Software testing handout
Edit java files using Notepad
1. Configure java path
1.1 select the computer icon / right-click to select the properties of the drop-down menu/
1.2 select the advanced system Settings link
1.3 select the environment variable button in the system properties window
1.4 select the "classpath" path in the system variable and add the symbol in English as,;
Note: if there is no "CLASSPAT" in the system variable, click new and add "name of the variable" and "value"
2. System command generation class files and accessing files in java
CMD > javac java file name Suffix / / edit the java file to generate a Java class file
CMD > java file name. / / the output content in Java is displayed
3. Solve the problem of garbled code
Text file name txt "changed to" java file name java"
Files containing java code in Notepad: first, "save as" another file name; Then, the suffix of notepad file is "file name. Java"; Finally, change the text code to ANSI and click OK
4. java basic coding
4.1 calculate Fahrenheit temperature
package test_softwareTEst; class Test2{ public static void main(String[] args){ //dh is the temperature in Celsius and ds is the temperature in Fahrenheit double dh = 0; double ds = 0; System.out.println("Please enter the value of Fahrenheit!"); java.util.Scanner input = new java.util.Scanner(System.in); dh = input.nextDouble(); System.out.println("The value converted to Celsius is:"+dh); ds =(dh-32)*(5.0/9);//5 here is double type, and 5.0 is entered!! System.out.println("The value converted to Celsius is:"+ds); } }
4.2 multiple judgment statements
package test_softwareTEst; class Test3 { public static void main(String[] args) { int cls = 0; String sex = " ";//Syntax: text assignment format,, cannot be null!! java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter any number 1 and 2 for the class,3:"); cls = input.nextInt(); System.out.println("Please enter the gender as female or male:"); sex = input.next(); if (cls == 1 && sex.equals("schoolboy")) {//Text judgment syntax!! System.out.println("Boys' mineral water"); } else if (cls == 2 && sex.equals("schoolboy")) { System.out.println("Boys Iced Black Tea"); } else if (cls == 1 && sex.equals("girl student")) { System.out.println("Girl farmer spring"); } else if (cls == 3 && sex.equals("schoolboy")) { System.out.println("Boy pulse"); } else if (cls == 3 && sex.equals("girl student")) { System.out.println("Girl asham milk tea"); } else if (cls == 2 && sex.equals("girl student")) { System.out.println("Kunlun mountain spring"); } else { System.out.println("No such class!"); } } }
4.3 triangle problem
package test_softwareTEst; class Test4 { public static void main(String[] args) { java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter a"); int a=input.nextInt(); //i is the number of rows for(int i =1;i<=a;i++){ for(int j =1;j<=i;j++){ System.out.print("*"); } System.out.println(); } } }
4.4 99 multiplication table
I. Law:
package test_softwareTEst; class Test4 { public static void main(String[] args) { java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter a"); int a=input.nextInt(); //i is the number of rows for(int i =1;i<=a;i++){ for(int j =1;j<=i;j++){ System.out.print("*"); } System.out.println(); } } }
Method 2:
package test_softwareTEst; class Test5 { public static void main(String[] args) { int sum =0; //i is the number of rows for(int i =1;i<=9;i++){ for(int j =1;j<=i;j++){//j=2 is the number of printed lines 2 System.out.print(i+"X"+j+"="+i*j+"\t"); } System.out.println(); } } }
4.5 function call
Create a table
Method 1:
package test_softwareTEst; public class Test8 { static int tiji(int a,int b,int c) { int area = a*b*c; return area; } public static void main(String[] args) { System.err.print("The area of a rectangle is"+tiji(3,2,5)); } }
Method 2:
public class Test8 { static int tiji(int a,int b,int c) { return a*b*c; } public static void main(String[] args) { System.err.print("The area of a rectangle is"+tiji(3,2,5)); } } if (cls == 1 && sex.equals("schoolboy")) {//Text judgment syntax!! System.out.println("Boys' mineral water"); } else if (cls == 2 && sex.equals("schoolboy")) { System.out.println("Boys Iced Black Tea"); } else if (cls == 1 && sex.equals("girl student")) { System.out.println("Girl farmer spring"); } else if (cls == 3 && sex.equals("schoolboy")) { System.out.println("Boy pulse"); } else if (cls == 3 && sex.equals("girl student")) { System.out.println("Girl asham milk tea"); } else if (cls == 2 && sex.equals("girl student")) { System.out.println("Kunlun mountain spring"); } else { System.out.println("No such class!"); }
