Background: it is needless to say how to write Java code more standardized. The most important points are to improve code performance, keep the code away from bugs and make the code more elegant.
1, MyBatis do not write 1 = 1 for multiple query criteria
When multiple query conditions are encountered, using where 1=1 can easily solve our problem, but it is likely to cause great performance loss, because after adding the "where 1=1" filter condition, the database system cannot use query optimization strategies such as index, The database system will be forced to scan each row of data (i.e. full table scan) to compare whether this row meets the filtering conditions. When the amount of data in the table is large, the query speed will be very slow. In addition, there will be the risk of SQL injection.
Counterexample:
<select id="queryBookInfo" parameterType="com.tjt.platform.entity.BookInfo" resultType="java.lang.Integer"> select count(*) from t_rule_BookInfo t where 1=1 <if test="title !=null and title !='' "> AND title = #{title} </if> <if test="author !=null and author !='' "> AND author = #{author} </if> </select>
Positive example:
<select id="queryBookInfo" parameterType="com.tjt.platform.entity.BookInfo" resultType="java.lang.Integer"> select count(*) from t_rule_BookInfo t <where> <if test="title !=null and title !='' "> title = #{title} </if> <if test="author !=null and author !='' "> AND author = #{author} </if> </where> </select>
The UPDATE operation is the same. You can use the < set > tag instead of 1 = 1.
2, Iterate entrySet() to get the key and value of the Map
When only the primary key of the Map needs to be obtained in the loop, the iterative keySet() is correct; However, when the primary key key and value are required, it is more efficient to iterate entrySet(), which has better performance than iterating keySet() first and then get ting value.
Counterexample:
//Counter example of getting value from Map: HashMap<String, String> map = new HashMap<>(); for (String key : map.keySet()){ String value = map.get(key); }
Positive example:
//Positive example of getting key & value from Map: HashMap<String, String> map = new HashMap<>(); for (Map.Entry<String,String> entry : map.entrySet()){ String key = entry.getKey(); String value = entry.getValue(); }
3, Using collection Isempty() detected null
Using collection Size () to detect whether it is empty is not a logical problem, but use collection Isempty () makes the code easier to read and provides better performance; In addition, any collection The time complexity of isempty() implementation is O(1), which does not require multiple loop traversal, but some are through collection The time complexity of the implementation of the size () method may be O(n). Example of O(1) latitude reduction cycle times.
Counterexample:
LinkedList<Object> collection = new LinkedList<>(); if (collection.size() == 0){ System.out.println("collection is empty."); }
Positive example:
LinkedList<Object> collection = new LinkedList<>(); if (collection.isEmpty()){ System.out.println("collection is empty."); } //To detect whether it is null, collectionutils. Can be used isEmpty() if (CollectionUtils.isEmpty(collection)){ System.out.println("collection is null."); }
4, When initializing a collection, try to specify its size
Specifying the size of the set during initialization can effectively reduce the number of expansion of the set, because the time complexity of each expansion of the set is likely to be O(n), which consumes time and performance.
Counterexample:
//Initialize the list and add elements to the list. Counterexample: int[] arr = new int[]{1,2,3,4}; List<Integer> list = new ArrayList<>(); for (int i : arr){ list.add(i); }
Positive example:
//Initialize the list and add elements to the list. Positive example: int[] arr = new int[]{1,2,3,4}; //Specifies the capacity size of the collection list List<Integer> list = new ArrayList<>(arr.length); for (int i : arr){ list.add(i); }
5, Splicing strings using StringBuilder
Generally, string splicing will be optimized by Java at compile time, but string splicing in the loop cannot be optimized at compile time, so it needs to be replaced by StringBuilder.
