Hello, everyone. Meet again. I'm Jun Quan.
1: Overview SSM framework is often used in project development. Compared with SSH framework, it is more widely used in only a few years of development.
- As a lightweight framework, Spring has many extended functions. The most important ones we use in general projects are IOC and AOP.
- Spring MVC is a Web layer implemented by spring, which is equivalent to the framework of Struts, but it is more flexible and powerful than Struts!
- Mybatis is a persistence layer framework, which is more flexible than Hibernate in use. It can control the preparation of sql and use XML or annotations for related configuration!
According to the above description, learning SSM framework is very important!
2: The process of building an SSM
- Managing projects with Maven Create a webapp project in Eclipse using Maven. The specific creation process is not demonstrated. If there is one, it will not be created Create project You can also use the Maven command to create, enter the specified directory in the Dos window, and execute the following command:
mvn archetype:create -DgroupId=org.ssm.dufy -DartifactId=ssm-demo -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
Note that Maven is installed in the system and the environment variables are configured! Maven installation and environment variable configuration
- Import project (named creation), add dependency The imported project is in the IDE or created directly in the IDE. Generally, the default is [src/main/java]. Manually create [src/test/resources] and [src/test/java] folders. The project structure is as follows:
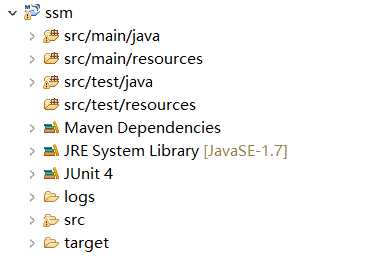
Then configure POM directly Package dependency in XML file!
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.dufy</groupId> <artifactId>ssm</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>ssmDemo</name> <url>http://maven.apache.org</url> <properties> <spring.version>4.0.5.RELEASE</spring.version> <mybatis.version>3.2.1</mybatis.version> <slf4j.version>1.6.6</slf4j.version> <log4j.version>1.2.12</log4j.version> <mysql.version>5.1.35</mysql.version> </properties> <dependencies> <!-- add to Spring rely on --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-tx</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!--spring Unit test dependency --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>${spring.version}</version> <scope>test</scope> </dependency> <!-- spring webmvc relevant jar --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!-- mysql Driver package --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.version}</version> </dependency> <!-- alibaba data source relevant jar package--> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>0.2.23</version> </dependency> <!