Article catalogue
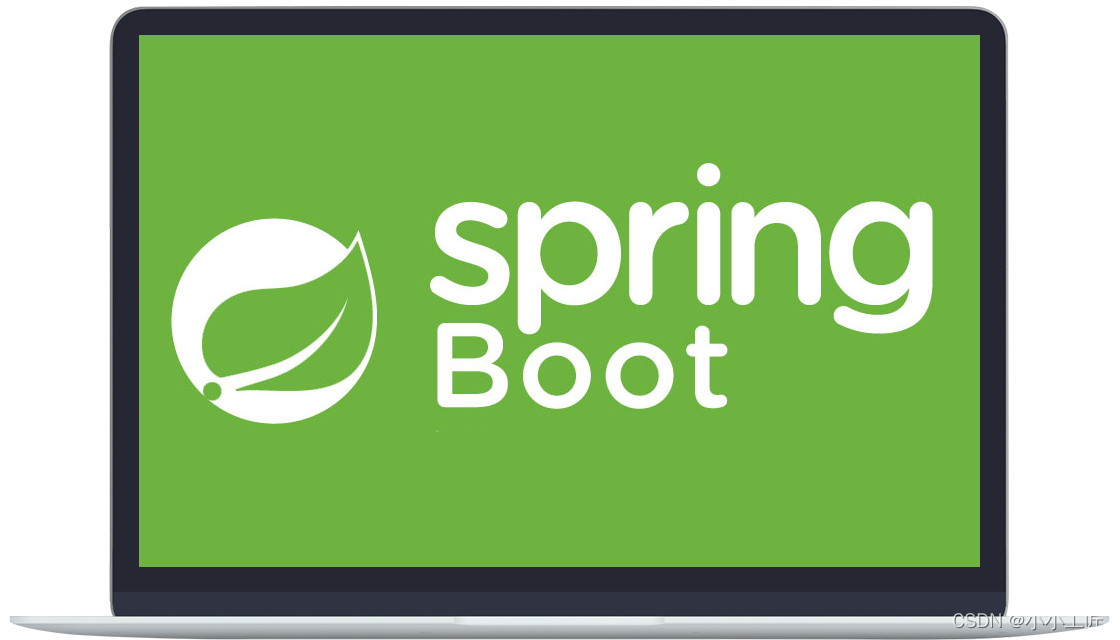
demand
One parameter is very important. If it is not configured or configured incorrectly, the application cannot be started.
We use the Java Validation function provided by Spring to meet this requirement
trilogy
Step1 Validation related configuration on the properties class
package com.artisan.startvalidator.config; import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component; import org.springframework.validation.annotation.Validated; import javax.validation.constraints.NotEmpty; /** * @author Small craftsman * @version 1.0 * @mark: show me the code , change the world */ @Validated @Component @Data @ConfigurationProperties(prefix = "artisan") public class ArtisanConfigProperties { @NotEmpty(message = "Must be configured[artisan.code]attribute") private String code; }
The above configuration will verify whether artisan is configured in the application configuration file code . If it is not configured, the project startup will fail and a verification exception will be thrown.
