1. In the distributed system environment, a method can only be executed by one thread of one machine at the same time;
2. High availability and high performance acquisition lock and release lock;
3. Reentrant feature;
4. Have lock failure mechanism to prevent deadlock;
5. It has the non blocking lock feature, that is, if the lock is not obtained, it will directly return the failure of obtaining the lock.
3, Three implementation methods of distributed lock
=================
At present, almost many large websites and applications are deployed distributed, and the problem of data consistency in distributed scenarios has always been an important topic. The distributed CAP theory tells us that "any distributed system can not meet consistency, availability and partition fault tolerance at the same time, and can only meet two requirements at most." Therefore, many systems have to choose between these three at the beginning of design. In most scenarios in the Internet field, consistency needs to be sacrificed in exchange for high availability of the system. The system often only needs to ensure the final consistency, as long as the final time is within the range acceptable to users.
In many scenarios, many technical solutions are needed to ensure the final consistency of data, such as distributed transactions, distributed locks, etc.
Three implementations of distributed locks:
1. Realize distributed lock based on database;
2. Implement distributed lock based on Redis;
3. Distributed based on Zookeeper;
Although there are these three schemes, different businesses should also be selected according to their own conditions. There is no best between them, only more suitable!
4, Implementation of distributed lock based on Database
=================
The core idea of the implementation method based on database is to create a table in the database, which contains fields such as method name, and create a unique index on the method name field. If you want to execute a method, you can use this method name to insert data into the table. If you insert successfully, you will get the lock, and delete the corresponding row data after execution to release the lock.
1. Create a table
DROP TABLE IF EXISTS `method_lock`; CREATE TABLE `method_lock` ( `id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT 'Primary key', `method_name` varchar(64) NOT NULL COMMENT 'Locked method name', `desc` varchar(255) NOT NULL COMMENT 'Remark information', `update_time` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP, PRIMARY KEY (`id`), UNIQUE KEY `uidx_method_name` (`method_name`) USING BTREE ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8 COMMENT='Methods in locking';
2. To execute a method, insert data into the table using the method name
INSERT INTO method_lock (method_name, desc) VALUES ('methodName', 'Tested methodName');
Because we are interested in method_name makes a uniqueness constraint. If multiple requests are submitted to the database at the same time, the database will ensure that only one operation can succeed. Then we can think that the thread that succeeded in the operation has obtained the lock of the method and can execute the content of the method body.
3. If the insertion is successful, the lock will be obtained. After execution, the corresponding row data will be deleted to release the lock
delete from method_lock where method_name ='methodName';
Note: This is only a method based on database. There are many other methods to use database to realize distributed lock.
4. Some existing problems
(1) Because it is implemented based on the database, the high availability and performance of the database will directly affect the availability and performance of the distributed lock. Therefore, the database needs dual machine hot standby, data synchronization and ready switching.
(2) It does not have the reentrant feature, because the row data always exists before the same thread releases the lock, and the data cannot be successfully inserted again. Therefore, it is necessary to add a new column in the table to record the information of the machine and thread currently obtaining the lock. When obtaining the lock again, first query whether the information of the machine and thread in the table is the current machine and thread. If they are the same, they will be directly obtained Remove the lock.
(3) There is no lock invalidation mechanism, because it is possible that after successfully inserting data, the server goes down and the corresponding data is not deleted. After the service is restored, the lock cannot be obtained. Therefore, a new column needs to be added in the table to record the invalidation time, and these invalid data need to be eliminated regularly.
(4) It does not have the blocking lock feature, and the failure is directly returned if the lock is not obtained. Therefore, it is necessary to optimize the acquisition logic and cycle for multiple times.
(5) Various problems are encountered in the implementation process. In order to solve these problems, the implementation method will be more and more complex. Relying on the database requires a certain resource overhead, and the performance problem needs to be considered.
