Introduction to redis
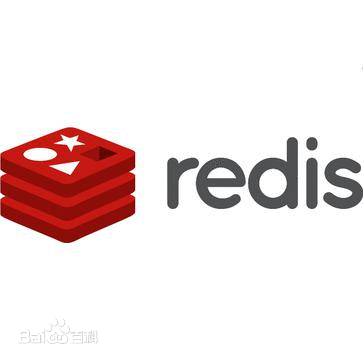
Configure Redis under Windows and change the password
Download Redis Windows version of GitHub Link, download the zip file directly and unzip it into the specified folder or download the msi installation file to install as prompted above.
Open redis.windows.conf with Notepad or Notepad++ and find the line'# requirepass foobared'. Add a line''requirepass your password'below this line
The cmd command goes to the Redis installation directory and enters redis-server redis.windows.conf, as shown in the figure
If you want to exit, press ctrl+C
Do not close the cmd console, double-click to open redis-cli.exe, connect to the local server if no error occurs, and then test, for example, the set command, the get command, the first time you enter the set command, you will find out (error) NOAUTH Authentication required. This is because you have changed the configuration password for redis.windows.conf, and thenType "auth your password" If OK appears, you can already use the Redis client.You can insert deleted data at will in the console, as shown in the figure
redis in java
1. Related Jar Files
Download and import the following three Jar files:
commons-pool2-2.4.2.jar,jedis-2.3.1.jar,spring-data-redis-1.3.4.RELEASE.jar.
2. Redis Profile
Create a new redis.properties file under the src folder to set some properties of the connection Redis.
redis.host=127.0.0.1 redis.port=6379 redis.password=123456 redis.timeout=100000 redis.minIdle=50 redis.maxIdle=100 redis.maxTotal=600 redis.maxActive=300 redis.maxWait=1000 redis.testOnBorrow=true redis.testOnReturn=true
Create a new redis.xml file.
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd"> <!-- Load redis.properties The contents of the file, redis.properties File key There must be some special rules for naming --> <context:property-placeholder location="classpath:redis.properties"/> <bean id="jedisConfig" class="redis.clients.jedis.JedisPoolConfig"> <property name="maxTotal" value="${redis.maxTotal}" /> <property name="maxIdle" value="${redis.maxIdle}" /> <property name="minIdle" value="${redis.minIdle}" /> <property name="maxWaitMillis" value="${redis.maxWait}" /> <property name="testOnBorrow" value="${redis.testOnBorrow}" /> <property name="testOnReturn" value="${redis.testOnReturn}" /> </bean> <!-- redis Connect Factory --> <bean id="jedisConnectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory"> <property name="hostName" value="${redis.host}"></property> <property name="port" value="${redis.port}"></property> <property name="password" value="${redis.password}"></property> <property name="poolConfig" ref="jedisConfig"></property> <property name="database" value="2"></property> </bean> <!-- redis Operational templates, which are as object-oriented as possible --> <bean id="redisTemplate" class="org.springframework.data.redis.core.RedisTemplate"> <property name="connectionFactory" ref="jedisConnectionFactory" /> <!-- If not configured Serializer,Then only use when storing String,If stored in object type, an error will be prompted can't cast to String!!!--> <property name="keySerializer"> <bean class="org.springframework.data.redis.serializer.StringRedisSerializer" /> </property> <property name="valueSerializer"> <bean class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" /> </property> <property name="HashKeySerializer"> <bean class="org.springframework.data.redis.serializer.StringRedisSerializer"> </bean> </property> <property name="HashValueSerializer"> <bean class="org.springframework.data.redis.serializer.StringRedisSerializer"/> </property> </bean>
3. Redis Test
1. Read the configuration file
public static void main(String[] args) { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("classpath:redis.xml"); RedisTemplate redisTemplate = (RedisTemplate) applicationContext.getBean("redisTemplate"); redisTemplate.boundValueOps("miaopeng").set("hello word"); System.out.println("redis The stored string is: "+ redisTemplate.boundValueOps("miaopeng").get()); }
2. Use jedis to not read configuration files
public static void main(String[] args) { Jedis jedis = new Jedis("127.0.0.1", 6379); //Logon Password jedis.auth("123456"); System.out.println("Connection Successful"); //Check to see if the service is running System.out.println("Service is running: " + jedis.ping()); jedis.set("miaopeng","hello world"); System.out.println(jedis.get("miaopeng"));//Value by key }