Warm tip: when using configuration file verification, you must use @ configurationproperties annotation, which @ value does not support
Step 2 start test
Binding to target org.springframework.boot.context.properties.bind.BindException: Failed to bind properties under 'artisan' to com.artisan.startvalidator.config.ArtisanConfigProperties failed: Property: artisan.code Value: null Reason: Must be configured[artisan.code]attribute
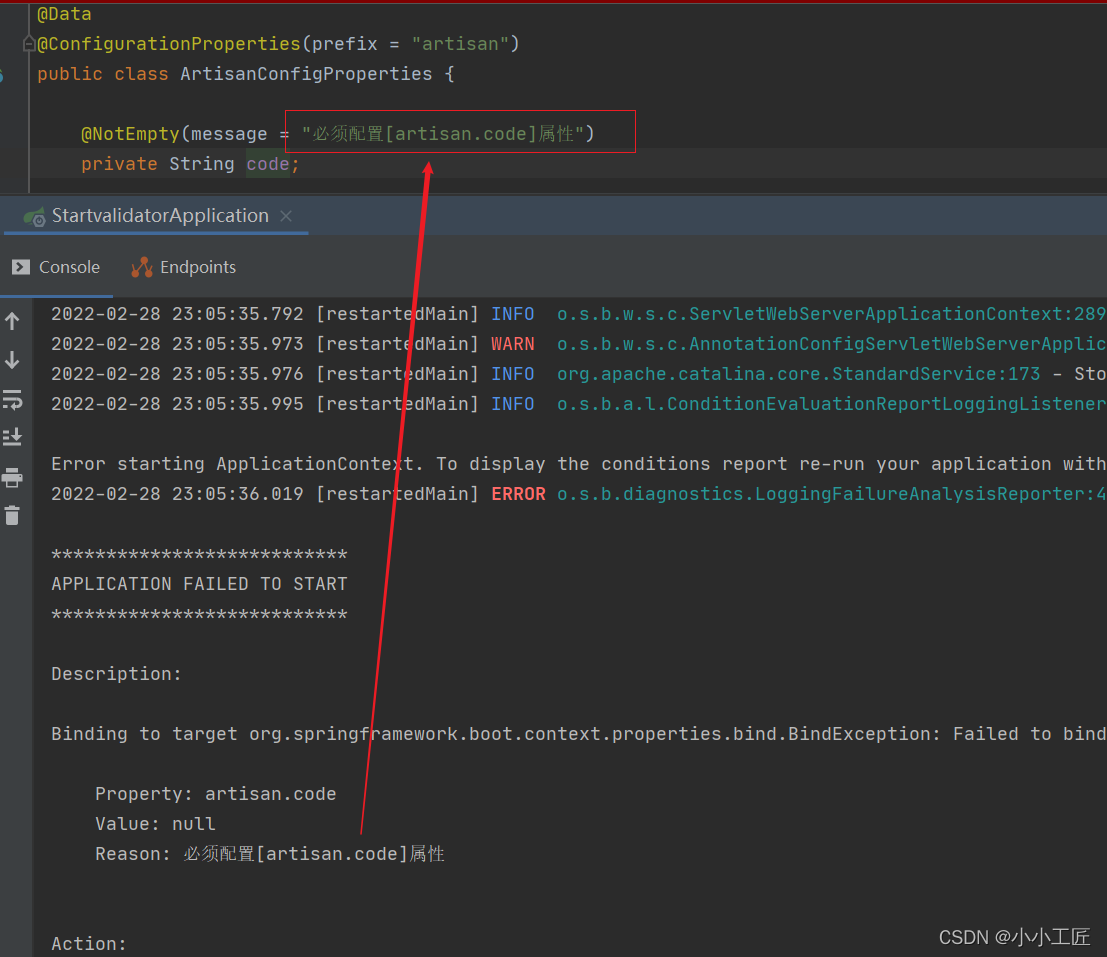
Step 3 with try
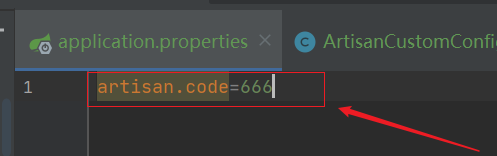
Do a random test
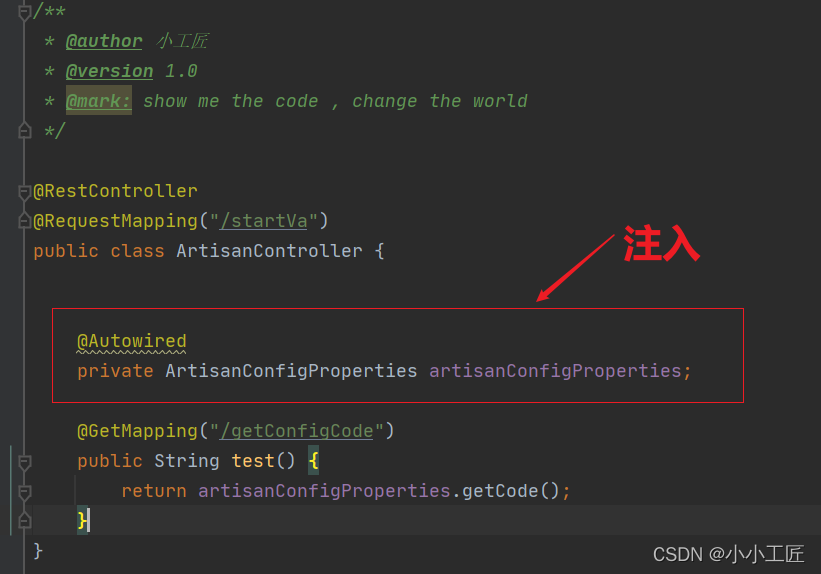
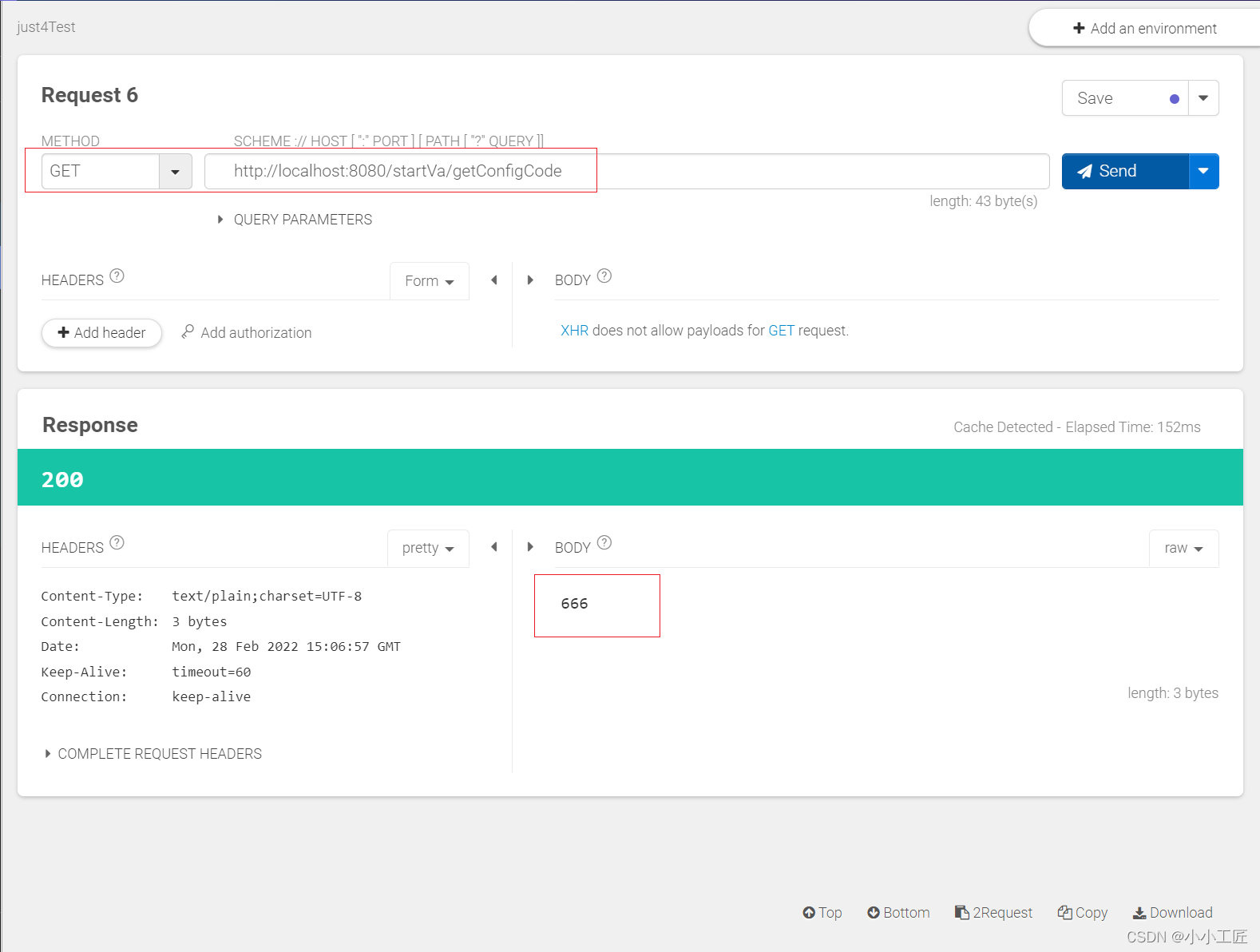
Of course, depending on your needs, you can also use other annotations provided by the framework
Verification rules | Rule description |
---|---|
@Null | Restriction can only be null |
@NotNull | Restriction must not be null |
@AssertFalse | Restriction must be false |
@AssertTrue | Restriction must be true |
@DecimalMax(value) | The limit must be a number no greater than the specified value |
@DecimalMin(value) | The limit must be a number not less than the specified value |
@Digits(integer,fraction) | The limit must be one decimal, and the number of digits in the integer part cannot exceed integer, and the number of digits in the decimal part cannot exceed fraction |
@Future | Limit must be a future date |
@Max(value) | The limit must be a number no greater than the specified value |
@Min(value) | The limit must be a number not less than the specified value |
