Previous remarks
For PHP, there are many template engines to choose from, but Smart is a PHP-based template engine, which is the most famous and powerful in the industry. Smart, like PHP, has a rich library of functions. It can be used directly from counting words to automatic indentation, text wrapping and regular expressions. If it is not enough, SMARTY also has a strong expansion capability, which can be expanded by plug-in. In addition, Smart is also a kind of free software, users can freely use, modify, and redistribute the software. This article will introduce the Smart Template Engine in detail.
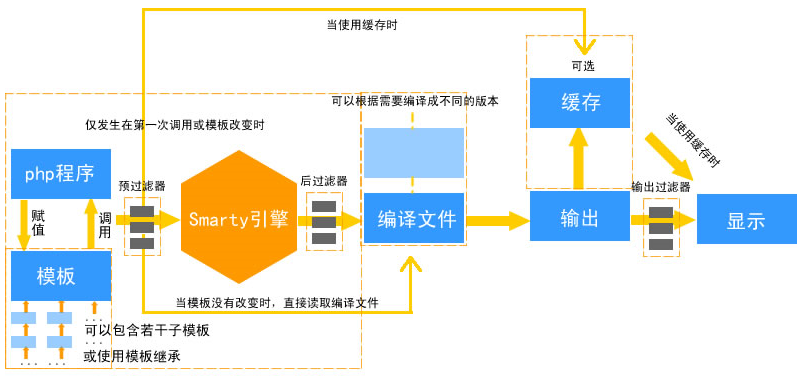
Summary
Smart is a php template engine. More precisely, it separates the logical program from the external content and provides an easy-to-manage method. Smart's overall design philosophy is to separate business logic from performance logic. Its advantages are summarized as follows:
Speed - Comparing with other template engine technologies, programs written in Smart can achieve maximum speed improvement
Compiler - Smarty programs are compiled into a non-template PHP file at runtime. This file uses a hybrid approach of PHP and HTML, and converts Web requests directly to this file the next time you access the template, without template recompilation (without changing the source program). Using subsequent calls is faster
Caching technology - Smart provides an optional caching technology that caches the HTML files that the user eventually sees into a static HTML page. When the user opens the Smart cache, and within a set time, the user's Web request is directly converted to the static HTML file, which is equivalent to calling a static HTML file.
Plug-in technology - Smart template engine is implemented by PHP object-oriented technology. It can not only be modified in the original code, but also customize some function plug-ins (functions customized according to rules).
Powerful presentation logic, which can process data through conditional judgment and iteration in Smart template, is actually a programming language, but the grammar is simple, and designers can learn it quickly without requiring programming knowledge.
Template Inheritance - Template Inheritance is a new thing in Smarty3. In template inheritance, you can manipulate content blocks to inherit templates as separate pages without loading other pages. This makes templates more intuitive, effective and manageable
Of course, there are also places where Smarty is not suitable for use. For example, content that needs to be updated in real time and templates need to be recompiled frequently, so using Smart in this type of program can slow down template processing. In addition, Smarty templates are not suitable for small projects, because small projects are simple and have front-end and back-end projects, Smarty will lose the advantages of rapid PHP development to a certain extent.
To configure
[Installation]
Installation of Smart is simple, to Smart official website Download the latest stable version, and then decompress the package. In the decompressed directory, you can see a Smart class library directory named libs. Just copy the LIBS folder directly to the main folder of the program.
