1 - Controller
- The complex controller provides the behavior of accessing the application program, which is usually realized by interface definition or annotation definition.
- The controller is responsible for parsing the user's request and transforming it into a model.
- In Spring MVC, a Controller class can contain multiple methods. In Spring MVC, there are many ways to configure the Controller
1.1 implement Controller interface
1.1.1 project directory
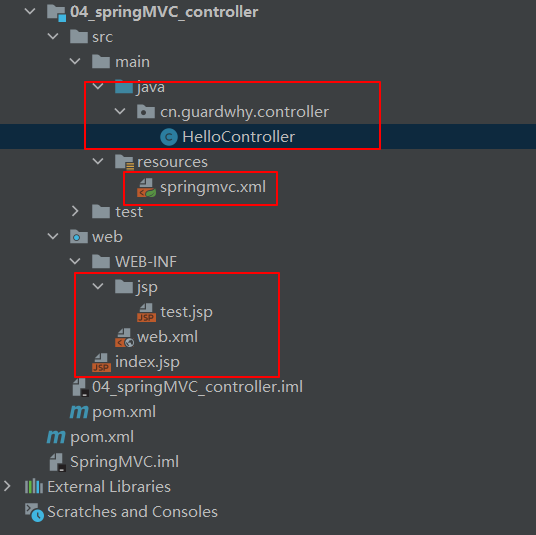
1.1.2 operation business Controller
Implement the Controller interface to obtain the Controller function
package cn.guardwhy.controller; import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.mvc.Controller; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Controller Explain in detail */ public class TestController1 implements Controller { @Override public ModelAndView handleRequest(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) throws Exception { // 1. Return a model view object ModelAndView mv = new ModelAndView(); // 2. Encapsulate the object and put it in ModelAndView. mv.addObject("msg", "TestController1"); // 3. View of package jump mv.setViewName("test"); return mv; } }
1.1.3 configuration file of spring MVC
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!--register bean,Submit class to springIOC Container to manage--> <bean id="/test1" class="cn.guardwhy.controller.TestController1"/> <!--4.view resolver --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" id="InternalResourceViewResolver"> <!--prefix--> <property name="prefix" value="/WEB-INF/jsp/"/> <!--suffix--> <property name="suffix" value=".jsp"/> </bean> </beans>
1.1.4 create view layer
Create hello.jsp in the WEB-INF/ jsp directory JSP, the view can directly take out and display the information brought back from the Controller. You can get the value or object stored in the Model through EL representation.
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>guardwhy</title> </head> <body> ${msg} </body> </html>
4.1.5 execution results
Attention
- It is an old method to implement the interface Controller and define the Controller.
- There is only one method in a Controller. If you want multiple methods, you need to define multiple controllers. The way of definition is troublesome.
1.2 using annotation @ Controller
1.2.1 operation business Controller
package cn.guardwhy.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; /** * Annotation form */ @Controller // Represents that this class will be taken over by Spring. If the return value of all methods in the annotated class is Spring // And if there is a specific page that can jump, it will be parsed by the view parser. public class TestController2 { // Map access path @RequestMapping("/test2") public String Test(Model model){ // Automatically instantiate a Model object to pass values to the view model.addAttribute("msg", "ControllerTest2"); return "test"; // It will be spliced into / / WEB-INF / / JSP / / test jsp } }
1.2.2 configuration file of spring MVC
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!-- Automatically scan the specified package and submit all the following annotation classes to IOC Container management --> <context:component-scan base-package="cn.guardwhy.controller"/> <!--4.view resolver --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" id="InternalResourceViewResolver"> <!--prefix--> <property name="prefix" value="/WEB-INF/jsp/"/> <!--suffix--> <property name="suffix" value=".jsp"/> </bean> </beans>
1.2.3 implementation results
1.3 RequestMapping
@The RequestMapping annotation is used to map URLs to a controller class or a specific handler method.
It can be used on a class or method. For a class, it means that all methods in the class that respond to requests take this address as the parent path.
1.3.1 notes on Methods
package cn.guardwhy.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class TestController3 { @RequestMapping("/test3") public String Test3(Model model){ // Automatically instantiate a Model object to pass values to the view model.addAttribute("msg", "ControllerTest3"); return "test"; } }
1.3.2 annotate classes and methods at the same time
package cn.guardwhy.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; @Controller @RequestMapping("/guardwhy") public class TestController3 { @RequestMapping("/test3") public String Test3(Model model){ // Automatically instantiate a Model object to pass values to the view model.addAttribute("msg", "ControllerTest3"); return "test"; } }
1.4 RestFul style
Restful is a style of resource positioning and resource operation. It's not a standard or agreement, it's just a style. The software designed based on this style can be more concise, more hierarchical, and easier to implement caching and other mechanisms.
1.4.1 project directory
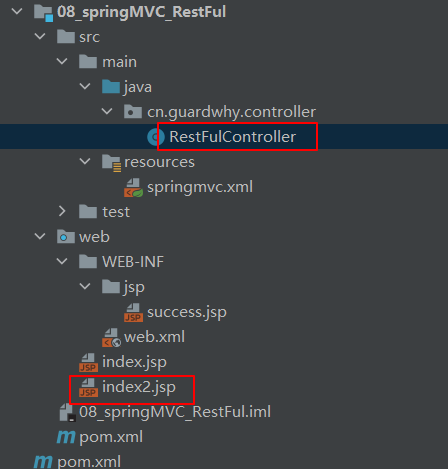
1.4.2 basic functions
Resources: all things on the Internet can be abstracted as resources.
Resource operation: use POST, DELETE, PUT and GET to operate resources with different methods. Add, DELETE, modify and query respectively.
Operating resources in traditional ways
Through different parameters to achieve different effects!!!
- http://localhost:8080/query.stu?id=1 Query, GET
- http://localhost:8080/save.stu New, POST
- http://localhost:8080/update.stu Update, POST
- http://localhost:8080/delete.stu?id=1 Delete POST
Operating resources with RESTful
Different effects can be achieved through different request methods!! Note that the request address is the same, but the function can be different!!!
- http://localhost:8080/stu/1 Query, GET
- http://localhost:8080/stu New, POST
- http://localhost:8080/stu Update, PUT
- http://localhost:8080/stu/1 DELETE delete
1.4.3 controller
In Spring MVC, you can use the @ PathVariable annotation to bind the value of the method parameter to a URL template variable.
package cn.guardwhy.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; /** * RestFul Introduction to style */ @Controller public class RestFulController { // 1. Access mapping path @RequestMapping(value = "/commit/{a}/{b}", method = RequestMethod.GET) public String test1(@PathVariable int a, @PathVariable String b, Model model){ // Return result set String res = a + b; model.addAttribute("msg", "Result return value 1:" + res); return "success"; } // post method @PostMapping("/commit/{a}/{b}") public String test2(@PathVariable int a, @PathVariable String b, Model model){ // Return result set String res = a + b; model.addAttribute("msg", "Result return value 2:" + res); return "success"; } }
View layer
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>post Submit</title> </head> <body> <form action="commit/1/6" method="post"> <input type="submit" value="Submit"> </form> </body> </html>
1.4.4 implementation results
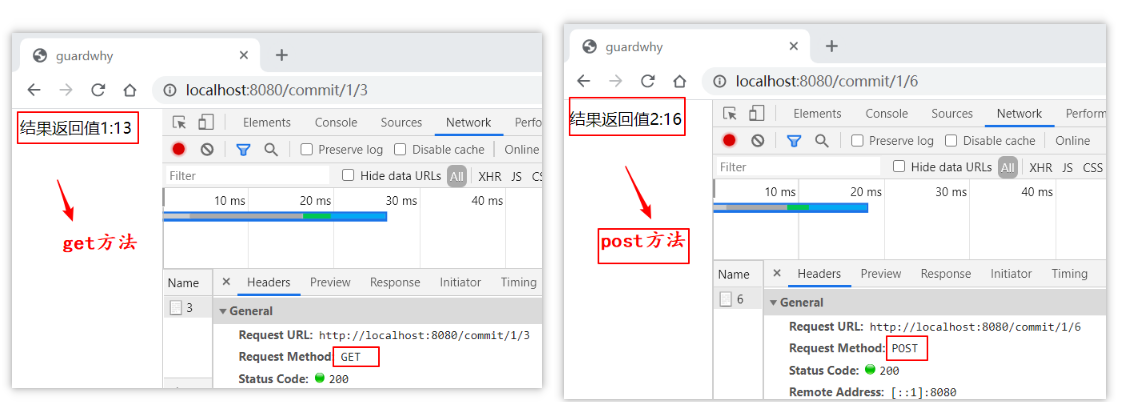
1.4.5 summary
The @ RequestMapping annotation of Spring MVC can handle HTTP requests, such as get, put, post and delete. All address bar requests will be of HTTP GET type by default.
Combined notes:
@GetMapping @PostMapping @PutMapping @DeleteMapping @PatchMapping