SpringBoot-06 employee management system
1, Environment construction
1. Create a new SpringBoot project
The specific steps are shown in the figure:
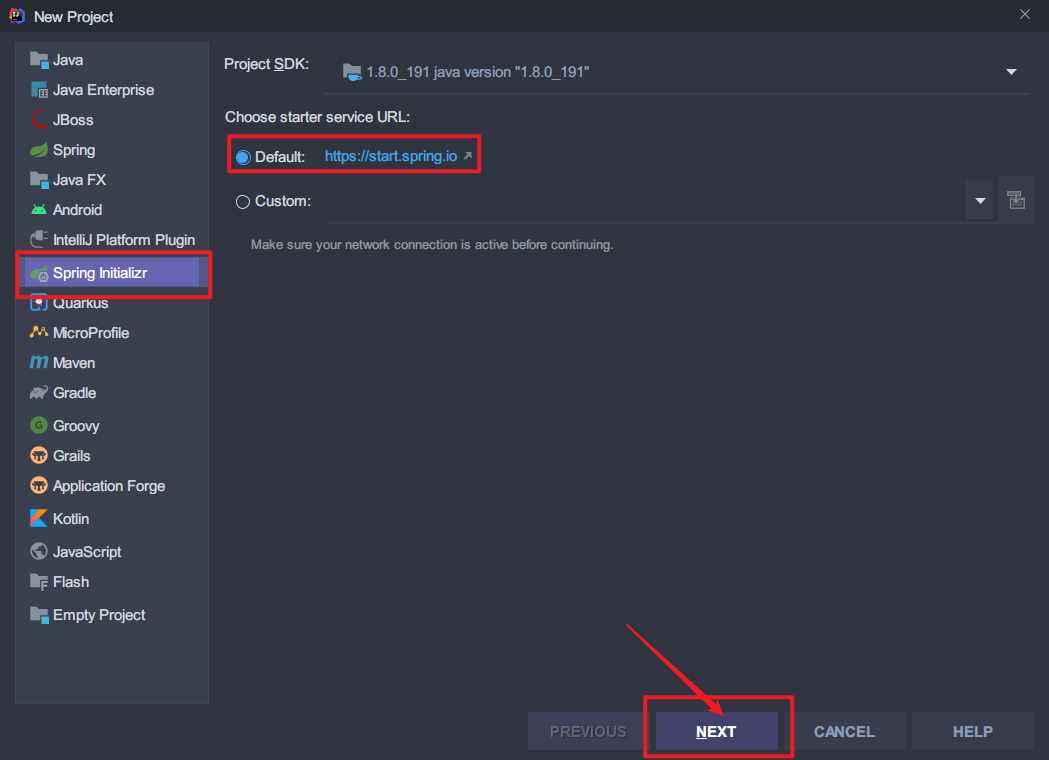
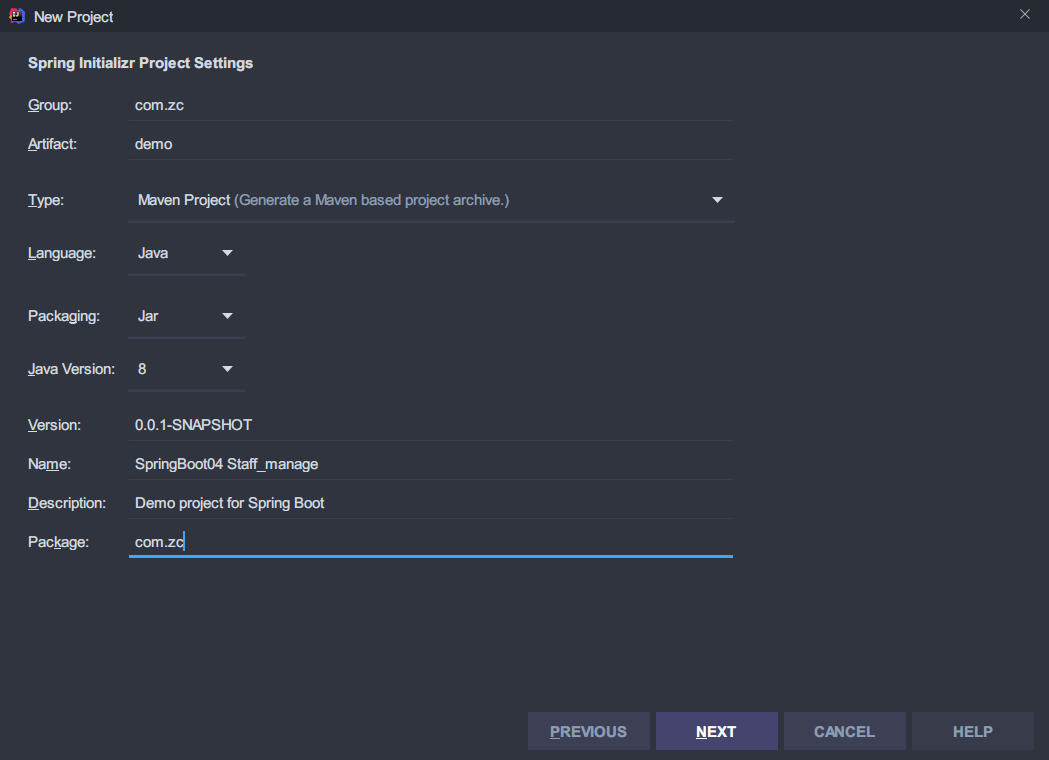
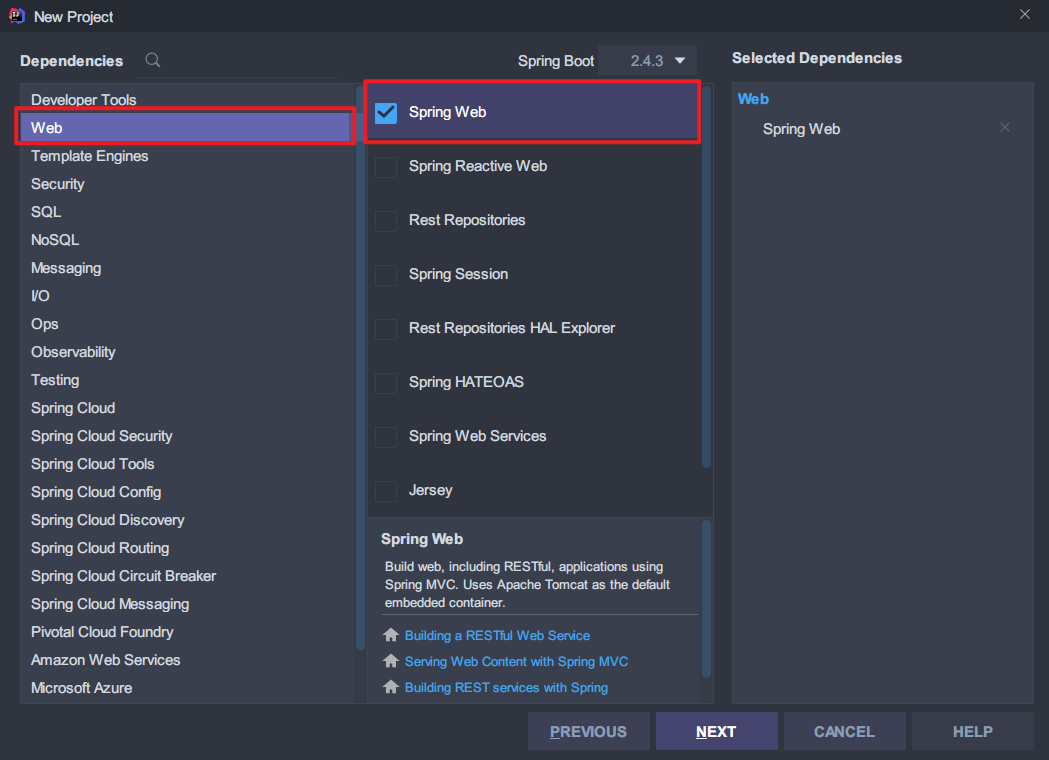
After that, the project name is given, and then the creation is completed.
