Abstract: original source: www.bysocket.com Com Mason BYSocket hopes to reprint and keep the summary, thank you!
Thymeleaf is a Template language. What is the Template language or Template Engine? Common Template languages contain the following concepts: Data, Template, Template Engine, and Result Documents.
- Data is the manifestation and carrier of information, which can be symbols, words, numbers, voice, images, videos, etc. Data and information are inseparable. Data is the expression of information and information is the connotation of data. Data itself has no meaning. Data becomes information only when it has an impact on entity behavior.
- A template is a blueprint, that is, a type independent class. When using a template, the compiler instantiates the template according to the template arguments to get a type related class.
- Template engine template engine (here specifically refers to the template engine for Web Development) is generated to separate the user interface from business data (content). It can generate documents in specific formats, and the template engine for the website will generate a standard HTML document.
- Result document a document in a specific format, such as a template engine for a website, will generate a standard HTML document.
Template language has a wide range of uses. The common uses are as follows:
- Page rendering
- Document generation
- code generation
- All application scenarios of "data + template = text"
The purpose of the case here is naturally page rendering. Next, integrate Thymeleaf in Spring Boot to implement a complete Web case.
1, Run chapter-2-spring-boot-quick-start
The chapter-2-spring-boot-quick-start project uses an in memory database and does not need to configure a data source. Download and run.
1. Download project
git clone download project springboot learning example. See GitHub for the project address: https://github.com/JeffLi1993/springboot-learning-example , i.e.:
git clone https://github.com/JeffLi1993/springboot-learning-example.git
2. Engineering structure
Open the project with IDEA, and you can see the sub project chapter-2-spring-boot-quick-start. Its directory is as follows:
├── pom.xml └── src ├── main │ ├── java │ │ └── spring │ │ └── boot │ │ └── core │ │ ├── QuickStartApplication.java │ │ ├── domain │ │ │ ├── User.java │ │ │ └── UserRepository.java │ │ ├── service │ │ │ ├── UserService.java │ │ │ └── impl │ │ │ └── UserServiceImpl.java │ │ └── web │ │ └── UserController.java │ └── resources │ ├── application.properties │ ├── static │ │ ├── css │ │ │ └── default.css │ │ └── images │ │ └── favicon.ico │ └── templates │ ├── userForm.html │ └── userList.html └── test └── java └── spring └── boot └── core ├── QuickStartApplicationTests.java └── domain └── UserRepositoryTests.java
Corresponding directory:
- org.spring.springboot.controller - Controller layer
- org.spring.springboot.dao - data operation layer DAO
- org.spring.springboot.domain - entity class
- org.spring.springboot.service - business logic layer
- Application - application startup class
- application.properties - Application Profile
The template will use the following two directories
- static directory is used to store CSS, JS and other resource files
- The templates directory is where the views are stored
3. Compile and run the project
In the root directory of the project, run the maven command to compile:
cd chapter-2-spring-boot-quick-start mvn clean install