jedis tool class
/** * Gets the value of the specified key, returns null if the key does not exist, and throws an error if the key does not store a string * * @param key * @return */ public static String get(String key) { Jedis jedis = getJedis(); String value = null; value = jedis.get(key); return value; } /** * Set the value of key to value * * @param key * @param value * @return */ public static String set(String key, String value) { Jedis jedis = getJedis(); return jedis.set(key, value); } /** * Delete the specified key or pass in an array containing the key * * @param keys * @return */ public static Long del(String... keys) { Jedis jedis = getJedis(); return jedis.del(keys); } /** * Appends a value to the specified value by key * * @param key * @param str * @return */ public static Long append(String key, String str) { Jedis jedis = getJedis(); return jedis.append(key, str); } /** * Determine if key exists * * @param key * @return */ public static Boolean exists(String key) { Jedis jedis = getJedis(); return jedis.exists(key); } /** * Set key value and return 0 if key already exists * * @param key * @param value * @return */ public static Long setnx(String key, String value) { Jedis jedis = getJedis(); return jedis.setnx(key, value); } /** * Set the key value and specify the validity period of the key value * * @param key * @param seconds * @param value * @return */ public static String setex(String key, int seconds, String value) { Jedis jedis = getJedis(); return jedis.setex(key, seconds, value); } /** * Replace the original value from the specified location with key and offset * * @param key * @param offset * @param str * @return */ public static Long setrange(String key, int offset, String str) { Jedis jedis = getJedis(); return jedis.setrange(key, offset, str); } /** * Get batch value from batch key * * @param keys * @return */ public static List<String> mget(String... keys) { Jedis jedis = getJedis(); return jedis.mget(keys); } /** * Batch settings key:value, or one * * @param keysValues * @return */ public static String mset(String... keysValues) { Jedis jedis = getJedis(); return jedis.mset(keysValues); } /** * Batch set key:value, can be one, if key already exists will fail, operation will roll back * * @param keysValues * @return */ public static Long msetnx(String... keysValues) { Jedis jedis = getJedis(); return jedis.msetnx(keysValues); } /** * Set the value of key and return an old value * * @param key * @param value * @return */ public static String getSet(String key, String value) { Jedis jedis = getJedis(); return jedis.getSet(key, value); } /** * Gets the value of the specified subscript position from the subscript and key * * @param key * @param startOffset * @param endOffset * @return */ public static String getrange(String key, int startOffset, int endOffset) { Jedis jedis = getJedis(); return jedis.getrange(key, startOffset, endOffset); } /** * Value is added by key + 1, which returns an error when value is not of type int, and value is 1 when key does not exist * * @param key * @return */ public static Long incr(String key) { Jedis jedis = getJedis(); return jedis.incr(key); } /** * Value is added to the specified value by key, which is the value if the key does not exist * * @param key * @param integer * @return */ public static Long incrBy(String key, long integer) { Jedis jedis = getJedis(); return jedis.incrBy(key, integer); } /** * Subtract the value of the key, or set the key to -1 if it does not exist * * @param key * @return */ public static Long decr(String key) { Jedis jedis = getJedis(); return jedis.decr(key); } /** * Subtract the specified value * * @param key * @param integer * @return */ public static Long decrBy(String key, long integer) { Jedis jedis = getJedis(); return jedis.decrBy(key, integer); } /** * Get the length of the value by key * * @param key * @return */ public static Long strLen(String key) { Jedis jedis = getJedis(); return jedis.strlen(key); } /** * Set the specified value to the field by key, create it first if the key does not exist, and return 0 if the field already exists * * @param key * @param field * @param value * @return */ public static Long hsetnx(String key, String field, String value) { Jedis jedis = getJedis(); return jedis.hsetnx(key, field, value); } /** * Set the specified value to the field by key, and if the