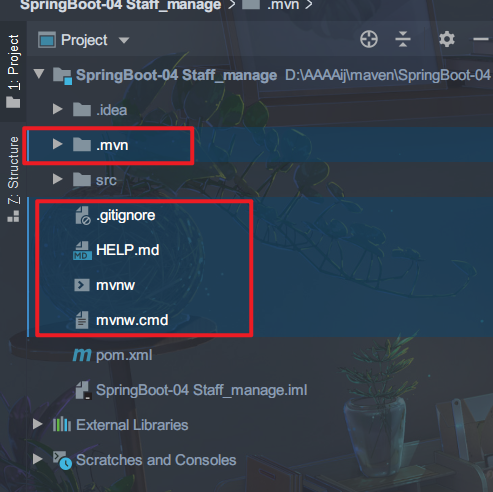
The in the red box can be deleted by yourself. It's OK not to delete it.
2. Import static resources
**Static resource file:** https://pan.baidu.com/s/1xjkUFp0ke73tUxM6SUJaSw
**Extraction code: * * m2yf
Or you can download a static resource file yourself:
**BootStrap static template:** https://getbootstrap.com/docs/4.0/examples/
- Put the html file in the templates folder
- Put the css, js and img folders in assets into the static folder (or public and resources, depending on the mood)
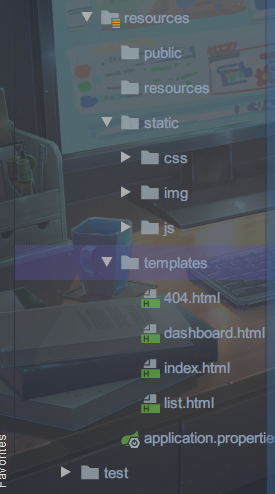
3. Simulation database
When creating pojo, dao and Controller packages, remember to create them under the same level directory of the initiator.
- pojo entity class as database table
- dao implementation class is used to add raw data and database operations.
3.1 pojo entity class
Department class, Department:
//Department table public class Department { private Integer id; //Department id private String departmentName; //Department name // Parametric / nonparametric method // Get/Set method // toString() method }
Employee:
//Employee table public class Employee { private Integer id; //Employee id private String lastName; //Employee name private String email; //Employee email private Integer gender; //Employee gender 0 female 1 male private Department department; //Employee Department private Date birth; //Employee birthday // Parametric / nonparametric method // Get/Set method // toString() method }
Here, you need to pay attention to the employee's birthday. You can let him create it himself in the entity class:
// Delete date from parameter public Employee(Integer id, String lastName, String email, Integer gender, Department department) { this.id = id; this.lastName = lastName; this.email = email; this.gender = gender; this.department = department; this.birth = new Date(); //Default creation date }
Of course, you can also write new Date() in the corresponding position of date every time you add data later;
3.2 dao implementation class
Department dao class, DepartmentDao:
@Repository //Register into the Spring container public class DepartmentDao { //Simulate data in database private static Map<Integer, Department> departments = null; static { departments = new HashMap<>(); //Create department table departments.put(101,new Department(101,"Teaching Department")); departments.put(102,new Department(102,"Marketing Department")); departments.put(103,new Department(103,"Teaching and Research Department")); departments.put(104,new Department(104,"Operation Department")); departments.put(105,new Department(105,"Logistics Department")); } //Database operation //Get all department information public Collection<Department> getDepartments(){ return departments.values(); } //Get department by id public Department getDepartment(Integer id){ return departments.get(id); } }
Employee dao, EmployeeDao:
@Repository public class EmployeeDao { //Simulation database private static Map<Integer, Employee> employees = null; @Autowired private DepartmentDao departmentDao; static { employees = new HashMap<Integer, Employee>(); employees.put(1001,new Employee(1001,"AA","1@qq.com",1,new Department(101,"Teaching Department"))); employees.put(1002,new Employee(1002,"BB","2@qq.com",0,new Department(102,"Marketing Department"))); employees.put(1003,new Employee(1003,"CC","3@qq.com",1,new Department(103,"Teaching and Research Department"))); employees.put(1004,new Employee(1004,"DD","4@qq.com",1,new Department(104,"Operation Department"))); employees.put(1005,new Employee(1005,"EE","5@qq.com",0,new Department(105,"Logistics Department"))); } //Primary key auto increment private static Integer id=1006; //Data operation //Add an employee public void save(Employee employee){ if (employee.getId()==null){ employee.setId(id++); } employee.setDepartment(departmentDao.getDepartment(employee.getDepartment().getId())); employees.put(employee.getId(),employee); } //Query all employee information public Collection<Employee> getEmployees(){ return employees.values(); } //Query employees by id public Employee getEmployee(Integer id){ return employees.get(id); } //Delete employee public void delEmployee(Integer id){ employees.remove(id); } }
Above, the environment construction is completed.
2, Home page and front-end path rewriting
1. Let's go first http://localhost:8080/ This path can directly access index html
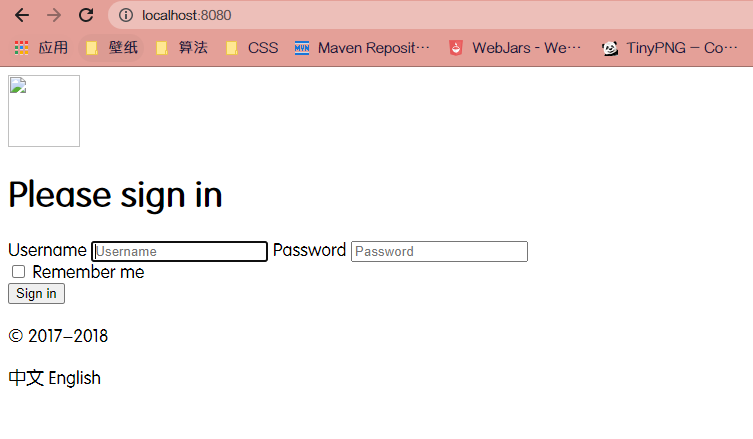
At this time, you can see that the web page is accessed normally, but the static resources are not loaded.
