Pay attention to the official account number: CoderBuff, reply to "redis" and get the complete version of Redis5.x tutorial PDF.
- Redis5.x tutorial directory
- Chapter I preparation
- Chapter II data types
- Chapter III orders
- Chapter IV configuration
- Chapter 5 Java client (I)
- Chapter VI affairs
- Chapter 7 distributed lock
- Chapter 8 Java client (2)
Chapter III orders
According to the steps in Chapter 1, we enter the redis command line interaction through the redis cli command.
This chapter will focus on the data types in the previous chapter and operate different Redis commands for different data types.
String (string)
Read / write / delete simple command
Write command is implemented by set keyword, set [key] [value].
127.0.0.1:6379> set hello world OK
The read command is implemented by the get keyword, get [key].
127.0.0.1:6379> get hello "world"
Delete command is implemented by del keyword (delete command can be applied to all data types), del [key].
127.0.0.1:6379> del hello (integer) 1
The string data type also has an mset to write one or more string values at the same time, mset [key1] [value1] [key2] [value2].
127.0.0.1:6379> mset key1 value1 key2 value2 OK
Auto increment / Auto decrement command
The auto increment + 1 command is implemented by the incr keyword, incr [key].
127.0.0.1:6379> set hello 1 #Write a value of 1 with key as hello OK 127.0.0.1:6379> get hello #Read the value with key as hello "1" 127.0.0.1:6379> incr hello #Increase the value of key to hello by + 1 (integer) 2 127.0.0.1:6379> get hello #Read the value with key as hello "2"
The self decrement-1 command is implemented by decr keyword, decr [key].
127.0.0.1:6379> set world 1 #Write a value of 1 with key as world OK 127.0.0.1:6379> get world #Read the value with key as world "1" 127.0.0.1:6379> decr world #Reduce the value of key to world by - 1 (integer) 0 127.0.0.1:6379> get world #Read the value with key as world "0"
The auto increment arbitrary integer is implemented by incrby, incrby [key] [number].
127.0.0.1:6379> set coderbuff 1 #Write a value of 1 with key as coderbuff OK 127.0.0.1:6379> get coderbuff #Read the value with key as coderbuff "1" 127.0.0.1:6379> incrby coderbuff 10 #Increase the value of key to coderbuff by + 10 (integer) 11 127.0.0.1:6379> get coderbuff #Read the value with key as coderbuff "11"
Any integer of self subtraction is implemented by decrby, decrby [key] [number].
127.0.0.1:6379> set coderbook 1 #Write a value of 1 with key as coderbook OK 127.0.0.1:6379> get coderbook #Read the value with key as coderbook "1" 127.0.0.1:6379> decrby coderbook 10 #Increase the value of key coderbook by - 10 (integer) -9 127.0.0.1:6379> get coderbook #Read the value with key as coderbook "-9"
Add any floating-point number through incrbyfloat, incrbyfloat [key] [float].
127.0.0.1:6379> set coderchat 1 #Write a value of 1 with key as coderchat OK 127.0.0.1:6379> get coderchat #Read the value with key as coderchat "1" 127.0.0.1:6379> incrbyfloat coderchat 1.1 #Increase the value of key as coderchat by + 1.1 "2.1" 127.0.0.1:6379> get coderchat #Read the value with key as coderchat "2.1"
String operation command
In redis, you can not only use the above commands for string data types, but also add values and get substrings like Java.
Append the value value to the specified key to the end through append, append [key] [append [u string].
127.0.0.1:6379> set say hello #Write the value of "key" as "say" as "hello" OK 127.0.0.1:6379> get say #Read the value with key as say "hello" 127.0.0.1:6379> append say world #Add world at the end of value value with key as say (integer) 10 #Return the length of the current value value of key 127.0.0.1:6379> get say #Read the value with key as say "helloworld"
Next, this example obtains the substring through the command getrange, getrange [key] [start] [end].
127.0.0.1:6379> getrange say 1 10 #Get substrings at positions 1 to 10 "elloworld" 127.0.0.1:6379> get say #As you can see, although we got the substring above, we did not modify the original string "helloworld"
In the same example, we use the command setrange to replace the substring with the given value, and we will give two key parameters. The first parameter is [start] to indicate where to start the replacement, the second parameter is [value] to indicate the content of the replacement, and setrange [key] [start] [value].
127.0.0.1:6379> setrange say 1 i (integer) 10 127.0.0.1:6379> get say "hilloworld"
Binary command
Any data is stored in the form of binary bits in the operating system. redis provides binary operation for it in string type. In general, it may not be used much, but it can be used to achieve some "clever" design.
