Getting to know spring
Two advantages of spring framework:
1.== Simplified development = = simplifies the complexity of enterprise development
2.== Framework inheritance = = effectively integrates other mainstream frameworks to improve operation and development efficiency
Core idea:
1. IOC (control reversal)
2. AOC (aspect oriented programming)
History of spring:
In 2004, Rod Johnson created a more efficient spring framework,
spring1.0: Programming in the form of full configuration
spring2.0: added annotation function to improve development efficiency
spring3.0: it has completely become pure annotation development
spring4.0: individual jdks have been adjusted according to the update of jdks
spring5.0: jdk8 is fully supported. If you want to use the spring framework at this time, you need to use jdk8 or above
Architecture diagram of spring framework:
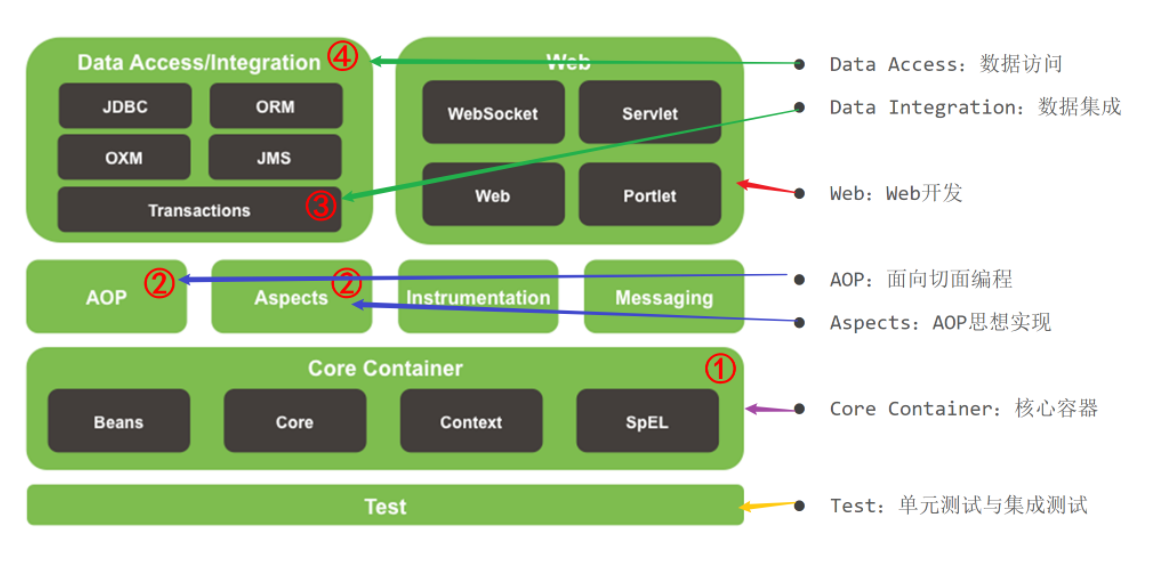
IOC and DI core ideas:
IOC(Inversion of Control)
The coupling between new objects will be very high. IOC transfers the right to create objects to the outside, that is, spring. The spring framework creates an IOC container outside, and the created objects are called beans and stored in the container When we need to use an object, the container provides us with a method, we can get the object and decouple it
DI(Dependency Injection)
Often, the execution of an object depends on the support of other objects. At this time, the spring framework will bind the dependent bean s stored in the IOC container
For the implementation of IOC and DI, in order to achieve sufficient decoupling, we can not only get bean s directly from the container, but also get all dependencies
Simple IOC control inversion using configuration file
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!--stay spring Configuration management in configuration file bean--> <!--Configuration data implementation layer bean--> <!-- id attribute: On behalf of the designated bean name,Get from container in bean When,You can get this with this name bean--> <!-- class attribute: appoint bean Type of,Here, the full class name is specified to the implementation class of the data implementation layer BookDaoImpl,Created on behalf of bean yes BookDaoImpl type--> <bean id="bookDao" class="com.itheima.dao.impl.BookDaoImpl"> </bean> <!--Configure business implementation layer bean--> <!-- id attribute: On behalf of the designated bean name,Get from container in bean When,You can get this with this name bean--> <!-- class attribute: appoint bean Type of,Here, the full class name is specified to the implementation class of the data implementation layer BookServiceImpl,Created on behalf of bean yes BookServiceImpl type--> <bean id="bookService" class="com.itheima.service.impl.BookServiceImpl"> <!--The business implementation layer object depends on the data implementation layer object,therefore service Layer dependence dao layer,use DI Dependency injection--> <!--name attribute:Which specific attribute represents the configuration--> <!--ref attribute: Which one does it represent bean,What we rely on here is dao Layered bean--> <property name="bookDao" ref="bookDao"/> </bean> </beans>
There are three implementations of instantiated bean s:
Construction method:
<bean id="bookDao" class="com.itheima.dao.impl.BookDaoImpl">
Static / non static factory method: (some older programs create object factories for decoupling)
Upgrading method of example chemical plant:
The lifecycle of a bean
1. Use attributes:
Init method = "initialization method" destroy method = "method executed before destruction"
2. Use interface
InitializingBean, DisposableBean
Implement these two classes and override methods
bean destruction time:
The bean destruction method is executed only when the container is destroyed,
1. Manually close the container:
close() method of ConfigurableApplicationContext interface
2. Register the close hook (close the container before closing the virtual machine)
registerShutdownHook() method of ConfigurableApplicationContext interface
Simple implementation of DI
setter injection:
Use the < property > tag
<property name="bookDao" ref="bookDao"/>
The name attribute refers to the set method, and the ref attribute refers to the bean object in the corresponding container
Constructor injection:
Make the constructor Arg > tag
<constructor-arg name="bookDao" ref="bookDao"/>
The name attribute refers to the parameter name in the constructor, and the ref attribute refers to the bean object in the corresponding container
Injection basic data type:
Replace the < var > tag with < value >
Automatic assembly:
Concept:
The IOC container automatically finds the corresponding bean in the container for assembly injection according to the dependency required by the bean
Use the autowire property keyword:
autowire="byType" automatically assemble according to the type (it is recommended to ensure the uniqueness of the bean)
autowire="byName" assemble by bean name (not recommended)
Set injection:
<!--Inject array data--> <property name="array"> <array> <value>100</value> <value>200</value> </array> </property> <!--injection list Aggregate data--> <property name="list"> <list> <value>aa</value> <value>bb</value> </list> </property> <!--injection set Aggregate data(Duplicate data will be deleted automatically)--> <property name="set"> <set> <value>aa</value> <value>aa</value><!--Will be filtered out--> </set> </property> <!--injection map Aggregate data--> <property name="map"> <map> <entry key="Key 1" value="Value 1"/> <entry key="Key 2" value="Value 2"/> </map> </property> <property name="properties"> <props> <prop key="country">china</prop> <prop key="province">henan</prop> <prop key="city">kaifeng</prop> </props> </property>
The property tag indicates setter injection, and the construction mode is injected into the constructor Arg tag. The < array >, < list >, < set >, < Map >, < props > tags can also be written inside