Shell overview
Getting started with Shell scripts
1. Script format
2. Create a Shell script: HelloWorld
tdx96@ubuntu:~/datas$ touch hellworld.sh tdx96@ubuntu:~/datas$ vim hellworld.sh
Input:
#!/bin/bash echo "helloworld today"
Execution:
tdx96@ubuntu:~/datas$ sh hellworld.sh helloworld today
perhaps
tdx96@ubuntu:~/datas$ bash hellworld.sh helloworld today
If you directly call Hellworld sh
tdx96@ubuntu:~/datas$ ./hellworld.sh -bash: ./hellworld.sh: insufficient privilege
resolvent:
tdx96@ubuntu:~/datas$ chmod 777 hellworld.sh tdx96@ubuntu:~/datas$ ll Total consumption 12 drwxrwxr-x 2 tdx96 tdx96 4096 8 January 2:25 ./ drwxr-xr-x 29 tdx96 tdx96 4096 8 January 2:25 ../ -rwxrwxrwx 1 tdx96 tdx96 37 8 January 2:25 hellworld.sh*
Re call
tdx96@ubuntu:~/datas$ ./hellworld.sh helloworld today
About chmod 777
The chmod (change mode) command is a command that controls the user's permissions on files
Followed by three user permission levels
Because the permissions of files are divided into three types: u (file owner), g (file group user) and O (other users), 777 means that u, g and o are 777 permissions
The first is the permission of u, that is, the permission of the file Owner. 4 table read, r table d write, x Table execution permission.
The second is the permission of g, that is, the permission of the group user (Group) of the file.
The third is the permission of o, that is, the permission of Other Users.
777 permission is an unsafe permission, because each user has all permissions. For some files or execution files, any user can modify and execute them. In fact, it is not friendly to the system, because permission restrictions cannot be achieved, so when authorizing files later, you should think about the file permissions~~
3. Create a Shell script: multi command processing
Steps:
1. Create sh file
tdx96@ubuntu:~/datas$ touch batch.sh tdx96@ubuntu:~/datas$ ls batch.sh hellworld.sh
2. Enter the command to be executed in the sh file
tdx96@ubuntu:~/datas$ vim batch.sh #!/bin/bash cd /home/tdx96/datas/ touch banzhang.txt echo "I Love You">>banzhang.txt
3. Implementation
tdx96@ubuntu:~/datas$ bash batch.sh
4. View the effect
tdx96@ubuntu:~/datas$ cat banzhang.txt I Love You
Variables in Shell
1. System variables
2. User defined variables
1. Define variables
No declaration type is required
tdx96@ubuntu:/$ A=1 tdx96@ubuntu:/$ echo $A 1
2. Undo variables
tdx96@ubuntu:/$ unset A tdx96@ubuntu:/$ echo $A tdx96@ubuntu:/$
3. Declare static variables
readonly variable. Note that unset is not allowed
tdx96@ubuntu:/$ readonly B=3 tdx96@ubuntu:/$ echo $B 3 tdx96@ubuntu:/$ unset B bash: unset: B: Unable to cancel the setting: read-only variable tdx96@ubuntu:/$
Variable definition rules:
For (3):
tdx96@ubuntu:/$ C=1+1 tdx96@ubuntu:/$ echo $C 1+1 tdx96@ubuntu:/$
resolvent:
tdx96@ubuntu:/$ C=$((1+1)) tdx96@ubuntu:/$ echo $C 2
For (4)
tdx96@ubuntu:/$ D="banzhang love you" tdx96@ubuntu:/$ echo $D banzhang love you tdx96@ubuntu:/$