Counterexample:
//Concatenate string Counterexamples in loops String str = ""; for (int i = 0; i < 10; i++){ //String splicing in a loop is not optimized by Java str += i; }
Positive example:
//Splicing positive examples of strings in a loop String str1 = "Love"; String str2 = "Courage"; String strConcat = str1 + str2; //The Java compiler optimizes string splicing in this normal mode StringBuilder sb = new StringBuilder(); for (int i = 0; i < 10; i++){ //In the loop, the Java compiler cannot optimize, so use StringBuilder manually sb.append(i); }
6, If you need to call collection frequently The contains method uses Set
In the Java collection class library, the general time complexity of the contains method of list is O(n). If the contains method needs to be called frequently in the code to find data, first convert the collection list into a HashSet implementation, and the time complexity of O(n) will be O(1).
Counterexample:
//Call collection frequently Contains() counterexample List<Object> list = new ArrayList<>(); for (int i = 0; i <= Integer.MAX_VALUE; i++){ //The time complexity is O(n) if (list.contains(i)) System.out.println("list contains "+ i); }
Positive example:
//Call collection frequently Contains() positive example List<Object> list = new ArrayList<>(); Set<Object> set = new HashSet<>(); for (int i = 0; i <= Integer.MAX_VALUE; i++){ //The time complexity is O(1) if (set.contains(i)){ System.out.println("list contains "+ i); } }
7, Using static code blocks to assign static member variables
For static member variables of collection type, static code block assignment should be used instead of collection implementation.
Counterexample:
//Counterexample of assigning static member variables private static Map<String, Integer> map = new HashMap<String, Integer>(){ { map.put("Leo",1); map.put("Family-loving",2); map.put("Cold on the out side passionate on the inside",3); } }; private static List<String> list = new ArrayList<>(){ { list.add("Sagittarius"); list.add("Charming"); list.add("Perfectionist"); } };
Positive example:
//Positive example of static member variable assignment private static Map<String, Integer> map = new HashMap<String, Integer>(); static { map.put("Leo",1); map.put("Family-loving",2); map.put("Cold on the out side passionate on the inside",3); } private static List<String> list = new ArrayList<>(); static { list.add("Sagittarius"); list.add("Charming"); list.add("Perfectionist"); }
8, Remove unused local variables, method parameters, private methods, fields, and extra parentheses.
9, Mask constructors in tool classes
A tool class is a collection of static fields and functions, which should not be instantiated; However, Java adds an implicit public constructor for each class that does not explicitly define a constructor. In order to avoid unnecessary instantiation, you should explicitly define a private constructor to mask the implicit public constructor.
Counterexample:
public class PasswordUtils { //Counterexample of tool class constructor private static final Logger LOG = LoggerFactory.getLogger(PasswordUtils.class); public static final String DEFAULT_CRYPT_ALGO = "PBEWithMD5AndDES"; public static String encryptPassword(String aPassword) throws IOException { return new PasswordUtils(aPassword).encrypt(); }
Positive example:
public class PasswordUtils { //Positive example of tool class constructor private static final Logger LOG = LoggerFactory.getLogger(PasswordUtils.class); //Define a private constructor to mask this implicit public constructor private PasswordUtils(){} public static final String DEFAULT_CRYPT_ALGO = "PBEWithMD5AndDES"; public static String encryptPassword(String aPassword) throws IOException { return new PasswordUtils(aPassword).encrypt(); }
10, Remove redundant exception catches and throw
After catching an exception with a catch statement, if nothing is handled, just let the exception be thrown again. This is the same as the effect of not catching an exception. You can delete this code or add other processing.
Counterexample:
//Redundant exception counterexample private static String fileReader(String fileName)throws IOException{ try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; StringBuilder builder = new StringBuilder(); while ((line = reader.readLine()) != null) { builder.append(line); } return builder.toString(); } catch (Exception e) { //The exception is only thrown repeatedly without any processing throw e; } }
Positive example:
//Positive example of redundant abnormality private static String fileReader(String fileName)throws IOException{ try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; StringBuilder builder = new StringBuilder(); while ((line = reader.readLine()) != null) { builder.append(line); } return builder.toString(); //Delete redundant throwing exceptions or add other processing: /*catch (Exception e) { return "fileReader exception"; }*/ } }
11, String conversion uses string Valueof (value) instead of "" + value
When converting other objects or types to strings, use string Valueof (value) is more efficient than "" + value.
