Qt uses gSoap to implement web service server and client
-
gSoap official website. In case of problems, the official website is often the most helpful place.
-
Links worthy of reference.
- gSoap usage experience: http://www.cppblog.com/qiujian5628/archive/2008/10/11/54019.html
- gSoap interface definition: http://blog.sina.com.cn/s/blog_5ee9235c0100de3g.html
-
Qt: use gSoap to build a simple CS system_ Jason's home CSDN blog
-
Environment: Qt 5.14,win10, msvc2017 compiler
I didn't use mingw for work reasons. I'll confirm it later. It's estimated that there's nothing wrong.
gSoap tool introduction
gSOAP is a cross platform tool for developing Web Service server and client. It is encoded in C and C + + languages under Windows, Linux, MAC OS and UNIX, and integrates SSL functions.
1. Download
- Download link: https://www.genivia.com/products.html , download the open source version

- Page Jump, automatic download
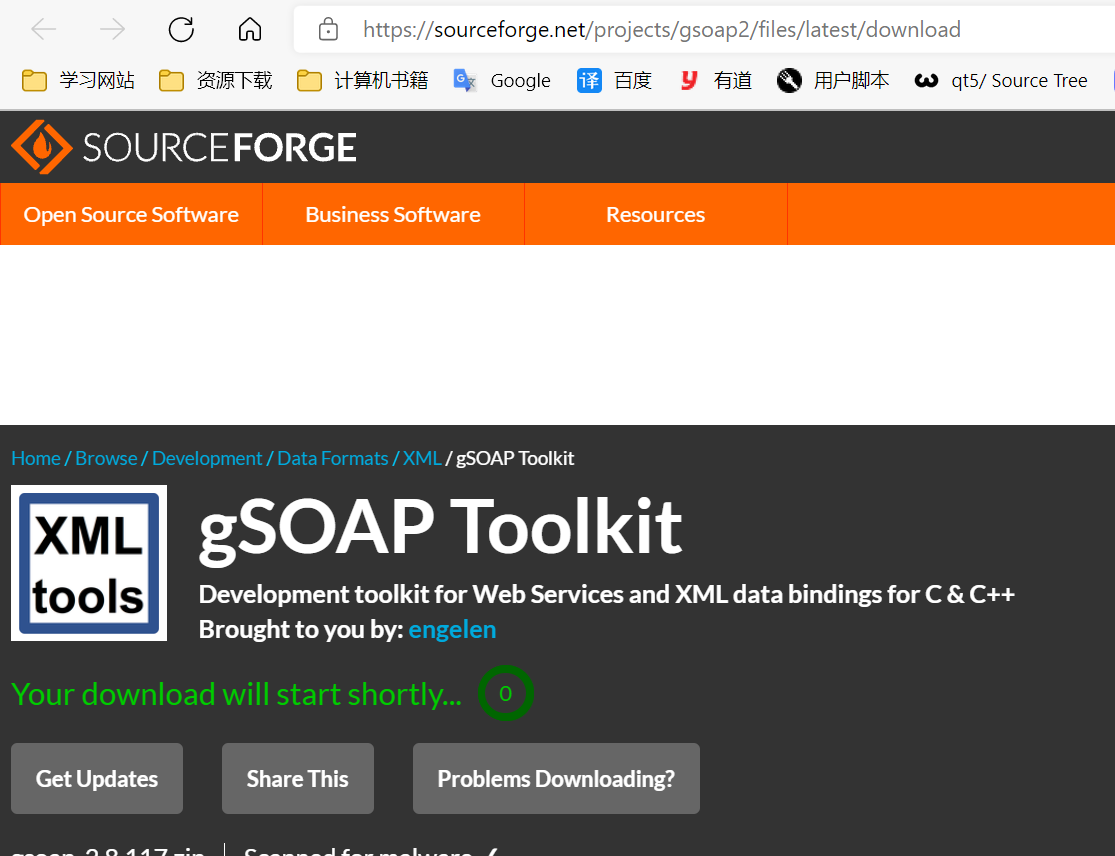
-
Compressed package
-
Unzip to Disk C
For example: C:\gsoap-2.8\
2. Hello world: start using the SOAP api
Using the gSOAP auto encoding CLI tools and libraries, you can easily use and deploy the SOAP/XML Web Service api.
CLI command line interface (English: command line interface) is the most widely used user interface before the popularity of graphical user interface (GUI). Users input instructions through the keyboard and the computer executes them after receiving the instructions. It is also called character user interface (CUI).
For example, let's implement a simple SOAP hello API that accepts a name and returns the greeting "hello name".
