Guide reading
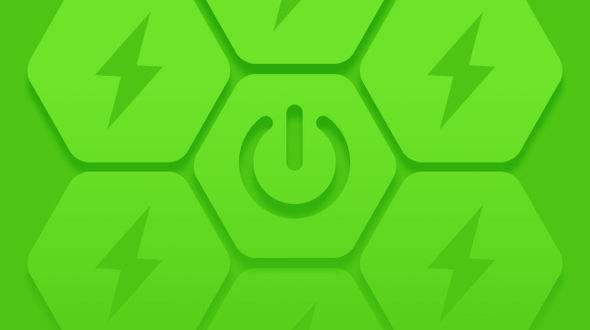
Spring Boot project development has gradually become the mainstream framework in the field of Java application development. It can not only create production-level Spring applications easily, but also easily integrate with the hot micro-service framework Spring Cloud through some annotation configurations.
The core reason why Spring Boot can easily create applications and integrate with other frameworks is that it greatly simplifies the configuration of projects and maximizes the principle of "agreement is greater than configuration". However, Spring Boot is a great convenience for development, but it is also easy to let people "in the clouds", especially the various annotations are easy to let people "know but don't know why".
Therefore, in order to make good use of Spring Boot, we must have a comprehensive and clear understanding of the various functional annotations it provides. On the one hand, it can improve the development efficiency based on Spring Boot, on the other hand, it is also the necessary knowledge points to be mastered when the framework principle is asked in the interview. In the following content, Xiaobian will take you to explore some of Spring Boot's commonly used annotations!
Spring related 6 annotations

Some of Spring Boot's annotations also need to be used with Spring's annotations. Here's a summary of the annotations of the six Spring basic frameworks that most closely cooperate with Spring Boot's annotations in the project. For example (1)
1,@Configuration
From Spring 3.0, @Configuration is used to define configuration classes, which can replace xml configuration files. The annotated classes contain one or more methods annotated by @Bean. These methods will be scanned by Annotation Config Application Context or Annotation Config Web Application Context classes and used to build bean definitions, initially. Spring container.
@Configuration public class TaskAutoConfiguration { @Bean @Profile("biz-electrfence-controller") public BizElectrfenceControllerJob bizElectrfenceControllerJob() { return new BizElectrfenceControllerJob(); } @Bean @Profile("biz-consume-1-datasync") public BizBikeElectrFenceTradeSyncJob bizBikeElectrFenceTradeSyncJob() { return new BizBikeElectrFenceTradeSyncJob(); } }
2,@ComponentScan
Students who have done web development must have used the @Controller,@Service,@Repository annotations. Looking at the source code, you will find that they have a common annotation @Component. Yes, the @ComponentScan annotation will assemble the classes with the @Controller,@Service,@Repository,@Component annotations into the spring container by default.
@ComponentScan(value = "com.abacus.check.api") public class CheckApiApplication { public static void main(String[] args) { SpringApplication.run(CheckApiApplication.class, args); } }
@ The SpringBootApplication annotation also contains the @ComponentScan annotation, so we can configure it in use through the scanBasePackages attribute of the @SpringBootApplication annotation.
@SpringBootApplication(scanBasePackages = {"com.abacus.check.api", "com.abacus.check.service"}) public class CheckApiApplication { public static void main(String[] args) { SpringApplication.run(CheckApiApplication.class, args); } }
3,@Conditional
@ Conditional is a new annotation provided by Spring 4. Different beans can be loaded according to the conditions set in the code through the @Conditional annotation. Before setting the condition annotation, the loaded bean class is first used to implement the Condition interface, and then the condition whether the class implementing the interface is loaded or not is set. The @ConditionalOnProperty,@ConditionalOnBean and other annotations in the Spring Book annotations that start with @Conditional* are all implemented by integrating @Conditional.
4,@Import
The instance is added to the spring IOC container by importing. Classes not managed by Spring containers can be imported into Spring containers as needed.
//Class Definition public class Square {} public class Circular {} //Import @Import({Square.class,Circular.class}) @Configuration public class MainConfig{}
5,@ImportResource
Similar to @Import, the difference is that @ImportResource imports configuration files.
@ImportResource("classpath:spring-redis.xml") //Import xml configuration public class CheckApiApplication { public static void main(String[] args) { SpringApplication.run(CheckApiApplication.class, args); } }
6,@Component
@ Component is a meta-annotation, meaning that other class annotations can be annotated, such as @Controller@Service@Repository. Classes with this annotation are considered components and instantiated when using annotation-based configuration and class path scanning. Other class-level annotations can also be identified as a special type of component, such as @Controller Controller Controller (Injection Service), @Service Service (Injection dao), @Repository dao (Implementing dao Access). @ Component refers to components. When components are not categorized properly, we can use this annotation to annotate them, which is equivalent to XML configuration, <bean id=""class="/>.
Twenty Annotations at the Core of Spring Boot
Having said a few basic Spring annotations that are closely related to Spring Boot, let's take a look at the core annotations provided by Spring Boot.
