Original title: Spring certified China Education Management Center - learn how to use spring's RESTful Web Service (spring China Education Management Center)
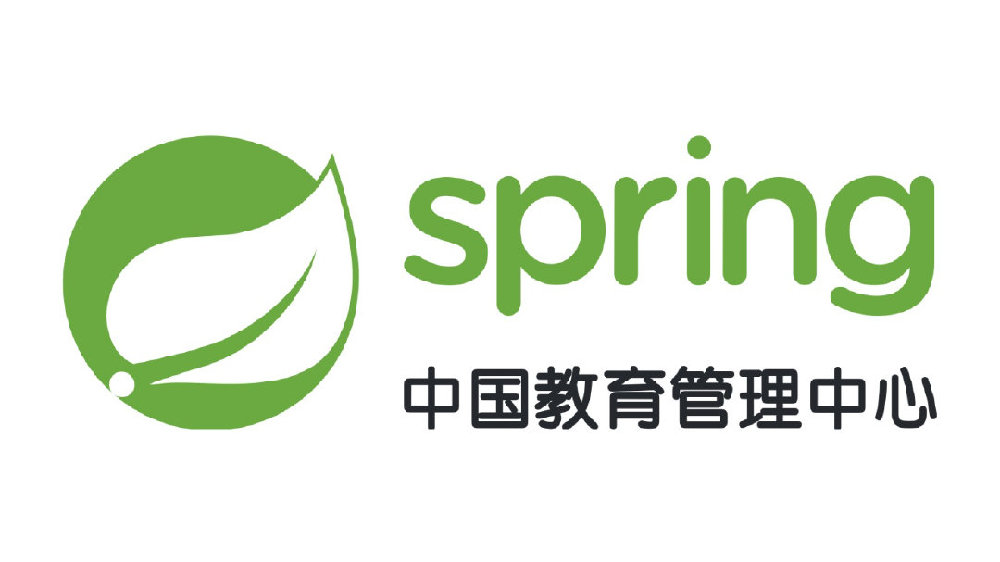
This guide will guide you through the process of creating applications that use RESTful Web services.
What will you build
You will build an application that uses SpringRestTemplate to https://quoters.apps.pcfone.io/api/random Retrieve random Spring Boot quotes.
What do you need?
- About 15 minutes
- Favorite text editor or IDE
- JDK 1.8 or later
- Gradle 4 + or Maven 3.2+
- You can also import the code directly into the IDE: spring tool kit (STS)IntelliJ IDEA
How to complete this guide
Like most Spring getting started guides, you can start from scratch and complete each step, or you can bypass the basic setup steps you are already familiar with. Either way, you'll end up with working code.
To start from scratch, continue with Spring Initializr.
To skip the basics:
- Download and unzip the source repository for this guide, or clone it using Git: git clone https://github.com/spring-guides/gs-consuming-rest.git
- The CD goes to GS consuming rest / initial
- Jump to get REST resources.
When finished, you can check the results against the code in gs-consuming-rest/complete.
Start with Spring Initializr
You can use this pre initialized project and click Generate to download the ZIP file. This project is configured to fit the examples in this tutorial.
Manually initialize the project:
- Navigate to https://start.spring.io . The service extracts all the dependencies required by the application and completes most of the settings for you.
- Select Gradle or Maven and the language you want to use. This guide assumes that you have chosen Java.
- Click Dependencies and select Spring Web.
- Click generate.
- Download the generated ZIP file, which is an archive of the Web application configured according to your choice.
If your IDE has spring initializer integration, you can complete this process from your IDE.
You can also fork the project from Github and open it in your IDE or other editor.
Get REST resources
After setting up the project, you can create a simple application that uses RESTful services.
A RESTful service is already in use https://quoters.apps.pcfone.io/api/random Build it up. It randomly takes references to Spring Boot and returns them as JSON documents.
If you request the URL through a Web browser or curl, you will receive a JSON document as follows:
{ type: "success", value: { id: 10, quote: "Really loving Spring Boot, makes stand alone Spring apps easy." } }
This is easy, but not very useful when getting through a browser or curl.
A more useful way to programmatically use REST Web services. To help you accomplish this task, Spring provides a convenient template class called resttemplate Resttemplate makes interaction with most RESTful services a one-way mantra. It can even bind this data to a custom field type.