For example, in the pin message, we send a message to show "read" and "unread" people. We need to store these two information in redis. How should we design it?
We designed a message's key value structure as "[user [ID]: [MSG [ID]", so key = "1:100" means "message ID sent with user ID 1 is 100". Note that at this time, if the user ID=2 reads the message, it will be written through the command setbit 1:100 21. If the user ID=100 reads the message, it will be written through setbit 1:100 101. The meaning of this command is to write 1 to binary bit 2 of key=1:100, 1 to binary bit 10 of key=1:100, 1 to read, and 0 to unread.
127.0.0.1:6379> set 1:100 0 #Define key=1:100, which means message ID 100 sent by user ID 1 OK 127.0.0.1:6379> setbit 1:100 0 0 #Initialization 1:100 bit 0 to bit 10 binary is 0, which means that just sent out is unread. (integer) 0 127.0.0.1:6379> setbit 1:100 1 0 (integer) 0 127.0.0.1:6379> setbit 1:100 2 0 (integer) 1 127.0.0.1:6379> setbit 1:100 3 0 (integer) 1 127.0.0.1:6379> setbit 1:100 4 0 (integer) 0 127.0.0.1:6379> setbit 1:100 5 0 (integer) 0 127.0.0.1:6379> setbit 1:100 6 0 (integer) 0 127.0.0.1:6379> setbit 1:100 7 0 (integer) 0 127.0.0.1:6379> setbit 1:100 8 0 (integer) 0 127.0.0.1:6379> setbit 1:100 9 0 (integer) 0 127.0.0.1:6379> setbit 1:100 10 0
Above we initialize a bitmap. Next, when user ID=2 and user ID=10 read the message.
127.0.0.1:6379> setbit 1:100 1 1 #In fact, while sending out the message, I have read it myself (integer) 0 127.0.0.1:6379> setbit 1:100 2 1 #User ID=2 read this message (integer) 0 127.0.0.1:6379> setbit 1:100 10 1 #User ID=10 read this message (integer) 0
We can judge which users have read the message through the getbit command. For example, we can judge whether user ID=3 has read the message.
127.0.0.1:6379> getbit 1:100 3 #Read binary bit 3 binary value with key 1:100 (integer) 0 #Return 0 for unread
Determine whether user ID=10 has read this message.
127.0.0.1:6379> getbit 1:100 10 #Read the binary value of bit 10 with key 1:100 (integer) 1 #Return 1 for read
We can also count the number of 1 by bitcount, that is, how many people have read the message.
127.0.0.1:6379> bitcount 1:100 #Count the number of binary bits 1 (integer) 3 #There are only three, which is consistent with the above assumption. Users themselves (user ID=1) and users with user ID=2 and user ID=10 read this message
Finally, there is a command about binary bits, bitop [operation] [result] [key1] [key2], which can perform binary operations on the binary bits of multiple key values, including AND, OR, XOR, NOT not. The calculation results are saved in [result].
List (list)
Push / Pop common commands
Push one or more values to the right or left through rpush, lpush.
rpush [key] [value1] [value2], push the value value to the right end of the list.
lpush [key] [value1] [value2], push the value value to the left end of the list.
127.0.0.1:6379> rpush books java #Push the value java to the right side of the list with the key value of books (integer) 1 127.0.0.1:6379> lpush books c #Push the value c to the left of the list with the key value of books (integer) 2 127.0.0.1:6379> rpush books python #Push the value python to the right of the list with the key value of books (integer) 3 127.0.0.1:6379> lrange books 0 -1 #View the list with the key value of books 1) "c" 2) "java" 3) "python"
Next, the above example, through rpop and lpop, removes and returns the most backend and leftmost elements in the list.
rpop [key], remove the last element of the list.
lpop [key], remove the leftmost element from the list.
127.0.0.1:6379> rpop books #Remove the element whose list key value is the rightmost end of books and return "python" 127.0.0.1:6379> lrange books 0 -1 #View all elements of the list with key as books 1) "c" 2) "java" 127.0.0.1:6379> lpop books #Remove the leftmost element of the list whose key value is books and return "c" 127.0.0.1:6379> lrange books 0 -1 #View all elements of the list with key as books 1) "java"
After introducing push and pop-up commands, the commands for viewing with the list range are described next.
lrange [key] [start] [end] command is used to return all elements in the list from [start] to [end]. Note that elements in [start] and [end] will also be returned. The above example has shown this command.
