tinylog( https://tinylog.org/v2/ ), like other things that begin with tiny, is a lightweight open source logging solution. It itself contains only two JAR files (one for API and the other for Implementation), without any external dependencies. The total size of the two JAR files is only 178KB.
Although it is a lightweight scheme, the basic log management functions we commonly use are very complete. It has API design similar to other popular log frameworks, a variety of configurable log output options and excellent performance (this is the official Benchmark)( https://tinylog.org/v2/benchmark/ )).
Today, let's learn how to use tinylog to record logs in Spring Boot.
Integrated tinylog
Pass the previous spring boot 2 X basic tutorial: logging with log4j2( https://blog.didispace.com/spring-boot-learning-2-8-2 )After studying the article, recall that integrating other log frameworks can be summarized into the following steps:
- Exclude Spring Boot default logging framework dependencies
- Introduce log framework dependency to use
- Add the configuration file of the new log framework
OK, let's follow this step to practice:
Step 1: exclude the dependency of Spring Boot default logging framework
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <exclusions> <exclusion> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-logging</artifactId> </exclusion> </exclusions> </dependency> </dependencies>
Step 2: introduce tinylog dependency
<properties> <tinylog.version>2.4.1</tinylog.version> </properties> <dependencies> <dependency> <groupId>org.tinylog</groupId> <artifactId>tinylog-api</artifactId> <version>${tinylog.version}</version> </dependency> <dependency> <groupId>org.tinylog</groupId> <artifactId>tinylog-impl</artifactId> <version>${tinylog.version}</version> </dependency> <dependency> <groupId>org.tinylog</groupId> <artifactId>slf4j-tinylog</artifactId> <version>${tinylog.version}</version> </dependency> <dependency> <groupId>org.tinylog</groupId> <artifactId>jcl-tinylog</artifactId> <version>${tinylog.version}</version> </dependency> <dependency> <groupId>org.tinylog</groupId> <artifactId>log4j1.2-api</artifactId> <version>${tinylog.version}</version> </dependency> </dependencies>
Testing and verification
Here, the basic integration has been completed. We are not in a hurry to make a detailed configuration of tinylog. First verify whether everything here is correct. Like the previous example of log integration, write a main class and print logs at all levels.
@Slf4j @SpringBootApplication public class Chapter83Application { public static void main(String[] args) { SpringApplication.run(Chapter83Application.class, args); log.error("Hello World"); log.warn("Hello World"); log.info("Hello World"); log.debug("Hello World"); log.trace("Hello World"); } }
Lombok @ Slf4j is used here. If you don't understand it, please read this article: Lombok: make JAVA code more elegant( https://blog.didispace.com/java-lombok-1/ )
Run it and you can see the following output from the console:
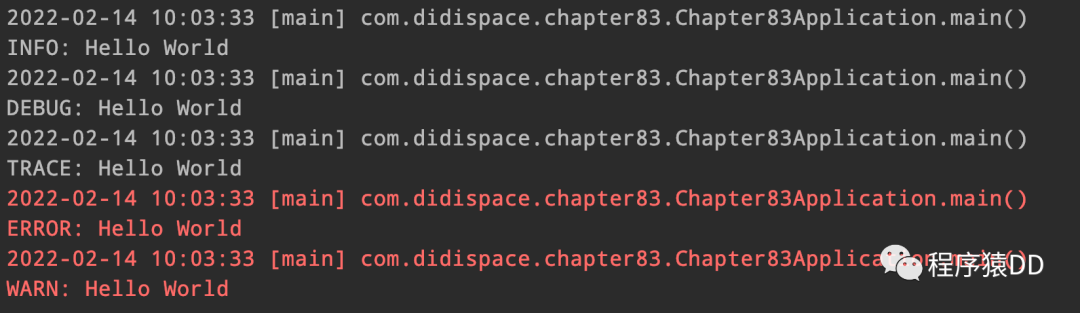
Through debug ging, we can see that the log at this time is TinylogLogger
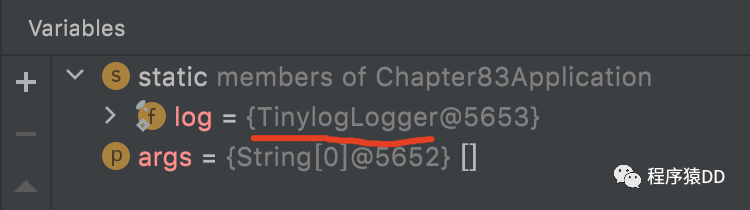
Step 3: add tinylog's configuration file
Through the previous step, although we have completed the integration, is the above format what you want? Add configuration adjustment!
Create a file in the resources directory: tinylog Properties, add the following configuration:
writer=console writer.format={date: HH:mm:ss.SSS} {level}: {message}
Run the test again. Does the console output look better
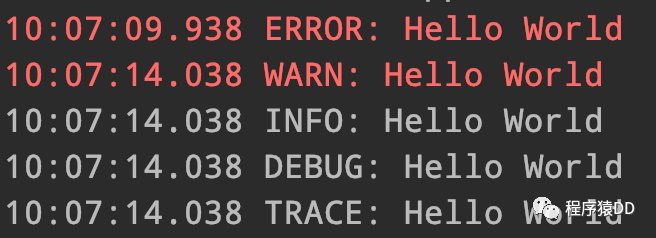
More configurations, such as file output and level control, will not be described in detail here. You can check the official documents( https://tinylog.org/v2/configuration/ ), it is basically similar to other frameworks and is easy to configure.
Code example
The complete project of this article can be viewed in the following warehouse 2 chapter8-3 project under X Directory:
- Github: https://github.com/dyc87112/SpringBoot-Learning/
- Gitee: https://gitee.com/didispace/SpringBoot-Learning/
If you think this article is good, welcome Star's support. Your attention is the driving force of my persistence!
Copyright declaration: This article is the official account of the program "monkey DD". Reprint: reprint completely without complete deletion, and the source of the official account is the number of the source and the author, and the card or the two-dimensional code for the official account. Non standard reprint, plagiarism and draft washing shall all complain of infringement.
Hello, I'm program ape DD. I've developed old driver, Alibaba cloud MVP, Tencent cloud TVP for 10 years, published books, started business, state-owned enterprise for 4 years and Internet for 6 years. 10 years ago, I graduated and joined the universe industry. My salary is not high and I am not too busy. I insist on studying technology and doing what I want to do in my spare time. Four years later, he left the state-owned enterprise and joined the entrepreneurial team of Yonghui Internet, from development, to architecture, to partners. Along the way, my deepest feeling is that I must keep learning and pay attention to the frontier. As long as you can stick to it, think more, complain less and do it frequently, it's easy to overtake in corners! So don't ask me if I can do it in time. If you are optimistic about a thing, you must adhere to it to see hope, rather than adhere to it only when you see hope. Believe me, as long as you stick to it, you will be better than now