After the project is compiled successfully, right-click to run quickstartapplication The Java application starts the main function of the class, and then the browser accesses localhost:8080/users: user list page:
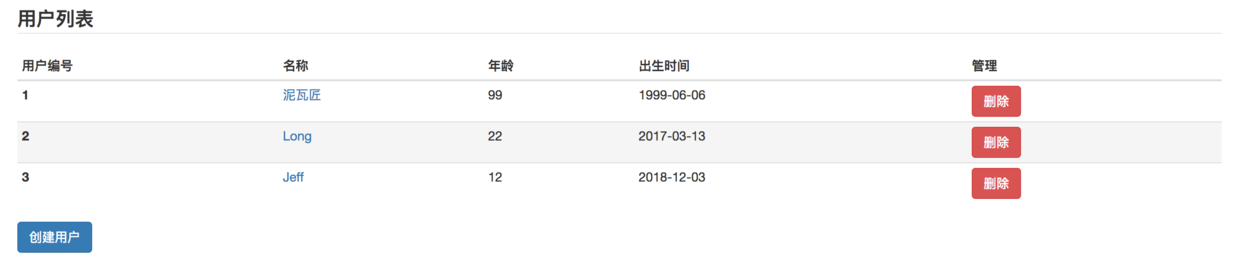
User edit page:
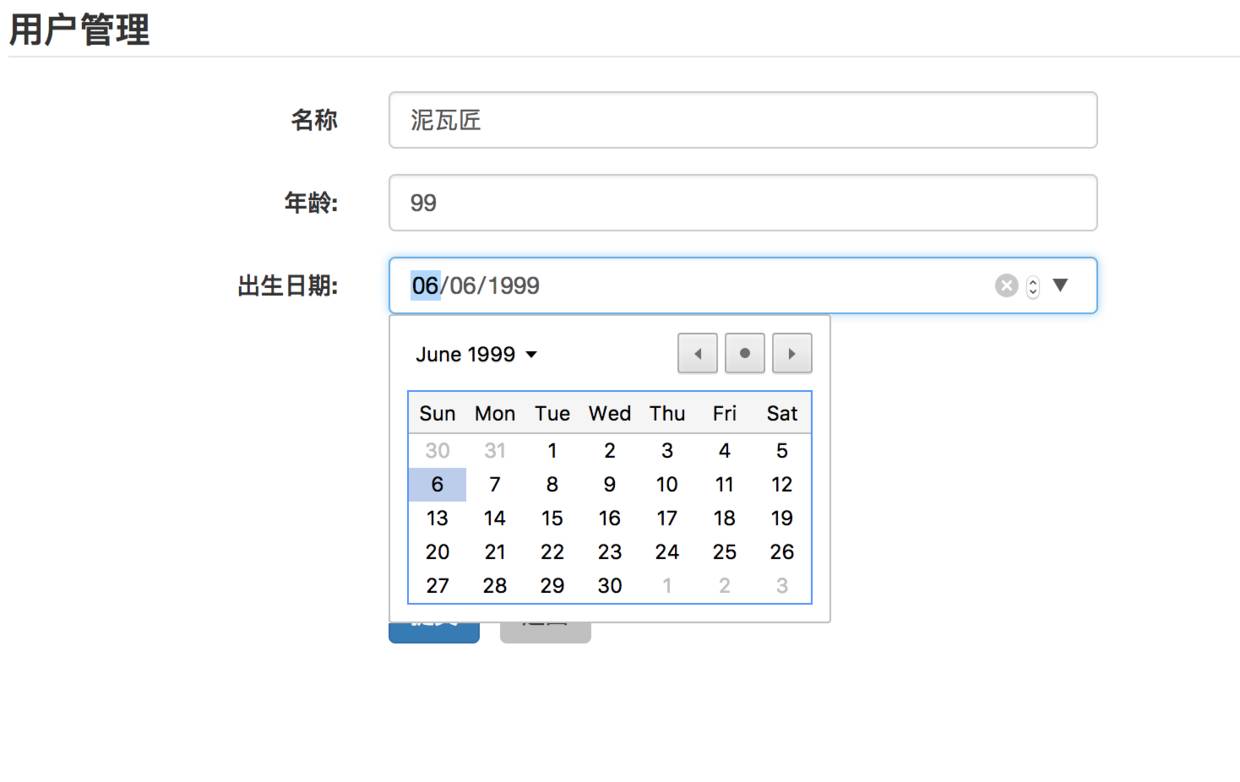
2, chapter-2-spring-boot-quick-start
Project code:
1. pom.xml Thymeleaf dependency
Use the template engine, just in POM Add Thymeleaf component dependency to XML:
<!-- template engine Thymeleaf rely on --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
What is thymeleaf? Thymeleaf is a modern server-side Java template engine for both web and standalone environments.
Thymeleaf's main goal is to bring elegant natural templates to your development workflow — HTML that can be correctly displayed in browsers and also work as static prototypes, allowing for stronger collaboration in development teams.
Thymeleaf is a new generation of Java template engine, which is recommended after Spring 4.
A POM The XML configuration is as follows:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>spring.boot.core</groupId> <artifactId>chapter-2-spring-boot-quick-start</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>chapter-2-spring-boot-quick-start</name> <description>Chapter II quick start cases</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.7.RELEASE</version> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <!-- Web rely on --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- Unit test dependency --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!-- Spring Data JPA rely on :: Data persistence layer framework --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <!-- h2 Data source connection driver --> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> <!-- template engine Thymeleaf rely on --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies> <build> <plugins> <!-- Spring Boot Maven plug-in unit --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
2. Thymeleaf dependency configuration
Add the Thymeleaf dependency to the Spring Boot project to start its default configuration. If you want to customize the configuration, you can go to application The properties configuration is as follows:
spring.thymeleaf.cache=true # Enable template caching. spring.thymeleaf.check-template=true # Check that the template exists before rendering it. spring.thymeleaf.check-template-location=true # Check that the templates location exists. spring.thymeleaf.enabled=true # Enable Thymeleaf view resolution for Web frameworks. spring.thymeleaf.encoding=UTF-8 # Template files encoding. spring.thymeleaf.excluded-view-names= # Comma-separated list of view names that should be excluded from resolution. spring.thymeleaf.mode=HTML5 # Template mode to be applied to templates. See also StandardTemplateModeHandlers. spring.thymeleaf.prefix=classpath:/templates/ # Prefix that gets prepended to view names when building a URL. spring.thymeleaf.reactive.max-chunk-size= # Maximum size of data buffers used for writing to the response, in bytes. spring.thymeleaf.reactive.media-types= # Media types supported by the view technology. spring.thymeleaf.servlet.content-type=text/html # Content-Type value written to HTTP responses. spring.thymeleaf.suffix=.html # Suffix that gets appended to view names when building a URL. spring.thymeleaf.template-resolver-order= # Order of the template resolver in the chain. spring.thymeleaf.view-names= # Comma-separated list of view names that can be resolved.