-- alibaba fastjson Format pair --> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.1.41</version> </dependency> <!-- logback start --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>${log4j.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>${slf4j.version}</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.1.2</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-core</artifactId> <version>1.1.2</version> </dependency> <dependency> <groupId>org.logback-extensions</groupId> <artifactId>logback-ext-spring</artifactId> <version>0.1.1</version> </dependency> <!--mybatis rely on --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>${mybatis.version}</version> </dependency> <!-- mybatis/spring package --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>1.2.0</version> </dependency> <!-- add to servlet3.0 Core package --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.0.1</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>javax.servlet.jsp-api</artifactId> <version>2.3.2-b01</version> </dependency> <!-- jstl --> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <!--Unit test dependency --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> <build> <finalName>ssmDemo</finalName> </build> </project>
- Create databases and tables and generate code To create a database, I refer to other people's blog database design. I don't write this by myself. I add code directly
DROP TABLE IF EXISTS `user_t`; CREATE TABLE `user_t` ( `id` int(11) NOT NULL AUTO_INCREMENT, `user_name` varchar(40) NOT NULL, `password` varchar(255) NOT NULL, `age` int(4) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8; /*Data for the table `user_t` */ insert into `user_t`(`id`,`user_name`,`password`,`age`) values (1,'test','sfasgfaf',24)
Please check the generated code: Mybatis automatically generates code
Explanation of the generated code import picture:
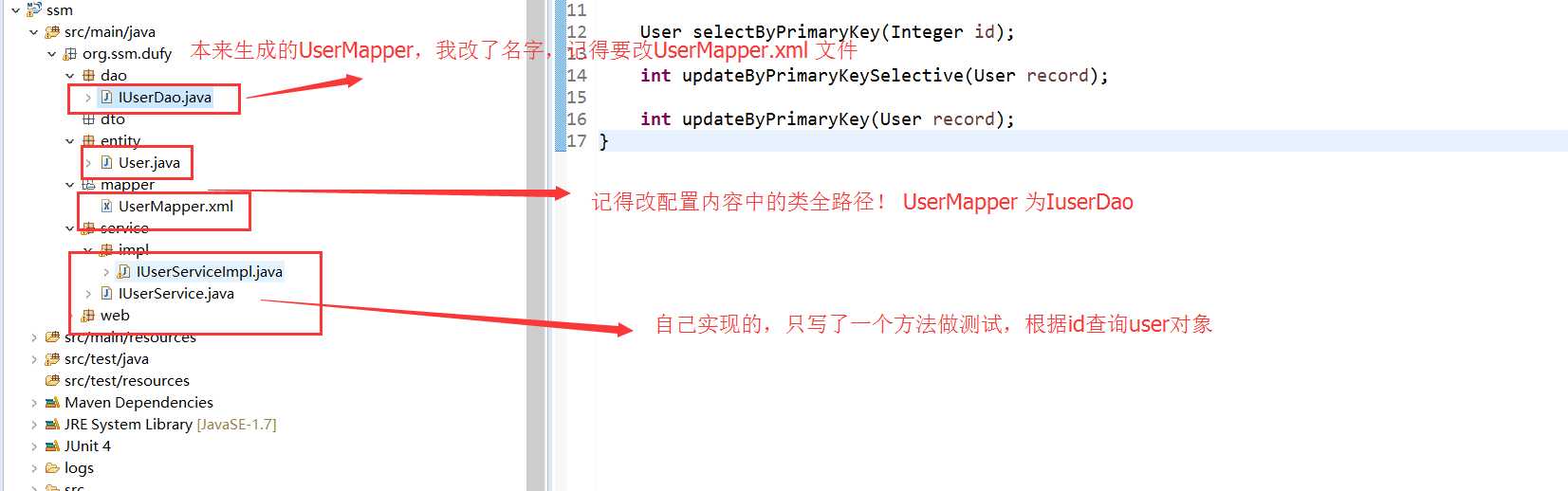
- Spring and mybatis are integrated, connected to the database and tested by Junit Copy the generated code into the project, then integrate Spring and Mybatis, and add the configuration file!
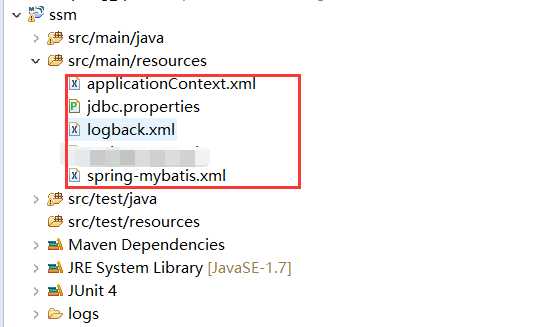
Mainly applicationContent.xml: Spring related configuration! Spring-mhbatis.xml: spring and Mybatis integrated configuration! jdbc.properties: database information configuration! logback.xml: log output information configuration! (no introduction, please check the source code for details)
Mainly introduces ApplicationContext xml,Spring-mhbatis.xml,jdbc.properties. The main contents are as follows:
jdbc.properties
jdbc_driverClassName =com.mysql.jdbc.Driver jdbc_url=jdbc:mysql://localhost:3306/ssm?useUnicode=true&characterEncoding=utf8 jdbc_username=root jdbc_password=root