The SOAP API uses XML, but it is not necessary to use XML when using gSoup tool in actual development, because we use gSOAP XML data binding in c + +, which makes our code easier to use than DOM or SAX. Our hello API is simply declared as a function (XML namespace with * * ns _ * *) in the hello.h header file:
2.1 new folder
For example: gSoapFoundation folder;
2.2 create a new service interface header file hello.h
The contents are as follows:
// hello.h int ns__hello(std::string name, std::string& greeting);
2.3 using soapcpp2.exe
Copy soapcpp2.exe to gSoapFoundation. Together with hello.h, you can also set path instead
Program path: C:\gsoap-2.8\gsoap\bin\win64\soapcpp2.exe, where the program on win64 platform is used
Now just use the gSOAP soapcpp2 CLI to generate the API source code from the hello.h service interface header file:
soapcpp2 hello.h
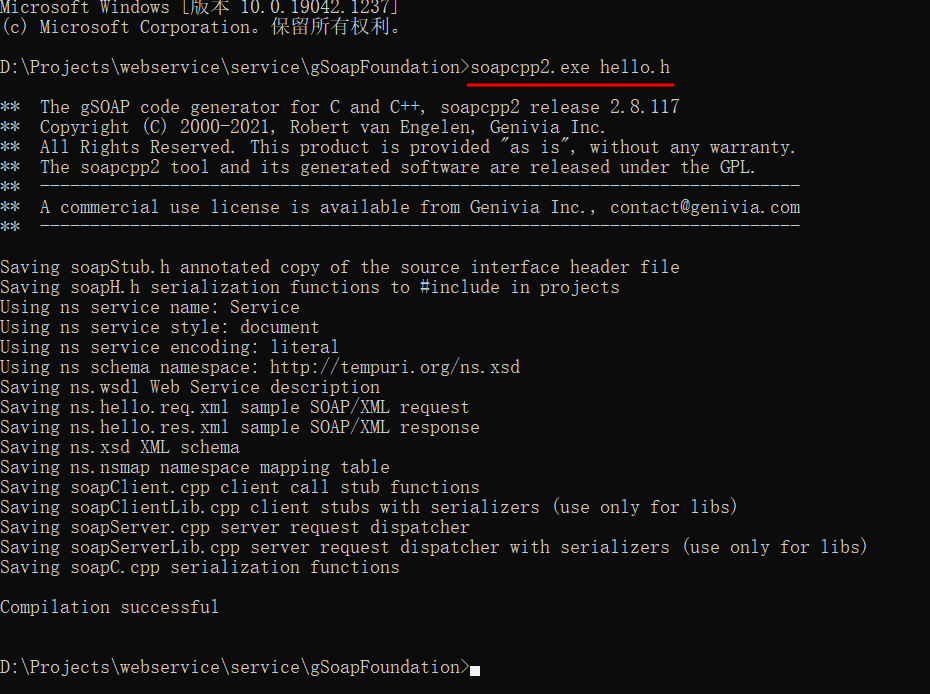
2.4. Copy source code base files:
-
stdsoap2.h
-
stdsoap2.cpp
Copy from C:\gsoap-2.8\gsoap \ path to gSoapFoundation
2.5 Qt server program
Create a new server project and copy the gSoapFoundation folder to the working directory.
#include "soapH.h" // include the generated source code headers #include "ns.nsmap" // include XML namespaces #include <QDebug> int main(int argc, char *argv[]) { // Define soap environment struct soap recieveSoap; // Initialize environment soap_init(&recieveSoap); // Binding environment / host / port / backlog soap_bind(&recieveSoap, "127.0.0.1", 23410, 100); while (true) { // Dead loop, similar to monitoring // Accept client connections int s = soap_accept(&recieveSoap); if (s < 0) { soap_print_fault(&recieveSoap, stderr); qDebug() << "error"; exit(-1); } qDebug() << "Socket connection successful: slave socket =" << s; soap_serve(&recieveSoap); // serve request, one thread, CGI style soap_end(&recieveSoap); // dealloc data and clean up } return 0; } //The implementation function on the server side is the same as that declared in hello.h, but there is an additional parameter of the current soap connection int ns__hello(struct soap *soap, std::string name, std::string& greeting) { greeting = "Hello " + name ; return SOAP_OK; }
The files to be imported by the server are as follows:
The compilation and operation should be correct. You can open the page through the web browser, as shown below:
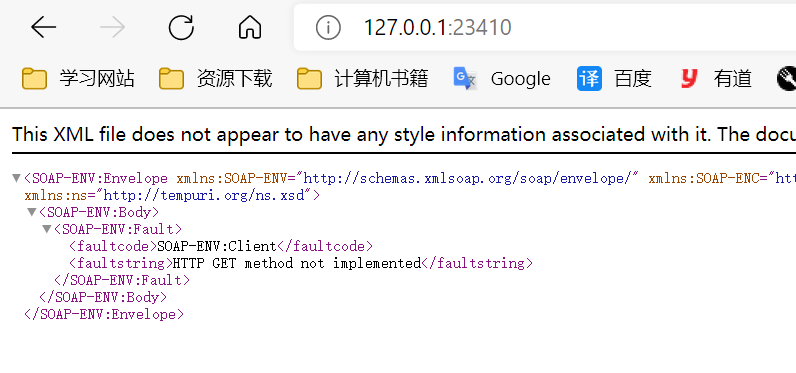
2.6 client
#include "soapH.h" // include the generated source code headers #include "ns.nsmap" // include XML namespaces #include <QDebug> int main(int argc, char *argv[]) { struct soap *soap = soap_new(); // new context std::string greeting; //This function is the main function called by the client. The following parameters are the same as those declared in hello.h. There are three more parameters in front. The function name is the interface function name ns__ Add soap before hello_ call_ if (soap_call_ns__hello(soap, "127.0.0.1:23410", NULL, "world", greeting) == SOAP_OK) qDebug() << greeting.data (); else soap_stream_fault(soap, std::cerr); soap_destroy(soap); // delete managed deserialized C++ instances soap_end(soap); // delete other managed data soap_free(soap); // free context return 0; }
Compile and run to ensure that the server is running, call the server service, obtain data and print out:
3. Summary
A very simple example, as an example of getting started. 😄