5, Implementing distributed lock based on Redis
=======================
1. Reasons for using Redis to implement distributed locks
(1) Redis has high performance;
(2) Redis command supports this well and is easy to implement
2. Introduction to using commands
(1) setnx
SETNX key val: when the key does not exist, set a string with key val and return 1; If the key exists, do nothing and return 0.
(2)expire
expire key timeout: set a timeout for the key, in seconds. After this time, the lock will be automatically released to avoid deadlock.
(3)delete
Delete key.
3. Realization thought
(1) When acquiring a lock, use setnx to lock it, and use the expire command to add a timeout time for the lock. If the timeout time is exceeded, the lock will be released automatically. The value of the lock is a randomly generated UUID, which can be judged when releasing the lock.
(2) When acquiring a lock, a timeout time is also set. If it exceeds this time, the acquisition of the lock will be abandoned.
(3) When releasing a lock, judge whether it is the lock through UUID. If it is the lock, execute delete to release the lock.
4. Simple code of distributed lock
/** * Simple implementation code of distributed lock * Created by Su Xiaowen on 2020/2/12 */ public class DistributedLock { private final JedisPool jedisPool; public DistributedLock(JedisPool jedisPool) { this.jedisPool = jedisPool; } /** * Lock * @param lockName Lock key * @param acquireTimeout Get timeout * @param timeout Lock timeout * @return Lock identification */ public String lockWithTimeout(String lockName, long acquireTimeout, long timeout) { Jedis conn = null; String retIdentifier = null; try { // Get connection conn = jedisPool.getResource(); // Randomly generate a value String identifier = UUID.randomUUID().toString(); // Lock name, i.e. key value String lockKey = "lock:" + lockName; // Timeout. After locking, the lock will be released automatically int lockExpire = (int) (timeout / 1000); // The timeout period for acquiring the lock. If it exceeds this time, the acquisition of the lock will be abandoned long end = System.currentTimeMillis() + acquireTimeout; while (System.currentTimeMillis() < end) { if (conn.setnx(lockKey, identifier) == 1) { conn.expire(lockKey, lockExpire); // Returns a value to confirm the lock release time retIdentifier = identifier; return retIdentifier; } // If - 1 is returned, it means that no timeout is set for the key. Set a timeout for the key if (conn.ttl(lockKey) == -1) { conn.expire(lockKey, lockExpire); } try { Thread.sleep(10); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } } catch (JedisException e) { e.printStackTrace(); } finally { if (conn != null) { conn.close(); } } return retIdentifier; } /** * Release lock * @param lockName Lock key * @param identifier Identification of release lock * @return */ public boolean releaseLock(String lockName, String identifier) { Jedis conn = null; String lockKey = "lock:" + lockName; boolean retFlag = false; try { conn = jedisPool.getResource(); while (true) { // Monitor the lock and prepare to start the transaction conn.watch(lockKey); // Judge whether the lock is based on the previously returned value. If it is, delete and release the lock if (identifier.equals(conn.get(lockKey))) { Transaction transaction = conn.multi(); transaction.del(lockKey); List<Object> results = transaction.exec(); if (results == null) { continue; } retFlag = true; } conn.unwatch(); break; } } catch (JedisException e) { e.printStackTrace(); } finally { if (conn != null) { conn.close(); } } return retFlag; } }
5. Testing
In the example, 50 threads are used to simulate the second killing of a commodity, and the – operator is used to reduce the commodity. From the order of the results, we can see whether it is in the locked state.
Simulate the second kill service, in which the jedis thread pool is configured and passed to the distributed lock for use during initialization.