Promote variables to global variables that can be used by other Shell programs
Let's first look at the local variable D
tdx96@ubuntu:~/datas$ vim hellworld.sh #!/bin/bash echo "helloworld today" echo $D
tdx96@ubuntu:~/datas$ sh hellworld.sh helloworld today
Variable D cannot be used
Promote D to a global variable:
tdx96@ubuntu:~/datas$ export D tdx96@ubuntu:~/datas$ sh hellworld.sh helloworld today banzhang love you
3. Special variables
1.$n
example:
tdx96@ubuntu:~/datas$ touch parameter.sh tdx96@ubuntu:~/datas$ vim parameter.sh
#!/bin/bash echo "$0 $1 $2 $3"
$0 means script name: parameter sh
tdx96@ubuntu:~/datas$ sh parameter.sh parameter.sh
$1 $2 $3 can be assigned on the command line
tdx96@ubuntu:~/datas$ sh parameter.sh banzhang parameter.sh banzhang tdx96@ubuntu:~/datas$ sh parameter.sh banzhang love parameter.sh banzhang love tdx96@ubuntu:~/datas$ sh parameter.sh banzhang love you parameter.sh banzhang love you
2.$#
example:
vim parameter.sh
#!/bin/bash echo "$0 $1 $2 $3" echo "$#"
tdx96@ubuntu:~/datas$ sh parameter.sh banzhang parameter.sh banzhang 1
tdx96@ubuntu:~/datas$ sh parameter.sh banzhang love parameter.sh banzhang love 2
tdx96@ubuntu:~/datas$ sh parameter.sh banzhang love you parameter.sh banzhang love you 3
3.$* $@
example:
tdx96@ubuntu:~/datas$ vim parameter.sh
#!/bin/bash echo "$0 $1 $2 $3" echo "$#" echo $* echo $@ ~
tdx96@ubuntu:~/datas$ sh parameter.sh banzhang love you parameter.sh banzhang love you 3 banzhang love you banzhang love you
4.$?
example:
tdx96@ubuntu:~/datas$ lsd Command 'lsd' not found, but can be installed with: sudo snap install lsd tdx96@ubuntu:~/datas$ echo $? 127 tdx96@ubuntu:~/datas$ ls banzhang.txt batch.sh hellworld.sh parameter.sh tdx96@ubuntu:~/datas$ echo $? 0
operator
example:
expr:
tdx96@ubuntu:~/datas$ expr 3 + 2 5 tdx96@ubuntu:~/datas$ expr 3+2 3+2 tdx96@ubuntu:~/datas$
tdx96@ubuntu:~/datas$ expr `expr 2 + 3` \* 4 20
$[]
tdx96@ubuntu:~/datas$ s=$[(2+3)*4] tdx96@ubuntu:~/datas$ s s: Command not found tdx96@ubuntu:~/datas$ echo $s 20 tdx96@ubuntu:~/datas$
Conditional judgment
1. Basic grammar
2. Common judgment conditions
3. Examples:
Judge whether 23 is greater than or equal to 22
tdx96@ubuntu:~/datas$ [ 23 -ge 22 ] tdx96@ubuntu:~/datas$ echo $? 0
A return value of 0 indicates that the previous command was executed correctly
Judge whether 23 is less than or equal to 22
tdx96@ubuntu:~/datas$ [ 23 -le 22 ] tdx96@ubuntu:~/datas$ echo $? 1
A return value of 1 indicates that the previous command was not executed correctly
Determine whether hellworld has writable permissions
tdx96@ubuntu:~/datas$ [ -w hellworld.sh ] tdx96@ubuntu:~/datas$ echo $? 0
Have write permission
Determine whether a file exists
tdx96@ubuntu:~/datas$ [ -e /home/tdx96/datas/banzhang.txt ] tdx96@ubuntu:~/datas$ echo $? 0
Multi condition judgment: two or more [] are connected by & & or 𞓜