4.7 object oriented
In the test class, instantiate the name The name of the method body; Instance alias Attribute name of noun (assigned here)
5. Flow chart of white box test
if condition override 1
5.1 java file coding
package test_softwareTEst; class Test11 { public static void main(String[] args) { java.util.Scanner input = new java.util.Scanner(System.in);//Line 1 System.out.println("Please enter a Score: ");//Line 2 int Score = input.nextInt();//Line 3 if (Score >=80) { //Line 4 System.out.println("excellent");//Line 5 } else if (Score>=60) {//Line 6 System.out.println("pass");//Line 7 } else { System.out.println("fail,");//Line 8 } } }
5.2 procedure flow chart
5.3. Control flow chart
be careful; There are three branches in the control flow chart, including the background color, excluding the color in the circle (three colors: small square, large square and the background color of the outermost layer)
if conditional coverage test case
Cycle coverage test case
for loop override 1
1.java file code
package test_softwareTEst; class Test10 { public static void main(String[] args) { java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter a"); int a=input.nextInt(); //i is the number of rows for(int m =1;m<=100;m++){ System.out.print("service"+m);//m is the number of services } } }
2. Procedure flow chart
4.for loop test case
for loop override 2
1.java code
package test_softwareTEst; class Multi symbol output { public static void main(String[] args) { java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter m"); int m=input.nextInt(); //i is the number of rows for(int i =1;i<=m;i++){ for(int j =1;j<=10;j++){//Do not participate in the flowchart System.out.print("*");//Do not participate in the flowchart }//Do not participate in the flowchart System.out.println();//Do not participate in the flowchart } } }
2. Procedure and control flow chart
Note: for the flow control diagram of circular statements here, just draw a circular line. Another for loop inside has nothing to do with another for loop, so some statements do not participate!!
for loop override 3
Number before range - number after range
Value: first, first + 1; Take the middle value of two numbers; Last number, last number + 1
1.java code
package test_softwareTEst; class Test12 { static void print() { java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Please enter m"); int m=input.nextInt();//0 System.out.println("Please enter n"); int n=input.nextInt();//1st //i is the number of rows for(int i =1;i<=m;i++){//2nd for(int j =1;j<=n;j++){//3rd System.out.print("*");//4th }// System.out.println();//6th } } public static void main(String[] args) { Test12 t12 = new Test12(); t12.print(); } }
Operation results:
2. Procedure and control flow chart
6. Black box test flow chart
Black box test "case"
Note: remove the head and tail numbers that meet the conditions
Effective equivalence class: the value range that meets the conditions
Invalid equivalence class: take the range that does not meet the conditions (beyond the range)
1. Equivalence class!
The test case is similar to the interval cannot be. The invalid equivalence class is the condition that is not satisfied, and the valid equivalence class is the condition that is satisfied (the range of variables)
Equivalence problem
Requirements for valid equivalence classes:
Micro signal: 2-10 digits, which can only be underlined later. It starts with the letter of USD $
Password: 6 ~ 16 digits, which must only start with a letter
Note: the conditions of valid equivalence classes must be disassembled one by one for the convenience of later testing!!
Test cases for equivalence classes:
Test case table (test each variable at least once if the number before and after each variable exceeds the boundary range)
2. Boundary value table
subject
Given score:0~750, write the boundary value table
Boundary value table
Test case!
Analysis: the boundary value table takes the number in front of the header, the header itself, and the header + 1; Take the total value / 2; The number before the mantissa, the mantissa itself, the mantissa + 1
6X + 1 = 6 + 7 (determined by the above seven analysis values) + 1 = 14
3. Decision table
Text definition
Topic 1
Requirements: 3 if the total score is higher than 450, the average score of each subject is higher than 75 or excellent graduates, they can be admitted directly. Otherwise to be determined
plan
1. Truth table judgment
(the latter step in the middle needs to be merged!!)