key does not exist, create it first * * @param key * @param field * @param value * @return */ public static Long hset(String key, String field, String value) { Jedis jedis = getJedis(); return jedis.hset(key, field, value); } /** * Setting multiple field s of hash simultaneously by key * * @param key * @param hash * @return */ public static String hmset(String key, Map<String, String> hash) { Jedis jedis = getJedis(); return jedis.hmset(key, hash); } /** * Get the specified value through key and field * * @param key * @param failed * @return */ public static String hget(String key, String failed) { Jedis jedis = getJedis(); return jedis.hget(key, failed); } /** * Set key's timeout to seconds * * @param key * @param seconds * @return */ public static Long expire(String key, int seconds) { Jedis jedis = getJedis(); return jedis.expire(key, seconds); } /** * Gets the specified value by key and fields and returns null if there is no corresponding value * * @param key * @param fields Can be a String or an array of Strings * @return */ public static List<String> hmget(String key, String... fields) { Jedis jedis = getJedis(); return jedis.hmget(key, fields); } /** * Add a given value to the value of the specified field by key * * @param key * @param field * @param value * @return */ public static Long hincrby(String key, String field, Long value) { Jedis jedis = getJedis(); return jedis.hincrBy(key, field, value); } /** * Determine whether a specified value exists by key and field * * @param key * @param field * @return */ public static Boolean hexists(String key, String field) { Jedis jedis = getJedis(); return jedis.hexists(key, field); } /** * Number of field s returned by key * * @param key * @return */ public static Long hlen(String key) { Jedis jedis = getJedis(); return jedis.hlen(key); } /** * Delete the specified field by key * * @param key * @param fields Can be a field or an array * @return */ public static Long hdel(String key, String... fields) { Jedis jedis = getJedis(); return jedis.hdel(key, fields); } /** * Return all field s by key * * @param key * @return */ public static Set<String> hkeys(String key) { Jedis jedis = getJedis(); return jedis.hkeys(key); } /** * Returns all key-related value s by key * * @param key * @return */ public static List<String> hvals(String key) { Jedis jedis = getJedis(); return jedis.hvals(key); } /** * Get all field s and value s by key * * @param key * @return */ public static Map<String, String> hgetall(String key) { Jedis jedis = getJedis(); return jedis.hgetAll(key); } /** * Add string to list header via key * * @param key * @param strs Can be a string or a string array * @return Number of value s returned to list */ public static Long lpush(String key, String... strs) { Jedis jedis = getJedis(); return jedis.lpush(key, strs); } /** * Add a string to the end of the list by key * * @param key * @param strs Can be a string or a string array * @return Number of value s returned to list */ public static Long rpush(String key, String... strs) { Jedis jedis = getJedis(); return jedis.rpush(key, strs); } /** * Add string elements before or after the position specified in the list by key * * @param key * @param where LIST_POSITION Enumeration Type * @param pivot list value inside * @param value Added value * @return */ public static Long linsert(String key, BinaryClient.LIST_POSITION where, String pivot, String value) { Jedis jedis = getJedis(); return jedis.linsert(key, where, pivot, value); } /** * Set list value to specify subscript position by key * Error if subscript exceeds number of value s in list * * @param key * @param index Start from 0 * @param value * @return Successfully returned OK */ public static String lset(String key, Long index, String value) { Jedis jedis = getJedis(); return jedis.lset(key, index, value); } /** * Remove the specified count and value identical elements from the corresponding list by key * * @param key * @param count Delete all when count is 0 * @param value * @return Returns the number of deletions */ public static Long lrem(String key, long count, String value) { Jedis jedis = getJedis(); return jedis.lrem(key, count, value); } /** * Keep the value in the list from the strat subscript to the end subscript by key * * @param key * @param start * @param end * @return Successfully returned OK */ public static String ltrim(String key, long start, long end) { Jedis