Specify the number, sum, and minimum of arrays to get a set of random numbers
- The original requirements are as follows:
Given sum SUM(A), given minimum number M, given length S of sequence, and precision P of each number in sequence, It is required that each number in the sequence must meet the requirements of a > = m, accuracy P, and uniformly increasing length S. Return to the sequence For example, SUM(A)=39, the minimum number is M1, the length S of the sequence is 6, and the precision P is 1. Here is a possible solution. 1.0,3.2,5.1,7.3,9.8,12.6.
- Java_random_numbers.java
This code is to implement the specified array number, sum and minimum value to obtain a set of random numbers
/*package whatever //do not write package name here */ import java.io.*; import java.util.Arrays; class GFG { public static double[] getRandDistArray(int n, double m, double min) { double randArray[] = new double[n]; double sum = 0; // Generate n random numbers for (int i = 0; i < randArray.length; i++) { randArray[i] = Math.random(); sum += randArray[i]; } // check number value int cnt = 0; while (true) { if ((randArray[cnt] / sum * m) < min) { sum -= randArray[cnt]; randArray[cnt] = Math.random(); sum += randArray[cnt]; cnt = -1; } cnt++; if (cnt >= randArray.length) { break; } } // Normalize sum to m for (int i = 0; i < randArray.length; i++) { randArray[i] /= sum; randArray[i] *= m; } return randArray; } public static void main (String[] args) { double sum[] = getRandDistArray(50, 1, 0.0001); Arrays.sort(sum); System.out.println(Arrays.toString(sum)); } }
Put the code on the following website to test:
https://ide.geeksforgeeks.org/index.php
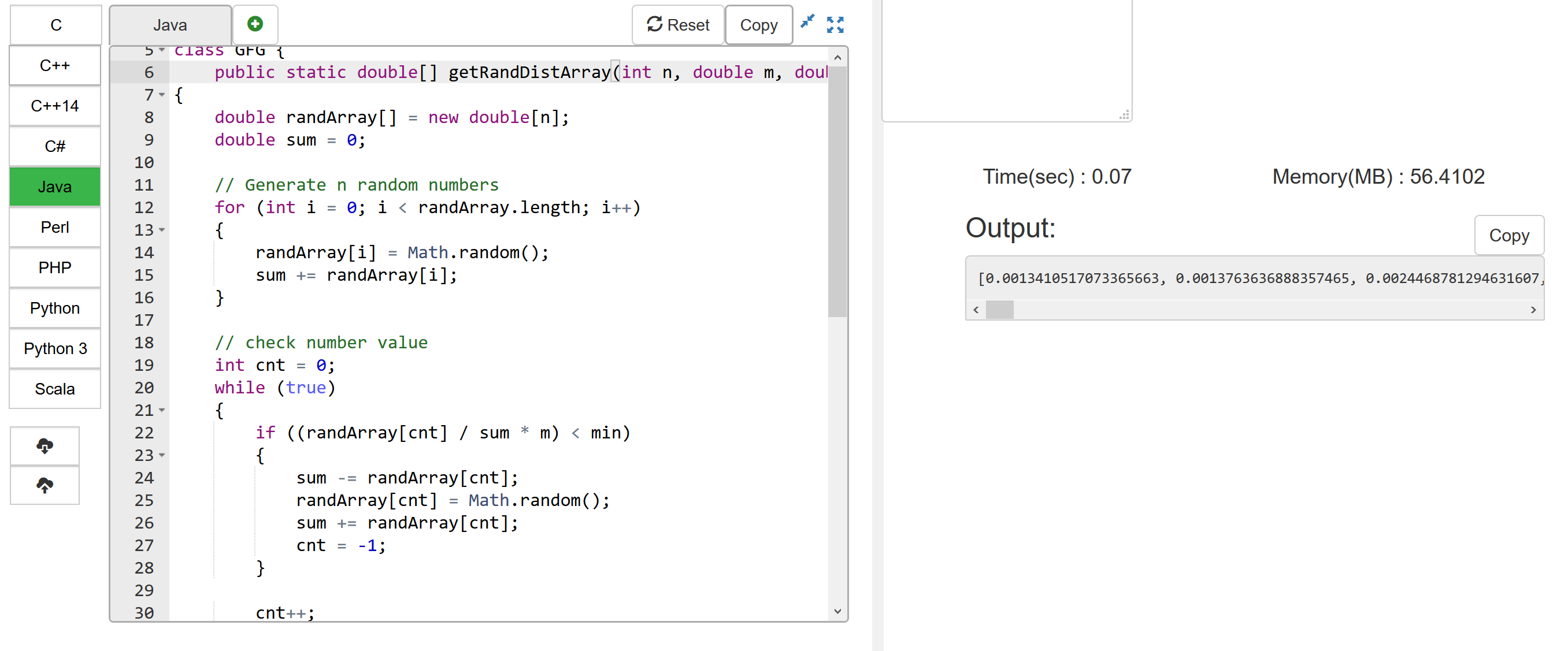
01.png
- Java_random_numbers_with_precision.java
This code is: specify the number of arrays, sum and minimum value, and generate a set of random numbers according to a certain interval and precision
The source code is: https://github.com/toniz/Java_random_numbers