2. Final consolidation (- any value can satisfy the condition)
Note: the combination needs to be interpreted according to the admission and pending. If the admission of their columns and columns are checked, the combination can be carried out. Consolidation conditions: the types checked are the same; The first two numbers correspond to the same; If the last three items have the same number, they can also be combined. The values used here are 0 and 1
2. Topic 2!
plan
Note 1: Note: in conditions and situations, the values marked 0 and 1 need to be checked. Find the two conditions in the table below and judge the following conditions. Finally, judge the value of the third condition
1. Truth table
Note 2: in conditions and situations, the values marked 0 and 1 need to be checked. Find the two conditions in the table below and judge the following conditions. Finally, judge the value of the third condition
2. Final merger
3. Test case!
Symbol for text!
3. Title:
Score > 60, pass; If the score is 50 ~ 60 points, and the score is greater than 80 at ordinary times, it will pass, otherwise it will not pass; Score < 60, fail
1. Planning
2. Analysis
3. Merge the same type
4. Title
Title: score > 60, pass; Score 50 ~ 60 points, good performance and pass; Score < 60, fail
1. Planning
2. Analysis
3. Consolidation of the same type
5. Title
1. Planning
2. Analysis
3. Merge the same type
7. Use of dateuntil tool class
7.1DateUtil.java
package Test2; public class DateUtil { public String createDate(int year,int month,int day) { String str=""; // if(year <1950 || year >2100) { return "The year can only be 1950~2100 between"; } // if(month <1 || month >12) { return "The month can only be in January~12 between"; } // if(day <1 || day >31) { return "Days can only be in 1~31 between"; } // if( (month ==4 || month ==6 || month ==9 ||month ==11) && day >30) { return "A small month cannot exceed 30 days"; } if(month ==2) { if(year%4==0 && year%100!=0) { if(day>29) { return "February in leap year cannot exceed 29 days"; }else { return "February of a leap year cannot exceed 28 days"; } } } return year +"year" +month +"month" +day +"day"; } }
7.2 Test1.java / / single test
package Test2; public class Test1{ public static void main(String [] args){ DateUtil du = new DateUtil(); // Assertequals ("May 12, 2000", Du. CreateDate (May 12, 2000))// verification System.out.println(du.createDate(2000, 5, 12)); } }
7.3 test table (31 test cases in total)
3.1 equivalence test table!
3.2 boundary test table!
Note: normal - as expected, remove duplicate lines
3.3 other test tables
7.4DateUtil_TestCase.java / / multiple tests
package Test2; import Test2.DateUtil; import junit.framework.TestCase; public class DateUtil_TestCase extends TestCase { DateUtil du = new DateUtil(); /*********************1.Equivalence list test (7 items) start*********************************/ // Note: the verification methods of n methods are the same. Use junit tool class. After the test, the running result appears green, indicating that the value verified in the test of these n methods is public void test001() { //The character text is the same as the value of the expected result in the equivalence class table!! assertEquals("2000 May 12",du.createDate(2000, 5, 12));// verification } public void test002() { assertEquals("The year can only be 1950~2100 between",du.createDate(1949, 2, 2)); } public void test003() { assertEquals("The year can only be 1950~2100 between",du.createDate(2101, 11, 30)); } public void test004() { assertEquals("The month can only be in January~12 between",du.createDate(1990,-5,31)); } public void test005() { assertEquals("The month can only be in January~12 between",du.createDate(1999,0,5)); } public void test006() { assertEquals("Days can only be in 1~31 between",du.createDate(1998,6,-5)); } public void test007() { assertEquals("Days can only be in 1~31 between",du.createDate(1992,8,0)); } /*********************1.Equivalent class list test (7 items)*********************************/ /*********************2.Boundary value table test (Article 19) start*********************************/ public void test008() { //The character text is the same as the value of the expected result in the equivalence class table!! assertEquals("The year can only be 1950~2100 between",du.createDate(1949,6,16));// verification } public void test009() { assertEquals("1950 June 16",du.createDate(1950,6,16)); } public void test010() { assertEquals("1951 June 16",du.createDate(1951,6,16)); } public void test011() { assertEquals("1999 June 16",du.createDate(1999,6,16)); } public void test012() { assertEquals("2000 June 16",du.createDate(2000,6,16)); } public void test013() { assertEquals("2100 June 16",du.createDate(2100,6,16)); } public void test014() { assertEquals("The year can only be 1950~2100 between",du.createDate(2101,6,16)); } public void test015() { //The character text is the same as the value of the expected result in the equivalence class table!! assertEquals("The month can only be in