What is a Scroller?
When translated into elastic sliding object, you can realize the elastic sliding animation of View. What is related to Scroller is the familiar scrollTo and scrollBy methods, which can be used to realize the sliding of View, but their disadvantages are that they are completed in an instant and cannot be smoothly transitioned, and Scroller can help us smooth the elastic sliding.
Use
Generally used in custom View, it can realize the elastic sliding effect of View
1. Customize a View with detailed comments
/** * There is a Scroller in the custom View, which can achieve a very smooth scrolling effect. Like animation, it can control how long it takes to scroll to the pointing position * * @author yangfan * */ public class DIYView extends LinearLayout { // Create a Scroller private Scroller mScroller; public DIYView(Context context) { this(context, null); } // 1. Create a Scroller public DIYView(Context context, AttributeSet attrs) { super(context, attrs); mScroller = new Scroller(context); } // 2, touch callback, add 100 to X axis every time, then call smoothScrollTo. @Override public boolean onTouchEvent(MotionEvent event) { int disX = mScroller.getFinalX() + 100; Log.e("***************", "onTouchEvent"); smoothScrollTo(disX, 0); return super.onTouchEvent(event); } // 3. Call smoothScrollBy according to the coordinate difference public void smoothScrollTo(int fx, int fy) { int dx = fx - mScroller.getFinalX(); int dy = fy - mScroller.getFinalY(); smoothScrollBy(dx, dy); } // 4. Call startScroll to set the coordinates, and then invalidate the redraw public void smoothScrollBy(int dx, int dy) { // Parameter 1: startX parameter 2: startY is the position to start rolling, dx,dy is the offset of rolling, 1500ms is the time to complete rolling mScroller.startScroll(mScroller.getFinalX(), mScroller.getFinalY(), dx, dy, 3000); invalidate(); } // 5. The redraw process will call computeScroll to actually call scrollTo to scroll and then redraw @Override public void computeScroll() { // Judge whether the scrolling is complete true is incomplete if (mScroller.computeScrollOffset()) { scrollTo(mScroller.getCurrX(), mScroller.getCurrY()); // In this case, both postInvalidate and invalidate can be called invalidate(); } super.computeScroll(); } }
2. Use custom View in layout
<com.abc.edu.scroll.DIYView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#ffffff" > <!-- Get a tip text --> <TextView android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#ff0000" android:gravity="center" android:text="Please slide left" android:textSize="30sp" /> </com.abc.edu.scroll.DIYView>
3. Run the test, and then swipe your finger on the screen for several times
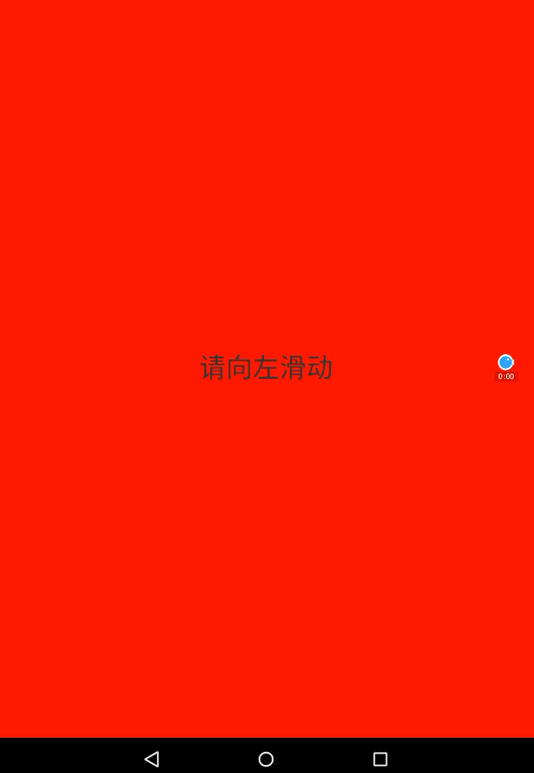
Attention points
Scroller itself is not able to achieve View slipping. In essence, View redraws, redraws the computeScroll method invoking View, implements the specific implementation of the sliding method in this method, and then calls the redraw function, so as to form a continuous sliding animation on the interface.