2. Load static resources
<html lang="en" xmlns:th="http://www.thymeleaf.org">
-
Add thymeleaf header file in the header of html file
-
Change the tag of the static resource of the html page to th:xxx
-
Loading path rules: @ {xxx}
<link th:href="@{/css/bootstrap.min.css}" rel="stylesheet"> <link th:href="@{/css/signin.css}" rel="stylesheet"> <img class="mb-4" th:src="@{/img/bootstrap-solid.svg}" alt="" width="72" height="72">
The last step after: turn off the template engine cache
spring.thymeleaf.cache=false
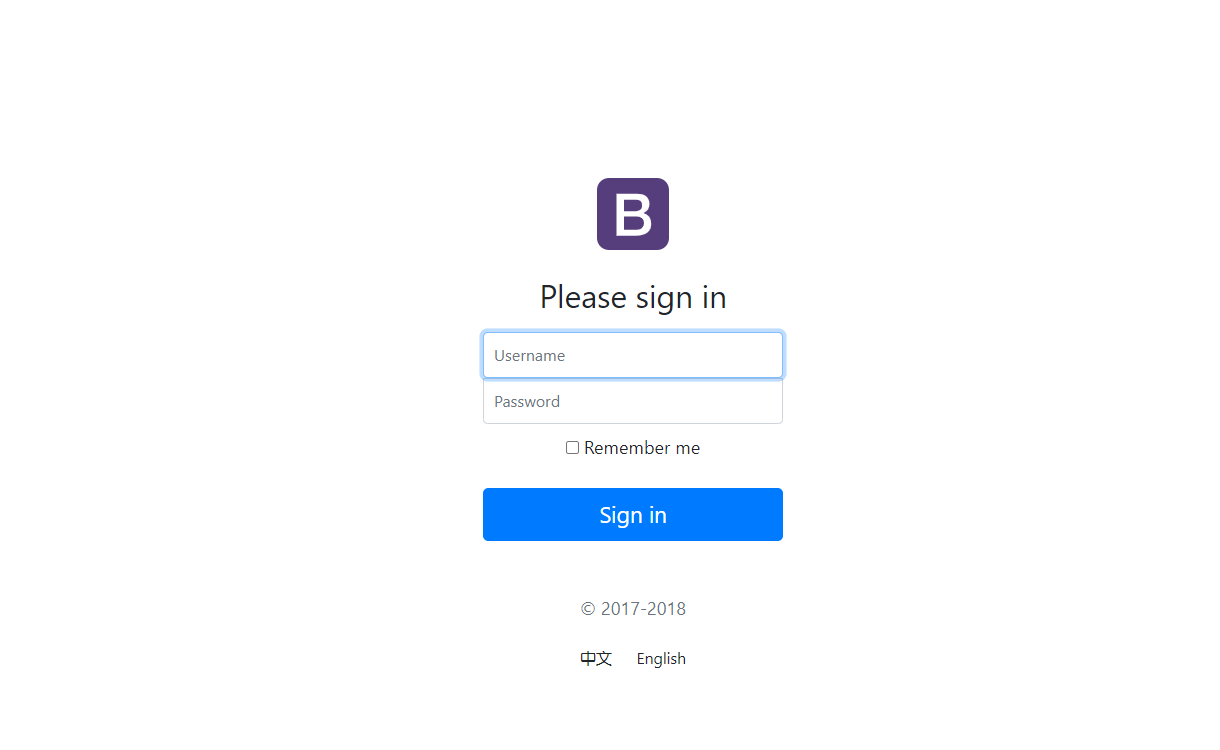
Then rewrite all the front-end pages.
3, Page internationalization
First, make sure your project is UTF-8:
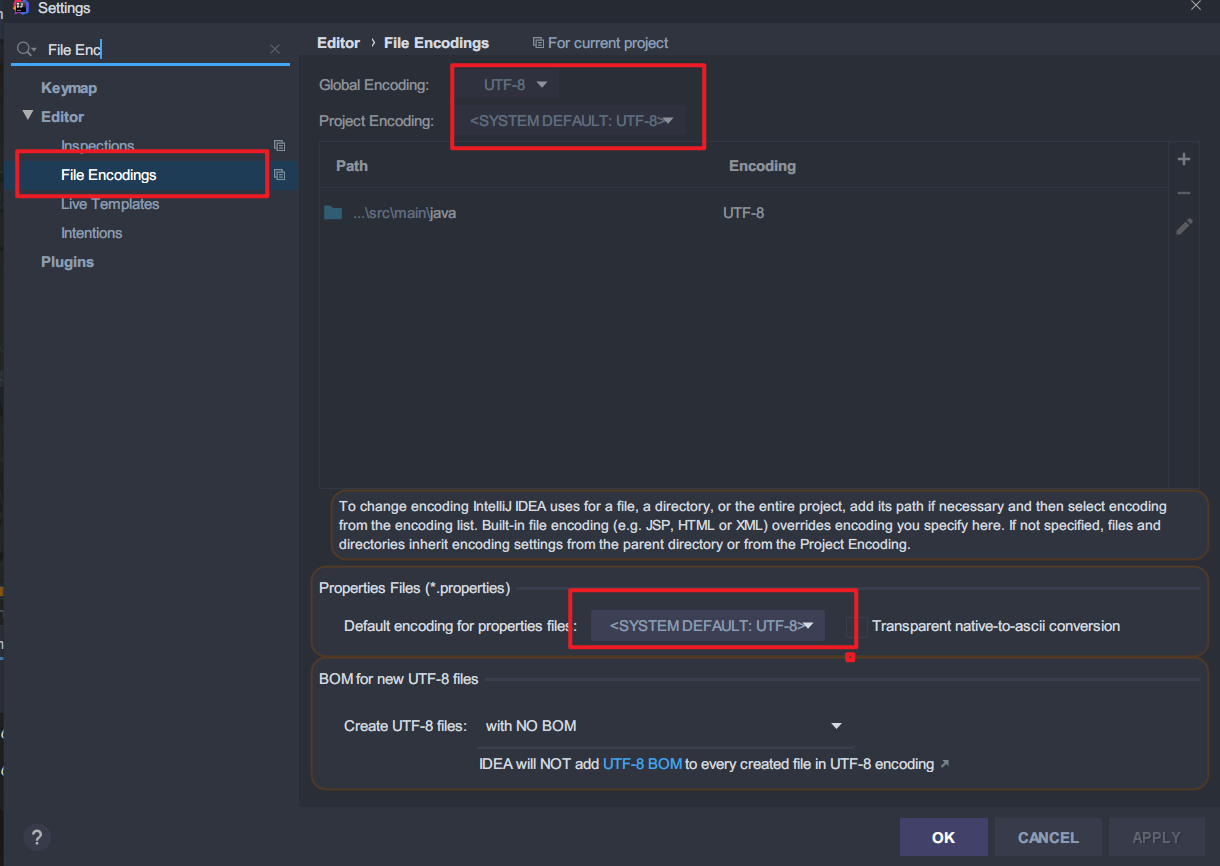
3.1 realize the following browser language switching
1. Create an i18n folder in resources and create login in the middle Properties (default language), login_ zh_ CN. Properties (Chinese), * * login_ en_ US. Properties (English) * * [create directly and merge automatically]
2. To configure the language, you can select one file, one file input, or visual input
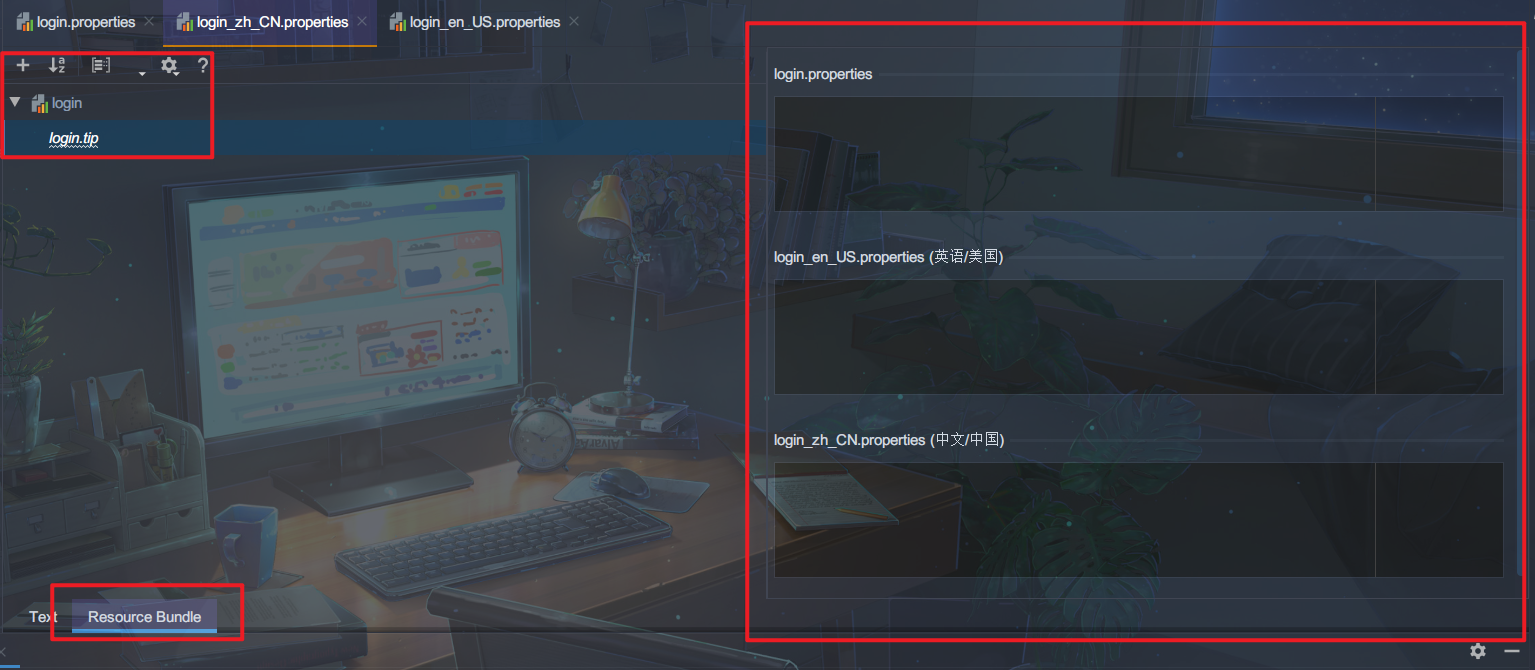
login.password=password login.tip=Please login login.remember=Remember me login.btn=Sign in login.username=user name
login.password=password login.tip=Please sign in login.remember=Remember me login.btn=Sign in login.username=username
3. Open login under internationalization i18n
spring.messages.basename=i18n.login
4. Modify the html file to index html as an example
For international information, use #{xxxx}
<body class="text-center"> <form class="form-signin" action="dashboard.html"> <img class="mb-4" th:src="@{/img/bootstrap-solid.svg}" alt="" width="72" height="72"> <h1 class="h3 mb-3 font-weight-normal" th:text="#{login.tip}">Please sign in</h1> <label class="sr-only" th:text="#{login.username}">Username</label> <input