Note: This article does not teach how to download and install software, but only provides installation precautions and environment construction methods. Please change all file paths involved in this article according to your actual installation location, which will not be repeated below.
1, Documents to be prepared before setting up the environment
Qt download address:
http://download.qt.io/archive/
VS2017 download address:
https://visualstudio.microsoft.com/zh-hans/vs/older-downloads/
MySQL download address:
https://dev.mysql.com/downloads/mysql/
Software version used in this article:
Qt5.14.2
VS2017
MySQL8.0.26
2, Precautions for installing software
2.1 components that must be checked when installing Qt
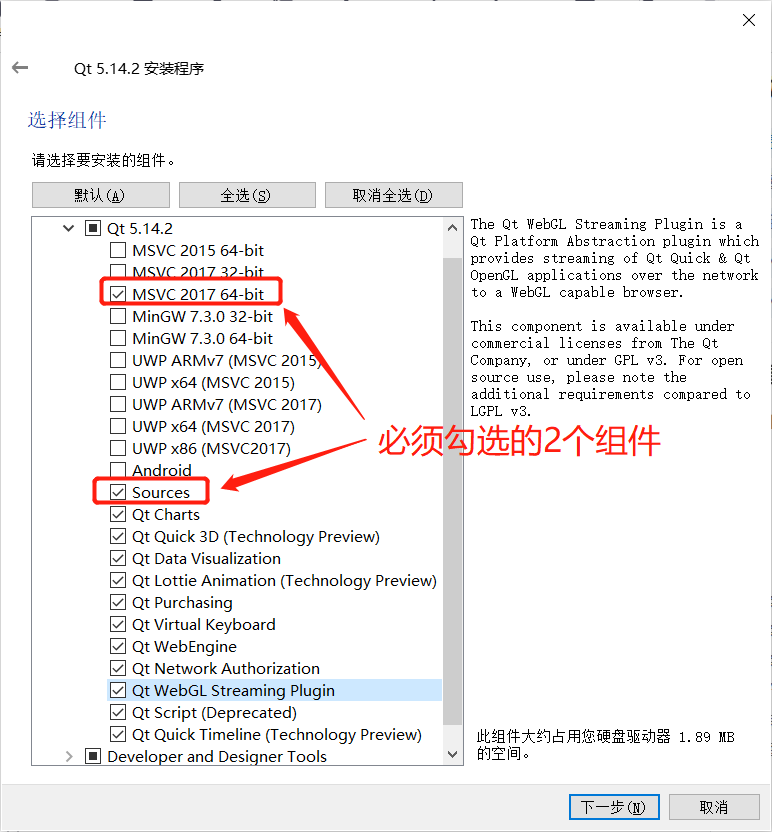
3, Qt+VS environment construction
3.1 installing VS extensions
Open VS2017, find Tools > extensions and updates, search for qt in the online option, find Qt Visual Studio Tools and install it.
3.2 configuring Qt VS Tools
① Open QT vs Tools > Qt VerSions
② Add "qmake.exe" (default path: C:\Qt\Qt5.14.2.14.2\msvc2017_64\bin\qmake.exe)
③ Note: since the configuration options of "Qt VS Tools" in most online articles are somewhat different from my configuration, other online configuration methods are also put here. The final effect of this operation is actually the same as that of step ②, and the selection of step ② and ③ is the same as that of your actual situation.
4, QT+MySQL environment construction
For various reasons, Qt does not provide MySQL database driver, so you need to compile a dynamic library yourself.
