The user makes a request, and the Dispatcher Servlet receives and intercepts the request.
Let's assume the requested URL is: http://localhost:8080/sm/hello
As above, the URL is split into three parts:
-
http://localhost:8080 Represents a server domain name
-
sm represents a web site deployed on a server
-
hello representation controller
Through analysis, as indicated by the URL above:
-
The hello controller for the sm site located on server localhost:8080 is requested, and the user makes the request.
-
HandlerMapping is a processor mapping.
Dispatcher Servlet calls Handler Mapping, which looks for Handler based on the request url.
-
Handler Execution represents a specific handler.
The main purpose is to find the controller based on the url, such as hello.
-
HandlerExecution passes parsed information to Dispatcher Servlet, such as resolving controller mappings.
-
A Handler Adapter represents a processor adapter that executes a Handler according to specific rules.
-
Handler lets the specific Controller execute.
-
Controller returns specific execution information to the Handler Adapter, such as ModelAndView.
-
HandlerAdapter passes the view logical name or model to Dispatcher Servlet.
-
Dispatcher Servlet calls ViewResolver to resolve the logical view name passed by Handler Adapter.
-
The view parser passes the parsed logical view name to Dispatcher Servlet.
-
Dispatcher Servlet calls a specific view based on the result of the view parsed by the view parser.
-
The final view is presented to the user.
==========================================================================
The xml configuration version is just a try. It will not be used in development, but will be more annotated.
Create a new Moudle, springmvc-hello, to add web support.
2. pom. Dependency on Importing SpringMVC in XML
<dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.2.6.RELEASE</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> </dependencies>
3. Configure the web.xml, register Dispatcher Servlet
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--1.register DispatcherServlet--> <servlet> <servlet-name>springmvc</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!--Associate one springmvc Profile:[servlet-name]-servlet.xml--> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:springmvc-servlet.xml</param-value> </init-param> <!--Startup Level-1--> <load-on-startup>1</load-on-startup> </servlet> <!--/ Match all requests; (excluding.jsp)--> <!--/* Match all requests; (Includes.jsp)--> <servlet-mapping> <servlet-name>springmvc</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
4. Write the configuration file for SpringMVC: springmvc-servlet.xml
Create the configuration file springmvc-servlet under resources. XML to which you add the following configuration:
-
Processing Mapper (BeanNameUrlHandlerMapping)
-
Processing adapter (SimpleController HandlerAdapter)
-
View Resolver
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!--Add Processing Mapper--> <bean class="org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping"/> <!--Add Processing Adapter--> <bean class="org.springframework.web.servlet.mvc.SimpleControllerHandlerAdapter"/> <!--view resolver:DispatcherServlet For him ModelAndView--> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" id="InternalResourceViewResolver"> <!--prefix--> <property name="prefix" value="/WEB-INF/jsp/"/> <!--Suffix--> <property name="suffix" value=".jsp"/> </bean> <!--Handler--> <bean id="/hello" class="com.yusael.controller.HelloController"/> </beans>
5. Write Operational Business Controller
Controller either implements the Controller interface or uses the @Controller annotation;
- Implementing Controller requires returning a ModelAndView, encapsulating data and views;
package com.yusael.controller; import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.mvc.Controller; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; // Implement Controller interface public class HelloController implements Controller { public ModelAndView handleRequest(HttpServletRequest request, HttpServletResponse response) throws Exception { // ModelAndView Model and View ModelAndView mv = new ModelAndView(); // Encapsulate the object and place it in ModelAndView mv.addObject("msg", "HelloSpringMVC!"); // Encapsulate the view you want to jump to and place it in ModelAndView mv.setViewName("hello"); // Automatically stitch into/WEB-INF/jsp/hello according to the view parser. JSP return mv; } }
6. Register bean s in configuration files
Springmvc-servlet. Give your class to the SpringIOC container in XML to register the bean s:
<!--Handler--> <bean id="/hello" class="com.yusael.controller.HelloController"/>
7. Create a view layer hello.jsp
Write a shell to jump to. JSP page, showing the data stored in ModelandView, as well as our normal page;
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>yusael</title> </head> <body> ${msg} </body> </html>
8. Configure Tomcat to start the test!
URL: http:localhost:8080/hello
Add lib dependencies to IDEA project publishing!
- Restart Tomcat to solve!
======================================================================
Create a new Moudle, springmvc-hello-annotation, and add web support.
2. pom. Dependency on Importing SpringMVC in XML
There are mainly Spring Framework Core Library, Spring MVC, servlet, JSTL and so on.
<dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.2.6.RELEASE</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> </dependencies>
Resource filtering issues
Because Maven may have resource filtering problems, pom.xml adds the following configuration:
<build> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> <resource> <directory>src/main/resources</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> </resources> </build>
3. Configure the web.xml, register Dispatcher Servlet
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--1.register servlet--> <servlet> <servlet-name>SpringMVC</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!--Specify by initialization parameters SpringMVC Configuration file location, associated--> <init-param> <param-name>contextConfigLocation</param-name> <!-- Relation SpringMVC configuration file --> <param-value>classpath:springmvc-servlet.xml</param-value> </init-param> <!-- Start sequence, the smaller the number, the earlier to start --> # summary Other content can be familiar, learned, digested one by one according to the knowledge points sorted out in the roadmap. It is not recommended that you go to a book to learn. It is best to watch more videos and read them repeatedly where you don't understand them. You must review a video content the next day and summarize it into a mind map to form a tree-like knowledge network structure for easy review in the future. There's also a good copy of " Java Foundation Core Summary Notes, shared with you intentionally,[Click here for free if you need it](https://gitee.com/vip204888/java-p7) **Catalog:** 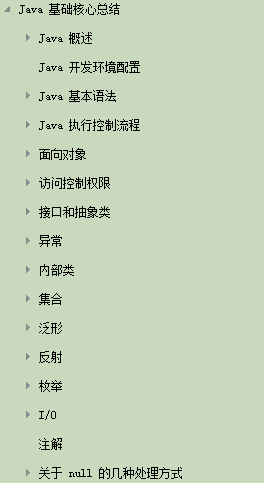 **Partial screenshots of content:** 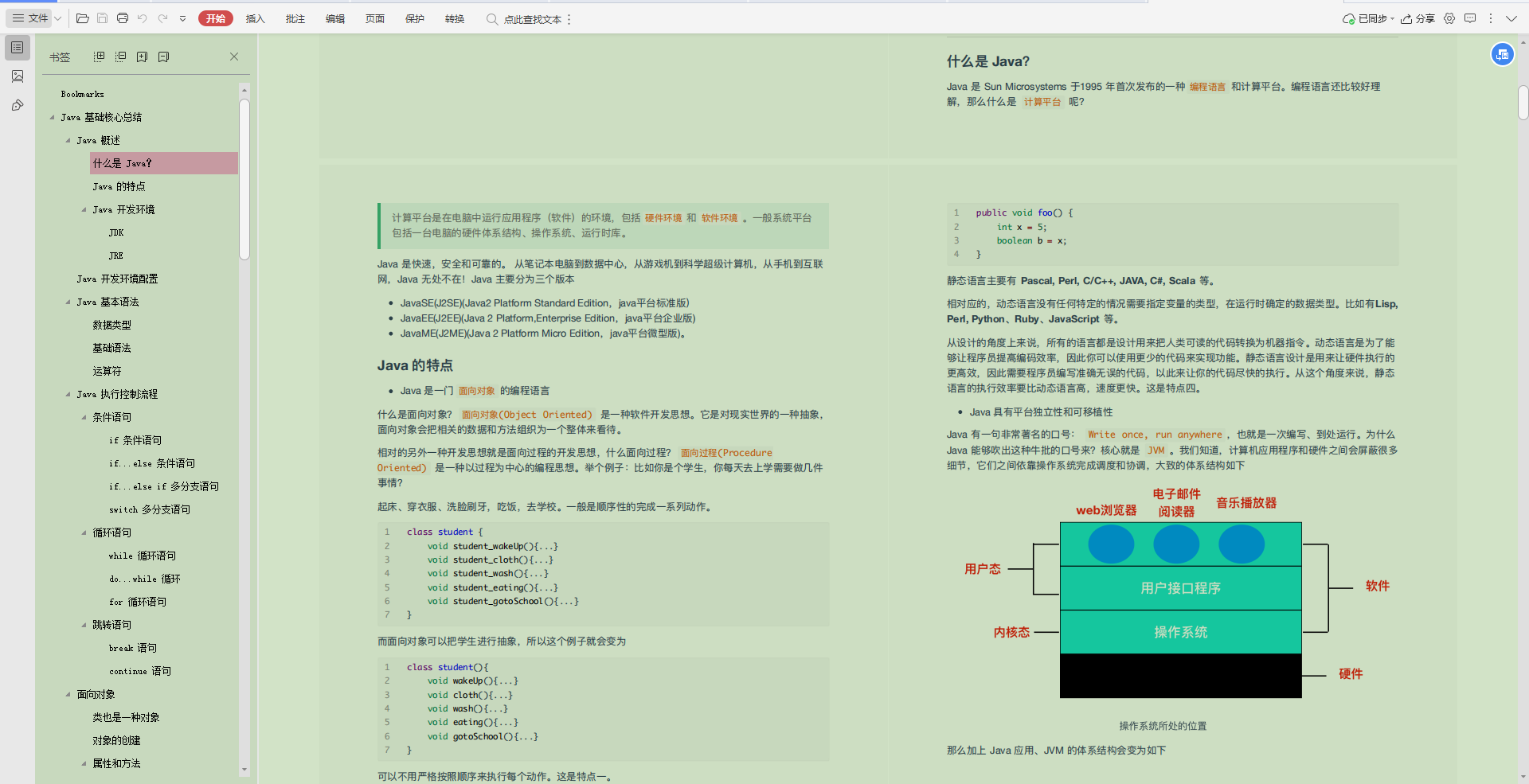 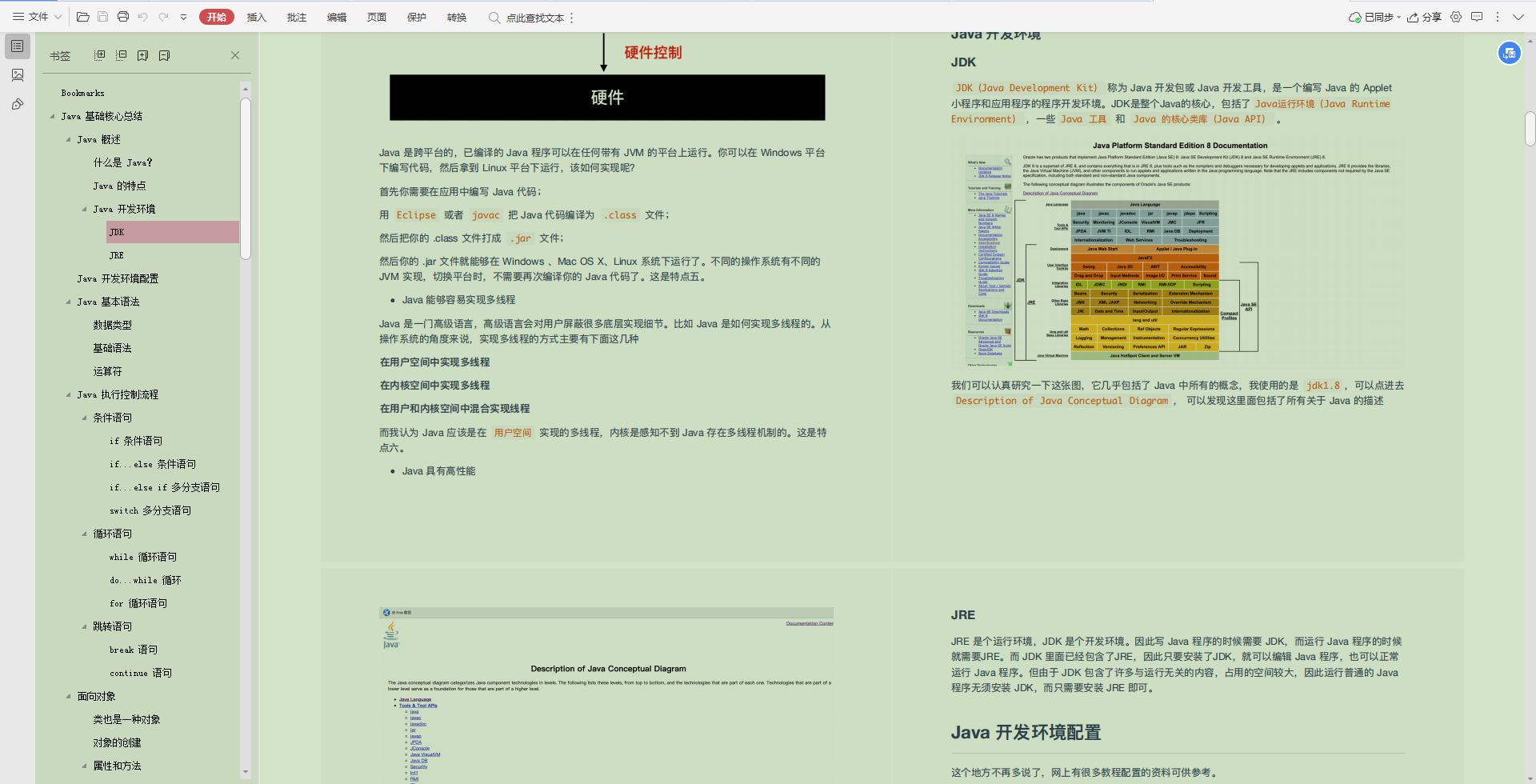 <init-param> <param-name>contextConfigLocation</param-name> <!-- Relation SpringMVC configuration file --> <param-value>classpath:springmvc-servlet.xml</param-value> </init-param> <!-- Start sequence, the smaller the number, the earlier to start --> # summary Other content can be familiar, learned, digested one by one according to the knowledge points sorted out in the roadmap. It is not recommended that you go to a book to learn. It is best to watch more videos and read them repeatedly where you don't understand them. You must review a video content the next day and summarize it into a mind map to form a tree-like knowledge network structure for easy review in the future. There's also a good copy of " Java Foundation Core Summary Notes, shared with you intentionally,[Click here for free if you need it](https://gitee.com/vip204888/java-p7) **Catalog:** [Outer Chain Picture Transfer in Progress...(img-I3J1mYXw-1628600322209)] **Partial screenshots of content:** [Outer Chain Picture Transfer in Progress...(img-KEMBRNJC-1628600322212)] [Outer Chain Picture Transfer in Progress...(img-fn38q2xq-1628600322213)]