Spring boot uses Druid data sources
When the program operates the database, it needs to use the database connection, and the performance of the database connection is related to the database connection pool. Druid is an open source database connection pool implementation of Alibaba. It combines the advantages of database connection pools such as C3P0 and DBCP, and adds log monitoring. Druid's strength lies in its application in monitoring. It can well monitor the execution of database connection pool and SQL.
When using JDBC in SpringBoot, you can see that its default data source is Hikari data source. Both Hikari and Druid are the best data sources in the Java Web. Now try integrating Druid data sources in SpringBoot!
1. Configure data source
Go directly to the Maven warehouse to find a Druid dependency. Here I use the springboot starter version
<!-- Druid --> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid-spring-boot-starter</artifactId> <version>1.2.6</version> </dependency>
Then in application Configure the data source in yaml, specifically the type attribute of the data source
spring: datasource: username: root password: '0723' # serverTimezone=UTC resolves time zone issues url: jdbc:mysql://localhost:3306/mybatis?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8 # Use cj above mysql8 driver-class-name: com.mysql.cj.jdbc.Driver type: com.alibaba.druid.pool.DruidDataSource
Then the configuration is finished, that's it. Directly test the previous test program and check the data source and the obtained connection
@SpringBootTest class SpringBoot05DataApplicationTests { // Automatic assembly data source @Autowired DataSource dataSource; @Test void contextLoads() throws SQLException { // View data source: class com alibaba. druid. spring. boot. autoconfigure. DruidDataSourceWrapper System.out.println(dataSource.getClass()); // Get database connection Connection connection = dataSource.getConnection(); // View the connections you've got. Com mysql. cj. jdbc. ConnectionImpl@2d3ef181 System.out.println(connection); connection.close(); } }
It can be seen that the data source has become Druid, but the database connection is still JDBC, indicating that no matter what data source, its bottom layer is JDBC!
Then you can further configure Druid in yaml, for example (log4j is used here, so you need to import the dependency of log4j, otherwise an error will be reported)
type: com.alibaba.druid.pool.DruidDataSource #druid data source proprietary configuration druid: initialSize: 5 minIdle: 5 maxActive: 20 maxWait: 60000 timeBetweenEvictionRunsMillis: 60000 minEvictableIdleTimeMillis: 300000 validationQuery: SELECT 1 FROM DUAL testWhileIdle: true testOnBorrow: false testOnReturn: false poolPreparedStatements: true #Configure filters for monitoring statistics interception, stat: monitoring statistics log4j: logging wall: Defense sql injection filters: stat,wall,log4j maxPoolPreparedStatementPerConnectionSize: 20 useGlobalDataSourceStat: true connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
After configuration, the operations in the previous JDBC controller will not change, because the bottom layer is the implementation of JDBC! However, the method of running the test connection is visible
@SpringBootTest class SpringBoot05DataApplicationTests { // Automatic assembly data source @Autowired DataSource dataSource; @Test void contextLoads() throws SQLException { // View data source: class com alibaba. druid. spring. boot. autoconfigure. DruidDataSourceWrapper System.out.println(dataSource.getClass()); // Get database connection Connection connection = dataSource.getConnection(); // View the connections you've got. Com alibaba. druid. proxy. jdbc. ConnectionProxyImpl@75b6dd5b System.out.println(connection); DruidDataSource druidDataSource = (DruidDataSource) dataSource; System.out.println("druidDataSource Maximum connections to data source:" + druidDataSource.getMaxActive()); System.out.println("druidDataSource Number of data source initialization connections:" + druidDataSource.getInitialSize()); connection.close(); } } /* class com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceWrapper com.alibaba.druid.proxy.jdbc.ConnectionProxyImpl@75b6dd5b druidDataSource Maximum connections to data source: 20 druidDataSource Number of data source initialization connections: 5 */
Visible configuration has taken effect!
In addition to specifying the attribute configuration of Druid in the global configuration file, you can also create the DruidConfig class to inject the attributes in the configuration file into com alibaba. druid. pool. Druiddatasource parameter with the same name. Try it below.
2. Configuration monitoring
The Druid data source and some of its properties are simply configured above, but these works can be done by other data sources. Now let's try to use Druid's monitoring function, which is really powerful.
