-
If objects implement both the InitializingBean and the common initialization methods provided, the order of execution?
Answer: InitializingBean is executed first, then general initialization method is executed.
-
The injection must occur before the initialization operation.
-
What exactly is an initialization operation?
Initialization of resources: database, IO, network,...
Destruction phase - DisposableBean, destroy-method
Before Spring destroys an object, it calls the object's destroy method to complete the destroy operation.
When does Spring destroy the created object? ctx.close();
Destruction methods are provided: Programmers define the destruction method and complete the destruction operation based on business needs
Destroy method call: Spring factory calls.
The development process, like the initialization operation, provides two methods of destruction:
- DisposableBean interface:
public class Product implements DisposableBean { // Programmers define destruction methods and complete the destruction according to their needs @Override public void destroy() throws Exception { System.out.println("Product.destroy"); } }
- The object provides a common destroy method, the configuration file type configures destroy-method:
public class Product { // Programmers define destruction methods and complete the destruction according to their needs public void myDestory() { System.out.println("Product.myDestory"); } }
* * * **Detailed analysis of the destruction phase**: 1. Destroy method operation is only applicable `scope="singleton"`,Initialization works. 2. What exactly is a destruction operation? Release of resources:`io.close()`,`connection.close()`,... [](https://Gitee. Summary of the life cycle of com/vip204888/java-p7) objects ----------------------------------------------------------------------------
public class Product implements InitializingBean, DisposableBean {
private String name; public String getName() { return name; } public void setName(String name) { System.out.println("Product.setName"); this.name = name; } Product() { // Establish System.out.println("Product.Product"); } // The programmer completes the initialization according to the method implemented by the requirements public void myInit() { System.out.println("Product.myInit"); } // The programmer completes the initialization according to the method implemented by the requirements @Override public void afterPropertiesSet() throws Exception { System.out.println("Product.afterPropertiesSet"); } public void myDestory() { System.out.println("Product.myDestory"); } // Programmers define destruction methods and complete the destruction according to their needs @Override public void destroy() throws Exception { System.out.println("Product.destroy"); }
}
<bean id="product" class="com.yusael.life.Product" init-method="myInit" destroy-method="myDestory"> <property name="name" value="yusael"/> </bean> ``` 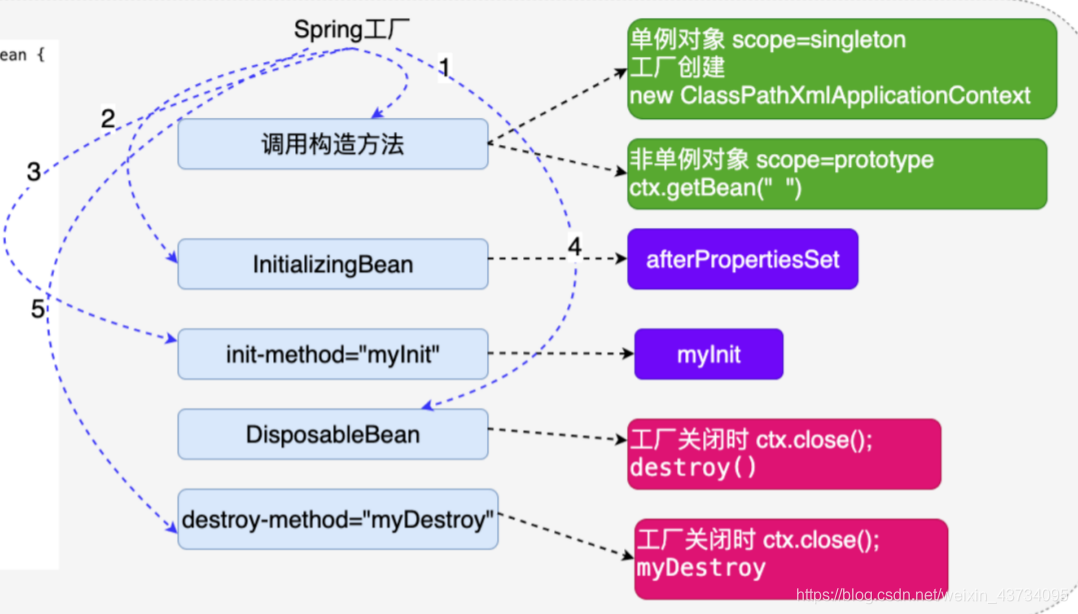 [](https://gitee.com/vip204888/java-p7) Profile parameterization ========================================================================== **Profile parameterization**: hold Spring The string information in the configuration file that needs to be modified frequently is transferred to smaller configuration files. 1. Spring Are there strings in the configuration file that need to be modified frequently? Existing: Parameters related to database connection... 