4.1 configuring MySQL environment variables
(default path: C:\Program Files\MySQL\MySQL Server 8.0\bin)
4.2 test whether MySQL environment variable configuration is successful
Open cmd and use the "mysql -u username - p" command to test, as shown in the following figure:
4.3 problems may be encountered when connecting to the database
Find the "MySQL80" service in the "services" of the operating system and start it, as shown in the following figure
4.4 compiling mysql dynamic library using Qt
① Open the "mysql.pro" project in the Qt source code, and change the code of the following two files:
(default path: C:\Qt\Qt5.14.2.14.2\Src\qtbase\src\plugins\sqldrivers\mysql\mysql.pro)
② Change the code in the "mysql.pro" file as follows:
TARGET = qsqlmysql HEADERS += $$PWD/qsql_mysql_p.h SOURCES += $$PWD/qsql_mysql.cpp $$PWD/main.cpp #QMAKE_USE += mysql #Comment out this line of code OTHER_FILES += mysql.json PLUGIN_CLASS_NAME = QMYSQLDriverPlugin #New code INCLUDEPATH += $$quote(C:/Program Files/MySQL/MySQL Server 8.0/include) DEPENDPATH += $$quote(C:/Program Files/MySQL/MySQL Server 8.0/include) LIBS += $$quote(C:/Program Files/MySQL/MySQL Server 8.0/lib/libmysql.lib) #New code #Note: the slash direction may be reversed when copying the path include(../qsqldriverbase.pri)
