Simple Factory Mode
Basic Definition:
Simple factory mode, also known as static factory method, is a creation mode.In simple factory mode, instances of different classes can be returned depending on the parameters passed.The Simple Factory pattern defines a class specifically for creating instances of other classes that share a common parent.
Pattern structure:
1. Factory: Factory role.A factory dedicated to creating instance classes provides a method for returning specific instances of different classes depending on the parameters passed.
2. Product: Abstract product role.Is the parent of all products.
3. ConcreteProduct: Specific product roles.
Code implementation:
1. Product abstract product class
/** * @Description Product Abstract product class * @Author yanyuan * @Date 18:27 2020/4/14 * @Version 1.0 **/ public interface Phone { void make(); void call(); }
2. Concrete Product Specific Product Class
import lombok.extern.slf4j.Slf4j; /** * @Description Huawei Mobile Phone * @Author yanyuan * @Date 18:30 2020/4/14 * @Version 1.0 **/ @Slf4j public class HuaweiPhone implements Phone { @Override public void make() { log.info("Huawei Mobile Phone Production Completed"); } @Override public void call() { log.info("Huawei Mobile Phone Call"); } }
/** * @Description Concrete Product Specific product classes * @Author yanyuan * @Date 18:28 2020/4/14 * @Version 1.0 **/ @Slf4j public class IPhone implements Phone{ @Override public void make() { log.info("Make iPhone Mobile phone"); } @Override public void call() { log.info("iPhone Phone Call"); } }
3. Factory Factory Role Class
import lombok.extern.slf4j.Slf4j; /** * @Description Factory Factory Role Class * @Author yanyuan * @Date 22:06 2020/4/14 * @Version 1.0 **/ @Slf4j public class SimpleFactory { public Phone createPhone(String brand){ Phone phone = null; switch (brand){ case "HUAWEI": phone = new HuaweiPhone(); break; case "IPHONE": phone = new IPhone(); break; default: log.error("This brand of mobile phone is not supported yet"); break; } return phone; } }
4. Test Classes
/** * @Description Simple Factory Mode Test Class * @Author yanyuan * @Date 22:10 2020/4/14 * @Version 1.0 **/ public class Test { public static void main(String[] args) { SimpleFactory factory = new SimpleFactory(); Phone huaweiPhone = factory.createPhone("HUAWEI"); huaweiPhone.make(); huaweiPhone.call(); Phone iPhone = factory.createPhone("IPHONE"); iPhone.make(); iPhone.call(); } }
Output Results
22:16:19.325 [main] INFO com.yanyuan.gof.build.simpleFactory.v2.HuaweiPhone - Huawei Mobile Phone Completed
22:16:19.343 [main] INFO com.yanyuan.gof.build.simpleFactory.v2.HuaweiPhone - Huawei Phone makes phone calls for mobile phones
22:16:19.344 [main] INFO com.yanyuan.gof.build.simpleFactory.v2.IPhone - Make an iPhone phone
22:16:19.344 [main] INFO com.yanyuan.gof.build.simpleFactory.v2.IPhone - Phone call
Advantages and disadvantages
Advantage
Separation of responsibilities is achieved, and specialized factory class creation objects are provided.
Users do not need to know the specific product name.
Configuration files allow you to change/add new products without modifying client (consumer) code, which improves the flexibility of the system in some programs.
shortcoming
Because factory classes are centralized, product creation logic can affect the entire system if it does not work properly.
Using the simple factory mode will increase the number of system classes and, to some extent, increase the complexity and understanding of the system.
System expansion is difficult, once new products are added, factory logic has to be modified. When there are many types of products, it may cause too complex factory logic, which is not conducive to system expansion and maintenance.
summary
The point of the simple factory model is that when you need something, you only need to know the correct parameters to get what you want without knowing the details of its creation.
The best advantage of simple factory mode is to create objects and separate them, but it can lead to complex factory codes when there are too many products.
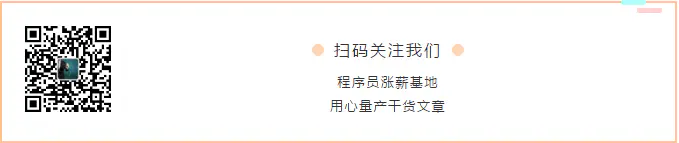