preface
In addition to the properties configuration file, spring boot can also use yaml configuration file, which is more intuitive.
brief introduction
YAML is not an acronym for recursive markup language. When developing this language, YAML actually means "Yet Another Markup Language".
It is very suitable for data centric configuration files
usage
Basic grammar
- key: value; There is a space between kv
- Case sensitive
- Use indents to indicate hierarchical relationships
- tab is not allowed for indentation, only spaces are allowed
- The number of indented spaces is not important, as long as the elements of the same level are aligned to the left
- #: indicates a comment
- There is no need to quote the string. If you want to add, '' and '' means that the string content will be escaped / not escaped
data type
Examples
Create a new SpringBoot project

In POM The Lombok dependency is introduced into the XML configuration file.
Create a new bean folder in src/main/java/boot directory, and write Pet and Person classes:
Pet class:
import lombok.Data; import lombok.ToString; @Data @ToString public class Pet { private String name; private Double weight; }
Person class:
import lombok.Data; import lombok.ToString; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component; import java.util.Date; import java.util.List; import java.util.Map; import java.util.Set; @Component @ConfigurationProperties(prefix = "person") @Data @ToString public class Person { private String userName; private Boolean boss; private Date birth; private Integer age; private String[] interests; private List<String> animal; private Map<String, Object> score; private Set<Double> salarys; private Pet pet; private Map<String, List<Pet>> allPets; }
@ConfigurationProperties(prefix = "person"): identifies the class in the configuration file
Create a new application in src/main/resources directory Yaml configuration file (take configuring Person class as an example):
person: userName: zhangsan boss: true birth: 2020/12/9 age: 18 interests: - Basketball - Football animal: [cat,dog] score: english: 80 math: 90 salarys: [9999.98, 9999.99] pet: name: Dog weight: 32.34 allPets: sick: - {name: dog, weight: 12.23} - name: cat weight: 9.32 - name: pig weight: 100.98 health: [{name: cattle, weight: 128.23},{name: sheep, weight: 124.23}]
You should pay attention to the writing of pet and allPets attributes
Test, create a new controller folder in src/main/java/boot directory, and write HelloController class:
import boot.bean.Person; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class HelloController { @Autowired Person person; @RequestMapping("/person") public Person person() { String userName = person.getUserName(); System.out.println(userName); return person; } }
Start the service, browser access: http://localhost:8080/person
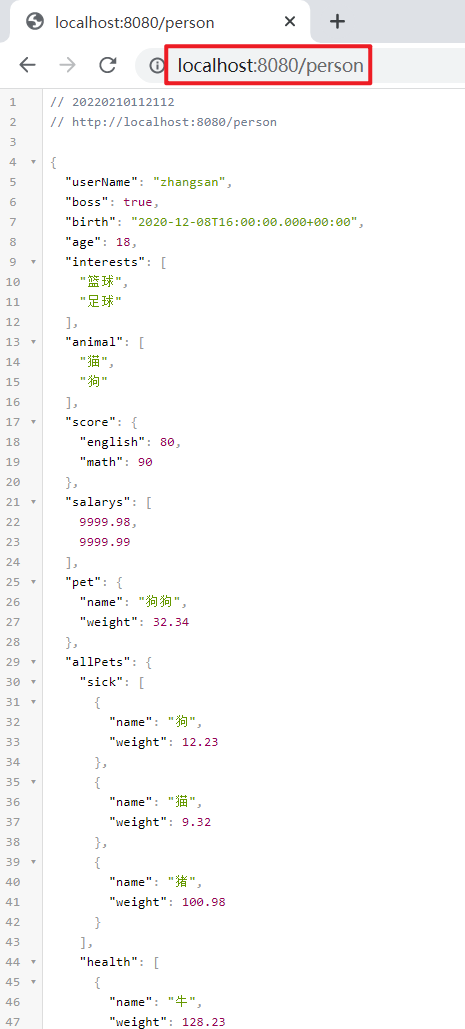
be careful
Quotation mark wrapping problem of string
Questions about strings wrapped by '' and '':
Single quotation mark, escape
person: userName: 'zhangsan \n lisi'
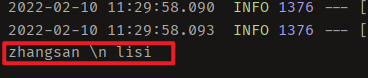
Double quotation marks, no escape
person: userName: "zhangsan \n lisi"
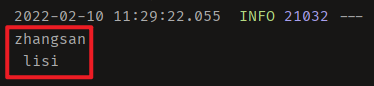
How to understand escape and non escape: Originally \ n means line feed. When wrapped in single quotation marks, it will be escaped, that is, the newline will be invalid; Double quotation mark wrapping does not escape, that is, it retains the original line feed effect.
properties and priority of yaml and yml
When the configuration files of properties, yaml and yml are written at the same time, and the contents are in conflict, the loading order is:
yml > yaml > properties
Since the later loaded content will overwrite the first loaded content, in terms of display effect, the order is as follows:
properties > yaml > yml
Example, in application Properties and application Configure userName in yaml configuration file at the same time:
application.properties:
person.userName=lisi
application.yaml:
person: userName: zhangsan
After operation, the effect is as follows:

supplement
Syntax tips for yaml configuration files
There is no automatic syntax prompt when writing yaml configuration file:

Need to be in POM The following dependencies are introduced into the XML configuration file:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <configuration> <excludes> <exclude> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> </exclude> </excludes> </configuration> </plugin> </plugins> </build>
explain:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency>
Indicates a syntax hint
<configuration> <excludes> <exclude> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> </exclude> </excludes> </configuration>
Represents a dependency that does not add syntax hints when packaging
effect:
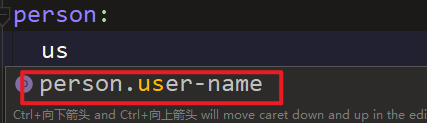
explain: person. User name: - N means capital n
Postscript
Using yml/yaml configuration file is more convenient for visual display and understanding.