January~12 between",du.createDate(1972,0,16));// verification } public void test016() { assertEquals("1972 January 16",du.createDate(1972,1,16)); } public void test017() { assertEquals("February of a leap year cannot exceed 28 days",du.createDate(1972,2,16)); } public void test018() { assertEquals("1972 November 16",du.createDate(1972,11,16)); } public void test019() { assertEquals("1972 December 16",du.createDate(1972,12,16)); } public void test020() { assertEquals("The month can only be in January~12 between",du.createDate(1972,13,0)); } public void test021() { assertEquals("1972 June 1",du.createDate(1972,6,1)); } public void test022() { assertEquals("1950 June 2",du.createDate(1950,6,2)); } public void test023() { assertEquals("1972 June 30",du.createDate(1972,6,30)); } public void test024() { assertEquals("1972 June 30",du.createDate(1972,6,30)); } public void test025() { assertEquals("A small month cannot exceed 30 days",du.createDate(1972,6,31)); } public void test026() { assertEquals("Days can only be in 1~31 between",du.createDate(1972,6,32)); } /*********************2.Equivalence list test (19 items)*********************************/ /*********************3.Boundary value table test (5 articles) start*********************************/ public void test027() { assertEquals("The year can only be 1950~2100 between",du.createDate(1949,4,31)); } public void test028() { assertEquals("The year can only be 1950~2100 between",du.createDate(1949,4,32)); } public void test029() { assertEquals("The year can only be 1950~2100 between",du.createDate(1949,9,33)); } public void test030() { assertEquals("2000 February 29",du.createDate(2000,2,29)); } public void test031() { assertEquals("2000 February 28",du.createDate(2000,2,28)); } /*********************3.Equivalent class list test (5 items)*********************************/ public static void main(String[] args) { junit.textui.TestRunner.run(DateUtil_TestCase.class);// Installation is required here. Import JUnit package } }
8. Database
Using database in cmd command
mysql -u root -p / / you only need a password to log in to the database
show databases; // Show all databases
create database database name// create database
Database name// use database
select database(); // query data base
Note: one Chinese character accounts for two characters; Table creation ends with a semicolon
desc table name// View the structure of the table
create table table name(
/ / student ID is of string type
/ / for the definition of the last variable at the end, there is no need to write comma interval
sno varchar(4), / / student number
sname varchar(8),
sex varchar(4),
age int(4)
);
select * from table name// Look up table
insert into table name (column name 1, column name 1...) values (data type of column name 1, data type of column name 2...);
Stop the database first and delete the database
update table name set column name 1 = 'column name value 1', column name n = 'column name value n' where PK column name = 'pK value' / / modify n columns in the row
delete from table name where student number = 'value'// Delete a data record
9.tomcat settings
9.1 installation path of Tomcat
It is best to use the default installation method of the system help, that is, the installation path of tomcat does not need to be modified!!
9.2 configuration path of Tomcat
Installation path attribute of computer tomcat / advanced settings / system variable / classpath / bin directory of tomcat installation
9.3 test configuration
Check whether the path has been configured in cmd or test it in the command prompt cmd of the computer. 8 is the version number of tomcat!!
9.4 check whether tomcat8 is installed successfully
Method 1: check whether the path has been configured in cmd or test it in the command prompt cmd of the computer. 8 is the version number of tomcat!!
If you use the command line to enter tomcat8, the system will display the following configuration information, and the configuration has been successful in classpath
Method 2: test in browser: http://localhost:8080/
1. Run the internal Tomcat file (double click the tomcat.exe file)
2. Note: if the main interface of tomcat cannot be loaded in the browser, it means that the executable exe of tomcat has not been run!!
example: http://localhost:8080/lanykey/login.jsp
Note: after compiling the files created by yourself in webapps under tomcat8 directory every time, click Run to the exe file under bin directory in tomcat8, otherwise the modified page will not display normally
Protocol: / / ip address: port number / the directory created by the user under the webapps folder of tomcat8 / the file in the same left directory (the file is composed of content)
Physical IP address of host 1
http://192.168.51.173:8080/lanykey/222.txt
2. The following two ip addresses are common
http://127.0.0.1:8080/lanykey/222.txt
http://localhost:8080/lanykey/222.txt
Note: 222 Txt file must be in the webapps path under the tomcat installation path, and the contents of the file must be included!!!