③ Change the code in the "qsqldriverbase.pri" file as follows:
QT = core core-private sql-private # For QMAKE_USE in the parent projects. #include($$shadowed($$PWD)/qtsqldrivers-config.pri) #Comment out this line of code include(./configure.pri) #New code PLUGIN_TYPE = sqldrivers load(qt_plugin) DEFINES += QT_NO_CAST_TO_ASCII QT_NO_CAST_FROM_ASCII
④ Save and build the project when the changes are complete
⑤ After building the project, you can find the following files under the path "C:\plugins\sqldrivers"
⑥ Copy the above two files "qsqlmysql.dll" and "qsqlmysqld.dll" to the following path:
C:\Qt\Qt5.14.2\5.14.2\msvc2017_64\plugins\sqldrivers
⑦ Find "libmysql.lib" in the path "C:\Program Files\MySQL\MySQL Server 8.0\lib"
And copy it to "C:\Qt\Qt5.14.2.14.2\msvc2017_64\bin"
5, Use VS to write code to test the connection between Qt and MySQL
5.1 create Qt Widgets project
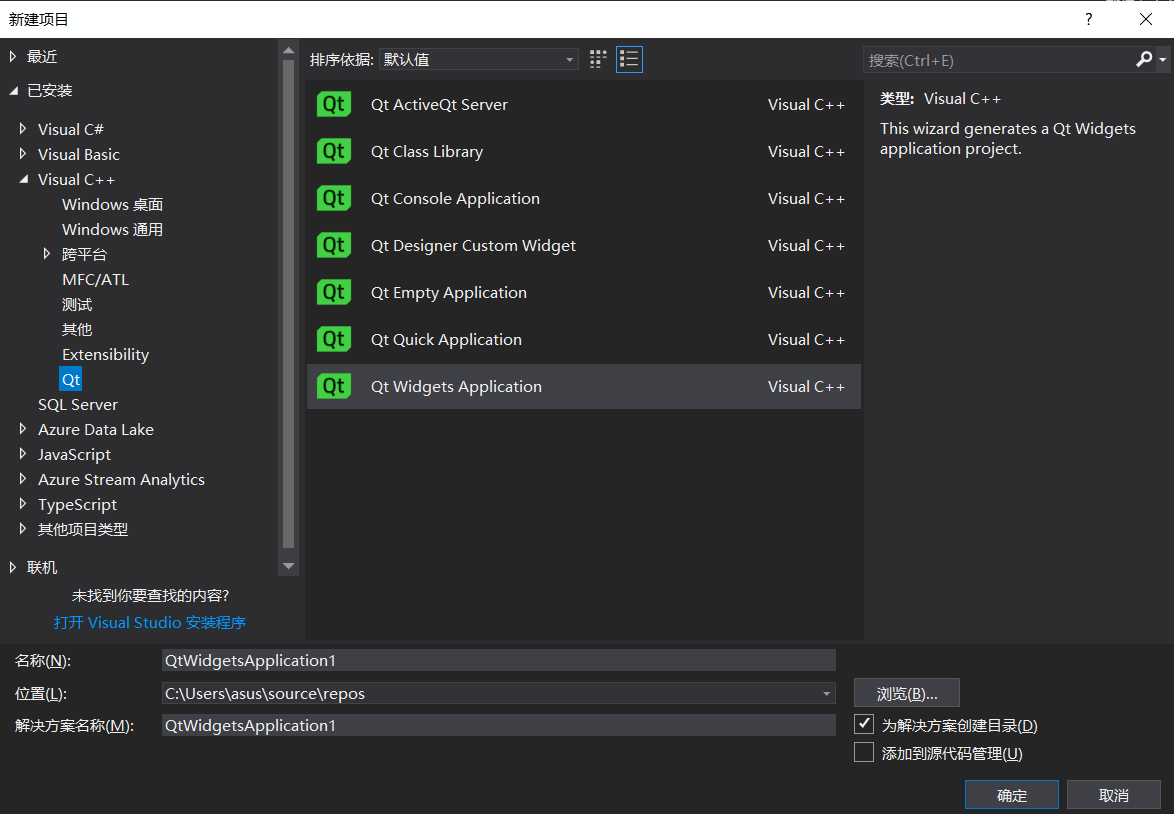
Note: when creating a project, pay attention to check "SQL" in Qt Modules, as shown in the following figure:
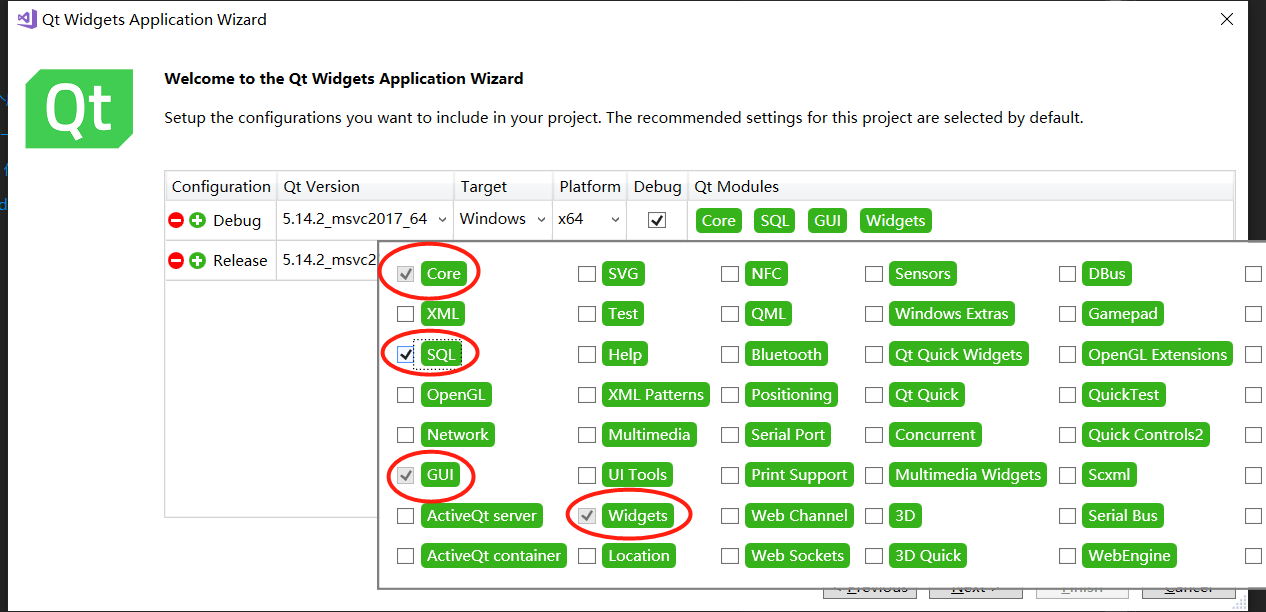