First, you need to create a domain class to contain the data you need. The following listing shows the classes that a Quote can use as a domain class:
src/main/java/com/example/consumingrest/Quote.java
package com.example.consumingrest; import com.fasterxml.jackson.annotation.JsonIgnoreProperties; @JsonIgnoreProperties(ignoreUnknown = true) public class Quote { private String type; private Value value; public Quote() { } public String getType() { return type; } public void setType(String type) { this.type = type; } public Value getValue() { return value; } public void setValue(Value value) { this.value = value; } @Override public String toString() { return "Quote{" + "type='" + type + '\'' + ", value=" + value + '}'; } }
This simple Java class has some properties and matching getter methods. It is annotated with @ JsonIgnoreProperties from the Jackson JSON processing library, indicating that any properties not bound to this type should be ignored.
To bind your data directly to your custom type, you need to specify the variable name to be exactly the same as the key in the JSON document returned from the API. If the variable names and keys in your JSON document do not match, you can use the @ JsonProperty annotation to specify the exact key of the JSON document. (this example matches each variable name with the JSON key, so this comment is not required here.)
You also need an extra class to embed the internal reference itself. This class Value meets this requirement and is shown in the following list (at) src/main/java/com/example/consumingrest/Value.java):
package com.example.consumingrest; import com.fasterxml.jackson.annotation.JsonIgnoreProperties; @JsonIgnoreProperties(ignoreUnknown = true) public class Value { private Long id; private String quote; public Value() { } public Long getId() { return this.id; } public String getQuote() { return this.quote; } public void setId(Long id) { this.id = id; } public void setQuote(String quote) { this.quote = quote; } @Override public String toString() { return "Value{" + "id=" + id + ", quote='" + quote + '\'' + '}'; } }
This uses the same annotation, but maps to other data fields.
Complete the application
Initalizr creates a class with a main() method. The following listing shows the class (at) created by Initializr src/main/java/com/example/consumingrest/ConsumingRestApplication.java):
package com.example.consumingrest; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class ConsumingRestApplication { public static void main(String[] args) { SpringApplication.run(ConsumingRestApplication.class, args); } }
Now you need to add something else to the ConsumingRestApplication class to show references from our RESTful source. You need to add:
- A logger that sends output to the log (console in this example).
- A RestTemplate, which uses Jackson JSON processing library to process incoming data.
- A runs RestTemplate when starting CommandLineRunner (and therefore gets our quotation).
The following listing shows the completed ConsumingRestApplication class (at src/main/java/com/example/consumingrest/ConsumingRestApplication.java):
package com.example.consumingrest; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.web.client.RestTemplateBuilder; import org.springframework.context.annotation.Bean; import org.springframework.web.client.RestTemplate; @SpringBootApplication public class ConsumingRestApplication { private static final Logger log = LoggerFactory.getLogger(ConsumingRestApplication.class); public static void main(String[] args) { SpringApplication.run(ConsumingRestApplication.class, args); } @Bean public RestTemplate restTemplate(RestTemplateBuilder builder) { return builder.build(); } @Bean public CommandLineRunner run(RestTemplate restTemplate) throws Exception { return args -> { Quote quote = restTemplate.getForObject( "https://quoters.apps.pcfone.io/api/random", Quote.class); log.info(quote.toString()); }; } }
Run the application
You can run the application from the command line using Gradle or Maven. You can also build and run a single executable jar file that contains all the necessary dependencies, classes, and resources. Building executable jars can be easily used as application delivery, versioning and deployment services throughout the development life cycle, across different environments, and so on.
If you use Gradle, you can use/ gradlew bootRun. Alternatively, you can use to build JAR files/ gradlew build, and then run the} JAR file as follows:
java -jar build/libs/gs-sumption-rest-0.1.0.jar
If you use Maven, you can use/ mvnw spring-boot:run. Alternatively, you can use the build JAR file/ mvnw clean package and run the JAR file as follows:
java -jar target/gs-consumption-rest-0.1.0.jar
The steps described here create a runnable JAR. You can also build classic WAR files.
You should see output similar to the following, but with random References:
2019-08-22 14:06:46.506 INFO 42940 --- [main] cecConsumingRestApplication : Quote{type='success', value=Value{id
If you see an error displayed as, Could not extract response: no suitable HttpMessageConverter found for response type [class com.example.consumingrest.Quote] you may be in an environment where you cannot connect to the back-end service (it will send JSON if you can access it). Maybe you're behind the agency. Try http Proxyhost and HTTP Set the proxyport system property to a value appropriate for your environment.
congratulations! You just developed a simple REST client using Spring Boot.