type="text" class="form-control" th:placeholder="#{login.username}" required="" autofocus=""> <label class="sr-only" th:text="#{login.password}">Password</label> <input type="password" class="form-control" th:placeholder="#{login.password}" required=""> <div class="checkbox mb-3"> <label> <input type="checkbox" value="remember-me" th:text="#{login.remember}"> </label> </div> <button class="btn btn-lg btn-primary btn-block" type="submit" >[[#{login.btn}]]</button> <p class="mt-5 mb-3 text-muted">© 2017-2018</p> <a class="btn btn-sm">chinese</a> <a class="btn btn-sm">English</a> </form> </body>
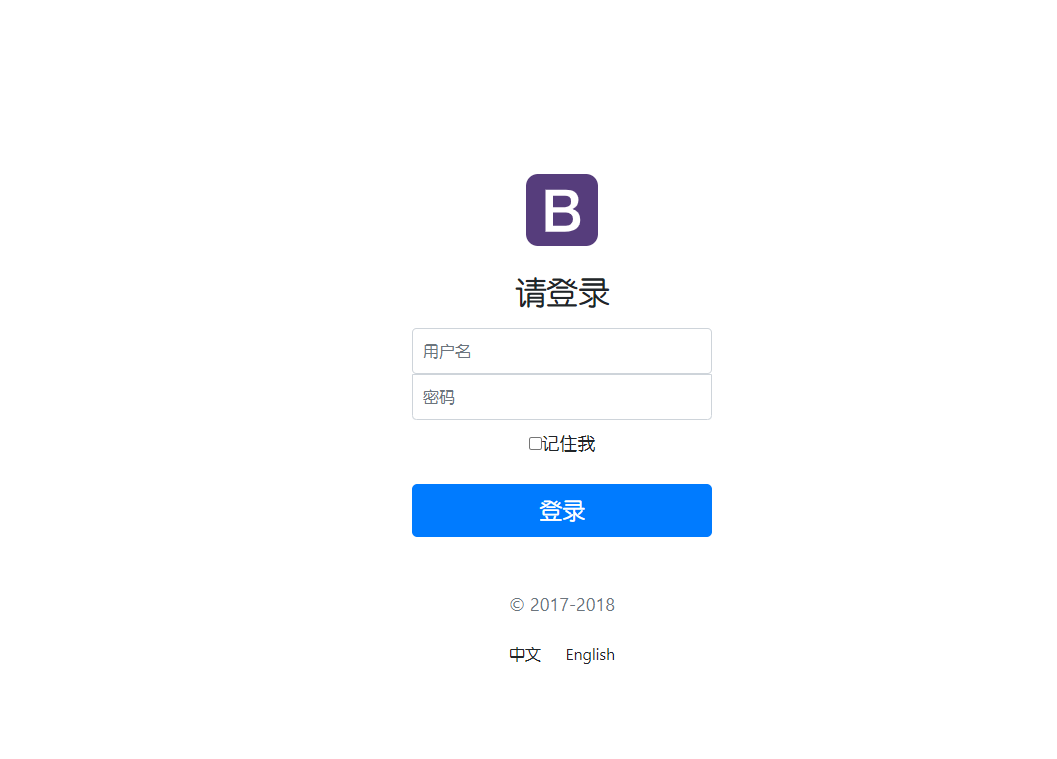
3.2 realize the following button switching
1. First use the jump address for the two buttons
<a class="btn btn-sm" th:href="@{/index.html(l='zh_CN')}">chinese</a> <a class="btn btn-sm" th:href="@{/index.html(l='en_US')}">English</a>
2. Customize internationalization components
You can create a MyLocalResolver class under the config folder
public class MyLocalResolver implements LocaleResolver { @Override public Locale resolveLocale(HttpServletRequest httpServletRequest) { //Get parameters in request String language = httpServletRequest.getParameter("l"); //If not, default Locale locale = Locale.getDefault(); //If the requested connection carries internationalization parameters if (!StringUtils.isEmpty(language)){ String[] l = language.split("_"); //Country and region locale=new Locale(l[0], l[1]); } return locale; } @Override public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) { } }
3. Register as Bean in MyMvcConfig to make it effective
@Bean public LocaleResolver localeResolver(){ return new MyLocalResolver(); }
In this way, after running the software, you can click the button to switch between Chinese and English.
