Simple understanding of SpringBoot distributed system
Next, after understanding the basic concept of distributed system and setting up the environment, use a simple example to experience distributed services.
1. Environmental construction
Create an empty new project and name it SpringBoot-09-DS.
Create a provider server module of the SpringBoot project, and select Web dependency to represent the service provider.
The book is first written in one of the services
package com.qiyuan.service; public interface BookService { public String getBook(); }
Then is the implementation class of the interface
package com.qiyuan.service; public class BookServiceImpl implements BookService{ @Override public String getBook() { return "Qiyuanc My notes"; } }
In this way, the service of the service provider is completed, and consumers can get books through the service of BookService.
Create a new SpringBoot project module consumer server, which only selects Web dependency as the service consumer, and there is only one book buying service
package com.qiyuan.service; public class UserService { // Consumers are going to buy books! }
Now, what we need to do is to enable the consumer service program to use the services provided by the provider service program through distributed services!
2. Service providers
When it comes to distributed services, Dubbo and ZooKeeper need to be imported
<dependency> <groupId>org.apache.dubbo</groupId> <artifactId>dubbo-spring-boot-starter</artifactId> <version>2.7.3</version> </dependency> <!-- zkclient --> <dependency> <groupId>com.github.sgroschupf</groupId> <artifactId>zkclient</artifactId> <version>0.1</version> </dependency> <!-- zookeeper --> <dependency> <groupId>org.apache.curator</groupId> <artifactId>curator-framework</artifactId> <version>2.12.0</version> </dependency> <dependency> <groupId>org.apache.curator</groupId> <artifactId>curator-recipes</artifactId> <version>2.12.0</version> </dependency> <dependency> <groupId>org.apache.zookeeper</groupId> <artifactId>zookeeper</artifactId> <version>3.4.14</version> <!-- exclude slf4j-log4j12 --> <exclusions> <exclusion> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
After the dependency is imported, it can be in the SpringBoot configuration file application Configure the properties of dubbo in properties
#Current app name dubbo.application.name=provider-server #Address of Registration Center dubbo.registry.address=zookeeper://127.0.0.1:2181 #Scan the services under the specified package dubbo.scan.base-packages=com.qiyuan.service #Application service WEB access port server.port=8001 #In order not to conflict with Dubbo admin, you need to change the port dubbo.protocol.port=20881
After the configuration is completed, you can publish the Service to the registry through the @ Service annotation. Note that this @ Service is Dubbo's, not Spring's (after Dubbo 2.7, the @ DubboService annotation was changed to avoid conflict, but there is a problem with the higher version, so you can only honestly use the old version!)
package com.qiyuan.service; import org.apache.dubbo.config.annotation.DubboService; import org.springframework.stereotype.Service; // Publish to the registry as Dubbo's service @Service // Put this class into the Spring container @Component public class BookServiceImpl implements BookService{ @Override public String getBook() { return "Qiyuanc My notes"; } }
After the application starts, Dubbo will scan the Service with @ Service annotation under the specified package and publish it to the specified registry.
3. Service consumers
Like service providers, service consumers also need to import dependencies related to Dubbo and ZooKeeper.
Then configure it in the configuration file of the service consumer
#Current app name dubbo.application.name=consumer-server #Address of Registration Center dubbo.registry.address=zookeeper://127.0.0.1:2181 #Application service WEB access port server.port=8002
At this time, the provider's interface should be packaged and delivered to consumers to import in the form of pom, so that consumers can use the provider's interface when programming. However, for simple testing, the required interface is directly added to the consumer here, and the interface path is consistent with that in the provider.
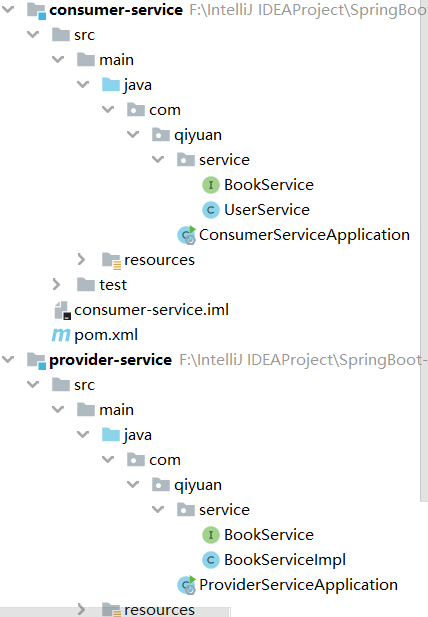
Then you can use the provider's services among consumers
package com.qiyuan.service; import org.apache.dubbo.config.annotation.DubboReference; import org.springframework.stereotype.Service; // Give it to Spring @Service public class UserService { // Consumers are going to buy books! // If the specified service is referenced remotely, the interface will be found in the registry @Reference BookService bookService; public void buyBook(){ String book = bookService.getBook(); System.out.println("Using the service of the registry:"+book+"!"); } }
Write a test class in the consumer to test
@SpringBootTest class ConsumerServiceApplicationTests { @Autowired UserService userService; @Test void contextLoads() { // Consumers call the interface provided by the service provider to buy books! userService.buyBook(); } }
The service startup sequence is
- Open ZooKeeper registration center;
- Open Dubbo admin for monitoring;
- Open the service provider application;
- Open the service consumer application and use the service!