tdx96@ubuntu:~/datas$ [ 20 -le 25 ] && [ ] tdx96@ubuntu:~/datas$ echo $? 1
Process control
1.if judgment
example
tdx96@ubuntu:~/datas$ touch if.sh tdx96@ubuntu:~/datas$ vim if.sh
#!/bin/bash if [ $1 -eq 1 ] then echo "banzhang zhen shuai" elif [ $1 -eq 2 ] then echo "cls zhen mei" fi
tdx96@ubuntu:~/datas$ bash if.sh 1 banzhang zhen shuai tdx96@ubuntu:~/datas$ bash if.sh 2 cls zhen mei
$1 represents the first parameter entered
2.case statement
example
tdx96@ubuntu:~/datas$ touch case.sh tdx96@ubuntu:~/datas$ vim case.sh
#!/bin/bash case $1 in 1) echo "banzhang" ;; 2) echo "cls" ;; *) echo "renyao" ;; esac
tdx96@ubuntu:~/datas$ sh case.sh 1 banzhang tdx96@ubuntu:~/datas$ sh case.sh 2 cls tdx96@ubuntu:~/datas$ sh case.sh 3 renyao
3.for loop
Basic grammar 1
example
tdx96@ubuntu:~/datas$ touch for.sh tdx96@ubuntu:~/datas$ vim for.sh
#!/bin/bash s=0 for((i=1;i<=100;i++)) do s=$[$s+$i] done echo $s
tdx96@ubuntu:~/datas$ bash for.sh 5050
Basic grammar 2
example
tdx96@ubuntu:~/datas$ touch for2.sh tdx96@ubuntu:~/datas$ vim for2.sh
Writing 1:
#!/bin/bash for i in $* do echo "banzhang love $i" done
tdx96@ubuntu:~/datas$ bash for2.sh mm banzhang love mm tdx96@ubuntu:~/datas$ bash for2.sh cls mm xiaoze banzhang love cls banzhang love mm banzhang love xiaoze
Writing 2:
#!/bin/bash for j in $@ do echo "banzhang love $j" done
tdx96@ubuntu:~/datas$ bash for2.sh cls mm xiaoze banzhang love cls banzhang love mm banzhang love xiaoze
It's no different from writing 1
Writing 3:
Enclose $* $@ with ""
$* becomes a whole
$@ or individual
#!/bin/bash for i in "$*" do echo "banzhang love $i" done for j in "$@" do echo "banzhang love $j" done
tdx96@ubuntu:~/datas$ bash for2.sh cls mm xiaoze banzhang love cls mm xiaoze banzhang love cls banzhang love mm banzhang love xiaoze
4.while loop
1. Basic grammar
2. Examples
while loop: from 1 to 100
tdx96@ubuntu:~/datas$ touch while.sh tdx96@ubuntu:~/datas$ vim while.sh
#!/bin/bash s=0 i=1 while [ $i -le 100 ] do s=$[$s+$i] i=$[$i+1] done echo $s
tdx96@ubuntu:~/datas$ bash while.sh 5050
Read read console input
1. Basic grammar
2. Examples
#!/bin/bash read -t 7 -p "Enter the name you love" NAME echo $NAME ~
tdx96@ubuntu:~/datas$ bash read.sh Enter the name you loveheihei heihei
Functions: system functions
1.basename basic syntax
2.basename instance
Extract banzhang Txt name and name without suffix
tdx96@ubuntu:~/datas$ basename /home/tdx96/datas/banzhang.txt banzhang.txt tdx96@ubuntu:~/datas$ basename /home/tdx96/datas/banzhang.txt .txt banzhang
3.dirname basic syntax
4.dirname instance
Set banzhang Txt path extraction
tdx96@ubuntu:~/datas$ dirname /home/tdx96/datas/banzhang.txt /home/tdx96/datas
Functions: custom functions
1. Basic grammar
Experience and skills:
2. Examples
tdx96@ubuntu:~/datas$ touch fun.sh tdx96@ubuntu:~/datas$ vim fun.sh #!/bin/bash function sum() { s=0 s=$[$1+$2] echo $s } read -p "input your paratermer1:" p1 read -p "input your paratermer2:" p2 sum $p1 $p2 ~
