Two steps
1. Implement filter interface and filter method
2. Add @ Configuration annotation to add the custom filter to the filter chain
@Configuration public class WebConfiguration { @Bean public RemoteIpFilter remoteIpFilter() { return new RemoteIpFilter(); } @Bean public FilterRegistrationBean testFilterRegistration() { FilterRegistrationBean registration = new FilterRegistrationBean(); registration.setFilter(new MyFilter()); registration.addUrlPatterns("/*"); registration.addInitParameter("paramName", "paramValue"); registration.setName("MyFilter"); registration.setOrder(1); return registration; } public class MyFilter implements Filter { @Override public void destroy() { // TODO Auto-generated method stub } @Override public void doFilter(ServletRequest srequest, ServletResponse sresponse, FilterChain filterChain) throws IOException, ServletException { // TODO Auto-generated method stub HttpServletRequest request = (HttpServletRequest) srequest; System.out.println("this is MyFilter,url :"+request.getRequestURI()); filterChain.doFilter(srequest, sresponse); } @Override public void init(FilterConfig arg0) throws ServletException { // TODO Auto-generated method stub } } }
4, Custom property
In the process of Web development, I often need to customize some configuration files. How to use them?
1. Configure in application Properties
server.port=8081 #spring.profiles.active=dev # idea\uFF0Cproperties\u914D\u7F6E\u6587\u4EF6utf-8 # \uFFFD\uFFFD\uFFFD\uFFFDperson\uFFFD\uFFFD\u05B5 person.last-name=\u5F20\u4E09${random.uuid} person.age=${random.int} person.birth=2017/12/15 person.boss=false person.maps.k1=v1 person.maps.k2=14 person.lists=a,b,c person.dog.name=${person.hello:hello}_dog person.dog.age=15
2. Custom configuration class
@Component public class NeoProperties { @Value("${person.last-name}") private String last-name;//Last name here is the same as lastName @Value("${person.age}") private int age; ... //Omit getter settet method }
3. log configuration
Configure the address and output level of the output
logging.path=/user/local/log logging.level.com.favorites=DEBUG logging.level.org.springframework.web=INFO logging.level.org.hibernate=ERROR
Path is the local log address, logging After level, you can configure the log level of different resources according to the package path
5, Database operation
Here we focus on the use of MySQL, spring data jpa. jpa uses hibernate to generate various automated sql. If it is just a simple addition, deletion, modification and query, there is basically no need to write by hand. Spring has been encapsulated internally.
The following describes the use of spring boot
1. Add related jar packages
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
2. Add profile
spring.datasource.url=jdbc:mysql://localhost:3306/test spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.jpa.properties.hibernate.hbm2ddl.auto=update spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect spring.jpa.show-sql= true
In fact, this hibernate hbm2ddl. The auto parameter is mainly used for: automatic creation.
Verify that the database table structure has four values:
-
create: every time hibernate is loaded, the last generated table will be deleted, and then a new table will be generated again according to your model class, even if; This is an important reason for the loss of database table data.
-
Create drop: each time hibernate is loaded, a table is generated according to the model class, but the table is automatically deleted as soon as sessionFactory is closed.
-
update: the most commonly used attribute. When hibernate is loaded for the first time, the target structure will be automatically established according to the model class. When hibernate is loaded later, the table structure will be automatically updated according to the model class. Even if the table structure is changed, the rows in the table still exist and the previous rows will not be deleted. It should be noted that when deployed to the server, the table structure will not be established immediately. It will not be established until the application runs for the first time.