Test successful (find BUG and find hemp)! You can also see the interface provided by the service provider in Dubbo admin
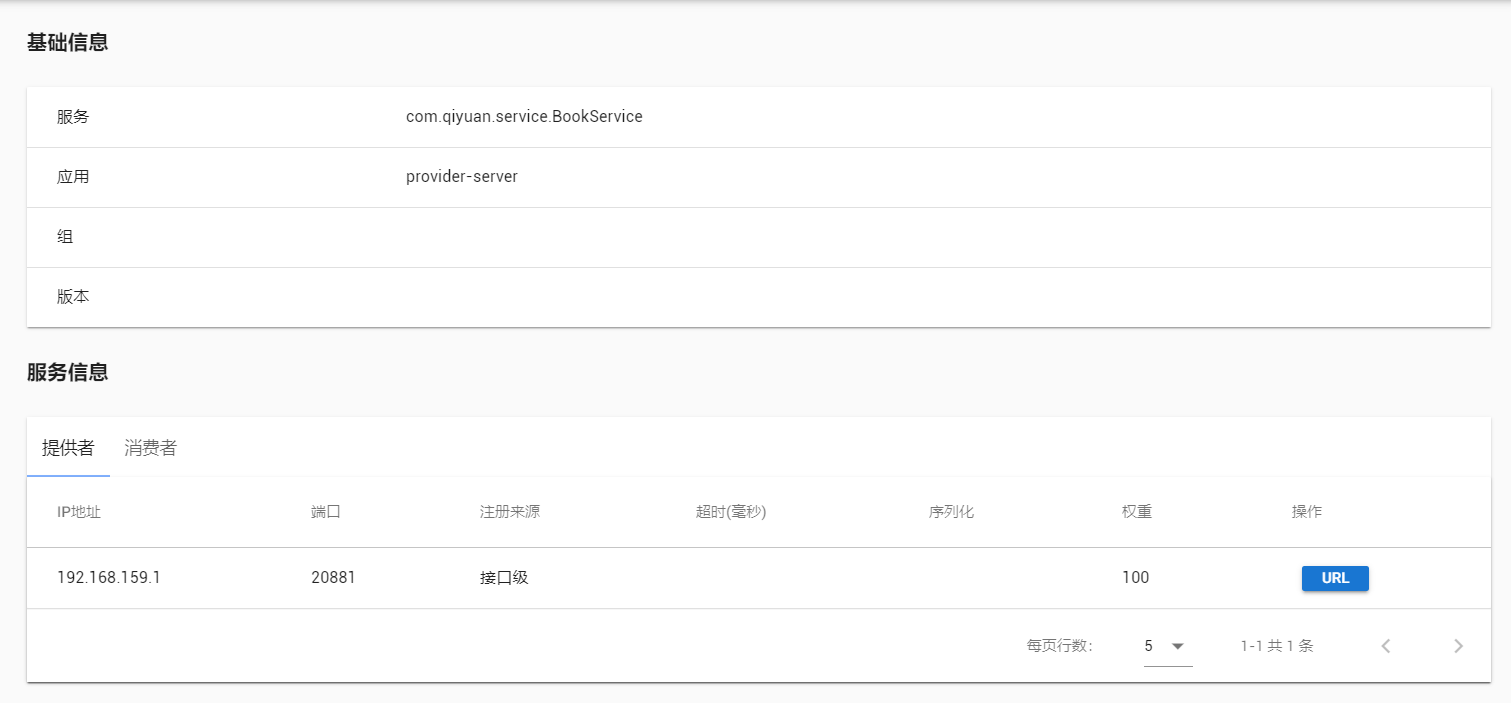
The simple distributed service test is over... One is the version problem, the other is the port problem, which makes me confused.
3. Summary
We tried to use distributed services through SpringBoot + Dubbo + ZooKeeper. The basic concept and operation are relatively simple, that is, through the registry, different applications can use the network to communicate and use the services provided by other applications. The follow-up still needs to be further deepened.