127.0.0.1:6379> rpush level A B C D #Push value: A B C D continuously to the right side of the list with key level (integer) 4 127.0.0.1:6379> lrange level 0 1 #Returns the elements of positions 0-1 with key level 1) "A" 2) "B" 127.0.0.1:6379> lrange level 0 -1 #View all elements whose key is level in the list 1) "A" 2) "B" 2) "C" 2) "D"
The lindex [key] [index] command is used to return the element at the specified location [index], and the above example is still used.
127.0.0.1:6379> lindex level 3 "D"
Neither lrange nor lindex modify the original list value, but ltrim is used to "build" the list value.
ltrim [key] [start] [end] means that only all elements of the list from [start] to [end] are reserved. Note that the above example is also used to include elements located in [start], [end].
127.0.0.1:6379> lrange level 0 -1 #View all elements whose key is level in the list 1) "A" 2) "B" 2) "C" 2) "D" 127.0.0.1:6379> ltrim level 1 2 #Keep elements with list positions 1-2 OK 127.0.0.1:6379> lrange level 0 -1 #View all elements whose key is level in the list 1) "B" 2) "C"
These are the basic commands of the list. There are also some more "advanced" commands: move elements from one queue to another, or execute pop-up commands in blocking mode until new elements are added to the list. I will not introduce it here. I will talk about some applications of redis later.
Hash (hash)
The hmset command can write a value of hash type, hmset [key] [field1] [value1] [field2] [value2].
127.0.0.1:6379> hmset okevin name kevin sex male OK
The hmget command can read the value of hash type, hmget [key] [field].
127.0.0.1:6379> hmget okevin name 1) "kevin"
hlen returns the number of key value pairs contained in the hash, hlen [key].
127.0.0.1:6379> hlen okevin (integer) 2
Hmgetall returns all key value pairs contained in the hash, hmgetall [key].
127.0.0.1:6379> hgetall okevin #Return all key value pairs, odd column is field, even column is value 1) "name" 2) "kevin" 3) "sex" 4) "male"
The hexists command checks whether the given field exists in the hash value, returns 0 for nonexistence, 1 for existence, and hexists [key] [field].
127.0.0.1:6379> hexists okevin name (integer) 1 127.0.0.1:6379> hexists okevin age (integer) 0
Hkeys gets all the field keys contained in the hash, hkeys [key].
127.0.0.1:6379> hkeys okevin 1) "name" 2) "sex"
Hvals gets the value value of all field s contained in the hash, hvals [key].
127.0.0.1:6379> hvals okevin 1) "kevin" 2) "male"
Hincrby automatically increments any integer (similar to incrby of string type) to the field key specified in hash, hincrby [key] [field] [number].
127.0.0.1:6379> hmset okevin age 0 #New age field value is 0 OK 127.0.0.1:6379> hmget okevin age #Get age 1) "0" 127.0.0.1:6379> hincrby okevin age 25 #Add "25" integer to "age" field (integer) 25 127.0.0.1:6379> hmget okevin age #Get age 1) "25"
Hincrbyfloat adds any floating-point number to the file key specified in hash (similar to incrbyfloat of string type), hincrbyfloat [key] [field] [number].
127.0.0.1:6379> hincrbyfloat okevin age 0.5 "25.5" 127.0.0.1:6379> hmget okevin age 1) "25.5"
hdel deletes the file D specified in the hash, hmdel [key] [field].
127.0.0.1:6379> hgetall okevin 1) "name" 2) "kevin" 3) "sex" 4) "male" 5) "age" 6) "25.5" 127.0.0.1:6379> hdel okevin age (integer) 1 127.0.0.1:6379> hgetall okevin 1) "name" 2) "kevin" 3) "sex" 4) "male"
Set (set)
As mentioned in the previous chapter, a set is a data type that stores different elements in an unordered manner. It is similar to the set type in Java. It also has the basic operations of adding, deleting, reading, and the operation between two sets.
Common commands such as read / write
The sadd command adds one or more elements to the collection and returns the number of elements in the collection that do not exist in the added elements. sadd [key] [member] [member].
127.0.0.1:6379> sadd students kevin yulinfeng (integer) 2
The sismember command checks whether the element exists in the collection, returns 1 for existence, 0 for nonexistence, and sismember [key] [member].
127.0.0.1:6379> sismember students kevin (integer) 1 127.0.0.1:6379> sismember students linfeng (integer) 0
The smembers command returns all elements contained in the collection, smembers [key].
127.0.0.1:6379> smembers students 1) "yulinfeng" 2) "kevin"
The scar command returns the number of elements in the collection, scard s [key].
127.0.0.1:6379> scard students (integer) 2
The srandmember command returns one or more elements randomly from the collection. When the number of returned elements is set to a positive number, the elements will not repeat. When the number of returned elements is set to a negative number, the elements may repeat. srandmember [key] [count].