tdx96@ubuntu:~/datas$ bash fun.sh input your paratermer1:5 input your paratermer2:12 17
Shell common tools
1.cut
Basic grammar
Option parameter description 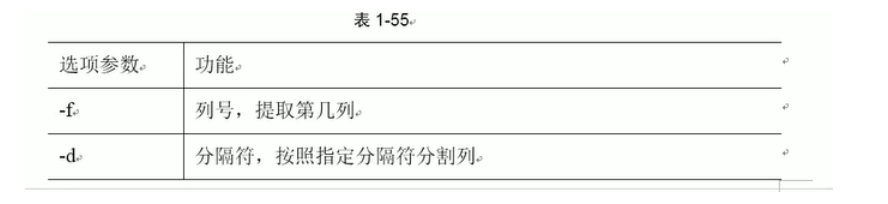
example:
Take out the first column of data with a space as a separator
tdx96@ubuntu:~/datas$ touch cut.txt tdx96@ubuntu:~/datas$ vim cut.txt
dong shen guan zhen wo wo lai lai le le
tdx96@ubuntu:~/datas$ cut -d " " -f 1 cut.txt dong guan wo lai le
Get the second column, the third column
tdx96@ubuntu:~/datas$ cut -d " " -f 2,3 cut.txt shen zhen wo lai le
Cut out guan separately
tdx96@ubuntu:~/datas$ cat cut.txt | grep guan |cut -d " " -f 1 guan
Select the system PATH variable value, and all paths after the beginning of the second ":
3 -: from 3 to last
tdx96@ubuntu:~/datas$ echo $PATH /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin tdx96@ubuntu:~/datas$ echo $PATH |cut -d : -f 3- /usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin
IP address printed after cutting ifconfig
tdx96@ubuntu:~/datas$ ifconfig ens33: flags=4163<UP,BROADCAST,RUNNING,MULTICAST> mtu 1500 inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255 inet6 fe80::680e:366e:9846:fc9d prefixlen 64 scopeid 0x20<link> ether 00:0c:29:e5:c9:60 txqueuelen 1000 (Ethernet) RX packets 24170 bytes 5840656 (5.8 MB) RX errors 0 dropped 0 overruns 0 frame 0 TX packets 27355 bytes 3135983 (3.1 MB) TX errors 0 dropped 0 overruns 0 carrier 0 collisions 0 lo: flags=73<UP,LOOPBACK,RUNNING> mtu 65536 inet 127.0.0.1 netmask 255.0.0.0 inet6 ::1 prefixlen 128 scopeid 0x10<host> loop txqueuelen 1000 (Local loopback) RX packets 1277 bytes 118508 (118.5 KB) RX errors 0 dropped 0 overruns 0 frame 0 TX packets 1277 bytes 118508 (118.5 KB) TX errors 0 dropped 0 overruns 0
tdx96@ubuntu:~/datas$ ifconfig ens33 ens33: flags=4163<UP,BROADCAST,RUNNING,MULTICAST> mtu 1500 inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255 inet6 fe80::680e:366e:9846:fc9d prefixlen 64 scopeid 0x20<link> ether 00:0c:29:e5:c9:60 txqueuelen 1000 (Ethernet) RX packets 24649 bytes 5888483 (5.8 MB) RX errors 0 dropped 0 overruns 0 frame 0 TX packets 27987 bytes 3207709 (3.2 MB) TX errors 0 dropped 0 overruns 0 carrier 0 collisions 0
tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "inet" inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255 inet6 fe80::680e:366e:9846:fc9d prefixlen 64 scopeid 0x20<link>
tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "inet" |grep broadcast inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255
tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "inet" |grep broadcast|cut -d "n" -f 2 et 192.168.1.161 tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "inet" |grep broadcast|cut -d "n" -f 2| cut -d " " -f 2 192.168.1.161
2.sed
1. Basic usage
2. Parameter description
3. Command function description
4. Examples
Insert the word meinv into the document and display it, but do not change the data of the original file