[Note] Smart requires the web server to run versions above PHP 4.0
The libs folder contains the following six files
Smarty.class.php(Main document) SmartyBC.class.php(Compatible with other versions Smarty) sysplugins/* (System Function Plug-in) plugins/* (Custom function plug-in) Autoloader.php debug.tpl
[Instance]
/* And specify the location of Smarty.class.php, note that'S'is capitalized*/ require './libs/Smarty.class.php'; /* Object $smarty that instantiates the Smart class */ $smarty = new Smarty();
[init]
Smart requires four directories, named tempalates, templates_c, configs, and cache by default. Each is customizable and can modify Smart class attributes or related methods separately: $template_dir, $compile_dir, $config_dir and $cache_dir
/** file: init.inc.php Smarty Object Instance and Initial File */ define("ROOT", str_replace("\\", "/",dirname(__FILE__)).'/'); //Specify the root path of the project require ROOT.'libs/Smarty.class.php'; //Load Smarty Class file $smarty = new Smarty(); //instantiation Smarty Objects of classes $smarty /* It is recommended that the default path be set in Smarty version 3 or above, and that the $smarty object itself be returned after success, which can be operated coherently. */ $smarty ->setTemplateDir(ROOT.'templates/') //Set up directories for all template files // -> Add Template Dir (ROOT.'templates 2/')// / You can add multiple template directories ->setCompileDir(ROOT.'templates_c/') //Set up directories for all compiled template files ->addPluginsDir(ROOT.'plugins/') //Add toDirectory for Template Extension Plug-ins ->setCacheDir(ROOT.'cache/') //Setting the directory where cached files are stored ->setConfigDir(ROOT.'configs'); //Setting the directory where template configuration files are stored $smarty->caching = false; //Set up Smarty Cache switch function $smarty->cache_lifetime = 60*60*24; //Set the effective time period of template cache to 1 day $smarty->left_delimiter = '<{'; //Setting the left terminator in the template language $smarty->right_delimiter = '}>'; //Setting the right terminator in the template language
[demo]
<!-- main.tpl -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<{$content}>
</body>
</html>
<?php require './init.inc.php'; $smarty -> assign('content','this is content.....'); $smarty -> display('main.tpl'); ?>

Basic Grammar
[Notes]
Template comments are surrounded by * asterisks, and asterisks on both sides are surrounded by delimiters. Annotations exist only in templates and are not visible on the output page
<{* this is a comment *} >
[Variables]
Template variables start with the dollar sign $and can contain numbers, letters, and underscores, much like php variables. You can refer to numeric or non-numeric indexes of arrays, as well as object attributes and methods.
A configuration file variable is a variable that does not use the dollar sign $, but surrounds it with a # sign (# hashmarks #), or is a variable in the form of $smarty.config
[Note] Smart recognizes variables embedded in double quotes
Mathematics and Embedded Labels {$x+$y} // output x+y And {assign var=foo value=$x+$y} // Variables in attributes {$foo[$x+3]} // Variables are indexed as arrays {$foo={counter}+3} // Nested labels in labels {$foo="this is message {counter}"} // Use labels in quotation marks //Define arrays {assign var=foo value=[1,2,3]} {assign var=foo value=['y'=>'yellow','b'=>'blue']} {assign var=foo value=[1,[9,8],3]} // Can be nested //Short variable allocation {$foo=$bar+2} {$foo = strlen($bar)} {$foo = myfunct( ($x+$y)*3 )} // As a function parameter {$foo.bar=1} // Assign a value to the specified array index {$foo.bar.baz=1} {$foo[]=1} //Point grammar {$foo.a.b.c} => $foo['a']['b']['c'] {$foo.a.$b.c} => $foo['a'][$b]['c'] {$foo.a.{$b+4}.c} => $foo['a'][$b+4]['c'] {$foo.a.{$b.c}} => $foo['a'][$b['c']] PHP grammar {$foo[1]} {$foo['bar']} {$foo['bar'][1]} {$foo[$x+$x]} {$foo[$bar[1]]} {$foo[section_name]}
<!-- main.tpl --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <{***Define arrays:10***}> <{assign var=foo value=[10,20,30]}> <{$foo[0]}><br> <{***Short variable allocation:0***}> <{$foo=0}> <{$foo}><br> <{***Point grammar:1***}> <{$test.a}><br> <{***PHP grammar:1***}> <{$test['a']}><br> <{***Mathematical operations:3***}> <{$test.a+$test.b}><br> </body> </html>
<?php require './init.inc.php'; $smarty -> assign('test',['a'=>1,'b'=>2]); $smarty -> display('main.tpl'); ?>
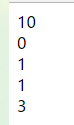
[Function]
Each smarty tag outputs a variable or calls a function. Within the delimiter, functions and their attributes will be processed and output
<{funcname attr1="val" attr2="val"}>
{config_load file="colors.conf"} {include file="header.tpl"} {if $highlight_name} Welcome, <div style="color:{#fontColor#}">{$name}!</div> {else} Welcome, {$name}! {/if} {include file="footer.tpl"}
[Attributes]
Most functions have their own attributes to specify or modify their behavior, and smarty functions have attributes similar to those in HTML. Quotation marks are not required for static values, but quotation marks are recommended for strings. Ordinary smarty variables can be used, or variables with adjusters can be used as attribute values, without quotation marks. You can even use php function return values and complex expressions as attribute values
Some properties use Boolean values (true or false), which indicate true or false. If no Boolean values are assigned to these attributes, then true is used as the default value.