jedis = getJedis(); return jedis.ltrim(key, start, end); } /** * Remove a value from the head of the list by key and return it * * @param key * @return */ public static synchronized String lpop(String key) { Jedis jedis = getJedis(); return jedis.lpop(key); } /** * Remove a value from the end of the list by key and return the element * * @param key * @return */ synchronized public static String rpop(String key) { Jedis jedis = getJedis(); return jedis.rpop(key); } /** * Delete a value from the tail of one list and add it to the head of another list by key, and return the value * Returns null if the first list is empty or does not exist * * @param srckey * @param dstkey * @return */ public static String rpoplpush(String srckey, String dstkey) { Jedis jedis = getJedis(); return jedis.rpoplpush(srckey, dstkey); } /** * Get the value of the subscript position specified in the list by key * * @param key * @param index * @return If no null is returned */ public static String lindex(String key, long index) { Jedis jedis = getJedis(); return jedis.lindex(key, index); } /** * Returns the length of the list by key * * @param key * @return */ public static Long llen(String key) { Jedis jedis = getJedis(); return jedis.llen(key); } /** * Get the value of the list at the specified subscript position by key * Returns value s in all list s if start is 0 end and -1 * * @param key * @param start * @param end * @return */ public static List<String> lrange(String key, long start, long end) { Jedis jedis = getJedis(); return jedis.lrange(key, start, end); } /** * Add value to specified set via key * * @param key * @param members Can be a String or an array of Strings * @return Number of Successful Adds */ public static Long sadd(String key, String... members) { Jedis jedis = getJedis(); return jedis.sadd(key, members); } /** * Delete the corresponding value in the set by key * * @param key * @param members Can be a String or an array of Strings * @return Number of deletions */ public static Long srem(String key, String... members) { Jedis jedis = getJedis(); return jedis.srem(key, members); } /** * Randomly delete a value from a set by key and return it * * @param key * @return */ public static String spop(String key) { Jedis jedis = getJedis(); return jedis.spop(key); } /** * Get the difference set in the set by key * First set as standard * * @param keys Either a string returns all the value s in the set or a string array * @return */ public static Set<String> sdiff(String... keys) { Jedis jedis = getJedis(); return jedis.sdiff(keys); } /** * Get the difference set from the set by key and save it in another key * First set as standard * * @param dstkey key stored in difference set * @param keys Either a string returns all the value s in the set or a string array * @return */ public static Long sdiffstore(String dstkey, String... keys) { Jedis jedis = getJedis(); return jedis.sdiffstore(dstkey, keys); } /** * Gets the intersection in the specified set by key * * @param keys Can be a string or a string array * @return */ public static Set<String> sinter(String... keys) { Jedis jedis = getJedis(); return jedis.sinter(keys); } /** * Get the intersection in the specified set by key and save the result in a new set * * @param dstkey * @param keys Can be a string or a string array * @return */ public static Long sinterstore(String dstkey, String... keys) { Jedis jedis = getJedis(); return jedis.sinterstore(dstkey, keys); } /** * Returns the union of all set s by key * * @param keys Can be a string or a string array * @return */ public static Set<String> sunion(String... keys) { Jedis jedis = getJedis(); return jedis.sunion(keys); } /** * Returns the union of all sets via key and stores them in a new set * * @param dstkey * @param keys Can be a string or a string array * @return */ public static Long sunionstore(String dstkey, String... keys) { Jedis jedis = getJedis(); return jedis.sunionstore(dstkey, keys); } /** * Remove the value from the set by key and add it to the second set * * @param srckey Need to be removed * @param dstkey Added * @param member set value in * @return */ public static Long smove(String srckey, String dstkey, String member) { Jedis jedis = getJedis(); return jedis.smove(srckey, dstkey, member); } /** * Get the number of value s in the set by key * * @param key * @return */ public static Long scard(String key) { Jedis jedis = getJedis(); return jedis.scard(key); } /** * Use key to determine if value is an element in a set * * @param key * @param member * @return */ public static Boolean sismember(String key, String member) { Jedis jedis = getJedis(); return jedis.sismember(key, member); } /** * Get the random value in the set by key without deleting the element * * @param key * @return */ public static String srandmember(String key) { Jedis jedis = getJedis(); return jedis.srandmember(key); } /** * Get all value s in the set by key * * @param key * @return */ public static Set<String> smembers(String key) { Jedis jedis = getJedis(); return jedis.smembers(key); } /** * Add value,score to zset by key, where score is used to sort * Update elements based on score if the value already exists * * @param key * @param score * @param member * @return */ public static Long zadd(String key, double score, String member) { Jedis jedis = getJedis(); return jedis.zadd(key, score, member); } /** * Delete the value specified in zset by key * * @param key * @param members Can be a string or a string array * @return */ public static Long zrem(String key, String... members) { Jedis jedis = getJedis(); return jedis.zrem(key, members); } /** * Increase the value of the score of the value in the zset by key * * @param key * @param score * @param member * @return */ public static Double zincrby(String key, double score, String member) { Jedis jedis = getJedis(); return jedis.zincrby(key, score, member); } /** * Returns the rank of value in zset by key * Sort subscripts from smallest to largest * * @param key * @param member * @return */ public static Long zrank(String key, String member) { Jedis jedis = getJedis(); return jedis.zrank(key, member); } /** * Returns the rank of value in zset by key * Sort subscripts from large to small * * @param key * @param member * @return */ public static Long zrevrank(String key, String member) { Jedis jedis = getJedis(); return jedis.zrevrank(key, member); } /** * Get the value of the zset in the scope from start to end by key * socre Sort from large to small * Return all when start is 0 end and -1 * * @param key * @param start * @param end * @return */ public static Set<String> zrevrange(String key, long start, long end) { Jedis jedis = getJedis(); return jedis.zrevrange(key, start, end); } /** * Returns the value in the zset within the specified scope by key * * @param key * @param max * @param min * @return */ public static Set<String> zrangebyscore(String key, String max, String min) { Jedis jedis = getJedis(); return jedis.zrevrangeByScore(key, max, min); } /** * Returns the value in the zset within the specified scope by key * * @param key * @param max * @param min * @return */ public static Set<String> zrangeByScore(String key, double max, double min) { Jedis jedis = getJedis(); return jedis.zrevrangeByScore(key, max, min); } /** * Returns the number of value s in the zset within a specified interval * * @param key * @param min * @param max * @return */ public static Long zcount(String key, String min, String max) { Jedis jedis = getJedis(); return jedis.zcount(key, min, max); } /** * Returns the number of value s in zset by key * * @param key * @return */ public static Long zcard(String key) { Jedis jedis = getJedis(); return jedis.zcard(key); } /** * Get the score value of value in zset by key * * @param key * @param member * @return */ public static Double zscore(String key, String member) { Jedis jedis = getJedis(); return jedis.zscore(key, member); } /** * Delete elements within a given interval by key * * @param key * @param start * @param end * @return */ public static Long zremrangeByRank(String key, long start, long end) { Jedis jedis = getJedis(); return jedis.zremrangeByRank(key, start, end); } /** * Delete elements within a specified scope by key * * @param key * @param start * @param end * @return */ public static Long zremrangeByScore(String key, double start, double end) { Jedis jedis = getJedis(); return jedis.zremrangeByScore(key, start, end); } /** * Returns all key s that satisfy the pattern expression * keys(*) * Return all key s * * @param pattern * @return */ public static Set<String> keys(String pattern) { Jedis jedis = getJedis(); return jedis.keys(pattern); } /** * Judging the type of value by key * * @param key * @return */ public static String type(String key) { Jedis jedis = getJedis(); return jedis.type(key); }