catalogue
1.1 spring MVC core: dispatcher servlet (central scheduler)
2. Create the first spring MVC project
2.1 create a web maven template
2.2 editing web XML file, configuring the central scheduler
Version 2.2.1 is too low, replace with a higher version
2.2.2 declare and register the DispatcherServlet, the core object of spring MVC
2.2.3 the overall code is as follows:
2.3.1 delete its index JSP, create a new index jsp
2.4 spring MVC configuration file
2.5 processing flow of spring MVC request
2.6 spring MVC execution process source code analysis
3. Small details of the first project
4.@ Detailed introduction to requestmapping
4.1 extract the common part and module name
4.2 definition of request submission method get} post
5. Parameters of processor method
5.1 accept parameters one by one
5.4 accept multiple parameters at one time (object parameter receiving)
6, Return value of processor method
1. Add dependency in pom file, no dependency found.
1. Basic concepts
Spring MVC is a framework based on spring. In fact, it is a spring module specialized in web development.
The bottom layer of web development is servlet. The framework is to add some functions on the basis of servlet to facilitate web development. It can be understood as an upgrade of servlet.
What we need to do is to use @ controller to create a controller object, put the object into the spring MVC container, and use the created object as a controller. The controller object can accept the user's request and display the processing results as a servlet.
Note: the @ converter annotation creates an ordinary class object, not a servlet. Spring MVC gives the controller object some additional functionality.
1.1 spring MVC core: dispatcher servlet (central scheduler)
The bottom layer of web development is servlet, and one object in spring MVC is servlet: dispatcher servlet (central scheduler)
DispatcherServlet: it is responsible for receiving all the user's requests. The user gives the request to the DispatcherServlet, and then the DispatcherServlet forwards the request to our Controller object. Finally, the Controller object processes the request.
2. Create the first spring MVC project
Requirements: the user initiates a request on the page, which is handed over to the controller object of spring MVC, and displays the processing result of the request
Implementation steps:
1. New web maven project
2. Add dependencies, spring webmvc dependencies, and indirectly add spring dependencies to the project, jsp and servlet dependencies
3. Key points: on the web The core object DispatcherServlet of spring MVC framework is registered in XML
1) the dispatcher servlet is called the central scheduler. It is a servlet, and its parent class inherits HttpServlet
2) dispatcher servlet is also called from controller
3) dispatcher servlet: it is responsible for receiving the request submitted by the user, calling other controller objects, and displaying the processing results of the request to the user
4. Create a page that initiates the request index jsp
5. Create controller class
1) add the @ Controller annotation on the class, create the object, and put it into the spring MVC container
2) add @ RequestMapping annotation to the method in the class
6. Create a jsp as the result and display the processing result of the request.
7. Create spring MVC configuration file (same as spring configuration file)
1) declare the component scanner and specify the package name where the @ Controller annotation is located
2) declare the view parser to help process the view
2.1 create a web maven template
2.2 editing web XML file, configuring the central scheduler
Version 2.2.1 is too low, replace with a higher version
2.2.2 declare and register the DispatcherServlet, the core object of spring MVC
2.2.3 the overall code is as follows:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--Declaration, registration springmvc Core object of DispatcherServlet--> <servlet> <servlet-name>myweb</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!--custom springMVC Location of configuration files for--> <init-param> <!--springmvc Properties of the location of the configuration file--> <param-name>contextConfigLocation</param-name> <!--Specify the location of the customization file--> <param-value>classpath:springmvc.xml</param-value> </init-param> <!--stay Tomcat After startup, create servlet object--> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>myweb</servlet-name> <!--When using frames, url-pattern Two values can be used--> <url-pattern>*.do</url-pattern> </servlet-mapping> </web-app>
2.3 requested page
2.3.1 delete its index JSP, create a new index jsp
Because of his index JSP, no coding method.
2.3.2show.jsp page
2.4 spring MVC configuration file
2.5 processing flow of spring MVC request
2.6 spring MVC execution process source code analysis
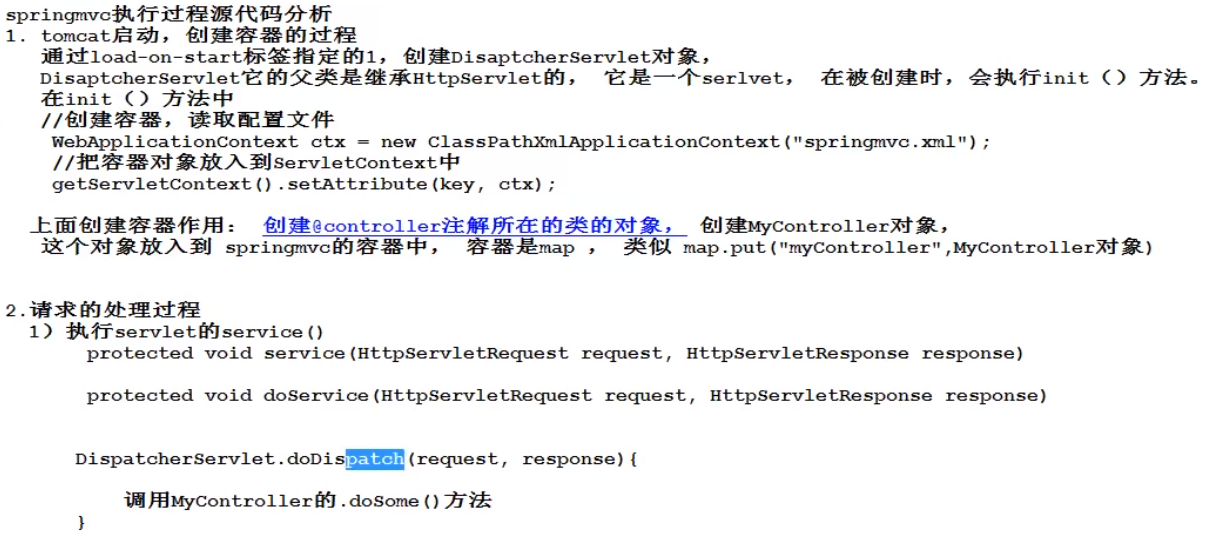
2.7 total code
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--Declaration, registration springmvc Core object of DispatcherServlet--> <servlet> <servlet-name>myweb</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!--custom springMVC Location of configuration files for--> <init-param> <!--springmvc Properties of the location of the configuration file--> <param-name>contextConfigLocation</param-name> <!--Specify the location of the customization file--> <param-value>classpath:springmvc.xml</param-value> </init-param> <!--stay Tomcat After startup, create servlet object--> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>myweb</servlet-name> <!--When using frames, url-pattern Two values can be used--> <url-pattern>*.do</url-pattern> </servlet-mapping> </web-app>
index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <p>first springmvc project</p> <p><a href="some.do">send out some.do request</a></p> </body> </html>
MyController.java
import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.servlet.ModelAndView; /** * @Controller:Create a processor (controller) object, which is placed in the spring MVC container * Location: above the class * And @ Service,@Component in spring * * Controllers (processors) can handle requests: MyController can handle requests, * It's called the back controller * */ @Controller public class MyController { /** * Spring MVC uses methods to process requests submitted by users. * Methods are user-defined, and can have multiple return values, multiple parameters, and method names. */ /** * Prepare to use the dosome method to handle some Do request. *@RequestMapping:Request mapping is used to bind a request address to a method. * A request formulates a processing method. * Attribute: 1 Value is a string indicating the URL address of the request (such as some.do). * value The value must be unique and cannot be repeated. When using, it is recommended to start with "/" * Location: 1 In the method above, commonly used. * 2.Above the class. * Note: the method modified by RequestMapping is called processor method or controller method. * The method decorated with @ RequestMapping can handle requests, similar to doGet(),doPost(), in servlet; * * Return value: ModelAndView indicates the processing result of this request * Model:Data, the data to be displayed to the user after the request processing is completed * View:Views, such as jsp and so on. */ @RequestMapping(value="/some.do") public ModelAndView dosome(){ //Doget() -- service request processing //Process some I asked. It is equivalent to that the service call processing is completed. ModelAndView mv=new ModelAndView(); //Add data, and the framework puts the data into the send request scope at the end of the request //request.setAttribute("msg", "welcome to spring MVC for web development"); mv.addObject("msg","Welcome springMVC do web development"); mv.addObject("fun","The implementation is dosome method"); //Make the view, make the full path of the view //Forward operation performed by the framework on the view, request getRequestDispather("/shoe.jsp"). forward(...) mv.setViewName("/show.jsp"); //Return mv return mv; } }
show.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <h3>show.jsp from request Get data from scope</h3><br/> <h3>msg Data: ${msg}</h3><br/> <h3>fun Data: ${fun}</h3> </body> </html>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!--Declaration component scanner--> <context:component-scan base-package="com.lin.controller"/> </beans>
The first spring MVC project was completed
Scatter flowers
3. Small details of the first project
3.1 if you directly enter show in the browser The JSP address can access this page, but it is not from some For the request initiated by do, this page has no data, so this access is meaningless. Then, we don't want the customer to enter the website in the website bar to access it and make it invisible. We put this page in the WEB-INF directory and build a subdirectory under the WEB-INF directory. It can be built or not. What is under the WEB-INF is inaccessible to users.
3.2 configuring the view parser
4.@ Detailed introduction to requestmapping
4.1 extract the common part and module name
/** * @RequestMapping: * value:The common part of all request addresses is called the module name * Location: on top of the class */
4.2 definition of request submission method get} post
/** * @RequestMapping:Request mapping * Property: method indicates the method of the request. Its value is the enumeration value of the RequestMethod class * Request method, for example, request. get GET * post Request method, requestmethod POST * * If it is different from the specified method, an error will be reported: HTTP status 405 - method not allowed * If the request method is not specified, there is no limit. Both post and get can be used */
5. Parameters of processor method
5.1 accept parameters one by one
5.2 Chinese garbled code
When submitting request parameters, the Chinese version of the get request method is not garbled; If you submit a request in post mode, there is garbled code in Chinese. You need to use a filter to deal with the problem of garbled code.
Filters can be customized, or you can use the filter characterencoding filter provided in the framework
The configuration code is as follows:
<filter> <filter-name>characterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <!--Sets the character encoding used in the project--> <init-param> <param-name>encoding</param-name> <param-value>utf-8</param-value> </init-param> <!--Force request object(HttpServletRequest)use encoding Encoded value--> <init-param> <param-name>forceRequestEncoding</param-name> <param-value>true</param-value> </init-param> <!--Forced response object(HttpServletResponse)use encoding Encoded value--> <init-param> <param-name>forceResponseEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>characterEncodingFilter</filter-name> <!--/*Means to force all requests to be processed through the filter first--> <url-pattern>/*</url-pattern> </filter-mapping>
5.3 the parameter name in the request is different from the formal parameter name in the method in the processor
Use @ RequestParam annotation
5.4 accept multiple parameters at one time (object parameter receiving)
Create a student Java class with properties and get and set methods.
6, Return value of processor method
Errors encountered
1. Add dependency in pom file, no dependency found.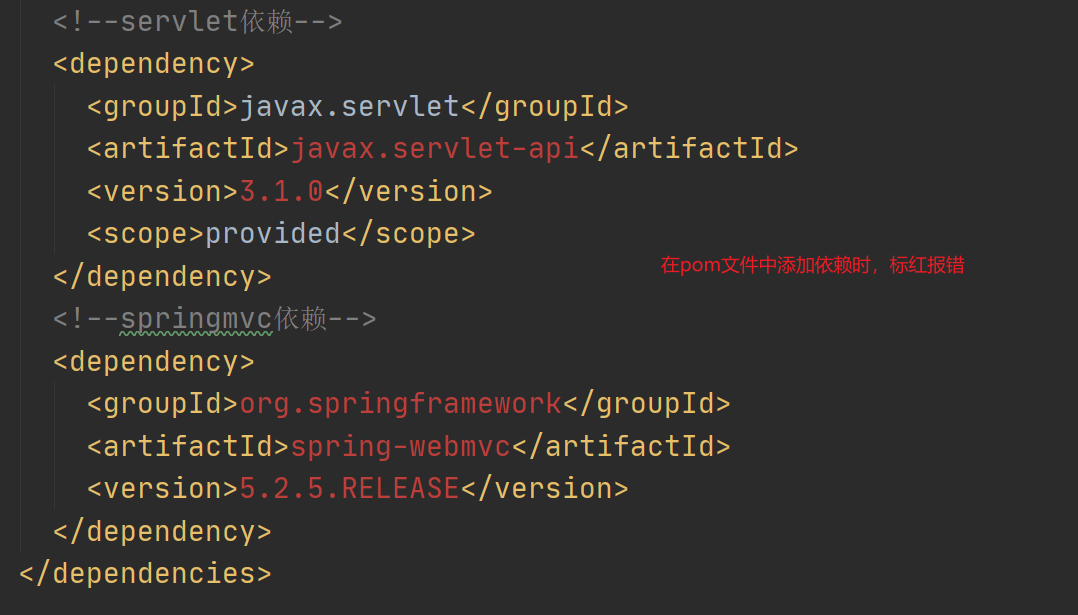
Solution:
Some references of the article: https://blog.csdn.net/weixin_40350981/article/details/109645897