127.0.0.1:6379> srandmember students 1 1) "yulinfeng" 127.0.0.1:6379> srandmember students 1 1) "kevin" 127.0.0.1:6379> srandmember students 3 1) "kevin" 2) "yulinfeng" 127.0.0.1:6379> srandmember students -3 1) "yulinfeng" 2) "kevin" 3) "kevin"
The srem command removes one or more specified elements from the collection and returns the number of elements removed, srem [key] [member] [member].
Multiple collection commands
Redis can operate on multiple sets, such as difference set operation and intersection operation in mathematics. The following is just a list of commands for multiple set operations, no more examples, but you must know that redis can also perform multiple set operations if you have scenarios.
sdiff [key1] [key2]…… The command returns elements (subtraction operations) that exist in key1 but do not exist in other sets (key2).
sdiff [dest-key] [key1] [key2]…… The command is also the same as the subtraction operation, but it stores the element in the dest key key key.
sinter [key1] [key2]…… The command returns the elements (intersection operation) in all sets (key1, key2) at the same time.
sinterstore [dest-key] [key1] [key2]…… The command is also the same as the intersection operation, but it stores the element in the dest key key key.
sunion [key1] [key2]…… The command returns the elements (Union operation) in all sets (key1, key2).
sunionstore [dest-key] [key1] [key2]…… The command is also the same as the union operation, but it stores the element in the dest key key key.
Ordered set
In the previous chapter, we talked about that although the data type of "ordered set" is more like the ordered version of "set", it is more similar to "hash" in terms of data structure. It is the same as the hash type, the value is also k-v form, the difference is that the ordered set of v represents the "fraction" used for sorting.
Common commands such as read / write
Zadd is used for write operation of ordered collection, zadd [key] [score1] [member1] [score2] [member2].
127.0.0.1:6379> zadd sorted 2 a 3 b (integer) 2
The zcard command returns the number of members in an ordered collection, zcard [key].
127.0.0.1:6379> zcard sorted (integer) 2
Zrank is used to return the ranking of members in an ordered set (sorted by score), zrank [key] [member].
127.0.0.1:6379> zrank sorted a (integer) 0 127.0.0.1:6379> zrank sorted b (integer) 1
The zrevrank command also returns the ranking of member, but it is the opposite of the zrank sorting rule. It is arranged by score from large to small, zrevrank [key] [member].
127.0.0.1:6379> zrevrank sorted a (integer) 1 127.0.0.1:6379> zrevrank sorted b (integer) 0
The zscore command returns the score of the member, zscore [key] [member].
127.0.0.1:6379> zscore sorted a "2"
The zrange [key] [start] [stop] (WithCores) command is used to return the members ranking between start and stop in an ordered set (sorted by score from small to large). The WithCores parameter can optionally indicate whether to return a score. Start and end refer to the number of members ranking from 0.
127.0.0.1:6379> zrange sorted 0 0 withscores 1) "a" 2) "2" 127.0.0.1:6379> zrange sorted 0 1 withscores 1) "a" 2) "2" 3) "b" 4) "3"
Zrevrange is similar to zrange, but it is arranged by fractions from large to small, zrevrange [key] [start] [End] (with cores).
127.0.0.1:6379> zrevrange sorted 0 0 withscores 1) "b" 2) "3" 127.0.0.1:6379> zrevrange sorted 0 1 withscores 1) "b" 2) "3" 3) "a" 4) "2"
Zcount [key] [min'score] [max'score] returns the number of members whose score is between min'score and max'score.
127.0.0.1:6379> zcount sorted 0 2 (integer) 1 127.0.0.1:6379> zcount sorted 0 3 (integer) 2
The zincrby [key] [increment] [MEMBER] command is used to add an increment to the score of a member.
127.0.0.1:6379> zincrby sorted 2 a "4"
The zrem command is used to delete the members specified in the ordered collection, zrem [key] [member1] [member2].
127.0.0.1:6379> zrem sorted a (integer) 1
Multiple ordered set commands
Like a set, an ordered set can operate on multiple sets, such as intersection, union, and so on.
zinterstore is used for intersection operations.
zunionstore is used for union operation.
Pay attention to the official account number: CoderBuff, reply to "redis" and get the complete version of Redis5.x tutorial PDF.
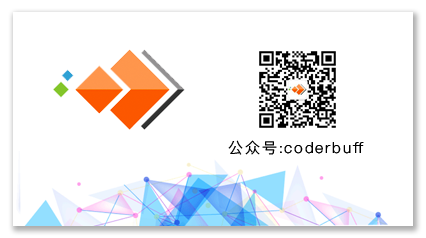