Kaptcha is a very practical verification code generation tool. It can generate a variety of verification codes through configuration, which can be displayed in the form of pictures, so it can not be copied and pasted.
Contents of this article
Introduction to Kaptcha2. Spring Boot Integration Kaptcha1. Introducing jar package into pom.xml2. Add Kaptcha configuration class3. Adding Control Layer Code4. View the generated authentication code
Introduction to Kaptcha
Kaptcha is a highly configurable practical verification code generation tool with freely configurable options such as:
- Verification Code Font
- Verification code font size
- Verification code font color
- Validation Code Content Range (Numbers, Letters, Chinese Characters)
- Verification Code Picture Size, Border, Border Thickness, Border Color
- Jamming line of verification code
- Style of Verification Code (Fisheye Style, 3D, Ordinary Fuzzy, etc.)
2. Spring Boot Integration Kaptcha
1. Introducing jar package into pom.xml
<!-- Verification Code -->
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
2. Add Kaptcha configuration class
The core code is as follows:
/**
* Verification Code Generated Beans
*/
@Bean
public DefaultKaptcha captchaProducer() {
DefaultKaptcha captchaProducer = new DefaultKaptcha();
Properties properties = new Properties();
properties.setProperty(Constants.KAPTCHA_IMAGE_WIDTH, "100");
properties.setProperty(Constants.KAPTCHA_IMAGE_HEIGHT, "30");
properties.setProperty(Constants.KAPTCHA_TEXTPRODUCER_FONT_SIZE, "22");
properties.setProperty(Constants.KAPTCHA_TEXTPRODUCER_CHAR_LENGTH, "4");
properties.setProperty(Constants.KAPTCHA_TEXTPRODUCER_CHAR_SPACE, "6");
properties.setProperty(Constants.KAPTCHA_TEXTPRODUCER_FONT_COLOR, "black");
properties.setProperty(Constants.KAPTCHA_BORDER_COLOR, "LIGHT_GRAY");
properties.setProperty(Constants.KAPTCHA_BACKGROUND_CLR_FROM, "WHITE");
properties.setProperty(Constants.KAPTCHA_NOISE_IMPL, "com.google.code.kaptcha.impl.NoNoise");
properties.setProperty(Constants.KAPTCHA_OBSCURIFICATOR_IMPL, "com.google.code.kaptcha.impl.ShadowGimpy");
properties.setProperty(Constants.KAPTCHA_TEXTPRODUCER_CHAR_STRING, "0123456789");
properties.setProperty(Constants.KAPTCHA_SESSION_CONFIG_KEY, "checkCode");
Config config = new Config(properties);
captchaProducer.setConfig(config);
return captchaProducer;
}
3. Adding Control Layer Code
The core code is as follows:
@Autowired
private Producer captchaProducer;
@RequestMapping("/getCode")
public ModelAndView getKaptchaImage(HttpServletRequest request, HttpServletResponse response) throws Exception {
response.setDateHeader("Expires", 0);
// Set standard HTTP/1.1 no-cache headers.
response.setHeader("Cache-Control", "no-store, no-cache, must-revalidate");
// Set IE extended HTTP/1.1 no-cache headers (use addHeader).
response.addHeader("Cache-Control", "post-check=0, pre-check=0");
// Set standard HTTP/1.0 no-cache header.
response.setHeader("Pragma", "no-cache");
response.setContentType("image/jpeg");
// create the text for the image
String capText = captchaProducer.createText();
log.info("******************Verification code is: " + capText + "******************");
// store the text in the session
request.getSession().setAttribute(Constants.KAPTCHA_SESSION_KEY, capText);
// create the image with the text
BufferedImage bi = captchaProducer.createImage(capText);
ServletOutputStream out = response.getOutputStream();
// write the data out
ImageIO.write(bi, "jpg", out);
try {
out.flush();
} finally {
out.close();
}
return null;
}
4. View the generated authentication code
Open the browser and visit http://localhost:8081/kaptcha/getCode. You can see the generated validation code. The screenshots of the generated validation code are as follows:
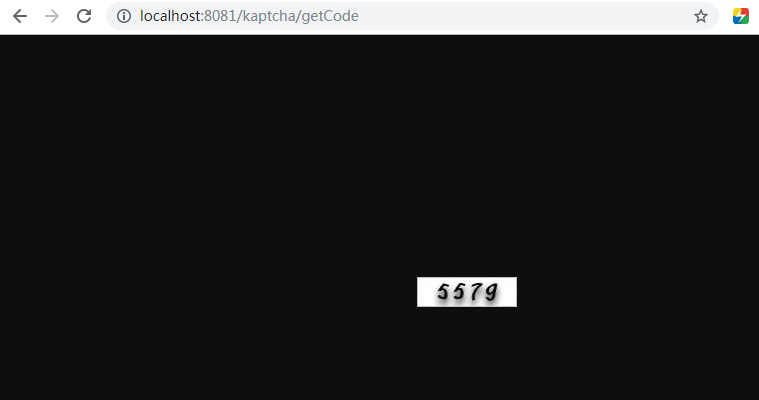
At this point, Spring Boot quickly integrates kaptcha to generate verification codes. If you have any questions, please leave a message to communicate.
Full source address: https://github.com/suisui2019/spring boot-study
Recommended reading
1.Spring Boot 2.X Integration Redis
2. How does Spring Boot 2.X gracefully solve cross-domain problems?
3.Spring Boot 2.X Integrates spring session to Realize session Sharing
4.Spring conditional annotation @Conditional
5.SpringBoot 2.X from 0 to 1 to realize mail sending function
6.Redis batch key removal tips, you know?
7. How can Spring Boot 2.X integrate jpa quickly?
8. Profile of Spring Boot--Quickly Solve Multi-environment Use and Switching
Timely access to free Java-related information covers Java, Redis, MongoDB, MySQL, Zookeeper, Spring Cloud, Dubbo/Kafka, Hadoop, Hbase, Flink and other high-concurrent distributed, large data, machine learning technology.
Pay attention to the following public numbers for free:
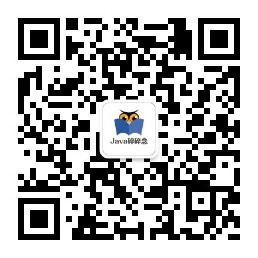