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd "> <!-- 1.to configure jdbc file --> <bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="locations" value="classpath:jdbc.properties"/> </bean> <!-- 2.Scanned packet path, which is not scanned here@Controller Annotated class --><!--use<context:component-scan/> Can not be configured<context:annotation-config/> --> <context:component-scan base-package="org.ssm.dufy"> <context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <import resource="classpath:spring-mybatis.xml" /> </beans>
spring-mybatis.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd"> <!-- 3.Configure data source, using alibba Database of--> <bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource" init-method="init" destroy-method="close"> <!-- Basic properties url,user,password --> <property name="driverClassName" value="${jdbc_driverClassName}"/> <property name="url" value="${jdbc_url}"/> <property name="username" value="${jdbc_username}"/> <property name="password" value="${jdbc_password}"/> <!-- Configure initialization size, minimum and maximum --> <property name="initialSize" value="10"/> <property name="minIdle" value="10"/> <property name="maxActive" value="50"/> <!-- Configure the timeout time for getting connections --> <property name="maxWait" value="60000"/> <!-- Configure how often to detect idle connections that need to be closed. The unit is milliseconds --> <property name="timeBetweenEvictionRunsMillis" value="60000" /> <!-- Configure the minimum lifetime of a connection in the pool, in milliseconds --> <property name="minEvictableIdleTimeMillis" value="300000" /> <property name="validationQuery" value="SELECT 'x'" /> <property name="testWhileIdle" value="true" /> <property name="testOnBorrow" value="false" /> <property name="testOnReturn" value="false" /> <!-- open PSCache,And specify on each connection PSCache If you use Oracle,Then poolPreparedStatements Configure as true,mysql Can be configured as false. --> <property name="poolPreparedStatements" value="false" /> <property name="maxPoolPreparedStatementPerConnectionSize" value="20" /> <!-- Configure the of monitoring statistics interception filters --> <property name="filters" value="wall,stat" /> </bean> <!-- spring and MyBatis Perfect integration, no need mybatis Configuration mapping file for --> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource" /> <!-- Automatic scanning mapping.xml file --> <property name="mapperLocations" value="classpath:org/ssm/dufy/mapper/*.xml"></property> </bean> <!-- DAO Package name of the interface, Spring The class under it will be found automatically ,Automatically scanned all XxxxMapper.xml Corresponding mapper Interface file,as long as Mapper Interface classes and Mapper The mapping file corresponds to it--> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="org.ssm.dufy.dao" /> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"></property> </bean> <!