4, Landing page implementation
1. Modify index html
<body class="text-center"> <!--Modify the running path to th:action="@{/login}" /login by Controller Class setting path --> <form class="form-signin" th:action="@{/login}"> <img class="mb-4" th:src="@{/img/bootstrap-solid.svg}" alt="" width="72" height="72"> <h1 class="h3 mb-3 font-weight-normal" th:text="#{login.tip}">Please sign in</h1> <!--Add an error statement. If there is a login error, it can be displayed --> <p style="color: red" th:text="${msg}" th:if="${not #strings.isEmpty(msg)}"></p> <label class="sr-only" th:text="#{login.username}">Username</label> <!--add to name="username",Values can be passed to the back end --> <input type="text" class="form-control" th:placeholder="#{login.username}" name="username" required="" autofocus=""> <label class="sr-only" th:text="#{login.password}">Password</label> <!--add to name="password",Values can be passed to the back end --> <input type="password" class="form-control" th:placeholder="#{login.password}" name="password" required=""> <div class="checkbox mb-3"> <label> <input type="checkbox" value="remember-me" th:text="#{login.remember}"> </label> </div> <button class="btn btn-lg btn-primary btn-block" type="submit" >[[#{login.btn}]]</button> <p class="mt-5 mb-3 text-muted">© 2017-2018</p> <a class="btn btn-sm" th:href="@{/index.html(l='zh_CN')}">chinese</a> <a class="btn btn-sm" th:href="@{/index.html(l='en_US')}">English</a> </form> </body>
2. Write control class
@Controller public class LoginController { @RequestMapping("/login") public String login(@RequestParam("username") String username, @RequestParam("password") String password, Model model){ // For specific business, you can log in when the username is not empty and the password is 123456, otherwise the error message msg is returned if (!StringUtils.isEmpty(username)&&password.equals("123456")){ return "dashboard"; }else { model.addAttribute("msg","Wrong user name or password"); return "index"; } } }
3. Test run
When input error:
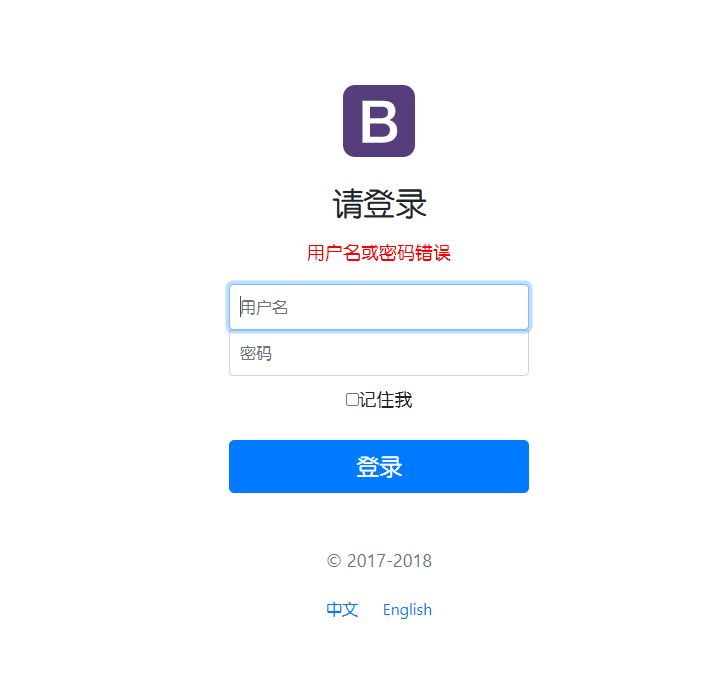
On successful login:
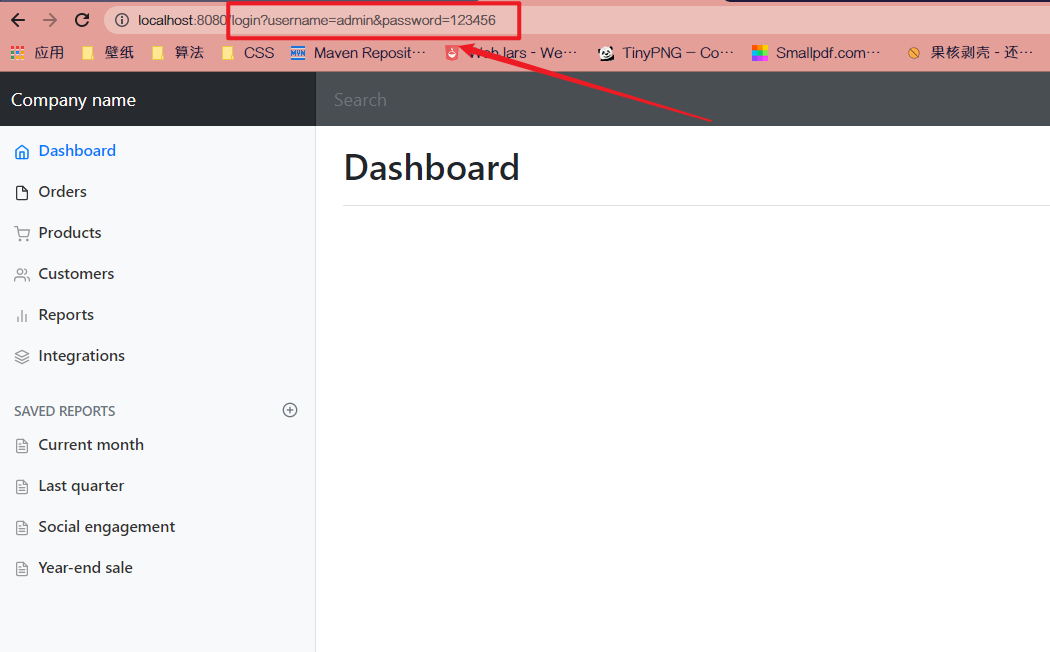
4. Path optimization
Looking at the successful login page path in the figure above, you can see that the login parameters are at a glance;
This is definitely unsafe, so we need to optimize the path
Idea: when we visit http://localhost:8080/main.html You can enter the main page
Step 1: add a main in MyMvcConfig HTML mapping
public void addViewControllers(ViewControllerRegistry registry) { registry.addViewController("/").setViewName("index"); registry.addViewController("/index.html").setViewName("index"); //Add a main HTML mapping registry.addViewController("/main.html").setViewName("dashboard.html"); }
Step 2: when the login is successful, redirect to main HTML, modify Controller
public String login(@RequestParam("username") String username, @RequestParam("password") String password, Model model){ // Specific business if (!StringUtils.isEmpty(username)&&password.equals("123456")){ //Redirect to main html return "redirect:/main.html"; }else { model.addAttribute("msg","Wrong user name or password"); return "index"; } }
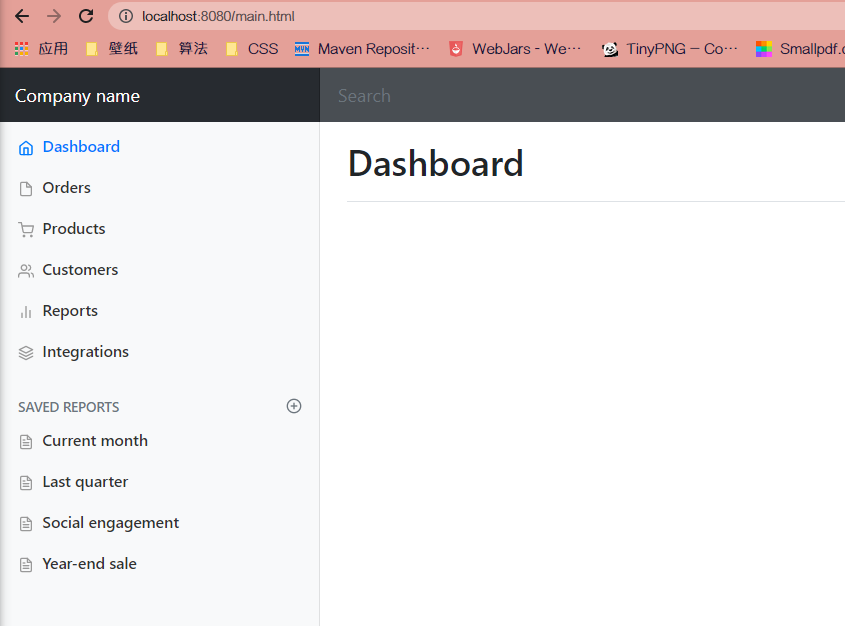
Login success page successfully changed
5, Login interceptor
1. Change the Controller class and save the username into the session domain after logging in
@RequestMapping("/login") // @ResponseBody public String login(@RequestParam("username") String username, @RequestParam("password") String password, Model model, HttpSession session){ // Specific business if (!StringUtils.isEmpty(username)&&password.equals("123456")){ // Save to session session.setAttribute("loginUser",username); return "redirect:/main.html"; }else { model.addAttribute("msg","Wrong user name or password"); return "index"; } } }
2. Create interceptor class in config
public class LoginHandlerInterceptor implements HandlerInterceptor { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { //After successful login, there should be a user Session Object loginUser = request.getSession().getAttribute("loginUser"); if (loginUser==null){ //No login request.setAttribute("msg","You don't have permission, please login"); request.getRequestDispatcher("/index.html").forward(request,response); return false; } else { return true; } } }
3. Add an interceptor mapping in MyMvcConfig
@Override public void addInterceptors(InterceptorRegistry registry) { // /**Intercept all resources; Excluding /, / login, / index HTML, / CSS / *, / img / *, / JS / * resources under the path. registry.addInterceptor(new LoginHandlerInterceptor()).addPathPatterns("/**") .excludePathPatterns("/","/login","/index.html","/css/*","/img/*","/js/*"); }
6, Show employee list
6.1 import employee page
The jump links in the directly imported web pages are self-contained by default. We need to modify them to our own pages.