To configure Druid, you need to go to com qiyuan. The DruidConfig class is created under the config package, annotated as a configuration class, and bound to the attributes in the yaml configuration file
@Configuration public class DruidConfig { /* @ConfigurationProperties(prefix = "spring.datasource") The function is to add the global configuration file The prefix is spring datasource. The attribute value of Druid is injected into com alibaba. druid. pool. Druiddatasource is in a parameter with the same name */ // In fact, spring can be used directly in yaml datasource. Druid, that is, above. Why am I doing this? @ConfigurationProperties(prefix = "spring.datasource") @Bean public DataSource druidDataSource() { return new DruidDataSource(); } }
Then set up Druid's background management page. You need to register a Servlet in SpringBoot, which is equivalent to the previous web The steps of configuring Servlet in XML
@Configuration public class DruidConfig { ... // SpringBoot has a built-in Servlet container. This method is on the web XML register Servlet replacement! @Bean public ServletRegistrationBean statViewServlet(){ ServletRegistrationBean bean = new ServletRegistrationBean(new StatViewServlet(), "/druid/*"); Map<String,String> initParams = new HashMap<>(); // Set the login account and password of the background interface. The key is fixed initParams.put("loginUsername","qiyuanc"); initParams.put("loginPassword","0723"); // Sets the objects that are allowed to be accessed initParams.put("allow",""); // Set objects that are not accessible //initParams.put("deny","192.168.1.1"); bean.setInitParameters(initParams); return bean; } }
After configuring the Servlet of the page in DruidConfig, you can enter the home page of Druid through localhost: 8080/druid. Log in according to the set user name and password, and you can see that there are many fancy functions!
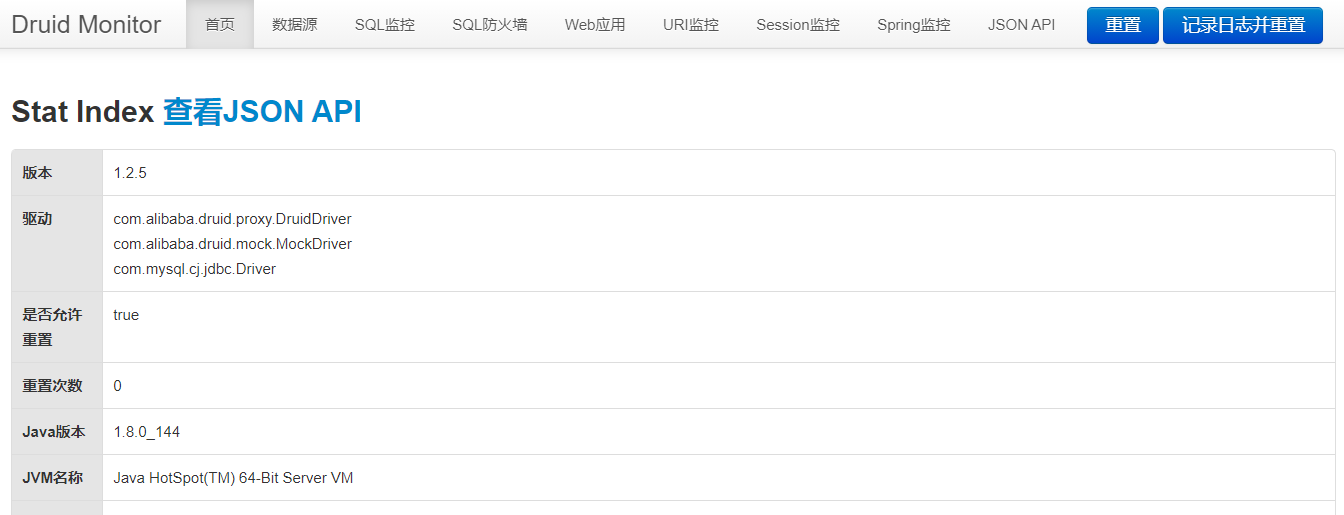
Enter the SQL monitoring interface, and then execute the SQL test in the previous JDBC controller to see the specific monitoring report!

It's true. It's very powerful!
In addition, you can also configure the management association between the Web and Druid data sources. The monitoring statistics WebStatFilter is similar to registering the Servlet above. Just register a filter
@Configuration public class DruidConfig { ... @Bean public FilterRegistrationBean webStatFilter(){ FilterRegistrationBean bean = new FilterRegistrationBean(); bean.setFilter(new WebStatFilter()); Map<String, String> initParams = new HashMap<>(); // exclusions sets which requests are not filtered, so statistics are not performed initParams.put("exclusions", "*.js,*.css,/druid/*,/jdbc/*"); bean.setInitParameters(initParams); return bean; } }
I don't know the specific role. Anyway, I know that Servlet and Filter can also be configured in SpringBoot!
3. Summary
Try to use Druid data source. Its own web page monitoring function is simple to configure and powerful. It is really very easy to use. At the same time, try to configure Servlet and Filter in SpringBoot, and dream back half a year ago.