2. Changing strings frequently, in Spring Direct modification in the configuration file is detrimental to project maintenance (modification) 3. Transfer to a small configuration file (.properties)Favorable for maintenance (modification) Advantages: Benefits Spring Maintenance (modification) of configuration files [](https://gitee.com/vip204888/java-p7) Steps for developing configuration file parameters ------------------------------------------------------------------------------ * Provide a small configuration file (.properities) Name: No requirement Placement: No requirement ``` jdbc.driverClassName = com.mysql.jdbc.Driver jdbc.url = jdbc:mysql://localhost:3306/spring?useSSL=false jdbc.username = root jdbc.password = 1234 ``` * Spring Configuration files are integrated with small configurations: ``` <!--Spring Configuration Files and_Configuration Files Integration--> <!--resources The following files will be placed after the entire program has been compiled classpath In the directory, src.main.java The files in are also--> <context:property-placeholder location="classpath:/db.properties"/> ``` stay Spring Configuration file passed through `${key}` Get the corresponding value in the small configuration file: ``` <bean id="conn" class="com.yusael.factorybean.ConnectionFactoryBean"> <property name="driverClassName" value="${jdbc.driverClassName}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </bean> ``` [](https://gitee.com/vip204888/java-p7) Custom Type Converter =========================================================================== [](https://gitee.com/vip204888/java-p7) Type Converter ------------------------------------------------------------------------ Effect: Spring The injection is completed by converting the string type data in the configuration file to the corresponding type data of the member variables in the object through a type converter. 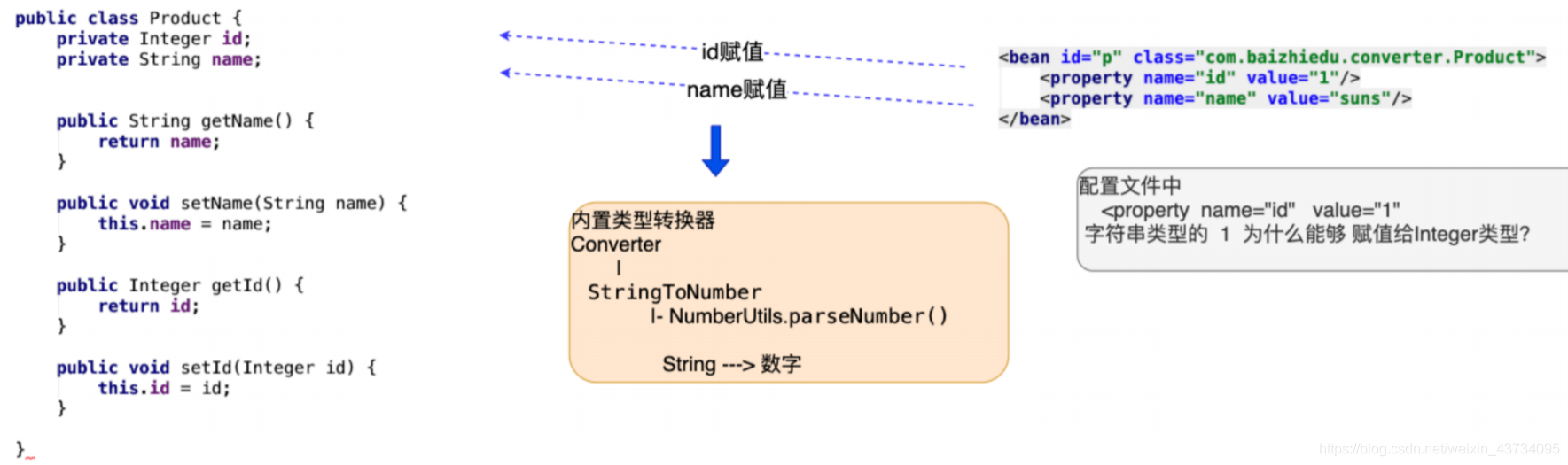 [](https://gitee.com/vip204888/java-p7) Custom Type Converter --------------------------------------------------------------------------- Cause: When Spring When there is no specific type converter available internally and the programmer needs to use it in the application, the programmer needs to define the type converter himself. **\[Development steps\]**: * class implements Converter Interface ``` public class MyDateConverter implements Converter<String, Date> { /* convert Method action: String ---> Date SimpleDateFormat sdf = new SimpleDateFormat(); sdf.parset(String) ---> Date Parameters: source : Represents the date string <value>2020-10-11</value>in the configuration file return : When the converted Date is used as the return value of the convert method, Spring _Move to inject (assign) the birthday attribute */ @Override public Date convert(String source) { Date date = null; try { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); date = sdf.parse(source); } catch (ParseException e) { e.printStackTrace(); } return date; } } ``` * stay Spring Configure in the configuration file; Create first `MyDateConverter` Object, then register the type converter; ``` <!--Establish MyDateConverter object--> <bean id="myDateConverter" class="com.yusael.converter.MyDateConverter"/> <!--Used to register type converters--> <bean id="conversionService" class="org.springframework.context.support.ConversionServiceFactoryBean"> <property name="converters"> <set> <ref bean="myDateConverter"/> </set> </property> </bean> <bean id="good" class="com.yusael.converter.Good"> <property name="name" value="zhenyu"/> <property name="birthday" value="2012-12-12"/> </bean> ``` [](https://gitee.com/vip204888/java-p7) Custom Type Converter Details ----------------------------------------------------------------------------- * `MyDateConverter` In**Format of date**,adopt **Dependent Injection** The assignment is done by the configuration file. ``` public class MyDateConverter implements Converter<String, Date> { private String pattern; @Override public Date convert(String source) { Date date = null; try { SimpleDateFormat sdf = new SimpleDateFormat(pattern); date = sdf.parse(source); } catch (ParseException e) { e.printStackTrace(); } return date; } public String getPattern() { return pattern; } public void setPattern(String pattern) { this.pattern = pattern; } }