10. Install JUnit tool
Basic steps for downloading and installing JUnit tools
1. Download from the official website
2. Unzip the JUnit package to the local disk
3. Import this package in java Enterprise Edition
3.1 in java Enterprise Edition, select the item and click Properties in the tab
3.2 select three places: Java buy path / libraries / add external jars... / open to import "mysql-connector-java-5.1.49.jar"
3.3 select three places in the project and check use mysql... jar package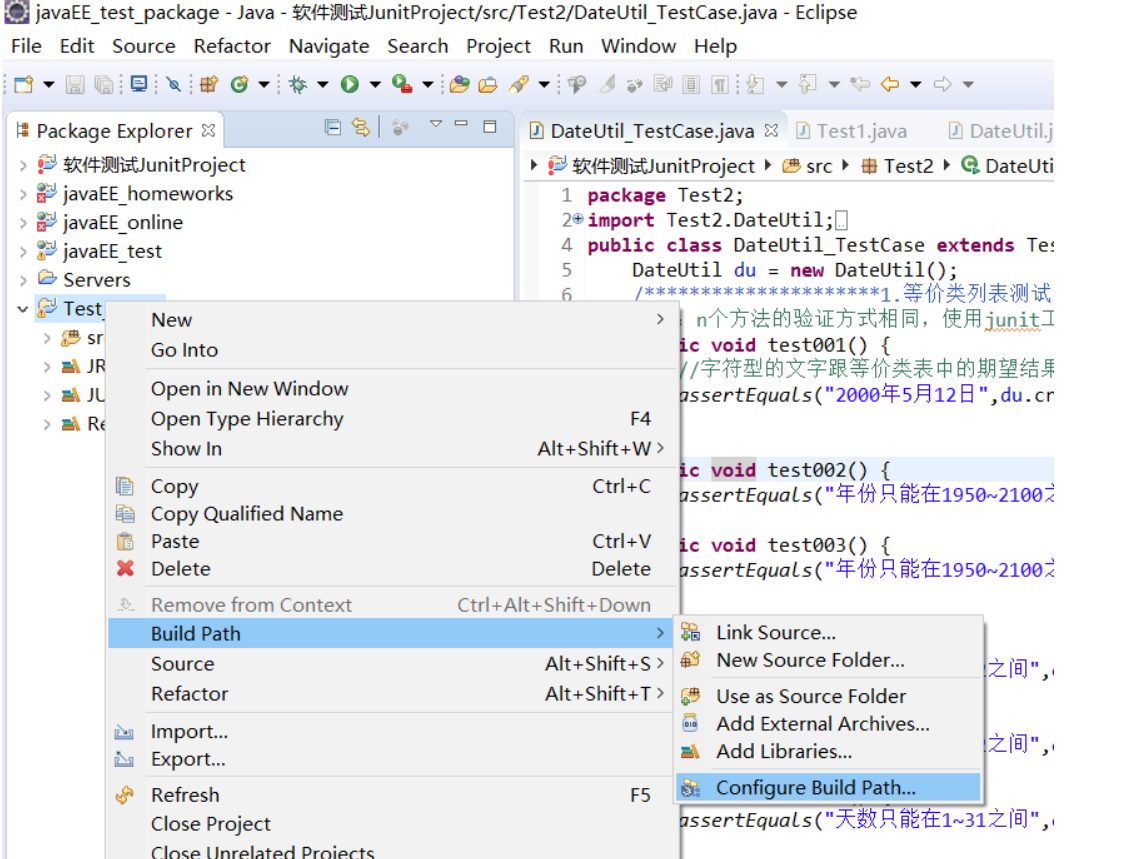
3.4 choose the JUnit tool you downloaded
"Test_finally" / "D:\mysql installation package \ mysql-connector-java-5.1.49\mysql-connector-java-5.1.49.jar" / "com.mysql.jdbc" / "Driver.class"
3.5 view JUnit driver name
"Test_finally" / "D:\mysql installation package \ mysql-connector-java-5.1.49\mysql-connector-java-5.1.49.jar" / "com.mysql.jdbc" / double click "Driver.class" / to find the name of the drive as "com.mysql.jdbc.Driver"
11.out.println and system out. The difference between println
out.println: output in the browser (in the browser accessed arbitrarily, the information in java is not displayed internally)
System.out.println: output in dos command line (displayed in command line of tomcat8)
12. Configure tomcat8 to access the web page
12.1 create four new folders (lanykey\WEB-INF) under the path webapps directory under tomcat8
C:\Program Files\Apache Software Foundation\Tomcat 8.0\webapps\lanykey\WEB-INF
Create two new folders under the path webapps directory under tomcat8, namely, the classes folder and the lib folder
12.2 for the class file in Test1 folder, copy the file of the whole project from java software to the desktop. Find the bin folder inside and copy the Test1 folder inside the bin, including several The classes file to the following directory, as shown in the following figure
Path: C:\Program Files\Apache Software Foundation\Tomcat 8.0\webapps\lanykey\WEB-INF\classes\Test1
12.3 copy local mysql driver package
C:\Program Files\Apache Software Foundation\Tomcat 8.0\webapps\lanykey\WEB-INF\lib
1. Login Class
Note: jsp, txt and other files are placed in the webapps folder in tomcat!!
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <html> <head>Login interface</head> <meta charset="UTF-8" /> <body> <form action="welcome.jsp"> user name:<input type="text" name="username"></input></br> password:<input type="text" name="password"></input></br> <input type="button" value="Sign in" /> <input type="reset" value="Reset" /> <input type="submit" /> <% String err= request.getParameter("error"); if(err!=null){ out.print("<span style='color:red;' >Wrong user name or password</span>"); } %> </form> </body> </html>
2. Welcome interface
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="Test1.UserInfoDao"%> <html> <head></head> <meta charset="UTF-8" /> <body> <% String str1 = request.getParameter("username"); String str2 = request.getParameter("password"); UserInfoDao dao= new UserInfoDao(); if(!dao.validateUserInfo(str1 ,str2 )){ response.sendRedirect("login.jsp?error=no"); } %> <h1>welcome! a warm welcome!</h1> </body> </html>
3.cmd command prompt
Enter the mysql command
mysql -u root -p
//new table create table UserInfo( account varchar(16), password varchar(16), username varchar(16) ); show databases;//Displays all existing database names create database lanykey; use lanykey;//Only after the database is created can the database name be used and other statements be inserted, modified and so on!! select * from UserInfo;// insert into UserInfo values('lanykey','123456','adff');// insert into UserInfo values('koki','1111','a');//