-- (transaction management)transaction manager, use JtaTransactionManager for global tx --> <!-- Configure transaction manager --> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource" /> </bean> <!--======= Transaction configuration End =================== --> <!-- Configure annotation based declarative transactions --> <!-- enables scanning for @Transactional annotations --> <tx:annotation-driven transaction-manager="transactionManager" /> </beans>
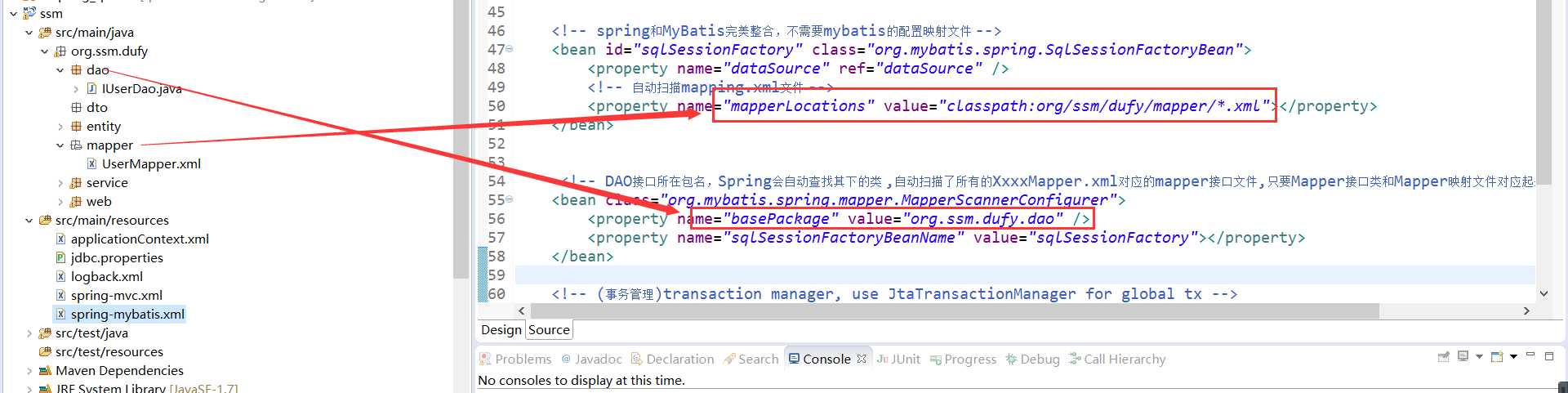
Test code in two ways:
Test 1:
package org.ssm.dufy.service; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import org.ssm.dufy.entity.User; /** * Configure the integration of spring and junit. When junit starts, load the spring IOC container spring test, junit */ @RunWith(SpringJUnit4ClassRunner.class) // Tell JUnit the spring configuration file @ContextConfiguration({ "classpath:applicationContext.xml"}) public class IUserServiceTest { @Autowired public IUserService userService; @Test public void getUserByIdTest(){ User user = userService.getUserById(1); System.out.println(user.getUserName()); } }
Test 2:
package org.ssm.dufy.service; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.ssm.dufy.entity.User; public class IUserServiceTest2 { public static void main(String[] args) { ApplicationContext application = new ClassPathXmlApplicationContext("applicationContext.xml"); IUserService uService = (IUserService) application.getBean("userService"); User user = uService.getUserById(1); System.out.println(user.getUserName()); } }
5: Integrate spring MVC, add configuration and create jsp Spring MVC needs to rely on POM XML has been added. Now you need to add web.xml in the web project Add the spring MVC startup configuration and spring integration spring MVC configuration in the XML. Add the following two files:
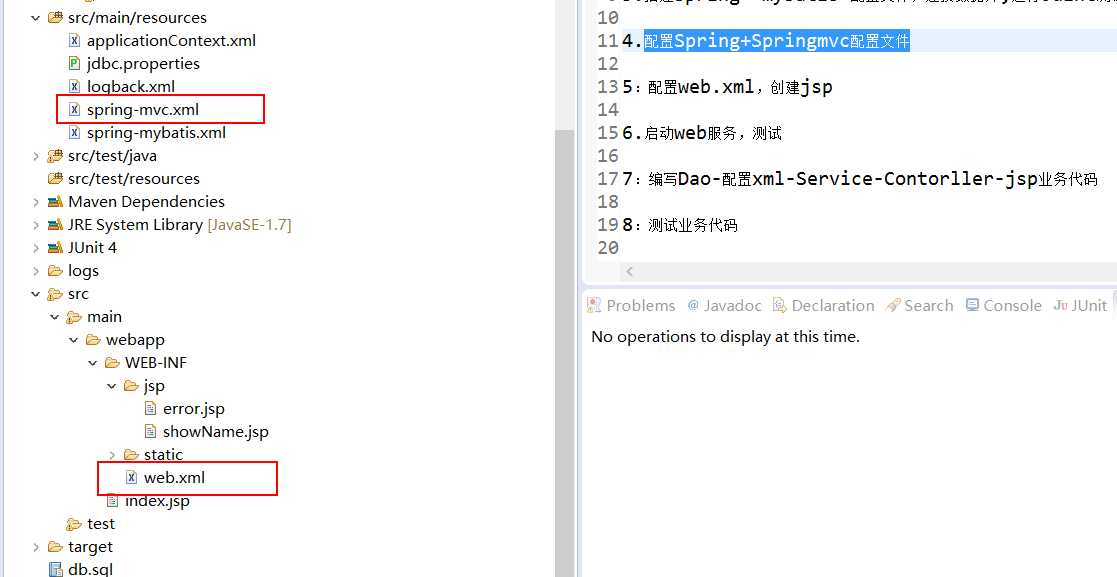
spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:p="http://www.springframework.org/schema/p" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd"> <!-- scanning controller(controller Layer injection) --> <context:component-scan base-package="org.ssm.dufy.web" use-default-filters="false"> <context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <mvc:annotation-driven /> <!-- Content Negotiation Manager --> <!--1,First check the path extension (e.g my.pdf);2,Second, check Parameter(as my?format=pdf);3,inspect Accept Header--> <bean id="contentNegotiationManager" class="org.springframework.web.accept.ContentNegotiationManagerFactoryBean"> <!-- Extension to mimeType Mapping of,Namely /user.json => application/json --> <property name="favorPathExtension" value="true"/> <!