Section 5 Conditions and Cycles
Posted by derchris on Tue, 30 Jul 2019 01:41:41 +0200
1. Conditional statements
(1) Run if satisfied, jump to else if not satisfied, run else content
For example:
a=1;b=2
if a>b: # If statement must end with a colon, if must be followed by a judgment statement
print('a Larger') # To execute a statement, you must have
elif a==b: # elif can make many or none
print('b and b The same big as that')
elif a<b:
print('b Larger')
else: # Can be understood as final condition(otherwise),Or not
pass # placeholder
Run result:
2. Hint input: built-in function input()
b=input('Please enter b: ') #Define what you enter as b and what you enter as a string
Run result:
3. Make a small game using conditional function: check the results
a=input('Please enter a 0-100 Number:')
a=int(a) #Cast type to int
if 100>=a>=90:
print('Excellent')
elif 90>a>=75:
print('Good')
elif 75>a>=60:
print('pass')
elif 60>a>=0:
print('Failed')
else:
print('wrong range of input numbers')
Output results:

4. Random number random
Import random #import random number module
a=random.randint(1,10)#Defines a as a random number within 1 to 10, both 1 and 10 are accessible, randInt represents a positive integer
print(a)
Output: Random numbers in 1 to 10

Random.random() # [0,1) random floating point number, left closed right open
Random numbers within random.randrange(10)#10, excluding 10
Random.sample (range (10, 6)# Random numbers within 10 produce 6, also excluding 10
2. Circular statements
1. while Loop
a=1
while a<4: # After while, you must add a judgment that True is running the execution statement
print('666',end=' ') # Execute Statement
a+=1 # Execute Statement
Output results:
2. break and continue
while True:
continue #Break the cycle, jump out of this cycle, the cycle continues
break #End the cycle, terminate the entire cycle
3,range
Represent scope
range(10) #Left Close Right Open, default step is 1, 0-9 range
range(1,10) #Represents the range between 1 and 9
range(1,10,2) #Represents a range from 1 to 9 with a step of 2
4. for loop
for i in range(1,10): # i is item and the range (1,10) section needs to have iterative elements
print(i,end=' ') # Execute Statement
Output results:
5,else
while True:
break
else:
print('OK')
for item in iterable:
break
else:
print('OK')
#Only loops that end normally will the else part be executed by loops that do not end with break s
6. The similarities and differences between while and for
Same thing: they all do a repeating thing in a cycle
The difference: while stops looping when conditions are unsuccessful
for stops looping when Iterable objects are exhausted
7. Nested loops
for a in range(1,6):
for b in range(1,6):
print('No.%d That's ok%s position'%(a,b),end='')
print()
#The outer loop runs one first, the inner loop traverses once, and so on until the outer loop traverses to the end
Run result:
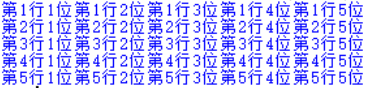
3. Operations
1. Write a game of guessing numbers, which requires: the system generates a random number (1-10), and the user has three opportunities to enter numbers to guess.If the number of inputs is small or large, the corresponding prompt is given.If the input number is equal to the random number, the prompt is "Congratulations on your guess!"
import random #Import Random Numbers
a=int(random.randint(1,10)) #Casts a random number and defines it as a
c=1
while c<4: #Set up a cycle, from which you can see that the cycle runs three times
b=input('Please enter the number you guessed (1)-10)b: ') #Input instructions, defined as b
if b.isdigit(): #Run the execution command if b is a positive integer, otherwise output follows the rules of the game
b=int(b)
if b==a:
print('Congratulations on your correct answer')
break #When the guess is right, output the guessed result and terminate the loop
elif b>a:
print('Big')
else:
print('Small')
else:
print('Please follow the rules of the game')
c+=1 #Origin of the cubic cycle
Output results:
2. Output 9*9 Multiplication Tips
for x in range(1,10): # x traverses 1 to 10
print() # Run once to wrap lines
for y in range(1,x+1): # y iterates through 1 to x+1 and jumps out of the loop after running the execution command when x is the same
print('%d*%d=%d'%(y,x,x*y),end=' ') #Output desired format
for x in range(1,10):
for y in range(1,10):
print('%d*%d=%d'%(y,x,x*y),end=' ')
if x==y:
print('')
break #Each time x==y, terminate the inner loop and jump to the outer loop
Run result:
Both can be output
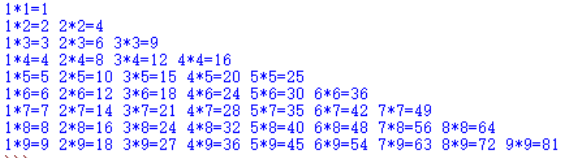