<property name="pattern" value="yyyy-MM-dd"/>
* `ConversionSeviceFactoryBean` Definition id Property, value must be `conversionService`;
General Catalog Display
The note has eight nodes (shallow to deep) and is divided into three modules.
High performance. Secondary killing involves a large number of concurrent reads and writes, so supporting high concurrent access is critical. This note will focus on four aspects: static and dynamic separation of design data, hot spot discovery and isolation, peak clipping and hierarchical filtering of requests, and ultimate optimization of service side.
Uniformity. The way goods are reduced in stock in seconds killing is also critical. It can be imagined that a limited number of goods are requested to reduce the inventory at the same time many times. Reducing the inventory is divided into "take down the inventory", "pay down the inventory" and "withholding". In the process of large concurrent updates, the accuracy of the data should be guaranteed, and its difficulty can be imagined. Therefore, a node will be dedicated to the design of a second-kill inventory plan.
High availability. Although a lot of extreme optimization ideas have been introduced, there are always some situations in reality that we can't take into account, so to ensure the high availability and correctness of the system, we need to design a PlanB to cover the bottom so that we can still cope with the worst case. At the end of your notes, you'll be asked to think about what links you can use to design your pocket plan.
The space is limited, so it is impossible to show each module in detail (all of these points are collected in this Top Tutorial of High Concurrent Secondary Killing). If you feel you need it, please forward it (to help more people see yo!) Click here to get a free download!!
Because there is too much content, only part of it is intercepted here. Need a partner for this Top Tutorial of High Concurrency Secondary Kills, please share your support (can help more people see yo!)
Be requested many times at the same time to reduce inventory, inventory reduction is divided into "take down inventory", "pay down inventory" and withholding several kinds, in the process of large concurrent updates to ensure the accuracy of data, its difficulty is imaginable. Therefore, a node will be dedicated to the design of a second-kill inventory plan.
High availability. Although a lot of extreme optimization ideas have been introduced, there are always some situations in reality that we can't take into account, so to ensure the high availability and correctness of the system, we need to design a PlanB to cover the bottom so that we can still cope with the worst case. At the end of your notes, you'll be asked to think about what links you can use to design your pocket plan.
The space is limited, so it is impossible to show each module in detail (all of these points are collected in this Top Tutorial of High Concurrent Secondary Killing). If you feel you need it, please forward it (to help more people see yo!) Click here to get a free download!!
[img-bzJ2mprc-1628599982452)]
[img-H5qneUlF-1628599982456)]
Because there is too much content, only part of it is intercepted here. Need a partner for this Top Tutorial of High Concurrency Secondary Kills, please share your support (can help more people see yo!)