{include file="header.tpl"} {include file="header.tpl" nocache} // Be equal to nocache=true {include file="header.tpl" attrib_name="attrib value"} {include file=$includeFile} {include file=#includeFile# title="My Title"} {assign var=foo value={counter}} {assign var=foo value=substr($bar,2,5)} {assign var=foo value=$bar|strlen} {assign var=foo value=$buh+$bar|strlen} {html_select_date display_days=true} {mailto address="smarty@example.com"} <select name="company_id"> {html_options options=$companies selected=$company_id} </select>
variable
Smart has several different types of variables, and the type of variable depends on what prefix symbol it is (or is surrounded by). Smart variables can be output directly or as parameters of function attributes and modifiers, or used for internal conditional expressions, etc. If you want to output a variable, just enclose it with a delimiter
1. Variables allocated from PHP
index.php: $smarty = new Smarty; $smarty->assign('Contacts',array('fax' => '555-222-9876','email' => 'zaphod@slartibartfast.com')); $smarty->display('index.tpl'); index.tpl: <{$Contacts.fax}><br> <{$Contacts.email}><br> OUTPUT: 555-222-9876<br> zaphod@slartibartfast.com<br>
2. Variables read from configuration files
After loading the configuration file, the variables in the configuration file need to be surrounded by two well numbers "#" or smarty's reserved variable $smarty.config.
foo.conf: pageTitle = "This is mine" tableBgColor = "#bbbbbb" rowBgColor = "#cccccc" <!-- main.tpl --> <{config_load file='foo.conf'}> <!DOCTYPE> <html> <title><{#pageTitle#}></title> <body> <table style="background:<{#tableBgColor#}>"> <tr style="background:<{#rowBgColor#}>"> <td>First</td> <td>Last</td> <td>Address</td> </tr> </table> </body> </html>
<?php require './init.inc.php'; $smarty -> display('main.tpl'); ?>
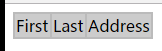
3. Variables of the partition in the configuration file
foo.conf: [a] x=1 [b] x=2 [c] x=3 <!-- main.tpl --> <{config_load file='foo.conf' section="a"}> <{#x#}> output: 1
4. Variables defined in the template
<{$name='Bob'}> The value of $name is <{$name}>. output: The value of $name is Bob.
5. Reserving variables
$smarty.get $smarty.post $smarty.cookies $smarty.server $smarty.env $smarty.session $smarty.request $smarty.now //Current timestamp $smarty.const //Visit php constant $smarty.capture //Capture built-in{capture}...{/capture}Template output $smarty.config //Gets the configuration variable.{$smarty.config.foo}yes{#foo#} Synonyms $smarty.section //point{section}Attributes of loops $smarty.template //Returns the current template name processed $smarty.current_dir //Returns the name of the current template directory processed $smarty.version //Returns compiled Smarty Template version number
<!-- main.tpl --> <{$smarty.now}><br> <{date('Y-m-d',$smarty.now)}><br> <{$smarty.template }><br> <{$smarty.current_dir }><br> <{$smarty.version }><br>
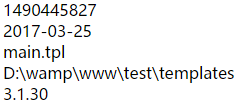
Variable regulator
Variable regulators act on variables, custom functions, or strings. The use of variable regulator is:'|'sign right to the name of the regulator. Variable regulator can receive additional parameters to influence its behavior. The parameters are located on the right side of the regulator and are separated by the':'symbol.
[Note] Multiple modifiers can be used for the same variable. They will be combined from left to right in a set order. The "|" character must be used as a separator between them.
capitalize [capital first character]
Capitalize all words in a variable, similar to the ucwords() function of php. The default parameter, false, is used to determine whether words with numbers need to be capitalized.
<{$articleTitle="next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|capitalize}><br> <{$articleTitle|capitalize:true}> <br> output: next x-men film, x3, delayed. Next X-Men Film, x3, Delayed. Next X-Men Film, X3, Delayed.
lower [lowercase]
The variable string is lowercase, which acts as the strtolower() function of php
<{$articleTitle="Next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|lower}><br> output: Next x-men film, x3, delayed. next x-men film, x3, delayed.
upper [capitalization]
Change variables to uppercase, equivalent to php's strtoupper() function
<{$articleTitle="Next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|upper}><br> output: Next x-men film, x3, delayed. NEXT X-MEN FILM, X3, DELAYED.
cat [connection string]
Connect the value in cat to the given variable
<{$articleTitle="next x-men film, x3, delayed."}> <{$articleTitle|cat:" yesterday."}> OUTPUT: next x-men film, x3, delayed. yesterday.
count_characters [character count]
Calculate the number of characters in a variable. The default parameter is false, which is used to determine whether a space character is calculated or not.