3. Use of thymeleaf
How does the Controller point the View to Thymeleaf
The user control layer code is as follows:
@Controller @RequestMapping(value = "/users") // Configure here so that the following mappings are in / users public class UserController { @Autowired UserService userService; // User service layer /** * Get user list * Process the GET request of "/ users" to GET the list of users * Pass parameters through @ RequestParam to further realize conditional query or paging query */ @RequestMapping(method = RequestMethod.GET) public String getUserList(ModelMap map) { map.addAttribute("userList", userService.findAll()); return "userList"; } /** * Show create user form * */ @RequestMapping(value = "/create", method = RequestMethod.GET) public String createUserForm(ModelMap map) { map.addAttribute("user", new User()); map.addAttribute("action", "create"); return "userForm"; } /** * Create user * Handle the POST request of "/ users" to get the user list * Bind parameters through @ ModelAttribute and pass parameters from the page through @ RequestParam */ @RequestMapping(value = "/create", method = RequestMethod.POST) public String postUser(@ModelAttribute User user) { userService.insertByUser(user); return "redirect:/users/"; } /** * Displays the user form that needs to be updated * Process the GET request of "/ users/{id}", and obtain User information through the id value in the URL * URL id in, bind parameters through @ PathVariable */ @RequestMapping(value = "/update/{id}", method = RequestMethod.GET) public String getUser(@PathVariable Long id, ModelMap map) { map.addAttribute("user", userService.findById(id)); map.addAttribute("action", "update"); return "userForm"; } /** * Process PUT request of "/ users/{id}" to update User information * */ @RequestMapping(value = "/update", method = RequestMethod.POST) public String putUser(@ModelAttribute User user) { userService.update(user); return "redirect:/users/"; } /** * Process the GET request of "/ users/{id}" to delete User information */ @RequestMapping(value = "/delete/{id}", method = RequestMethod.GET) public String deleteUser(@PathVariable Long id) { userService.delete(id); return "redirect:/users/"; } }
ModelMap object to bind data to the view. return string, which corresponds to the template name of the directory under resources/templates@ The ModelAttribute annotation is used to obtain the data submitted by the page Form and bind it to the User data object.
Form form page
Core code:
<form th:action="@{/users/{action}(action=${action})}" method="post" class="form-horizontal"> <input type="hidden" name="id" th:value="${user.id}"/> <div class="form-group"> <label for="user_name" class="col-sm-2 control-label">name</label> <div class="col-xs-4"> <input type="text" class="form-control" id="user_name" name="name" th:value="${user.name}" /> </div> </div> <div class="form-group"> <label for="user_age" class="col-sm-2 control-label">Age:</label> <div class="col-xs-4"> <input type="text" class="form-control" id="user_age" name="age" th:value="${user.age}"/> </div> </div> <div class="form-group"> <label for="user_birthday" class="col-sm-2 control-label">date of birth:</label> <div class="col-xs-4"> <input type="date" class="form-control" id="user_birthday" name="birthday" th:value="${user.birthday}"/> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <input class="btn btn-primary" type="submit" value="Submit"/> <input class="btn" type="button" value="return" onclick="history.back()"/> </div> </div> </form>
A Form is defined here to add or update users.
List page
The code is as follows:
<table class="table table-hover table-condensed"> <legend> <strong>User list</strong> </legend> <thead> <tr> <th>User number</th> <th>name</th> <th>Age</th> <th>time of birth</th> <th>Administration</th> </tr> </thead> <tbody> <tr th:each="user : ${userList}"> <th scope="row" th:text="${user.id}"></th> <td><a th:href="@{/users/update/{userId}(userId=${user.id})}" th:text="${user.name}"></a></td> <td th:text="${user.age}"></td> <td th:text="${user.birthday}"></td> <td><a class="btn btn-danger" th:href="@{/users/delete/{userId}(userId=${user.id})}">delete</a></td> </tr> </tbody> </table>
The list of users is looped here.
Syntax of Tymeleaf
I won't expand in detail. Let's look at what others wrote http://www.cnblogs.com/nuoyiamy/p/5591559.html Or look at the official documents http://www.thymeleaf.org/documentation.html
3, Summary of this paper
This paper makes a Web CRUD case using Thymeleaf. Let's give more advice~
If the above articles or links are helpful to you, don't forget to comment at the end of the article ~ you can also click the "share" button on the right side of the page to let more people read this article.