Counterexample:
//Convert other objects or types to strings counterexamples: int num = 520; // "" + value String strLove = "" + num;
Positive example:
//Convert other objects or types to string positive examples: int num = 520; // String.valueOf() is more efficient String strLove = String.valueOf(num);
12, Avoid using BigDecimal(double)
BigDecimal(double) has the risk of accuracy loss, which may lead to business logic exceptions in the scenario of accurate calculation or value comparison.
Counterexample:
// BigDecimal counterexample BigDecimal bigDecimal = new BigDecimal(0.11D);
Positive example:
// BigDecimal positive example BigDecimal bigDecimal1 = bigDecimal.valueOf(0.11D);
Figure 1 Loss of accuracy
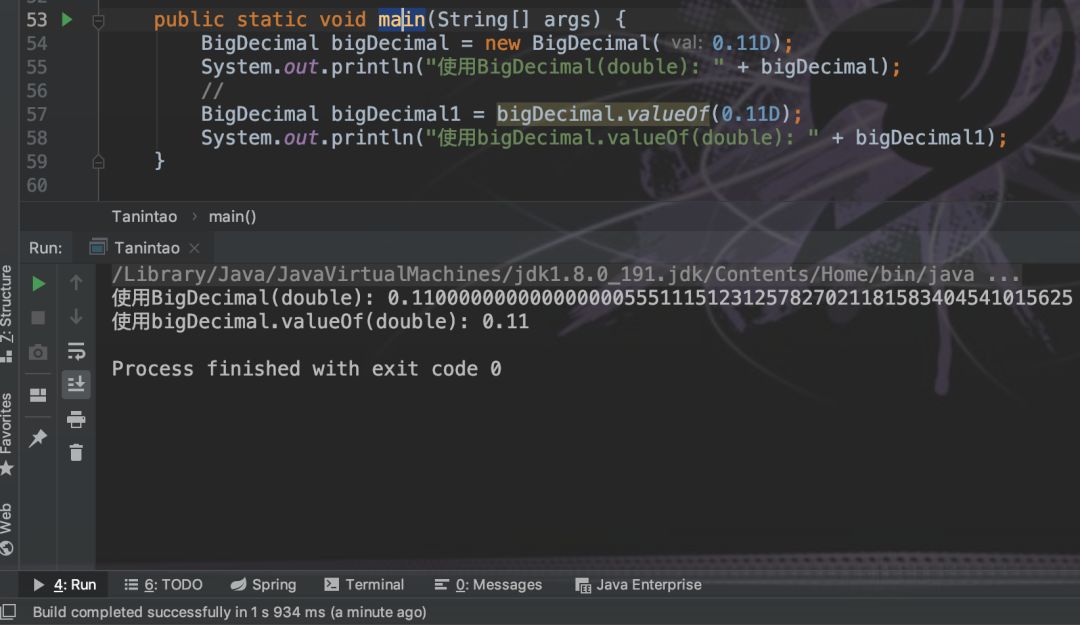
13, Returns empty arrays and collections instead of null
If the program returns null, the caller needs to force null detection, otherwise a null pointer exception will be thrown; Returning an empty array or empty collection effectively avoids the caller throwing a null pointer exception because it does not detect null. It can also delete the caller's null detection statement to make the code more concise.
Counterexample:
//Return null counterexample public static Result[] getResults() { return null; } public static List<Result> getResultList() { return null; } public static Map<String, Result> getResultMap() { return null; }
Positive example:
//Returns a positive example of an empty array and an empty set public static Result[] getResults() { return new Result[0]; } public static List<Result> getResultList() { return Collections.emptyList(); } public static Map<String, Result> getResultMap() { return Collections.emptyMap(); }
14, The equals method is called preferentially with constants or fixed values
The equals method of an object is easy to throw null pointer exceptions. You should call the equals method with a constant or an object with a certain value.