@Past | Verify that the element value (date type) of the annotation is earlier than the current time |
@Pattern(value) | The restriction must conform to the specified regular expression |
@Size(max,min) | The limit character length must be between min and max |
@NotEmpty | The element value of the validation annotation is not null and empty (string length is not 0, collection size is not 0) |
@NotBlank | The element value of the verification annotation is not null (it is not null, and the length is 0 after removing the first space). Unlike @ NotEmpty, @ NotBlank is only applied to strings, and the spaces of strings will be removed during comparison |
Verify that the element value of the annotation is email. You can also specify a custom email format through regular expression and flag |
Custom verification rules
When none of the above comments can satisfy you
Then just customize one
Step1 interface implementation
Custom verification logic rules, implementation org springframework. validation. Validator
package com.artisan.startvalidator.validator; import com.artisan.startvalidator.config.ArtisanConfigProperties; import org.springframework.util.StringUtils; import org.springframework.validation.Errors; import org.springframework.validation.Validator; /** * @author Small craftsman * @version 1.0 * @mark: show me the code , change the world */ public class ArtisanCustomConfigPropertiesValidator implements Validator { @Override public boolean supports(Class<?> clazz) { // Parent class class. Isassignablefrom (subclass. class) return ArtisanConfigProperties.class.isAssignableFrom(clazz); } @Override public void validate(Object target, Errors errors) { ArtisanConfigProperties config = (ArtisanConfigProperties) target; if (StringUtils.isEmpty(config.getCode())) { errors.rejectValue("code", "artisan.code.empty", "[artisan.code] Property must be in the configuration file application.properties Medium configuration"); } else if (config.getCode().length() < 8) { errors.rejectValue("id", "artisan.code.short", "[artisan.code] The length of the property needs to be greater than 8"); } } }
Step 2 property file
If you use custom verification rules, you don't need to use the native @ NotEmpty. You can delete it
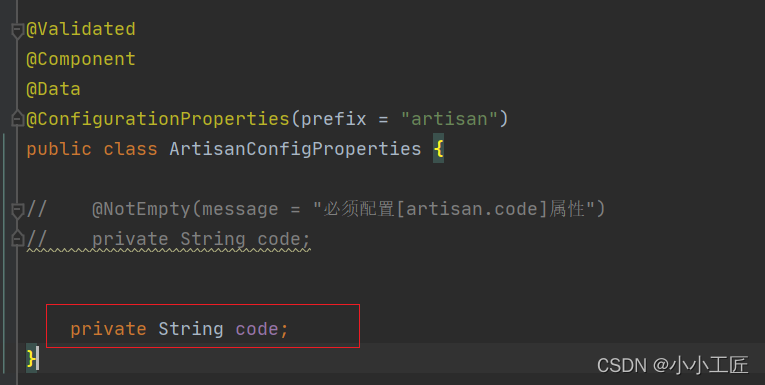
Step 3 user defined verification rules
Inject custom verification rules, write a configuration class, @ Bean
package com.artisan.startvalidator.config; import com.artisan.startvalidator.validator.ArtisanCustomConfigPropertiesValidator; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; /** * @author Small craftsman * @version 1.0 * @mark: show me the code , change the world */ @Configuration public class AppConfiguration { /** * bean The method name of must be configurationPropertiesValidator, otherwise the verification will not be performed at startup * * @return */ @Bean public ArtisanCustomConfigPropertiesValidator configurationPropertiesValidator() { return new ArtisanCustomConfigPropertiesValidator(); } }
The method name of the bean must be configurationPropertiesValidator, otherwise it will not take effect.