/** * Created by Su Xiaowen on 2020/2/12 */ public class Service { private static JedisPool pool = null; private DistributedLock lock = new DistributedLock(pool); int n = 500; static { ## summary Generally, large enterprises like this have several rounds of interviews, so they must take some time to collect and sort out the company's background and corporate culture, as the saying goes「Know yourself and the enemy, and you will never be defeated in a hundred battles」,Don't go to the interview blindly. There are many people who care about how to talk to you HR Talk about salary. Here's a suggestion. If your ideal salary is 30 K,You can talk to me HR Talk 33~35K,Instead of exposing your cards all at once, but you can't say it so directly. For example, your company was 25 K,You can talk to me HR What's the original salary? What can you give me? You said I wanted a 20%Pay rise. Finally, say a few more words about the recruitment platform. In short, before submitting your resume to the company, please confirm what the company is like. Go to Baidu first. Don't be trapped. There are some ill intentioned advertising parties waiting for you on each platform. Don't be fooled!!! Provide [free] Java Structure learning materials, learning technology contents include: Spring,Dubbo,MyBatis, RPC, Source code analysis, high concurrency, high performance, distributed,Performance optimization, advanced architecture development of microservices, etc. **[Java A full set of advanced information is available here for free](https://codechina.csdn.net/m0_60958482/java-p7)** also Java Core knowledge points+Full set of architect learning materials and videos+Interview Dictionary of first-line large factories+The interview resume template can be obtained+Ali group NetEase Tencent NetEase Iqiyi Kwai beep interview questions+Spring Source code collection+Java Architecture practice e-book. Interview, there are many people who care about how to talk to you HR Talk about salary. Here's a suggestion. If your ideal salary is 30 K,You can talk to me HR Talk 33~35K,Instead of exposing your cards all at once, but you can't say it so directly. For example, your company was 25 K,You can talk to me HR What's the original salary? What can you give me? You said I wanted a 20%Pay rise. Finally, say a few more words about the recruitment platform. In short, before submitting your resume to the company, please confirm what the company is like. Go to Baidu first. Don't be trapped. There are some ill intentioned advertising parties waiting for you on each platform. Don't be fooled!!! Provide [free] Java Structure learning materials, learning technology contents include: Spring,Dubbo,MyBatis, RPC, Source code analysis, high concurrency, high performance, distributed,Performance optimization, advanced architecture development of microservices, etc. **[Java A full set of advanced information is available here for free](https://codechina.csdn.net/m0_60958482/java-p7)** also Java Core knowledge points+Full set of architect learning materials and videos+Interview Dictionary of first-line large factories+The interview resume template can be obtained+Ali group NetEase Tencent NetEase Iqiyi Kwai beep interview questions+Spring Source code collection+Java Architecture practice e-book. 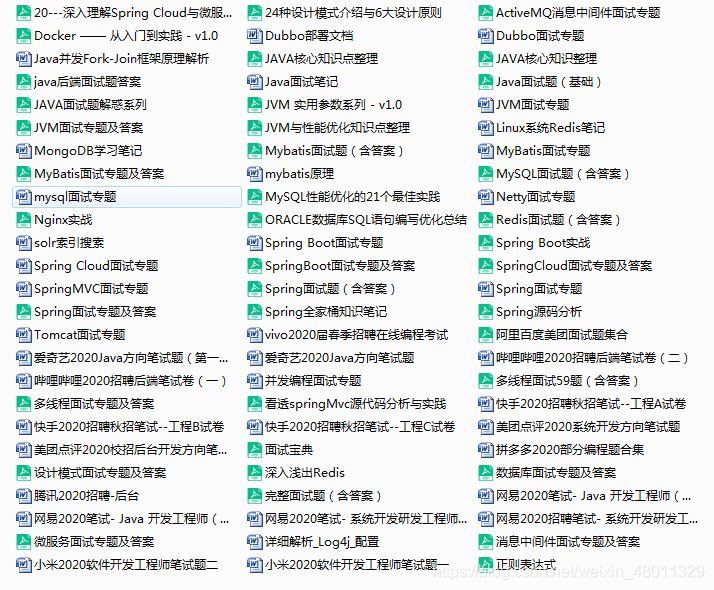