<{$articleTitle="next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|count_characters}><br> <{$articleTitle|count_characters:true}><br> OUTPUT: next x-men film, x3, delayed. 25 29
count_paragraphs [Number of calculated segments]
Calculate the number of paragraphs in a variable
<{$articleTitle="next x-men\n film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|count_paragraphs}><br> OUTPUT: next x-men film, x3, delayed. 2
count_sentences [counting sentences]
Calculate the number of sentences in a variable
<{$articleTitle="next x-men. film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|count_sentences}><br> OUTPUT: next x-men. film, x3, delayed. 2
count_words [counts words]
Calculate the number of words in a variable
<{$articleTitle="next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|count_words}><br> OUTPUT: next x-men film, x3, delayed. 5
date_format [format date]
% a - Abbreviation of the current regional day of the week % A - Full name of the current regional week % b - Abbreviation of current regional month % B - Full name of current regional month % c - The preferred date and time expression for the current region % C - Century Value (Year divided by 100, ranging from 00 to 99) % d - On the day of the month, decimal digits (ranging from 01 to 31) % D - is the same as% m/%d/%y % On the day of the e-month, decimal digits and one-digit digits are preceded by a space (ranging from'1'to'31'). % G - Same as% G, but no Century % G - 4 digit years, in line with ISO weeks (see% V). The format and value of% V are the same except for the year in which ISO weeks belong to the previous year or the following year. % h - Same as% b % Decimal Hours in H - 24 Hours (ranging from 00 to 23) % Decimal Hours in I - 12 Hours (ranging from 00 to 12) % On the day of the j - year, decimal numbers (ranging from 001 to 366) % m - decimal months (ranging from 01 to 12) % M-decimal minutes % n - newline character % p - Based on a given time value of `am'or `pm', or the corresponding string in the current locale % r - Time with a.m. and p.m. symbols % R - 24 hour symbol time % S-decimal seconds % t - tab % T - Current time, same as% H:%M:%S % u - The decimal number of the day of the week represents [1,7], and 1 represents Monday. % U - Weeks of the year, starting with the first Sunday of the first week as the first day % V - ISO 8601:1988 format for the first few weeks of the year, ranging from 01 to 53. The first week is the first week of the year with at least four days, and Monday is the first day of the week. (Composition of years with% G or% g as the corresponding number of weeks for the specified timestamp.) % W - Weeks of the year, starting with the first Monday of the first week as the first day % w - The day of the week, Sunday is 0 % x - preferred time representation for the current region, excluding time % X - The preferred time representation for the current region, excluding dates % y - decimal years without centuries (ranging from 00 to 99) % Y - decimal years including centuries % z or% z - Time Zone Name or Abbreviation %%- The `%'character on the text
<{$smarty.now|date_format}><br> <{$smarty.now|date_format:"%D"}><br> output: Mar 25, 2017 03/25/17
Default [default value]
Set a default value for the variable. When a variable is not set or empty, its output is replaced by a given default value
<{$articleTitle|default:'a'}><br> <{$articleTitle="next x-men film, x3, delayed."}> <{$articleTitle|default:'a'}><br> output: a next x-men film, x3, delayed.
Escape [escape]
escape acts on variables for transcoding or escaping html, url, single quotation marks, hexadecimal, hexadecimal entities, javascript, mail. The first parameter defaults to'html', and the optional parameters are'html,htmlall,url,quotes,hex,hexentity,javascript'; the second parameter defaults to'utf-8', and the optional parameters are'ISO-8859-1,UTF-8'.
<{$articleTitle="'Stiff Opposition Expected to Casketless Funeral Plan'"}> <{$articleTitle|escape}><br> <{$articleTitle|escape:'url'}> output: 'Stiff Opposition Expected to Casketless Funeral Plan' %27Stiff%20Opposition%20Expected%20to%20Casketless%20Funeral%20Plan%27
Indent [indent]
In each line of indented string, the default is 4 characters. For the first optional parameter, you can specify the number of indented characters, and for the second optional parameter, you can specify what character indentation to use, such as't'as a tab.
<{$articleTitle="'Stiff Opposition Expected to Casketless Funeral Plan'"}> <{$articleTitle}><br> <{$articleTitle|indent}> output: 'Stiff Opposition Expected to Casketless Funeral Plan' 'Stiff Opposition Expected to Casketless Funeral Plan'
nl2br [line break replaced by < br />]
All newline characters will be replaced with < br />, functioning the same as the nl2br() function in PHP
<{$articleTitle="Next x-men\nfilm, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|nl2br}><br> output: Next x-men film, x3, delayed. Next x-men film, x3, delayed.
regex_replace [regular replacement]
Search and replace variables with regular expressions, and the grammar comes from the preg_replace() function of php
<{$articleTitle="Next x-men\nfilm, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|regex_replace:"/[\r\t\n]/":" "}><br> output: Next x-men film, x3, delayed. Next x-men film, x3, delayed.