1, Write Controller
@Controller public class EmployeeController { @Autowired EmployeeDao employeeDao; @RequestMapping("/emps") public String getAllemployee(Model model){ Collection<Employee> employees = employeeDao.getEmployees(); model.addAttribute("emp",employees); //A new emp folder is created and the list HTML inside return "emp/list"; } }
2, Modify the jump link in the employee section of the sidebar
<li class="nav-item"> <!-- th:href="@{/emp}" function Controller Class method, jump list.html --> <a th:class="${active=='list.html'?'nav-link active':'nav-link' }" th:href="@{/emps}" > <svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-users"> <path d="M17 21v-2a4 4 0 0 0-4-4H5a4 4 0 0 0-4 4v2"></path> <circle cx="9" cy="7" r="4"></circle> <path d="M23 21v-2a4 4 0 0 0-3-3.87"></path> <path d="M16 3.13a4 4 0 0 1 0 7.75"></path> </svg> Customers </a> </li>
6.2 prepare extraction page
We can see that the header and sidebar of each page are repeated, so we can extract the repeated part and directly introduce it into the html file.
- Extract file: th:fragment="xxxxx"
- Import file: th:replace="{xxxx}" * *, * * th:insert="{xxxx}"
The first step is to create a commons folder in templates, where commons html.
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <!-- Top th:fragment="topbar" --> <nav class="navbar navbar-dark sticky-top bg-dark flex-md-nowrap p-0" th:fragment="topbar"> <a class="navbar-brand col-sm-3 col-md-2 mr-0" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">[[${session.loginUser}]]</a> <input class="form-control form-control-dark w-100" type="text" placeholder="Search" aria-label="Search"> <ul class="navbar-nav px-3"> <li class="nav-item text-nowrap"> <a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">Sign out</a> </li> </ul> </nav > <!-- sidebar th:fragment="sidebar" --> <nav class="col-md-2 d-none d-md-block bg-light sidebar" th:fragment="sidebar"> <div class="sidebar-sticky"> <ul class="nav flex-column"> <li class="nav-item"> <a th:class="${active=='main.html'?'nav-link active':'nav-link' }" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#"> <svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-home"> <path d="M3 9l9-7 9 7v11a2 2 0 0 1-2 2H5a2 2 0 0 1-2-2z"></path> <polyline points="9 22 9 12 15 12 15 22"></polyline> </svg> Dashboard <span class="sr-only">(current)</span> </a> </li> ..........Middle omission <li class="nav-item"> <a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#"> <svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file-text"> <path d="M14 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V8z"></path> <polyline points="14 2 14 8 20 8"></polyline> <line x1="16" y1="13" x2="8" y2="13"></line> <line x1="16" y1="17" x2="8" y2="17"></line> <polyline points="10 9 9 9 8 9"></polyline> </svg> Year-end sale </a> </li> </ul> </div> </nav> </html>
The second step is to delete the original header and side code in other html pages and introduce them in the same position
<!-- Top--> <div th:replace="~{commons/commons::topbar}"></div> <!-- sidebar --> <div th:replace="~{commons/commons::sidebar}" ></div>
The third step is to click which module on the side of the web page and the text corresponding to which module will be highlighted
Idea:
- Pass a parameter when jumping
- The parameters of each module are represented by the corresponding html name
- Judge in the sidebar code and highlight the corresponding part of the corresponding name
1. Add parameters
For example, dashboard HTML and list HTML page:
<div th:replace="~{commons/commons::sidebar(active='main.html')}" ></div>
<div th:replace="~{commons/commons::sidebar(active='list.html')}"></div>
2. Judgment
Use th:class="${active = = 'xxx'? 'NAV link active': 'NAV link'}" to judge
<li class="nav-item"> <a th:class="${active=='main.html'?'nav-link active':'nav-link' }" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#"> <svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-home"> <path d="M3 9l9-7 9 7v11a2 2 0 0 1-2 2H5a2 2 0 0 1-2-2z"></path> <polyline points="9 22 9 12 15 12 15 22"></polyline> </svg> Dashboard <span class="sr-only">(current)</span> </a> </li>
<li class="nav-item"> <a th:class="${active=='list.html'?'nav-link active':'nav-link' }" th:href="@{/emps}" > <svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-users"> <path d="M17 21v-2a4 4 0 0 0-4-4H5a4 4 0 0 0-4 4v2"></path> <circle cx="9" cy="7" r="4"></circle> <path d="M23 21v-2a4 4 0 0 0-3-3.87"></path> <path d="M16 3.13a4 4 0 0 1 0 7.75"></path> </svg> Customers </a> </li>
6.3 show employee list
At this stage, the preparatory work is basically completed and starts on the list HTML presentation