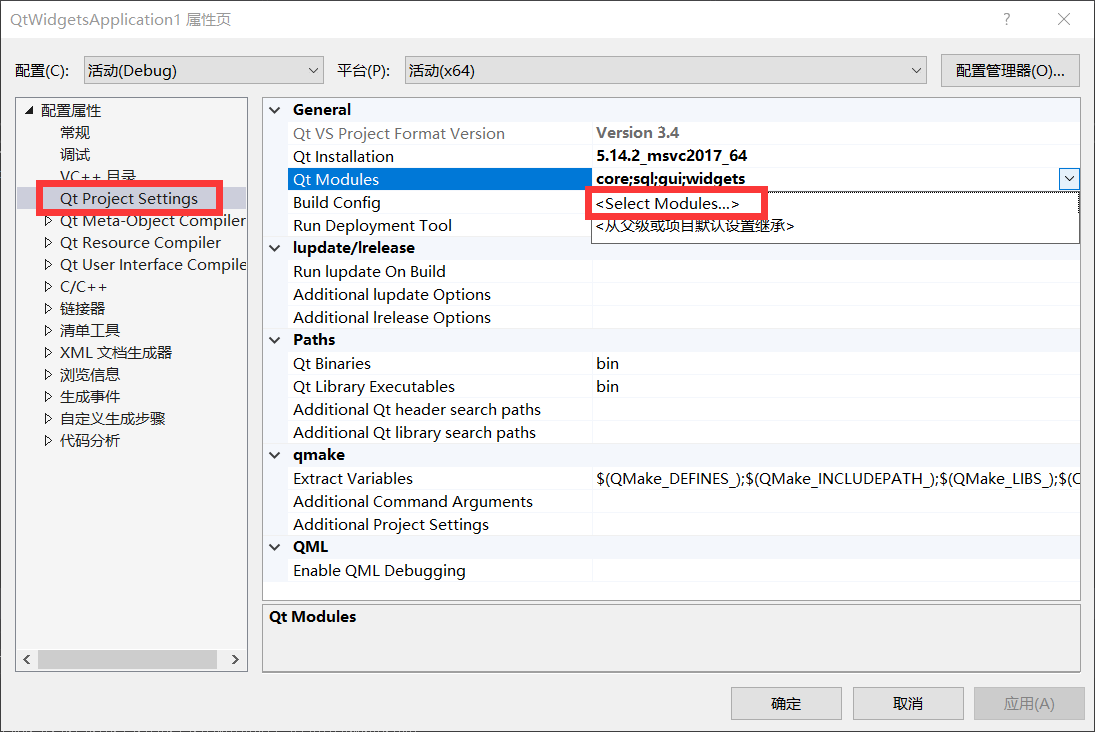
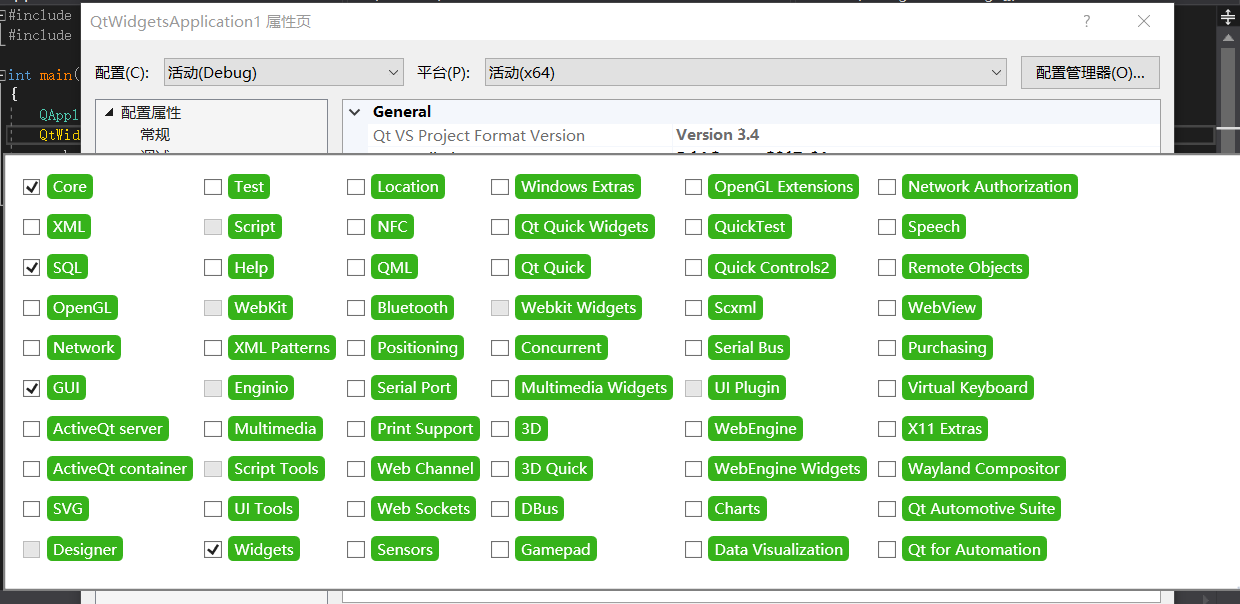
#include "QtWidgetsApplication1.h" #include <QtWidgets/QApplication> #include <QtWidgets/QMessageBox> #include <QtSql/QSqlDataBase> #include <QtSql/QSqlError> int main(int argc, char *argv[]) { QApplication a(argc, argv); QtWidgetsApplication1 w; w.show(); //Create a database object and select qmmysql as the database driver QSqlDatabase *db = new QSqlDatabase(QSqlDatabase::addDatabase("QMYSQL")); db->setHostName("localhost");//Database address db->setPort(3306);//Database port number, MySQL default port number 3306 db->setDatabaseName("Database name");//Database name db->setUserName("user name");//user name db->setPassword("password");//password //Open database if (db->open()) { QMessageBox::information(NULL, "info", "Sql Connected."); } else { QMessageBox::information(NULL, "info", "connect failed:" + db->lastError().text()); } //close database QString dbname = db->connectionName(); db->close(); delete db; db = NULL; QSqlDatabase::removeDatabase(dbname); return a.exec(); }
5.3 commissioning procedure
As shown in the figure below, the data connection is successful: