The basic steps for using a JDBC transaction are as follows:
@Test public void testTX(){ String url = "jdbc:mysql://127.0.0.1:3306/test"; String username = "root"; String password = "1234"; String sourceUserId = "leo"; String desUserId = "xnn"; int money = 500; Connection connection = null; try { //1. Load database driver Class.forName("com.mysql.jdbc.Driver"); //Get database connection connection = DriverManager.getConnection(url, username, password); //Open transaction connection.setAutoCommit(false);//If true, the sql statements will be executed separately to modify the database; If false, the transaction is activated //Multiple data operation data Statement sql = connection.createStatement(); sql.executeUpdate("UPDATE user_info SET balance = balance-" + money + " WHERE user_id = '" + sourceUserId+"'"); sql.executeUpdate("UPDATE user_info SET balance = balance+" + money + " WHERE user_id = '" + desUserId+"'"); //Commit transaction connection.commit(); } catch (SQLException e) { e.printStackTrace(); try{ //RollBACK connection.rollback(); }catch (SQLException ex){ } } catch (ClassNotFoundException e) { e.printStackTrace(); } finally { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } }
From the code, we can see the disadvantages of JDBC transactions:
1. Lengthy and repetitive 2 Transaction control needs to be displayed 3 Need to display and handle checked exceptions
Moreover, JDBC only provides basic API support to complete transaction operations. By operating JDBC, we can put multiple sql statements into the same transaction to ensure ACID characteristics. However, when cross database and cross table sql is encountered, simple JDBC transactions cannot be satisfied. Based on this problem, JTA transactions occur
JTA transaction
JTA(Java Transaction API) provides the transaction management capability of cross database connection (or other JTA resources). JTA transaction management is implemented by JTA container, and the transaction communication between transaction manager and application program, resource manager and application server in J2ee framework
Composition of JTA
There are several important concepts in JTA:
1). The high-level application transaction definition interface allows transaction clients to define transaction boundaries
2). The standard Java mapping of X / open XA protocol (a standardized interface between resources), which enables transactional resource managers to participate in transactions controlled by external transaction managers
3). The high-level transaction manager interface allows applications to define the boundaries of transactions for the programs they manage
Important interfaces in JTA
The important interfaces in JTA are mainly located in javax Transaction package
1).UserTransaction: enables the application to control the start, suspend, commit and rollback of transactions, which is called by java or ejb components
2). Transaction manager: used to manage transaction status of application service
3).Transaction: used to control the execution of transaction operations
4).XAResource: used to coordinate the work of transaction manager and resource manager in distributed transaction environment
5).XID: java mapping id used to mark the transaction
Note that the first three interfaces only exist in Java EE Jar does not exist in javaSe.
Basic steps of JTA transaction programming
Let's look at the basic steps of using JTA transactions to complete a simple JTA transaction process through simple java coding:
//Configure JTA transactions and establish corresponding data sources //1. Create transaction: start a transaction by creating an instance of UserTransaction class Context ctx = new InitialContext(p) ; UserTransaction trans = (UserTransaction) ctx.lookup("javax. Transaction.UserTransaction"); //Start transaction trans.begin(); //Find the data source and bind it DataSource ds = (DataSource) ctx.lookup("mysqldb"); //Establish database connection Connection mycon = ds.getConnection(); //sql operation performed stmt.executeUpdate(sqlS); //Commit transaction trans.commit(); //Close connection mycon.close();
Advantages and disadvantages of JTA transactions
It can be seen that JTA has obvious advantages. It provides a distributed transaction solution and performs strict ACID operations. However, standard JTA transactions are not commonly used in daily development. The reason is due to the shortcomings of JTA. For example, the implementation of JTA is quite complex, and JTA UserTransaction needs to be obtained from JNDI, That is, if we want to implement JTA, we generally also need to implement JNDI, and JTA is just a simple container, which is complex to use, and it is difficult to realize code reuse under flexible requirements. Therefore, we need a framework that can give us a perfect container transaction operation
Spring transaction and transaction abstraction
Spring encapsulates a set of transaction mechanism, provides perfect transaction abstraction, abstractly divides the steps required by transactions, and provides a standard API programmatically, as follows:
try{ //1. Start transaction //2. Execute database operation //3. Submission of services }catch(Exception ex){ //Handling exceptions //4. Rollback transaction }finally{ //Close the connection and clean up the resources }
Transaction abstraction in Spring
Spring's abstract transaction model is based on the interface PlatformTransactionManager, which has different implementations. Each implementation has a corresponding specific data access technology, which is generally as follows:
It can be seen that Spring does not provide a complete transaction operation Api, but provides a variety of transaction managers, which trust the transaction responsibilities to Hibernate, JTA and other persistence mechanism platform frameworks, and only provides a general transaction manager interface - org springframework. transaction. Platformtransactionmanager also provides corresponding transaction managers for each persistent transaction platform framework to limit its general behavior, but the specific transaction implementation will be implemented by each platform itself:
Public interface PlatformTransactionManager{ // The TransactionStatus object is obtained from the TransactionDefinition TransactionStatus getTransaction(TransactionDefinition definition) throws TransactionException; // Submit Void commit(TransactionStatus status) throws TransactionException; // RollBACK Void rollback(TransactionStatus status) throws TransactionException; }
Using individual transactions in Spring
Next, let's take a look at how to use Spring transaction abstraction to use various transaction platforms:
Spring JDBC transactions
If JDBC is directly used for persistence in the application, you can use DataSourceTransactionManager to handle the transaction boundary, while using DataSourceTransactionManager, you need to assemble it into the application context using xml configuration:
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource" /> </bean>
After configuration, in the JDBC instance, the DataSourceTransactionManager calls Java sql. Connection to manage transactions. After the SQL method is executed, it will automatically call the commit() method of the connection to commit transactions. Similarly, if an exception occurs during execution, it will trigger the call of rollback() method to rollback.
Hibernate transaction
If the persistence of the application is realized through Hibernate, you need to use Hibernate transaction manager. For Hibernate 3 and above, you need to add the following Bean declaration in the Spring context definition:
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean>
Java persistent Api transactions – JPA
In fact, the implementation of JPA specification comes from Hibernate. If you want to use JPA transactions, you need to use JPA transaction manager to manage transactions. The configuration is as follows:
# summary Here, due to the interview MySQL I asked a lot, so I'm here MySQL Take as an example to summarize and share. But you often have to learn more than this, as well as the use of some mainstream frameworks, Spring Learning the source code, Mybatis The learning of source code and so on need to be mastered, and I have sorted out these knowledge points **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** 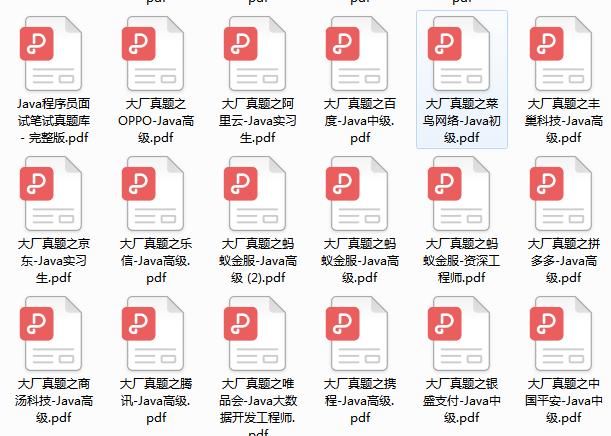 use, Spring Learning the source code, Mybatis The learning of source code and so on need to be mastered, and I have sorted out these knowledge points **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** [External chain picture transfer...(img-hraNjunx-1630391856798)] 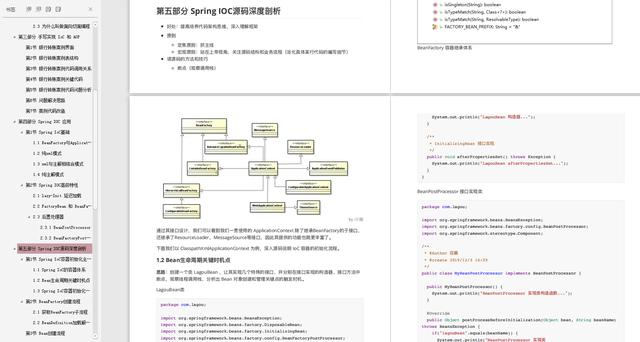