Replace [replace]
A simple search and substitution of strings in variables. str_replace() function equivalent to php
<{$articleTitle="Next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|replace:"x":"y"}><br> output: Next x-men film, x3, delayed. Neyt y-men film, y3, delayed.
spacify [interpolation]
Interpolation is a method of inserting spaces or other characters (strings) between each character of a variable's string.
<{$articleTitle="Next x-men film, x3, delayed."}> <{$articleTitle}><br> <{$articleTitle|spacify}><br> <{$articleTitle|spacify:"^"}><br> output: Next x-men film, x3, delayed. N e x t x - m e n f i l m , x 3 , d e l a y e d . N^e^x^t^ ^x^-^m^e^n^ ^f^i^l^m^,^ ^x^3^,^ ^d^e^l^a^y^e^d^.
string_format [string formatting]
A method of formatting strings, such as decimal numbers, etc. The actual application is the sprintf() function of php
<{$number=23.5678}> <{$number}><br> <{$number|string_format:"%.2f"}><br> <{$number|string_format:"%d"}> output: 23.5678 23.57 23
strip [Remove (Redundant Spaces)]
Replace all duplicate spaces, newlines, and tabs with a space or a given character
<{$articleTitle="Grandmother of\neight makes\t hole in one."}> <{$articleTitle}><br> <{$articleTitle|strip}><br> <{$articleTitle|strip:' '}><br> output: Grandmother of eight makes hole in one. Grandmother of eight makes hole in one. Grandmother of eight makes hole in one.
strip_tags [remove html tags]
Remove < and > tags, including all content between < and >
<{$articleTitle="Blind Woman Gets New Kidney from Dad she Hasn't Seen in <b>years</b>."}> <{$articleTitle}><br> <{$articleTitle|strip_tags}><br> output: Blind Woman Gets New Kidney from Dad she Hasn't Seen in <b>years</b>.<br> Blind Woman Gets New Kidney from Dad she Hasn't Seen in years .<br>
truncate [intercept]
To intercept a length of character from the beginning of the string, the default is 80. You can also specify the second parameter as the text string appended after the intercepted string. The additional string is calculated in the interception length. By default, smarty intercepts the end of a word. If you want to intercept exactly how many characters, change the third parameter to "true" and the fourth parameter to FALSE by default, which means that it will be intercepted to the end of the string and TRUE to the middle. Note that if TRUE is set, character boundaries are ignored
<{$articleTitle='Two Sisters Reunite after Eighteen Years at Checkout Counter.'}> <{$articleTitle}><br> <{$articleTitle|truncate}><br> <{$articleTitle|truncate:30}><br> <{$articleTitle|truncate:30:""}><br> <{$articleTitle|truncate:30:"---"}><br> <{$articleTitle|truncate:30:"":true}><br> <{$articleTitle|truncate:30:"...":true}><br> <{$articleTitle|truncate:30:'..':true:true}><br> output: Two Sisters Reunite after Eighteen Years at Checkout Counter. Two Sisters Reunite after Eighteen Years at Checkout Counter. Two Sisters Reunite after... Two Sisters Reunite after Two Sisters Reunite after--- Two Sisters Reunite after Eigh Two Sisters Reunite after E... Two Sisters Re..ckout Counter.
wordwrap [line width constraint]
You can specify the column width of a paragraph (that is, how many characters in a row, more than the number of characters in a newline), by default of 80. The second parameter is optional, specifying what newline character to use at the constraint point, defaulting to " n". By default, smarty will be truncated to the end of the word. If you want to be precise enough to set the length of the character, set the third parameter to ture. This regulator is equivalent to the wordwrap() function of php
<{$articleTitle="Blind woman gets new kidney from dad she hasn't seen in years."}> <{$articleTitle}><br> <{$articleTitle|wordwrap:30}><br> <{$articleTitle|wordwrap:20}><br> <{$articleTitle|wordwrap:30:"<br />\n"}><br> <{$articleTitle|wordwrap:26:"\n":true}><br> output: Blind woman gets new kidney from dad she hasn't seen in years.<br> Blind woman gets new kidney from dad she hasn't seen in years.<br> Blind woman gets new kidney from dad she hasn't seen in years.<br> Blind woman gets new kidney<br /> from dad she hasn't seen in<br /> years.<br> Blind woman gets new kidney from dad she hasn't seen in years.<br>