4. Visit the web page
http://localhost:8080/
http://localhost:8080/lanykey/login.jsp
http://localhost:8080/lanykey/login.jsp
After entering the correct information, click login first, and then click Submit
Note: localhost is a local account, 127.0.0.1 is accessible to every user, and 192.168.16.214 is the ip address of your host. Only this address can be accessed
Check the ip address of your computer. Enter ipconfig/all in the cmd command window of your computer to view the address of ipv4
5. In Tomcat directory class file
5.1 Student.java
package Test1; public class Student { public String sno; public String sname; public String sex; public int age; // @Override // public String toString() { // return "Student [sno=" + sno + ", sname=" + sname + ", sex=" + sex + ", age=" + age + "]"; // } }
5.2 StudentDao_test1 .java
package Test1; import java.util.List; public class StudentDao_test1 { /** * @param args */ public static void main(String[] args) { StudentDao sd = new StudentDao(); Student s = new Student(); s.sno="s012"; s.sname="ggg"; s.sex="female"; s.age=15; StudentDao.addStudent(s);//Add the value of s to the body of addStudent method sd.deleteStudent("s012"); s.sno="s008"; s.sname="QWQ"; s.sex="female"; s.age=111;;//Add the value of s to the body of addStudent method StudentDao.addStudent(s);//Add the value of s to the body of addStudent method List<Student> list = sd.getAll(); for(Student c:list){//foreach System.out.print(c.sno+"\t"); System.out.print(c.sname+"\t"); System.out.print(c.sex+"\t"); System.out.println(c.age+"\t"); } } }
5.3 StudentDao.java
package Test1; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.List; import javax.naming.ldap.Rdn; public class StudentDao { static void addStudent(Student student){ //1. Get connection try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction String sql = "insert into student " + "values('"+student.sno+"'," + " ' "+student.sname+"'," + " '"+student.sex+"'," +student.age+")"; //3.2 create statement executor through connection Statement st = conn.createStatement(); //3.3 operate the database through the statement executor st.execute(sql); st.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } } void deleteStudent(String ac){ //1. Get connection try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction String sql = "delete from student where sno='"+ac+"' "; // insert into student values('s030','ax','w',0); // insert into student values('s010','bx','m',0); // insert into student values('s020','cx','w',0); //3.2 preprocessing statement executor by connection Statement st = conn.prepareStatement(sql);//!! //3.3 operation database st.execute(sql); st.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } } // static void updateStudent(Student student){ //1. Get connection try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction String sql ="update student set sname='"+student.sname +"',sex='"+student.sex +"',age="+student.age +" where sno='"+student.sno+"'"; System.out.println(sql);//Used to check whether the statement is wrong!! //3.2 create statement executor through connection Statement st = conn.prepareStatement(sql); // if(st.execute(sql)){ // System.out.println("insert succeeded!"); // }else{ // System.out.println("insert failed!"); // } //3.3 operate the database through the statement executor st.execute(sql); st.