Counterexample:
//Counterexample of calling the equals method private static boolean fileReader(String fileName)throws IOException{ // Possible null pointer exception return fileName.equals("Charming"); }
Positive example:
//Positive example of calling equals method private static boolean fileReader(String fileName)throws IOException{ // The equals method is called using a constant or an object that is determined to have a value return "Charming".equals(fileName); //Or use: Java util. Objects. Equals() method return Objects.equals("Charming",fileName); }
15, The property fields of an enumeration must be private and immutable
Enumerations are usually used as constants. If there are public attribute fields or setting field methods in enumerations, the attributes of these enumerating constants can be easily modified; Ideally, the attribute field in the enumeration is private and assigned in the private constructor. There is no corresponding Setter method. It is best to add the final modifier.
Counterexample:
public enum SwitchStatus { // Counter examples of enumerated attribute fields DISABLED(0, "Disable"), ENABLED(1, "Enable"); public int value; private String description; private SwitchStatus(int value, String description) { this.value = value; this.description = description; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } }
Positive example:
public enum SwitchStatus { // Enumerated property field positive example DISABLED(0, "Disable"), ENABLED(1, "Enable"); // final modification private final int value; private final String description; private SwitchStatus(int value, String description) { this.value = value; this.description = description; } // There is no Setter method public int getValue() { return value; } public String getDescription() { return description; } }
16, Tring Some keywords of split (string regex) need to be translated
When using the plit method of String string, if the incoming delimited String is a regular expression, some keywords (such as. [] () \ |) need to be escaped.
Counterexample:
// String.split(String regex) counterexample String[] split = "a.ab.abc".split("."); System.out.println(Arrays.toString(split)); // The result is [] String[] split1 = "a|ab|abc".split("|"); System.out.println(Arrays.toString(split1)); // The result is ["a", "|," a "," B "," |, "a", "B", "C"]
Positive example:
// String. Positive example of split (string regex) // . Translation required String[] split2 = "a.ab.abc".split("\\."); System.out.println(Arrays.toString(split2)); // The result is ["a", "ab", "abc"] // |Translation required String[] split3 = "a|ab|abc".split("\\|"); System.out.println(Arrays.toString(split3)); // The result is ["a", "ab", "abc"]
Figure 2 String. Split (string regex) positive and negative examples
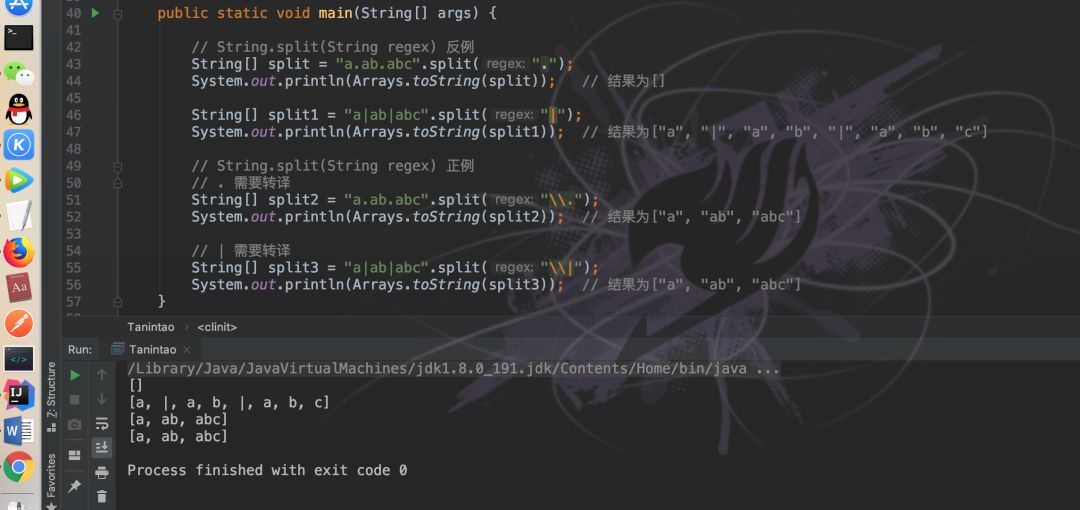