<main role="main" class="col-md-9 ml-sm-auto col-lg-10 pt-3 px-4"> <h2>Section title</h2> <div class="table-responsive"> <table class="table table-striped table-sm"> <thead> <tr> <th>id</th> <th>lastName</th> <th>email</th> <th>gender</th> <th>department</th> <th>birth</th> </tr> </thead> <tbody th:each="emp:${emps}"> <tr> <td th:text="${emp.getId()}"></td> <td th:text="${emp.getLastName()}"></td> <td th:text="${emp.getEmail()}"></td> <td th:text="${emp.getGender()==0?'female':'male '}"></td> <td th:text="${emp.getDepartment().getDepartmentName()}"></td> <td th:text="${#dates.format(emp.getBirth(),'yyyy-mm-dd hh:mm:ss')}"></td> <td> <button class="btn btn-sm btn-primary">edit</button> <button class="btn btn-sm btn-danger">delete</button> </td> </tr> </tbody> </table> </div> </main>
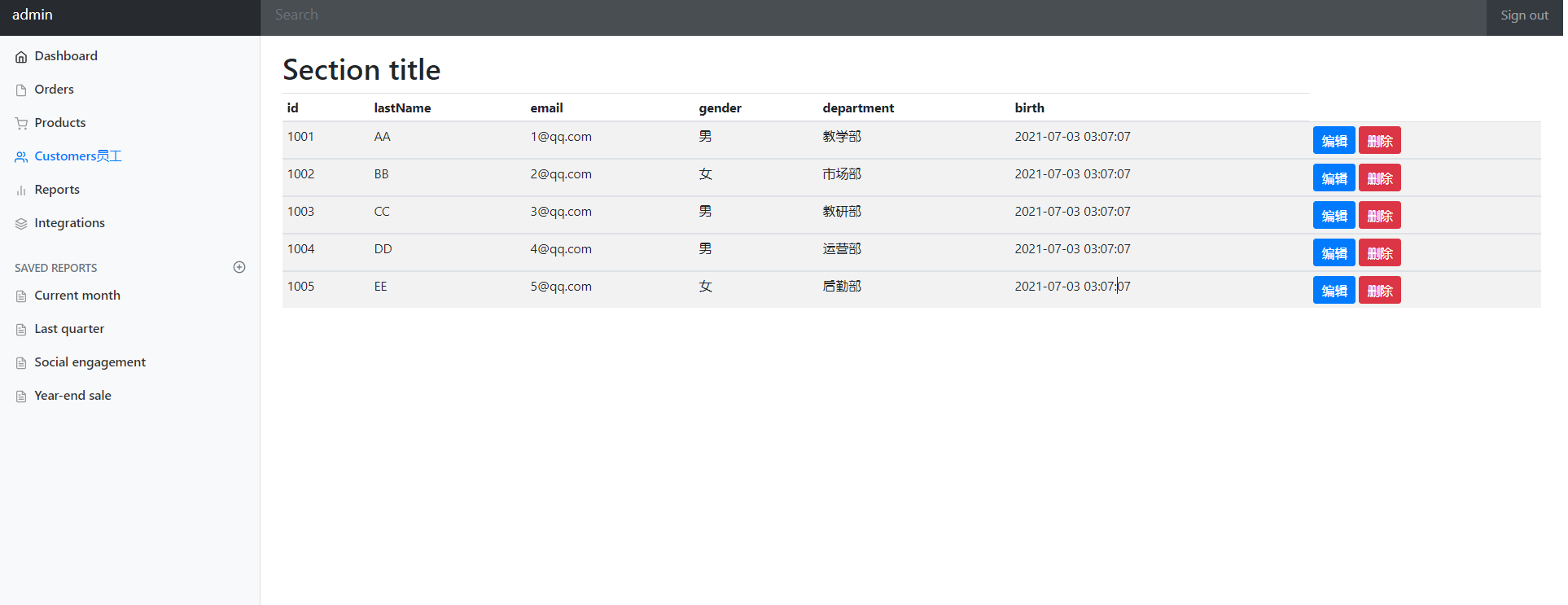
7, New employee information
1. First write a method to jump to the added page
@GetMapping("/emp") public String toAdd(Model model){ //Find out all department information Collection<Department> departments = departmentDao.getDepartments(); model.addAttribute("depts",departments); return "emp/add"; }
2. Add another add page in the emp folder:
from form method to use post
<!DOCTYPE html> <!-- saved from url=(0052)http://getbootstrap.com/docs/4.0/examples/dashboard/ --> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <meta name="description" content=""> <meta name="author" content=""> <title>Dashboard Template for Bootstrap</title> <!-- Bootstrap core CSS --> <link th:href="@{/css/bootstrap.min.css}" rel="stylesheet"> <!-- Custom styles for this template --> <link th:href="@{/css/dashboard.css}" rel="stylesheet"> <style type="text/css"> /* Chart.js */ @-webkit-keyframes chartjs-render-animation { from { opacity: 0.99 } to { opacity: 1 } } @keyframes chartjs-render-animation { from { opacity: 0.99 } to { opacity: 1 } } .chartjs-render-monitor { -webkit-animation: chartjs-render-animation 0.001s; animation: chartjs-render-animation 0.001s; } </style> </head> <body> <div th:replace="~{commons/commons::topbar}"></div> <div class="container-fluid"> <div class="row"> <div th:replace="~{commons/commons::sidebar(active='list.html')}"></div> <main role="main" class="col-md-9 ml-sm-auto col-lg-10 pt-3 px-4"> <form class="form-horizontal" th:action="@{/emp}" method="post"> <div class="form-group"> <label class="col-sm-2 control-label">full name</label> <div class="col-sm-10"> <input type="text" class="form-control" name="lastName" id="lastName" placeholder="lastName"> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">mailbox</label> <div class="col-sm-10"> <input type="email" class="form-control" name="email" id="Email" placeholder="Email"> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">Gender</label> <div class="col-sm-10"> <input type="radio" class="form-check-input" name="gender" value="0"> <label class="col-sm-2 control-label">female</label> <input type="radio" class="form-check-input" name="gender" value="1"> <label class="col-sm-2 control-label">male</label> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">department</label> <div class="col-sm-10"> <select class="form-control" name="department.id"> <option th:each="dept:${depts}" th:text="${dept.getDepartmentName()}" th:value="${dept.getId()}">Teaching Department</option> </select> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">date</label> <div class="col-sm-10"> <input type="text" class="form-control" name="birth" id="birth" placeholder="birth"> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-success">Submit</button> </div> </div> </form> </main> </div> </div> <!-- Bootstrap core JavaScript ================================================== --> <!-- Placed at the end of the document so the pages load faster --> <script type="text/javascript" src="asserts/js/jquery-3.2.1.slim.min.js"></script> <script type="text/javascript" src="asserts/js/popper.min.js"></script> <script type="text/javascript" src="asserts/js/bootstrap.min.js"></script> <!-- Icons --> <script type="text/javascript" src="asserts/js/feather.min.js"></script> <script> feather.replace() </script> <!-- Graphs --> <script type="text/javascript" src="asserts/js/Chart.min.js"></script> <script> var ctx = document.getElementById("myChart"); var myChart = new Chart(ctx, { type: 'line', data: { labels: ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"], datasets: [{ data: [15339, 21345, 18483, 24003, 23489, 24092, 12034], lineTension: 0, backgroundColor: 'transparent', borderColor: '#007bff', borderWidth: 4, pointBackgroundColor: '#007bff' }] }, options: { scales: { yAxes: [{ ticks: { beginAtZero: false } }] }, legend: { display: false, } } }); </script> </body> </html>
3. Write the method of adding employee Controller
At this time, the path used is still emp, but because it is passed by post, it conforms to the Restful style.