-- For opening /userinfo/123?format=json Support of --> <property name="favorParameter" value="true"/> <property name="parameterName" value="format"/> <!-- Ignore Accept Header --> <property name="ignoreAcceptHeader" value="false"/> <property name="mediaTypes"> <!--Extension to MIME Mapping of; favorPathExtension, favorParameter yes true Time works --> <value> json=application/json xml=application/xml html=text/html </value> </property> <!-- default content type --> <property name="defaultContentType" value="text/html"/> </bean> <!-- When in web.xml in DispatcherServlet use <url-pattern>/</url-pattern> When mapping, static resources can be mapped --> <mvc:default-servlet-handler /> <!-- Static resource mapping --> <mvc:resources mapping="/static/**" location="/WEB-INF/static/"/> <!-- Add pre suffix to model view --> <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:prefix="/WEB-INF/jsp/" p:suffix=".jsp"/> </beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app> <display-name>SSM-DEMO</display-name> <!-- read spring configuration file --> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </context-param> <!-- Design path variable value <context-param> <param-name>webAppRootKey</param-name> <param-value>springmvc.root</param-value> </context-param> --> <!-- Spring Character set filter --> <filter> <filter-name>SpringEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>SpringEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- Add log listener --> <context-param> <param-name>logbackConfigLocation</param-name> <param-value>classpath:logback.xml</param-value> </context-param> <listener> <listener-class>ch.qos.logback.ext.spring.web.LogbackConfigListener</listener-class> </listener> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <!-- springMVC Core configuration --> <servlet> <servlet-name>dispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <!--spingMVC Configuration path for --> <param-value>classpath:spring-mvc.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <!-- Interception settings --> <servlet-mapping> <servlet-name>dispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
New index JSP file
<%@ page contentType="text/html; charset=utf-8"%> <!doctype html> <html> <body> <h2>Hello World!</h2> </body> </html>
6. Start the web service and test Add the above items to Tomcat, start it, there is no error on the console, and access it in the address bar, http://localhost:8080/ssm . Page display Hello World! Project configuration ok!
7: Write Controller and corresponding business interface Add a new UserController and obtain the user through parameter passing uid. If the user exists, jump to showname If the user does not exist, go to. JSP JSP and return prompt information!