-
validate: every time hibernate is loaded, the database table structure will be verified and created. It will only be compared with the table in the database. No new table will be created, but new values will be inserted.
dialect mainly specifies that the storage engine for generating table names is InnoDBD
Show SQL: whether to print the automatically generated SQL to facilitate viewing during debugging
3. Add entity class and dao
@Entity public class User implements Serializable { private static final long serialVersionUID = 1L; @Id @GeneratedValue private Long id; @Column(nullable = false, unique = true) private String userName; @Column(nullable = false) private String passWord; @Column(nullable = false, unique = true) private String email; @Column(nullable = true, unique = true) private String nickName; @Column(nullable = false) private String regTime; //Omit getter, settet method and constructor }
dao only needs to inherit the JpaRepository class. There is almost no need to write methods. There is also a very good function, that is, it can automatically generate SQL according to the method name. For example, findByUserName , will automatically generate a query method with , userName , as the parameter. For example, , findAlll , will automatically query all the data in the table, Such as automatic paging and so on..
The field in Entity should not be a column, but must be added @Transient Annotation, without annotation, will also be mapped into columns
public interface UserRepository extends JpaRepository<User, Long> { User findByUserName(String userName); User findByUserNameOrEmail(String username, String email); }
4. Testing
@RunWith(SpringJUnit4ClassRunner.class) @SpringApplicationConfiguration(Application.class) public class UserRepositoryTests { @Autowired private UserRepository userRepository; @Test public void test() throws Exception { Date date = new Date(); DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG); String formattedDate = dateFormat.format(date); userRepository.save(new User("aa1", "aa@126.com", "aa", "aa123456",formattedDate)); userRepository.save(new User("bb2", "bb@126.com", "bb", "bb123456",formattedDate)); userRepository.save(new User("cc3", "cc@126.com", "cc", "cc123456",formattedDate)); Assert.assertEquals(9, userRepository.findAll().size()); Assert.assertEquals("bb", userRepository.findByUserNameOrEmail("bb", "cc@126.com").getNickName()); userRepository.delete(userRepository.findByUserName("aa1")); } }
6, Thymeleaf template
Spring Boot recommends using Thymeleaf instead of Jsp.
1. Introduction to Thymeleaf
Thymeleaf is a template engine for rendering XML/XHTML/HTML5 content. Similar to JSP. It can be easily integrated with spring MVC framework as a template engine for web applications. Compared with other template engines, the biggest feature of thymeleaf is that it can directly open and correctly display the template page in the browser without starting the whole web application.
Thymeleaf uses natural template technology, which means that thymeleaf's template syntax will not destroy the structure of the document, and the template is still a valid XML document. Templates can also be used as working prototypes, and thymeleaf # will replace static values at run time.
URLs play a very important role in Web application templates. It should be noted that thymeleaf processes URLs through syntax @ {...} To handle it. Thymeleaf supports absolute path URLs:
<a th:href="@{http://www.thymeleaf.org}">Thymeleaf</a>
Conditional evaluation
<a th:href="@{/login}" th:unless=${session.user != null}>Login</a>
for loop
<tr th:each="prod : ${prods}"> <td th:text="${prod.name}">Onions</td> <td th:text="${prod.price}">2.41</td> <td th:text="${prod.inStock}? #{true} : #{false}">yes</td> </tr>
2. Page is prototype
In the traditional Java Web development process, front-end engineers, like back-end engineers, also need to install a complete development environment, and then modify templates and static resource files in various Java ides, start / restart / reload the application server, refresh the page to see the final effect.
But in fact, the front-end engineer should pay more attention to the page. It is difficult to do this with JSP, because JSP can only see the effect in the browser after rendering in the application server. However, Thymeleaf can never keep up with this problem. Template rendering through attributes will not introduce any new tags that cannot be recognized by the browser, such as JSP, No more expressions will be written inside Tag. The whole page is directly opened as an HTML file with a browser, and you can almost see the final effect, which greatly liberates the productivity of front-end engineers. Their final delivery is pure HTML/CSS/JavaScript files.
7, Gradle build tool
spring projects suggest using Maven/Gradle to build projects. Compared with maven, gradle is more concise and more suitable for building large and complex projects. Gradle absorbs the features of Maven and ant, but Maven is still the mainstream in java. Let's learn about it first.
