EhCache is a relatively mature Java cache framework, which was first developed from hibernate. It is an in-process cache system. It provides a variety of flexible cache management schemes such as memory, disk file storage and distributed storage, which is fast and simple.
Springboot supports the use of ehcache very much, so you only need to make some configuration to use it in springboot, and the use method is also simple.
Configure the following steps on your project
First, the old rule, POM XML plus dependency;
<!-- Spring Boot Cache support initiator --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-cache</artifactId> </dependency> <!-- Ehcache coordinate --> <dependency> <groupId>net.sf.ehcache</groupId> <artifactId>ehcache</artifactId> </dependency>
Step 2: create ehcache XML configuration file
Location: under the classpath directory, i.e. Src / main / resources / ehcache xml
When developing the file content, you can refer to the jar package imported in the first step. For details, see the following:
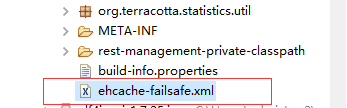
Look at the code again:
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../config/ehcache.xsd"> <diskStore path="java.io.tmpdir"/> <!--defaultCache:echcache Default cache policy for --> <defaultCache maxElementsInMemory="10000" eternal="false" timeToIdleSeconds="120" timeToLiveSeconds="120" maxElementsOnDisk="10000000" diskExpiryThreadIntervalSeconds="120" memoryStoreEvictionPolicy="LRU"> <persistence strategy="localTempSwap"/> </defaultCache> <cache name="users" maxElementsInMemory="10000" eternal="false" timeToIdleSeconds="120" timeToLiveSeconds="120" maxElementsOnDisk="10000000" diskExpiryThreadIntervalSeconds="120" memoryStoreEvictionPolicy="LRU"> <persistence strategy="localTempSwap"/> </cache> </ehcache>
Description:
<diskStore path="java.io.tmpdir"/>This is the disk storage path. When the memory cache is full, it will be put here, java.io.tmdir It is the temporary directory of the operating system cache. The cache directory of different operating systems is different.
maxElementsInMemory The maximum number of elements that can be stored in the memory cache,If put Cache The element in exceeds this value,There are two situations 1)if overflowToDisk=true,Will Cache Put the extra elements in the disk file 2)if overflowToDisk=false,Then according to memoryStoreEvictionPolicy Policy replacement Cache Original element in
overflowToDisk When memory is low,Enable disk caching
eternal Is the object in the cache permanently valid
timeToIdleSeconds Allowed idle time of cached data before invalidation(Company:second),Only if eternal=false When using,The default value is 0, which means the idle time is infinite,If you don't access this after this time Cache An element in,Then this element will be removed from Cache Clear in
timeToLiveSeconds Total lifetime of cached data (in seconds), only if eternal=false When used, it is timed from creation to expiration.
maxElementsOnDisk The maximum number of elements that can be stored in the disk cache,0 Represents infinity
diskExpiryThreadIntervalSeconds Disk cache cleanup thread run interval,The default is 120 seconds
memoryStoreEvictionPolicy Memory storage and release strategy,Namely achieve maxElementsInMemory When restricted,Ehcache The memory will be cleaned according to the specified policy. There are three policies,Respectively LRU(Least recently used),LFU(Most commonly used),FIFO(fifo) In addition, defaultCache Is the default caching method, cache It is a custom caching method, which can be set by yourself name Step three, in Springboot In the configuration file ehcache.xml Configuration; Namely in application.properties Add the following configuration code to the
spring.cache.ehcache.config=ehcache.xml
At the end of the third step, the ehcache is configured in Springboot. Here is how to use it in Springboot
Step 4: add the @ EnableCaching annotation before starting the class; In this case, the cache initiator will be started when the startup class is started.
@SpringBootApplication @MapperScan("com.yunxing.mapper") @EnableCaching public class app { public static void main(String[] args) { SpringApplication.run(app.class, args); } }
Step 5: the entity class implements the Serializable interface; Since entity classes are required to support disk storage in the cache, entity classes are required to implement Serializable interfaces
public class user implements Serializable{ private Integer id; private String name; private Integer age; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } }
Step 6: use @ Cacheable to store the data in the cache. The following is to store the method return value in the cache
@Service @Transactional //Transaction, which means that all under this class are controlled by transaction public class userServiceImpl implements userService { @Autowired private userMapper usermapper; @Override @Cacheable(value="users") public user selectUserById(int id) { user user=this.usermapper.selectUserById(id); System.out.println("1111111111111111111111111"); return user; } }
Note: @ Cacheable can be marked on a method or a class. When marked on a method, it means that the method supports caching. When marked on a class, it means that all methods of the class support caching. For a method that supports caching, spring will cache its return value after it is called to ensure that the next time the method is executed with the same parameters, the results can be obtained directly from the cache without executing the method again. Spring caches the return value of a method as a key value pair, and the value is the return result of the method.
@Cacheable can specify three attributes, value, key and condition.
The value attribute specifies the name of the cache (that is, which cache method in ehcache.xml is selected for storage)
The key attribute is used to specify the key corresponding to the returned result of the Spring cache method. This property supports Spring El expressions. When we do not specify this attribute, Spring will use the default policy to generate the key. We can also directly use "# parameter name" or "#p parameter index". Here are some examples of using parameters as keys
@Cacheable(value="users", key="#id") public User find(Integer id) { returnnull; } @Cacheable(value="users", key="#p0") public User find(Integer id) { returnnull; } @Cacheable(value="users", key="#user.id") public User find(User user) { returnnull; } @Cacheable(value="users", key="#p0.id") public User find(User user) { returnnull; }
Finally, use @ CacheEvict to clear the cache;
@CacheEvict(value="users",allEntries=true) public void saveUsers(Users users) { this.usersRepository.save(users); }
Note: @ CacheEvict is used to mark the methods or classes that need to clear cache elements. When marked on a class, it means that the execution of all methods in it will trigger the cache cleanup operation@ The attributes CacheEvict can specify are value, key, condition, allEntries, and beforeInvocation.
The semantics of value, key and condition are similar to the attributes corresponding to @ Cacheable; allEntries is a boolean type, indicating whether all elements in the cache need to be cleared. The default value is false, which means it is unnecessary.
When allEntries is specified as true, Spring Cache will ignore the specified key. Sometimes we need to clear all elements by Cache, which is more efficient than clearing elements one by one.