Step 4 verify one
Change application Properties code
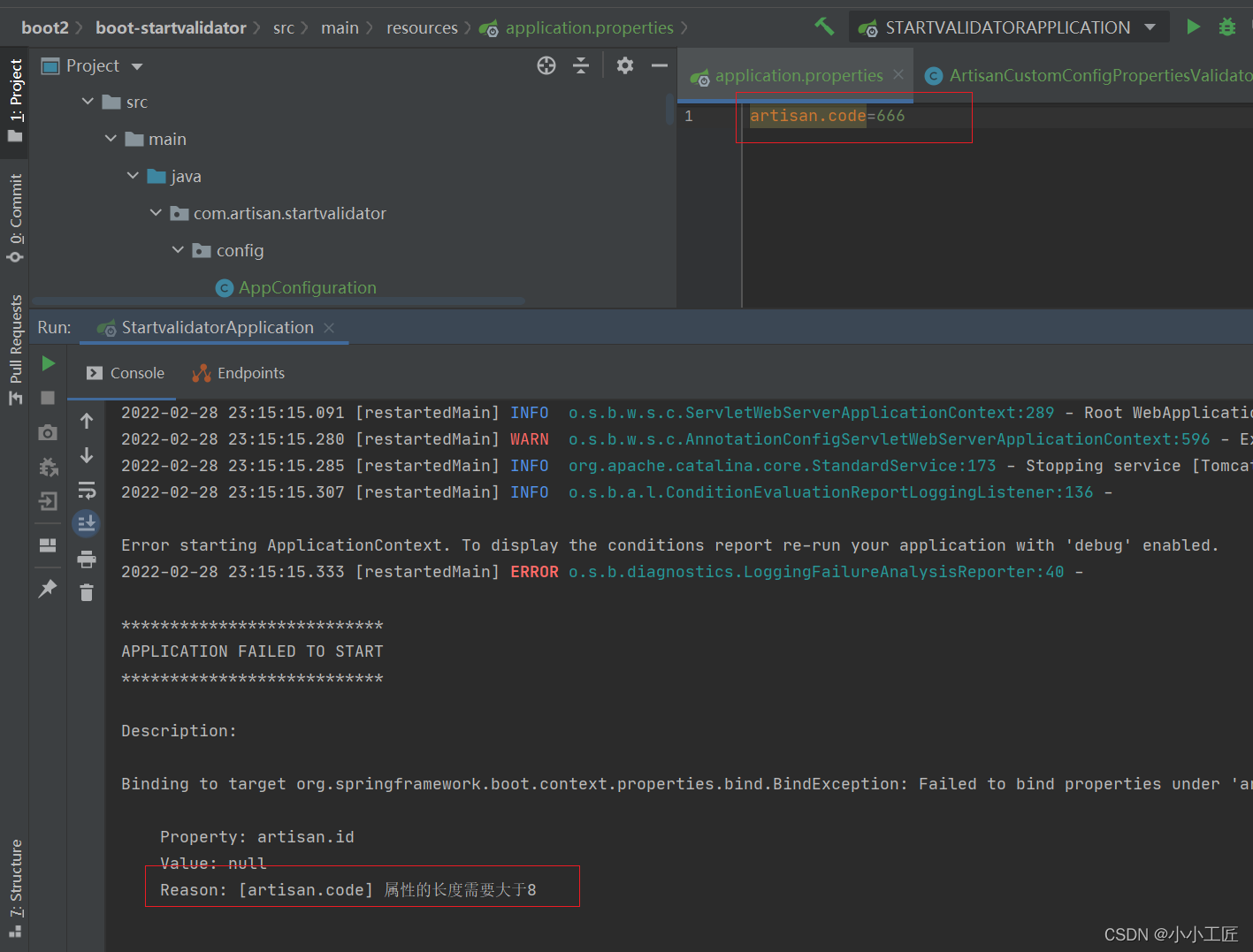
No, try it