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } } public List<Student> getAll(){ List<Student> list = new ArrayList<Student>();//ArrayList is a subclass of List!! //1. Get connection try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction String sql = "select * from student"; Statement st = conn.createStatement(); //3.3 operation database ResultSet rs = st.executeQuery(sql);//sql package while(rs.next()){ Student stu = new Student(); stu.sno = rs.getString("sno"); stu.sname = rs.getString("sname"); stu.sex = rs.getString("sex"); stu.age = rs.getInt("age"); list.add(stu);//!! } st.execute(sql); st.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } return list; } }
5.4 Test1.java
package Test1; //Basic framework of jdb import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class Test1 { public static void main(String[] args) { //Get connection //Tool class in memory try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password //String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; String url = "jdbc:mysql://127.0.0.1:3306/lanykey?useUnicode=true&characterEncoding=GBK"; //String url = "jdbc:mysql://127.0.0.1:3306/lanykey?useUnicode=true&characterEncoding=gb2312","root","123456"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction //String sql = "insert into student values('s001','QAQ ',' female ', 15)"; //String sql = "update student set sname='QBQ',sex =' male ', age = 15, where SnO ='s001'"; //String sql = "delete from student where sno='s001' "; String sql = "select * from student"; //3.2 create statement executor through connection Statement st = conn.createStatement(); //3.3 operate the database through the statement executor //st.execute(sql); //st.execute(sql); ResultSet rs = st.executeQuery(sql); while(rs.next()){ String sno = rs.getString("sno"); String sname = rs.getString("sname"); String sex = rs.getString("sex"); int age = rs.getInt("age"); System.out.print(sno+"\t"+sname+"\t"+sex+"\t"+age+"\n"); } } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } } }
5.5 UserInfo.java
package Test1; public class UserInfo { public String account; public String password; public String username; }
5.6 UserInfoDao .java
package Test1; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class UserInfoDao { public boolean validateUserInfo(String ac, String pwd){ boolean flag=false; //1. Get connection try { //1. Load driver class Class.forName("com.mysql.jdbc.Driver"); //2. Set connection information String username="root"; //Database user name String password="123456";//Database password String url ="jdbc:mysql://127.0.0.1:3306/lanykey"; //3. Equipment connection Connection conn = DriverManager.getConnection(url, username, password); System.out.println(conn); //3.1 setting instruction String sql = "select * from UserInfo " + "where account='"+ac+"'" + " and password='"+pwd+"'"; //3.2 create statement executor through connection Statement st = conn.createStatement(); ResultSet rs = st.executeQuery(sql);//sql package!! flag = rs.next(); //3.3 operate the database through the statement executor st.execute(sql); st.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } return flag; } }
5.7 UserInfoDaoTest.java
package Test1; public class UserInfoDaoTest { public static void main(String[] args) { UserInfoDao userInfoDao = new UserInfoDao(); System.err.println(userInfoDao.validateUserInfo("lanykey", "123456")); } }