@PostMapping("/emp") public String Addemp(Employee employee){ System.out.println(employee); //Save employee information employeeDao.save(employee); return "redirect:/emps"; }
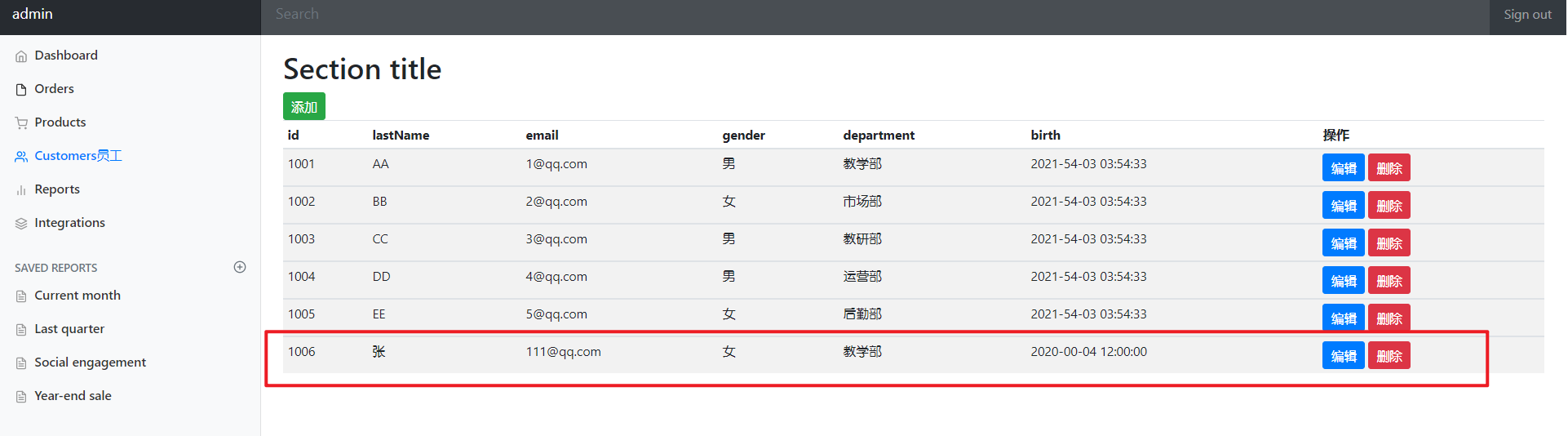
8, Modify employee information
1. First write a method to jump to the modification page
@GetMapping("/emp/{id}") public String toUpdateEmp(@PathVariable("id") Integer id, Model model){ //Find out the corresponding data Employee employee = employeeDao.getEmployee(id); model.addAttribute("emp",employee); //Find out all department information Collection<Department> departments = departmentDao.getDepartments(); model.addAttribute("depts",departments); return "emp/update"; }
2. Modify the jump path of the Edit button in the list page
<a class="btn btn-sm btn-primary" th:href="@{/emp/}+${emp.getId()}">edit</a>
Note: the '+' here will be red. Don't worry
3. Add another update page in the emp folder:
from form method to use post
The update page can directly copy the add page
The following is * * * *
<form class="form-horizontal" th:action="@{/updateEmp}" method="post"> <input type="hidden" name="id" th:value="${emp.getId()}"> <div class="form-group"> <label class="col-sm-2 control-label">full name</label> <div class="col-sm-10"> <input th:value="${emp.getLastName()}" type="text" class="form-control" name="lastName" id="lastName" placeholder="lastName"> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">mailbox</label> <div class="col-sm-10"> <input th:value="${emp.getEmail()}" type="email" class="form-control" name="email" id="Email" placeholder="Email"> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">Gender</label> <div class="col-sm-10"> <input th:checked="${emp.getGender()==0}" type="radio" class="form-check-input" name="gender" value="0"> <label class="col-sm-2 control-label">female</label> <input th:checked="${emp.getGender()==1}" type="radio" class="form-check-input" name="gender" value="1"> <label class="col-sm-2 control-label">male</label> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">department</label> <div class="col-sm-10"> <select class="form-control" name="department.id"> <option th:selected="${dept.getId()==emp.getDepartment().getId()}" th:each="dept:${depts}" th:text="${dept.getDepartmentName()}" th:value="${dept.getId()}"></option> </select> </div> </div> <div class="form-group"> <label class="col-sm-2 control-label">date</label> <div class="col-sm-10"> <input th:value="${#dates.format(emp.getBirth(),'yyyy-MM-dd hh:mm:ss')}" type="text" class="form-control" name="birth" id="birth" placeholder="birth"> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-success">Submit</button> </div> </div> </form>
4. Write and modify employee Controller class methods
@PostMapping("/updateEmp") public String UpdateEmp(Employee employee){ employeeDao.save(employee); return "redirect:/emps"; }
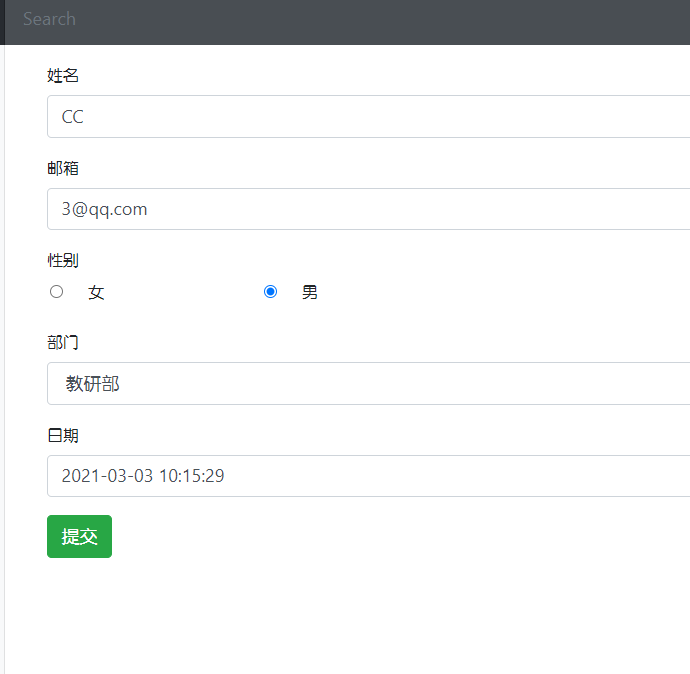
9, Delete employee information
1. Modify list page - delete path
<a class="btn btn-sm btn-danger" th:href="@{/delemp/}+${emp.getId()}">delete</a>
2. Modify the Controller class
@GetMapping("/delemp/{id}") public String DelEmp(@PathVariable("id") Integer id){ employeeDao.delEmployee(id); return "redirect:/emps"; }
10, 404 page, logout operation
10.1 page 404
404 page is the simplest function in SpringBoot:
- Create the error folder in templates
- Move 404 pages into it
- Done!
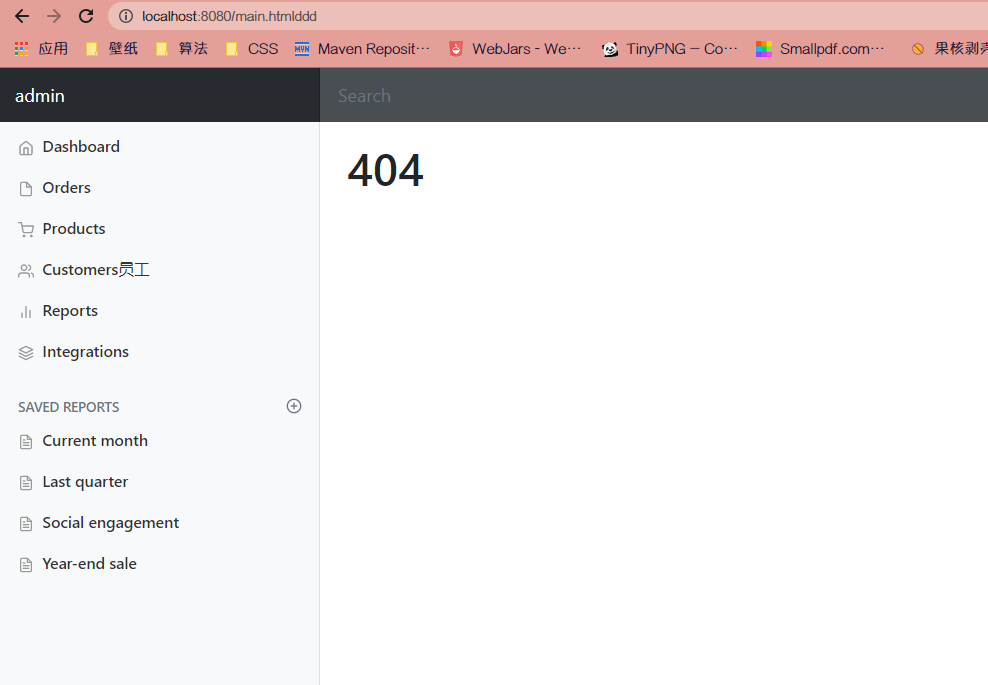
10.2 logout
1. Write Controller method
@RequestMapping("/loginout") public String loginout(HttpSession session){ //session.invalidate(); session.removeAttribute("loginUser"); return "redirect:index.html"; }
2. Modify the jump path at the logout
<nav class="navbar navbar-dark sticky-top bg-dark flex-md-nowrap p-0" th:fragment="topbar"> <a class="navbar-brand col-sm-3 col-md-2 mr-0" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">[[${session.loginUser}]]</a> <input class="form-control form-control-dark w-100" type="text" placeholder="Search" aria-label="Search"> <ul class="navbar-nav px-3"> <li class="nav-item text-nowrap"> <!--Jump path modification--> <a class="nav-link" th:href="@{/loginout}">Sign out</a> </li> </ul> </nav >
Such a CRUD project is basically completed!