tdx96@ubuntu:~/datas$ touch sed.txt tdx96@ubuntu:~/datas$ vim sed.txt tdx96@ubuntu:~/datas$ cat sed.txt dong shen guan zhen wo wo lai lai le le tdx96@ubuntu:~/datas$ sed "2a mei nv" sed.txt dong shen guan zhen mei nv wo wo lai lai le le
Delete sed Txt file and display all lines containing wo
tdx96@ubuntu:~/datas$ sed "/wo/d" sed.txt dong shen guan zhen lai lai le le tdx96@ubuntu:~/datas$ cat sed.txt dong shen guan zhen wo wo lai lai le le
Replace wo in sed file with ni and display
g stands for global
tdx96@ubuntu:~/datas$ sed "s/wo/ni/g" sed.txt dong shen guan zhen ni ni lai lai le le tdx96@ubuntu:~/datas$ cat sed.txt dong shen guan zhen wo wo lai lai le le
Delete the second line in the sed file and replace wo with ni
tdx96@ubuntu:~/datas$ sed -e "2d" -e "s/wo/ni/g" sed.txt dong shen ni ni lai lai le le tdx96@ubuntu:~/datas$ cat sed.txt dong shen guan zhen wo wo lai lai le le
3.awk
1. Basic usage
2. Description of option parameters
3. Examples
Example 1:
1. Copy the passwd file to the current folder
tdx96@ubuntu:~/datas$ sudo cp /etc/passwd ./ [sudo] tdx96 Password for:
2. Modify file permissions
tdx96@ubuntu:~/datas$ sudo chown tdx96:tdx96 passwd
3. Find and print the seventh column
tdx96@ubuntu:~/datas$ awk -F : '/^root/ {print $7}' passwd /bin/bash
Example 2:
tdx96@ubuntu:~/datas$ awk -F : '/^root/ {print $1","$7}' passwd root,/bin/bash
Example 3:
tdx96@ubuntu:~/datas$ awk -F : 'BEGIN{print "user,shell"}{print $1","$7} END{print "dahaige,bin/zuishuai"}' passwd
Example 4:
tdx96@ubuntu:~/datas$ awk -F : -v i=1 '{print $3+$i}' passwd 0 1 2 3 4 5 ...
4. Built in variables of awk
5. Built in variable cases
tdx96@ubuntu:~/datas$ awk -F : '{print FILENAME"," NR"," NF}' passwd passwd,1,7 passwd,2,7 passwd,3,7 passwd,4,7 . . .
tdx96@ubuntu:~/datas$ ifconfig ens33 ens33: flags=4163<UP,BROADCAST,RUNNING,MULTICAST> mtu 1500 inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255 inet6 fe80::680e:366e:9846:fc9d prefixlen 64 scopeid 0x20<link> ether 00:0c:29:e5:c9:60 txqueuelen 1000 (Ethernet) RX packets 13827 bytes 2727675 (2.7 MB) RX errors 0 dropped 0 overruns 0 frame 0 TX packets 16804 bytes 1942372 (1.9 MB) TX errors 0 dropped 0 overruns 0 carrier 0 collisions 0 tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "inet" inet 192.168.1.161 netmask 255.255.255.0 broadcast 192.168.1.255 inet6 fe80::680e:366e:9846:fc9d prefixlen 64 scopeid 0x20<link>
tdx96@ubuntu:~/datas$ ifconfig ens33 |grep "netmask" | awk -F " " '{print $2}' 192.168.1.161
tdx96@ubuntu:~/datas$ awk '/^$/{print NR}' sed.txt 4 tdx96@ubuntu:~/datas$ cat sed.txt dong shen guan zhen wo wo lai lai le le
4.sort
1. Basic usage
2. Examples
tdx96@ubuntu:~/datas$ sort -t : -nrk2 sort.sh xz:50:2.3 bb:40:5.4 ss:30:1.6 bd:20:4.2 cls:10:3.5 #!/bin/bash tdx96@ubuntu:~/datas$ sort -t : -nk2 sort.sh #!/bin/bash cls:10:3.5 bd:20:4.2 ss:30:1.6 bb:40:5.4 xz:50:2.3
Interview questions