A project configured with Gradle:
buildscript { repositories { maven { url "http://repo.spring.io/libs-snapshot" } mavenLocal() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:1.3.6.RELEASE") } } apply plugin: 'java' //Adding a Java plug-in indicates that this is a java project apply plugin: 'spring-boot' //Add spring boot support apply plugin: 'war' //Add a War plug-in to export the War package apply plugin: 'eclipse' //Add Eclipse Plug-in, add Eclipse IDE support, and Intellij Idea is "idea" war { baseName = 'favorites' version = '0.1.0' } sourceCompatibility = 1.7 //Minimum compatible version jdk1.0 seven targetCompatibility = 1.7 //Target compatible version jdk1 seven repositories { // Maven warehouse mavenLocal() //Use local warehouse mavenCentral() //Use central warehouse maven { url "http://repo. spring. IO / LIBS snapshot "} / / use remote warehouse } dependencies { // Various dependent jar packages compile("org.springframework.boot:spring-boot-starter-web:1.3.6.RELEASE") compile("org.springframework.boot:spring-boot-starter-thymeleaf:1.3.6.RELEASE") compile("org.springframework.boot:spring-boot-starter-data-jpa:1.3.6.RELEASE") compile group: 'mysql', name: 'mysql-connector-java', version: '5.1.6' compile group: 'org.apache.commons', name: 'commons-lang3', version: '3.4' compile("org.springframework.boot:spring-boot-devtools:1.3.6.RELEASE") compile("org.springframework.boot:spring-boot-starter-test:1.3.6.RELEASE") compile 'org.webjars.bower:bootstrap:3.3.6' compile 'org.webjars.bower:jquery:2.2.4' compile("org.webjars:vue:1.0.24") compile 'org.webjars.bower:vue-resource:0.7.0' } bootRun { addResources = true }
8, WebJars
WebJars # is a magical thing that allows you to use various frameworks and components of the front end in the form of jar packages.
Data sharing
Receiving method: you can get it for free by stamping here At the same time, you can also "whore" to a detailed explanation of the Redis transaction source code.
1. Big algorithm factory -- byte jump interview question
2. 2000 pages of Internet Java interview questions
3. High order essential, algorithm learning
g-boot-starter-test:1.3.6.RELEASE")
compile 'org.webjars.bower:bootstrap:3.3.6' compile 'org.webjars.bower:jquery:2.2.4' compile("org.webjars:vue:1.0.24") compile 'org.webjars.bower:vue-resource:0.7.0'
}
bootRun {
addResources = true
}
VIII WebJars ---------- WebJars Is a very magical thing that can make everyone jar Package to use various frameworks and components of the front end. # Data sharing > **[Receiving method: you can get it for free by stamping here](https://gitee.com/vip204888/java-p7), and you can also "whore" to a detailed explanation of the Redis transaction source code** **1,Big algorithm factory -- byte jump interview question** [External chain picture transfer...(img-zZG3Cvsi-1628388959105)] **2,2000 Page Internet Java Interview questions** [External chain picture transfer...(img-rDJCNClr-1628388959107)] **3,High order essential, algorithm learning** 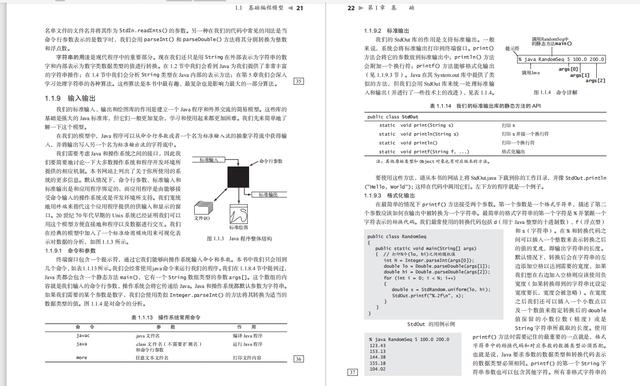