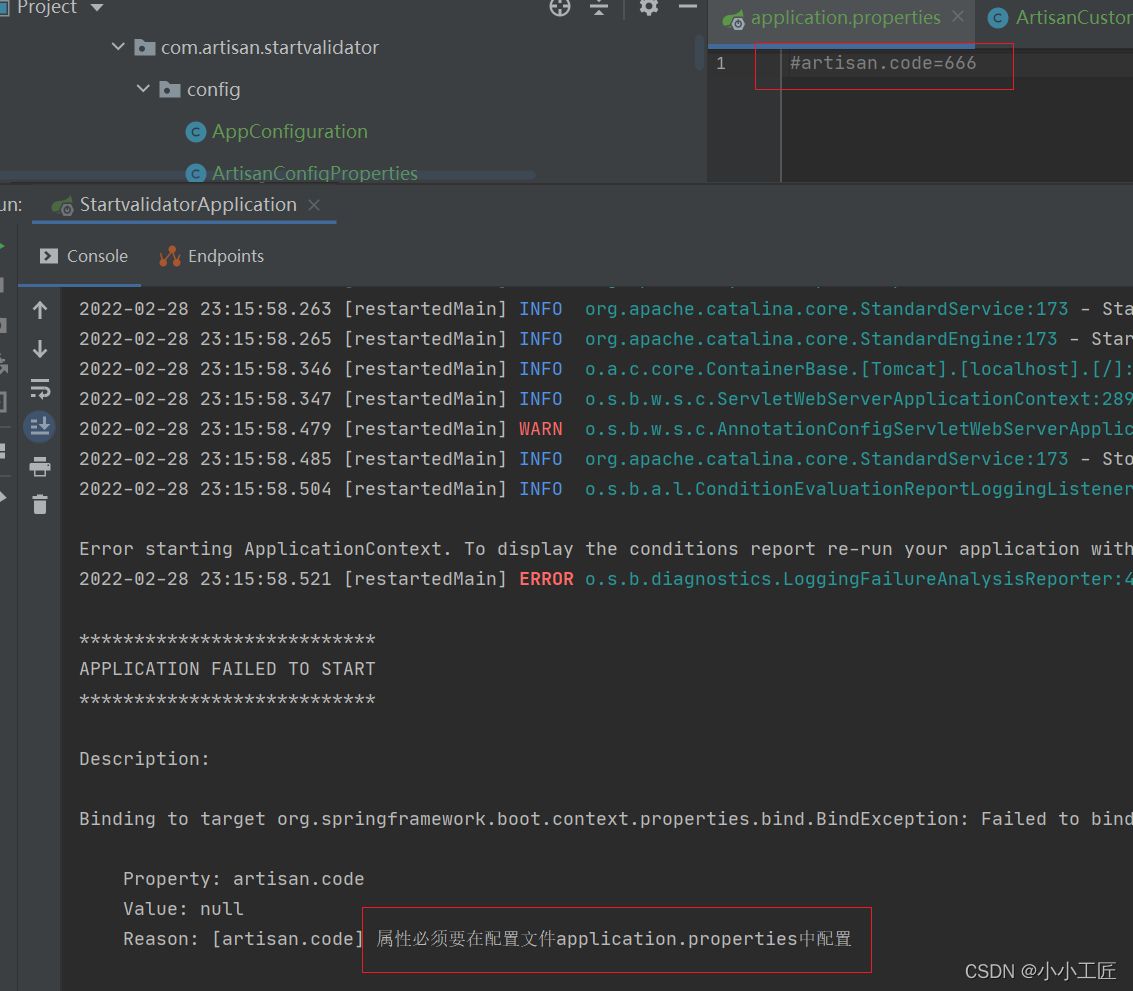
You can see that the error message is the output of user-defined verification