1. When the system boot program starts the Linux kernel, the kernel will record various data structures and drivers. After loading, the Android system starts and loads the first user level process: Init.c(system\core\init)
//main() method in Init.c
int main(int argc, char **argv)
{
//Parsing execution init.rc configuration file
init_parse_config_file("/init.rc");
}
2. Execute the instructions defined in the configuration file init.rc(system\core\rootdir) to initialize the environment; execute many bin instructions to start system services
//Start the incubator process and execute app ﹣ process (a compiled executable)
service zygote /system/bin/app_process -Xzygote /system/bin --zygote --start-system-server
socket zygote stream 666
onrestart write /sys/android_power/request_state wake
onrestart write /sys/power/state on
onrestart restart media onrestart restart netd
3. Find app main.cpp in the app process file and check the main() method
int main(int argc, const char* const argv[])
{
//Start a system service: ZygoteInit
runtime.start("com.android.internal.os.ZygoteInit",startSystemServer);
}
4. In ZygoteInit.java, look at the main() method
public static void main(String argv[]) {
//Classes required for preloading Android system
preloadClasses();
if (argv[1].equals("true")) {
//Call method to start system service
startSystemServer();
}
}
preloadClasses() to load the Android runtime environment
/**
* The name of a resource file that contains classes to preload.
*/
//The "preloaded classes" file contains all the full package names that need to be loaded in Android
private static final String PRELOADED_CLASSES = "preloaded-classes";
private static void preloadClasses() {
//Use class loader to load resources as an input stream according to the file name
InputStream is = ZygoteInit.class.getClassLoader().getResourceAsStream(PRELOADED_CLASSES);
BufferedReader br = new BufferedReader(new InputStreamReader(is), 256);
String line;
//Read full package name line by line
while ((line = br.readLine()) != null) {
//Load classes by full package name
Class.forName(line);
}
}
startSystemServer(), which starts system services
String args[] = {
"--setuid=1000",
"--setgid=1000",
"--setgroups=1001,1002,1003,1004,1005,1006,1007,1008,
1009,1010,1018,3001,3002,3003",
"--capabilities=130104352,130104352",
"--runtime-init",
"--nice-name=system_server",
//Open system server service
"com.android.server.SystemServer",
};
5. Start system server service
//init1() method in C library
native public static void init1(String[] args);
public static void main(String[] args) {
//Loading C Library
System.loadLibrary("android_servers");
//Execute init1 method in C library
init1(args);
}
In the OM Android server systemserver.cpp file, find the init1() method
static JNINativeMethod gMethods[] = {
/* name, signature, funcPtr */
//Mapping a pointer to the init1 method, finally calling the system_init() method, while system_init() has no method body.
{ "init1", "([Ljava/lang/String;)V", (void*)
android_server_SystemServer_init1 },
};
static void android_server_SystemServer_init1(JNIEnv* env, jobject clazz)
{
system_init();
}
extern "C" int system_init();
Find the system init() method in the system init.cpp file
extern "C" status_t system_init()
{
//In this method, hardware management service is mainly enabled
SensorService::instantiate();
AudioFlinger::instantiate();
MediaPlayerService::instantiate();
CameraService::instantiate();
//Execute init2() method in SystemServer class
runtime->callStatic("com/android/server/SystemServer", "init2");
}
Go back to the init2() method in the SystemServer class
public static final void init2() {
//Create system service thread
Thread thr = new ServerThread();
thr.setName("android.server.ServerThread");
thr.start();
}
6. In the run() method of the ServerThread class, start other services of the system
//Prepare message poller
Looper.prepare();
//Start a large number of system services and add them to service manager one by one
ServiceManager.addService(Context.WINDOW_SERVICE, wm);
//Call the systemReady() method in the ActivityManagerService class to create the first activity
((ActivityManagerService)ActivityManagerNative.getDefault()).systemReady(new Runnable() {});
7. In ActivityManagerService.java, find systemReady() method
public ActivityStack mMainStack;
public void systemReady(final Runnable goingCallback) {
//Launch laucher
mMainStack.resumeTopActivityLocked(null);
}
Find the resumeTopActivityLocked() method in ActivityStack.java
final boolean resumeTopActivityLocked(ActivityRecord prev) {
ActivityRecord next = topRunningActivityLocked(null);
//Judge whether there is activity on the top of the stack. If not, start the laucher directly
if (next == null) {
// There are no more activities! Let's just start up the Launcher...
if (mMainStack) {
return mService.startHomeActivityLocked();
}
}
}
So far, Laucher has been started and Android system has been started;
Declaration: This article only does the study exchange, welcome everybody to raise each kind of question, lets us progress together!!!
Finally, attach a time sequence diagram
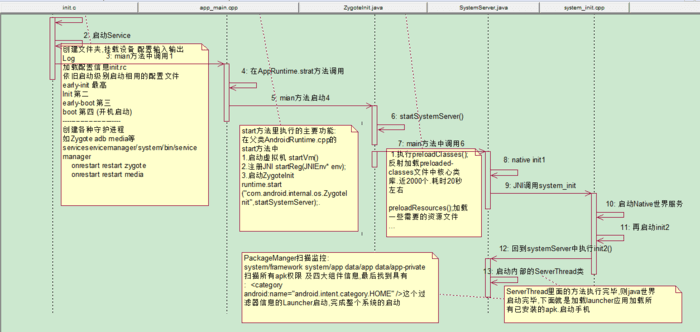