package org.ssm.dufy.web; import javax.servlet.http.HttpServletRequest; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.servlet.ModelAndView; import org.ssm.dufy.entity.User; import org.ssm.dufy.service.IUserService; @Controller public class UserController { @Autowired private IUserService userService; @RequestMapping(value="/showname",method=RequestMethod.GET) public String showUserName(@RequestParam("uid") int uid,HttpServletRequest request,Model model){ System.out.println("showname"); User user = userService.getUserById(uid); if(user != null){ request.setAttribute("name", user.getUserName()); model.addAttribute("mame", user.getUserName()); return "showName"; } request.setAttribute("error", "The user was not found!"); return "error"; } }
The content of Jsp is as follows:
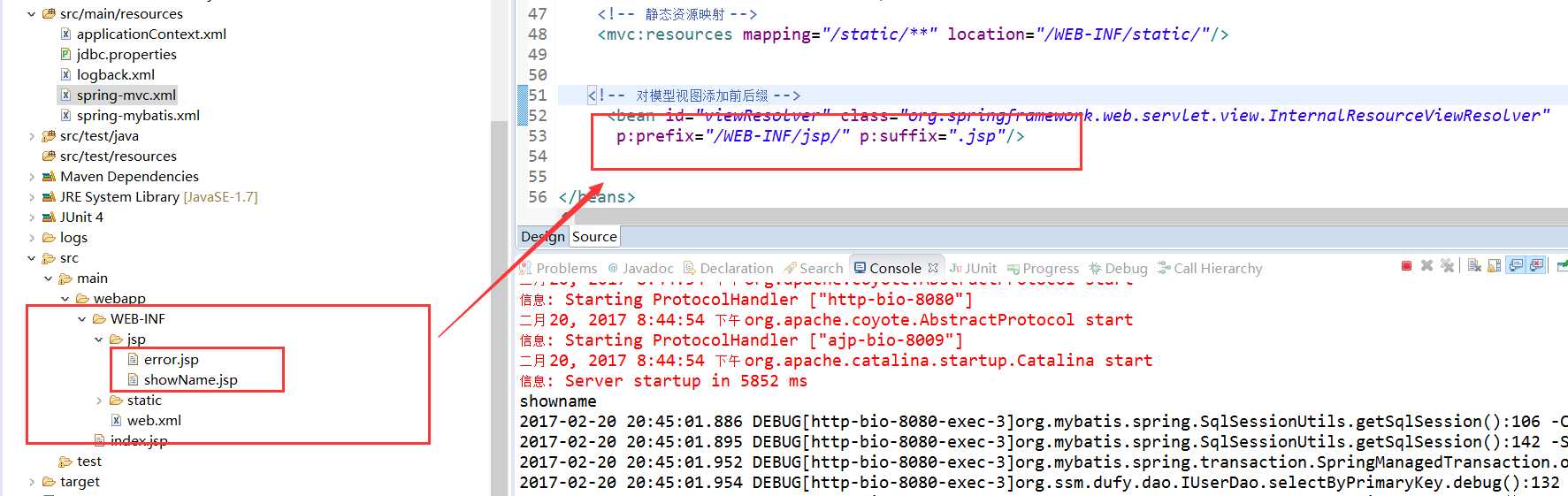
showName.jsp
<%@ page contentType="text/html; charset=utf-8"%> <!doctype html> <html> <head> <title>show name</title> </head> <body> <h1>Welcome</h1> ${name }<h1>Visit this page</h1> </body> </html>
error.jsp
<%@ page contentType="text/html; charset=utf-8"%> <!doctype html> <html> <head> <title>error page</title> </head> <body> <h2> ${error } </h2> </body> </html>
3: Problems encountered 1: The UserService cannot be found and an error is reported
It may be the problem of the package scanning path. Check whether the Service is within the scanning range
2: jsp cannot receive request setAttribute(“”,””); content
According to the information, it is because of the version problem of Jsp. You can add <% @ page iselignored = "false"% > on Jsp Or modify the web XML, add version!
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0" metadata-complete="true">
4: Summary of experience Maybe these are built on the basis of others, but reading an SSM Building Article may be fast and feel that you understand it, but I suggest that you must build it yourself, read it again and do it again. It is completely two concepts!
5: Reference articles
SSM framework - detailed integration tutorial (Spring + spring MVC + mybatis)
Mybatis3.x and spring 4 X integration
Attachment: Project source code
Publisher: full stack programmer, stack length, please indicate the source for Reprint: https://javaforall.cn/121189.html Original link: https://javaforall.cn