By default, the Spring Boot project error page and information will be displayed directly on the browser. As shown below:
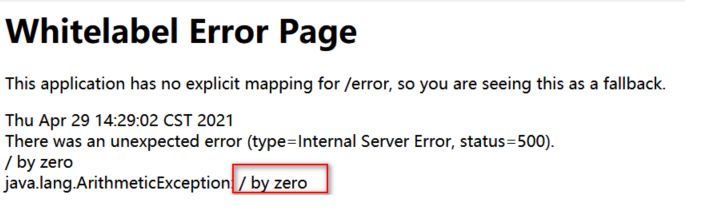
However, after the project is actually launched, the user experience is absolutely terrible. You should give users a more friendly error prompt after encountering an error.
Error page prompt
- Set specific status code page
Create a new error folder in the templates directory and a new page of status code. HTML in the error directory. For example, when 500 appears, the displayed page is 500.html. - Fuzzy matching using x
When the error of status code starting with 5 occurs, the display page can be named 5xx.html;
When a status code error starting with 50 occurs, the display page can be named 50x.html; - Unified error display page
Create error.html under templates. If there is no page with specific status code or no page successfully matched with x in the project, error.html will be displayed as the error display page.
Using spring MVC exception handling mechanism
We can also use the spring MVC exception to solve it.
Introduction to spring MVC exceptions
There are two types of exceptions in the system:
- Expected exception (check exception)
Exception information can be obtained by capturing exceptions. - Runtime exception RuntimeException
The occurrence of runtime exceptions can be reduced by standardizing code development, testing and other means;
Exceptions in the dao, service and controller of the system can be thrown upward through the throws Exception. Finally, the spring MVC front-end controller is handed over to the exception handler for exception handling, as shown in the following figure:
Specific embodiment of exception handling
1. Use @ ExceptionHandler annotation to handle exceptions
Using this annotation to handle exceptions has a disadvantage: it can only handle exceptions in the current Controller.
@Controller public class ControllerDemo1 { @RequestMapping("test1.action") public String test1(){ int i = 1/0; return "success.jsp"; } @RequestMapping("test2.action") public String test2(){ String s =null; System.out.println(s.length()); return "success.jsp"; } @ExceptionHandler(value ={ArithmeticException.class,NullPointerException.class} ) public ModelAndView handelException(){ ModelAndView mv =new ModelAndView(); mv.setViewName("error1.jsp"); return mv; } }
Where @ ExceptionHandler(value ={ArithmeticException.class,NullPointerException.class}) can handle errors and respond to the error1.jsp page.
2. Use @ ControllerAdvice+@ExceptionHandler
Using this method, the priority is lower than local exception handling
@ControllerAdvice public class GloableExceptionHandler1 { @ExceptionHandler(value ={ArithmeticException.class,NullPointerException.class} ) public ModelAndView handelException(){ ModelAndView mv =new ModelAndView(); mv.setViewName("error1"); return mv; } }
3. Use SimpleMappingExceptionResolver
This method can do global exceptions.
/** * Global exception */ @Configurationpublic class GloableException2 { @Bean public SimpleMappingExceptionResolver getSimpleMappingExceptionResolver(){ SimpleMappingExceptionResolver resolver = new SimpleMappingExceptionResolver(); Properties prop = new Properties(); prop.put("java.lang.NullPointerException","error1"); prop.put("java.lang.ArithmeticException","error2"); resolver.setExceptionMappings(prop); return resolver; } }
4. Customized HandlerExceptionResolver
/** * Global exception * HandlerExceptionResolve */ @Configuration public class GloableException3 implements HandlerExceptionResolver { @Override public ModelAndView resolveException(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Object o, Exception e) { ModelAndView mv = new ModelAndView(); if(e instanceof NullPointerException){ mv.setViewName("error1"); } if(e instanceof ArithmeticException){ mv.setViewName("error2"); } mv.addObject("msg",e); return mv; } }
Continuous update, if wrong, welcome to discuss and point out, learn together