1,@SpringBootApplication
This annotation is the core of Spring Boot annotations, which are used on Spring Boot's main classes. It identifies this as a Spring Boot application, which is used to open the capabilities of Spring Boot. In fact, this annotation is a combination of @Configuration, @Enable AutoConfiguration, @ComponentScan annotations. Since these annotations are generally used together, Spring Boot provides a unified annotation @Spring Boot Application.
@SpringBootApplication(exclude = { MongoAutoConfiguration.class, MongoDataAutoConfiguration.class, DataSourceAutoConfiguration.class, ValidationAutoConfiguration.class, MybatisAutoConfiguration.class, MailSenderAutoConfiguration.class, }) public class API { public static void main(String[] args) { SpringApplication.run(API.class, args); } }
2,@EnableAutoConfiguration
Allow Spring Boot to automatically configure annotations, and after opening the annotation, Spring Boot can configure Spring Bean based on the package or class under the current class path.
For example, Mybatis is a JAR package under the current class path, and the Mybatis AutoConfiguration annotation can configure Mybatis Spring Bean s according to the relevant parameters.
@ The key to the implementation of Enable AutoConfiguration lies in the introduction of AutoConfiguration Import Selector, whose core logic is the selectImports method. The logic is as follows:
Load all possible automatic configuration classes from the configuration file META-INF/spring.factories;
Remove duplication and exclude classes carried by exclude and excludeName attributes.
Filter and return the automatic configuration class that satisfies the condition (@Conditional);
@Target({ElementType.TYPE}) @Retention(RetentionPolicy.RUNTIME) @Documented @Inherited @AutoConfigurationPackage//Import the subclass @Import ({Enable AutoConfiguration ImportSelector. class}) of AutoConfiguration ImportSelector public @interface EnableAutoConfiguration { String ENABLED_OVERRIDE_PROPERTY = "spring.boot.enableautoconfiguration"; Class<?>[] exclude() default {}; String[] excludeName() default {}; }
3,@SpringBootConfiguration
This annotation is a variant of the @Configuration annotation, which is only used to modify the Spring Boot configuration, or to facilitate subsequent Spring Boot extensions.
4,@ConditionalOnBean
@ Conditional OnBean (A.class) instantiates a Bean only if an A object exists in the current context, which means that the current bean can be created only if A.class exists in spring's application context.
@Bean //The RocketMQ Producer Lifecycle Bean can only be created when there is a DefaultMQ Producer instance in the current environment context @ConditionalOnBean(DefaultMQProducer.class) public RocketMQProducerLifecycle rocketMQLifecycle() { return new RocketMQProducerLifecycle(); }
5,@ConditionalOnMissingBean
Combining @Conditional annotations, as opposed to @Conditional OnBean annotations, instantiates a Bean only if there is no A object in the current context.
@Bean //Creation of RocketMQProducer Bean objects is allowed only if the RocketMQProducer object is missing from the current environment context @ConditionalOnMissingBean(RocketMQProducer.class) public RocketMQProducer mqProducer() { return new RocketMQProducer(); }
6,@ConditionalOnClass
Combining @Conditional annotations allows you to create a Bean only if certain classes exist on the classpath.
@Bean //When the HealthIndicator class exists in the classpath, the HealthIndicator Bean object is created. @ConditionalOnClass(HealthIndicator.class) public HealthIndicator rocketMQProducerHealthIndicator(Map<String, DefaultMQProducer> producers) { if (producers.size() == 1) { return new RocketMQProducerHealthIndicator(producers.values().iterator().next()); } }
7,@ConditionalOnMissingClass
Combining the @Conditional annotation, as opposed to the @Conditional OnMissing Class annotation, opens the configuration when no Class is specified in the classpath.
8,@ConditionalOnWebApplication
Combining the @Conditional annotation, the current project type is a WEB project to open the configuration. There are three types of current projects: ANY (any Web project matches), SERVLET (only basic Servelet projects match), and REACTIVE (only response-based web applications match).
9,@ConditionalOnNotWebApplication
Combining @Conditional annotations, as opposed to @Conditional On WEB Application annotations, the current project type is not a WEB project to open the configuration.
10,@ConditionalOnProperty
Combine the @Conditional annotation to open the configuration when the specified property has a specified value. The specific operation is achieved by its two attribute names and havingValue, where name is used from application.properties Read an attribute value and return false if it is empty; if it is not empty, compare it with the value specified by havingValue and return true if it is the same; otherwise return false. If the return value is false, the configuration does not take effect; if true, it takes effect.
@Bean //Whether the value of matching attribute rocketmq.producer.enabled is true @ConditionalOnProperty(value = "rocketmq.producer.enabled", havingValue = "true", matchIfMissing = true) public RocketMQProducer mqProducer() { return new RocketMQProducer(); }
11,@ConditionalOnExpression
Combine @Conditional annotations to open the configuration when the SpEL expression is true.
