://javaforall.cn/120088.html Original link: https://javaforall.cn