Several methods to find the maximum and minimum values in an integer array
Posted by gmp on Sun, 19 Jul 2020 16:30:03 +0200
In integer arrays, we need to get the maximum and minimum values of array elements from them:
Method 1: Sort using Array first, then from the sorted array, the first element is the smallest and the last element is the largest.
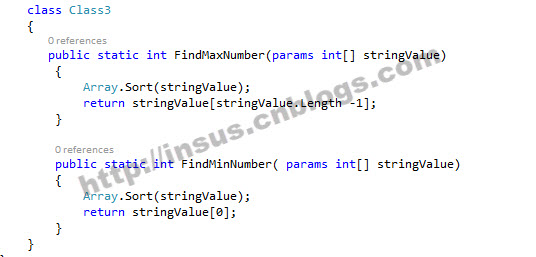

public static int FindMaxNumber(params int[] stringValue)
{
Array.Sort(stringValue);
return stringValue[stringValue.Length -1];
}
public static int FindMinNumber( params int[] stringValue)
{
Array.Sort(stringValue);
return stringValue[0];
}
Source Code
Method 2: This method declares a variable whose value is the first element value in the array.Then start the loop from the second element and compare it with the variable.
Find the maximum element, and assign the value of the element to the variable if the value being compared is larger than the variable.
Finding the minimum element is the opposite of finding the maximum value. If the value being compared is less than the variable, the value being compared is assigned to the variable.
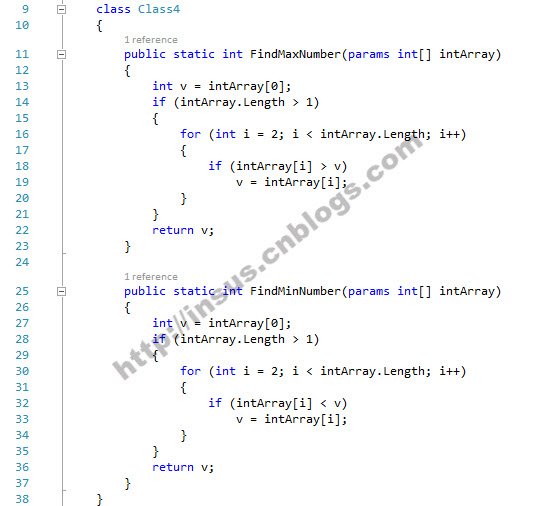

public static int FindMaxNumber(params int[] intArray)
{
int v = intArray[0];
if (intArray.Length > 1)
{
for (int i = 2; i < intArray.Length; i++)
{
if (intArray[i] > v)
v = intArray[i];
}
}
return v;
}
public static int FindMinNumber(params int[] intArray)
{
int v = intArray[0];
if (intArray.Length > 1)
{
for (int i = 2; i < intArray.Length; i++)
{
if (intArray[i] < v)
v = intArray[i];
}
}
return v;
}
Source Code