@Configuration @ConditionalOnExpression("${enabled:false}") public class BigpipeConfiguration { @Bean public OrderMessageMonitor orderMessageMonitor(ConfigContext configContext) { return new OrderMessageMonitor(configContext); } }
12,@ConditionalOnJava
Combine @Conditional annotations to open the configuration when the running Java JVM is within the specified version range.
13,@ConditionalOnResource
Combine the @Conditional annotation to open the configuration when there is a specified resource under the class path.
@Bean @ConditionalOnResource(resources="classpath:shiro.ini") protected Realm iniClasspathRealm(){ return new Realm(); }
14,@ConditionalOnJndi
Combine @Conditional annotations to open the configuration when the specified JNDI exists.
15,@ConditionalOnCloudPlatform
Combine @Conditional annotations to open the configuration when the specified cloud platform is activated.
16,@ConditionalOnSingleCandidate
Combine the @Conditional annotation to open the configuration when the specified class has only one Bean in the container or multiple but preferred classes at the same time.
17,@ConfigurationProperties
Spring Boot can use annotations to map custom properties files to entity bean s, such as config.properties files.
@Data @ConfigurationProperties("rocketmq.consumer") public class RocketMQConsumerProperties extends RocketMQProperties { private boolean enabled = true; private String consumerGroup; private MessageModel messageModel = MessageModel.CLUSTERING; private ConsumeFromWhere consumeFromWhere = ConsumeFromWhere.CONSUME_FROM_LAST_OFFSET; private int consumeThreadMin = 20; private int consumeThreadMax = 64; private int consumeConcurrentlyMaxSpan = 2000; private int pullThresholdForQueue = 1000; private int pullInterval = 0; private int consumeMessageBatchMaxSize = 1; private int pullBatchSize = 32; }
18,@EnableConfigurationProperties
When the @Enable Configuration Properties annotation is applied to your @Configuration, any beans annotated by @Configuration Properties will be automatically configured by the Environment property. This style of configuration is particularly suitable for use in conjunction with Spring Application's external YAML configuration.
@Configuration @EnableConfigurationProperties({ RocketMQProducerProperties.class, RocketMQConsumerProperties.class, }) @AutoConfigureOrder public class RocketMQAutoConfiguration { @Value("${spring.application.name}") private String applicationName; }
19,@AutoConfigureAfter
Used on an automatic configuration class to indicate that the automatic configuration class needs to be configured after another specified automatic configuration class has been configured.
For example, Mybatis's automatic configuration class needs to be after the data source's automatic configuration class.
@AutoConfigureAfter(DataSourceAutoConfiguration.class) public class MybatisAutoConfiguration { }
20,@AutoConfigureBefore
Contrary to the @AutoConfigureAfter annotation, this indicates that the automatic configuration class needs to be configured before another specified automatic configuration class.
21,@AutoConfigureOrder
Spring Boot 1.3.0 has a new annotation @AutoConfigureOrder to determine the priority of configuration loading.
@AutoConfigureOrder(Ordered.HIGHEST_PRECEDENCE) // Automatically configure the highest priority @Configuration @ConditionalOnWebApplication // web applications only @Import(BeanPostProcessorsRegistrar.class) // Settings for importing built-in containers public class EmbeddedServletContainerAutoConfiguration { @Configuration @ConditionalOnClass({ Servlet.class, Tomcat.class }) @ConditionalOnMissingBean(value = EmbeddedServletContainerFactory.class, search = SearchStrategy.CURRENT) public static class EmbeddedTomcat { // ... } @Configuration @ConditionalOnClass({ Servlet.class, Server.class, Loader.class, WebAppContext.class }) @ConditionalOnMissingBean(value = EmbeddedServletContainerFactory.class, search = SearchStrategy.CURRENT) public static class EmbeddedJetty { // ... } }
Last
Here I recommend an architecture learning circle to you. Click to join the communication circle Senior architects will share some organized video recordings and BATJ interview questions: Spring, MyBatis, Netty source code analysis, principles of high concurrency, high performance, distributed, micro-service architecture, JVM performance optimization, distributed architecture and so on, which become necessary knowledge systems for architects. They can also receive free learning resources, which have benefited a lot at present.
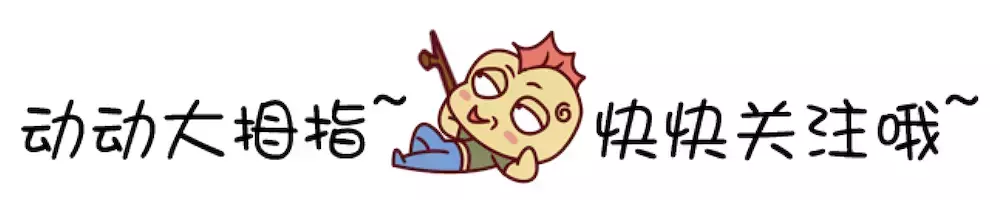
Note:
Author: Invincible Coder
Original: https://www.cnblogs.com/wudimanong/p/10457211.html